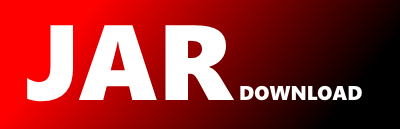
cognitect.aws.batch.specs.clj Maven / Gradle / Ivy
;; Copyright (c) Cognitect, Inc.
;; All rights reserved.
(ns cognitect.aws.batch.specs
(:require [clojure.spec.alpha :as s] [clojure.spec.gen.alpha :as gen]))
(s/def :cognitect.aws/client map?)
(s/def :core.async/channel any?)
(s/def
:cognitect.aws.batch/EFSVolumeConfiguration
(s/keys
:req-un
[:cognitect.aws.batch.EFSVolumeConfiguration/fileSystemId]
:opt-un
[:cognitect.aws.batch.EFSVolumeConfiguration/authorizationConfig
:cognitect.aws.batch.EFSVolumeConfiguration/rootDirectory
:cognitect.aws.batch.EFSVolumeConfiguration/transitEncryptionPort
:cognitect.aws.batch.EFSVolumeConfiguration/transitEncryption]))
(s/def :cognitect.aws.batch/DeregisterJobDefinitionResponse (s/keys))
(s/def
:cognitect.aws.batch/TaskPropertiesOverride
(s/keys :opt-un [:cognitect.aws.batch.TaskPropertiesOverride/containers]))
(s/def :cognitect.aws.batch/JobDependencyList (s/coll-of :cognitect.aws.batch/JobDependency))
(s/def
:cognitect.aws.batch/JobStateTimeLimitActions
(s/coll-of :cognitect.aws.batch/JobStateTimeLimitAction))
(s/def
:cognitect.aws.batch/TagResourceRequest
(s/keys
:req-un
[:cognitect.aws.batch.TagResourceRequest/resourceArn
:cognitect.aws.batch.TagResourceRequest/tags]))
(s/def
:cognitect.aws.batch/EksConfiguration
(s/keys
:req-un
[:cognitect.aws.batch.EksConfiguration/eksClusterArn
:cognitect.aws.batch.EksConfiguration/kubernetesNamespace]))
(s/def
:cognitect.aws.batch/JobQueueDetail
(s/keys
:req-un
[:cognitect.aws.batch.JobQueueDetail/jobQueueName
:cognitect.aws.batch.JobQueueDetail/jobQueueArn
:cognitect.aws.batch.JobQueueDetail/state
:cognitect.aws.batch.JobQueueDetail/priority
:cognitect.aws.batch.JobQueueDetail/computeEnvironmentOrder]
:opt-un
[:cognitect.aws.batch.JobQueueDetail/tags
:cognitect.aws.batch.JobQueueDetail/status
:cognitect.aws.batch.JobQueueDetail/statusReason
:cognitect.aws.batch.JobQueueDetail/jobStateTimeLimitActions
:cognitect.aws.batch.JobQueueDetail/schedulingPolicyArn]))
(s/def :cognitect.aws.batch/ResourceType (s/spec string? :gen #(s/gen #{"MEMORY" "GPU" "VCPU"})))
(s/def
:cognitect.aws.batch/TaskContainerDependencyList
(s/coll-of :cognitect.aws.batch/TaskContainerDependency))
(s/def
:cognitect.aws.batch/CreateJobQueueResponse
(s/keys
:req-un
[:cognitect.aws.batch.CreateJobQueueResponse/jobQueueName
:cognitect.aws.batch.CreateJobQueueResponse/jobQueueArn]))
(s/def :cognitect.aws.batch/TmpfsList (s/coll-of :cognitect.aws.batch/Tmpfs))
(s/def
:cognitect.aws.batch/ComputeEnvironmentDetailList
(s/coll-of :cognitect.aws.batch/ComputeEnvironmentDetail))
(s/def
:cognitect.aws.batch/NodeProperties
(s/keys
:req-un
[:cognitect.aws.batch.NodeProperties/numNodes
:cognitect.aws.batch.NodeProperties/mainNode
:cognitect.aws.batch.NodeProperties/nodeRangeProperties]))
(s/def
:cognitect.aws.batch/EksContainerOverride
(s/keys
:opt-un
[:cognitect.aws.batch.EksContainerOverride/command
:cognitect.aws.batch.EksContainerOverride/args
:cognitect.aws.batch.EksContainerOverride/image
:cognitect.aws.batch.EksContainerOverride/name
:cognitect.aws.batch.EksContainerOverride/env
:cognitect.aws.batch.EksContainerOverride/resources]))
(s/def :cognitect.aws.batch/EksVolumes (s/coll-of :cognitect.aws.batch/EksVolume))
(s/def
:cognitect.aws.batch/EksContainerResourceRequirements
(s/keys
:opt-un
[:cognitect.aws.batch.EksContainerResourceRequirements/limits
:cognitect.aws.batch.EksContainerResourceRequirements/requests]))
(s/def
:cognitect.aws.batch/DescribeJobsRequest
(s/keys :req-un [:cognitect.aws.batch.DescribeJobsRequest/jobs]))
(s/def
:cognitect.aws.batch/AttemptTaskContainerDetails
(s/keys
:opt-un
[:cognitect.aws.batch.AttemptTaskContainerDetails/networkInterfaces
:cognitect.aws.batch.AttemptTaskContainerDetails/exitCode
:cognitect.aws.batch.AttemptTaskContainerDetails/name
:cognitect.aws.batch.AttemptTaskContainerDetails/logStreamName
:cognitect.aws.batch.AttemptTaskContainerDetails/reason]))
(s/def
:cognitect.aws.batch/FrontOfQueueDetail
(s/keys
:opt-un
[:cognitect.aws.batch.FrontOfQueueDetail/lastUpdatedAt
:cognitect.aws.batch.FrontOfQueueDetail/jobs]))
(s/def
:cognitect.aws.batch/ShareAttributes
(s/keys
:req-un
[:cognitect.aws.batch.ShareAttributes/shareIdentifier]
:opt-un
[:cognitect.aws.batch.ShareAttributes/weightFactor]))
(s/def
:cognitect.aws.batch/ListTaskContainerOverrides
(s/coll-of :cognitect.aws.batch/TaskContainerOverrides))
(s/def
:cognitect.aws.batch/ListJobsResponse
(s/keys
:req-un
[:cognitect.aws.batch.ListJobsResponse/jobSummaryList]
:opt-un
[:cognitect.aws.batch.ListJobsResponse/nextToken]))
(s/def
:cognitect.aws.batch/ContainerProperties
(s/keys
:opt-un
[:cognitect.aws.batch.ContainerProperties/runtimePlatform
:cognitect.aws.batch.ContainerProperties/ulimits
:cognitect.aws.batch.ContainerProperties/linuxParameters
:cognitect.aws.batch.ContainerProperties/command
:cognitect.aws.batch.ContainerProperties/mountPoints
:cognitect.aws.batch.ContainerProperties/image
:cognitect.aws.batch.ContainerProperties/resourceRequirements
:cognitect.aws.batch.ContainerProperties/user
:cognitect.aws.batch.ContainerProperties/volumes
:cognitect.aws.batch.ContainerProperties/repositoryCredentials
:cognitect.aws.batch.ContainerProperties/privileged
:cognitect.aws.batch.ContainerProperties/vcpus
:cognitect.aws.batch.ContainerProperties/readonlyRootFilesystem
:cognitect.aws.batch.ContainerProperties/fargatePlatformConfiguration
:cognitect.aws.batch.ContainerProperties/logConfiguration
:cognitect.aws.batch.ContainerProperties/memory
:cognitect.aws.batch.ContainerProperties/instanceType
:cognitect.aws.batch.ContainerProperties/environment
:cognitect.aws.batch.ContainerProperties/networkConfiguration
:cognitect.aws.batch.ContainerProperties/executionRoleArn
:cognitect.aws.batch.ContainerProperties/secrets
:cognitect.aws.batch.ContainerProperties/jobRoleArn
:cognitect.aws.batch.ContainerProperties/ephemeralStorage]))
(s/def
:cognitect.aws.batch/EcsPropertiesOverride
(s/keys :opt-un [:cognitect.aws.batch.EcsPropertiesOverride/taskProperties]))
(s/def
:cognitect.aws.batch/CreateComputeEnvironmentRequest
(s/keys
:req-un
[:cognitect.aws.batch.CreateComputeEnvironmentRequest/computeEnvironmentName
:cognitect.aws.batch.CreateComputeEnvironmentRequest/type]
:opt-un
[:cognitect.aws.batch.CreateComputeEnvironmentRequest/serviceRole
:cognitect.aws.batch.CreateComputeEnvironmentRequest/eksConfiguration
:cognitect.aws.batch.CreateComputeEnvironmentRequest/tags
:cognitect.aws.batch.CreateComputeEnvironmentRequest/context
:cognitect.aws.batch.CreateComputeEnvironmentRequest/unmanagedvCpus
:cognitect.aws.batch.CreateComputeEnvironmentRequest/computeResources
:cognitect.aws.batch.CreateComputeEnvironmentRequest/state]))
(s/def
:cognitect.aws.batch/NodePropertyOverrides
(s/coll-of :cognitect.aws.batch/NodePropertyOverride))
(s/def
:cognitect.aws.batch/LogConfiguration
(s/keys
:req-un
[:cognitect.aws.batch.LogConfiguration/logDriver]
:opt-un
[:cognitect.aws.batch.LogConfiguration/options
:cognitect.aws.batch.LogConfiguration/secretOptions]))
(s/def :cognitect.aws.batch/TagResourceResponse (s/keys))
(s/def :cognitect.aws.batch/MountPoints (s/coll-of :cognitect.aws.batch/MountPoint))
(s/def :cognitect.aws.batch/EvaluateOnExitList (s/coll-of :cognitect.aws.batch/EvaluateOnExit))
(s/def
:cognitect.aws.batch/CreateSchedulingPolicyRequest
(s/keys
:req-un
[:cognitect.aws.batch.CreateSchedulingPolicyRequest/name]
:opt-un
[:cognitect.aws.batch.CreateSchedulingPolicyRequest/tags
:cognitect.aws.batch.CreateSchedulingPolicyRequest/fairsharePolicy]))
(s/def :cognitect.aws.batch/JQState (s/spec string? :gen #(s/gen #{"DISABLED" "ENABLED"})))
(s/def
:cognitect.aws.batch/ParametersMap
(s/map-of :cognitect.aws.batch/String :cognitect.aws.batch/String))
(s/def
:cognitect.aws.batch/AttemptDetail
(s/keys
:opt-un
[:cognitect.aws.batch.AttemptDetail/taskProperties
:cognitect.aws.batch.AttemptDetail/startedAt
:cognitect.aws.batch.AttemptDetail/stoppedAt
:cognitect.aws.batch.AttemptDetail/statusReason
:cognitect.aws.batch.AttemptDetail/container]))
(s/def
:cognitect.aws.batch/EksAttemptContainerDetails
(s/coll-of :cognitect.aws.batch/EksAttemptContainerDetail))
(s/def
:cognitect.aws.batch/EvaluateOnExit
(s/keys
:req-un
[:cognitect.aws.batch.EvaluateOnExit/action]
:opt-un
[:cognitect.aws.batch.EvaluateOnExit/onReason
:cognitect.aws.batch.EvaluateOnExit/onStatusReason
:cognitect.aws.batch.EvaluateOnExit/onExitCode]))
(s/def
:cognitect.aws.batch/JobDependency
(s/keys
:opt-un
[:cognitect.aws.batch.JobDependency/type :cognitect.aws.batch.JobDependency/jobId]))
(s/def
:cognitect.aws.batch/KeyValuePair
(s/keys :opt-un [:cognitect.aws.batch.KeyValuePair/name :cognitect.aws.batch.KeyValuePair/value]))
(s/def
:cognitect.aws.batch/RetryStrategy
(s/keys
:opt-un
[:cognitect.aws.batch.RetryStrategy/evaluateOnExit
:cognitect.aws.batch.RetryStrategy/attempts]))
(s/def
:cognitect.aws.batch/EksEmptyDir
(s/keys
:opt-un
[:cognitect.aws.batch.EksEmptyDir/medium :cognitect.aws.batch.EksEmptyDir/sizeLimit]))
(s/def
:cognitect.aws.batch/CreateComputeEnvironmentResponse
(s/keys
:opt-un
[:cognitect.aws.batch.CreateComputeEnvironmentResponse/computeEnvironmentArn
:cognitect.aws.batch.CreateComputeEnvironmentResponse/computeEnvironmentName]))
(s/def :cognitect.aws.batch/PlatformCapability (s/spec string? :gen #(s/gen #{"EC2" "FARGATE"})))
(s/def
:cognitect.aws.batch/DescribeJobsResponse
(s/keys :opt-un [:cognitect.aws.batch.DescribeJobsResponse/jobs]))
(s/def :cognitect.aws.batch/ShareAttributesList (s/coll-of :cognitect.aws.batch/ShareAttributes))
(s/def
:cognitect.aws.batch/ListEcsTaskProperties
(s/coll-of :cognitect.aws.batch/EcsTaskProperties))
(s/def
:cognitect.aws.batch/EksPropertiesOverride
(s/keys :opt-un [:cognitect.aws.batch.EksPropertiesOverride/podProperties]))
(s/def :cognitect.aws.batch/JobDetailList (s/coll-of :cognitect.aws.batch/JobDetail))
(s/def
:cognitect.aws.batch/SubmitJobRequest
(s/keys
:req-un
[:cognitect.aws.batch.SubmitJobRequest/jobName
:cognitect.aws.batch.SubmitJobRequest/jobQueue
:cognitect.aws.batch.SubmitJobRequest/jobDefinition]
:opt-un
[:cognitect.aws.batch.SubmitJobRequest/eksPropertiesOverride
:cognitect.aws.batch.SubmitJobRequest/retryStrategy
:cognitect.aws.batch.SubmitJobRequest/propagateTags
:cognitect.aws.batch.SubmitJobRequest/tags
:cognitect.aws.batch.SubmitJobRequest/nodeOverrides
:cognitect.aws.batch.SubmitJobRequest/containerOverrides
:cognitect.aws.batch.SubmitJobRequest/timeout
:cognitect.aws.batch.SubmitJobRequest/ecsPropertiesOverride
:cognitect.aws.batch.SubmitJobRequest/schedulingPriorityOverride
:cognitect.aws.batch.SubmitJobRequest/shareIdentifier
:cognitect.aws.batch.SubmitJobRequest/dependsOn
:cognitect.aws.batch.SubmitJobRequest/parameters
:cognitect.aws.batch.SubmitJobRequest/arrayProperties]))
(s/def
:cognitect.aws.batch/EphemeralStorage
(s/keys :req-un [:cognitect.aws.batch.EphemeralStorage/sizeInGiB]))
(s/def
:cognitect.aws.batch/LogConfigurationOptionsMap
(s/map-of :cognitect.aws.batch/String :cognitect.aws.batch/String))
(s/def
:cognitect.aws.batch/UpdateSchedulingPolicyRequest
(s/keys
:req-un
[:cognitect.aws.batch.UpdateSchedulingPolicyRequest/arn]
:opt-un
[:cognitect.aws.batch.UpdateSchedulingPolicyRequest/fairsharePolicy]))
(s/def
:cognitect.aws.batch/DescribeSchedulingPoliciesRequest
(s/keys :req-un [:cognitect.aws.batch.DescribeSchedulingPoliciesRequest/arns]))
(s/def
:cognitect.aws.batch/NodeRangeProperty
(s/keys
:req-un
[:cognitect.aws.batch.NodeRangeProperty/targetNodes]
:opt-un
[:cognitect.aws.batch.NodeRangeProperty/eksProperties
:cognitect.aws.batch.NodeRangeProperty/ecsProperties
:cognitect.aws.batch.NodeRangeProperty/instanceTypes
:cognitect.aws.batch.NodeRangeProperty/container]))
(s/def
:cognitect.aws.batch/EksContainerDetail
(s/keys
:opt-un
[:cognitect.aws.batch.EksContainerDetail/command
:cognitect.aws.batch.EksContainerDetail/args
:cognitect.aws.batch.EksContainerDetail/image
:cognitect.aws.batch.EksContainerDetail/exitCode
:cognitect.aws.batch.EksContainerDetail/securityContext
:cognitect.aws.batch.EksContainerDetail/name
:cognitect.aws.batch.EksContainerDetail/env
:cognitect.aws.batch.EksContainerDetail/reason
:cognitect.aws.batch.EksContainerDetail/volumeMounts
:cognitect.aws.batch.EksContainerDetail/resources
:cognitect.aws.batch.EksContainerDetail/imagePullPolicy]))
(s/def
:cognitect.aws.batch/JobStateTimeLimitActionsAction
(s/spec string? :gen #(s/gen #{"CANCEL"})))
(s/def
:cognitect.aws.batch/DeviceCgroupPermission
(s/spec string? :gen #(s/gen #{"WRITE" "MKNOD" "READ"})))
(s/def
:cognitect.aws.batch/ListAttemptTaskContainerDetails
(s/coll-of :cognitect.aws.batch/AttemptTaskContainerDetails))
(s/def
:cognitect.aws.batch/JobTimeout
(s/keys :opt-un [:cognitect.aws.batch.JobTimeout/attemptDurationSeconds]))
(s/def
:cognitect.aws.batch/JobStatus
(s/spec
string?
:gen
#(s/gen #{"RUNNABLE" "PENDING" "SUBMITTED" "SUCCEEDED" "STARTING" "FAILED" "RUNNING"})))
(s/def
:cognitect.aws.batch/EksContainerEnvironmentVariables
(s/coll-of :cognitect.aws.batch/EksContainerEnvironmentVariable))
(s/def
:cognitect.aws.batch/EcsTaskDetails
(s/keys
:opt-un
[:cognitect.aws.batch.EcsTaskDetails/runtimePlatform
:cognitect.aws.batch.EcsTaskDetails/volumes
:cognitect.aws.batch.EcsTaskDetails/taskRoleArn
:cognitect.aws.batch.EcsTaskDetails/taskArn
:cognitect.aws.batch.EcsTaskDetails/containerInstanceArn
:cognitect.aws.batch.EcsTaskDetails/networkConfiguration
:cognitect.aws.batch.EcsTaskDetails/platformVersion
:cognitect.aws.batch.EcsTaskDetails/executionRoleArn
:cognitect.aws.batch.EcsTaskDetails/ipcMode
:cognitect.aws.batch.EcsTaskDetails/containers
:cognitect.aws.batch.EcsTaskDetails/pidMode
:cognitect.aws.batch.EcsTaskDetails/ephemeralStorage]))
(s/def
:cognitect.aws.batch/MountPoint
(s/keys
:opt-un
[:cognitect.aws.batch.MountPoint/sourceVolume
:cognitect.aws.batch.MountPoint/readOnly
:cognitect.aws.batch.MountPoint/containerPath]))
(s/def :cognitect.aws.batch/DeleteJobQueueResponse (s/keys))
(s/def
:cognitect.aws.batch/NetworkInterface
(s/keys
:opt-un
[:cognitect.aws.batch.NetworkInterface/privateIpv4Address
:cognitect.aws.batch.NetworkInterface/ipv6Address
:cognitect.aws.batch.NetworkInterface/attachmentId]))
(s/def
:cognitect.aws.batch/ListJobsRequest
(s/keys
:opt-un
[:cognitect.aws.batch.ListJobsRequest/jobQueue
:cognitect.aws.batch.ListJobsRequest/filters
:cognitect.aws.batch.ListJobsRequest/maxResults
:cognitect.aws.batch.ListJobsRequest/nextToken
:cognitect.aws.batch.ListJobsRequest/arrayJobId
:cognitect.aws.batch.ListJobsRequest/multiNodeJobId
:cognitect.aws.batch.ListJobsRequest/jobStatus]))
(s/def
:cognitect.aws.batch/DescribeJobQueuesRequest
(s/keys
:opt-un
[:cognitect.aws.batch.DescribeJobQueuesRequest/jobQueues
:cognitect.aws.batch.DescribeJobQueuesRequest/maxResults
:cognitect.aws.batch.DescribeJobQueuesRequest/nextToken]))
(s/def
:cognitect.aws.batch/ContainerOverrides
(s/keys
:opt-un
[:cognitect.aws.batch.ContainerOverrides/command
:cognitect.aws.batch.ContainerOverrides/resourceRequirements
:cognitect.aws.batch.ContainerOverrides/vcpus
:cognitect.aws.batch.ContainerOverrides/memory
:cognitect.aws.batch.ContainerOverrides/instanceType
:cognitect.aws.batch.ContainerOverrides/environment]))
(s/def
:cognitect.aws.batch/SchedulingPolicyListingDetail
(s/keys :req-un [:cognitect.aws.batch.SchedulingPolicyListingDetail/arn]))
(s/def
:cognitect.aws.batch/SchedulingPolicyListingDetailList
(s/coll-of :cognitect.aws.batch/SchedulingPolicyListingDetail))
(s/def :cognitect.aws.batch/JobDefinitionList (s/coll-of :cognitect.aws.batch/JobDefinition))
(s/def
:cognitect.aws.batch/EFSTransitEncryption
(s/spec string? :gen #(s/gen #{"DISABLED" "ENABLED"})))
(s/def
:cognitect.aws.batch/KubernetesVersion
(s/spec
(s/and string? #(<= 1 (count %) 256))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 256) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.batch/NodeDetails
(s/keys
:opt-un
[:cognitect.aws.batch.NodeDetails/isMainNode :cognitect.aws.batch.NodeDetails/nodeIndex]))
(s/def
:cognitect.aws.batch/RuntimePlatform
(s/keys
:opt-un
[:cognitect.aws.batch.RuntimePlatform/operatingSystemFamily
:cognitect.aws.batch.RuntimePlatform/cpuArchitecture]))
(s/def
:cognitect.aws.batch/Tmpfs
(s/keys
:req-un
[:cognitect.aws.batch.Tmpfs/containerPath :cognitect.aws.batch.Tmpfs/size]
:opt-un
[:cognitect.aws.batch.Tmpfs/mountOptions]))
(s/def
:cognitect.aws.batch/ListSchedulingPoliciesResponse
(s/keys
:opt-un
[:cognitect.aws.batch.ListSchedulingPoliciesResponse/nextToken
:cognitect.aws.batch.ListSchedulingPoliciesResponse/schedulingPolicies]))
(s/def
:cognitect.aws.batch/ResourceRequirements
(s/coll-of :cognitect.aws.batch/ResourceRequirement))
(s/def :cognitect.aws.batch/Boolean boolean?)
(s/def
:cognitect.aws.batch/ContainerDetail
(s/keys
:opt-un
[:cognitect.aws.batch.ContainerDetail/runtimePlatform
:cognitect.aws.batch.ContainerDetail/ulimits
:cognitect.aws.batch.ContainerDetail/linuxParameters
:cognitect.aws.batch.ContainerDetail/command
:cognitect.aws.batch.ContainerDetail/mountPoints
:cognitect.aws.batch.ContainerDetail/networkInterfaces
:cognitect.aws.batch.ContainerDetail/image
:cognitect.aws.batch.ContainerDetail/exitCode
:cognitect.aws.batch.ContainerDetail/resourceRequirements
:cognitect.aws.batch.ContainerDetail/user
:cognitect.aws.batch.ContainerDetail/volumes
:cognitect.aws.batch.ContainerDetail/repositoryCredentials
:cognitect.aws.batch.ContainerDetail/privileged
:cognitect.aws.batch.ContainerDetail/vcpus
:cognitect.aws.batch.ContainerDetail/readonlyRootFilesystem
:cognitect.aws.batch.ContainerDetail/fargatePlatformConfiguration
:cognitect.aws.batch.ContainerDetail/logConfiguration
:cognitect.aws.batch.ContainerDetail/taskArn
:cognitect.aws.batch.ContainerDetail/memory
:cognitect.aws.batch.ContainerDetail/logStreamName
:cognitect.aws.batch.ContainerDetail/instanceType
:cognitect.aws.batch.ContainerDetail/containerInstanceArn
:cognitect.aws.batch.ContainerDetail/environment
:cognitect.aws.batch.ContainerDetail/networkConfiguration
:cognitect.aws.batch.ContainerDetail/reason
:cognitect.aws.batch.ContainerDetail/executionRoleArn
:cognitect.aws.batch.ContainerDetail/secrets
:cognitect.aws.batch.ContainerDetail/jobRoleArn
:cognitect.aws.batch.ContainerDetail/ephemeralStorage]))
(s/def
:cognitect.aws.batch/ListTaskPropertiesOverride
(s/coll-of :cognitect.aws.batch/TaskPropertiesOverride))
(s/def
:cognitect.aws.batch/CreateSchedulingPolicyResponse
(s/keys
:req-un
[:cognitect.aws.batch.CreateSchedulingPolicyResponse/name
:cognitect.aws.batch.CreateSchedulingPolicyResponse/arn]))
(s/def
:cognitect.aws.batch/DeleteSchedulingPolicyRequest
(s/keys :req-un [:cognitect.aws.batch.DeleteSchedulingPolicyRequest/arn]))
(s/def
:cognitect.aws.batch/ListSchedulingPoliciesRequest
(s/keys
:opt-un
[:cognitect.aws.batch.ListSchedulingPoliciesRequest/maxResults
:cognitect.aws.batch.ListSchedulingPoliciesRequest/nextToken]))
(s/def
:cognitect.aws.batch/EksPodPropertiesDetail
(s/keys
:opt-un
[:cognitect.aws.batch.EksPodPropertiesDetail/serviceAccountName
:cognitect.aws.batch.EksPodPropertiesDetail/hostNetwork
:cognitect.aws.batch.EksPodPropertiesDetail/shareProcessNamespace
:cognitect.aws.batch.EksPodPropertiesDetail/initContainers
:cognitect.aws.batch.EksPodPropertiesDetail/volumes
:cognitect.aws.batch.EksPodPropertiesDetail/nodeName
:cognitect.aws.batch.EksPodPropertiesDetail/metadata
:cognitect.aws.batch.EksPodPropertiesDetail/imagePullSecrets
:cognitect.aws.batch.EksPodPropertiesDetail/containers
:cognitect.aws.batch.EksPodPropertiesDetail/podName
:cognitect.aws.batch.EksPodPropertiesDetail/dnsPolicy]))
(s/def
:cognitect.aws.batch/EksRequests
(s/map-of :cognitect.aws.batch/String :cognitect.aws.batch/Quantity))
(s/def
:cognitect.aws.batch/UpdatePolicy
(s/keys
:opt-un
[:cognitect.aws.batch.UpdatePolicy/terminateJobsOnUpdate
:cognitect.aws.batch.UpdatePolicy/jobExecutionTimeoutMinutes]))
(s/def
:cognitect.aws.batch/TaskContainerOverrides
(s/keys
:opt-un
[:cognitect.aws.batch.TaskContainerOverrides/command
:cognitect.aws.batch.TaskContainerOverrides/resourceRequirements
:cognitect.aws.batch.TaskContainerOverrides/name
:cognitect.aws.batch.TaskContainerOverrides/environment]))
(s/def
:cognitect.aws.batch/ArrayJobStatusSummary
(s/map-of :cognitect.aws.batch/String :cognitect.aws.batch/Integer))
(s/def :cognitect.aws.batch/EksContainerDetails (s/coll-of :cognitect.aws.batch/EksContainerDetail))
(s/def :cognitect.aws.batch/Ec2ConfigurationList (s/coll-of :cognitect.aws.batch/Ec2Configuration))
(s/def
:cognitect.aws.batch/EksContainerVolumeMount
(s/keys
:opt-un
[:cognitect.aws.batch.EksContainerVolumeMount/name
:cognitect.aws.batch.EksContainerVolumeMount/readOnly
:cognitect.aws.batch.EksContainerVolumeMount/mountPath]))
(s/def
:cognitect.aws.batch/TaskContainerDetails
(s/keys
:opt-un
[:cognitect.aws.batch.TaskContainerDetails/ulimits
:cognitect.aws.batch.TaskContainerDetails/linuxParameters
:cognitect.aws.batch.TaskContainerDetails/command
:cognitect.aws.batch.TaskContainerDetails/mountPoints
:cognitect.aws.batch.TaskContainerDetails/networkInterfaces
:cognitect.aws.batch.TaskContainerDetails/image
:cognitect.aws.batch.TaskContainerDetails/exitCode
:cognitect.aws.batch.TaskContainerDetails/resourceRequirements
:cognitect.aws.batch.TaskContainerDetails/user
:cognitect.aws.batch.TaskContainerDetails/repositoryCredentials
:cognitect.aws.batch.TaskContainerDetails/privileged
:cognitect.aws.batch.TaskContainerDetails/readonlyRootFilesystem
:cognitect.aws.batch.TaskContainerDetails/name
:cognitect.aws.batch.TaskContainerDetails/logConfiguration
:cognitect.aws.batch.TaskContainerDetails/essential
:cognitect.aws.batch.TaskContainerDetails/logStreamName
:cognitect.aws.batch.TaskContainerDetails/environment
:cognitect.aws.batch.TaskContainerDetails/reason
:cognitect.aws.batch.TaskContainerDetails/dependsOn
:cognitect.aws.batch.TaskContainerDetails/secrets]))
(s/def :cognitect.aws.batch/OrchestrationType (s/spec string? :gen #(s/gen #{"ECS" "EKS"})))
(s/def
:cognitect.aws.batch/EksContainerOverrideList
(s/coll-of :cognitect.aws.batch/EksContainerOverride))
(s/def :cognitect.aws.batch/CEState (s/spec string? :gen #(s/gen #{"DISABLED" "ENABLED"})))
(s/def
:cognitect.aws.batch/TagKeysList
(s/coll-of :cognitect.aws.batch/TagKey :min-count 1 :max-count 50))
(s/def
:cognitect.aws.batch/AttemptEcsTaskDetails
(s/keys
:opt-un
[:cognitect.aws.batch.AttemptEcsTaskDetails/taskArn
:cognitect.aws.batch.AttemptEcsTaskDetails/containerInstanceArn
:cognitect.aws.batch.AttemptEcsTaskDetails/containers]))
(s/def :cognitect.aws.batch/NetworkInterfaceList (s/coll-of :cognitect.aws.batch/NetworkInterface))
(s/def
:cognitect.aws.batch/TagrisTagsMap
(s/map-of :cognitect.aws.batch/TagKey :cognitect.aws.batch/TagValue :min-count 1 :max-count 50))
(s/def
:cognitect.aws.batch/Secret
(s/keys :req-un [:cognitect.aws.batch.Secret/name :cognitect.aws.batch.Secret/valueFrom]))
(s/def :cognitect.aws.batch/DevicesList (s/coll-of :cognitect.aws.batch/Device))
(s/def :cognitect.aws.batch/Integer (s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.batch/EksVolume
(s/keys
:req-un
[:cognitect.aws.batch.EksVolume/name]
:opt-un
[:cognitect.aws.batch.EksVolume/emptyDir
:cognitect.aws.batch.EksVolume/hostPath
:cognitect.aws.batch.EksVolume/secret]))
(s/def
:cognitect.aws.batch/DeleteJobQueueRequest
(s/keys :req-un [:cognitect.aws.batch.DeleteJobQueueRequest/jobQueue]))
(s/def
:cognitect.aws.batch/JobDefinition
(s/keys
:req-un
[:cognitect.aws.batch.JobDefinition/jobDefinitionName
:cognitect.aws.batch.JobDefinition/jobDefinitionArn
:cognitect.aws.batch.JobDefinition/revision
:cognitect.aws.batch.JobDefinition/type]
:opt-un
[:cognitect.aws.batch.JobDefinition/retryStrategy
:cognitect.aws.batch.JobDefinition/platformCapabilities
:cognitect.aws.batch.JobDefinition/propagateTags
:cognitect.aws.batch.JobDefinition/containerProperties
:cognitect.aws.batch.JobDefinition/tags
:cognitect.aws.batch.JobDefinition/eksProperties
:cognitect.aws.batch.JobDefinition/nodeProperties
:cognitect.aws.batch.JobDefinition/ecsProperties
:cognitect.aws.batch.JobDefinition/containerOrchestrationType
:cognitect.aws.batch.JobDefinition/status
:cognitect.aws.batch.JobDefinition/timeout
:cognitect.aws.batch.JobDefinition/schedulingPriority
:cognitect.aws.batch.JobDefinition/parameters]))
(s/def :cognitect.aws.batch/EksHostPath (s/keys :opt-un [:cognitect.aws.batch.EksHostPath/path]))
(s/def :cognitect.aws.batch/CancelJobResponse (s/keys))
(s/def :cognitect.aws.batch/TerminateJobResponse (s/keys))
(s/def
:cognitect.aws.batch/TagValue
(s/spec
(s/and string? #(>= 256 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 256) #(gen/vector (gen/char-alpha) %))))))
(s/def :cognitect.aws.batch/AssignPublicIp (s/spec string? :gen #(s/gen #{"DISABLED" "ENABLED"})))
(s/def
:cognitect.aws.batch/JobStateTimeLimitActionsState
(s/spec string? :gen #(s/gen #{"RUNNABLE"})))
(s/def :cognitect.aws.batch/NodeRangeProperties (s/coll-of :cognitect.aws.batch/NodeRangeProperty))
(s/def
:cognitect.aws.batch/Volume
(s/keys
:opt-un
[:cognitect.aws.batch.Volume/efsVolumeConfiguration
:cognitect.aws.batch.Volume/host
:cognitect.aws.batch.Volume/name]))
(s/def
:cognitect.aws.batch/UpdateComputeEnvironmentResponse
(s/keys
:opt-un
[:cognitect.aws.batch.UpdateComputeEnvironmentResponse/computeEnvironmentArn
:cognitect.aws.batch.UpdateComputeEnvironmentResponse/computeEnvironmentName]))
(s/def
:cognitect.aws.batch/ListTagsForResourceRequest
(s/keys :req-un [:cognitect.aws.batch.ListTagsForResourceRequest/resourceArn]))
(s/def
:cognitect.aws.batch/ArrayProperties
(s/keys :opt-un [:cognitect.aws.batch.ArrayProperties/size]))
(s/def :cognitect.aws.batch/AttemptDetails (s/coll-of :cognitect.aws.batch/AttemptDetail))
(s/def :cognitect.aws.batch/DeleteSchedulingPolicyResponse (s/keys))
(s/def
:cognitect.aws.batch/ArrayJobDependency
(s/spec string? :gen #(s/gen #{"SEQUENTIAL" "N_TO_N"})))
(s/def :cognitect.aws.batch/SecretList (s/coll-of :cognitect.aws.batch/Secret))
(s/def :cognitect.aws.batch/UntagResourceResponse (s/keys))
(s/def
:cognitect.aws.batch/ContainerSummary
(s/keys
:opt-un
[:cognitect.aws.batch.ContainerSummary/exitCode :cognitect.aws.batch.ContainerSummary/reason]))
(s/def
:cognitect.aws.batch/KeyValuesPair
(s/keys
:opt-un
[:cognitect.aws.batch.KeyValuesPair/values :cognitect.aws.batch.KeyValuesPair/name]))
(s/def
:cognitect.aws.batch/EksContainer
(s/keys
:req-un
[:cognitect.aws.batch.EksContainer/image]
:opt-un
[:cognitect.aws.batch.EksContainer/command
:cognitect.aws.batch.EksContainer/args
:cognitect.aws.batch.EksContainer/securityContext
:cognitect.aws.batch.EksContainer/name
:cognitect.aws.batch.EksContainer/env
:cognitect.aws.batch.EksContainer/volumeMounts
:cognitect.aws.batch.EksContainer/resources
:cognitect.aws.batch.EksContainer/imagePullPolicy]))
(s/def
:cognitect.aws.batch/ResourceRequirement
(s/keys
:req-un
[:cognitect.aws.batch.ResourceRequirement/value :cognitect.aws.batch.ResourceRequirement/type]))
(s/def :cognitect.aws.batch/DeleteComputeEnvironmentResponse (s/keys))
(s/def :cognitect.aws.batch/EksAttemptDetails (s/coll-of :cognitect.aws.batch/EksAttemptDetail))
(s/def
:cognitect.aws.batch/ComputeEnvironmentDetail
(s/keys
:req-un
[:cognitect.aws.batch.ComputeEnvironmentDetail/computeEnvironmentName
:cognitect.aws.batch.ComputeEnvironmentDetail/computeEnvironmentArn]
:opt-un
[:cognitect.aws.batch.ComputeEnvironmentDetail/serviceRole
:cognitect.aws.batch.ComputeEnvironmentDetail/eksConfiguration
:cognitect.aws.batch.ComputeEnvironmentDetail/tags
:cognitect.aws.batch.ComputeEnvironmentDetail/uuid
:cognitect.aws.batch.ComputeEnvironmentDetail/containerOrchestrationType
:cognitect.aws.batch.ComputeEnvironmentDetail/context
:cognitect.aws.batch.ComputeEnvironmentDetail/ecsClusterArn
:cognitect.aws.batch.ComputeEnvironmentDetail/status
:cognitect.aws.batch.ComputeEnvironmentDetail/unmanagedvCpus
:cognitect.aws.batch.ComputeEnvironmentDetail/computeResources
:cognitect.aws.batch.ComputeEnvironmentDetail/updatePolicy
:cognitect.aws.batch.ComputeEnvironmentDetail/type
:cognitect.aws.batch.ComputeEnvironmentDetail/state
:cognitect.aws.batch.ComputeEnvironmentDetail/statusReason]))
(s/def
:cognitect.aws.batch/ComputeEnvironmentOrder
(s/keys
:req-un
[:cognitect.aws.batch.ComputeEnvironmentOrder/order
:cognitect.aws.batch.ComputeEnvironmentOrder/computeEnvironment]))
(s/def :cognitect.aws.batch/ListJobsFilterList (s/coll-of :cognitect.aws.batch/KeyValuesPair))
(s/def
:cognitect.aws.batch/NetworkConfiguration
(s/keys :opt-un [:cognitect.aws.batch.NetworkConfiguration/assignPublicIp]))
(s/def
:cognitect.aws.batch/JobDefinitionType
(s/spec string? :gen #(s/gen #{"multinode" "container"})))
(s/def
:cognitect.aws.batch/EksContainerSecurityContext
(s/keys
:opt-un
[:cognitect.aws.batch.EksContainerSecurityContext/runAsNonRoot
:cognitect.aws.batch.EksContainerSecurityContext/privileged
:cognitect.aws.batch.EksContainerSecurityContext/readOnlyRootFilesystem
:cognitect.aws.batch.EksContainerSecurityContext/allowPrivilegeEscalation
:cognitect.aws.batch.EksContainerSecurityContext/runAsUser
:cognitect.aws.batch.EksContainerSecurityContext/runAsGroup]))
(s/def
:cognitect.aws.batch/JobDetail
(s/keys
:req-un
[:cognitect.aws.batch.JobDetail/jobName
:cognitect.aws.batch.JobDetail/jobId
:cognitect.aws.batch.JobDetail/jobQueue
:cognitect.aws.batch.JobDetail/status
:cognitect.aws.batch.JobDetail/startedAt
:cognitect.aws.batch.JobDetail/jobDefinition]
:opt-un
[:cognitect.aws.batch.JobDetail/retryStrategy
:cognitect.aws.batch.JobDetail/eksAttempts
:cognitect.aws.batch.JobDetail/platformCapabilities
:cognitect.aws.batch.JobDetail/propagateTags
:cognitect.aws.batch.JobDetail/createdAt
:cognitect.aws.batch.JobDetail/isCancelled
:cognitect.aws.batch.JobDetail/nodeDetails
:cognitect.aws.batch.JobDetail/tags
:cognitect.aws.batch.JobDetail/eksProperties
:cognitect.aws.batch.JobDetail/nodeProperties
:cognitect.aws.batch.JobDetail/ecsProperties
:cognitect.aws.batch.JobDetail/stoppedAt
:cognitect.aws.batch.JobDetail/timeout
:cognitect.aws.batch.JobDetail/attempts
:cognitect.aws.batch.JobDetail/jobArn
:cognitect.aws.batch.JobDetail/schedulingPriority
:cognitect.aws.batch.JobDetail/shareIdentifier
:cognitect.aws.batch.JobDetail/statusReason
:cognitect.aws.batch.JobDetail/isTerminated
:cognitect.aws.batch.JobDetail/dependsOn
:cognitect.aws.batch.JobDetail/parameters
:cognitect.aws.batch.JobDetail/arrayProperties
:cognitect.aws.batch.JobDetail/container]))
(s/def
:cognitect.aws.batch/TaskContainerProperties
(s/keys
:req-un
[:cognitect.aws.batch.TaskContainerProperties/image]
:opt-un
[:cognitect.aws.batch.TaskContainerProperties/ulimits
:cognitect.aws.batch.TaskContainerProperties/linuxParameters
:cognitect.aws.batch.TaskContainerProperties/command
:cognitect.aws.batch.TaskContainerProperties/mountPoints
:cognitect.aws.batch.TaskContainerProperties/resourceRequirements
:cognitect.aws.batch.TaskContainerProperties/user
:cognitect.aws.batch.TaskContainerProperties/repositoryCredentials
:cognitect.aws.batch.TaskContainerProperties/privileged
:cognitect.aws.batch.TaskContainerProperties/readonlyRootFilesystem
:cognitect.aws.batch.TaskContainerProperties/name
:cognitect.aws.batch.TaskContainerProperties/logConfiguration
:cognitect.aws.batch.TaskContainerProperties/essential
:cognitect.aws.batch.TaskContainerProperties/environment
:cognitect.aws.batch.TaskContainerProperties/dependsOn
:cognitect.aws.batch.TaskContainerProperties/secrets]))
(s/def
:cognitect.aws.batch/CreateJobQueueRequest
(s/keys
:req-un
[:cognitect.aws.batch.CreateJobQueueRequest/jobQueueName
:cognitect.aws.batch.CreateJobQueueRequest/priority
:cognitect.aws.batch.CreateJobQueueRequest/computeEnvironmentOrder]
:opt-un
[:cognitect.aws.batch.CreateJobQueueRequest/tags
:cognitect.aws.batch.CreateJobQueueRequest/state
:cognitect.aws.batch.CreateJobQueueRequest/jobStateTimeLimitActions
:cognitect.aws.batch.CreateJobQueueRequest/schedulingPolicyArn]))
(s/def
:cognitect.aws.batch/Ec2Configuration
(s/keys
:req-un
[:cognitect.aws.batch.Ec2Configuration/imageType]
:opt-un
[:cognitect.aws.batch.Ec2Configuration/imageKubernetesVersion
:cognitect.aws.batch.Ec2Configuration/imageIdOverride]))
(s/def
:cognitect.aws.batch/CRAllocationStrategy
(s/spec
string?
:gen
#(s/gen
#{"SPOT_PRICE_CAPACITY_OPTIMIZED"
"BEST_FIT_PROGRESSIVE"
"BEST_FIT"
"SPOT_CAPACITY_OPTIMIZED"})))
(s/def
:cognitect.aws.batch/JobExecutionTimeoutMinutes
(s/spec (s/and int? #(<= 1 % 360)) :gen #(gen/choose 1 360)))
(s/def
:cognitect.aws.batch/EksPodPropertiesOverride
(s/keys
:opt-un
[:cognitect.aws.batch.EksPodPropertiesOverride/initContainers
:cognitect.aws.batch.EksPodPropertiesOverride/metadata
:cognitect.aws.batch.EksPodPropertiesOverride/containers]))
(s/def
:cognitect.aws.batch/ImageType
(s/spec
(s/and string? #(<= 1 (count %) 256))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 256) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.batch/RepositoryCredentials
(s/keys :req-un [:cognitect.aws.batch.RepositoryCredentials/credentialsParameter]))
(s/def
:cognitect.aws.batch/DescribeJobDefinitionsRequest
(s/keys
:opt-un
[:cognitect.aws.batch.DescribeJobDefinitionsRequest/jobDefinitions
:cognitect.aws.batch.DescribeJobDefinitionsRequest/jobDefinitionName
:cognitect.aws.batch.DescribeJobDefinitionsRequest/maxResults
:cognitect.aws.batch.DescribeJobDefinitionsRequest/nextToken
:cognitect.aws.batch.DescribeJobDefinitionsRequest/status]))
(s/def :cognitect.aws.batch/EnvironmentVariables (s/coll-of :cognitect.aws.batch/KeyValuePair))
(s/def
:cognitect.aws.batch/EksSecret
(s/keys
:req-un
[:cognitect.aws.batch.EksSecret/secretName]
:opt-un
[:cognitect.aws.batch.EksSecret/optional]))
(s/def
:cognitect.aws.batch/Float
(s/spec double? :gen #(gen/double* {:infinite? false, :NaN? false})))
(s/def
:cognitect.aws.batch/EFSAuthorizationConfig
(s/keys
:opt-un
[:cognitect.aws.batch.EFSAuthorizationConfig/accessPointId
:cognitect.aws.batch.EFSAuthorizationConfig/iam]))
(s/def
:cognitect.aws.batch/RegisterJobDefinitionRequest
(s/keys
:req-un
[:cognitect.aws.batch.RegisterJobDefinitionRequest/jobDefinitionName
:cognitect.aws.batch.RegisterJobDefinitionRequest/type]
:opt-un
[:cognitect.aws.batch.RegisterJobDefinitionRequest/retryStrategy
:cognitect.aws.batch.RegisterJobDefinitionRequest/platformCapabilities
:cognitect.aws.batch.RegisterJobDefinitionRequest/propagateTags
:cognitect.aws.batch.RegisterJobDefinitionRequest/containerProperties
:cognitect.aws.batch.RegisterJobDefinitionRequest/tags
:cognitect.aws.batch.RegisterJobDefinitionRequest/eksProperties
:cognitect.aws.batch.RegisterJobDefinitionRequest/nodeProperties
:cognitect.aws.batch.RegisterJobDefinitionRequest/ecsProperties
:cognitect.aws.batch.RegisterJobDefinitionRequest/timeout
:cognitect.aws.batch.RegisterJobDefinitionRequest/schedulingPriority
:cognitect.aws.batch.RegisterJobDefinitionRequest/parameters]))
(s/def
:cognitect.aws.batch/ComputeEnvironmentOrders
(s/coll-of :cognitect.aws.batch/ComputeEnvironmentOrder))
(s/def :cognitect.aws.batch/Ulimits (s/coll-of :cognitect.aws.batch/Ulimit))
(s/def
:cognitect.aws.batch/EksContainerEnvironmentVariable
(s/keys
:req-un
[:cognitect.aws.batch.EksContainerEnvironmentVariable/name]
:opt-un
[:cognitect.aws.batch.EksContainerEnvironmentVariable/value]))
(s/def
:cognitect.aws.batch/TaskContainerDependency
(s/keys
:opt-un
[:cognitect.aws.batch.TaskContainerDependency/condition
:cognitect.aws.batch.TaskContainerDependency/containerName]))
(s/def
:cognitect.aws.batch/EcsTaskProperties
(s/keys
:req-un
[:cognitect.aws.batch.EcsTaskProperties/containers]
:opt-un
[:cognitect.aws.batch.EcsTaskProperties/runtimePlatform
:cognitect.aws.batch.EcsTaskProperties/volumes
:cognitect.aws.batch.EcsTaskProperties/taskRoleArn
:cognitect.aws.batch.EcsTaskProperties/networkConfiguration
:cognitect.aws.batch.EcsTaskProperties/platformVersion
:cognitect.aws.batch.EcsTaskProperties/executionRoleArn
:cognitect.aws.batch.EcsTaskProperties/ipcMode
:cognitect.aws.batch.EcsTaskProperties/pidMode
:cognitect.aws.batch.EcsTaskProperties/ephemeralStorage]))
(s/def
:cognitect.aws.batch/EksLabelsMap
(s/map-of :cognitect.aws.batch/String :cognitect.aws.batch/String))
(s/def :cognitect.aws.batch/Long (s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.batch/EcsProperties
(s/keys :req-un [:cognitect.aws.batch.EcsProperties/taskProperties]))
(s/def
:cognitect.aws.batch/FargatePlatformConfiguration
(s/keys :opt-un [:cognitect.aws.batch.FargatePlatformConfiguration/platformVersion]))
(s/def :cognitect.aws.batch/ImagePullSecrets (s/coll-of :cognitect.aws.batch/ImagePullSecret))
(s/def
:cognitect.aws.batch/CRType
(s/spec string? :gen #(s/gen #{"EC2" "FARGATE" "SPOT" "FARGATE_SPOT"})))
(s/def
:cognitect.aws.batch/DescribeComputeEnvironmentsResponse
(s/keys
:opt-un
[:cognitect.aws.batch.DescribeComputeEnvironmentsResponse/computeEnvironments
:cognitect.aws.batch.DescribeComputeEnvironmentsResponse/nextToken]))
(s/def
:cognitect.aws.batch/JQStatus
(s/spec string? :gen #(s/gen #{"UPDATING" "INVALID" "DELETING" "CREATING" "DELETED" "VALID"})))
(s/def
:cognitect.aws.batch/UpdateComputeEnvironmentRequest
(s/keys
:req-un
[:cognitect.aws.batch.UpdateComputeEnvironmentRequest/computeEnvironment]
:opt-un
[:cognitect.aws.batch.UpdateComputeEnvironmentRequest/serviceRole
:cognitect.aws.batch.UpdateComputeEnvironmentRequest/context
:cognitect.aws.batch.UpdateComputeEnvironmentRequest/unmanagedvCpus
:cognitect.aws.batch.UpdateComputeEnvironmentRequest/computeResources
:cognitect.aws.batch.UpdateComputeEnvironmentRequest/updatePolicy
:cognitect.aws.batch.UpdateComputeEnvironmentRequest/state]))
(s/def :cognitect.aws.batch/UpdateSchedulingPolicyResponse (s/keys))
(s/def
:cognitect.aws.batch/UpdateJobQueueResponse
(s/keys
:opt-un
[:cognitect.aws.batch.UpdateJobQueueResponse/jobQueueName
:cognitect.aws.batch.UpdateJobQueueResponse/jobQueueArn]))
(s/def :cognitect.aws.batch/EksContainers (s/coll-of :cognitect.aws.batch/EksContainer))
(s/def
:cognitect.aws.batch/EksProperties
(s/keys :opt-un [:cognitect.aws.batch.EksProperties/podProperties]))
(s/def
:cognitect.aws.batch/EksAttemptContainerDetail
(s/keys
:opt-un
[:cognitect.aws.batch.EksAttemptContainerDetail/exitCode
:cognitect.aws.batch.EksAttemptContainerDetail/name
:cognitect.aws.batch.EksAttemptContainerDetail/reason]))
(s/def
:cognitect.aws.batch/EksLimits
(s/map-of :cognitect.aws.batch/String :cognitect.aws.batch/Quantity))
(s/def :cognitect.aws.batch/Volumes (s/coll-of :cognitect.aws.batch/Volume))
(s/def
:cognitect.aws.batch/RegisterJobDefinitionResponse
(s/keys
:req-un
[:cognitect.aws.batch.RegisterJobDefinitionResponse/jobDefinitionName
:cognitect.aws.batch.RegisterJobDefinitionResponse/jobDefinitionArn
:cognitect.aws.batch.RegisterJobDefinitionResponse/revision]))
(s/def :cognitect.aws.batch/CEType (s/spec string? :gen #(s/gen #{"MANAGED" "UNMANAGED"})))
(s/def
:cognitect.aws.batch/UntagResourceRequest
(s/keys
:req-un
[:cognitect.aws.batch.UntagResourceRequest/resourceArn
:cognitect.aws.batch.UntagResourceRequest/tagKeys]))
(s/def
:cognitect.aws.batch/NodeOverrides
(s/keys
:opt-un
[:cognitect.aws.batch.NodeOverrides/numNodes
:cognitect.aws.batch.NodeOverrides/nodePropertyOverrides]))
(s/def
:cognitect.aws.batch/TagKey
(s/spec
(s/and string? #(<= 1 (count %) 128))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 128) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.batch/JobSummary
(s/keys
:req-un
[:cognitect.aws.batch.JobSummary/jobId :cognitect.aws.batch.JobSummary/jobName]
:opt-un
[:cognitect.aws.batch.JobSummary/createdAt
:cognitect.aws.batch.JobSummary/startedAt
:cognitect.aws.batch.JobSummary/nodeProperties
:cognitect.aws.batch.JobSummary/stoppedAt
:cognitect.aws.batch.JobSummary/status
:cognitect.aws.batch.JobSummary/jobArn
:cognitect.aws.batch.JobSummary/statusReason
:cognitect.aws.batch.JobSummary/arrayProperties
:cognitect.aws.batch.JobSummary/jobDefinition
:cognitect.aws.batch.JobSummary/container]))
(s/def
:cognitect.aws.batch/SchedulingPolicyDetail
(s/keys
:req-un
[:cognitect.aws.batch.SchedulingPolicyDetail/name
:cognitect.aws.batch.SchedulingPolicyDetail/arn]
:opt-un
[:cognitect.aws.batch.SchedulingPolicyDetail/tags
:cognitect.aws.batch.SchedulingPolicyDetail/fairsharePolicy]))
(s/def
:cognitect.aws.batch/GetJobQueueSnapshotRequest
(s/keys :req-un [:cognitect.aws.batch.GetJobQueueSnapshotRequest/jobQueue]))
(s/def :cognitect.aws.batch/EksMetadata (s/keys :opt-un [:cognitect.aws.batch.EksMetadata/labels]))
(s/def
:cognitect.aws.batch/SchedulingPolicyDetailList
(s/coll-of :cognitect.aws.batch/SchedulingPolicyDetail))
(s/def
:cognitect.aws.batch/ListAttemptEcsTaskDetails
(s/coll-of :cognitect.aws.batch/AttemptEcsTaskDetails))
(s/def
:cognitect.aws.batch/Ulimit
(s/keys
:req-un
[:cognitect.aws.batch.Ulimit/hardLimit
:cognitect.aws.batch.Ulimit/name
:cognitect.aws.batch.Ulimit/softLimit]))
(s/def
:cognitect.aws.batch/DeviceCgroupPermissions
(s/coll-of :cognitect.aws.batch/DeviceCgroupPermission))
(s/def
:cognitect.aws.batch/LinuxParameters
(s/keys
:opt-un
[:cognitect.aws.batch.LinuxParameters/initProcessEnabled
:cognitect.aws.batch.LinuxParameters/maxSwap
:cognitect.aws.batch.LinuxParameters/devices
:cognitect.aws.batch.LinuxParameters/tmpfs
:cognitect.aws.batch.LinuxParameters/sharedMemorySize
:cognitect.aws.batch.LinuxParameters/swappiness]))
(s/def :cognitect.aws.batch/JobSummaryList (s/coll-of :cognitect.aws.batch/JobSummary))
(s/def
:cognitect.aws.batch/ComputeResource
(s/keys
:req-un
[:cognitect.aws.batch.ComputeResource/type
:cognitect.aws.batch.ComputeResource/maxvCpus
:cognitect.aws.batch.ComputeResource/subnets]
:opt-un
[:cognitect.aws.batch.ComputeResource/ec2KeyPair
:cognitect.aws.batch.ComputeResource/spotIamFleetRole
:cognitect.aws.batch.ComputeResource/bidPercentage
:cognitect.aws.batch.ComputeResource/tags
:cognitect.aws.batch.ComputeResource/allocationStrategy
:cognitect.aws.batch.ComputeResource/launchTemplate
:cognitect.aws.batch.ComputeResource/imageId
:cognitect.aws.batch.ComputeResource/placementGroup
:cognitect.aws.batch.ComputeResource/instanceTypes
:cognitect.aws.batch.ComputeResource/ec2Configuration
:cognitect.aws.batch.ComputeResource/desiredvCpus
:cognitect.aws.batch.ComputeResource/securityGroupIds
:cognitect.aws.batch.ComputeResource/minvCpus
:cognitect.aws.batch.ComputeResource/instanceRole]))
(s/def
:cognitect.aws.batch/TagsMap
(s/map-of :cognitect.aws.batch/String :cognitect.aws.batch/String))
(s/def
:cognitect.aws.batch/TerminateJobRequest
(s/keys
:req-un
[:cognitect.aws.batch.TerminateJobRequest/jobId
:cognitect.aws.batch.TerminateJobRequest/reason]))
(s/def :cognitect.aws.batch/StringList (s/coll-of :cognitect.aws.batch/String))
(s/def
:cognitect.aws.batch/ImageIdOverride
(s/spec
(s/and string? #(<= 1 (count %) 256))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 256) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.batch/EFSAuthorizationConfigIAM
(s/spec string? :gen #(s/gen #{"DISABLED" "ENABLED"})))
(s/def
:cognitect.aws.batch/ImagePullSecret
(s/keys :req-un [:cognitect.aws.batch.ImagePullSecret/name]))
(s/def
:cognitect.aws.batch/EcsPropertiesDetail
(s/keys :opt-un [:cognitect.aws.batch.EcsPropertiesDetail/taskProperties]))
(s/def :cognitect.aws.batch/Host (s/keys :opt-un [:cognitect.aws.batch.Host/sourcePath]))
(s/def
:cognitect.aws.batch/DescribeComputeEnvironmentsRequest
(s/keys
:opt-un
[:cognitect.aws.batch.DescribeComputeEnvironmentsRequest/computeEnvironments
:cognitect.aws.batch.DescribeComputeEnvironmentsRequest/maxResults
:cognitect.aws.batch.DescribeComputeEnvironmentsRequest/nextToken]))
(s/def
:cognitect.aws.batch/FrontOfQueueJobSummaryList
(s/coll-of :cognitect.aws.batch/FrontOfQueueJobSummary))
(s/def
:cognitect.aws.batch/EksPodProperties
(s/keys
:opt-un
[:cognitect.aws.batch.EksPodProperties/serviceAccountName
:cognitect.aws.batch.EksPodProperties/hostNetwork
:cognitect.aws.batch.EksPodProperties/shareProcessNamespace
:cognitect.aws.batch.EksPodProperties/initContainers
:cognitect.aws.batch.EksPodProperties/volumes
:cognitect.aws.batch.EksPodProperties/metadata
:cognitect.aws.batch.EksPodProperties/imagePullSecrets
:cognitect.aws.batch.EksPodProperties/containers
:cognitect.aws.batch.EksPodProperties/dnsPolicy]))
(s/def
:cognitect.aws.batch/JobStateTimeLimitAction
(s/keys
:req-un
[:cognitect.aws.batch.JobStateTimeLimitAction/reason
:cognitect.aws.batch.JobStateTimeLimitAction/state
:cognitect.aws.batch.JobStateTimeLimitAction/maxTimeSeconds
:cognitect.aws.batch.JobStateTimeLimitAction/action]))
(s/def
:cognitect.aws.batch/EksContainerVolumeMounts
(s/coll-of :cognitect.aws.batch/EksContainerVolumeMount))
(s/def
:cognitect.aws.batch/NodePropertyOverride
(s/keys
:req-un
[:cognitect.aws.batch.NodePropertyOverride/targetNodes]
:opt-un
[:cognitect.aws.batch.NodePropertyOverride/eksPropertiesOverride
:cognitect.aws.batch.NodePropertyOverride/containerOverrides
:cognitect.aws.batch.NodePropertyOverride/instanceTypes
:cognitect.aws.batch.NodePropertyOverride/ecsPropertiesOverride]))
(s/def :cognitect.aws.batch/ListEcsTaskDetails (s/coll-of :cognitect.aws.batch/EcsTaskDetails))
(s/def
:cognitect.aws.batch/ComputeResourceUpdate
(s/keys
:opt-un
[:cognitect.aws.batch.ComputeResourceUpdate/ec2KeyPair
:cognitect.aws.batch.ComputeResourceUpdate/subnets
:cognitect.aws.batch.ComputeResourceUpdate/bidPercentage
:cognitect.aws.batch.ComputeResourceUpdate/tags
:cognitect.aws.batch.ComputeResourceUpdate/allocationStrategy
:cognitect.aws.batch.ComputeResourceUpdate/launchTemplate
:cognitect.aws.batch.ComputeResourceUpdate/maxvCpus
:cognitect.aws.batch.ComputeResourceUpdate/updateToLatestImageVersion
:cognitect.aws.batch.ComputeResourceUpdate/imageId
:cognitect.aws.batch.ComputeResourceUpdate/placementGroup
:cognitect.aws.batch.ComputeResourceUpdate/instanceTypes
:cognitect.aws.batch.ComputeResourceUpdate/ec2Configuration
:cognitect.aws.batch.ComputeResourceUpdate/desiredvCpus
:cognitect.aws.batch.ComputeResourceUpdate/type
:cognitect.aws.batch.ComputeResourceUpdate/securityGroupIds
:cognitect.aws.batch.ComputeResourceUpdate/minvCpus
:cognitect.aws.batch.ComputeResourceUpdate/instanceRole]))
(s/def
:cognitect.aws.batch/Quantity
(s/spec
(s/and string? #(<= 1 (count %) 256))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 256) #(gen/vector (gen/char-alpha) %))))))
(s/def :cognitect.aws.batch/RetryAction (s/spec string? :gen #(s/gen #{"RETRY" "EXIT"})))
(s/def
:cognitect.aws.batch/FairsharePolicy
(s/keys
:opt-un
[:cognitect.aws.batch.FairsharePolicy/shareDecaySeconds
:cognitect.aws.batch.FairsharePolicy/shareDistribution
:cognitect.aws.batch.FairsharePolicy/computeReservation]))
(s/def
:cognitect.aws.batch/DescribeSchedulingPoliciesResponse
(s/keys :opt-un [:cognitect.aws.batch.DescribeSchedulingPoliciesResponse/schedulingPolicies]))
(s/def :cognitect.aws.batch/JobQueueDetailList (s/coll-of :cognitect.aws.batch/JobQueueDetail))
(s/def
:cognitect.aws.batch/LogDriver
(s/spec
string?
:gen
#(s/gen #{"gelf" "json-file" "fluentd" "journald" "syslog" "splunk" "awslogs"})))
(s/def
:cognitect.aws.batch/Device
(s/keys
:req-un
[:cognitect.aws.batch.Device/hostPath]
:opt-un
[:cognitect.aws.batch.Device/permissions :cognitect.aws.batch.Device/containerPath]))
(s/def
:cognitect.aws.batch/LaunchTemplateSpecification
(s/keys
:opt-un
[:cognitect.aws.batch.LaunchTemplateSpecification/launchTemplateName
:cognitect.aws.batch.LaunchTemplateSpecification/launchTemplateId
:cognitect.aws.batch.LaunchTemplateSpecification/version]))
(s/def
:cognitect.aws.batch/EksAttemptDetail
(s/keys
:opt-un
[:cognitect.aws.batch.EksAttemptDetail/initContainers
:cognitect.aws.batch.EksAttemptDetail/startedAt
:cognitect.aws.batch.EksAttemptDetail/nodeName
:cognitect.aws.batch.EksAttemptDetail/stoppedAt
:cognitect.aws.batch.EksAttemptDetail/eksClusterArn
:cognitect.aws.batch.EksAttemptDetail/statusReason
:cognitect.aws.batch.EksAttemptDetail/containers
:cognitect.aws.batch.EksAttemptDetail/podName]))
(s/def
:cognitect.aws.batch/DescribeJobDefinitionsResponse
(s/keys
:opt-un
[:cognitect.aws.batch.DescribeJobDefinitionsResponse/jobDefinitions
:cognitect.aws.batch.DescribeJobDefinitionsResponse/nextToken]))
(s/def
:cognitect.aws.batch/CancelJobRequest
(s/keys
:req-un
[:cognitect.aws.batch.CancelJobRequest/jobId :cognitect.aws.batch.CancelJobRequest/reason]))
(s/def
:cognitect.aws.batch/AttemptContainerDetail
(s/keys
:opt-un
[:cognitect.aws.batch.AttemptContainerDetail/networkInterfaces
:cognitect.aws.batch.AttemptContainerDetail/exitCode
:cognitect.aws.batch.AttemptContainerDetail/taskArn
:cognitect.aws.batch.AttemptContainerDetail/logStreamName
:cognitect.aws.batch.AttemptContainerDetail/containerInstanceArn
:cognitect.aws.batch.AttemptContainerDetail/reason]))
(s/def
:cognitect.aws.batch/CEStatus
(s/spec string? :gen #(s/gen #{"UPDATING" "INVALID" "DELETING" "CREATING" "DELETED" "VALID"})))
(s/def
:cognitect.aws.batch/DescribeJobQueuesResponse
(s/keys
:opt-un
[:cognitect.aws.batch.DescribeJobQueuesResponse/jobQueues
:cognitect.aws.batch.DescribeJobQueuesResponse/nextToken]))
(s/def
:cognitect.aws.batch/ListTagsForResourceResponse
(s/keys :opt-un [:cognitect.aws.batch.ListTagsForResourceResponse/tags]))
(s/def
:cognitect.aws.batch/ListTaskContainerProperties
(s/coll-of :cognitect.aws.batch/TaskContainerProperties))
(s/def
:cognitect.aws.batch/DeleteComputeEnvironmentRequest
(s/keys :req-un [:cognitect.aws.batch.DeleteComputeEnvironmentRequest/computeEnvironment]))
(s/def
:cognitect.aws.batch/FrontOfQueueJobSummary
(s/keys
:opt-un
[:cognitect.aws.batch.FrontOfQueueJobSummary/earliestTimeAtPosition
:cognitect.aws.batch.FrontOfQueueJobSummary/jobArn]))
(s/def
:cognitect.aws.batch/ListTaskContainerDetails
(s/coll-of :cognitect.aws.batch/TaskContainerDetails))
(s/def
:cognitect.aws.batch/EksPropertiesDetail
(s/keys :opt-un [:cognitect.aws.batch.EksPropertiesDetail/podProperties]))
(s/def
:cognitect.aws.batch/SubmitJobResponse
(s/keys
:req-un
[:cognitect.aws.batch.SubmitJobResponse/jobName :cognitect.aws.batch.SubmitJobResponse/jobId]
:opt-un
[:cognitect.aws.batch.SubmitJobResponse/jobArn]))
(s/def
:cognitect.aws.batch/ArrayPropertiesDetail
(s/keys
:opt-un
[:cognitect.aws.batch.ArrayPropertiesDetail/statusSummary
:cognitect.aws.batch.ArrayPropertiesDetail/index
:cognitect.aws.batch.ArrayPropertiesDetail/size]))
(s/def
:cognitect.aws.batch/CRUpdateAllocationStrategy
(s/spec
string?
:gen
#(s/gen #{"SPOT_PRICE_CAPACITY_OPTIMIZED" "BEST_FIT_PROGRESSIVE" "SPOT_CAPACITY_OPTIMIZED"})))
(s/def
:cognitect.aws.batch/DeregisterJobDefinitionRequest
(s/keys :req-un [:cognitect.aws.batch.DeregisterJobDefinitionRequest/jobDefinition]))
(s/def
:cognitect.aws.batch/NodePropertiesSummary
(s/keys
:opt-un
[:cognitect.aws.batch.NodePropertiesSummary/isMainNode
:cognitect.aws.batch.NodePropertiesSummary/numNodes
:cognitect.aws.batch.NodePropertiesSummary/nodeIndex]))
(s/def :cognitect.aws.batch/String string?)
(s/def
:cognitect.aws.batch/PlatformCapabilityList
(s/coll-of :cognitect.aws.batch/PlatformCapability))
(s/def
:cognitect.aws.batch/UpdateJobQueueRequest
(s/keys
:req-un
[:cognitect.aws.batch.UpdateJobQueueRequest/jobQueue]
:opt-un
[:cognitect.aws.batch.UpdateJobQueueRequest/priority
:cognitect.aws.batch.UpdateJobQueueRequest/state
:cognitect.aws.batch.UpdateJobQueueRequest/computeEnvironmentOrder
:cognitect.aws.batch.UpdateJobQueueRequest/jobStateTimeLimitActions
:cognitect.aws.batch.UpdateJobQueueRequest/schedulingPolicyArn]))
(s/def
:cognitect.aws.batch/ArrayPropertiesSummary
(s/keys
:opt-un
[:cognitect.aws.batch.ArrayPropertiesSummary/index
:cognitect.aws.batch.ArrayPropertiesSummary/size]))
(s/def
:cognitect.aws.batch/GetJobQueueSnapshotResponse
(s/keys :opt-un [:cognitect.aws.batch.GetJobQueueSnapshotResponse/frontOfQueue]))
(s/def :cognitect.aws.batch.EFSVolumeConfiguration/fileSystemId :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EFSVolumeConfiguration/rootDirectory :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.EFSVolumeConfiguration/transitEncryption
:cognitect.aws.batch/EFSTransitEncryption)
(s/def
:cognitect.aws.batch.EFSVolumeConfiguration/transitEncryptionPort
:cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.EFSVolumeConfiguration/authorizationConfig
:cognitect.aws.batch/EFSAuthorizationConfig)
(s/def
:cognitect.aws.batch.TaskPropertiesOverride/containers
:cognitect.aws.batch/ListTaskContainerOverrides)
(s/def :cognitect.aws.batch.TagResourceRequest/resourceArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.TagResourceRequest/tags :cognitect.aws.batch/TagrisTagsMap)
(s/def :cognitect.aws.batch.EksConfiguration/eksClusterArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksConfiguration/kubernetesNamespace :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobQueueDetail/tags :cognitect.aws.batch/TagrisTagsMap)
(s/def :cognitect.aws.batch.JobQueueDetail/jobQueueName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobQueueDetail/schedulingPolicyArn :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.JobQueueDetail/computeEnvironmentOrder
:cognitect.aws.batch/ComputeEnvironmentOrders)
(s/def
:cognitect.aws.batch.JobQueueDetail/jobStateTimeLimitActions
:cognitect.aws.batch/JobStateTimeLimitActions)
(s/def :cognitect.aws.batch.JobQueueDetail/statusReason :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobQueueDetail/state :cognitect.aws.batch/JQState)
(s/def :cognitect.aws.batch.JobQueueDetail/priority :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.JobQueueDetail/status :cognitect.aws.batch/JQStatus)
(s/def :cognitect.aws.batch.JobQueueDetail/jobQueueArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.CreateJobQueueResponse/jobQueueName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.CreateJobQueueResponse/jobQueueArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.NodeProperties/numNodes :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.NodeProperties/mainNode :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.NodeProperties/nodeRangeProperties
:cognitect.aws.batch/NodeRangeProperties)
(s/def :cognitect.aws.batch.EksContainerOverride/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksContainerOverride/image :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksContainerOverride/command :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.EksContainerOverride/args :cognitect.aws.batch/StringList)
(s/def
:cognitect.aws.batch.EksContainerOverride/env
:cognitect.aws.batch/EksContainerEnvironmentVariables)
(s/def
:cognitect.aws.batch.EksContainerOverride/resources
:cognitect.aws.batch/EksContainerResourceRequirements)
(s/def :cognitect.aws.batch.EksContainerResourceRequirements/limits :cognitect.aws.batch/EksLimits)
(s/def
:cognitect.aws.batch.EksContainerResourceRequirements/requests
:cognitect.aws.batch/EksRequests)
(s/def :cognitect.aws.batch.DescribeJobsRequest/jobs :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.AttemptTaskContainerDetails/exitCode :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.AttemptTaskContainerDetails/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.AttemptTaskContainerDetails/reason :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.AttemptTaskContainerDetails/logStreamName :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.AttemptTaskContainerDetails/networkInterfaces
:cognitect.aws.batch/NetworkInterfaceList)
(s/def :cognitect.aws.batch.FrontOfQueueDetail/jobs :cognitect.aws.batch/FrontOfQueueJobSummaryList)
(s/def :cognitect.aws.batch.FrontOfQueueDetail/lastUpdatedAt :cognitect.aws.batch/Long)
(s/def :cognitect.aws.batch.ShareAttributes/shareIdentifier :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ShareAttributes/weightFactor :cognitect.aws.batch/Float)
(s/def :cognitect.aws.batch.ListJobsResponse/jobSummaryList :cognitect.aws.batch/JobSummaryList)
(s/def :cognitect.aws.batch.ListJobsResponse/nextToken :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ContainerProperties/vcpus :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.ContainerProperties/logConfiguration
:cognitect.aws.batch/LogConfiguration)
(s/def :cognitect.aws.batch.ContainerProperties/privileged :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.ContainerProperties/ulimits :cognitect.aws.batch/Ulimits)
(s/def :cognitect.aws.batch.ContainerProperties/instanceType :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.ContainerProperties/networkConfiguration
:cognitect.aws.batch/NetworkConfiguration)
(s/def
:cognitect.aws.batch.ContainerProperties/ephemeralStorage
:cognitect.aws.batch/EphemeralStorage)
(s/def :cognitect.aws.batch.ContainerProperties/mountPoints :cognitect.aws.batch/MountPoints)
(s/def :cognitect.aws.batch.ContainerProperties/secrets :cognitect.aws.batch/SecretList)
(s/def :cognitect.aws.batch.ContainerProperties/memory :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ContainerProperties/command :cognitect.aws.batch/StringList)
(s/def
:cognitect.aws.batch.ContainerProperties/runtimePlatform
:cognitect.aws.batch/RuntimePlatform)
(s/def
:cognitect.aws.batch.ContainerProperties/linuxParameters
:cognitect.aws.batch/LinuxParameters)
(s/def :cognitect.aws.batch.ContainerProperties/volumes :cognitect.aws.batch/Volumes)
(s/def :cognitect.aws.batch.ContainerProperties/image :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ContainerProperties/readonlyRootFilesystem :cognitect.aws.batch/Boolean)
(s/def
:cognitect.aws.batch.ContainerProperties/environment
:cognitect.aws.batch/EnvironmentVariables)
(s/def :cognitect.aws.batch.ContainerProperties/jobRoleArn :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.ContainerProperties/resourceRequirements
:cognitect.aws.batch/ResourceRequirements)
(s/def :cognitect.aws.batch.ContainerProperties/user :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.ContainerProperties/repositoryCredentials
:cognitect.aws.batch/RepositoryCredentials)
(s/def
:cognitect.aws.batch.ContainerProperties/fargatePlatformConfiguration
:cognitect.aws.batch/FargatePlatformConfiguration)
(s/def :cognitect.aws.batch.ContainerProperties/executionRoleArn :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.EcsPropertiesOverride/taskProperties
:cognitect.aws.batch/ListTaskPropertiesOverride)
(s/def :cognitect.aws.batch.CreateComputeEnvironmentRequest/tags :cognitect.aws.batch/TagrisTagsMap)
(s/def
:cognitect.aws.batch.CreateComputeEnvironmentRequest/computeResources
:cognitect.aws.batch/ComputeResource)
(s/def
:cognitect.aws.batch.CreateComputeEnvironmentRequest/unmanagedvCpus
:cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.CreateComputeEnvironmentRequest/type :cognitect.aws.batch/CEType)
(s/def :cognitect.aws.batch.CreateComputeEnvironmentRequest/state :cognitect.aws.batch/CEState)
(s/def :cognitect.aws.batch.CreateComputeEnvironmentRequest/serviceRole :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.CreateComputeEnvironmentRequest/eksConfiguration
:cognitect.aws.batch/EksConfiguration)
(s/def :cognitect.aws.batch.CreateComputeEnvironmentRequest/context :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.CreateComputeEnvironmentRequest/computeEnvironmentName
:cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.LogConfiguration/logDriver :cognitect.aws.batch/LogDriver)
(s/def
:cognitect.aws.batch.LogConfiguration/options
:cognitect.aws.batch/LogConfigurationOptionsMap)
(s/def :cognitect.aws.batch.LogConfiguration/secretOptions :cognitect.aws.batch/SecretList)
(s/def :cognitect.aws.batch.CreateSchedulingPolicyRequest/name :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.CreateSchedulingPolicyRequest/fairsharePolicy
:cognitect.aws.batch/FairsharePolicy)
(s/def :cognitect.aws.batch.CreateSchedulingPolicyRequest/tags :cognitect.aws.batch/TagrisTagsMap)
(s/def :cognitect.aws.batch.AttemptDetail/container :cognitect.aws.batch/AttemptContainerDetail)
(s/def :cognitect.aws.batch.AttemptDetail/startedAt :cognitect.aws.batch/Long)
(s/def :cognitect.aws.batch.AttemptDetail/stoppedAt :cognitect.aws.batch/Long)
(s/def :cognitect.aws.batch.AttemptDetail/statusReason :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.AttemptDetail/taskProperties
:cognitect.aws.batch/ListAttemptEcsTaskDetails)
(s/def :cognitect.aws.batch.EvaluateOnExit/onStatusReason :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EvaluateOnExit/onReason :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EvaluateOnExit/onExitCode :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EvaluateOnExit/action :cognitect.aws.batch/RetryAction)
(s/def :cognitect.aws.batch.JobDependency/jobId :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobDependency/type :cognitect.aws.batch/ArrayJobDependency)
(s/def :cognitect.aws.batch.KeyValuePair/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.KeyValuePair/value :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.RetryStrategy/attempts :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.RetryStrategy/evaluateOnExit :cognitect.aws.batch/EvaluateOnExitList)
(s/def :cognitect.aws.batch.EksEmptyDir/medium :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksEmptyDir/sizeLimit :cognitect.aws.batch/Quantity)
(s/def
:cognitect.aws.batch.CreateComputeEnvironmentResponse/computeEnvironmentName
:cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.CreateComputeEnvironmentResponse/computeEnvironmentArn
:cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.DescribeJobsResponse/jobs :cognitect.aws.batch/JobDetailList)
(s/def
:cognitect.aws.batch.EksPropertiesOverride/podProperties
:cognitect.aws.batch/EksPodPropertiesOverride)
(s/def
:cognitect.aws.batch.SubmitJobRequest/containerOverrides
:cognitect.aws.batch/ContainerOverrides)
(s/def :cognitect.aws.batch.SubmitJobRequest/tags :cognitect.aws.batch/TagrisTagsMap)
(s/def
:cognitect.aws.batch.SubmitJobRequest/schedulingPriorityOverride
:cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.SubmitJobRequest/jobName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.SubmitJobRequest/arrayProperties :cognitect.aws.batch/ArrayProperties)
(s/def :cognitect.aws.batch.SubmitJobRequest/dependsOn :cognitect.aws.batch/JobDependencyList)
(s/def
:cognitect.aws.batch.SubmitJobRequest/ecsPropertiesOverride
:cognitect.aws.batch/EcsPropertiesOverride)
(s/def :cognitect.aws.batch.SubmitJobRequest/jobDefinition :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.SubmitJobRequest/shareIdentifier :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.SubmitJobRequest/propagateTags :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.SubmitJobRequest/jobQueue :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.SubmitJobRequest/nodeOverrides :cognitect.aws.batch/NodeOverrides)
(s/def :cognitect.aws.batch.SubmitJobRequest/timeout :cognitect.aws.batch/JobTimeout)
(s/def :cognitect.aws.batch.SubmitJobRequest/parameters :cognitect.aws.batch/ParametersMap)
(s/def
:cognitect.aws.batch.SubmitJobRequest/eksPropertiesOverride
:cognitect.aws.batch/EksPropertiesOverride)
(s/def :cognitect.aws.batch.SubmitJobRequest/retryStrategy :cognitect.aws.batch/RetryStrategy)
(s/def :cognitect.aws.batch.EphemeralStorage/sizeInGiB :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.UpdateSchedulingPolicyRequest/arn :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.UpdateSchedulingPolicyRequest/fairsharePolicy
:cognitect.aws.batch/FairsharePolicy)
(s/def :cognitect.aws.batch.DescribeSchedulingPoliciesRequest/arns :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.NodeRangeProperty/targetNodes :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.NodeRangeProperty/container :cognitect.aws.batch/ContainerProperties)
(s/def :cognitect.aws.batch.NodeRangeProperty/instanceTypes :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.NodeRangeProperty/ecsProperties :cognitect.aws.batch/EcsProperties)
(s/def :cognitect.aws.batch.NodeRangeProperty/eksProperties :cognitect.aws.batch/EksProperties)
(s/def :cognitect.aws.batch.EksContainerDetail/args :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.EksContainerDetail/exitCode :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.EksContainerDetail/securityContext
:cognitect.aws.batch/EksContainerSecurityContext)
(s/def
:cognitect.aws.batch.EksContainerDetail/volumeMounts
:cognitect.aws.batch/EksContainerVolumeMounts)
(s/def :cognitect.aws.batch.EksContainerDetail/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksContainerDetail/command :cognitect.aws.batch/StringList)
(s/def
:cognitect.aws.batch.EksContainerDetail/env
:cognitect.aws.batch/EksContainerEnvironmentVariables)
(s/def :cognitect.aws.batch.EksContainerDetail/reason :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksContainerDetail/imagePullPolicy :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksContainerDetail/image :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.EksContainerDetail/resources
:cognitect.aws.batch/EksContainerResourceRequirements)
(s/def :cognitect.aws.batch.JobTimeout/attemptDurationSeconds :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.EcsTaskDetails/platformVersion :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EcsTaskDetails/pidMode :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.EcsTaskDetails/networkConfiguration
:cognitect.aws.batch/NetworkConfiguration)
(s/def :cognitect.aws.batch.EcsTaskDetails/ephemeralStorage :cognitect.aws.batch/EphemeralStorage)
(s/def :cognitect.aws.batch.EcsTaskDetails/runtimePlatform :cognitect.aws.batch/RuntimePlatform)
(s/def :cognitect.aws.batch.EcsTaskDetails/containers :cognitect.aws.batch/ListTaskContainerDetails)
(s/def :cognitect.aws.batch.EcsTaskDetails/taskArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EcsTaskDetails/taskRoleArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EcsTaskDetails/volumes :cognitect.aws.batch/Volumes)
(s/def :cognitect.aws.batch.EcsTaskDetails/containerInstanceArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EcsTaskDetails/executionRoleArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EcsTaskDetails/ipcMode :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.MountPoint/containerPath :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.MountPoint/readOnly :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.MountPoint/sourceVolume :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.NetworkInterface/attachmentId :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.NetworkInterface/ipv6Address :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.NetworkInterface/privateIpv4Address :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ListJobsRequest/jobQueue :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ListJobsRequest/arrayJobId :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ListJobsRequest/multiNodeJobId :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ListJobsRequest/jobStatus :cognitect.aws.batch/JobStatus)
(s/def :cognitect.aws.batch.ListJobsRequest/maxResults :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ListJobsRequest/nextToken :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ListJobsRequest/filters :cognitect.aws.batch/ListJobsFilterList)
(s/def :cognitect.aws.batch.DescribeJobQueuesRequest/jobQueues :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.DescribeJobQueuesRequest/maxResults :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.DescribeJobQueuesRequest/nextToken :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ContainerOverrides/vcpus :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ContainerOverrides/memory :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ContainerOverrides/command :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.ContainerOverrides/instanceType :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.ContainerOverrides/environment
:cognitect.aws.batch/EnvironmentVariables)
(s/def
:cognitect.aws.batch.ContainerOverrides/resourceRequirements
:cognitect.aws.batch/ResourceRequirements)
(s/def :cognitect.aws.batch.SchedulingPolicyListingDetail/arn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.NodeDetails/nodeIndex :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.NodeDetails/isMainNode :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.RuntimePlatform/operatingSystemFamily :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.RuntimePlatform/cpuArchitecture :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.Tmpfs/containerPath :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.Tmpfs/size :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.Tmpfs/mountOptions :cognitect.aws.batch/StringList)
(s/def
:cognitect.aws.batch.ListSchedulingPoliciesResponse/schedulingPolicies
:cognitect.aws.batch/SchedulingPolicyListingDetailList)
(s/def :cognitect.aws.batch.ListSchedulingPoliciesResponse/nextToken :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ContainerDetail/exitCode :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ContainerDetail/vcpus :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ContainerDetail/logConfiguration :cognitect.aws.batch/LogConfiguration)
(s/def :cognitect.aws.batch.ContainerDetail/privileged :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.ContainerDetail/ulimits :cognitect.aws.batch/Ulimits)
(s/def :cognitect.aws.batch.ContainerDetail/instanceType :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.ContainerDetail/networkConfiguration
:cognitect.aws.batch/NetworkConfiguration)
(s/def :cognitect.aws.batch.ContainerDetail/ephemeralStorage :cognitect.aws.batch/EphemeralStorage)
(s/def :cognitect.aws.batch.ContainerDetail/mountPoints :cognitect.aws.batch/MountPoints)
(s/def :cognitect.aws.batch.ContainerDetail/secrets :cognitect.aws.batch/SecretList)
(s/def :cognitect.aws.batch.ContainerDetail/memory :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ContainerDetail/command :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.ContainerDetail/runtimePlatform :cognitect.aws.batch/RuntimePlatform)
(s/def :cognitect.aws.batch.ContainerDetail/taskArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ContainerDetail/linuxParameters :cognitect.aws.batch/LinuxParameters)
(s/def :cognitect.aws.batch.ContainerDetail/volumes :cognitect.aws.batch/Volumes)
(s/def :cognitect.aws.batch.ContainerDetail/containerInstanceArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ContainerDetail/reason :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ContainerDetail/logStreamName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ContainerDetail/image :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ContainerDetail/readonlyRootFilesystem :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.ContainerDetail/environment :cognitect.aws.batch/EnvironmentVariables)
(s/def
:cognitect.aws.batch.ContainerDetail/networkInterfaces
:cognitect.aws.batch/NetworkInterfaceList)
(s/def :cognitect.aws.batch.ContainerDetail/jobRoleArn :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.ContainerDetail/resourceRequirements
:cognitect.aws.batch/ResourceRequirements)
(s/def :cognitect.aws.batch.ContainerDetail/user :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.ContainerDetail/repositoryCredentials
:cognitect.aws.batch/RepositoryCredentials)
(s/def
:cognitect.aws.batch.ContainerDetail/fargatePlatformConfiguration
:cognitect.aws.batch/FargatePlatformConfiguration)
(s/def :cognitect.aws.batch.ContainerDetail/executionRoleArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.CreateSchedulingPolicyResponse/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.CreateSchedulingPolicyResponse/arn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.DeleteSchedulingPolicyRequest/arn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ListSchedulingPoliciesRequest/maxResults :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ListSchedulingPoliciesRequest/nextToken :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.EksPodPropertiesDetail/imagePullSecrets
:cognitect.aws.batch/ImagePullSecrets)
(s/def :cognitect.aws.batch.EksPodPropertiesDetail/hostNetwork :cognitect.aws.batch/Boolean)
(s/def
:cognitect.aws.batch.EksPodPropertiesDetail/containers
:cognitect.aws.batch/EksContainerDetails)
(s/def
:cognitect.aws.batch.EksPodPropertiesDetail/shareProcessNamespace
:cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.EksPodPropertiesDetail/volumes :cognitect.aws.batch/EksVolumes)
(s/def :cognitect.aws.batch.EksPodPropertiesDetail/nodeName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksPodPropertiesDetail/dnsPolicy :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.EksPodPropertiesDetail/initContainers
:cognitect.aws.batch/EksContainerDetails)
(s/def :cognitect.aws.batch.EksPodPropertiesDetail/serviceAccountName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksPodPropertiesDetail/metadata :cognitect.aws.batch/EksMetadata)
(s/def :cognitect.aws.batch.EksPodPropertiesDetail/podName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.UpdatePolicy/terminateJobsOnUpdate :cognitect.aws.batch/Boolean)
(s/def
:cognitect.aws.batch.UpdatePolicy/jobExecutionTimeoutMinutes
:cognitect.aws.batch/JobExecutionTimeoutMinutes)
(s/def :cognitect.aws.batch.TaskContainerOverrides/command :cognitect.aws.batch/StringList)
(s/def
:cognitect.aws.batch.TaskContainerOverrides/environment
:cognitect.aws.batch/EnvironmentVariables)
(s/def :cognitect.aws.batch.TaskContainerOverrides/name :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.TaskContainerOverrides/resourceRequirements
:cognitect.aws.batch/ResourceRequirements)
(s/def :cognitect.aws.batch.EksContainerVolumeMount/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksContainerVolumeMount/mountPath :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksContainerVolumeMount/readOnly :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.TaskContainerDetails/exitCode :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.TaskContainerDetails/logConfiguration
:cognitect.aws.batch/LogConfiguration)
(s/def :cognitect.aws.batch.TaskContainerDetails/privileged :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.TaskContainerDetails/ulimits :cognitect.aws.batch/Ulimits)
(s/def
:cognitect.aws.batch.TaskContainerDetails/dependsOn
:cognitect.aws.batch/TaskContainerDependencyList)
(s/def :cognitect.aws.batch.TaskContainerDetails/mountPoints :cognitect.aws.batch/MountPoints)
(s/def :cognitect.aws.batch.TaskContainerDetails/secrets :cognitect.aws.batch/SecretList)
(s/def :cognitect.aws.batch.TaskContainerDetails/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.TaskContainerDetails/command :cognitect.aws.batch/StringList)
(s/def
:cognitect.aws.batch.TaskContainerDetails/linuxParameters
:cognitect.aws.batch/LinuxParameters)
(s/def :cognitect.aws.batch.TaskContainerDetails/reason :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.TaskContainerDetails/logStreamName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.TaskContainerDetails/image :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.TaskContainerDetails/readonlyRootFilesystem
:cognitect.aws.batch/Boolean)
(s/def
:cognitect.aws.batch.TaskContainerDetails/environment
:cognitect.aws.batch/EnvironmentVariables)
(s/def
:cognitect.aws.batch.TaskContainerDetails/networkInterfaces
:cognitect.aws.batch/NetworkInterfaceList)
(s/def :cognitect.aws.batch.TaskContainerDetails/essential :cognitect.aws.batch/Boolean)
(s/def
:cognitect.aws.batch.TaskContainerDetails/resourceRequirements
:cognitect.aws.batch/ResourceRequirements)
(s/def :cognitect.aws.batch.TaskContainerDetails/user :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.TaskContainerDetails/repositoryCredentials
:cognitect.aws.batch/RepositoryCredentials)
(s/def :cognitect.aws.batch.AttemptEcsTaskDetails/containerInstanceArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.AttemptEcsTaskDetails/taskArn :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.AttemptEcsTaskDetails/containers
:cognitect.aws.batch/ListAttemptTaskContainerDetails)
(s/def :cognitect.aws.batch.Secret/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.Secret/valueFrom :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksVolume/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksVolume/hostPath :cognitect.aws.batch/EksHostPath)
(s/def :cognitect.aws.batch.EksVolume/emptyDir :cognitect.aws.batch/EksEmptyDir)
(s/def :cognitect.aws.batch.EksVolume/secret :cognitect.aws.batch/EksSecret)
(s/def :cognitect.aws.batch.DeleteJobQueueRequest/jobQueue :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobDefinition/tags :cognitect.aws.batch/TagrisTagsMap)
(s/def :cognitect.aws.batch.JobDefinition/ecsProperties :cognitect.aws.batch/EcsProperties)
(s/def :cognitect.aws.batch.JobDefinition/revision :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.JobDefinition/eksProperties :cognitect.aws.batch/EksProperties)
(s/def :cognitect.aws.batch.JobDefinition/type :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobDefinition/jobDefinitionName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobDefinition/nodeProperties :cognitect.aws.batch/NodeProperties)
(s/def
:cognitect.aws.batch.JobDefinition/containerOrchestrationType
:cognitect.aws.batch/OrchestrationType)
(s/def :cognitect.aws.batch.JobDefinition/propagateTags :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.JobDefinition/status :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobDefinition/jobDefinitionArn :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.JobDefinition/containerProperties
:cognitect.aws.batch/ContainerProperties)
(s/def :cognitect.aws.batch.JobDefinition/schedulingPriority :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.JobDefinition/timeout :cognitect.aws.batch/JobTimeout)
(s/def
:cognitect.aws.batch.JobDefinition/platformCapabilities
:cognitect.aws.batch/PlatformCapabilityList)
(s/def :cognitect.aws.batch.JobDefinition/parameters :cognitect.aws.batch/ParametersMap)
(s/def :cognitect.aws.batch.JobDefinition/retryStrategy :cognitect.aws.batch/RetryStrategy)
(s/def :cognitect.aws.batch.EksHostPath/path :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.Volume/host :cognitect.aws.batch/Host)
(s/def :cognitect.aws.batch.Volume/name :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.Volume/efsVolumeConfiguration
:cognitect.aws.batch/EFSVolumeConfiguration)
(s/def
:cognitect.aws.batch.UpdateComputeEnvironmentResponse/computeEnvironmentName
:cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.UpdateComputeEnvironmentResponse/computeEnvironmentArn
:cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ListTagsForResourceRequest/resourceArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ArrayProperties/size :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ContainerSummary/exitCode :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ContainerSummary/reason :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.KeyValuesPair/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.KeyValuesPair/values :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.EksContainer/args :cognitect.aws.batch/StringList)
(s/def
:cognitect.aws.batch.EksContainer/securityContext
:cognitect.aws.batch/EksContainerSecurityContext)
(s/def :cognitect.aws.batch.EksContainer/volumeMounts :cognitect.aws.batch/EksContainerVolumeMounts)
(s/def :cognitect.aws.batch.EksContainer/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksContainer/command :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.EksContainer/env :cognitect.aws.batch/EksContainerEnvironmentVariables)
(s/def :cognitect.aws.batch.EksContainer/imagePullPolicy :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksContainer/image :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.EksContainer/resources
:cognitect.aws.batch/EksContainerResourceRequirements)
(s/def :cognitect.aws.batch.ResourceRequirement/value :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ResourceRequirement/type :cognitect.aws.batch/ResourceType)
(s/def :cognitect.aws.batch.ComputeEnvironmentDetail/tags :cognitect.aws.batch/TagrisTagsMap)
(s/def :cognitect.aws.batch.ComputeEnvironmentDetail/updatePolicy :cognitect.aws.batch/UpdatePolicy)
(s/def
:cognitect.aws.batch.ComputeEnvironmentDetail/computeResources
:cognitect.aws.batch/ComputeResource)
(s/def :cognitect.aws.batch.ComputeEnvironmentDetail/unmanagedvCpus :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ComputeEnvironmentDetail/type :cognitect.aws.batch/CEType)
(s/def :cognitect.aws.batch.ComputeEnvironmentDetail/statusReason :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ComputeEnvironmentDetail/state :cognitect.aws.batch/CEState)
(s/def :cognitect.aws.batch.ComputeEnvironmentDetail/serviceRole :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.ComputeEnvironmentDetail/containerOrchestrationType
:cognitect.aws.batch/OrchestrationType)
(s/def
:cognitect.aws.batch.ComputeEnvironmentDetail/eksConfiguration
:cognitect.aws.batch/EksConfiguration)
(s/def :cognitect.aws.batch.ComputeEnvironmentDetail/status :cognitect.aws.batch/CEStatus)
(s/def
:cognitect.aws.batch.ComputeEnvironmentDetail/computeEnvironmentArn
:cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ComputeEnvironmentDetail/ecsClusterArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ComputeEnvironmentDetail/context :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ComputeEnvironmentDetail/uuid :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.ComputeEnvironmentDetail/computeEnvironmentName
:cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ComputeEnvironmentOrder/order :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ComputeEnvironmentOrder/computeEnvironment :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.NetworkConfiguration/assignPublicIp :cognitect.aws.batch/AssignPublicIp)
(s/def :cognitect.aws.batch.EksContainerSecurityContext/runAsUser :cognitect.aws.batch/Long)
(s/def :cognitect.aws.batch.EksContainerSecurityContext/runAsGroup :cognitect.aws.batch/Long)
(s/def :cognitect.aws.batch.EksContainerSecurityContext/privileged :cognitect.aws.batch/Boolean)
(s/def
:cognitect.aws.batch.EksContainerSecurityContext/allowPrivilegeEscalation
:cognitect.aws.batch/Boolean)
(s/def
:cognitect.aws.batch.EksContainerSecurityContext/readOnlyRootFilesystem
:cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.EksContainerSecurityContext/runAsNonRoot :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.JobDetail/tags :cognitect.aws.batch/TagrisTagsMap)
(s/def :cognitect.aws.batch.JobDetail/startedAt :cognitect.aws.batch/Long)
(s/def :cognitect.aws.batch.JobDetail/ecsProperties :cognitect.aws.batch/EcsPropertiesDetail)
(s/def :cognitect.aws.batch.JobDetail/stoppedAt :cognitect.aws.batch/Long)
(s/def :cognitect.aws.batch.JobDetail/nodeDetails :cognitect.aws.batch/NodeDetails)
(s/def :cognitect.aws.batch.JobDetail/jobName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobDetail/arrayProperties :cognitect.aws.batch/ArrayPropertiesDetail)
(s/def :cognitect.aws.batch.JobDetail/eksProperties :cognitect.aws.batch/EksPropertiesDetail)
(s/def :cognitect.aws.batch.JobDetail/dependsOn :cognitect.aws.batch/JobDependencyList)
(s/def :cognitect.aws.batch.JobDetail/attempts :cognitect.aws.batch/AttemptDetails)
(s/def :cognitect.aws.batch.JobDetail/createdAt :cognitect.aws.batch/Long)
(s/def :cognitect.aws.batch.JobDetail/statusReason :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobDetail/isCancelled :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.JobDetail/nodeProperties :cognitect.aws.batch/NodeProperties)
(s/def :cognitect.aws.batch.JobDetail/jobDefinition :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobDetail/shareIdentifier :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobDetail/propagateTags :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.JobDetail/status :cognitect.aws.batch/JobStatus)
(s/def :cognitect.aws.batch.JobDetail/schedulingPriority :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.JobDetail/jobQueue :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobDetail/isTerminated :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.JobDetail/eksAttempts :cognitect.aws.batch/EksAttemptDetails)
(s/def :cognitect.aws.batch.JobDetail/container :cognitect.aws.batch/ContainerDetail)
(s/def :cognitect.aws.batch.JobDetail/jobArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobDetail/timeout :cognitect.aws.batch/JobTimeout)
(s/def :cognitect.aws.batch.JobDetail/jobId :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.JobDetail/platformCapabilities
:cognitect.aws.batch/PlatformCapabilityList)
(s/def :cognitect.aws.batch.JobDetail/parameters :cognitect.aws.batch/ParametersMap)
(s/def :cognitect.aws.batch.JobDetail/retryStrategy :cognitect.aws.batch/RetryStrategy)
(s/def
:cognitect.aws.batch.TaskContainerProperties/logConfiguration
:cognitect.aws.batch/LogConfiguration)
(s/def :cognitect.aws.batch.TaskContainerProperties/privileged :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.TaskContainerProperties/ulimits :cognitect.aws.batch/Ulimits)
(s/def
:cognitect.aws.batch.TaskContainerProperties/dependsOn
:cognitect.aws.batch/TaskContainerDependencyList)
(s/def :cognitect.aws.batch.TaskContainerProperties/mountPoints :cognitect.aws.batch/MountPoints)
(s/def :cognitect.aws.batch.TaskContainerProperties/secrets :cognitect.aws.batch/SecretList)
(s/def :cognitect.aws.batch.TaskContainerProperties/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.TaskContainerProperties/command :cognitect.aws.batch/StringList)
(s/def
:cognitect.aws.batch.TaskContainerProperties/linuxParameters
:cognitect.aws.batch/LinuxParameters)
(s/def :cognitect.aws.batch.TaskContainerProperties/image :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.TaskContainerProperties/readonlyRootFilesystem
:cognitect.aws.batch/Boolean)
(s/def
:cognitect.aws.batch.TaskContainerProperties/environment
:cognitect.aws.batch/EnvironmentVariables)
(s/def :cognitect.aws.batch.TaskContainerProperties/essential :cognitect.aws.batch/Boolean)
(s/def
:cognitect.aws.batch.TaskContainerProperties/resourceRequirements
:cognitect.aws.batch/ResourceRequirements)
(s/def :cognitect.aws.batch.TaskContainerProperties/user :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.TaskContainerProperties/repositoryCredentials
:cognitect.aws.batch/RepositoryCredentials)
(s/def :cognitect.aws.batch.CreateJobQueueRequest/jobQueueName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.CreateJobQueueRequest/state :cognitect.aws.batch/JQState)
(s/def :cognitect.aws.batch.CreateJobQueueRequest/schedulingPolicyArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.CreateJobQueueRequest/priority :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.CreateJobQueueRequest/computeEnvironmentOrder
:cognitect.aws.batch/ComputeEnvironmentOrders)
(s/def :cognitect.aws.batch.CreateJobQueueRequest/tags :cognitect.aws.batch/TagrisTagsMap)
(s/def
:cognitect.aws.batch.CreateJobQueueRequest/jobStateTimeLimitActions
:cognitect.aws.batch/JobStateTimeLimitActions)
(s/def :cognitect.aws.batch.Ec2Configuration/imageType :cognitect.aws.batch/ImageType)
(s/def :cognitect.aws.batch.Ec2Configuration/imageIdOverride :cognitect.aws.batch/ImageIdOverride)
(s/def
:cognitect.aws.batch.Ec2Configuration/imageKubernetesVersion
:cognitect.aws.batch/KubernetesVersion)
(s/def
:cognitect.aws.batch.EksPodPropertiesOverride/containers
:cognitect.aws.batch/EksContainerOverrideList)
(s/def
:cognitect.aws.batch.EksPodPropertiesOverride/initContainers
:cognitect.aws.batch/EksContainerOverrideList)
(s/def :cognitect.aws.batch.EksPodPropertiesOverride/metadata :cognitect.aws.batch/EksMetadata)
(s/def :cognitect.aws.batch.RepositoryCredentials/credentialsParameter :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.DescribeJobDefinitionsRequest/jobDefinitions
:cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.DescribeJobDefinitionsRequest/maxResults :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.DescribeJobDefinitionsRequest/jobDefinitionName
:cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.DescribeJobDefinitionsRequest/status :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.DescribeJobDefinitionsRequest/nextToken :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksSecret/secretName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksSecret/optional :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.EFSAuthorizationConfig/accessPointId :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.EFSAuthorizationConfig/iam
:cognitect.aws.batch/EFSAuthorizationConfigIAM)
(s/def :cognitect.aws.batch.RegisterJobDefinitionRequest/tags :cognitect.aws.batch/TagrisTagsMap)
(s/def
:cognitect.aws.batch.RegisterJobDefinitionRequest/ecsProperties
:cognitect.aws.batch/EcsProperties)
(s/def
:cognitect.aws.batch.RegisterJobDefinitionRequest/eksProperties
:cognitect.aws.batch/EksProperties)
(s/def
:cognitect.aws.batch.RegisterJobDefinitionRequest/type
:cognitect.aws.batch/JobDefinitionType)
(s/def
:cognitect.aws.batch.RegisterJobDefinitionRequest/jobDefinitionName
:cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.RegisterJobDefinitionRequest/nodeProperties
:cognitect.aws.batch/NodeProperties)
(s/def :cognitect.aws.batch.RegisterJobDefinitionRequest/propagateTags :cognitect.aws.batch/Boolean)
(s/def
:cognitect.aws.batch.RegisterJobDefinitionRequest/containerProperties
:cognitect.aws.batch/ContainerProperties)
(s/def
:cognitect.aws.batch.RegisterJobDefinitionRequest/schedulingPriority
:cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.RegisterJobDefinitionRequest/timeout :cognitect.aws.batch/JobTimeout)
(s/def
:cognitect.aws.batch.RegisterJobDefinitionRequest/platformCapabilities
:cognitect.aws.batch/PlatformCapabilityList)
(s/def
:cognitect.aws.batch.RegisterJobDefinitionRequest/parameters
:cognitect.aws.batch/ParametersMap)
(s/def
:cognitect.aws.batch.RegisterJobDefinitionRequest/retryStrategy
:cognitect.aws.batch/RetryStrategy)
(s/def :cognitect.aws.batch.EksContainerEnvironmentVariable/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksContainerEnvironmentVariable/value :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.TaskContainerDependency/containerName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.TaskContainerDependency/condition :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EcsTaskProperties/platformVersion :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EcsTaskProperties/pidMode :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.EcsTaskProperties/networkConfiguration
:cognitect.aws.batch/NetworkConfiguration)
(s/def
:cognitect.aws.batch.EcsTaskProperties/ephemeralStorage
:cognitect.aws.batch/EphemeralStorage)
(s/def :cognitect.aws.batch.EcsTaskProperties/runtimePlatform :cognitect.aws.batch/RuntimePlatform)
(s/def
:cognitect.aws.batch.EcsTaskProperties/containers
:cognitect.aws.batch/ListTaskContainerProperties)
(s/def :cognitect.aws.batch.EcsTaskProperties/taskRoleArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EcsTaskProperties/volumes :cognitect.aws.batch/Volumes)
(s/def :cognitect.aws.batch.EcsTaskProperties/executionRoleArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EcsTaskProperties/ipcMode :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EcsProperties/taskProperties :cognitect.aws.batch/ListEcsTaskProperties)
(s/def
:cognitect.aws.batch.FargatePlatformConfiguration/platformVersion
:cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.DescribeComputeEnvironmentsResponse/computeEnvironments
:cognitect.aws.batch/ComputeEnvironmentDetailList)
(s/def
:cognitect.aws.batch.DescribeComputeEnvironmentsResponse/nextToken
:cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.UpdateComputeEnvironmentRequest/computeEnvironment
:cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.UpdateComputeEnvironmentRequest/state :cognitect.aws.batch/CEState)
(s/def
:cognitect.aws.batch.UpdateComputeEnvironmentRequest/unmanagedvCpus
:cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.UpdateComputeEnvironmentRequest/computeResources
:cognitect.aws.batch/ComputeResourceUpdate)
(s/def :cognitect.aws.batch.UpdateComputeEnvironmentRequest/serviceRole :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.UpdateComputeEnvironmentRequest/updatePolicy
:cognitect.aws.batch/UpdatePolicy)
(s/def :cognitect.aws.batch.UpdateComputeEnvironmentRequest/context :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.UpdateJobQueueResponse/jobQueueName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.UpdateJobQueueResponse/jobQueueArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksProperties/podProperties :cognitect.aws.batch/EksPodProperties)
(s/def :cognitect.aws.batch.EksAttemptContainerDetail/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksAttemptContainerDetail/exitCode :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.EksAttemptContainerDetail/reason :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.RegisterJobDefinitionResponse/jobDefinitionName
:cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.RegisterJobDefinitionResponse/jobDefinitionArn
:cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.RegisterJobDefinitionResponse/revision :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.UntagResourceRequest/resourceArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.UntagResourceRequest/tagKeys :cognitect.aws.batch/TagKeysList)
(s/def :cognitect.aws.batch.NodeOverrides/numNodes :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.NodeOverrides/nodePropertyOverrides
:cognitect.aws.batch/NodePropertyOverrides)
(s/def :cognitect.aws.batch.JobSummary/startedAt :cognitect.aws.batch/Long)
(s/def :cognitect.aws.batch.JobSummary/stoppedAt :cognitect.aws.batch/Long)
(s/def :cognitect.aws.batch.JobSummary/jobName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobSummary/arrayProperties :cognitect.aws.batch/ArrayPropertiesSummary)
(s/def :cognitect.aws.batch.JobSummary/createdAt :cognitect.aws.batch/Long)
(s/def :cognitect.aws.batch.JobSummary/statusReason :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobSummary/nodeProperties :cognitect.aws.batch/NodePropertiesSummary)
(s/def :cognitect.aws.batch.JobSummary/jobDefinition :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobSummary/status :cognitect.aws.batch/JobStatus)
(s/def :cognitect.aws.batch.JobSummary/container :cognitect.aws.batch/ContainerSummary)
(s/def :cognitect.aws.batch.JobSummary/jobArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.JobSummary/jobId :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.SchedulingPolicyDetail/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.SchedulingPolicyDetail/arn :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.SchedulingPolicyDetail/fairsharePolicy
:cognitect.aws.batch/FairsharePolicy)
(s/def :cognitect.aws.batch.SchedulingPolicyDetail/tags :cognitect.aws.batch/TagrisTagsMap)
(s/def :cognitect.aws.batch.GetJobQueueSnapshotRequest/jobQueue :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksMetadata/labels :cognitect.aws.batch/EksLabelsMap)
(s/def :cognitect.aws.batch.Ulimit/hardLimit :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.Ulimit/name :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.Ulimit/softLimit :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.LinuxParameters/devices :cognitect.aws.batch/DevicesList)
(s/def :cognitect.aws.batch.LinuxParameters/initProcessEnabled :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.LinuxParameters/sharedMemorySize :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.LinuxParameters/tmpfs :cognitect.aws.batch/TmpfsList)
(s/def :cognitect.aws.batch.LinuxParameters/maxSwap :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.LinuxParameters/swappiness :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ComputeResource/tags :cognitect.aws.batch/TagsMap)
(s/def
:cognitect.aws.batch.ComputeResource/allocationStrategy
:cognitect.aws.batch/CRAllocationStrategy)
(s/def :cognitect.aws.batch.ComputeResource/ec2KeyPair :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ComputeResource/spotIamFleetRole :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ComputeResource/instanceRole :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ComputeResource/desiredvCpus :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ComputeResource/type :cognitect.aws.batch/CRType)
(s/def :cognitect.aws.batch.ComputeResource/maxvCpus :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.ComputeResource/launchTemplate
:cognitect.aws.batch/LaunchTemplateSpecification)
(s/def :cognitect.aws.batch.ComputeResource/subnets :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.ComputeResource/securityGroupIds :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.ComputeResource/placementGroup :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ComputeResource/minvCpus :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.ComputeResource/ec2Configuration
:cognitect.aws.batch/Ec2ConfigurationList)
(s/def :cognitect.aws.batch.ComputeResource/imageId :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ComputeResource/bidPercentage :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ComputeResource/instanceTypes :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.TerminateJobRequest/jobId :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.TerminateJobRequest/reason :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ImagePullSecret/name :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.EcsPropertiesDetail/taskProperties
:cognitect.aws.batch/ListEcsTaskDetails)
(s/def :cognitect.aws.batch.Host/sourcePath :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.DescribeComputeEnvironmentsRequest/computeEnvironments
:cognitect.aws.batch/StringList)
(s/def
:cognitect.aws.batch.DescribeComputeEnvironmentsRequest/maxResults
:cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.DescribeComputeEnvironmentsRequest/nextToken
:cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksPodProperties/imagePullSecrets :cognitect.aws.batch/ImagePullSecrets)
(s/def :cognitect.aws.batch.EksPodProperties/hostNetwork :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.EksPodProperties/containers :cognitect.aws.batch/EksContainers)
(s/def :cognitect.aws.batch.EksPodProperties/shareProcessNamespace :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.EksPodProperties/volumes :cognitect.aws.batch/EksVolumes)
(s/def :cognitect.aws.batch.EksPodProperties/dnsPolicy :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksPodProperties/initContainers :cognitect.aws.batch/EksContainers)
(s/def :cognitect.aws.batch.EksPodProperties/serviceAccountName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksPodProperties/metadata :cognitect.aws.batch/EksMetadata)
(s/def :cognitect.aws.batch.JobStateTimeLimitAction/reason :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.JobStateTimeLimitAction/state
:cognitect.aws.batch/JobStateTimeLimitActionsState)
(s/def :cognitect.aws.batch.JobStateTimeLimitAction/maxTimeSeconds :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.JobStateTimeLimitAction/action
:cognitect.aws.batch/JobStateTimeLimitActionsAction)
(s/def :cognitect.aws.batch.NodePropertyOverride/targetNodes :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.NodePropertyOverride/containerOverrides
:cognitect.aws.batch/ContainerOverrides)
(s/def
:cognitect.aws.batch.NodePropertyOverride/ecsPropertiesOverride
:cognitect.aws.batch/EcsPropertiesOverride)
(s/def :cognitect.aws.batch.NodePropertyOverride/instanceTypes :cognitect.aws.batch/StringList)
(s/def
:cognitect.aws.batch.NodePropertyOverride/eksPropertiesOverride
:cognitect.aws.batch/EksPropertiesOverride)
(s/def :cognitect.aws.batch.ComputeResourceUpdate/tags :cognitect.aws.batch/TagsMap)
(s/def
:cognitect.aws.batch.ComputeResourceUpdate/allocationStrategy
:cognitect.aws.batch/CRUpdateAllocationStrategy)
(s/def :cognitect.aws.batch.ComputeResourceUpdate/ec2KeyPair :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.ComputeResourceUpdate/updateToLatestImageVersion
:cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.ComputeResourceUpdate/instanceRole :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ComputeResourceUpdate/desiredvCpus :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ComputeResourceUpdate/type :cognitect.aws.batch/CRType)
(s/def :cognitect.aws.batch.ComputeResourceUpdate/maxvCpus :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.ComputeResourceUpdate/launchTemplate
:cognitect.aws.batch/LaunchTemplateSpecification)
(s/def :cognitect.aws.batch.ComputeResourceUpdate/subnets :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.ComputeResourceUpdate/securityGroupIds :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.ComputeResourceUpdate/placementGroup :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ComputeResourceUpdate/minvCpus :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.ComputeResourceUpdate/ec2Configuration
:cognitect.aws.batch/Ec2ConfigurationList)
(s/def :cognitect.aws.batch.ComputeResourceUpdate/imageId :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ComputeResourceUpdate/bidPercentage :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ComputeResourceUpdate/instanceTypes :cognitect.aws.batch/StringList)
(s/def :cognitect.aws.batch.FairsharePolicy/shareDecaySeconds :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.FairsharePolicy/computeReservation :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.FairsharePolicy/shareDistribution
:cognitect.aws.batch/ShareAttributesList)
(s/def
:cognitect.aws.batch.DescribeSchedulingPoliciesResponse/schedulingPolicies
:cognitect.aws.batch/SchedulingPolicyDetailList)
(s/def :cognitect.aws.batch.Device/hostPath :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.Device/containerPath :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.Device/permissions :cognitect.aws.batch/DeviceCgroupPermissions)
(s/def
:cognitect.aws.batch.LaunchTemplateSpecification/launchTemplateId
:cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.LaunchTemplateSpecification/launchTemplateName
:cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.LaunchTemplateSpecification/version :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.EksAttemptDetail/containers
:cognitect.aws.batch/EksAttemptContainerDetails)
(s/def
:cognitect.aws.batch.EksAttemptDetail/initContainers
:cognitect.aws.batch/EksAttemptContainerDetails)
(s/def :cognitect.aws.batch.EksAttemptDetail/eksClusterArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksAttemptDetail/podName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksAttemptDetail/nodeName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.EksAttemptDetail/startedAt :cognitect.aws.batch/Long)
(s/def :cognitect.aws.batch.EksAttemptDetail/stoppedAt :cognitect.aws.batch/Long)
(s/def :cognitect.aws.batch.EksAttemptDetail/statusReason :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.DescribeJobDefinitionsResponse/jobDefinitions
:cognitect.aws.batch/JobDefinitionList)
(s/def :cognitect.aws.batch.DescribeJobDefinitionsResponse/nextToken :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.CancelJobRequest/jobId :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.CancelJobRequest/reason :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.AttemptContainerDetail/containerInstanceArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.AttemptContainerDetail/taskArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.AttemptContainerDetail/exitCode :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.AttemptContainerDetail/reason :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.AttemptContainerDetail/logStreamName :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.AttemptContainerDetail/networkInterfaces
:cognitect.aws.batch/NetworkInterfaceList)
(s/def
:cognitect.aws.batch.DescribeJobQueuesResponse/jobQueues
:cognitect.aws.batch/JobQueueDetailList)
(s/def :cognitect.aws.batch.DescribeJobQueuesResponse/nextToken :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.ListTagsForResourceResponse/tags :cognitect.aws.batch/TagrisTagsMap)
(s/def
:cognitect.aws.batch.DeleteComputeEnvironmentRequest/computeEnvironment
:cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.FrontOfQueueJobSummary/jobArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.FrontOfQueueJobSummary/earliestTimeAtPosition :cognitect.aws.batch/Long)
(s/def
:cognitect.aws.batch.EksPropertiesDetail/podProperties
:cognitect.aws.batch/EksPodPropertiesDetail)
(s/def :cognitect.aws.batch.SubmitJobResponse/jobArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.SubmitJobResponse/jobName :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.SubmitJobResponse/jobId :cognitect.aws.batch/String)
(s/def
:cognitect.aws.batch.ArrayPropertiesDetail/statusSummary
:cognitect.aws.batch/ArrayJobStatusSummary)
(s/def :cognitect.aws.batch.ArrayPropertiesDetail/size :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ArrayPropertiesDetail/index :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.DeregisterJobDefinitionRequest/jobDefinition
:cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.NodePropertiesSummary/isMainNode :cognitect.aws.batch/Boolean)
(s/def :cognitect.aws.batch.NodePropertiesSummary/numNodes :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.NodePropertiesSummary/nodeIndex :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.UpdateJobQueueRequest/jobQueue :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.UpdateJobQueueRequest/state :cognitect.aws.batch/JQState)
(s/def :cognitect.aws.batch.UpdateJobQueueRequest/schedulingPolicyArn :cognitect.aws.batch/String)
(s/def :cognitect.aws.batch.UpdateJobQueueRequest/priority :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.UpdateJobQueueRequest/computeEnvironmentOrder
:cognitect.aws.batch/ComputeEnvironmentOrders)
(s/def
:cognitect.aws.batch.UpdateJobQueueRequest/jobStateTimeLimitActions
:cognitect.aws.batch/JobStateTimeLimitActions)
(s/def :cognitect.aws.batch.ArrayPropertiesSummary/size :cognitect.aws.batch/Integer)
(s/def :cognitect.aws.batch.ArrayPropertiesSummary/index :cognitect.aws.batch/Integer)
(s/def
:cognitect.aws.batch.GetJobQueueSnapshotResponse/frontOfQueue
:cognitect.aws.batch/FrontOfQueueDetail)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy