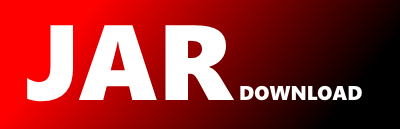
com.codepoetics.fluvius.test.matchers.AMap Maven / Gradle / Ivy
package com.codepoetics.fluvius.test.matchers;
import org.hamcrest.Description;
import org.hamcrest.Matcher;
import org.hamcrest.Matchers;
import org.hamcrest.TypeSafeDiagnosingMatcher;
import java.util.HashMap;
import java.util.Map;
public final class AMap extends TypeSafeDiagnosingMatcher
© 2015 - 2025 Weber Informatics LLC | Privacy Policy