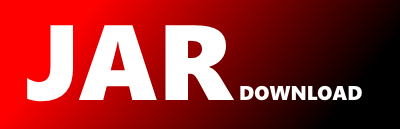
com.codingame.gameengine.core.RefereeMain Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
Core Project of the CodinGame engine toolkit. Core components to create a game and communicate with the view.
package com.codingame.gameengine.core;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.PrintStream;
import java.lang.reflect.Type;
import com.google.inject.Guice;
import com.google.inject.Injector;
import com.google.inject.Key;
import com.google.inject.util.Types;
/**
* Entry point for the local GameRunner
and CodinGame's server side game runner
*/
public class RefereeMain {
private static boolean inProduction = false;
/**
* Is overridden by CodinGame's server side game runner
* @return whether or not this execution is happening locally or on CodinGame
*/
public static boolean isInProduction() {
return inProduction;
}
/**
* CodinGame's game runner will launch the referee using this method.
*
* @param args unused
*/
public static void main(String[] args) {
inProduction = true;
InputStream in = System.in;
PrintStream out = System.out;
System.setOut(new PrintStream(new OutputStream() {
@Override
public void write(int b) throws IOException {
// Do nothing.
}
}));
System.setIn(new InputStream() {
@Override
public int read() throws IOException {
throw new RuntimeException("Impossible to read from the referee");
}
});
start(in, out);
}
/**
* The local GameRunner
will launch the referee using this method.
*
* @param is InputStream
used to capture the referee's stdin
* @param out PrintStream
used to capture the referee's stdout
*/
@SuppressWarnings("unchecked")
public static void start(InputStream is, PrintStream out) {
Injector injector = Guice.createInjector(new GameEngineModule());
Type type = Types.newParameterizedType(GameManager.class, AbstractPlayer.class);
GameManager gameManager = (GameManager) injector.getInstance(Key.get(type));
gameManager.start(is, out);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy