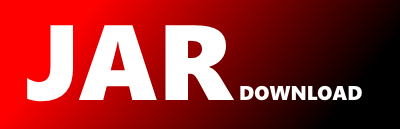
com.codingame.gameengine.module.entities.Entity Maven / Gradle / Ivy
package com.codingame.gameengine.module.entities;
import java.util.Optional;
/**
*
* A graphical entity, displayed on screen in the game's replay.
*
* The graphical counterpart's coordinates are converted from world units to pixel coordinates.
*
* @param
* a subclass inheriting Entity, used in order to return this as a T instead of an Entity.
*/
public abstract class Entity> {
final int id;
EntityState state;
private int x, y, zIndex;
private double scaleX = 1, scaleY = 1;
private double skewX = 0, skewY = 0;
private boolean visible = false;
private double rotation, alpha = 1;
ContainerBasedEntity> parent;
Mask mask;
static enum Type {
CIRCLE, LINE, RECTANGLE, SPRITE, TEXT, BITMAPTEXT, GROUP, BUFFERED_GROUP, SPRITEANIMATION, ROUNDED_RECTANGLE, POLYGON, TILING_SPRITE
}
Entity() {
id = ++GraphicEntityModule.ENTITY_COUNT;
state = new EntityState();
// World commits made before the creation of an entity should not affect that entity.
// This is why we set `visible` to false by default and immediately setVisible(true),
// this way the first commit of this entity will turn it into something visible.
// All other properties have no impact while the entity is invisible so it is effectively non-existant until it's first commit.
setVisible(true);
}
@SuppressWarnings("unchecked")
protected T self() {
return (T) this;
}
/**
* Returns a unique identifier for this Entity
.
*
* @return A unique identifier.
*/
public int getId() {
return id;
}
protected void set(String key, Object value, Curve curve) {
state.put(key, value, Optional.ofNullable(curve).orElse(Curve.DEFAULT));
}
protected void set(String key, Object value) {
set(key, value, null);
}
abstract Type getType();
/**
* Sets the X coordinate of this Entity
in world units.
*
* @param x
* the X coordinate for this Entity
.
* @return this Entity
.
*/
public T setX(int x) {
return setX(x, null);
}
/**
* Sets the X coordinate of this Entity
in world units.
*
* @param x
* the X coordinate for this Entity
.
* @param curve
* the transition to animate between values of this property.
* @return this Entity
.
*/
public T setX(int x, Curve curve) {
this.x = x;
set("x", x, curve);
return self();
}
/**
* Sets the Y coordinate of this Entity
in world units.
*
* @param y
* the Y coordinate for this Entity
.
* @return this Entity
.
*/
public T setY(int y) {
return setY(y, null);
}
/**
* Sets the Y coordinate of this Entity
in world units.
*
* @param y
* the Y coordinate for this Entity
.
* @param curve
* the transition to animate between values of this property.
* @return this Entity
.
*/
public T setY(int y, Curve curve) {
this.y = y;
set("y", y, curve);
return self();
}
/**
*
* Sets the z-index of this Entity
used to compute the display order for overlapping entities.
*
*
* An Entity
with a higher z-index is displayed over one with a smaller z-index.
*
* In case of equal values, the most recently created Entity
will be on top.
*
* @param zIndex
* the z-index for this Entity
.
* @return this Entity
.
*/
public T setZIndex(int zIndex) {
this.zIndex = zIndex;
set("zIndex", zIndex);
return self();
}
/**
* Sets the horizontal scale of this Entity
as a percentage.
*
* @param scaleX
* the horizontal scale for this Entity
.
* @return this Entity
.
*/
public T setScaleX(double scaleX) {
return setScaleX(scaleX, null);
}
/**
* Sets the horizontal scale of this Entity
as a percentage.
*
* @param scaleX
* the horizontal scale for this Entity
.
* @param curve
* the transition to animate between values of this property.
* @return this Entity
.
*/
public T setScaleX(double scaleX, Curve curve) {
this.scaleX = scaleX;
set("scaleX", scaleX, curve);
return self();
}
/**
* Sets the vertical scale of this Entity
as a percentage.
*
* @param scaleY
* the vertical scale for this Entity
.
* @return this Entity
.
*/
public T setScaleY(double scaleY) {
return setScaleY(scaleY, null);
}
/**
* Sets the vertical scale of this Entity
as a percentage.
*
* @param scaleY
* the vertical scale for this Entity
.
* @param curve
* the transition to animate between values of this property.
* @return this Entity
.
*/
public T setScaleY(double scaleY, Curve curve) {
this.scaleY = scaleY;
set("scaleY", scaleY, curve);
return self();
}
/**
* Sets the horizontal skew of this Entity
in radians.
*
* @param skewX
* the horizontal skew for this Entity
.
* @return this Entity
.
*/
public T setSkewX(double skewX) {
return setSkewX(skewX, null);
}
/**
* Sets the horizontal skew of this Entity
in radians.
*
* @param skewX
* the horizontal skew for this Entity
.
* @param curve
* the transition to animate between values of this property.
* @return this Entity
.
*/
public T setSkewX(double skewX, Curve curve) {
this.skewX = skewX;
set("skewX", skewX, curve);
return self();
}
/**
* Sets the vertical skew of this Entity
in radians.
*
* @param skewY
* the vertical skew for this Entity
.
* @return this Entity
.
*/
public T setSkewY(double skewY) {
return setSkewY(skewY, null);
}
/**
* Sets the vertical skew of this Entity
in radians.
*
* @param skewY
* the vertical skew for this Entity
.
* @param curve
* the transition to animate between values of this property.
* @return this Entity
.
*/
public T setSkewY(double skewY, Curve curve) {
this.skewY = skewY;
set("skewY", skewY, curve);
return self();
}
/**
*
* Sets the alpha of this Entity
as a percentage.
*
* 1 is opaque and 0 is invisible.
*
* @param alpha
* the alpha for this Entity
.
* @exception IllegalArgumentException
* if alpha < 0 or alpha > 1
* @return this Entity
.
*/
public T setAlpha(double alpha) {
return setAlpha(alpha, null);
}
/**
*
* Sets the alpha of this Entity
as a percentage.
*
* 1 is opaque and 0 is invisible.
*
* @param alpha
* the alpha for this Entity
.
*
* @param curve
* the transition to animate between values of this property.
* @return this Entity
.
* @exception IllegalArgumentException
* if alpha < 0 or alpha > 1
*/
public T setAlpha(double alpha, Curve curve) {
requireValidAlpha(alpha);
this.alpha = alpha;
set("alpha", alpha, curve);
return self();
}
/**
* Sets both the horizontal and vertical scale of this Entity
to the same percentage.
*
* @param scale
* the scale for this Entity
.
* @return this Entity
.
*/
public T setScale(double scale) {
return setScale(scale, null);
}
/**
* Sets both the horizontal and vertical scale of this Entity
to the same percentage.
*
* @param scale
* the scale for this Entity
.
* @param curve
* the transition to animate between values of this property.
* @return this Entity
.
*/
public T setScale(double scale, Curve curve) {
setScaleX(scale, curve);
setScaleY(scale, curve);
return self();
}
/**
* Sets the rotation of this Entity
in radians.
*
* @param rotation
* the rotation for this Entity
.
* @return this Entity
.
*/
public T setRotation(double rotation) {
return setRotation(rotation, null);
}
/**
* Sets the rotation of this Entity
in radians.
*
* @param rotation
* the rotation for this Entity
.
* @param curve
* the transition to animate between values of this property.
* @return this Entity
.
*/
public T setRotation(double rotation, Curve curve) {
this.rotation = rotation;
set("rotation", rotation, curve);
return self();
}
/**
* Flags this Entity
to be drawn on screen or not.
*
* Default is true.
*
*
* @param visible
* the value for this Entity
's visible flag.
* @return this Entity
.
*/
public T setVisible(boolean visible) {
this.visible = visible;
set("visible", visible);
return self();
}
/**
* Returns the X coordinate of this Entity
in world units.
*
* @return the X coordinate of this Entity
.
*/
public int getX() {
return x;
}
/**
* Returns the Y coordinate of this Entity
in world units.
*
* @return the Y coordinate of this Entity
.
*/
public int getY() {
return y;
}
/**
* Returns the z-index of this Entity
used to compute the display order for overlapping entities.
*
* @return the z-index of this Entity
.
*/
public int getZIndex() {
return zIndex;
}
/**
* Returns the horizontal scale of this Entity
as a percentage.
*
* Default is 1.
*
*
* @return the horizontal scale of this Entity
.
*/
public double getScaleX() {
return scaleX;
}
/**
* Returns the vertical scale of this Entity
as a percentage.
*
* Default is 1.
*
*
* @return the vertical scale of this Entity
.
*/
public double getScaleY() {
return scaleY;
}
/**
* Returns the horizontal skew of this Entity
in radians.
*
* Default is 0.
*
*
* @return the horizontal skew of this Entity
.
*/
public double getSkewX() {
return skewX;
}
/**
* Returns the vertical skew of this Entity
in radians.
*
* Default is 0.
*
*
* @return the vertical skew of this Entity
.
*/
public double getSkewY() {
return skewY;
}
/**
* Returns the alpha of this Entity
as a percentage.
*
* Default is 1.
*
*
* @return the alpha of this Entity
.
*/
public double getAlpha() {
return alpha;
}
/**
* Returns the rotation of this Entity
in radians.
*
* @return the rotation coordinate of this Entity
.
*/
public double getRotation() {
return rotation;
}
/**
* Returns whether this Entity
is flagged to be drawn on screen.
*
* @return the value of the visible flag of this Entity
.
*/
public boolean isVisible() {
return visible;
}
/**
*
* Sets a given Mask
as this Entity
's Mask
.
*
* Note: texture masks will only work on browsers which support WebGL
*
* @param mask
* the mask.
* @return this Entity
.
*/
public T setMask(Mask mask) {
return saveMask(mask);
}
/**
*
* Sets a given SpriteAnimation
as this Entity
's Mask
.
*
* Note: texture masks will only work on browsers which support WebGL
*
* @param animation
* the mask.
* @return this Entity
.
*/
public T setMask(SpriteAnimation animation) {
return saveMask(animation);
}
private T saveMask(Mask entity) {
mask = entity;
set("mask", entity == null ? -1 : entity.getId());
return self();
}
/**
*
* Returns this Entity
's Mask
.
*
* A Mask
can be:
*
* - a
Shape
* - a
Sprite
* - a
SpriteAnimation
*
*
* Default is null.
*
*
* @return this Entity
's Mask
.
*/
public Mask getMask() {
return mask;
}
protected static void requireValidAlpha(double alpha) {
if (alpha > 1) {
throw new IllegalArgumentException("An alpha may not exceed 1");
} else if (alpha < 0) {
throw new IllegalArgumentException("An alpha may not be less than 0");
}
}
protected static void requireValidColor(int color) {
if (color > 0xFFFFFF) {
throw new IllegalArgumentException(color + "is not a valid RGB integer.");
}
}
/**
* @return the Group or BufferedGroup which contains this entity
*/
public Optional> getParent() {
return Optional.ofNullable(parent);
}
}