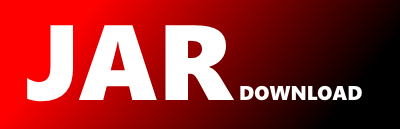
com.codeslap.apps.bean.Version Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codeslap-api Show documentation
Show all versions of codeslap-api Show documentation
Java library to use the apps.codeslap.com API
package com.codeslap.apps.bean;
import org.simpleframework.xml.Default;
import org.simpleframework.xml.DefaultType;
import org.simpleframework.xml.Element;
import org.simpleframework.xml.Root;
/**
* Example of XML version:
*
* {@code
*
*
*
*
*
* 4f0150968cfe354758000021
* Implemented top 100
* aabbccdd
* 2012-01-02T20:48:26Z
* 4f02181a8cfe35181000000e
* 2012-01-02T20:48:26Z
* 1
* alpha
* nightly-build
*
*
* }
*
*
* @author cristian
*/
@Root
@Default(DefaultType.FIELD)
public class Version {
private String id;
@Element(name = "app-id")
private String applicationId;
@Element(name = "change-set")
private String changeSet;
@Element(name = "version-code")
private String versionCode;
@Element(name = "version-name")
private String versionName;
@Element(name = "version-type")
private String versionType;
@Element(name = "commit-hash")
private String commitHash;
@Element(name = "apk-id")
private String apkId;
@Element(name = "apk-name")
private String apkName;
@Element(name = "apk-type")
private String apkType;
@Element(name = "apk-size")
private long apkSize;
@Element(name = "created-at")
private String created;
@Element(name = "updated-at")
private String modified;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getApplicationId() {
return applicationId;
}
public void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
public String getChangeSet() {
return changeSet;
}
public void setChangeSet(String changeSet) {
this.changeSet = changeSet;
}
public String getVersionCode() {
return versionCode;
}
public void setVersionCode(String versionCode) {
this.versionCode = versionCode;
}
public String getVersionName() {
return versionName;
}
public void setVersionName(String versionName) {
this.versionName = versionName;
}
public String getVersionType() {
return versionType;
}
public void setVersionType(String versionType) {
this.versionType = versionType;
}
public String getCommitHash() {
return commitHash;
}
public void setCommitHash(String commitHash) {
this.commitHash = commitHash;
}
public String getCreated() {
return created;
}
public void setCreated(String created) {
this.created = created;
}
public String getModified() {
return modified;
}
public void setModified(String modified) {
this.modified = modified;
}
public String getApkId() {
return apkId;
}
public void setApkId(String apkId) {
this.apkId = apkId;
}
public String getApkName() {
return apkName;
}
public void setApkName(String apkName) {
this.apkName = apkName;
}
public String getApkType() {
return apkType;
}
public void setApkType(String apkType) {
this.apkType = apkType;
}
public long getApkSize() {
return apkSize;
}
public void setApkSize(long apkSize) {
this.apkSize = apkSize;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Version version = (Version) o;
if (apkSize != version.apkSize) return false;
if (apkId != null ? !apkId.equals(version.apkId) : version.apkId != null) return false;
if (apkName != null ? !apkName.equals(version.apkName) : version.apkName != null) return false;
if (apkType != null ? !apkType.equals(version.apkType) : version.apkType != null) return false;
if (applicationId != null ? !applicationId.equals(version.applicationId) : version.applicationId != null) return false;
if (changeSet != null ? !changeSet.equals(version.changeSet) : version.changeSet != null) return false;
if (commitHash != null ? !commitHash.equals(version.commitHash) : version.commitHash != null) return false;
if (created != null ? !created.equals(version.created) : version.created != null) return false;
if (id != null ? !id.equals(version.id) : version.id != null) return false;
if (modified != null ? !modified.equals(version.modified) : version.modified != null) return false;
if (versionCode != null ? !versionCode.equals(version.versionCode) : version.versionCode != null) return false;
if (versionName != null ? !versionName.equals(version.versionName) : version.versionName != null) return false;
if (versionType != null ? !versionType.equals(version.versionType) : version.versionType != null) return false;
return true;
}
@Override
public int hashCode() {
int result = id != null ? id.hashCode() : 0;
result = 31 * result + (applicationId != null ? applicationId.hashCode() : 0);
result = 31 * result + (changeSet != null ? changeSet.hashCode() : 0);
result = 31 * result + (versionCode != null ? versionCode.hashCode() : 0);
result = 31 * result + (versionName != null ? versionName.hashCode() : 0);
result = 31 * result + (versionType != null ? versionType.hashCode() : 0);
result = 31 * result + (commitHash != null ? commitHash.hashCode() : 0);
result = 31 * result + (apkId != null ? apkId.hashCode() : 0);
result = 31 * result + (apkName != null ? apkName.hashCode() : 0);
result = 31 * result + (apkType != null ? apkType.hashCode() : 0);
result = 31 * result + (int) (apkSize ^ (apkSize >>> 32));
result = 31 * result + (created != null ? created.hashCode() : 0);
result = 31 * result + (modified != null ? modified.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "Version{" +
"id='" + id + '\'' +
", appId='" + applicationId + '\'' +
", changeSet='" + changeSet + '\'' +
", versionCode='" + versionCode + '\'' +
", versionName='" + versionName + '\'' +
", versionType='" + versionType + '\'' +
", commitHash='" + commitHash + '\'' +
", apkId='" + apkId + '\'' +
", apkName='" + apkName + '\'' +
", apkType='" + apkType + '\'' +
", apkSize=" + apkSize +
", created='" + created + '\'' +
", modified='" + modified + '\'' +
'}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy