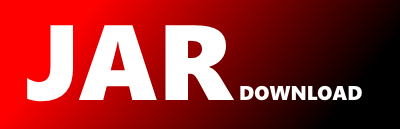
com.codurance.lightaccess.mapping.LAResultSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of light-access Show documentation
Show all versions of light-access Show documentation
Non-intrusive JDBC library supporting lambdas
The newest version!
package com.codurance.lightaccess.mapping;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.text.SimpleDateFormat;
import java.time.LocalDate;
import java.util.*;
import java.util.function.Function;
import static com.codurance.lightaccess.executables.Throwables.executeQuery;
public class LAResultSet {
private final SimpleDateFormat YYYY_MM_DD_date_format = new SimpleDateFormat("yyyy-MM-dd");
private ResultSet resultSet;
public LAResultSet(ResultSet resultSet) {
this.resultSet = resultSet;
}
public int getInt(int columnIndex) {
return executeQuery(() -> resultSet.getInt(columnIndex));
}
public String getString(int columnIndex) {
return executeQuery(() -> resultSet.getString(columnIndex));
}
public Optional getOptionalString(int columnIndex) {
return Optional.ofNullable(getString(columnIndex));
}
public LocalDate getLocalDate(int columnIndex) {
return utilDateToLocalDate(getDate(columnIndex));
}
public Date getDate(int columnIndex) {
return executeQuery(() -> sqlDateToUtilDate(columnIndex));
}
public Optional getOptionalLocalDate(int columnIndex) {
return Optional.ofNullable(getLocalDate(columnIndex));
}
public Optional onlyResult(Function mapOne) throws SQLException {
if (resultSet.next()) {
return Optional.of(mapOne.apply(this));
}
return Optional.empty();
}
public List mapResults(Function mapResults) {
List list = new ArrayList<>();
while (this.next()) {
list.add(mapResults.apply(this));
}
return list;
}
public OneToMany normaliseOneToMany(Function>> normalise) {
OneToMany oneToMany = new OneToMany<>();
while (this.next()) {
KeyValue> kv = normalise.apply(this);
oneToMany.put(kv);
}
return oneToMany;
}
public LAResultSet nextRecord() {
next();
return this;
}
private boolean next() {
return executeQuery(() -> resultSet.next());
}
private Date sqlDateToUtilDate(int columnIndex) throws SQLException {
java.sql.Date date = resultSet.getDate(columnIndex);
return (date != null) ? new Date(date.getTime()) : null;
}
private LocalDate utilDateToLocalDate(Date date) {
return (date != null) ? LocalDate.parse(YYYY_MM_DD_date_format.format(date)) : null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy