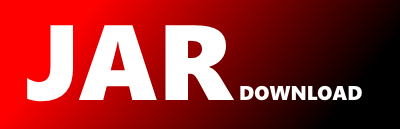
com.codepoetics.fluvius.test.matchers.AFlowEvent Maven / Gradle / Ivy
package com.codepoetics.fluvius.test.matchers;
import com.codepoetics.fluvius.api.history.*;
import org.hamcrest.Matcher;
import org.hamcrest.Matchers;
import java.util.Map;
import java.util.UUID;
public final class AFlowEvent extends BasePropertyMatcher> {
public static AFlowEvent stepStarted() {
return new AFlowEvent<>(StepStartedEvent.class);
}
public static AFlowEvent stepStarted(Map scratchpadState) {
return stepStarted(Matchers.equalTo(scratchpadState));
}
public static AFlowEvent stepStarted(Matcher super Map> scratchpadStateMatcher) {
AFlowEvent flowEventMatcher = stepStarted();
flowEventMatcher.scratchpadStateMatcher = scratchpadStateMatcher;
return flowEventMatcher;
}
public static AFlowEvent stepSucceeded() {
return new AFlowEvent<>(StepSucceededEvent.class);
}
public static AFlowEvent stepSucceeded(T result) {
return stepSucceeded(Matchers.equalTo(result));
}
public static AFlowEvent stepSucceeded(Matcher super T> resultMatcher) {
AFlowEvent flowEventMatcher = stepSucceeded();
flowEventMatcher.resultMatcher = resultMatcher;
return flowEventMatcher;
}
public static AFlowEvent stepFailed() {
return new AFlowEvent<>(StepFailedEvent.class);
}
public static AFlowEvent stepFailed(T result) {
return stepFailed(Matchers.equalTo(result));
}
public static AFlowEvent stepFailed(Matcher super T> reasonMatcher) {
AFlowEvent flowEventMatcher = stepFailed();
flowEventMatcher.reasonMatcher = reasonMatcher;
return flowEventMatcher;
}
private final Class extends FlowEvent> expectedEventType;
private Matcher super UUID> flowIdMatcher;
private Matcher super UUID> stepIdMatcher;
private Matcher super Long> timestampMatcher;
private Matcher super Map> scratchpadStateMatcher;
private Matcher super T> resultMatcher;
private Matcher super T> reasonMatcher;
private AFlowEvent(Class extends FlowEvent> expectedEventType) {
super(expectedEventType.getSimpleName());
this.expectedEventType = expectedEventType;
}
public AFlowEvent withFlowId(UUID flowId) {
return withFlowId(Matchers.equalTo(flowId));
}
public AFlowEvent withFlowId(Matcher super UUID> flowIdMatcher) {
this.flowIdMatcher = flowIdMatcher;
return this;
}
public AFlowEvent withStepId(UUID stepId) {
return withStepId(Matchers.equalTo(stepId));
}
public AFlowEvent withStepId(Matcher super UUID> stepIdMatcher) {
this.stepIdMatcher = stepIdMatcher;
return this;
}
public AFlowEvent withTimestamp(long timestamp) {
return withTimestamp(Matchers.equalTo(timestamp));
}
public AFlowEvent withTimestamp(Matcher super Long> timestampMatcher) {
this.timestampMatcher = timestampMatcher;
return this;
}
@Override
protected void describeProperties(PropertyDescriber describer) {
describer
.describeProperty("flowId", flowIdMatcher)
.describeProperty("stepId", stepIdMatcher)
.describeProperty("timestamp", timestampMatcher)
.describeProperty("scratchpadState", scratchpadStateMatcher)
.describeProperty("result", resultMatcher)
.describeProperty("reason", reasonMatcher);
}
@Override
protected void checkProperties(FlowEvent flowEvent, final PropertyMismatchDescriber describer) {
describer.check("type", flowEvent, Matchers.instanceOf(expectedEventType))
.check("flowId", flowEvent.getFlowId(), flowIdMatcher)
.check("stepId", flowEvent.getStepId(), stepIdMatcher)
.check("timestamp", flowEvent.getTimestamp(), timestampMatcher);
flowEvent.translate(new FlowEventTranslator() {
@Override
public PropertyMismatchDescriber translateStepStartedEvent(StepStartedEvent event) {
return describer.check("scratchpadState", event.getScratchpadState(), scratchpadStateMatcher);
}
@Override
public PropertyMismatchDescriber translateStepSucceededEvent(StepSucceededEvent event) {
return describer.check("result", event.getResult(), resultMatcher);
}
@Override
public PropertyMismatchDescriber translateStepFailedEvent(StepFailedEvent event) {
return describer.check("reason", event.getReason(), reasonMatcher);
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy