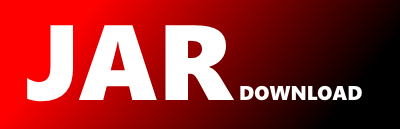
com.codota.CodotaUploaderMojo Maven / Gradle / Ivy
/*
* Copyright 2015 Codota
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.codota;
import com.codota.uploader.Uploader;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import java.io.File;
import java.io.IOException;
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
@SuppressWarnings({"JavaDoc", "unused"})
@Mojo(name = "upload")
public class CodotaUploaderMojo
extends AbstractMojo {
/**
* Location of the target files.
*/
@Parameter(defaultValue = "${project.build.directory}", required = true)
private File buildDirectory;
/**
* Source directory.
*/
@Parameter(defaultValue = "${project.build.sourceDirectory}", required = true)
private File sourceDirectory;
@Parameter(defaultValue = "${project.artifactId}", readonly = true)
private String artifactId;
@Parameter(defaultValue = "${project.groupId}", readonly = true)
private String groupId;
@Parameter(defaultValue = "${project.version}", readonly = true)
private String version;
/**
* Location of the result file.
*/
@Parameter(property = "outputDirectory", defaultValue = "${project.build.directory}", required = true)
private File outputDirectory;
/**
* Codota name passed to the uploader
*/
@Parameter(property = "token", defaultValue = "${codota.token}", required = true)
private String token;
/**
* Codota endpoint passed to the uploader
*/
@Parameter(property = "endpoint", defaultValue = "${codota.endpoint}", required = true)
private String endpoint;
@Parameter(property = "reportFileName")
private String reportFileName;
public void execute()
throws MojoExecutionException {
if (version == null) {
version = "NO_VERSION";
}
logParameters();
ensureExists(buildDirectory);
/**
* @todo: add sanitization to the endpoint url string
*/
final Uploader uploader;
uploader = new Uploader(uploadUrl(), token);
// Visit target directory and upload every jar file
FileVisitor fv = new SimpleFileVisitor() {
boolean root = true;
@Override
public FileVisitResult preVisitDirectory(Path dir, BasicFileAttributes attrs) throws IOException {
if (root) {
root = false;
return FileVisitResult.CONTINUE;
}
getLog().info("Not going into directory " + dir);
return FileVisitResult.SKIP_SUBTREE;
}
@Override
public FileVisitResult visitFile(Path path, BasicFileAttributes attrs)
throws IOException {
if (shouldSendFile(path)) {
try {
getLog().info("Uploading " + path);
uploader.uploadFile(path.toFile());
getLog().info("Done.");
} catch (Exception e) {
getLog().error("Error: " + e.getMessage());
}
}
return FileVisitResult.CONTINUE;
}
};
try {
Files.walkFileTree(buildDirectory.toPath(), fv);
} catch (IOException e) {
// print the task trace and give up on handling the exception
e.printStackTrace();
}
}
/**
* Construct artifact name from groupId and artifactId.
* Can add version if you care to make this distinction when uploading artifacts
*/
private String artifactName() {
return this.groupId + "." + this.artifactId;
}
private String uploadUrl() {
return endpoint + "/" + artifactName() + "/" + version;
}
private void ensureExists(File f) {
if (!f.exists()) {
if (f.mkdirs()) {
getLog().info("Created directory " + f);
}
}
}
private void logParameters() {
getLog().info("Codota Uploader - Started " + artifactName() + " version " + version);
getLog().info("Endpoint:" + endpoint);
getLog().info("Build directory:" + buildDirectory);
getLog().info("Source directory:" + sourceDirectory);
getLog().info("Codota token exists:" + (token != null));
}
private boolean shouldSendFile(Path path) {
return path.toString().toLowerCase().endsWith(".jar") || path.toString().toLowerCase().endsWith(".war");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy