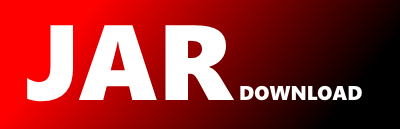
com.cognite.client.AutoValue_CogniteClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdf-sdk-java Show documentation
Show all versions of cdf-sdk-java Show documentation
Java SDK for reading and writing from/to CDF resources.
package com.cognite.client;
import com.cognite.client.config.ClientConfig;
import java.net.URL;
import java.util.function.Supplier;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import okhttp3.OkHttpClient;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_CogniteClient extends CogniteClient {
private final String project;
private final String clientId;
private final String clientSecret;
private final URL tokenUrl;
private final String apiKey;
private final Supplier tokenSupplier;
private final CogniteClient.AuthType authType;
private final String baseUrl;
private final ClientConfig clientConfig;
private final OkHttpClient httpClient;
private AutoValue_CogniteClient(
@Nullable String project,
@Nullable String clientId,
@Nullable String clientSecret,
@Nullable URL tokenUrl,
@Nullable String apiKey,
@Nullable Supplier tokenSupplier,
CogniteClient.AuthType authType,
String baseUrl,
ClientConfig clientConfig,
OkHttpClient httpClient) {
this.project = project;
this.clientId = clientId;
this.clientSecret = clientSecret;
this.tokenUrl = tokenUrl;
this.apiKey = apiKey;
this.tokenSupplier = tokenSupplier;
this.authType = authType;
this.baseUrl = baseUrl;
this.clientConfig = clientConfig;
this.httpClient = httpClient;
}
@Nullable
@Override
protected String getProject() {
return project;
}
@Nullable
@Override
protected String getClientId() {
return clientId;
}
@Nullable
@Override
protected String getClientSecret() {
return clientSecret;
}
@Nullable
@Override
protected URL getTokenUrl() {
return tokenUrl;
}
@Nullable
@Override
protected String getApiKey() {
return apiKey;
}
@Nullable
@Override
protected Supplier getTokenSupplier() {
return tokenSupplier;
}
@Override
protected CogniteClient.AuthType getAuthType() {
return authType;
}
@Override
protected String getBaseUrl() {
return baseUrl;
}
@Override
public ClientConfig getClientConfig() {
return clientConfig;
}
@Override
public OkHttpClient getHttpClient() {
return httpClient;
}
@Override
public String toString() {
return "CogniteClient{"
+ "project=" + project + ", "
+ "clientId=" + clientId + ", "
+ "clientSecret=" + clientSecret + ", "
+ "tokenUrl=" + tokenUrl + ", "
+ "apiKey=" + apiKey + ", "
+ "tokenSupplier=" + tokenSupplier + ", "
+ "authType=" + authType + ", "
+ "baseUrl=" + baseUrl + ", "
+ "clientConfig=" + clientConfig + ", "
+ "httpClient=" + httpClient
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof CogniteClient) {
CogniteClient that = (CogniteClient) o;
return (this.project == null ? that.getProject() == null : this.project.equals(that.getProject()))
&& (this.clientId == null ? that.getClientId() == null : this.clientId.equals(that.getClientId()))
&& (this.clientSecret == null ? that.getClientSecret() == null : this.clientSecret.equals(that.getClientSecret()))
&& (this.tokenUrl == null ? that.getTokenUrl() == null : this.tokenUrl.equals(that.getTokenUrl()))
&& (this.apiKey == null ? that.getApiKey() == null : this.apiKey.equals(that.getApiKey()))
&& (this.tokenSupplier == null ? that.getTokenSupplier() == null : this.tokenSupplier.equals(that.getTokenSupplier()))
&& this.authType.equals(that.getAuthType())
&& this.baseUrl.equals(that.getBaseUrl())
&& this.clientConfig.equals(that.getClientConfig())
&& this.httpClient.equals(that.getHttpClient());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (project == null) ? 0 : project.hashCode();
h$ *= 1000003;
h$ ^= (clientId == null) ? 0 : clientId.hashCode();
h$ *= 1000003;
h$ ^= (clientSecret == null) ? 0 : clientSecret.hashCode();
h$ *= 1000003;
h$ ^= (tokenUrl == null) ? 0 : tokenUrl.hashCode();
h$ *= 1000003;
h$ ^= (apiKey == null) ? 0 : apiKey.hashCode();
h$ *= 1000003;
h$ ^= (tokenSupplier == null) ? 0 : tokenSupplier.hashCode();
h$ *= 1000003;
h$ ^= authType.hashCode();
h$ *= 1000003;
h$ ^= baseUrl.hashCode();
h$ *= 1000003;
h$ ^= clientConfig.hashCode();
h$ *= 1000003;
h$ ^= httpClient.hashCode();
return h$;
}
@Override
protected CogniteClient.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends CogniteClient.Builder {
private String project;
private String clientId;
private String clientSecret;
private URL tokenUrl;
private String apiKey;
private Supplier tokenSupplier;
private CogniteClient.AuthType authType;
private String baseUrl;
private ClientConfig clientConfig;
private OkHttpClient httpClient;
Builder() {
}
private Builder(CogniteClient source) {
this.project = source.getProject();
this.clientId = source.getClientId();
this.clientSecret = source.getClientSecret();
this.tokenUrl = source.getTokenUrl();
this.apiKey = source.getApiKey();
this.tokenSupplier = source.getTokenSupplier();
this.authType = source.getAuthType();
this.baseUrl = source.getBaseUrl();
this.clientConfig = source.getClientConfig();
this.httpClient = source.getHttpClient();
}
@Override
CogniteClient.Builder setProject(String project) {
this.project = project;
return this;
}
@Override
CogniteClient.Builder setClientId(String clientId) {
this.clientId = clientId;
return this;
}
@Override
CogniteClient.Builder setClientSecret(String clientSecret) {
this.clientSecret = clientSecret;
return this;
}
@Override
CogniteClient.Builder setTokenUrl(URL tokenUrl) {
this.tokenUrl = tokenUrl;
return this;
}
@Override
CogniteClient.Builder setApiKey(String apiKey) {
this.apiKey = apiKey;
return this;
}
@Override
CogniteClient.Builder setTokenSupplier(Supplier tokenSupplier) {
this.tokenSupplier = tokenSupplier;
return this;
}
@Override
CogniteClient.Builder setAuthType(CogniteClient.AuthType authType) {
if (authType == null) {
throw new NullPointerException("Null authType");
}
this.authType = authType;
return this;
}
@Override
CogniteClient.Builder setBaseUrl(String baseUrl) {
if (baseUrl == null) {
throw new NullPointerException("Null baseUrl");
}
this.baseUrl = baseUrl;
return this;
}
@Override
CogniteClient.Builder setClientConfig(ClientConfig clientConfig) {
if (clientConfig == null) {
throw new NullPointerException("Null clientConfig");
}
this.clientConfig = clientConfig;
return this;
}
@Override
CogniteClient.Builder setHttpClient(OkHttpClient httpClient) {
if (httpClient == null) {
throw new NullPointerException("Null httpClient");
}
this.httpClient = httpClient;
return this;
}
@Override
CogniteClient build() {
if (this.authType == null
|| this.baseUrl == null
|| this.clientConfig == null
|| this.httpClient == null) {
StringBuilder missing = new StringBuilder();
if (this.authType == null) {
missing.append(" authType");
}
if (this.baseUrl == null) {
missing.append(" baseUrl");
}
if (this.clientConfig == null) {
missing.append(" clientConfig");
}
if (this.httpClient == null) {
missing.append(" httpClient");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_CogniteClient(
this.project,
this.clientId,
this.clientSecret,
this.tokenUrl,
this.apiKey,
this.tokenSupplier,
this.authType,
this.baseUrl,
this.clientConfig,
this.httpClient);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy