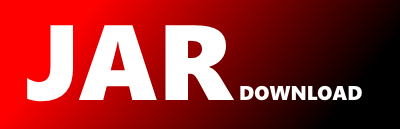
com.codurance.lightaccess.executables.Throwables Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of light-access Show documentation
Show all versions of light-access Show documentation
Non-intrusive JDBC library supporting lambdas
The newest version!
package com.codurance.lightaccess.executables;
import java.util.concurrent.Callable;
public class Throwables {
@FunctionalInterface
public interface Command {
void execute() throws Exception;
}
@FunctionalInterface
public interface Query extends Callable {
}
@FunctionalInterface
public interface ExceptionWrapper {
E wrap(Exception e);
}
public static void execute(Command command) throws RuntimeException {
execute(command, RuntimeException::new);
}
public static void execute(Command command, ExceptionWrapper wrapper) throws E {
try {
command.execute();
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
throw wrapper.wrap(e);
}
}
public static T executeQuery(Query callable) throws RuntimeException {
return executeQuery(callable, RuntimeException::new);
}
public static T executeQuery(Callable callable, ExceptionWrapper wrapper) throws E {
try {
return callable.call();
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
throw wrapper.wrap(e);
}
}
public static void executeWithResource(T closeableResource, Command command) {
try(T ignored = closeableResource) {
command.execute();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public static R executeWithResource(T closeableResource, Query callable) {
try(T ignored = closeableResource) {
return callable.call();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy