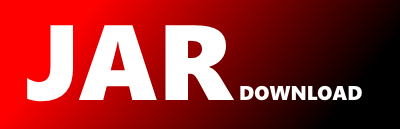
com.codeslap.apps.api.StringUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codeslap-api Show documentation
Show all versions of codeslap-api Show documentation
Java library to use the apps.codeslap.com API
package com.codeslap.apps.api;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.Map;
/**
* A series of String methods
*
* @author evelio
* @version 1.0
*/
class StringUtils {
private static final String TAG = "TwitVid.StringUtils";
/**
* Non instance constant class
*/
private StringUtils() {
}
/**
* Joins a Map to URL string like: baseUrl?key1=value1&key2=value2...
*
* @param baseUrl Optional base url to append to
* @param parameters Map with keys and values
* Note: Null values are omitted with its keys
* @param utf8Encode if true uses UTF-8 to encode values
* @return String
* - baseUrl if there is no parameters (can be null)
* - String starting with ? if there is no baseUrl i.e: ?key1=value1&key2=value2...
* - baseUrl plus parameters i.e: baseUrl?key1=value1&key2=value2...
*/
public static String urlEncode(String baseUrl, Map parameters, boolean utf8Encode) {
if (parameters != null && parameters.size() > 0) {
baseUrl = baseUrl == null ? "?" : baseUrl;//If it is null use empty to prevent NPE
StringBuilder paramBuilder = new StringBuilder();
for (Map.Entry entry : parameters.entrySet()) {
String key = entry.getKey();
String value = entry.getValue();
if (value != null && !baseUrl.contains(key + "=")) {
//Only parameters with values and non existent
if (utf8Encode) {
try {
value = URLEncoder.encode(value, "UTF-8");
} catch (UnsupportedEncodingException e) {
Logger.e(TAG, "Unable to encode param to UTF-8", e);
}//If UTF-8 is not supported does nothing
}
paramBuilder.append("&").append(key)
.append("=").append(value);
}
}
int paramStart = baseUrl.indexOf('?');
if (paramStart == -1) {//If doesn't has a ? add it
paramBuilder.setCharAt(0, '?');//Replaces the first & with ?
} else if (baseUrl.endsWith("?") || baseUrl.endsWith("&")) {
paramBuilder.deleteCharAt(0);//Removes the first &
}
baseUrl += paramBuilder.toString();
}
return baseUrl;
}
/**
* Returns the empty String "" if only if string is null
*
* @param string String to evaluate
* @return The empty string "" if only if string is null
* string itself otherwise
*/
public static String emptyIfNull(String string) {
if (string == null) {
return "";
}
return string;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy