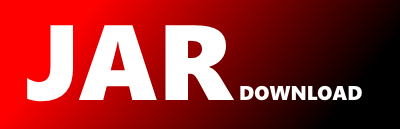
com.coditory.quark.context.HierarchyIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quark-context Show documentation
Show all versions of quark-context Show documentation
Coditory Quark Configuration Library
package com.coditory.quark.context;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.LinkedList;
import java.util.NoSuchElementException;
import java.util.Queue;
import java.util.Set;
import java.util.stream.Collectors;
import java.util.stream.StreamSupport;
final class HierarchyIterator implements Iterator> {
public static Set> getClassHierarchy(Class> forClass) {
Iterable> iterable = () -> new HierarchyIterator(forClass);
return StreamSupport
.stream(iterable.spliterator(), false)
.collect(Collectors.toSet());
}
private final Queue> remaining = new LinkedList<>();
private final Set> visited = new LinkedHashSet<>();
public HierarchyIterator(Class> initial) {
append(initial);
}
private void append(Class> toAppend) {
if (toAppend != null && !visited.contains(toAppend)) {
remaining.add(toAppend);
visited.add(toAppend);
}
}
@Override
public boolean hasNext() {
return remaining.size() > 0;
}
@Override
public Class> next() {
if (!hasNext()) {
throw new NoSuchElementException();
}
Class> polled = remaining.poll();
append(polled.getSuperclass());
for (Class> superInterface : polled.getInterfaces()) {
append(superInterface);
}
return polled;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy