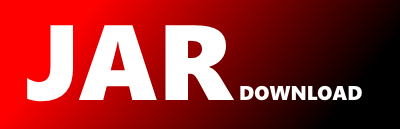
com.coditory.quark.context.ResolutionContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quark-context Show documentation
Show all versions of quark-context Show documentation
Coditory Quark Configuration Library
package com.coditory.quark.context;
import java.util.List;
import static com.coditory.quark.context.Args.checkNonNull;
import static com.coditory.quark.context.BeanDescriptor.descriptor;
public final class ResolutionContext {
private final Context context;
private final ResolutionPath path;
ResolutionContext(
Context context,
ResolutionPath path
) {
this.context = context;
this.path = path;
}
public T get(Class type) {
checkNonNull(type, "type");
return context.get(descriptor(type), path);
}
public T getOrNull(Class type) {
checkNonNull(type, "type");
return context.getOrNull(descriptor(type), path);
}
public T get(Class type, String name) {
checkNonNull(type, "type");
checkNonNull(name, "name");
return context.get(descriptor(type, name), path);
}
public T getOrNull(Class type, String name) {
checkNonNull(type, "type");
checkNonNull(name, "name");
return context.getOrNull(descriptor(type, name), path);
}
public boolean contains(Class> type) {
checkNonNull(type, "type");
return context.contains(type);
}
public boolean contains(String name) {
checkNonNull(name, "name");
return context.contains(name);
}
public boolean contains(Class> type, String name) {
checkNonNull(type, "type");
checkNonNull(name, "name");
return context.contains(type, name);
}
public List getAll(Class type) {
checkNonNull(type, "type");
return context.getAll(type, path);
}
public List getAllOrEmpty(Class type) {
checkNonNull(type, "type");
return context.getAllOrEmpty(type, path);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy