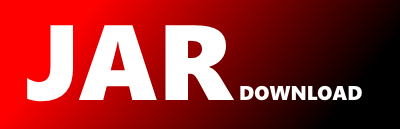
com.codepulsar.nils.api.NLS Maven / Gradle / Ivy
Show all versions of nils-core Show documentation
package com.codepulsar.nils.api;
import java.util.Locale;
import com.codepulsar.nils.core.handler.ClassPrefixResolver;
/**
* The NLS provides access to "national language support" related function for a
* specific Locale
.
*/
public interface NLS {
/**
* Get the translation for the requested key.
*
* @param key The key for the translation
* @return The translation
*/
String get(String key);
/**
* Get the translation for the requested key with arguments that should be replaced in the
* translation.
*
* @param key The key for the translation
* @param args Arguments for the translation
* @return The translation
*/
String get(String key, Object... args);
/**
* Get the translation for the requested key.
*
* The Class
is used a base key. The subKey as a further key. Both, the key and
* subKey, will be concatenated like 'MyClass.attrbute'.
*
* @param key A Class
as base key for the translation
* @param subKey The sub key, like an attribute of the class
* @return The translation
*/
String get(Class> key, String subKey);
/**
* Get the translation for the requested key with arguments that should be replaced in the
* translation.
*
*
The Class
is used a base key. The subKey as a further key. Both, the key and
* subKey, will be concatenated like 'MyClass.attrbute'.
*
* @param key A Class
as base key for the translation
* @param subKey The sub key, like an attribute of the class
* @param args Arguments for the translation
* @return The translation
*/
String get(Class> key, String subKey, Object... args);
/**
* Get the Locale
the NLS is responsible for.
*
* @return A Locale
object.
*/
Locale getLocale();
/**
* Get the {@link Formats} for the Locale
of NLS.
*
* @return A {@link Formats} object.
*/
Formats getFormats();
/**
* Get a {@link NLS} object for a specific context.
*
*
Only the keys beneath the context will be examined.
*
* @param context The context key.
* @return A {@link NLS} object.
*/
NLS context(String context);
/**
* Get a {@link NLS} object for a specific context based on a Class
.
*
*
Only the keys beneath the context will be examined.
*
*
The determination of the Class as key will be done with the configured {@link
* ClassPrefixResolver}.
*
* @param context The Class
for the context key.
* @return A {@link NLS} object.
* @see NilsConfig#getClassPrefixResolver()
*/
NLS context(Class> context);
}