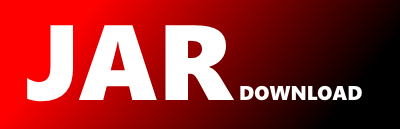
annotations.com.cognite.client.AutoValue_ApiBase_UpsertItems Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdf-sdk-java Show documentation
Show all versions of cdf-sdk-java Show documentation
Java SDK for reading and writing from/to CDF resources.
package com.cognite.client;
import com.cognite.client.config.AuthConfig;
import com.cognite.client.dto.Item;
import com.cognite.client.servicesV1.ConnectorServiceV1;
import com.google.common.collect.ImmutableMap;
import com.google.protobuf.Message;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.function.BiFunction;
import java.util.function.Function;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ApiBase_UpsertItems extends ApiBase.UpsertItems {
private final int maxBatchSize;
private final AuthConfig authConfig;
private final Function> idFunction;
private final Function> createMappingFunction;
private final Function, List>> batchingFunction;
private final Function> updateMappingFunction;
private final Function, List> retrieveFunction;
private final BiFunction equalFunction;
private final Function itemMappingFunction;
private final ConnectorServiceV1.ItemWriter createItemWriter;
private final ConnectorServiceV1.ItemWriter updateItemWriter;
private final ConnectorServiceV1.ItemWriter deleteItemWriter;
private final ImmutableMap deleteParameters;
private AutoValue_ApiBase_UpsertItems(
int maxBatchSize,
AuthConfig authConfig,
Function> idFunction,
Function> createMappingFunction,
Function, List>> batchingFunction,
@Nullable Function> updateMappingFunction,
@Nullable Function, List> retrieveFunction,
@Nullable BiFunction equalFunction,
@Nullable Function itemMappingFunction,
ConnectorServiceV1.ItemWriter createItemWriter,
@Nullable ConnectorServiceV1.ItemWriter updateItemWriter,
@Nullable ConnectorServiceV1.ItemWriter deleteItemWriter,
ImmutableMap deleteParameters) {
this.maxBatchSize = maxBatchSize;
this.authConfig = authConfig;
this.idFunction = idFunction;
this.createMappingFunction = createMappingFunction;
this.batchingFunction = batchingFunction;
this.updateMappingFunction = updateMappingFunction;
this.retrieveFunction = retrieveFunction;
this.equalFunction = equalFunction;
this.itemMappingFunction = itemMappingFunction;
this.createItemWriter = createItemWriter;
this.updateItemWriter = updateItemWriter;
this.deleteItemWriter = deleteItemWriter;
this.deleteParameters = deleteParameters;
}
@Override
int getMaxBatchSize() {
return maxBatchSize;
}
@Override
AuthConfig getAuthConfig() {
return authConfig;
}
@Override
Function> getIdFunction() {
return idFunction;
}
@Override
Function> getCreateMappingFunction() {
return createMappingFunction;
}
@Override
Function, List>> getBatchingFunction() {
return batchingFunction;
}
@Nullable
@Override
Function> getUpdateMappingFunction() {
return updateMappingFunction;
}
@Nullable
@Override
Function, List> getRetrieveFunction() {
return retrieveFunction;
}
@Nullable
@Override
BiFunction getEqualFunction() {
return equalFunction;
}
@Nullable
@Override
Function getItemMappingFunction() {
return itemMappingFunction;
}
@Override
ConnectorServiceV1.ItemWriter getCreateItemWriter() {
return createItemWriter;
}
@Nullable
@Override
ConnectorServiceV1.ItemWriter getUpdateItemWriter() {
return updateItemWriter;
}
@Nullable
@Override
ConnectorServiceV1.ItemWriter getDeleteItemWriter() {
return deleteItemWriter;
}
@Override
ImmutableMap getDeleteParameters() {
return deleteParameters;
}
@Override
public String toString() {
return "UpsertItems{"
+ "maxBatchSize=" + maxBatchSize + ", "
+ "authConfig=" + authConfig + ", "
+ "idFunction=" + idFunction + ", "
+ "createMappingFunction=" + createMappingFunction + ", "
+ "batchingFunction=" + batchingFunction + ", "
+ "updateMappingFunction=" + updateMappingFunction + ", "
+ "retrieveFunction=" + retrieveFunction + ", "
+ "equalFunction=" + equalFunction + ", "
+ "itemMappingFunction=" + itemMappingFunction + ", "
+ "createItemWriter=" + createItemWriter + ", "
+ "updateItemWriter=" + updateItemWriter + ", "
+ "deleteItemWriter=" + deleteItemWriter + ", "
+ "deleteParameters=" + deleteParameters
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ApiBase.UpsertItems) {
ApiBase.UpsertItems> that = (ApiBase.UpsertItems>) o;
return this.maxBatchSize == that.getMaxBatchSize()
&& this.authConfig.equals(that.getAuthConfig())
&& this.idFunction.equals(that.getIdFunction())
&& this.createMappingFunction.equals(that.getCreateMappingFunction())
&& this.batchingFunction.equals(that.getBatchingFunction())
&& (this.updateMappingFunction == null ? that.getUpdateMappingFunction() == null : this.updateMappingFunction.equals(that.getUpdateMappingFunction()))
&& (this.retrieveFunction == null ? that.getRetrieveFunction() == null : this.retrieveFunction.equals(that.getRetrieveFunction()))
&& (this.equalFunction == null ? that.getEqualFunction() == null : this.equalFunction.equals(that.getEqualFunction()))
&& (this.itemMappingFunction == null ? that.getItemMappingFunction() == null : this.itemMappingFunction.equals(that.getItemMappingFunction()))
&& this.createItemWriter.equals(that.getCreateItemWriter())
&& (this.updateItemWriter == null ? that.getUpdateItemWriter() == null : this.updateItemWriter.equals(that.getUpdateItemWriter()))
&& (this.deleteItemWriter == null ? that.getDeleteItemWriter() == null : this.deleteItemWriter.equals(that.getDeleteItemWriter()))
&& this.deleteParameters.equals(that.getDeleteParameters());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= maxBatchSize;
h$ *= 1000003;
h$ ^= authConfig.hashCode();
h$ *= 1000003;
h$ ^= idFunction.hashCode();
h$ *= 1000003;
h$ ^= createMappingFunction.hashCode();
h$ *= 1000003;
h$ ^= batchingFunction.hashCode();
h$ *= 1000003;
h$ ^= (updateMappingFunction == null) ? 0 : updateMappingFunction.hashCode();
h$ *= 1000003;
h$ ^= (retrieveFunction == null) ? 0 : retrieveFunction.hashCode();
h$ *= 1000003;
h$ ^= (equalFunction == null) ? 0 : equalFunction.hashCode();
h$ *= 1000003;
h$ ^= (itemMappingFunction == null) ? 0 : itemMappingFunction.hashCode();
h$ *= 1000003;
h$ ^= createItemWriter.hashCode();
h$ *= 1000003;
h$ ^= (updateItemWriter == null) ? 0 : updateItemWriter.hashCode();
h$ *= 1000003;
h$ ^= (deleteItemWriter == null) ? 0 : deleteItemWriter.hashCode();
h$ *= 1000003;
h$ ^= deleteParameters.hashCode();
return h$;
}
@Override
ApiBase.UpsertItems.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends ApiBase.UpsertItems.Builder {
private Integer maxBatchSize;
private AuthConfig authConfig;
private Function> idFunction;
private Function> createMappingFunction;
private Function, List>> batchingFunction;
private Function> updateMappingFunction;
private Function, List> retrieveFunction;
private BiFunction equalFunction;
private Function itemMappingFunction;
private ConnectorServiceV1.ItemWriter createItemWriter;
private ConnectorServiceV1.ItemWriter updateItemWriter;
private ConnectorServiceV1.ItemWriter deleteItemWriter;
private ImmutableMap.Builder deleteParametersBuilder$;
private ImmutableMap deleteParameters;
Builder() {
}
private Builder(ApiBase.UpsertItems source) {
this.maxBatchSize = source.getMaxBatchSize();
this.authConfig = source.getAuthConfig();
this.idFunction = source.getIdFunction();
this.createMappingFunction = source.getCreateMappingFunction();
this.batchingFunction = source.getBatchingFunction();
this.updateMappingFunction = source.getUpdateMappingFunction();
this.retrieveFunction = source.getRetrieveFunction();
this.equalFunction = source.getEqualFunction();
this.itemMappingFunction = source.getItemMappingFunction();
this.createItemWriter = source.getCreateItemWriter();
this.updateItemWriter = source.getUpdateItemWriter();
this.deleteItemWriter = source.getDeleteItemWriter();
this.deleteParameters = source.getDeleteParameters();
}
@Override
ApiBase.UpsertItems.Builder setMaxBatchSize(int maxBatchSize) {
this.maxBatchSize = maxBatchSize;
return this;
}
@Override
ApiBase.UpsertItems.Builder setAuthConfig(AuthConfig authConfig) {
if (authConfig == null) {
throw new NullPointerException("Null authConfig");
}
this.authConfig = authConfig;
return this;
}
@Override
ApiBase.UpsertItems.Builder setIdFunction(Function> idFunction) {
if (idFunction == null) {
throw new NullPointerException("Null idFunction");
}
this.idFunction = idFunction;
return this;
}
@Override
ApiBase.UpsertItems.Builder setCreateMappingFunction(Function> createMappingFunction) {
if (createMappingFunction == null) {
throw new NullPointerException("Null createMappingFunction");
}
this.createMappingFunction = createMappingFunction;
return this;
}
@Override
ApiBase.UpsertItems.Builder setBatchingFunction(Function, List>> batchingFunction) {
if (batchingFunction == null) {
throw new NullPointerException("Null batchingFunction");
}
this.batchingFunction = batchingFunction;
return this;
}
@Override
ApiBase.UpsertItems.Builder setUpdateMappingFunction(Function> updateMappingFunction) {
this.updateMappingFunction = updateMappingFunction;
return this;
}
@Override
ApiBase.UpsertItems.Builder setRetrieveFunction(Function, List> retrieveFunction) {
this.retrieveFunction = retrieveFunction;
return this;
}
@Override
ApiBase.UpsertItems.Builder setEqualFunction(BiFunction equalFunction) {
this.equalFunction = equalFunction;
return this;
}
@Override
ApiBase.UpsertItems.Builder setItemMappingFunction(Function itemMappingFunction) {
this.itemMappingFunction = itemMappingFunction;
return this;
}
@Override
ApiBase.UpsertItems.Builder setCreateItemWriter(ConnectorServiceV1.ItemWriter createItemWriter) {
if (createItemWriter == null) {
throw new NullPointerException("Null createItemWriter");
}
this.createItemWriter = createItemWriter;
return this;
}
@Override
ApiBase.UpsertItems.Builder setUpdateItemWriter(ConnectorServiceV1.ItemWriter updateItemWriter) {
this.updateItemWriter = updateItemWriter;
return this;
}
@Override
ApiBase.UpsertItems.Builder setDeleteItemWriter(ConnectorServiceV1.ItemWriter deleteItemWriter) {
this.deleteItemWriter = deleteItemWriter;
return this;
}
@Override
ImmutableMap.Builder deleteParametersBuilder() {
if (deleteParametersBuilder$ == null) {
if (deleteParameters == null) {
deleteParametersBuilder$ = ImmutableMap.builder();
} else {
deleteParametersBuilder$ = ImmutableMap.builder();
deleteParametersBuilder$.putAll(deleteParameters);
deleteParameters = null;
}
}
return deleteParametersBuilder$;
}
@Override
ApiBase.UpsertItems build() {
if (deleteParametersBuilder$ != null) {
this.deleteParameters = deleteParametersBuilder$.build();
} else if (this.deleteParameters == null) {
this.deleteParameters = ImmutableMap.of();
}
if (this.maxBatchSize == null
|| this.authConfig == null
|| this.idFunction == null
|| this.createMappingFunction == null
|| this.batchingFunction == null
|| this.createItemWriter == null) {
StringBuilder missing = new StringBuilder();
if (this.maxBatchSize == null) {
missing.append(" maxBatchSize");
}
if (this.authConfig == null) {
missing.append(" authConfig");
}
if (this.idFunction == null) {
missing.append(" idFunction");
}
if (this.createMappingFunction == null) {
missing.append(" createMappingFunction");
}
if (this.batchingFunction == null) {
missing.append(" batchingFunction");
}
if (this.createItemWriter == null) {
missing.append(" createItemWriter");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ApiBase_UpsertItems(
this.maxBatchSize,
this.authConfig,
this.idFunction,
this.createMappingFunction,
this.batchingFunction,
this.updateMappingFunction,
this.retrieveFunction,
this.equalFunction,
this.itemMappingFunction,
this.createItemWriter,
this.updateItemWriter,
this.deleteItemWriter,
this.deleteParameters);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy