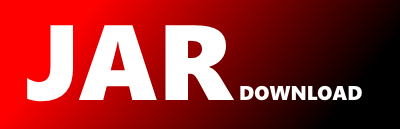
com.codeslap.topy.BaseActivity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of topy Show documentation
Show all versions of topy Show documentation
Some boilerplate code when using fragments API
/*
* Copyright 2011 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.codeslap.topy;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.view.KeyEvent;
import android.view.View;
import com.actionbarsherlock.app.SherlockFragmentActivity;
import com.actionbarsherlock.view.Window;
/**
* A base activity that defers common functionality across app activities to an
* {@link ActivityHelper}.
*/
public abstract class BaseActivity extends SherlockFragmentActivity {
public static final String URI_KEY = "_uri";
final ActivityHelper mActivityHelper = ActivityHelper.createInstance(this);
@Override
protected void onCreate(Bundle savedInstanceState) {
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
super.onCreate(savedInstanceState);
}
@Override
public void setContentView(int layoutResId) {
super.setContentView(layoutResId);
setSupportProgressBarIndeterminateVisibility(false);
}
@Override
public void setContentView(View view) {
super.setContentView(view);
setSupportProgressBarIndeterminateVisibility(false);
}
@Override
public boolean onKeyLongPress(int keyCode, KeyEvent event) {
return mActivityHelper.onKeyLongPress(keyCode, event)
|| super.onKeyLongPress(keyCode, event);
}
/**
* Returns the {@link ActivityHelper} object associated with this activity.
*/
protected ActivityHelper getActivityHelper() {
return mActivityHelper;
}
/**
* Converts an intent into a {@link Bundle} suitable for use as fragment
* arguments.
*/
public static Bundle intentToFragmentArguments(Intent intent) {
Bundle arguments = new Bundle();
if (intent == null) {
return arguments;
}
final Uri data = intent.getData();
if (data != null) {
arguments.putParcelable(URI_KEY, data);
}
final Bundle extras = intent.getExtras();
if (extras != null) {
arguments.putAll(intent.getExtras());
}
return arguments;
}
/**
* Converts a fragment arguments bundle into an intent.
*/
public static Intent fragmentArgumentsToIntent(Bundle arguments) {
Intent intent = new Intent();
if (arguments == null) {
return intent;
}
final Uri data = arguments.getParcelable(URI_KEY);
if (data != null) {
intent.setData(data);
}
intent.putExtras(arguments);
intent.removeExtra(URI_KEY);
return intent;
}
/**
* Takes a given intent and either starts a new ui to handle it (the default behavior),
* or creates/updates a fragment (in the case of a multi-pane ui) that can handle the
* intent.
*
* Must be called from the main (UI) thread.
*/
public void openActivityOrFragment(Intent intent) {
// Default implementation simply calls startActivity
startActivity(intent);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy