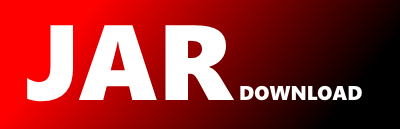
com.codepoetics.fluvius.predicates.Predicates Maven / Gradle / Ivy
package com.codepoetics.fluvius.predicates;
import com.codepoetics.fluvius.api.functional.Predicate;
import com.codepoetics.fluvius.api.functional.ScratchpadPredicate;
import com.codepoetics.fluvius.api.scratchpad.Key;
import com.codepoetics.fluvius.api.scratchpad.Scratchpad;
/**
* Utility class for constructing predicates.
*/
public final class Predicates {
private Predicates() {
}
/**
* Construct a ScratchpadPredicate that will be true if the value written for the specified key is a failure reason.
* @param key The key to test.
* @return The constructed ScratchpadPredicate.
*/
public static ScratchpadPredicate isFailure(final Key> key) {
return new ScratchpadPredicate() {
@Override
public boolean test(Scratchpad value) {
return !value.isSuccessful(key);
}
};
}
/**
* Construct a ScratchpadPredicate that will be true if the Scratchpad contains the specified key, and its value matches the supplied predicate.
*
* @param key The Key to test.
* @param predicate The predicate to apply to the key's value.
* @param The type of the Key/value.
* @return The constructed ScratchpadPredicate.
*/
public static ScratchpadPredicate keyMatches(Key key, Predicate predicate) {
return new MatchingKeyValuePredicate<>(predicate, key);
}
/**
* Construct a ScratchpadPredicate that will be true if the Scratchpad contains the specified key, and its value is equal to the expected value.
*
* @param key The Key to test.
* @param expected The expected value.
* @param The type of the Key/value.
* @return The constructed ScratchpadPredicate.
*/
public static ScratchpadPredicate keyEquals(Key key, T expected) {
return keyMatches(key, equalTo(expected));
}
/**
* Constructs a predicate that will return true if the supplied value is equal to the expected value.
*
* @param expected The expected value.
* @param The type of the expected value.
* @return The constructed predicate.
*/
public static Predicate equalTo(final T expected) {
return new Predicate() {
@Override
public boolean test(T value) {
return value.equals(expected);
}
};
}
private static final class MatchingKeyValuePredicate implements ScratchpadPredicate {
private final Predicate predicate;
private final Key key;
private MatchingKeyValuePredicate(Predicate predicate, Key key) {
this.predicate = predicate;
this.key = key;
}
@Override
public boolean test(Scratchpad scratchpad) {
return predicate.test(scratchpad.get(key));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy