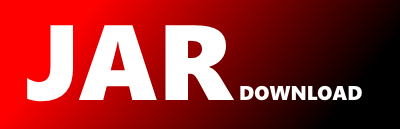
com.codeslap.apps.api.RawCodeSlapApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codeslap-api Show documentation
Show all versions of codeslap-api Show documentation
Java library to use the apps.codeslap.com API
package com.codeslap.apps.api;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.mime.MultipartEntity;
import org.apache.http.entity.mime.content.FileBody;
import org.apache.http.entity.mime.content.StringBody;
import org.apache.http.impl.client.DefaultHttpClient;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
/**
* Class to use to interact TwitVid API
*
* @author cristian
* @version 1.0
*/
class RawCodeSlapApi {
private final boolean mDebug;
private HttpClient mExecutor;
private final String mApiUrl;
RawCodeSlapApi(String apiUrl, boolean debug) {
mApiUrl = apiUrl;
mExecutor = HttpClient.getInstance();
mDebug = debug;
}
/**
* Execute the API's login method
*
* <request>
* <username>[email protected]</username>
* <password>123456</password>
* </request>
*
*
* @param username user username
* @param password user's password
* @return string xml response from server:
* @throws CodeSlapException
*/
public InputStream login(String username, String password) throws CodeSlapException {
Request request = getPostRequest(ApiConstants.METHOD_LOGIN);
request.addParameter(ApiConstants.PARAM_EMAIL, username);
request.addParameter(ApiConstants.PARAM_PASSWORD, password);
return executeRequest(request);
}
/**
* Execute the API's getApps method
*
* @return string xml response from server:
* @throws CodeSlapException
*/
public InputStream getApps(String userId) throws CodeSlapException {
Request request = getGetRequest(ApiConstants.METHOD_GET_APPS);
request.addParameter(ApiConstants.PARAM_USER_ID, userId);
return executeRequest(request);
}
/**
* Execute the API's getApps method
*
* @return string xml response from server:
* @throws CodeSlapException
*/
public InputStream getApp(String appCode) throws CodeSlapException {
Request request = getGetRequest(ApiConstants.METHOD_GET_APPS + "/" + appCode);
return executeRequest(request);
}
/**
* Execute the API's getVersions method
*
* @param appId application id
*/
public InputStream getVersions(String appId) throws CodeSlapException {
Request request = getGetRequest(ApiConstants.METHOD_GET_VERSIONS);
request.addParameter(ApiConstants.PARAM_APP_ID, appId);
return executeRequest(request);
}
/**
* @param name
* @param email
* @param subject
* @param body
* @return
*/
public InputStream sendFeedback(String name, String email, String subject, String body) throws CodeSlapException {
Request request = getPostRequest(ApiConstants.METHOD_SEND_FEEDBACK);
request.addParameter(ApiConstants.PARAM_FEEDBACK_NAME, name);
request.addParameter(ApiConstants.PARAM_FEEDBACK_EMAIL, email);
request.addParameter(ApiConstants.PARAM_FEEDBACK_SUBJECT, subject);
request.addParameter(ApiConstants.PARAM_FEEDBACK_BODY, body);
return executeRequest(request);
}
private InputStream executeRequest(Request request) {
try {
if (mDebug == true) {
System.out.println("Executing codeslap API URL: " + request.getUrl());
}
return mExecutor.execute(request);
} catch (Exception e) {
return null;
}
}
/**
* Uploads a log to the codeslap-apps platform
*
* @param appId the id of the application to attach the file to
* @param fileToUpload the path of the file to attach
* @param comment the comment that the file will have
* @param type the the of the attachment (e.g. log)
* @return an input stream of the result
*/
public InputStream attachFile(String appId, String fileToUpload, String comment, String type) throws CodeSlapException {
DefaultHttpClient httpclient = new DefaultHttpClient();
HttpPost httppost = new HttpPost(mApiUrl + ApiConstants.METHOD_ATTACHMENTS + ".xml");
FileBody bin = new FileBody(new File(fileToUpload));
try {
MultipartEntity reqEntity = new MultipartEntity();
reqEntity.addPart("attachment[file]", bin);
reqEntity.addPart("attachment[app_id]", new StringBody(appId));
reqEntity.addPart("attachment[comment]", new StringBody(comment));
reqEntity.addPart("attachment[type]", new StringBody(type));
httppost.setEntity(reqEntity);
HttpResponse response = httpclient.execute(httppost);
HttpEntity resEntity = response.getEntity();
return resEntity.getContent();
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
private Request getGetRequest(String action) {
return new Request(HttpClient.RequestMethod.GET, mApiUrl, action + ".xml");
}
private Request getPostRequest(String action) {
return new Request(HttpClient.RequestMethod.POST, mApiUrl, action + ".xml");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy