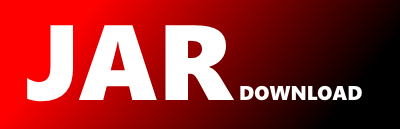
com.cognite.client.servicesV1.request.AutoValue_RawWriteTablesRequestProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdf-sdk-java Show documentation
Show all versions of cdf-sdk-java Show documentation
Java SDK for reading and writing from/to CDF resources.
package com.cognite.client.servicesV1.request;
import com.cognite.client.Request;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_RawWriteTablesRequestProvider extends RawWriteTablesRequestProvider {
private final String sdkIdentifier;
private final String appIdentifier;
private final String sessionIdentifier;
private final String endpoint;
private final Request request;
private final boolean betaEnabled;
private AutoValue_RawWriteTablesRequestProvider(
String sdkIdentifier,
String appIdentifier,
String sessionIdentifier,
String endpoint,
Request request,
boolean betaEnabled) {
this.sdkIdentifier = sdkIdentifier;
this.appIdentifier = appIdentifier;
this.sessionIdentifier = sessionIdentifier;
this.endpoint = endpoint;
this.request = request;
this.betaEnabled = betaEnabled;
}
@Override
public String getSdkIdentifier() {
return sdkIdentifier;
}
@Override
public String getAppIdentifier() {
return appIdentifier;
}
@Override
public String getSessionIdentifier() {
return sessionIdentifier;
}
@Override
public String getEndpoint() {
return endpoint;
}
@Override
public Request getRequest() {
return request;
}
@Override
public boolean isBetaEnabled() {
return betaEnabled;
}
@Override
public String toString() {
return "RawWriteTablesRequestProvider{"
+ "sdkIdentifier=" + sdkIdentifier + ", "
+ "appIdentifier=" + appIdentifier + ", "
+ "sessionIdentifier=" + sessionIdentifier + ", "
+ "endpoint=" + endpoint + ", "
+ "request=" + request + ", "
+ "betaEnabled=" + betaEnabled
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof RawWriteTablesRequestProvider) {
RawWriteTablesRequestProvider that = (RawWriteTablesRequestProvider) o;
return this.sdkIdentifier.equals(that.getSdkIdentifier())
&& this.appIdentifier.equals(that.getAppIdentifier())
&& this.sessionIdentifier.equals(that.getSessionIdentifier())
&& this.endpoint.equals(that.getEndpoint())
&& this.request.equals(that.getRequest())
&& this.betaEnabled == that.isBetaEnabled();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= sdkIdentifier.hashCode();
h$ *= 1000003;
h$ ^= appIdentifier.hashCode();
h$ *= 1000003;
h$ ^= sessionIdentifier.hashCode();
h$ *= 1000003;
h$ ^= endpoint.hashCode();
h$ *= 1000003;
h$ ^= request.hashCode();
h$ *= 1000003;
h$ ^= betaEnabled ? 1231 : 1237;
return h$;
}
@Override
public RawWriteTablesRequestProvider.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends RawWriteTablesRequestProvider.Builder {
private String sdkIdentifier;
private String appIdentifier;
private String sessionIdentifier;
private String endpoint;
private Request request;
private Boolean betaEnabled;
Builder() {
}
private Builder(RawWriteTablesRequestProvider source) {
this.sdkIdentifier = source.getSdkIdentifier();
this.appIdentifier = source.getAppIdentifier();
this.sessionIdentifier = source.getSessionIdentifier();
this.endpoint = source.getEndpoint();
this.request = source.getRequest();
this.betaEnabled = source.isBetaEnabled();
}
@Override
public RawWriteTablesRequestProvider.Builder setSdkIdentifier(String sdkIdentifier) {
if (sdkIdentifier == null) {
throw new NullPointerException("Null sdkIdentifier");
}
this.sdkIdentifier = sdkIdentifier;
return this;
}
@Override
public RawWriteTablesRequestProvider.Builder setAppIdentifier(String appIdentifier) {
if (appIdentifier == null) {
throw new NullPointerException("Null appIdentifier");
}
this.appIdentifier = appIdentifier;
return this;
}
@Override
public RawWriteTablesRequestProvider.Builder setSessionIdentifier(String sessionIdentifier) {
if (sessionIdentifier == null) {
throw new NullPointerException("Null sessionIdentifier");
}
this.sessionIdentifier = sessionIdentifier;
return this;
}
@Override
public RawWriteTablesRequestProvider.Builder setEndpoint(String endpoint) {
if (endpoint == null) {
throw new NullPointerException("Null endpoint");
}
this.endpoint = endpoint;
return this;
}
@Override
public RawWriteTablesRequestProvider.Builder setRequest(Request request) {
if (request == null) {
throw new NullPointerException("Null request");
}
this.request = request;
return this;
}
@Override
public RawWriteTablesRequestProvider.Builder setBetaEnabled(boolean betaEnabled) {
this.betaEnabled = betaEnabled;
return this;
}
@Override
public RawWriteTablesRequestProvider build() {
if (this.sdkIdentifier == null
|| this.appIdentifier == null
|| this.sessionIdentifier == null
|| this.endpoint == null
|| this.request == null
|| this.betaEnabled == null) {
StringBuilder missing = new StringBuilder();
if (this.sdkIdentifier == null) {
missing.append(" sdkIdentifier");
}
if (this.appIdentifier == null) {
missing.append(" appIdentifier");
}
if (this.sessionIdentifier == null) {
missing.append(" sessionIdentifier");
}
if (this.endpoint == null) {
missing.append(" endpoint");
}
if (this.request == null) {
missing.append(" request");
}
if (this.betaEnabled == null) {
missing.append(" betaEnabled");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_RawWriteTablesRequestProvider(
this.sdkIdentifier,
this.appIdentifier,
this.sessionIdentifier,
this.endpoint,
this.request,
this.betaEnabled);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy