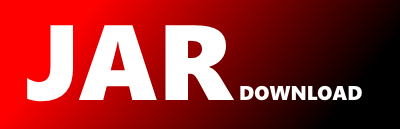
com.codeslap.apps.api.CodeSlapApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codeslap-api Show documentation
Show all versions of codeslap-api Show documentation
Java library to use the apps.codeslap.com API
package com.codeslap.apps.api;
import com.codeslap.apps.bean.*;
import org.simpleframework.xml.Default;
import org.simpleframework.xml.DefaultType;
import org.simpleframework.xml.ElementList;
import org.simpleframework.xml.Root;
import java.io.File;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Twitvid API implementation. This class uses RawTwitvidApi but returns objects for each API method, instead
* of a plain String.
*
* @author cristian
*/
public class CodeSlapApi {
private static final Map apiInstances = new HashMap();
private final RawCodeSlapApi mRawCodeSlapApi;
private final String mApiUrl;
private CodeSlapApi() {
mApiUrl = ApiConstants.BASE_URL;
mRawCodeSlapApi = new RawCodeSlapApi(mApiUrl, true);
}
private CodeSlapApi(String apiUrl) {
if (apiUrl == null) {
throw new NullPointerException("API url cannot bee null");
}
mApiUrl = apiUrl;
mRawCodeSlapApi = new RawCodeSlapApi(mApiUrl, true);
}
public static CodeSlapApi getApiInstance() {
if (!apiInstances.containsKey(ApiConstants.BASE_URL)) {
apiInstances.put(ApiConstants.BASE_URL, new CodeSlapApi());
}
return apiInstances.get(ApiConstants.BASE_URL);
}
public static CodeSlapApi getApiInstance(String apiUrl) {
if (!apiInstances.containsKey(apiUrl)) {
apiInstances.put(apiUrl, new CodeSlapApi(apiUrl));
}
return apiInstances.get(apiUrl);
}
public User login(String username, String password) throws CodeSlapException {
return XmlHelper.fromXml(User.class, mRawCodeSlapApi.login(username, password));
}
public List getApps(String userId) throws CodeSlapException {
AppsResponse appsResponse = XmlHelper.fromXml(AppsResponse.class, mRawCodeSlapApi.getApps(userId));
return appsResponse == null ? new ArrayList() : appsResponse.applications;
}
public Application getApp(String appId) throws CodeSlapException {
return XmlHelper.fromXml(Application.class, mRawCodeSlapApi.getApp(appId));
}
public List getVersions(String appId) throws CodeSlapException {
Versions appsResponse = XmlHelper.fromXml(Versions.class, mRawCodeSlapApi.getVersions(appId));
return (appsResponse == null || appsResponse.versions == null) ? new ArrayList() : appsResponse.versions;
}
/**
* Uploads a log to the codeslap-apps platform
*
* @param appId the id of the application to attach the file to
* @param fileToUpload the path of the file to attach
* @param comment the comment that the file will have
* @param type the the of the attachment (e.g. log)
* @return an attachment bean built from the server response
*/
public Attachment attachFile(String appId, String fileToUpload, String comment, String type) throws CodeSlapException {
if (appId == null) {
throw new NullPointerException("App ID cannot be null");
}
if (fileToUpload == null) {
throw new NullPointerException("File path cannot be null");
}
if (!new File(fileToUpload).exists()) {
throw new IllegalStateException("File does not exist");
}
return XmlHelper.fromXml(Attachment.class, mRawCodeSlapApi.attachFile(appId, fileToUpload, comment, type));
}
public Feedback sendFeedback(String name, String email, String subject, String body) throws CodeSlapException {
return XmlHelper.fromXml(Feedback.class, mRawCodeSlapApi.sendFeedback(name, email, subject, body));
}
/**
* @param objectId the object to get the url from
* @return returns the url that will make a gridfs file be accessible from the app
*/
public String getGridFsUrl(String objectId) {
return String.format("%sgrid/%s", mApiUrl, objectId);
}
@Root(name = "response")
@Default(DefaultType.FIELD)
private static class Versions {
@ElementList(entry = "version", required = false, inline = true, type = Version.class)
private List versions;
}
@Root(name = "apps")
@Default(value = DefaultType.FIELD)
private static class AppsResponse {
@ElementList(inline = true, entry = "app", type = Application.class)
private List applications;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy