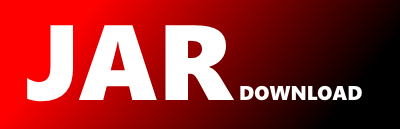
com.cognite.client.servicesV1.request.TSPointsReadProtoRequestProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdf-sdk-java Show documentation
Show all versions of cdf-sdk-java Show documentation
Java SDK for reading and writing from/to CDF resources.
/*
* Copyright (c) 2020 Cognite AS
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.cognite.client.servicesV1.request;
import com.cognite.client.servicesV1.ConnectorConstants;
import com.cognite.client.Request;
import com.cognite.client.servicesV1.util.TSIterationUtilities;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.ObjectReader;
import com.google.auto.value.AutoValue;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import okhttp3.MediaType;
import okhttp3.RequestBody;
import org.apache.commons.lang3.RandomStringUtils;
import java.util.*;
@AutoValue
public abstract class TSPointsReadProtoRequestProvider extends GenericRequestProvider {
private static final ObjectMapper mapper = new ObjectMapper();
private static final int MAX_TS_ITEMS = 100;
private final ObjectReader objectReader = mapper.reader();
public static Builder builder() {
return new com.cognite.client.servicesV1.request.AutoValue_TSPointsReadProtoRequestProvider.Builder()
.setRequest(Request.create())
.setSdkIdentifier(ConnectorConstants.SDK_IDENTIFIER)
.setAppIdentifier(ConnectorConstants.DEFAULT_APP_IDENTIFIER)
.setSessionIdentifier(ConnectorConstants.DEFAULT_SESSION_IDENTIFIER)
.setBetaEnabled(ConnectorConstants.DEFAULT_BETA_ENABLED);
}
public abstract Builder toBuilder();
public TSPointsReadProtoRequestProvider withRequest(Request parameters) {
Preconditions.checkNotNull(parameters, "Request parameters cannot be null.");
Preconditions.checkArgument(parameters.getItems().size() <= MAX_TS_ITEMS,
"Datapoints can only be requested for maximum " + MAX_TS_ITEMS + " time series per request.");
Preconditions.checkArgument(parameters.getItems().get(0).containsKey("id")
|| parameters.getItems().get(0).containsKey("externalId"),
"The request must contain an id or externalId");
for (Map item : parameters.getItems()) {
checkItemPreconditions(item);
}
return toBuilder().setRequest(parameters).build();
}
public okhttp3.Request buildRequest(Optional cursor) throws Exception {
final String randomString = RandomStringUtils.randomAlphanumeric(5);
final String logPrefix = "Build read TS datapoints request - ";
Request requestParameters = getRequest();
okhttp3.Request.Builder requestBuilder = buildGenericRequest();
// Check for limit
if (!requestParameters.getRequestParameters().containsKey("limit")) {
if (requestParameters.getRequestParameters().containsKey("aggregates")) {
requestParameters = requestParameters.withRootParameter("limit",
ConnectorConstants.DEFAULT_MAX_BATCH_SIZE_TS_DATAPOINTS_AGG);
LOG.info(logPrefix + "Request does not contain a *limit* parameter. Setting default limit: "
+ ConnectorConstants.DEFAULT_MAX_BATCH_SIZE_TS_DATAPOINTS);
} else {
requestParameters = requestParameters.withRootParameter("limit",
ConnectorConstants.DEFAULT_MAX_BATCH_SIZE_TS_DATAPOINTS);
LOG.info(logPrefix + "Request does not contain a *limit* parameter. Setting default limit: "
+ ConnectorConstants.DEFAULT_MAX_BATCH_SIZE_TS_DATAPOINTS);
}
}
if (cursor.isPresent()) {
LOG.debug(logPrefix + "Adding cursor to the request.");
LOG.debug(logPrefix + "Cursor: \r\n" + cursor.get());
// The cursor should be a map of all (valid) TS items and their start timestamp
ImmutableList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy