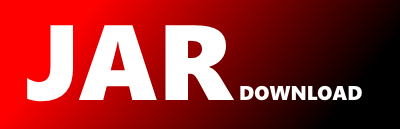
proto.com.cognite.client.dto.ThreeDNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdf-sdk-java Show documentation
Show all versions of cdf-sdk-java Show documentation
Java SDK for reading and writing from/to CDF resources.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: three_d_model.proto
package com.cognite.client.dto;
/**
* Protobuf type {@code com.cognite.beam.proto.ThreeDNode}
*/
public final class ThreeDNode extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.cognite.beam.proto.ThreeDNode)
ThreeDNodeOrBuilder {
private static final long serialVersionUID = 0L;
// Use ThreeDNode.newBuilder() to construct.
private ThreeDNode(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ThreeDNode() {
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ThreeDNode();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ThreeDNode(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
id_ = input.readInt64();
break;
}
case 16: {
bitField0_ |= 0x00000002;
treeIndex_ = input.readInt64();
break;
}
case 24: {
bitField0_ |= 0x00000004;
parentId_ = input.readInt64();
break;
}
case 32: {
bitField0_ |= 0x00000008;
depth_ = input.readInt64();
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
name_ = s;
break;
}
case 48: {
bitField0_ |= 0x00000020;
subtreeSize_ = input.readInt64();
break;
}
case 58: {
com.cognite.client.dto.ThreeDNode.Properties.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) != 0)) {
subBuilder = properties_.toBuilder();
}
properties_ = input.readMessage(com.cognite.client.dto.ThreeDNode.Properties.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(properties_);
properties_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
case 66: {
com.cognite.client.dto.ThreeDNode.BoundingBox.Builder subBuilder = null;
if (((bitField0_ & 0x00000080) != 0)) {
subBuilder = boundingBox_.toBuilder();
}
boundingBox_ = input.readMessage(com.cognite.client.dto.ThreeDNode.BoundingBox.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(boundingBox_);
boundingBox_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000080;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.cognite.client.dto.ThreeDNode.class, com.cognite.client.dto.ThreeDNode.Builder.class);
}
public interface BoundingBoxOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.cognite.beam.proto.ThreeDNode.BoundingBox)
com.google.protobuf.MessageOrBuilder {
/**
* repeated double max = 1;
* @return A list containing the max.
*/
java.util.List getMaxList();
/**
* repeated double max = 1;
* @return The count of max.
*/
int getMaxCount();
/**
* repeated double max = 1;
* @param index The index of the element to return.
* @return The max at the given index.
*/
double getMax(int index);
/**
* repeated double min = 2;
* @return A list containing the min.
*/
java.util.List getMinList();
/**
* repeated double min = 2;
* @return The count of min.
*/
int getMinCount();
/**
* repeated double min = 2;
* @param index The index of the element to return.
* @return The min at the given index.
*/
double getMin(int index);
}
/**
* Protobuf type {@code com.cognite.beam.proto.ThreeDNode.BoundingBox}
*/
public static final class BoundingBox extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.cognite.beam.proto.ThreeDNode.BoundingBox)
BoundingBoxOrBuilder {
private static final long serialVersionUID = 0L;
// Use BoundingBox.newBuilder() to construct.
private BoundingBox(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BoundingBox() {
max_ = emptyDoubleList();
min_ = emptyDoubleList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new BoundingBox();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BoundingBox(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 9: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
max_ = newDoubleList();
mutable_bitField0_ |= 0x00000001;
}
max_.addDouble(input.readDouble());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) != 0) && input.getBytesUntilLimit() > 0) {
max_ = newDoubleList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
max_.addDouble(input.readDouble());
}
input.popLimit(limit);
break;
}
case 17: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
min_ = newDoubleList();
mutable_bitField0_ |= 0x00000002;
}
min_.addDouble(input.readDouble());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) != 0) && input.getBytesUntilLimit() > 0) {
min_ = newDoubleList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
min_.addDouble(input.readDouble());
}
input.popLimit(limit);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
max_.makeImmutable(); // C
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
min_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_BoundingBox_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_BoundingBox_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.cognite.client.dto.ThreeDNode.BoundingBox.class, com.cognite.client.dto.ThreeDNode.BoundingBox.Builder.class);
}
public static final int MAX_FIELD_NUMBER = 1;
private com.google.protobuf.Internal.DoubleList max_;
/**
* repeated double max = 1;
* @return A list containing the max.
*/
@java.lang.Override
public java.util.List
getMaxList() {
return max_;
}
/**
* repeated double max = 1;
* @return The count of max.
*/
public int getMaxCount() {
return max_.size();
}
/**
* repeated double max = 1;
* @param index The index of the element to return.
* @return The max at the given index.
*/
public double getMax(int index) {
return max_.getDouble(index);
}
private int maxMemoizedSerializedSize = -1;
public static final int MIN_FIELD_NUMBER = 2;
private com.google.protobuf.Internal.DoubleList min_;
/**
* repeated double min = 2;
* @return A list containing the min.
*/
@java.lang.Override
public java.util.List
getMinList() {
return min_;
}
/**
* repeated double min = 2;
* @return The count of min.
*/
public int getMinCount() {
return min_.size();
}
/**
* repeated double min = 2;
* @param index The index of the element to return.
* @return The min at the given index.
*/
public double getMin(int index) {
return min_.getDouble(index);
}
private int minMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getMaxList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(maxMemoizedSerializedSize);
}
for (int i = 0; i < max_.size(); i++) {
output.writeDoubleNoTag(max_.getDouble(i));
}
if (getMinList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(minMemoizedSerializedSize);
}
for (int i = 0; i < min_.size(); i++) {
output.writeDoubleNoTag(min_.getDouble(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
dataSize = 8 * getMaxList().size();
size += dataSize;
if (!getMaxList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
maxMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
dataSize = 8 * getMinList().size();
size += dataSize;
if (!getMinList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
minMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.cognite.client.dto.ThreeDNode.BoundingBox)) {
return super.equals(obj);
}
com.cognite.client.dto.ThreeDNode.BoundingBox other = (com.cognite.client.dto.ThreeDNode.BoundingBox) obj;
if (!getMaxList()
.equals(other.getMaxList())) return false;
if (!getMinList()
.equals(other.getMinList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getMaxCount() > 0) {
hash = (37 * hash) + MAX_FIELD_NUMBER;
hash = (53 * hash) + getMaxList().hashCode();
}
if (getMinCount() > 0) {
hash = (37 * hash) + MIN_FIELD_NUMBER;
hash = (53 * hash) + getMinList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.cognite.client.dto.ThreeDNode.BoundingBox parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.BoundingBox parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.BoundingBox parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.BoundingBox parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.BoundingBox parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.BoundingBox parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.BoundingBox parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.BoundingBox parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.BoundingBox parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.BoundingBox parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.BoundingBox parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.BoundingBox parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.cognite.client.dto.ThreeDNode.BoundingBox prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.cognite.beam.proto.ThreeDNode.BoundingBox}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.cognite.beam.proto.ThreeDNode.BoundingBox)
com.cognite.client.dto.ThreeDNode.BoundingBoxOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_BoundingBox_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_BoundingBox_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.cognite.client.dto.ThreeDNode.BoundingBox.class, com.cognite.client.dto.ThreeDNode.BoundingBox.Builder.class);
}
// Construct using com.cognite.client.dto.ThreeDNode.BoundingBox.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
max_ = emptyDoubleList();
bitField0_ = (bitField0_ & ~0x00000001);
min_ = emptyDoubleList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_BoundingBox_descriptor;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.BoundingBox getDefaultInstanceForType() {
return com.cognite.client.dto.ThreeDNode.BoundingBox.getDefaultInstance();
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.BoundingBox build() {
com.cognite.client.dto.ThreeDNode.BoundingBox result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.BoundingBox buildPartial() {
com.cognite.client.dto.ThreeDNode.BoundingBox result = new com.cognite.client.dto.ThreeDNode.BoundingBox(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
max_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.max_ = max_;
if (((bitField0_ & 0x00000002) != 0)) {
min_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.min_ = min_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.cognite.client.dto.ThreeDNode.BoundingBox) {
return mergeFrom((com.cognite.client.dto.ThreeDNode.BoundingBox)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.cognite.client.dto.ThreeDNode.BoundingBox other) {
if (other == com.cognite.client.dto.ThreeDNode.BoundingBox.getDefaultInstance()) return this;
if (!other.max_.isEmpty()) {
if (max_.isEmpty()) {
max_ = other.max_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureMaxIsMutable();
max_.addAll(other.max_);
}
onChanged();
}
if (!other.min_.isEmpty()) {
if (min_.isEmpty()) {
min_ = other.min_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureMinIsMutable();
min_.addAll(other.min_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.cognite.client.dto.ThreeDNode.BoundingBox parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.cognite.client.dto.ThreeDNode.BoundingBox) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.Internal.DoubleList max_ = emptyDoubleList();
private void ensureMaxIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
max_ = mutableCopy(max_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated double max = 1;
* @return A list containing the max.
*/
public java.util.List
getMaxList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(max_) : max_;
}
/**
* repeated double max = 1;
* @return The count of max.
*/
public int getMaxCount() {
return max_.size();
}
/**
* repeated double max = 1;
* @param index The index of the element to return.
* @return The max at the given index.
*/
public double getMax(int index) {
return max_.getDouble(index);
}
/**
* repeated double max = 1;
* @param index The index to set the value at.
* @param value The max to set.
* @return This builder for chaining.
*/
public Builder setMax(
int index, double value) {
ensureMaxIsMutable();
max_.setDouble(index, value);
onChanged();
return this;
}
/**
* repeated double max = 1;
* @param value The max to add.
* @return This builder for chaining.
*/
public Builder addMax(double value) {
ensureMaxIsMutable();
max_.addDouble(value);
onChanged();
return this;
}
/**
* repeated double max = 1;
* @param values The max to add.
* @return This builder for chaining.
*/
public Builder addAllMax(
java.lang.Iterable extends java.lang.Double> values) {
ensureMaxIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, max_);
onChanged();
return this;
}
/**
* repeated double max = 1;
* @return This builder for chaining.
*/
public Builder clearMax() {
max_ = emptyDoubleList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private com.google.protobuf.Internal.DoubleList min_ = emptyDoubleList();
private void ensureMinIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
min_ = mutableCopy(min_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated double min = 2;
* @return A list containing the min.
*/
public java.util.List
getMinList() {
return ((bitField0_ & 0x00000002) != 0) ?
java.util.Collections.unmodifiableList(min_) : min_;
}
/**
* repeated double min = 2;
* @return The count of min.
*/
public int getMinCount() {
return min_.size();
}
/**
* repeated double min = 2;
* @param index The index of the element to return.
* @return The min at the given index.
*/
public double getMin(int index) {
return min_.getDouble(index);
}
/**
* repeated double min = 2;
* @param index The index to set the value at.
* @param value The min to set.
* @return This builder for chaining.
*/
public Builder setMin(
int index, double value) {
ensureMinIsMutable();
min_.setDouble(index, value);
onChanged();
return this;
}
/**
* repeated double min = 2;
* @param value The min to add.
* @return This builder for chaining.
*/
public Builder addMin(double value) {
ensureMinIsMutable();
min_.addDouble(value);
onChanged();
return this;
}
/**
* repeated double min = 2;
* @param values The min to add.
* @return This builder for chaining.
*/
public Builder addAllMin(
java.lang.Iterable extends java.lang.Double> values) {
ensureMinIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, min_);
onChanged();
return this;
}
/**
* repeated double min = 2;
* @return This builder for chaining.
*/
public Builder clearMin() {
min_ = emptyDoubleList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.cognite.beam.proto.ThreeDNode.BoundingBox)
}
// @@protoc_insertion_point(class_scope:com.cognite.beam.proto.ThreeDNode.BoundingBox)
private static final com.cognite.client.dto.ThreeDNode.BoundingBox DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.cognite.client.dto.ThreeDNode.BoundingBox();
}
public static com.cognite.client.dto.ThreeDNode.BoundingBox getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BoundingBox parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BoundingBox(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.BoundingBox getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PropertiesOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.cognite.beam.proto.ThreeDNode.Properties)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
java.util.List
getCategoriesList();
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
com.cognite.client.dto.ThreeDNode.Categories getCategories(int index);
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
int getCategoriesCount();
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
java.util.List extends com.cognite.client.dto.ThreeDNode.CategoriesOrBuilder>
getCategoriesOrBuilderList();
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
com.cognite.client.dto.ThreeDNode.CategoriesOrBuilder getCategoriesOrBuilder(
int index);
}
/**
* Protobuf type {@code com.cognite.beam.proto.ThreeDNode.Properties}
*/
public static final class Properties extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.cognite.beam.proto.ThreeDNode.Properties)
PropertiesOrBuilder {
private static final long serialVersionUID = 0L;
// Use Properties.newBuilder() to construct.
private Properties(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Properties() {
categories_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Properties();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Properties(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
categories_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
categories_.add(
input.readMessage(com.cognite.client.dto.ThreeDNode.Categories.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
categories_ = java.util.Collections.unmodifiableList(categories_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_Properties_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_Properties_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.cognite.client.dto.ThreeDNode.Properties.class, com.cognite.client.dto.ThreeDNode.Properties.Builder.class);
}
public static final int CATEGORIES_FIELD_NUMBER = 1;
private java.util.List categories_;
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
@java.lang.Override
public java.util.List getCategoriesList() {
return categories_;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
@java.lang.Override
public java.util.List extends com.cognite.client.dto.ThreeDNode.CategoriesOrBuilder>
getCategoriesOrBuilderList() {
return categories_;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
@java.lang.Override
public int getCategoriesCount() {
return categories_.size();
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.Categories getCategories(int index) {
return categories_.get(index);
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.CategoriesOrBuilder getCategoriesOrBuilder(
int index) {
return categories_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < categories_.size(); i++) {
output.writeMessage(1, categories_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < categories_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, categories_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.cognite.client.dto.ThreeDNode.Properties)) {
return super.equals(obj);
}
com.cognite.client.dto.ThreeDNode.Properties other = (com.cognite.client.dto.ThreeDNode.Properties) obj;
if (!getCategoriesList()
.equals(other.getCategoriesList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getCategoriesCount() > 0) {
hash = (37 * hash) + CATEGORIES_FIELD_NUMBER;
hash = (53 * hash) + getCategoriesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.cognite.client.dto.ThreeDNode.Properties parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.Properties parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.Properties parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.Properties parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.Properties parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.Properties parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.Properties parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.Properties parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.Properties parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.Properties parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.Properties parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.Properties parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.cognite.client.dto.ThreeDNode.Properties prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.cognite.beam.proto.ThreeDNode.Properties}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.cognite.beam.proto.ThreeDNode.Properties)
com.cognite.client.dto.ThreeDNode.PropertiesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_Properties_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_Properties_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.cognite.client.dto.ThreeDNode.Properties.class, com.cognite.client.dto.ThreeDNode.Properties.Builder.class);
}
// Construct using com.cognite.client.dto.ThreeDNode.Properties.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCategoriesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (categoriesBuilder_ == null) {
categories_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
categoriesBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_Properties_descriptor;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.Properties getDefaultInstanceForType() {
return com.cognite.client.dto.ThreeDNode.Properties.getDefaultInstance();
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.Properties build() {
com.cognite.client.dto.ThreeDNode.Properties result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.Properties buildPartial() {
com.cognite.client.dto.ThreeDNode.Properties result = new com.cognite.client.dto.ThreeDNode.Properties(this);
int from_bitField0_ = bitField0_;
if (categoriesBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
categories_ = java.util.Collections.unmodifiableList(categories_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.categories_ = categories_;
} else {
result.categories_ = categoriesBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.cognite.client.dto.ThreeDNode.Properties) {
return mergeFrom((com.cognite.client.dto.ThreeDNode.Properties)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.cognite.client.dto.ThreeDNode.Properties other) {
if (other == com.cognite.client.dto.ThreeDNode.Properties.getDefaultInstance()) return this;
if (categoriesBuilder_ == null) {
if (!other.categories_.isEmpty()) {
if (categories_.isEmpty()) {
categories_ = other.categories_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureCategoriesIsMutable();
categories_.addAll(other.categories_);
}
onChanged();
}
} else {
if (!other.categories_.isEmpty()) {
if (categoriesBuilder_.isEmpty()) {
categoriesBuilder_.dispose();
categoriesBuilder_ = null;
categories_ = other.categories_;
bitField0_ = (bitField0_ & ~0x00000001);
categoriesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCategoriesFieldBuilder() : null;
} else {
categoriesBuilder_.addAllMessages(other.categories_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.cognite.client.dto.ThreeDNode.Properties parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.cognite.client.dto.ThreeDNode.Properties) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List categories_ =
java.util.Collections.emptyList();
private void ensureCategoriesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
categories_ = new java.util.ArrayList(categories_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.cognite.client.dto.ThreeDNode.Categories, com.cognite.client.dto.ThreeDNode.Categories.Builder, com.cognite.client.dto.ThreeDNode.CategoriesOrBuilder> categoriesBuilder_;
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public java.util.List getCategoriesList() {
if (categoriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(categories_);
} else {
return categoriesBuilder_.getMessageList();
}
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public int getCategoriesCount() {
if (categoriesBuilder_ == null) {
return categories_.size();
} else {
return categoriesBuilder_.getCount();
}
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public com.cognite.client.dto.ThreeDNode.Categories getCategories(int index) {
if (categoriesBuilder_ == null) {
return categories_.get(index);
} else {
return categoriesBuilder_.getMessage(index);
}
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public Builder setCategories(
int index, com.cognite.client.dto.ThreeDNode.Categories value) {
if (categoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCategoriesIsMutable();
categories_.set(index, value);
onChanged();
} else {
categoriesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public Builder setCategories(
int index, com.cognite.client.dto.ThreeDNode.Categories.Builder builderForValue) {
if (categoriesBuilder_ == null) {
ensureCategoriesIsMutable();
categories_.set(index, builderForValue.build());
onChanged();
} else {
categoriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public Builder addCategories(com.cognite.client.dto.ThreeDNode.Categories value) {
if (categoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCategoriesIsMutable();
categories_.add(value);
onChanged();
} else {
categoriesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public Builder addCategories(
int index, com.cognite.client.dto.ThreeDNode.Categories value) {
if (categoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCategoriesIsMutable();
categories_.add(index, value);
onChanged();
} else {
categoriesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public Builder addCategories(
com.cognite.client.dto.ThreeDNode.Categories.Builder builderForValue) {
if (categoriesBuilder_ == null) {
ensureCategoriesIsMutable();
categories_.add(builderForValue.build());
onChanged();
} else {
categoriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public Builder addCategories(
int index, com.cognite.client.dto.ThreeDNode.Categories.Builder builderForValue) {
if (categoriesBuilder_ == null) {
ensureCategoriesIsMutable();
categories_.add(index, builderForValue.build());
onChanged();
} else {
categoriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public Builder addAllCategories(
java.lang.Iterable extends com.cognite.client.dto.ThreeDNode.Categories> values) {
if (categoriesBuilder_ == null) {
ensureCategoriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, categories_);
onChanged();
} else {
categoriesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public Builder clearCategories() {
if (categoriesBuilder_ == null) {
categories_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
categoriesBuilder_.clear();
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public Builder removeCategories(int index) {
if (categoriesBuilder_ == null) {
ensureCategoriesIsMutable();
categories_.remove(index);
onChanged();
} else {
categoriesBuilder_.remove(index);
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public com.cognite.client.dto.ThreeDNode.Categories.Builder getCategoriesBuilder(
int index) {
return getCategoriesFieldBuilder().getBuilder(index);
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public com.cognite.client.dto.ThreeDNode.CategoriesOrBuilder getCategoriesOrBuilder(
int index) {
if (categoriesBuilder_ == null) {
return categories_.get(index); } else {
return categoriesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public java.util.List extends com.cognite.client.dto.ThreeDNode.CategoriesOrBuilder>
getCategoriesOrBuilderList() {
if (categoriesBuilder_ != null) {
return categoriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(categories_);
}
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public com.cognite.client.dto.ThreeDNode.Categories.Builder addCategoriesBuilder() {
return getCategoriesFieldBuilder().addBuilder(
com.cognite.client.dto.ThreeDNode.Categories.getDefaultInstance());
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public com.cognite.client.dto.ThreeDNode.Categories.Builder addCategoriesBuilder(
int index) {
return getCategoriesFieldBuilder().addBuilder(
index, com.cognite.client.dto.ThreeDNode.Categories.getDefaultInstance());
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.Categories categories = 1;
*/
public java.util.List
getCategoriesBuilderList() {
return getCategoriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.cognite.client.dto.ThreeDNode.Categories, com.cognite.client.dto.ThreeDNode.Categories.Builder, com.cognite.client.dto.ThreeDNode.CategoriesOrBuilder>
getCategoriesFieldBuilder() {
if (categoriesBuilder_ == null) {
categoriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.cognite.client.dto.ThreeDNode.Categories, com.cognite.client.dto.ThreeDNode.Categories.Builder, com.cognite.client.dto.ThreeDNode.CategoriesOrBuilder>(
categories_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
categories_ = null;
}
return categoriesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.cognite.beam.proto.ThreeDNode.Properties)
}
// @@protoc_insertion_point(class_scope:com.cognite.beam.proto.ThreeDNode.Properties)
private static final com.cognite.client.dto.ThreeDNode.Properties DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.cognite.client.dto.ThreeDNode.Properties();
}
public static com.cognite.client.dto.ThreeDNode.Properties getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Properties parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Properties(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.Properties getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CategoriesOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.cognite.beam.proto.ThreeDNode.Categories)
com.google.protobuf.MessageOrBuilder {
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* optional string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* optional string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* map<string, string> values = 2;
*/
int getValuesCount();
/**
* map<string, string> values = 2;
*/
boolean containsValues(
java.lang.String key);
/**
* Use {@link #getValuesMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getValues();
/**
* map<string, string> values = 2;
*/
java.util.Map
getValuesMap();
/**
* map<string, string> values = 2;
*/
java.lang.String getValuesOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
* map<string, string> values = 2;
*/
java.lang.String getValuesOrThrow(
java.lang.String key);
}
/**
* Protobuf type {@code com.cognite.beam.proto.ThreeDNode.Categories}
*/
public static final class Categories extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.cognite.beam.proto.ThreeDNode.Categories)
CategoriesOrBuilder {
private static final long serialVersionUID = 0L;
// Use Categories.newBuilder() to construct.
private Categories(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Categories() {
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Categories();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Categories(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
name_ = s;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
values_ = com.google.protobuf.MapField.newMapField(
ValuesDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000002;
}
com.google.protobuf.MapEntry
values__ = input.readMessage(
ValuesDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
values_.getMutableMap().put(
values__.getKey(), values__.getValue());
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_Categories_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetValues();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_Categories_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.cognite.client.dto.ThreeDNode.Categories.class, com.cognite.client.dto.ThreeDNode.Categories.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* optional string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUES_FIELD_NUMBER = 2;
private static final class ValuesDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_Categories_ValuesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> values_;
private com.google.protobuf.MapField
internalGetValues() {
if (values_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ValuesDefaultEntryHolder.defaultEntry);
}
return values_;
}
public int getValuesCount() {
return internalGetValues().getMap().size();
}
/**
* map<string, string> values = 2;
*/
@java.lang.Override
public boolean containsValues(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetValues().getMap().containsKey(key);
}
/**
* Use {@link #getValuesMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getValues() {
return getValuesMap();
}
/**
* map<string, string> values = 2;
*/
@java.lang.Override
public java.util.Map getValuesMap() {
return internalGetValues().getMap();
}
/**
* map<string, string> values = 2;
*/
@java.lang.Override
public java.lang.String getValuesOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetValues().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> values = 2;
*/
@java.lang.Override
public java.lang.String getValuesOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetValues().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetValues(),
ValuesDefaultEntryHolder.defaultEntry,
2);
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
for (java.util.Map.Entry entry
: internalGetValues().getMap().entrySet()) {
com.google.protobuf.MapEntry
values__ = ValuesDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, values__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.cognite.client.dto.ThreeDNode.Categories)) {
return super.equals(obj);
}
com.cognite.client.dto.ThreeDNode.Categories other = (com.cognite.client.dto.ThreeDNode.Categories) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (!internalGetValues().equals(
other.internalGetValues())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (!internalGetValues().getMap().isEmpty()) {
hash = (37 * hash) + VALUES_FIELD_NUMBER;
hash = (53 * hash) + internalGetValues().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.cognite.client.dto.ThreeDNode.Categories parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.Categories parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.Categories parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.Categories parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.Categories parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.Categories parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.Categories parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.Categories parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.Categories parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.Categories parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.Categories parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.Categories parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.cognite.client.dto.ThreeDNode.Categories prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.cognite.beam.proto.ThreeDNode.Categories}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.cognite.beam.proto.ThreeDNode.Categories)
com.cognite.client.dto.ThreeDNode.CategoriesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_Categories_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetValues();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 2:
return internalGetMutableValues();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_Categories_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.cognite.client.dto.ThreeDNode.Categories.class, com.cognite.client.dto.ThreeDNode.Categories.Builder.class);
}
// Construct using com.cognite.client.dto.ThreeDNode.Categories.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
internalGetMutableValues().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_Categories_descriptor;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.Categories getDefaultInstanceForType() {
return com.cognite.client.dto.ThreeDNode.Categories.getDefaultInstance();
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.Categories build() {
com.cognite.client.dto.ThreeDNode.Categories result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.Categories buildPartial() {
com.cognite.client.dto.ThreeDNode.Categories result = new com.cognite.client.dto.ThreeDNode.Categories(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
result.values_ = internalGetValues();
result.values_.makeImmutable();
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.cognite.client.dto.ThreeDNode.Categories) {
return mergeFrom((com.cognite.client.dto.ThreeDNode.Categories)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.cognite.client.dto.ThreeDNode.Categories other) {
if (other == com.cognite.client.dto.ThreeDNode.Categories.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
internalGetMutableValues().mergeFrom(
other.internalGetValues());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.cognite.client.dto.ThreeDNode.Categories parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.cognite.client.dto.ThreeDNode.Categories) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> values_;
private com.google.protobuf.MapField
internalGetValues() {
if (values_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ValuesDefaultEntryHolder.defaultEntry);
}
return values_;
}
private com.google.protobuf.MapField
internalGetMutableValues() {
onChanged();;
if (values_ == null) {
values_ = com.google.protobuf.MapField.newMapField(
ValuesDefaultEntryHolder.defaultEntry);
}
if (!values_.isMutable()) {
values_ = values_.copy();
}
return values_;
}
public int getValuesCount() {
return internalGetValues().getMap().size();
}
/**
* map<string, string> values = 2;
*/
@java.lang.Override
public boolean containsValues(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetValues().getMap().containsKey(key);
}
/**
* Use {@link #getValuesMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getValues() {
return getValuesMap();
}
/**
* map<string, string> values = 2;
*/
@java.lang.Override
public java.util.Map getValuesMap() {
return internalGetValues().getMap();
}
/**
* map<string, string> values = 2;
*/
@java.lang.Override
public java.lang.String getValuesOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetValues().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> values = 2;
*/
@java.lang.Override
public java.lang.String getValuesOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetValues().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearValues() {
internalGetMutableValues().getMutableMap()
.clear();
return this;
}
/**
* map<string, string> values = 2;
*/
public Builder removeValues(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableValues().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableValues() {
return internalGetMutableValues().getMutableMap();
}
/**
* map<string, string> values = 2;
*/
public Builder putValues(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableValues().getMutableMap()
.put(key, value);
return this;
}
/**
* map<string, string> values = 2;
*/
public Builder putAllValues(
java.util.Map values) {
internalGetMutableValues().getMutableMap()
.putAll(values);
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.cognite.beam.proto.ThreeDNode.Categories)
}
// @@protoc_insertion_point(class_scope:com.cognite.beam.proto.ThreeDNode.Categories)
private static final com.cognite.client.dto.ThreeDNode.Categories DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.cognite.client.dto.ThreeDNode.Categories();
}
public static com.cognite.client.dto.ThreeDNode.Categories getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Categories parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Categories(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.Categories getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PropertiesFilterOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.cognite.beam.proto.ThreeDNode.PropertiesFilter)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
java.util.List
getCategoriesList();
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories getCategories(int index);
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
int getCategoriesCount();
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
java.util.List extends com.cognite.client.dto.ThreeDNode.PropertiesFilter.CategoriesOrBuilder>
getCategoriesOrBuilderList();
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
com.cognite.client.dto.ThreeDNode.PropertiesFilter.CategoriesOrBuilder getCategoriesOrBuilder(
int index);
}
/**
* Protobuf type {@code com.cognite.beam.proto.ThreeDNode.PropertiesFilter}
*/
public static final class PropertiesFilter extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.cognite.beam.proto.ThreeDNode.PropertiesFilter)
PropertiesFilterOrBuilder {
private static final long serialVersionUID = 0L;
// Use PropertiesFilter.newBuilder() to construct.
private PropertiesFilter(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PropertiesFilter() {
categories_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PropertiesFilter();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PropertiesFilter(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
categories_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
categories_.add(
input.readMessage(com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
categories_ = java.util.Collections.unmodifiableList(categories_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.cognite.client.dto.ThreeDNode.PropertiesFilter.class, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Builder.class);
}
public interface CategoriesOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories)
com.google.protobuf.MessageOrBuilder {
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* optional string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* optional string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
int getValuesCount();
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
boolean containsValues(
java.lang.String key);
/**
* Use {@link #getValuesMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getValues();
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
java.util.Map
getValuesMap();
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues getValuesOrDefault(
java.lang.String key,
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues defaultValue);
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues getValuesOrThrow(
java.lang.String key);
}
/**
* Protobuf type {@code com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories}
*/
public static final class Categories extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories)
CategoriesOrBuilder {
private static final long serialVersionUID = 0L;
// Use Categories.newBuilder() to construct.
private Categories(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Categories() {
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Categories();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Categories(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
name_ = s;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
values_ = com.google.protobuf.MapField.newMapField(
ValuesDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000002;
}
com.google.protobuf.MapEntry
values__ = input.readMessage(
ValuesDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
values_.getMutableMap().put(
values__.getKey(), values__.getValue());
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_Categories_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetValues();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_Categories_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.class, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.Builder.class);
}
public interface CategoriesValuesOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string valuesString = 1;
* @return A list containing the valuesString.
*/
java.util.List
getValuesStringList();
/**
* repeated string valuesString = 1;
* @return The count of valuesString.
*/
int getValuesStringCount();
/**
* repeated string valuesString = 1;
* @param index The index of the element to return.
* @return The valuesString at the given index.
*/
java.lang.String getValuesString(int index);
/**
* repeated string valuesString = 1;
* @param index The index of the value to return.
* @return The bytes of the valuesString at the given index.
*/
com.google.protobuf.ByteString
getValuesStringBytes(int index);
}
/**
* Protobuf type {@code com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues}
*/
public static final class CategoriesValues extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues)
CategoriesValuesOrBuilder {
private static final long serialVersionUID = 0L;
// Use CategoriesValues.newBuilder() to construct.
private CategoriesValues(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CategoriesValues() {
valuesString_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CategoriesValues();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CategoriesValues(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
valuesString_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
valuesString_.add(s);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
valuesString_ = valuesString_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_Categories_CategoriesValues_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_Categories_CategoriesValues_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues.class, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues.Builder.class);
}
public static final int VALUESSTRING_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList valuesString_;
/**
* repeated string valuesString = 1;
* @return A list containing the valuesString.
*/
public com.google.protobuf.ProtocolStringList
getValuesStringList() {
return valuesString_;
}
/**
* repeated string valuesString = 1;
* @return The count of valuesString.
*/
public int getValuesStringCount() {
return valuesString_.size();
}
/**
* repeated string valuesString = 1;
* @param index The index of the element to return.
* @return The valuesString at the given index.
*/
public java.lang.String getValuesString(int index) {
return valuesString_.get(index);
}
/**
* repeated string valuesString = 1;
* @param index The index of the value to return.
* @return The bytes of the valuesString at the given index.
*/
public com.google.protobuf.ByteString
getValuesStringBytes(int index) {
return valuesString_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < valuesString_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, valuesString_.getRaw(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < valuesString_.size(); i++) {
dataSize += computeStringSizeNoTag(valuesString_.getRaw(i));
}
size += dataSize;
size += 1 * getValuesStringList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues)) {
return super.equals(obj);
}
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues other = (com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues) obj;
if (!getValuesStringList()
.equals(other.getValuesStringList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getValuesStringCount() > 0) {
hash = (37 * hash) + VALUESSTRING_FIELD_NUMBER;
hash = (53 * hash) + getValuesStringList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues)
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValuesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_Categories_CategoriesValues_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_Categories_CategoriesValues_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues.class, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues.Builder.class);
}
// Construct using com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
valuesString_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_Categories_CategoriesValues_descriptor;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues getDefaultInstanceForType() {
return com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues.getDefaultInstance();
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues build() {
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues buildPartial() {
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues result = new com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
valuesString_ = valuesString_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.valuesString_ = valuesString_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues) {
return mergeFrom((com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues other) {
if (other == com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues.getDefaultInstance()) return this;
if (!other.valuesString_.isEmpty()) {
if (valuesString_.isEmpty()) {
valuesString_ = other.valuesString_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureValuesStringIsMutable();
valuesString_.addAll(other.valuesString_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList valuesString_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureValuesStringIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
valuesString_ = new com.google.protobuf.LazyStringArrayList(valuesString_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string valuesString = 1;
* @return A list containing the valuesString.
*/
public com.google.protobuf.ProtocolStringList
getValuesStringList() {
return valuesString_.getUnmodifiableView();
}
/**
* repeated string valuesString = 1;
* @return The count of valuesString.
*/
public int getValuesStringCount() {
return valuesString_.size();
}
/**
* repeated string valuesString = 1;
* @param index The index of the element to return.
* @return The valuesString at the given index.
*/
public java.lang.String getValuesString(int index) {
return valuesString_.get(index);
}
/**
* repeated string valuesString = 1;
* @param index The index of the value to return.
* @return The bytes of the valuesString at the given index.
*/
public com.google.protobuf.ByteString
getValuesStringBytes(int index) {
return valuesString_.getByteString(index);
}
/**
* repeated string valuesString = 1;
* @param index The index to set the value at.
* @param value The valuesString to set.
* @return This builder for chaining.
*/
public Builder setValuesString(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesStringIsMutable();
valuesString_.set(index, value);
onChanged();
return this;
}
/**
* repeated string valuesString = 1;
* @param value The valuesString to add.
* @return This builder for chaining.
*/
public Builder addValuesString(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesStringIsMutable();
valuesString_.add(value);
onChanged();
return this;
}
/**
* repeated string valuesString = 1;
* @param values The valuesString to add.
* @return This builder for chaining.
*/
public Builder addAllValuesString(
java.lang.Iterable values) {
ensureValuesStringIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, valuesString_);
onChanged();
return this;
}
/**
* repeated string valuesString = 1;
* @return This builder for chaining.
*/
public Builder clearValuesString() {
valuesString_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string valuesString = 1;
* @param value The bytes of the valuesString to add.
* @return This builder for chaining.
*/
public Builder addValuesStringBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureValuesStringIsMutable();
valuesString_.add(value);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues)
}
// @@protoc_insertion_point(class_scope:com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues)
private static final com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues();
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CategoriesValues parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CategoriesValues(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* optional string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUES_FIELD_NUMBER = 2;
private static final class ValuesDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_Categories_ValuesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values_;
private com.google.protobuf.MapField
internalGetValues() {
if (values_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ValuesDefaultEntryHolder.defaultEntry);
}
return values_;
}
public int getValuesCount() {
return internalGetValues().getMap().size();
}
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
@java.lang.Override
public boolean containsValues(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetValues().getMap().containsKey(key);
}
/**
* Use {@link #getValuesMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getValues() {
return getValuesMap();
}
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
@java.lang.Override
public java.util.Map getValuesMap() {
return internalGetValues().getMap();
}
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues getValuesOrDefault(
java.lang.String key,
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetValues().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues getValuesOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetValues().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetValues(),
ValuesDefaultEntryHolder.defaultEntry,
2);
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
for (java.util.Map.Entry entry
: internalGetValues().getMap().entrySet()) {
com.google.protobuf.MapEntry
values__ = ValuesDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, values__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories)) {
return super.equals(obj);
}
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories other = (com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (!internalGetValues().equals(
other.internalGetValues())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (!internalGetValues().getMap().isEmpty()) {
hash = (37 * hash) + VALUES_FIELD_NUMBER;
hash = (53 * hash) + internalGetValues().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories)
com.cognite.client.dto.ThreeDNode.PropertiesFilter.CategoriesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_Categories_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetValues();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 2:
return internalGetMutableValues();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_Categories_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.class, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.Builder.class);
}
// Construct using com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
internalGetMutableValues().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_Categories_descriptor;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories getDefaultInstanceForType() {
return com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.getDefaultInstance();
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories build() {
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories buildPartial() {
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories result = new com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
result.values_ = internalGetValues();
result.values_.makeImmutable();
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories) {
return mergeFrom((com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories other) {
if (other == com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
internalGetMutableValues().mergeFrom(
other.internalGetValues());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values_;
private com.google.protobuf.MapField
internalGetValues() {
if (values_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ValuesDefaultEntryHolder.defaultEntry);
}
return values_;
}
private com.google.protobuf.MapField
internalGetMutableValues() {
onChanged();;
if (values_ == null) {
values_ = com.google.protobuf.MapField.newMapField(
ValuesDefaultEntryHolder.defaultEntry);
}
if (!values_.isMutable()) {
values_ = values_.copy();
}
return values_;
}
public int getValuesCount() {
return internalGetValues().getMap().size();
}
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
@java.lang.Override
public boolean containsValues(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetValues().getMap().containsKey(key);
}
/**
* Use {@link #getValuesMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getValues() {
return getValuesMap();
}
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
@java.lang.Override
public java.util.Map getValuesMap() {
return internalGetValues().getMap();
}
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues getValuesOrDefault(
java.lang.String key,
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetValues().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues getValuesOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetValues().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearValues() {
internalGetMutableValues().getMutableMap()
.clear();
return this;
}
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
public Builder removeValues(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableValues().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableValues() {
return internalGetMutableValues().getMutableMap();
}
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
public Builder putValues(
java.lang.String key,
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableValues().getMutableMap()
.put(key, value);
return this;
}
/**
* map<string, .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories.CategoriesValues> values = 2;
*/
public Builder putAllValues(
java.util.Map values) {
internalGetMutableValues().getMutableMap()
.putAll(values);
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories)
}
// @@protoc_insertion_point(class_scope:com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories)
private static final com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories();
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Categories parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Categories(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int CATEGORIES_FIELD_NUMBER = 1;
private java.util.List categories_;
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
@java.lang.Override
public java.util.List getCategoriesList() {
return categories_;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
@java.lang.Override
public java.util.List extends com.cognite.client.dto.ThreeDNode.PropertiesFilter.CategoriesOrBuilder>
getCategoriesOrBuilderList() {
return categories_;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
@java.lang.Override
public int getCategoriesCount() {
return categories_.size();
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories getCategories(int index) {
return categories_.get(index);
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.CategoriesOrBuilder getCategoriesOrBuilder(
int index) {
return categories_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < categories_.size(); i++) {
output.writeMessage(1, categories_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < categories_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, categories_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.cognite.client.dto.ThreeDNode.PropertiesFilter)) {
return super.equals(obj);
}
com.cognite.client.dto.ThreeDNode.PropertiesFilter other = (com.cognite.client.dto.ThreeDNode.PropertiesFilter) obj;
if (!getCategoriesList()
.equals(other.getCategoriesList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getCategoriesCount() > 0) {
hash = (37 * hash) + CATEGORIES_FIELD_NUMBER;
hash = (53 * hash) + getCategoriesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.cognite.client.dto.ThreeDNode.PropertiesFilter prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.cognite.beam.proto.ThreeDNode.PropertiesFilter}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.cognite.beam.proto.ThreeDNode.PropertiesFilter)
com.cognite.client.dto.ThreeDNode.PropertiesFilterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.cognite.client.dto.ThreeDNode.PropertiesFilter.class, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Builder.class);
}
// Construct using com.cognite.client.dto.ThreeDNode.PropertiesFilter.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCategoriesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (categoriesBuilder_ == null) {
categories_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
categoriesBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_PropertiesFilter_descriptor;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter getDefaultInstanceForType() {
return com.cognite.client.dto.ThreeDNode.PropertiesFilter.getDefaultInstance();
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter build() {
com.cognite.client.dto.ThreeDNode.PropertiesFilter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter buildPartial() {
com.cognite.client.dto.ThreeDNode.PropertiesFilter result = new com.cognite.client.dto.ThreeDNode.PropertiesFilter(this);
int from_bitField0_ = bitField0_;
if (categoriesBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
categories_ = java.util.Collections.unmodifiableList(categories_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.categories_ = categories_;
} else {
result.categories_ = categoriesBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.cognite.client.dto.ThreeDNode.PropertiesFilter) {
return mergeFrom((com.cognite.client.dto.ThreeDNode.PropertiesFilter)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.cognite.client.dto.ThreeDNode.PropertiesFilter other) {
if (other == com.cognite.client.dto.ThreeDNode.PropertiesFilter.getDefaultInstance()) return this;
if (categoriesBuilder_ == null) {
if (!other.categories_.isEmpty()) {
if (categories_.isEmpty()) {
categories_ = other.categories_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureCategoriesIsMutable();
categories_.addAll(other.categories_);
}
onChanged();
}
} else {
if (!other.categories_.isEmpty()) {
if (categoriesBuilder_.isEmpty()) {
categoriesBuilder_.dispose();
categoriesBuilder_ = null;
categories_ = other.categories_;
bitField0_ = (bitField0_ & ~0x00000001);
categoriesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCategoriesFieldBuilder() : null;
} else {
categoriesBuilder_.addAllMessages(other.categories_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.cognite.client.dto.ThreeDNode.PropertiesFilter parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.cognite.client.dto.ThreeDNode.PropertiesFilter) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List categories_ =
java.util.Collections.emptyList();
private void ensureCategoriesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
categories_ = new java.util.ArrayList(categories_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.Builder, com.cognite.client.dto.ThreeDNode.PropertiesFilter.CategoriesOrBuilder> categoriesBuilder_;
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public java.util.List getCategoriesList() {
if (categoriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(categories_);
} else {
return categoriesBuilder_.getMessageList();
}
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public int getCategoriesCount() {
if (categoriesBuilder_ == null) {
return categories_.size();
} else {
return categoriesBuilder_.getCount();
}
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories getCategories(int index) {
if (categoriesBuilder_ == null) {
return categories_.get(index);
} else {
return categoriesBuilder_.getMessage(index);
}
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public Builder setCategories(
int index, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories value) {
if (categoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCategoriesIsMutable();
categories_.set(index, value);
onChanged();
} else {
categoriesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public Builder setCategories(
int index, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.Builder builderForValue) {
if (categoriesBuilder_ == null) {
ensureCategoriesIsMutable();
categories_.set(index, builderForValue.build());
onChanged();
} else {
categoriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public Builder addCategories(com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories value) {
if (categoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCategoriesIsMutable();
categories_.add(value);
onChanged();
} else {
categoriesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public Builder addCategories(
int index, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories value) {
if (categoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCategoriesIsMutable();
categories_.add(index, value);
onChanged();
} else {
categoriesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public Builder addCategories(
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.Builder builderForValue) {
if (categoriesBuilder_ == null) {
ensureCategoriesIsMutable();
categories_.add(builderForValue.build());
onChanged();
} else {
categoriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public Builder addCategories(
int index, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.Builder builderForValue) {
if (categoriesBuilder_ == null) {
ensureCategoriesIsMutable();
categories_.add(index, builderForValue.build());
onChanged();
} else {
categoriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public Builder addAllCategories(
java.lang.Iterable extends com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories> values) {
if (categoriesBuilder_ == null) {
ensureCategoriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, categories_);
onChanged();
} else {
categoriesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public Builder clearCategories() {
if (categoriesBuilder_ == null) {
categories_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
categoriesBuilder_.clear();
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public Builder removeCategories(int index) {
if (categoriesBuilder_ == null) {
ensureCategoriesIsMutable();
categories_.remove(index);
onChanged();
} else {
categoriesBuilder_.remove(index);
}
return this;
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.Builder getCategoriesBuilder(
int index) {
return getCategoriesFieldBuilder().getBuilder(index);
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.CategoriesOrBuilder getCategoriesOrBuilder(
int index) {
if (categoriesBuilder_ == null) {
return categories_.get(index); } else {
return categoriesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public java.util.List extends com.cognite.client.dto.ThreeDNode.PropertiesFilter.CategoriesOrBuilder>
getCategoriesOrBuilderList() {
if (categoriesBuilder_ != null) {
return categoriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(categories_);
}
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.Builder addCategoriesBuilder() {
return getCategoriesFieldBuilder().addBuilder(
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.getDefaultInstance());
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.Builder addCategoriesBuilder(
int index) {
return getCategoriesFieldBuilder().addBuilder(
index, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.getDefaultInstance());
}
/**
* repeated .com.cognite.beam.proto.ThreeDNode.PropertiesFilter.Categories categories = 1;
*/
public java.util.List
getCategoriesBuilderList() {
return getCategoriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.Builder, com.cognite.client.dto.ThreeDNode.PropertiesFilter.CategoriesOrBuilder>
getCategoriesFieldBuilder() {
if (categoriesBuilder_ == null) {
categoriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories, com.cognite.client.dto.ThreeDNode.PropertiesFilter.Categories.Builder, com.cognite.client.dto.ThreeDNode.PropertiesFilter.CategoriesOrBuilder>(
categories_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
categories_ = null;
}
return categoriesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.cognite.beam.proto.ThreeDNode.PropertiesFilter)
}
// @@protoc_insertion_point(class_scope:com.cognite.beam.proto.ThreeDNode.PropertiesFilter)
private static final com.cognite.client.dto.ThreeDNode.PropertiesFilter DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.cognite.client.dto.ThreeDNode.PropertiesFilter();
}
public static com.cognite.client.dto.ThreeDNode.PropertiesFilter getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PropertiesFilter parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PropertiesFilter(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesFilter getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private long id_;
/**
* optional int64 id = 1;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int64 id = 1;
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
public static final int TREEINDEX_FIELD_NUMBER = 2;
private long treeIndex_;
/**
* optional int64 treeIndex = 2;
* @return Whether the treeIndex field is set.
*/
@java.lang.Override
public boolean hasTreeIndex() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int64 treeIndex = 2;
* @return The treeIndex.
*/
@java.lang.Override
public long getTreeIndex() {
return treeIndex_;
}
public static final int PARENTID_FIELD_NUMBER = 3;
private long parentId_;
/**
* optional int64 parentId = 3;
* @return Whether the parentId field is set.
*/
@java.lang.Override
public boolean hasParentId() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional int64 parentId = 3;
* @return The parentId.
*/
@java.lang.Override
public long getParentId() {
return parentId_;
}
public static final int DEPTH_FIELD_NUMBER = 4;
private long depth_;
/**
* optional int64 depth = 4;
* @return Whether the depth field is set.
*/
@java.lang.Override
public boolean hasDepth() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional int64 depth = 4;
* @return The depth.
*/
@java.lang.Override
public long getDepth() {
return depth_;
}
public static final int NAME_FIELD_NUMBER = 5;
private volatile java.lang.Object name_;
/**
* optional string name = 5;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string name = 5;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* optional string name = 5;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBTREESIZE_FIELD_NUMBER = 6;
private long subtreeSize_;
/**
* optional int64 subtreeSize = 6;
* @return Whether the subtreeSize field is set.
*/
@java.lang.Override
public boolean hasSubtreeSize() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional int64 subtreeSize = 6;
* @return The subtreeSize.
*/
@java.lang.Override
public long getSubtreeSize() {
return subtreeSize_;
}
public static final int PROPERTIES_FIELD_NUMBER = 7;
private com.cognite.client.dto.ThreeDNode.Properties properties_;
/**
* optional .com.cognite.beam.proto.ThreeDNode.Properties properties = 7;
* @return Whether the properties field is set.
*/
@java.lang.Override
public boolean hasProperties() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.Properties properties = 7;
* @return The properties.
*/
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.Properties getProperties() {
return properties_ == null ? com.cognite.client.dto.ThreeDNode.Properties.getDefaultInstance() : properties_;
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.Properties properties = 7;
*/
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.PropertiesOrBuilder getPropertiesOrBuilder() {
return properties_ == null ? com.cognite.client.dto.ThreeDNode.Properties.getDefaultInstance() : properties_;
}
public static final int BOUNDINGBOX_FIELD_NUMBER = 8;
private com.cognite.client.dto.ThreeDNode.BoundingBox boundingBox_;
/**
* optional .com.cognite.beam.proto.ThreeDNode.BoundingBox boundingBox = 8;
* @return Whether the boundingBox field is set.
*/
@java.lang.Override
public boolean hasBoundingBox() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.BoundingBox boundingBox = 8;
* @return The boundingBox.
*/
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.BoundingBox getBoundingBox() {
return boundingBox_ == null ? com.cognite.client.dto.ThreeDNode.BoundingBox.getDefaultInstance() : boundingBox_;
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.BoundingBox boundingBox = 8;
*/
@java.lang.Override
public com.cognite.client.dto.ThreeDNode.BoundingBoxOrBuilder getBoundingBoxOrBuilder() {
return boundingBox_ == null ? com.cognite.client.dto.ThreeDNode.BoundingBox.getDefaultInstance() : boundingBox_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(1, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(2, treeIndex_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt64(3, parentId_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt64(4, depth_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, name_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeInt64(6, subtreeSize_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(7, getProperties());
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeMessage(8, getBoundingBox());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, treeIndex_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, parentId_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, depth_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, name_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(6, subtreeSize_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getProperties());
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getBoundingBox());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.cognite.client.dto.ThreeDNode)) {
return super.equals(obj);
}
com.cognite.client.dto.ThreeDNode other = (com.cognite.client.dto.ThreeDNode) obj;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId()
!= other.getId()) return false;
}
if (hasTreeIndex() != other.hasTreeIndex()) return false;
if (hasTreeIndex()) {
if (getTreeIndex()
!= other.getTreeIndex()) return false;
}
if (hasParentId() != other.hasParentId()) return false;
if (hasParentId()) {
if (getParentId()
!= other.getParentId()) return false;
}
if (hasDepth() != other.hasDepth()) return false;
if (hasDepth()) {
if (getDepth()
!= other.getDepth()) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasSubtreeSize() != other.hasSubtreeSize()) return false;
if (hasSubtreeSize()) {
if (getSubtreeSize()
!= other.getSubtreeSize()) return false;
}
if (hasProperties() != other.hasProperties()) return false;
if (hasProperties()) {
if (!getProperties()
.equals(other.getProperties())) return false;
}
if (hasBoundingBox() != other.hasBoundingBox()) return false;
if (hasBoundingBox()) {
if (!getBoundingBox()
.equals(other.getBoundingBox())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getId());
}
if (hasTreeIndex()) {
hash = (37 * hash) + TREEINDEX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTreeIndex());
}
if (hasParentId()) {
hash = (37 * hash) + PARENTID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getParentId());
}
if (hasDepth()) {
hash = (37 * hash) + DEPTH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getDepth());
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasSubtreeSize()) {
hash = (37 * hash) + SUBTREESIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSubtreeSize());
}
if (hasProperties()) {
hash = (37 * hash) + PROPERTIES_FIELD_NUMBER;
hash = (53 * hash) + getProperties().hashCode();
}
if (hasBoundingBox()) {
hash = (37 * hash) + BOUNDINGBOX_FIELD_NUMBER;
hash = (53 * hash) + getBoundingBox().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.cognite.client.dto.ThreeDNode parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.cognite.client.dto.ThreeDNode parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.cognite.client.dto.ThreeDNode parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.cognite.client.dto.ThreeDNode parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.cognite.client.dto.ThreeDNode prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.cognite.beam.proto.ThreeDNode}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.cognite.beam.proto.ThreeDNode)
com.cognite.client.dto.ThreeDNodeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.cognite.client.dto.ThreeDNode.class, com.cognite.client.dto.ThreeDNode.Builder.class);
}
// Construct using com.cognite.client.dto.ThreeDNode.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getPropertiesFieldBuilder();
getBoundingBoxFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
treeIndex_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
parentId_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
depth_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
subtreeSize_ = 0L;
bitField0_ = (bitField0_ & ~0x00000020);
if (propertiesBuilder_ == null) {
properties_ = null;
} else {
propertiesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
if (boundingBoxBuilder_ == null) {
boundingBox_ = null;
} else {
boundingBoxBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.cognite.client.dto.ThreeDModelOuterClass.internal_static_com_cognite_beam_proto_ThreeDNode_descriptor;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode getDefaultInstanceForType() {
return com.cognite.client.dto.ThreeDNode.getDefaultInstance();
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode build() {
com.cognite.client.dto.ThreeDNode result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode buildPartial() {
com.cognite.client.dto.ThreeDNode result = new com.cognite.client.dto.ThreeDNode(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.treeIndex_ = treeIndex_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.parentId_ = parentId_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.depth_ = depth_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000020) != 0)) {
result.subtreeSize_ = subtreeSize_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
if (propertiesBuilder_ == null) {
result.properties_ = properties_;
} else {
result.properties_ = propertiesBuilder_.build();
}
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
if (boundingBoxBuilder_ == null) {
result.boundingBox_ = boundingBox_;
} else {
result.boundingBox_ = boundingBoxBuilder_.build();
}
to_bitField0_ |= 0x00000080;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.cognite.client.dto.ThreeDNode) {
return mergeFrom((com.cognite.client.dto.ThreeDNode)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.cognite.client.dto.ThreeDNode other) {
if (other == com.cognite.client.dto.ThreeDNode.getDefaultInstance()) return this;
if (other.hasId()) {
setId(other.getId());
}
if (other.hasTreeIndex()) {
setTreeIndex(other.getTreeIndex());
}
if (other.hasParentId()) {
setParentId(other.getParentId());
}
if (other.hasDepth()) {
setDepth(other.getDepth());
}
if (other.hasName()) {
bitField0_ |= 0x00000010;
name_ = other.name_;
onChanged();
}
if (other.hasSubtreeSize()) {
setSubtreeSize(other.getSubtreeSize());
}
if (other.hasProperties()) {
mergeProperties(other.getProperties());
}
if (other.hasBoundingBox()) {
mergeBoundingBox(other.getBoundingBox());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.cognite.client.dto.ThreeDNode parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.cognite.client.dto.ThreeDNode) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long id_ ;
/**
* optional int64 id = 1;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int64 id = 1;
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
/**
* optional int64 id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(long value) {
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
* optional int64 id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0L;
onChanged();
return this;
}
private long treeIndex_ ;
/**
* optional int64 treeIndex = 2;
* @return Whether the treeIndex field is set.
*/
@java.lang.Override
public boolean hasTreeIndex() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int64 treeIndex = 2;
* @return The treeIndex.
*/
@java.lang.Override
public long getTreeIndex() {
return treeIndex_;
}
/**
* optional int64 treeIndex = 2;
* @param value The treeIndex to set.
* @return This builder for chaining.
*/
public Builder setTreeIndex(long value) {
bitField0_ |= 0x00000002;
treeIndex_ = value;
onChanged();
return this;
}
/**
* optional int64 treeIndex = 2;
* @return This builder for chaining.
*/
public Builder clearTreeIndex() {
bitField0_ = (bitField0_ & ~0x00000002);
treeIndex_ = 0L;
onChanged();
return this;
}
private long parentId_ ;
/**
* optional int64 parentId = 3;
* @return Whether the parentId field is set.
*/
@java.lang.Override
public boolean hasParentId() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional int64 parentId = 3;
* @return The parentId.
*/
@java.lang.Override
public long getParentId() {
return parentId_;
}
/**
* optional int64 parentId = 3;
* @param value The parentId to set.
* @return This builder for chaining.
*/
public Builder setParentId(long value) {
bitField0_ |= 0x00000004;
parentId_ = value;
onChanged();
return this;
}
/**
* optional int64 parentId = 3;
* @return This builder for chaining.
*/
public Builder clearParentId() {
bitField0_ = (bitField0_ & ~0x00000004);
parentId_ = 0L;
onChanged();
return this;
}
private long depth_ ;
/**
* optional int64 depth = 4;
* @return Whether the depth field is set.
*/
@java.lang.Override
public boolean hasDepth() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional int64 depth = 4;
* @return The depth.
*/
@java.lang.Override
public long getDepth() {
return depth_;
}
/**
* optional int64 depth = 4;
* @param value The depth to set.
* @return This builder for chaining.
*/
public Builder setDepth(long value) {
bitField0_ |= 0x00000008;
depth_ = value;
onChanged();
return this;
}
/**
* optional int64 depth = 4;
* @return This builder for chaining.
*/
public Builder clearDepth() {
bitField0_ = (bitField0_ & ~0x00000008);
depth_ = 0L;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* optional string name = 5;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string name = 5;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 5;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 5;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 5;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000010);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 5;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000010;
name_ = value;
onChanged();
return this;
}
private long subtreeSize_ ;
/**
* optional int64 subtreeSize = 6;
* @return Whether the subtreeSize field is set.
*/
@java.lang.Override
public boolean hasSubtreeSize() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional int64 subtreeSize = 6;
* @return The subtreeSize.
*/
@java.lang.Override
public long getSubtreeSize() {
return subtreeSize_;
}
/**
* optional int64 subtreeSize = 6;
* @param value The subtreeSize to set.
* @return This builder for chaining.
*/
public Builder setSubtreeSize(long value) {
bitField0_ |= 0x00000020;
subtreeSize_ = value;
onChanged();
return this;
}
/**
* optional int64 subtreeSize = 6;
* @return This builder for chaining.
*/
public Builder clearSubtreeSize() {
bitField0_ = (bitField0_ & ~0x00000020);
subtreeSize_ = 0L;
onChanged();
return this;
}
private com.cognite.client.dto.ThreeDNode.Properties properties_;
private com.google.protobuf.SingleFieldBuilderV3<
com.cognite.client.dto.ThreeDNode.Properties, com.cognite.client.dto.ThreeDNode.Properties.Builder, com.cognite.client.dto.ThreeDNode.PropertiesOrBuilder> propertiesBuilder_;
/**
* optional .com.cognite.beam.proto.ThreeDNode.Properties properties = 7;
* @return Whether the properties field is set.
*/
public boolean hasProperties() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.Properties properties = 7;
* @return The properties.
*/
public com.cognite.client.dto.ThreeDNode.Properties getProperties() {
if (propertiesBuilder_ == null) {
return properties_ == null ? com.cognite.client.dto.ThreeDNode.Properties.getDefaultInstance() : properties_;
} else {
return propertiesBuilder_.getMessage();
}
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.Properties properties = 7;
*/
public Builder setProperties(com.cognite.client.dto.ThreeDNode.Properties value) {
if (propertiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
properties_ = value;
onChanged();
} else {
propertiesBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.Properties properties = 7;
*/
public Builder setProperties(
com.cognite.client.dto.ThreeDNode.Properties.Builder builderForValue) {
if (propertiesBuilder_ == null) {
properties_ = builderForValue.build();
onChanged();
} else {
propertiesBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.Properties properties = 7;
*/
public Builder mergeProperties(com.cognite.client.dto.ThreeDNode.Properties value) {
if (propertiesBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0) &&
properties_ != null &&
properties_ != com.cognite.client.dto.ThreeDNode.Properties.getDefaultInstance()) {
properties_ =
com.cognite.client.dto.ThreeDNode.Properties.newBuilder(properties_).mergeFrom(value).buildPartial();
} else {
properties_ = value;
}
onChanged();
} else {
propertiesBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.Properties properties = 7;
*/
public Builder clearProperties() {
if (propertiesBuilder_ == null) {
properties_ = null;
onChanged();
} else {
propertiesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.Properties properties = 7;
*/
public com.cognite.client.dto.ThreeDNode.Properties.Builder getPropertiesBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getPropertiesFieldBuilder().getBuilder();
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.Properties properties = 7;
*/
public com.cognite.client.dto.ThreeDNode.PropertiesOrBuilder getPropertiesOrBuilder() {
if (propertiesBuilder_ != null) {
return propertiesBuilder_.getMessageOrBuilder();
} else {
return properties_ == null ?
com.cognite.client.dto.ThreeDNode.Properties.getDefaultInstance() : properties_;
}
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.Properties properties = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.cognite.client.dto.ThreeDNode.Properties, com.cognite.client.dto.ThreeDNode.Properties.Builder, com.cognite.client.dto.ThreeDNode.PropertiesOrBuilder>
getPropertiesFieldBuilder() {
if (propertiesBuilder_ == null) {
propertiesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.cognite.client.dto.ThreeDNode.Properties, com.cognite.client.dto.ThreeDNode.Properties.Builder, com.cognite.client.dto.ThreeDNode.PropertiesOrBuilder>(
getProperties(),
getParentForChildren(),
isClean());
properties_ = null;
}
return propertiesBuilder_;
}
private com.cognite.client.dto.ThreeDNode.BoundingBox boundingBox_;
private com.google.protobuf.SingleFieldBuilderV3<
com.cognite.client.dto.ThreeDNode.BoundingBox, com.cognite.client.dto.ThreeDNode.BoundingBox.Builder, com.cognite.client.dto.ThreeDNode.BoundingBoxOrBuilder> boundingBoxBuilder_;
/**
* optional .com.cognite.beam.proto.ThreeDNode.BoundingBox boundingBox = 8;
* @return Whether the boundingBox field is set.
*/
public boolean hasBoundingBox() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.BoundingBox boundingBox = 8;
* @return The boundingBox.
*/
public com.cognite.client.dto.ThreeDNode.BoundingBox getBoundingBox() {
if (boundingBoxBuilder_ == null) {
return boundingBox_ == null ? com.cognite.client.dto.ThreeDNode.BoundingBox.getDefaultInstance() : boundingBox_;
} else {
return boundingBoxBuilder_.getMessage();
}
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.BoundingBox boundingBox = 8;
*/
public Builder setBoundingBox(com.cognite.client.dto.ThreeDNode.BoundingBox value) {
if (boundingBoxBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
boundingBox_ = value;
onChanged();
} else {
boundingBoxBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.BoundingBox boundingBox = 8;
*/
public Builder setBoundingBox(
com.cognite.client.dto.ThreeDNode.BoundingBox.Builder builderForValue) {
if (boundingBoxBuilder_ == null) {
boundingBox_ = builderForValue.build();
onChanged();
} else {
boundingBoxBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.BoundingBox boundingBox = 8;
*/
public Builder mergeBoundingBox(com.cognite.client.dto.ThreeDNode.BoundingBox value) {
if (boundingBoxBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0) &&
boundingBox_ != null &&
boundingBox_ != com.cognite.client.dto.ThreeDNode.BoundingBox.getDefaultInstance()) {
boundingBox_ =
com.cognite.client.dto.ThreeDNode.BoundingBox.newBuilder(boundingBox_).mergeFrom(value).buildPartial();
} else {
boundingBox_ = value;
}
onChanged();
} else {
boundingBoxBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.BoundingBox boundingBox = 8;
*/
public Builder clearBoundingBox() {
if (boundingBoxBuilder_ == null) {
boundingBox_ = null;
onChanged();
} else {
boundingBoxBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.BoundingBox boundingBox = 8;
*/
public com.cognite.client.dto.ThreeDNode.BoundingBox.Builder getBoundingBoxBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getBoundingBoxFieldBuilder().getBuilder();
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.BoundingBox boundingBox = 8;
*/
public com.cognite.client.dto.ThreeDNode.BoundingBoxOrBuilder getBoundingBoxOrBuilder() {
if (boundingBoxBuilder_ != null) {
return boundingBoxBuilder_.getMessageOrBuilder();
} else {
return boundingBox_ == null ?
com.cognite.client.dto.ThreeDNode.BoundingBox.getDefaultInstance() : boundingBox_;
}
}
/**
* optional .com.cognite.beam.proto.ThreeDNode.BoundingBox boundingBox = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.cognite.client.dto.ThreeDNode.BoundingBox, com.cognite.client.dto.ThreeDNode.BoundingBox.Builder, com.cognite.client.dto.ThreeDNode.BoundingBoxOrBuilder>
getBoundingBoxFieldBuilder() {
if (boundingBoxBuilder_ == null) {
boundingBoxBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.cognite.client.dto.ThreeDNode.BoundingBox, com.cognite.client.dto.ThreeDNode.BoundingBox.Builder, com.cognite.client.dto.ThreeDNode.BoundingBoxOrBuilder>(
getBoundingBox(),
getParentForChildren(),
isClean());
boundingBox_ = null;
}
return boundingBoxBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.cognite.beam.proto.ThreeDNode)
}
// @@protoc_insertion_point(class_scope:com.cognite.beam.proto.ThreeDNode)
private static final com.cognite.client.dto.ThreeDNode DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.cognite.client.dto.ThreeDNode();
}
public static com.cognite.client.dto.ThreeDNode getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ThreeDNode parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ThreeDNode(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.cognite.client.dto.ThreeDNode getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy