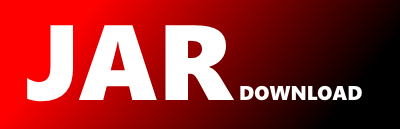
com.codingame.gameengine.core.MultiplayerGameManager Maven / Gradle / Ivy
package com.codingame.gameengine.core;
import java.io.IOException;
import java.io.StringReader;
import java.util.List;
import java.util.Properties;
import java.util.Scanner;
import java.util.Map.Entry;
import java.util.concurrent.ThreadLocalRandom;
import java.util.stream.Collectors;
import com.google.inject.Singleton;
/**
* The AbstractMultiplayerPlayer
takes care of running each turn of a multiplayer game and computing each visual frame of the replay. It
* provides many utility methods that handle instances of your implementation of AbstractMultiplayerPlayer.
*
* @param
* Your implementation of AbstractMultiplayerPlayer
*/
@Singleton
public final class MultiplayerGameManager extends GameManager {
private Properties gameParameters;
private Long seed;
@Override
protected void readGameProperties(InputCommand iCmd, Scanner s) {
// create game properties
gameParameters = new Properties();
if (iCmd.lineCount > 0) {
for (int i = 0; i < (iCmd.lineCount - 1); i++) {
try {
gameParameters.load(new StringReader(s.nextLine()));
} catch (IOException e) {
e.printStackTrace();
}
}
}
if (!gameParameters.containsKey("seed")) {
seed = ThreadLocalRandom.current().nextLong();
gameParameters.setProperty("seed", String.valueOf(seed));
} else {
try {
seed = Long.parseLong(gameParameters.getProperty("seed"));
} catch (NumberFormatException e) {
log.warn("The seed property is not a number, it is reserved by the CodinGame platform to run arena games.");
}
}
}
@Override
protected void dumpGameProperties() {
out.println(OutputCommand.UINPUT.format(gameParameters.size()));
for (Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy