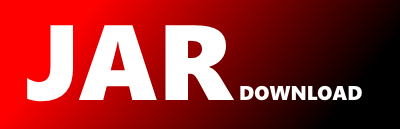
com.codeslap.apps.api.XmlHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codeslap-api Show documentation
Show all versions of codeslap-api Show documentation
Java library to use the apps.codeslap.com API
package com.codeslap.apps.api;
import org.simpleframework.xml.Serializer;
import org.simpleframework.xml.core.Persister;
import org.simpleframework.xml.transform.TransformException;
import java.io.ByteArrayOutputStream;
import java.io.InputStream;
import java.io.OutputStream;
public class XmlHelper {
private static final String XML_HEADER = "\n";
private static final Serializer SERIALIZER = new Persister();
public static String toXml(Object object) {
OutputStream out = new ByteArrayOutputStream();
try {
SERIALIZER.write(object, out);
String result = out.toString();
out.close();
return XML_HEADER + result;
} catch (Exception e) {
if (e instanceof TransformException) {
throw new RuntimeException(
"The specified object cannot be transformed to XML. "
+ "Did you forget to add SimpleXML annotations to it?");
} else {
e.printStackTrace();
}
}
return null;
}
public static T fromXml(Class extends T> clazz, String xml) {
try {
return SERIALIZER.read(clazz, xml, false);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
public static T fromXml(Class extends T> clazz, InputStream xml) {
try {
return SERIALIZER.read(clazz, xml, false);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy