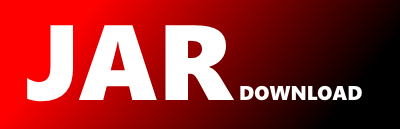
com.cognite.client.stream.AutoValue_Publisher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdf-sdk-java Show documentation
Show all versions of cdf-sdk-java Show documentation
Java SDK for reading and writing from/to CDF resources.
package com.cognite.client.stream;
import com.cognite.client.Request;
import java.time.Duration;
import java.time.Instant;
import java.util.List;
import java.util.function.Consumer;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Publisher extends Publisher {
private final Duration pollingInterval;
private final Duration pollingOffset;
private final Instant startTime;
private final Instant endTime;
private final ListSource source;
private final Request request;
private final Consumer> consumer;
private AutoValue_Publisher(
Duration pollingInterval,
Duration pollingOffset,
Instant startTime,
Instant endTime,
ListSource source,
Request request,
@Nullable Consumer> consumer) {
this.pollingInterval = pollingInterval;
this.pollingOffset = pollingOffset;
this.startTime = startTime;
this.endTime = endTime;
this.source = source;
this.request = request;
this.consumer = consumer;
}
@Override
protected Duration getPollingInterval() {
return pollingInterval;
}
@Override
protected Duration getPollingOffset() {
return pollingOffset;
}
@Override
protected Instant getStartTime() {
return startTime;
}
@Override
protected Instant getEndTime() {
return endTime;
}
@Override
ListSource getSource() {
return source;
}
@Override
Request getRequest() {
return request;
}
@Nullable
@Override
Consumer> getConsumer() {
return consumer;
}
@Override
public String toString() {
return "Publisher{"
+ "pollingInterval=" + pollingInterval + ", "
+ "pollingOffset=" + pollingOffset + ", "
+ "startTime=" + startTime + ", "
+ "endTime=" + endTime + ", "
+ "source=" + source + ", "
+ "request=" + request + ", "
+ "consumer=" + consumer
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Publisher) {
Publisher> that = (Publisher>) o;
return this.pollingInterval.equals(that.getPollingInterval())
&& this.pollingOffset.equals(that.getPollingOffset())
&& this.startTime.equals(that.getStartTime())
&& this.endTime.equals(that.getEndTime())
&& this.source.equals(that.getSource())
&& this.request.equals(that.getRequest())
&& (this.consumer == null ? that.getConsumer() == null : this.consumer.equals(that.getConsumer()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= pollingInterval.hashCode();
h$ *= 1000003;
h$ ^= pollingOffset.hashCode();
h$ *= 1000003;
h$ ^= startTime.hashCode();
h$ *= 1000003;
h$ ^= endTime.hashCode();
h$ *= 1000003;
h$ ^= source.hashCode();
h$ *= 1000003;
h$ ^= request.hashCode();
h$ *= 1000003;
h$ ^= (consumer == null) ? 0 : consumer.hashCode();
return h$;
}
@Override
Publisher.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends Publisher.Builder {
private Duration pollingInterval;
private Duration pollingOffset;
private Instant startTime;
private Instant endTime;
private ListSource source;
private Request request;
private Consumer> consumer;
Builder() {
}
private Builder(Publisher source) {
this.pollingInterval = source.getPollingInterval();
this.pollingOffset = source.getPollingOffset();
this.startTime = source.getStartTime();
this.endTime = source.getEndTime();
this.source = source.getSource();
this.request = source.getRequest();
this.consumer = source.getConsumer();
}
@Override
Publisher.Builder setPollingInterval(Duration pollingInterval) {
if (pollingInterval == null) {
throw new NullPointerException("Null pollingInterval");
}
this.pollingInterval = pollingInterval;
return this;
}
@Override
Publisher.Builder setPollingOffset(Duration pollingOffset) {
if (pollingOffset == null) {
throw new NullPointerException("Null pollingOffset");
}
this.pollingOffset = pollingOffset;
return this;
}
@Override
Publisher.Builder setStartTime(Instant startTime) {
if (startTime == null) {
throw new NullPointerException("Null startTime");
}
this.startTime = startTime;
return this;
}
@Override
Publisher.Builder setEndTime(Instant endTime) {
if (endTime == null) {
throw new NullPointerException("Null endTime");
}
this.endTime = endTime;
return this;
}
@Override
Publisher.Builder setSource(ListSource source) {
if (source == null) {
throw new NullPointerException("Null source");
}
this.source = source;
return this;
}
@Override
Publisher.Builder setRequest(Request request) {
if (request == null) {
throw new NullPointerException("Null request");
}
this.request = request;
return this;
}
@Override
Publisher.Builder setConsumer(Consumer> consumer) {
this.consumer = consumer;
return this;
}
@Override
Publisher build() {
if (this.pollingInterval == null
|| this.pollingOffset == null
|| this.startTime == null
|| this.endTime == null
|| this.source == null
|| this.request == null) {
StringBuilder missing = new StringBuilder();
if (this.pollingInterval == null) {
missing.append(" pollingInterval");
}
if (this.pollingOffset == null) {
missing.append(" pollingOffset");
}
if (this.startTime == null) {
missing.append(" startTime");
}
if (this.endTime == null) {
missing.append(" endTime");
}
if (this.source == null) {
missing.append(" source");
}
if (this.request == null) {
missing.append(" request");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_Publisher(
this.pollingInterval,
this.pollingOffset,
this.startTime,
this.endTime,
this.source,
this.request,
this.consumer);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy