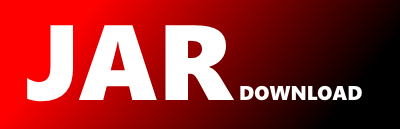
com.codeslap.apps.bean.Application Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codeslap-api Show documentation
Show all versions of codeslap-api Show documentation
Java library to use the apps.codeslap.com API
package com.codeslap.apps.bean;
import org.simpleframework.xml.Default;
import org.simpleframework.xml.DefaultType;
import org.simpleframework.xml.Element;
import org.simpleframework.xml.Root;
@Root(name = "app")
@Default(DefaultType.FIELD)
public class Application {
private String id;
private String name;
@Element(name = "app-code")
private String appCode;
@Element(name = "icon-id")
private String iconId;
@Element(name = "icon-name")
private String iconName;
@Element(name = "icon-size")
private String iconSize;
@Element(name = "icon-type")
private String iconType;
private String description;
@Element(name = "package-name", required = false)
private String packageName;
@Element(name = "created-at")
private String created;
@Element(name = "updated-at")
private String modified;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAppCode() {
return appCode;
}
public void setAppCode(String appCode) {
this.appCode = appCode;
}
public String getIconId() {
return iconId;
}
public void setIconId(String iconId) {
this.iconId = iconId;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getPackageName() {
return packageName;
}
public void setPackageName(String packageName) {
this.packageName = packageName;
}
public String getCreated() {
return created;
}
public void setCreated(String created) {
this.created = created;
}
public String getModified() {
return modified;
}
public void setModified(String modified) {
this.modified = modified;
}
public String getIconName() {
return iconName;
}
public void setIconName(String iconName) {
this.iconName = iconName;
}
public String getIconSize() {
return iconSize;
}
public void setIconSize(String iconSize) {
this.iconSize = iconSize;
}
public String getIconType() {
return iconType;
}
public void setIconType(String iconType) {
this.iconType = iconType;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof Application)) return false;
Application that = (Application) o;
if (appCode != null ? !appCode.equals(that.appCode) : that.appCode != null) return false;
if (created != null ? !created.equals(that.created) : that.created != null) return false;
if (description != null ? !description.equals(that.description) : that.description != null) return false;
if (iconId != null ? !iconId.equals(that.iconId) : that.iconId != null) return false;
if (iconName != null ? !iconName.equals(that.iconName) : that.iconName != null) return false;
if (iconSize != null ? !iconSize.equals(that.iconSize) : that.iconSize != null) return false;
if (iconType != null ? !iconType.equals(that.iconType) : that.iconType != null) return false;
if (id != null ? !id.equals(that.id) : that.id != null) return false;
if (modified != null ? !modified.equals(that.modified) : that.modified != null) return false;
if (name != null ? !name.equals(that.name) : that.name != null) return false;
if (packageName != null ? !packageName.equals(that.packageName) : that.packageName != null) return false;
return true;
}
@Override
public int hashCode() {
int result = id != null ? id.hashCode() : 0;
result = 31 * result + (name != null ? name.hashCode() : 0);
result = 31 * result + (appCode != null ? appCode.hashCode() : 0);
result = 31 * result + (iconId != null ? iconId.hashCode() : 0);
result = 31 * result + (iconName != null ? iconName.hashCode() : 0);
result = 31 * result + (iconSize != null ? iconSize.hashCode() : 0);
result = 31 * result + (iconType != null ? iconType.hashCode() : 0);
result = 31 * result + (description != null ? description.hashCode() : 0);
result = 31 * result + (packageName != null ? packageName.hashCode() : 0);
result = 31 * result + (created != null ? created.hashCode() : 0);
result = 31 * result + (modified != null ? modified.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "Application{" +
"id='" + id + '\'' +
", name='" + name + '\'' +
", appCode='" + appCode + '\'' +
", iconId='" + iconId + '\'' +
", iconName='" + iconName + '\'' +
", iconSize='" + iconSize + '\'' +
", iconType='" + iconType + '\'' +
", description='" + description + '\'' +
", packageName='" + packageName + '\'' +
", created='" + created + '\'' +
", modified='" + modified + '\'' +
'}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy