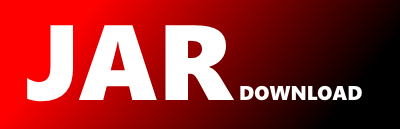
com.cognite.client.servicesV1.ResponseItems Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdf-sdk-java Show documentation
Show all versions of cdf-sdk-java Show documentation
Java SDK for reading and writing from/to CDF resources.
/*
* Copyright (c) 2020 Cognite AS
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.cognite.client.servicesV1;
import com.cognite.client.servicesV1.response.JsonErrorItemResponseParser;
import com.cognite.client.servicesV1.response.JsonStringAttributeResponseParser;
import com.cognite.client.servicesV1.response.ResponseParser;
import com.google.auto.value.AutoValue;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableList;
import javax.annotation.Nullable;
import java.io.Serializable;
/**
* This class represents a collection of response items from a Cognite API request.
*
*
*/
@AutoValue
public abstract class ResponseItems implements Serializable {
static Builder builder() {
return new com.cognite.client.servicesV1.AutoValue_ResponseItems.Builder()
.setDuplicateResponseParser(JsonErrorItemResponseParser.builder()
.setErrorSubPath("duplicated")
.build())
.setMissingResponseParser(JsonErrorItemResponseParser.builder()
.setErrorSubPath("missing")
.build())
.setErrorMessageResponseParser(JsonStringAttributeResponseParser.create()
.withAttributePath("error.message"))
.setStatusResponseParser(JsonStringAttributeResponseParser.create()
.withAttributePath("error.code"));
}
public static ResponseItems of(ResponseParser responseParser, ResponseBinary response) {
Preconditions.checkNotNull(responseParser, "Response parser cannot be null.");
Preconditions.checkNotNull(response, "Response binary cannot be null.");
return ResponseItems.builder()
.setResponseParser(responseParser)
.setResponseBinary(response)
.build();
}
abstract Builder toBuilder();
abstract ResponseParser getResponseParser();
abstract ResponseParser getDuplicateResponseParser();
abstract ResponseParser getMissingResponseParser();
abstract ResponseParser getErrorMessageResponseParser();
abstract ResponseParser getStatusResponseParser();
@Nullable
abstract ImmutableList getResultsItemsList();
public abstract ResponseBinary getResponseBinary();
/**
* Specifies the parser for extracting duplicate items from the response payload.
*
* The default parser looks in the *duplicated* object of the response json.
* @param parser
* @return
*/
public ResponseItems withDuplicateResponseParser(ResponseParser parser) {
Preconditions.checkNotNull(parser, "Parser cannot be null.");
return toBuilder().setDuplicateResponseParser(parser).build();
}
/**
* Specifies the parser for extracting missing items from the response payload.
*
* The default parser looks in the *missing* object of the response json.
* @param parser
* @return
*/
public ResponseItems withMissingResponseParser(ResponseParser parser) {
Preconditions.checkNotNull(parser, "Parser cannot be null.");
return toBuilder().setMissingResponseParser(parser).build();
}
/**
* Specifies the parser for extracting the error message from the response payload.
*
* The default parser looks in the *message* attribute of the response json.
* @param parser
* @return
*/
public ResponseItems withErrorMessageResponseParser(ResponseParser parser) {
Preconditions.checkNotNull(parser, "Parser cannot be null.");
return toBuilder().setErrorMessageResponseParser(parser).build();
}
/**
* Specifies the parser for extracting the error code (http status code) from the response payload.
*
* The default parser looks in the *code* attribute of the response json.
* @param parser
* @return
*/
public ResponseItems withStatusResponseParser(ResponseParser parser) {
Preconditions.checkNotNull(parser, "Parser cannot be null.");
return toBuilder().setStatusResponseParser(parser).build();
}
/**
* Specifies a results items list. By setting this list you will override the default behavior
* which is to parse the results items from the response body (bytes).
*
* That is, if a results items list is specified, the parser will not attempt to parse the response body.
*
* @param itemsList
* @return
*/
public ResponseItems withResultsItemsList(ImmutableList itemsList) {
return toBuilder().setResultsItemsList(itemsList).build();
}
/**
* Returns the main result items.
*
* @return A list of result items.
* @throws Exception
*/
public ImmutableList getResultsItems() throws Exception {
if (null != getResultsItemsList()) {
// a results items list is specified, so this will override the parser
return getResultsItemsList();
}
return getResponseParser().extractItems(getResponseBinary().getResponseBodyBytes().toByteArray());
}
/**
* Returns a list of duplicate items.
*
*
* @return A list of duplicate items.
* @throws Exception
*/
public ImmutableList getDuplicateItems() throws Exception {
return getDuplicateResponseParser().extractItems(getResponseBinary().getResponseBodyBytes().toByteArray());
}
public ImmutableList getMissingItems() throws Exception {
return getMissingResponseParser().extractItems(getResponseBinary().getResponseBodyBytes().toByteArray());
}
public ImmutableList getStatus() throws Exception {
return getStatusResponseParser().extractItems(getResponseBinary().getResponseBodyBytes().toByteArray());
}
public ImmutableList getErrorMessage() throws Exception {
return getErrorMessageResponseParser().extractItems(getResponseBinary().getResponseBodyBytes().toByteArray());
}
public String getResponseBodyAsString() {
return getResponseBinary().getResponseBodyBytes().toStringUtf8();
}
public boolean isSuccessful() {
return getResponseBinary().getResponse().isSuccessful();
}
@AutoValue.Builder
abstract static class Builder {
abstract Builder setResponseParser(ResponseParser value);
abstract Builder setDuplicateResponseParser(ResponseParser value);
abstract Builder setMissingResponseParser(ResponseParser value);
abstract Builder setErrorMessageResponseParser(ResponseParser value);
abstract Builder setStatusResponseParser(ResponseParser value);
abstract Builder setResponseBinary(ResponseBinary value);
abstract Builder setResultsItemsList(ImmutableList value);
abstract ResponseItems build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy