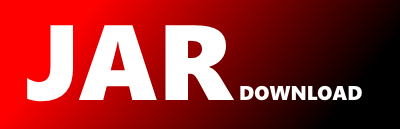
com.codeslap.apps.api.CompareUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codeslap-api Show documentation
Show all versions of codeslap-api Show documentation
Java library to use the apps.codeslap.com API
package com.codeslap.apps.api;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
/**
* Serie of methods useful to compare objects
*
* @author evelio
* @version 1.0
*/
abstract class CompareUtils {
/**
* Non instance constants class
*/
private CompareUtils() {
}
/**
* Compares with equals two objects if first is not null,
* if first is null compares it with ==
*
* @param obj1 first object to compare
* @param obj2 second object to compare
* @return true if the objects are equals false otherwise
*/
public static boolean areEquals(Object obj1, Object obj2) {
if (obj1 != null) {
return obj1.equals(obj2);
}
return obj1 == obj2;
}
/**
* Compare two maps by its contents
* It is an alternative to Map.equals function, it pretends to compare two maps.
* by comparing size, keys and values rater than if they are the same object.
* Note: In case one of the maps is not instance of map == operator will be used.
*
* @param map1 first map to compare
* @param map2 second map to compare
* @return true if both has the same size, keys and values
* true in case one of them is null and when compared with == returns true (i.e. null == null)).
* false otherwise
*/
@SuppressWarnings({"rawtypes", "unchecked"})
public static boolean areMapsContentEquals(Map map1, Map map2) {
if (map1 != null && map2 != null) {
if (!(map1.size() == map2.size() && areEquals(map1.keySet(), map2.keySet()))) {//If aren't same size and same keys
return false;
}
List values1 = new ArrayList(map1.values());
List values2 = new ArrayList(map2.values());
if (map1.size() > 1) {//If there are more than one element must be sorted
Collections.sort(values1);
Collections.sort(values2);
}
return areEquals(values1, values2);
}
return map1 == map2;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy