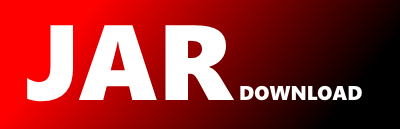
com.codepoetics.octarine.json.deserialisation.MapDeserialiser Maven / Gradle / Ivy
package com.codepoetics.octarine.json.deserialisation;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonToken;
import org.pcollections.HashTreePMap;
import org.pcollections.PMap;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import java.util.function.Function;
public final class MapDeserialiser implements SafeDeserialiser> {
public static MapDeserialiser readingValuesWith(Function valueDeserialiser) {
return new MapDeserialiser<>(valueDeserialiser);
}
public static MapDeserialiser readingStrings() {
return readingValuesWith(Deserialisers.ofString);
}
public static MapDeserialiser readingIntegers() {
return readingValuesWith(Deserialisers.ofInteger);
}
public static MapDeserialiser readingLongs() {
return readingValuesWith(Deserialisers.ofLong);
}
public static MapDeserialiser readingDoubles() {
return readingValuesWith(Deserialisers.ofDouble);
}
public static MapDeserialiser readingBooleans() {
return readingValuesWith(Deserialisers.ofBoolean);
}
private final Function valueDeserialiser;
private MapDeserialiser(Function valueDeserialiser) {
this.valueDeserialiser = valueDeserialiser;
}
@Override
public PMap applyUnsafe(JsonParser p) throws IOException {
Map values = new HashMap<>();
if (p.nextToken() == JsonToken.END_OBJECT) {
return HashTreePMap.empty();
}
while (p.nextValue() != JsonToken.END_OBJECT) {
String fieldName = p.getCurrentName();
values.put(fieldName, valueDeserialiser.apply(p));
}
return HashTreePMap.from(values);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy