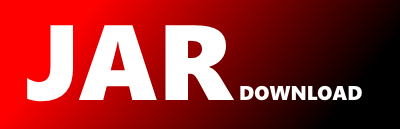
com.codetaco.date.CalendarFactoryImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of date Show documentation
Show all versions of date Show documentation
A better date function for JAVA
package com.codetaco.date;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.List;
class CalendarFactoryImpl implements ICalendarFactory {
LocalDateTime overrideForNowAndToday;
LocalDateTime applyAdjustments(final LocalDateTime startingDate,
final String... adjustmentsArray) {
try {
LocalDateTime modifiedDateTime = startingDate;
if (adjustmentsArray != null) {
final List adjustments = new ArrayList();
for (final String adjs : adjustmentsArray) {
if (adjs.trim().length() == 0) {
break;
}
final String[] singleAdjs = adjs.split(" +");
for (final String adj : singleAdjs) {
adjustments.add(new DateAdjustment(adj));
}
}
for (final DateAdjustment adj : adjustments) {
modifiedDateTime = adj.adjust(modifiedDateTime);
}
}
return modifiedDateTime;
} catch (Exception e) {
throw DateException.builder().cause(e).build();
}
}
@Override
public String asFormula(final LocalDateTime ldt) {
final char dir = AdjustmentDirection.AT.firstChar;
final StringBuilder sb = new StringBuilder();
sb.append("" + dir).append(ldt.getYear()).append("year");
sb.append(" " + dir).append(ldt.getMonthValue()).append("month");
sb.append(" " + dir).append(ldt.getDayOfMonth()).append("day");
sb.append(" " + dir).append(ldt.getHour()).append("hour");
sb.append(" " + dir).append(ldt.getMinute()).append("minute");
sb.append(" " + dir).append(ldt.getSecond()).append("second");
sb.append(" " + dir).append(ldt.getNano()).append("nanosecond");
return sb.toString();
}
@Override
public LocalDateTime modifyImpl(final LocalDateTime startingDate,
final String... adjustmentsArray) {
return applyAdjustments(startingDate, adjustmentsArray);
}
@Override
public LocalDateTime noTimeImpl(final LocalDateTime startingDate) {
return LocalDateTime.of(
startingDate.getYear(),
startingDate.getMonth(),
startingDate.getDayOfMonth(),
0, 0, 0, 0);
}
@Override
public LocalDateTime nowImpl(final String... adjustmentsArray) {
return applyAdjustments(overrideIfNecessary(LocalDateTime.now()), adjustmentsArray);
}
LocalDateTime overrideIfNecessary(final LocalDateTime startingDate) {
if (overrideForNowAndToday != null) {
return overrideForNowAndToday;
}
return startingDate;
}
@Override
public void setOverrideForSystemTime(final LocalDateTime overrideDateAndTime) {
overrideForNowAndToday = overrideDateAndTime;
}
@Override
public LocalDateTime todayImpl(final String... adjustmentsArray) {
return applyAdjustments(noTimeImpl(overrideIfNecessary(LocalDateTime.now())), adjustmentsArray);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy