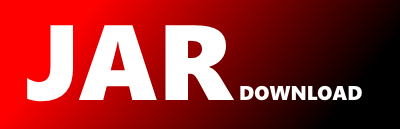
com.cognite.client.servicesV1.executor.AutoValue_FileBinaryRequestExecutor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdf-sdk-java Show documentation
Show all versions of cdf-sdk-java Show documentation
Java SDK for reading and writing from/to CDF resources.
package com.cognite.client.servicesV1.executor;
import java.net.URI;
import java.util.List;
import java.util.concurrent.Executor;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import okhttp3.OkHttpClient;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_FileBinaryRequestExecutor extends FileBinaryRequestExecutor {
private final OkHttpClient httpClient;
private final List validResponseCodes;
private final Executor executor;
private final int maxRetries;
private final boolean forceTempStorage;
private final boolean deleteTempFile;
private final URI tempStoragePath;
private AutoValue_FileBinaryRequestExecutor(
OkHttpClient httpClient,
List validResponseCodes,
Executor executor,
int maxRetries,
boolean forceTempStorage,
boolean deleteTempFile,
@Nullable URI tempStoragePath) {
this.httpClient = httpClient;
this.validResponseCodes = validResponseCodes;
this.executor = executor;
this.maxRetries = maxRetries;
this.forceTempStorage = forceTempStorage;
this.deleteTempFile = deleteTempFile;
this.tempStoragePath = tempStoragePath;
}
@Override
OkHttpClient getHttpClient() {
return httpClient;
}
@Override
List getValidResponseCodes() {
return validResponseCodes;
}
@Override
Executor getExecutor() {
return executor;
}
@Override
int getMaxRetries() {
return maxRetries;
}
@Override
boolean isForceTempStorage() {
return forceTempStorage;
}
@Override
boolean isDeleteTempFile() {
return deleteTempFile;
}
@Nullable
@Override
URI getTempStoragePath() {
return tempStoragePath;
}
@Override
public String toString() {
return "FileBinaryRequestExecutor{"
+ "httpClient=" + httpClient + ", "
+ "validResponseCodes=" + validResponseCodes + ", "
+ "executor=" + executor + ", "
+ "maxRetries=" + maxRetries + ", "
+ "forceTempStorage=" + forceTempStorage + ", "
+ "deleteTempFile=" + deleteTempFile + ", "
+ "tempStoragePath=" + tempStoragePath
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof FileBinaryRequestExecutor) {
FileBinaryRequestExecutor that = (FileBinaryRequestExecutor) o;
return this.httpClient.equals(that.getHttpClient())
&& this.validResponseCodes.equals(that.getValidResponseCodes())
&& this.executor.equals(that.getExecutor())
&& this.maxRetries == that.getMaxRetries()
&& this.forceTempStorage == that.isForceTempStorage()
&& this.deleteTempFile == that.isDeleteTempFile()
&& (this.tempStoragePath == null ? that.getTempStoragePath() == null : this.tempStoragePath.equals(that.getTempStoragePath()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= httpClient.hashCode();
h$ *= 1000003;
h$ ^= validResponseCodes.hashCode();
h$ *= 1000003;
h$ ^= executor.hashCode();
h$ *= 1000003;
h$ ^= maxRetries;
h$ *= 1000003;
h$ ^= forceTempStorage ? 1231 : 1237;
h$ *= 1000003;
h$ ^= deleteTempFile ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (tempStoragePath == null) ? 0 : tempStoragePath.hashCode();
return h$;
}
@Override
FileBinaryRequestExecutor.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends FileBinaryRequestExecutor.Builder {
private OkHttpClient httpClient;
private List validResponseCodes;
private Executor executor;
private Integer maxRetries;
private Boolean forceTempStorage;
private Boolean deleteTempFile;
private URI tempStoragePath;
Builder() {
}
private Builder(FileBinaryRequestExecutor source) {
this.httpClient = source.getHttpClient();
this.validResponseCodes = source.getValidResponseCodes();
this.executor = source.getExecutor();
this.maxRetries = source.getMaxRetries();
this.forceTempStorage = source.isForceTempStorage();
this.deleteTempFile = source.isDeleteTempFile();
this.tempStoragePath = source.getTempStoragePath();
}
@Override
FileBinaryRequestExecutor.Builder setHttpClient(OkHttpClient httpClient) {
if (httpClient == null) {
throw new NullPointerException("Null httpClient");
}
this.httpClient = httpClient;
return this;
}
@Override
FileBinaryRequestExecutor.Builder setValidResponseCodes(List validResponseCodes) {
if (validResponseCodes == null) {
throw new NullPointerException("Null validResponseCodes");
}
this.validResponseCodes = validResponseCodes;
return this;
}
@Override
FileBinaryRequestExecutor.Builder setExecutor(Executor executor) {
if (executor == null) {
throw new NullPointerException("Null executor");
}
this.executor = executor;
return this;
}
@Override
FileBinaryRequestExecutor.Builder setMaxRetries(int maxRetries) {
this.maxRetries = maxRetries;
return this;
}
@Override
FileBinaryRequestExecutor.Builder setForceTempStorage(boolean forceTempStorage) {
this.forceTempStorage = forceTempStorage;
return this;
}
@Override
FileBinaryRequestExecutor.Builder setDeleteTempFile(boolean deleteTempFile) {
this.deleteTempFile = deleteTempFile;
return this;
}
@Override
FileBinaryRequestExecutor.Builder setTempStoragePath(URI tempStoragePath) {
this.tempStoragePath = tempStoragePath;
return this;
}
@Override
FileBinaryRequestExecutor autoBuild() {
if (this.httpClient == null
|| this.validResponseCodes == null
|| this.executor == null
|| this.maxRetries == null
|| this.forceTempStorage == null
|| this.deleteTempFile == null) {
StringBuilder missing = new StringBuilder();
if (this.httpClient == null) {
missing.append(" httpClient");
}
if (this.validResponseCodes == null) {
missing.append(" validResponseCodes");
}
if (this.executor == null) {
missing.append(" executor");
}
if (this.maxRetries == null) {
missing.append(" maxRetries");
}
if (this.forceTempStorage == null) {
missing.append(" forceTempStorage");
}
if (this.deleteTempFile == null) {
missing.append(" deleteTempFile");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_FileBinaryRequestExecutor(
this.httpClient,
this.validResponseCodes,
this.executor,
this.maxRetries,
this.forceTempStorage,
this.deleteTempFile,
this.tempStoragePath);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy