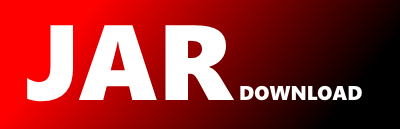
cognitect.aws.ecr_public.specs.clj Maven / Gradle / Ivy
The newest version!
;; Copyright (c) Cognitect, Inc.
;; All rights reserved.
(ns cognitect.aws.ecr-public.specs
(:require [clojure.spec.alpha :as s] [clojure.spec.gen.alpha :as gen]))
(s/def :cognitect.aws/client map?)
(s/def :core.async/channel any?)
(s/def :cognitect.aws.ecr-public/Url string?)
(s/def :cognitect.aws.ecr-public/RegistryVerified boolean?)
(s/def
:cognitect.aws.ecr-public/TagResourceRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.TagResourceRequest/resourceArn
:cognitect.aws.ecr-public.TagResourceRequest/tags]))
(s/def
:cognitect.aws.ecr-public/DescribeImageTagsResponse
(s/keys
:opt-un
[:cognitect.aws.ecr-public.DescribeImageTagsResponse/nextToken
:cognitect.aws.ecr-public.DescribeImageTagsResponse/imageTagDetails]))
(s/def
:cognitect.aws.ecr-public/DescribeRepositoriesRequest
(s/keys
:opt-un
[:cognitect.aws.ecr-public.DescribeRepositoriesRequest/registryId
:cognitect.aws.ecr-public.DescribeRepositoriesRequest/repositoryNames
:cognitect.aws.ecr-public.DescribeRepositoriesRequest/maxResults
:cognitect.aws.ecr-public.DescribeRepositoriesRequest/nextToken]))
(s/def
:cognitect.aws.ecr-public/RepositoryCatalogDataInput
(s/keys
:opt-un
[:cognitect.aws.ecr-public.RepositoryCatalogDataInput/architectures
:cognitect.aws.ecr-public.RepositoryCatalogDataInput/logoImageBlob
:cognitect.aws.ecr-public.RepositoryCatalogDataInput/operatingSystems
:cognitect.aws.ecr-public.RepositoryCatalogDataInput/usageText
:cognitect.aws.ecr-public.RepositoryCatalogDataInput/aboutText
:cognitect.aws.ecr-public.RepositoryCatalogDataInput/description]))
(s/def
:cognitect.aws.ecr-public/GetRepositoryCatalogDataResponse
(s/keys :opt-un [:cognitect.aws.ecr-public.GetRepositoryCatalogDataResponse/catalogData]))
(s/def :cognitect.aws.ecr-public/LayerList (s/coll-of :cognitect.aws.ecr-public/Layer))
(s/def
:cognitect.aws.ecr-public/RegistryCatalogData
(s/keys :opt-un [:cognitect.aws.ecr-public.RegistryCatalogData/displayName]))
(s/def
:cognitect.aws.ecr-public/RegistryAliasName
(s/spec #(re-matches (re-pattern "[a-z][a-z0-9]+(?:[._-][a-z0-9]+)*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.ecr-public/ArchitectureList
(s/coll-of :cognitect.aws.ecr-public/Architecture :max-count 50))
(s/def
:cognitect.aws.ecr-public/UploadLayerPartResponse
(s/keys
:opt-un
[:cognitect.aws.ecr-public.UploadLayerPartResponse/repositoryName
:cognitect.aws.ecr-public.UploadLayerPartResponse/registryId
:cognitect.aws.ecr-public.UploadLayerPartResponse/uploadId
:cognitect.aws.ecr-public.UploadLayerPartResponse/lastByteReceived]))
(s/def
:cognitect.aws.ecr-public/DescribeImagesRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.DescribeImagesRequest/repositoryName]
:opt-un
[:cognitect.aws.ecr-public.DescribeImagesRequest/registryId
:cognitect.aws.ecr-public.DescribeImagesRequest/maxResults
:cognitect.aws.ecr-public.DescribeImagesRequest/nextToken
:cognitect.aws.ecr-public.DescribeImagesRequest/imageIds]))
(s/def
:cognitect.aws.ecr-public/Tag
(s/keys :opt-un [:cognitect.aws.ecr-public.Tag/Key :cognitect.aws.ecr-public.Tag/Value]))
(s/def
:cognitect.aws.ecr-public/LayerPartTooSmallException
(s/keys :opt-un [:cognitect.aws.ecr-public.LayerPartTooSmallException/message]))
(s/def
:cognitect.aws.ecr-public/DeleteRepositoryResponse
(s/keys :opt-un [:cognitect.aws.ecr-public.DeleteRepositoryResponse/repository]))
(s/def
:cognitect.aws.ecr-public/GetRepositoryPolicyResponse
(s/keys
:opt-un
[:cognitect.aws.ecr-public.GetRepositoryPolicyResponse/repositoryName
:cognitect.aws.ecr-public.GetRepositoryPolicyResponse/registryId
:cognitect.aws.ecr-public.GetRepositoryPolicyResponse/policyText]))
(s/def
:cognitect.aws.ecr-public/RepositoryDescription
(s/spec
(s/and string? #(>= 1024 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 1024) #(gen/vector (gen/char-alpha) %))))))
(s/def :cognitect.aws.ecr-public/TagResourceResponse (s/keys))
(s/def :cognitect.aws.ecr-public/ExceptionMessage string?)
(s/def
:cognitect.aws.ecr-public/LayerPartBlob
(s/spec
(s/or
:byte-array
(s/and bytes? #(<= 0 (count %) 20971520))
:input-stream
#(instance? java.io.InputStream %))
:gen
(fn []
(gen/bind
(gen/choose (or 0 0) (or 20971520 100))
#(gen/return (byte-array % (repeatedly (fn [] (rand-int 256)))))))))
(s/def
:cognitect.aws.ecr-public/UploadId
(s/spec
#(re-matches
(re-pattern "[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12}")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.ecr-public/DescribeRegistriesRequest
(s/keys
:opt-un
[:cognitect.aws.ecr-public.DescribeRegistriesRequest/maxResults
:cognitect.aws.ecr-public.DescribeRegistriesRequest/nextToken]))
(s/def
:cognitect.aws.ecr-public/LayersNotFoundException
(s/keys :opt-un [:cognitect.aws.ecr-public.LayersNotFoundException/message]))
(s/def
:cognitect.aws.ecr-public/UnsupportedCommandException
(s/keys :opt-un [:cognitect.aws.ecr-public.UnsupportedCommandException/message]))
(s/def :cognitect.aws.ecr-public/ImageFailureReason string?)
(s/def
:cognitect.aws.ecr-public/Image
(s/keys
:opt-un
[:cognitect.aws.ecr-public.Image/repositoryName
:cognitect.aws.ecr-public.Image/registryId
:cognitect.aws.ecr-public.Image/imageManifest
:cognitect.aws.ecr-public.Image/imageId
:cognitect.aws.ecr-public.Image/imageManifestMediaType]))
(s/def
:cognitect.aws.ecr-public/CompleteLayerUploadResponse
(s/keys
:opt-un
[:cognitect.aws.ecr-public.CompleteLayerUploadResponse/repositoryName
:cognitect.aws.ecr-public.CompleteLayerUploadResponse/registryId
:cognitect.aws.ecr-public.CompleteLayerUploadResponse/layerDigest
:cognitect.aws.ecr-public.CompleteLayerUploadResponse/uploadId]))
(s/def
:cognitect.aws.ecr-public/BatchedOperationLayerDigest
(s/spec
(s/and string? #(<= 0 (count %) 1000))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 1000) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.ecr-public/ImageAlreadyExistsException
(s/keys :opt-un [:cognitect.aws.ecr-public.ImageAlreadyExistsException/message]))
(s/def
:cognitect.aws.ecr-public/ImageTagDetail
(s/keys
:opt-un
[:cognitect.aws.ecr-public.ImageTagDetail/imageDetail
:cognitect.aws.ecr-public.ImageTagDetail/createdAt
:cognitect.aws.ecr-public.ImageTagDetail/imageTag]))
(s/def
:cognitect.aws.ecr-public/TagList
(s/coll-of :cognitect.aws.ecr-public/Tag :min-count 0 :max-count 200))
(s/def
:cognitect.aws.ecr-public/RepositoryNotEmptyException
(s/keys :opt-un [:cognitect.aws.ecr-public.RepositoryNotEmptyException/message]))
(s/def
:cognitect.aws.ecr-public/ReferencedImageDetail
(s/keys
:opt-un
[:cognitect.aws.ecr-public.ReferencedImageDetail/imagePushedAt
:cognitect.aws.ecr-public.ReferencedImageDetail/imageManifestMediaType
:cognitect.aws.ecr-public.ReferencedImageDetail/imageDigest
:cognitect.aws.ecr-public.ReferencedImageDetail/imageSizeInBytes
:cognitect.aws.ecr-public.ReferencedImageDetail/artifactMediaType]))
(s/def
:cognitect.aws.ecr-public/RepositoryName
(s/spec
#(re-matches (re-pattern "(?:[a-z0-9]+(?:[._-][a-z0-9]+)*/)*[a-z0-9]+(?:[._-][a-z0-9]+)*") %)
:gen
#(gen/string)))
(s/def
:cognitect.aws.ecr-public/LayerDigestList
(s/coll-of :cognitect.aws.ecr-public/LayerDigest :min-count 1 :max-count 100))
(s/def
:cognitect.aws.ecr-public/ImageDigestDoesNotMatchException
(s/keys :opt-un [:cognitect.aws.ecr-public.ImageDigestDoesNotMatchException/message]))
(s/def
:cognitect.aws.ecr-public/UsageText
(s/spec
(s/and string? #(>= 25600 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 25600) #(gen/vector (gen/char-alpha) %))))))
(s/def :cognitect.aws.ecr-public/CreationTimestamp inst?)
(s/def
:cognitect.aws.ecr-public/RegistryNotFoundException
(s/keys :opt-un [:cognitect.aws.ecr-public.RegistryNotFoundException/message]))
(s/def
:cognitect.aws.ecr-public/Architecture
(s/spec
(s/and string? #(<= 1 (count %) 50))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 50) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.ecr-public/RepositoryNameList
(s/coll-of :cognitect.aws.ecr-public/RepositoryName :min-count 1 :max-count 100))
(s/def
:cognitect.aws.ecr-public/RegistryDisplayName
(s/spec
(s/and string? #(<= 0 (count %) 100))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 100) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.ecr-public/ImageManifest
(s/spec
(s/and string? #(<= 1 (count %) 4194304))
:gen
(fn []
(gen/fmap
#(apply str %)
(gen/bind (gen/choose 1 4194304) #(gen/vector (gen/char-alpha) %))))))
(s/def :cognitect.aws.ecr-public/GetAuthorizationTokenRequest (s/keys))
(s/def
:cognitect.aws.ecr-public/PutRepositoryCatalogDataResponse
(s/keys :opt-un [:cognitect.aws.ecr-public.PutRepositoryCatalogDataResponse/catalogData]))
(s/def
:cognitect.aws.ecr-public/LayerFailure
(s/keys
:opt-un
[:cognitect.aws.ecr-public.LayerFailure/layerDigest
:cognitect.aws.ecr-public.LayerFailure/failureCode
:cognitect.aws.ecr-public.LayerFailure/failureReason]))
(s/def :cognitect.aws.ecr-public/ForceFlag boolean?)
(s/def :cognitect.aws.ecr-public/MediaType string?)
(s/def
:cognitect.aws.ecr-public/RepositoryCatalogDataNotFoundException
(s/keys :opt-un [:cognitect.aws.ecr-public.RepositoryCatalogDataNotFoundException/message]))
(s/def
:cognitect.aws.ecr-public/GetRegistryCatalogDataResponse
(s/keys :req-un [:cognitect.aws.ecr-public.GetRegistryCatalogDataResponse/registryCatalogData]))
(s/def
:cognitect.aws.ecr-public/LayerAvailability
(s/spec string? :gen #(s/gen #{"AVAILABLE" "UNAVAILABLE"})))
(s/def
:cognitect.aws.ecr-public/SetRepositoryPolicyRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.SetRepositoryPolicyRequest/repositoryName
:cognitect.aws.ecr-public.SetRepositoryPolicyRequest/policyText]
:opt-un
[:cognitect.aws.ecr-public.SetRepositoryPolicyRequest/registryId
:cognitect.aws.ecr-public.SetRepositoryPolicyRequest/force]))
(s/def
:cognitect.aws.ecr-public/LayerFailureCode
(s/spec string? :gen #(s/gen #{"MissingLayerDigest" "InvalidLayerDigest"})))
(s/def
:cognitect.aws.ecr-public/RepositoryNotFoundException
(s/keys :opt-un [:cognitect.aws.ecr-public.RepositoryNotFoundException/message]))
(s/def :cognitect.aws.ecr-public/DefaultRegistryAliasFlag boolean?)
(s/def
:cognitect.aws.ecr-public/DescribeRegistriesResponse
(s/keys
:req-un
[:cognitect.aws.ecr-public.DescribeRegistriesResponse/registries]
:opt-un
[:cognitect.aws.ecr-public.DescribeRegistriesResponse/nextToken]))
(s/def
:cognitect.aws.ecr-public/DeleteRepositoryPolicyResponse
(s/keys
:opt-un
[:cognitect.aws.ecr-public.DeleteRepositoryPolicyResponse/repositoryName
:cognitect.aws.ecr-public.DeleteRepositoryPolicyResponse/registryId
:cognitect.aws.ecr-public.DeleteRepositoryPolicyResponse/policyText]))
(s/def
:cognitect.aws.ecr-public/LayerFailureList
(s/coll-of :cognitect.aws.ecr-public/LayerFailure))
(s/def :cognitect.aws.ecr-public/ExpirationTimestamp inst?)
(s/def
:cognitect.aws.ecr-public/ImageFailureList
(s/coll-of :cognitect.aws.ecr-public/ImageFailure))
(s/def
:cognitect.aws.ecr-public/PartSize
(s/spec (s/and int? #(<= 0 %)) :gen #(gen/choose 0 Long/MAX_VALUE)))
(s/def :cognitect.aws.ecr-public/NextToken string?)
(s/def
:cognitect.aws.ecr-public/TooManyTagsException
(s/keys :opt-un [:cognitect.aws.ecr-public.TooManyTagsException/message]))
(s/def :cognitect.aws.ecr-public/RegistryList (s/coll-of :cognitect.aws.ecr-public/Registry))
(s/def :cognitect.aws.ecr-public/PrimaryRegistryAliasFlag boolean?)
(s/def
:cognitect.aws.ecr-public/TagValue
(s/spec
(s/and string? #(<= 0 (count %) 256))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 256) #(gen/vector (gen/char-alpha) %))))))
(s/def :cognitect.aws.ecr-public/ImageTagList (s/coll-of :cognitect.aws.ecr-public/ImageTag))
(s/def
:cognitect.aws.ecr-public/PutRepositoryCatalogDataRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.PutRepositoryCatalogDataRequest/repositoryName
:cognitect.aws.ecr-public.PutRepositoryCatalogDataRequest/catalogData]
:opt-un
[:cognitect.aws.ecr-public.PutRepositoryCatalogDataRequest/registryId]))
(s/def
:cognitect.aws.ecr-public/PutRegistryCatalogDataResponse
(s/keys :req-un [:cognitect.aws.ecr-public.PutRegistryCatalogDataResponse/registryCatalogData]))
(s/def
:cognitect.aws.ecr-public/UploadLayerPartRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.UploadLayerPartRequest/repositoryName
:cognitect.aws.ecr-public.UploadLayerPartRequest/uploadId
:cognitect.aws.ecr-public.UploadLayerPartRequest/partFirstByte
:cognitect.aws.ecr-public.UploadLayerPartRequest/partLastByte
:cognitect.aws.ecr-public.UploadLayerPartRequest/layerPartBlob]
:opt-un
[:cognitect.aws.ecr-public.UploadLayerPartRequest/registryId]))
(s/def
:cognitect.aws.ecr-public/LayerSizeInBytes
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.ecr-public/ListTagsForResourceRequest
(s/keys :req-un [:cognitect.aws.ecr-public.ListTagsForResourceRequest/resourceArn]))
(s/def
:cognitect.aws.ecr-public/RegistryIdOrAlias
(s/spec
(s/and string? #(<= 2 (count %) 50))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 2 50) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.ecr-public/ImageNotFoundException
(s/keys :opt-un [:cognitect.aws.ecr-public.ImageNotFoundException/message]))
(s/def
:cognitect.aws.ecr-public/PutRegistryCatalogDataRequest
(s/keys :opt-un [:cognitect.aws.ecr-public.PutRegistryCatalogDataRequest/displayName]))
(s/def
:cognitect.aws.ecr-public/InvalidParameterException
(s/keys :opt-un [:cognitect.aws.ecr-public.InvalidParameterException/message]))
(s/def :cognitect.aws.ecr-public/UntagResourceResponse (s/keys))
(s/def
:cognitect.aws.ecr-public/EmptyUploadException
(s/keys :opt-un [:cognitect.aws.ecr-public.EmptyUploadException/message]))
(s/def
:cognitect.aws.ecr-public/InvalidLayerException
(s/keys :opt-un [:cognitect.aws.ecr-public.InvalidLayerException/message]))
(s/def
:cognitect.aws.ecr-public/RepositoryCatalogData
(s/keys
:opt-un
[:cognitect.aws.ecr-public.RepositoryCatalogData/architectures
:cognitect.aws.ecr-public.RepositoryCatalogData/marketplaceCertified
:cognitect.aws.ecr-public.RepositoryCatalogData/operatingSystems
:cognitect.aws.ecr-public.RepositoryCatalogData/usageText
:cognitect.aws.ecr-public.RepositoryCatalogData/aboutText
:cognitect.aws.ecr-public.RepositoryCatalogData/logoUrl
:cognitect.aws.ecr-public.RepositoryCatalogData/description]))
(s/def
:cognitect.aws.ecr-public/ImageFailureCode
(s/spec
string?
:gen
#(s/gen
#{"ImageNotFound"
"InvalidImageDigest"
"InvalidImageTag"
"ImageTagDoesNotMatchDigest"
"MissingDigestAndTag"
"ImageReferencedByManifestList"
"KmsError"})))
(s/def
:cognitect.aws.ecr-public/Registry
(s/keys
:req-un
[:cognitect.aws.ecr-public.Registry/registryId
:cognitect.aws.ecr-public.Registry/registryArn
:cognitect.aws.ecr-public.Registry/registryUri
:cognitect.aws.ecr-public.Registry/verified
:cognitect.aws.ecr-public.Registry/aliases]))
(s/def
:cognitect.aws.ecr-public/RepositoryPolicyText
(s/spec
(s/and string? #(<= 0 (count %) 10240))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 10240) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.ecr-public/LayerDigest
(s/spec #(re-matches (re-pattern "[a-zA-Z0-9-_+.]+:[a-fA-F0-9]+") %) :gen #(gen/string)))
(s/def
:cognitect.aws.ecr-public/CreateRepositoryResponse
(s/keys
:opt-un
[:cognitect.aws.ecr-public.CreateRepositoryResponse/repository
:cognitect.aws.ecr-public.CreateRepositoryResponse/catalogData]))
(s/def
:cognitect.aws.ecr-public/ReferencedImagesNotFoundException
(s/keys :opt-un [:cognitect.aws.ecr-public.ReferencedImagesNotFoundException/message]))
(s/def :cognitect.aws.ecr-public/ImageDigest string?)
(s/def
:cognitect.aws.ecr-public/UploadNotFoundException
(s/keys :opt-un [:cognitect.aws.ecr-public.UploadNotFoundException/message]))
(s/def
:cognitect.aws.ecr-public/InvalidTagParameterException
(s/keys :opt-un [:cognitect.aws.ecr-public.InvalidTagParameterException/message]))
(s/def
:cognitect.aws.ecr-public/InvalidLayerPartException
(s/keys
:opt-un
[:cognitect.aws.ecr-public.InvalidLayerPartException/message
:cognitect.aws.ecr-public.InvalidLayerPartException/repositoryName
:cognitect.aws.ecr-public.InvalidLayerPartException/registryId
:cognitect.aws.ecr-public.InvalidLayerPartException/lastValidByteReceived
:cognitect.aws.ecr-public.InvalidLayerPartException/uploadId]))
(s/def
:cognitect.aws.ecr-public/Base64
(s/spec #(re-matches (re-pattern "^\\S+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.ecr-public/CompleteLayerUploadRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.CompleteLayerUploadRequest/repositoryName
:cognitect.aws.ecr-public.CompleteLayerUploadRequest/uploadId
:cognitect.aws.ecr-public.CompleteLayerUploadRequest/layerDigests]
:opt-un
[:cognitect.aws.ecr-public.CompleteLayerUploadRequest/registryId]))
(s/def
:cognitect.aws.ecr-public/BatchDeleteImageRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.BatchDeleteImageRequest/repositoryName
:cognitect.aws.ecr-public.BatchDeleteImageRequest/imageIds]
:opt-un
[:cognitect.aws.ecr-public.BatchDeleteImageRequest/registryId]))
(s/def
:cognitect.aws.ecr-public/RepositoryPolicyNotFoundException
(s/keys :opt-un [:cognitect.aws.ecr-public.RepositoryPolicyNotFoundException/message]))
(s/def
:cognitect.aws.ecr-public/BatchCheckLayerAvailabilityResponse
(s/keys
:opt-un
[:cognitect.aws.ecr-public.BatchCheckLayerAvailabilityResponse/failures
:cognitect.aws.ecr-public.BatchCheckLayerAvailabilityResponse/layers]))
(s/def
:cognitect.aws.ecr-public/BatchCheckLayerAvailabilityRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.BatchCheckLayerAvailabilityRequest/repositoryName
:cognitect.aws.ecr-public.BatchCheckLayerAvailabilityRequest/layerDigests]
:opt-un
[:cognitect.aws.ecr-public.BatchCheckLayerAvailabilityRequest/registryId]))
(s/def
:cognitect.aws.ecr-public/InitiateLayerUploadRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.InitiateLayerUploadRequest/repositoryName]
:opt-un
[:cognitect.aws.ecr-public.InitiateLayerUploadRequest/registryId]))
(s/def
:cognitect.aws.ecr-public/ServerException
(s/keys :opt-un [:cognitect.aws.ecr-public.ServerException/message]))
(s/def
:cognitect.aws.ecr-public/GetRepositoryPolicyRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.GetRepositoryPolicyRequest/repositoryName]
:opt-un
[:cognitect.aws.ecr-public.GetRepositoryPolicyRequest/registryId]))
(s/def
:cognitect.aws.ecr-public/Arn
(s/spec
(s/and string? #(<= 1 (count %) 2048))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 2048) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.ecr-public/ImageTagDetailList
(s/coll-of :cognitect.aws.ecr-public/ImageTagDetail))
(s/def
:cognitect.aws.ecr-public/SetRepositoryPolicyResponse
(s/keys
:opt-un
[:cognitect.aws.ecr-public.SetRepositoryPolicyResponse/repositoryName
:cognitect.aws.ecr-public.SetRepositoryPolicyResponse/registryId
:cognitect.aws.ecr-public.SetRepositoryPolicyResponse/policyText]))
(s/def :cognitect.aws.ecr-public/MarketplaceCertified boolean?)
(s/def
:cognitect.aws.ecr-public/MaxResults
(s/spec (s/and int? #(<= 1 % 1000)) :gen #(gen/choose 1 1000)))
(s/def
:cognitect.aws.ecr-public/ImageDetail
(s/keys
:opt-un
[:cognitect.aws.ecr-public.ImageDetail/repositoryName
:cognitect.aws.ecr-public.ImageDetail/registryId
:cognitect.aws.ecr-public.ImageDetail/imagePushedAt
:cognitect.aws.ecr-public.ImageDetail/imageTags
:cognitect.aws.ecr-public.ImageDetail/imageManifestMediaType
:cognitect.aws.ecr-public.ImageDetail/imageDigest
:cognitect.aws.ecr-public.ImageDetail/imageSizeInBytes
:cognitect.aws.ecr-public.ImageDetail/artifactMediaType]))
(s/def
:cognitect.aws.ecr-public/UntagResourceRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.UntagResourceRequest/resourceArn
:cognitect.aws.ecr-public.UntagResourceRequest/tagKeys]))
(s/def
:cognitect.aws.ecr-public/TagKey
(s/spec
(s/and string? #(<= 1 (count %) 128))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 128) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.ecr-public/GetRepositoryCatalogDataRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.GetRepositoryCatalogDataRequest/repositoryName]
:opt-un
[:cognitect.aws.ecr-public.GetRepositoryCatalogDataRequest/registryId]))
(s/def
:cognitect.aws.ecr-public/LayerAlreadyExistsException
(s/keys :opt-un [:cognitect.aws.ecr-public.LayerAlreadyExistsException/message]))
(s/def
:cognitect.aws.ecr-public/LogoImageBlob
(s/spec
(s/or
:byte-array
(s/and bytes? #(<= 0 (count %) 512000))
:input-stream
#(instance? java.io.InputStream %))
:gen
(fn []
(gen/bind
(gen/choose (or 0 0) (or 512000 100))
#(gen/return (byte-array % (repeatedly (fn [] (rand-int 256)))))))))
(s/def :cognitect.aws.ecr-public/GetRegistryCatalogDataRequest (s/keys))
(s/def
:cognitect.aws.ecr-public/RepositoryAlreadyExistsException
(s/keys :opt-un [:cognitect.aws.ecr-public.RepositoryAlreadyExistsException/message]))
(s/def :cognitect.aws.ecr-public/LayerFailureReason string?)
(s/def
:cognitect.aws.ecr-public/DescribeRepositoriesResponse
(s/keys
:opt-un
[:cognitect.aws.ecr-public.DescribeRepositoriesResponse/nextToken
:cognitect.aws.ecr-public.DescribeRepositoriesResponse/repositories]))
(s/def
:cognitect.aws.ecr-public/OperatingSystem
(s/spec
(s/and string? #(<= 1 (count %) 50))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 50) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.ecr-public/GetAuthorizationTokenResponse
(s/keys :opt-un [:cognitect.aws.ecr-public.GetAuthorizationTokenResponse/authorizationData]))
(s/def
:cognitect.aws.ecr-public/DescribeImagesResponse
(s/keys
:opt-un
[:cognitect.aws.ecr-public.DescribeImagesResponse/nextToken
:cognitect.aws.ecr-public.DescribeImagesResponse/imageDetails]))
(s/def
:cognitect.aws.ecr-public/DeleteRepositoryRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.DeleteRepositoryRequest/repositoryName]
:opt-un
[:cognitect.aws.ecr-public.DeleteRepositoryRequest/registryId
:cognitect.aws.ecr-public.DeleteRepositoryRequest/force]))
(s/def
:cognitect.aws.ecr-public/DescribeImageTagsRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.DescribeImageTagsRequest/repositoryName]
:opt-un
[:cognitect.aws.ecr-public.DescribeImageTagsRequest/registryId
:cognitect.aws.ecr-public.DescribeImageTagsRequest/maxResults
:cognitect.aws.ecr-public.DescribeImageTagsRequest/nextToken]))
(s/def
:cognitect.aws.ecr-public/AuthorizationData
(s/keys
:opt-un
[:cognitect.aws.ecr-public.AuthorizationData/authorizationToken
:cognitect.aws.ecr-public.AuthorizationData/expiresAt]))
(s/def
:cognitect.aws.ecr-public/BatchedOperationLayerDigestList
(s/coll-of :cognitect.aws.ecr-public/BatchedOperationLayerDigest :min-count 1 :max-count 100))
(s/def :cognitect.aws.ecr-public/PushTimestamp inst?)
(s/def
:cognitect.aws.ecr-public/RegistryAliasStatus
(s/spec string? :gen #(s/gen #{"PENDING" "REJECTED" "ACTIVE"})))
(s/def
:cognitect.aws.ecr-public/ImageSizeInBytes
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.ecr-public/PutImageResponse
(s/keys :opt-un [:cognitect.aws.ecr-public.PutImageResponse/image]))
(s/def
:cognitect.aws.ecr-public/ImageIdentifierList
(s/coll-of :cognitect.aws.ecr-public/ImageIdentifier :min-count 1 :max-count 100))
(s/def
:cognitect.aws.ecr-public/RegistryAliasList
(s/coll-of :cognitect.aws.ecr-public/RegistryAlias))
(s/def :cognitect.aws.ecr-public/RepositoryList (s/coll-of :cognitect.aws.ecr-public/Repository))
(s/def
:cognitect.aws.ecr-public/Repository
(s/keys
:opt-un
[:cognitect.aws.ecr-public.Repository/repositoryName
:cognitect.aws.ecr-public.Repository/registryId
:cognitect.aws.ecr-public.Repository/createdAt
:cognitect.aws.ecr-public.Repository/repositoryUri
:cognitect.aws.ecr-public.Repository/repositoryArn]))
(s/def
:cognitect.aws.ecr-public/BatchDeleteImageResponse
(s/keys
:opt-un
[:cognitect.aws.ecr-public.BatchDeleteImageResponse/failures
:cognitect.aws.ecr-public.BatchDeleteImageResponse/imageIds]))
(s/def
:cognitect.aws.ecr-public/ImageTag
(s/spec
(s/and string? #(<= 1 (count %) 300))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 300) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.ecr-public/ImageFailure
(s/keys
:opt-un
[:cognitect.aws.ecr-public.ImageFailure/imageId
:cognitect.aws.ecr-public.ImageFailure/failureCode
:cognitect.aws.ecr-public.ImageFailure/failureReason]))
(s/def
:cognitect.aws.ecr-public/ImageIdentifier
(s/keys
:opt-un
[:cognitect.aws.ecr-public.ImageIdentifier/imageTag
:cognitect.aws.ecr-public.ImageIdentifier/imageDigest]))
(s/def
:cognitect.aws.ecr-public/PutImageRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.PutImageRequest/repositoryName
:cognitect.aws.ecr-public.PutImageRequest/imageManifest]
:opt-un
[:cognitect.aws.ecr-public.PutImageRequest/registryId
:cognitect.aws.ecr-public.PutImageRequest/imageTag
:cognitect.aws.ecr-public.PutImageRequest/imageManifestMediaType
:cognitect.aws.ecr-public.PutImageRequest/imageDigest]))
(s/def
:cognitect.aws.ecr-public/ListTagsForResourceResponse
(s/keys :opt-un [:cognitect.aws.ecr-public.ListTagsForResourceResponse/tags]))
(s/def
:cognitect.aws.ecr-public/LimitExceededException
(s/keys :opt-un [:cognitect.aws.ecr-public.LimitExceededException/message]))
(s/def
:cognitect.aws.ecr-public/OperatingSystemList
(s/coll-of :cognitect.aws.ecr-public/OperatingSystem :max-count 50))
(s/def
:cognitect.aws.ecr-public/RegistryId
(s/spec #(re-matches (re-pattern "[0-9]{12}") %) :gen #(gen/string)))
(s/def
:cognitect.aws.ecr-public/Layer
(s/keys
:opt-un
[:cognitect.aws.ecr-public.Layer/layerDigest
:cognitect.aws.ecr-public.Layer/layerAvailability
:cognitect.aws.ecr-public.Layer/mediaType
:cognitect.aws.ecr-public.Layer/layerSize]))
(s/def :cognitect.aws.ecr-public/ImageDetailList (s/coll-of :cognitect.aws.ecr-public/ImageDetail))
(s/def
:cognitect.aws.ecr-public/ResourceUrl
(s/spec
(s/and string? #(>= 2048 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 2048) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.ecr-public/InitiateLayerUploadResponse
(s/keys
:opt-un
[:cognitect.aws.ecr-public.InitiateLayerUploadResponse/uploadId
:cognitect.aws.ecr-public.InitiateLayerUploadResponse/partSize]))
(s/def
:cognitect.aws.ecr-public/CreateRepositoryRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.CreateRepositoryRequest/repositoryName]
:opt-un
[:cognitect.aws.ecr-public.CreateRepositoryRequest/tags
:cognitect.aws.ecr-public.CreateRepositoryRequest/catalogData]))
(s/def
:cognitect.aws.ecr-public/ImageTagAlreadyExistsException
(s/keys :opt-un [:cognitect.aws.ecr-public.ImageTagAlreadyExistsException/message]))
(s/def
:cognitect.aws.ecr-public/AboutText
(s/spec
(s/and string? #(>= 25600 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 25600) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.ecr-public/RegistryAlias
(s/keys
:req-un
[:cognitect.aws.ecr-public.RegistryAlias/name
:cognitect.aws.ecr-public.RegistryAlias/status
:cognitect.aws.ecr-public.RegistryAlias/primaryRegistryAlias
:cognitect.aws.ecr-public.RegistryAlias/defaultRegistryAlias]))
(s/def
:cognitect.aws.ecr-public/TagKeyList
(s/coll-of :cognitect.aws.ecr-public/TagKey :min-count 0 :max-count 200))
(s/def
:cognitect.aws.ecr-public/DeleteRepositoryPolicyRequest
(s/keys
:req-un
[:cognitect.aws.ecr-public.DeleteRepositoryPolicyRequest/repositoryName]
:opt-un
[:cognitect.aws.ecr-public.DeleteRepositoryPolicyRequest/registryId]))
(s/def :cognitect.aws.ecr-public.TagResourceRequest/resourceArn :cognitect.aws.ecr-public/Arn)
(s/def :cognitect.aws.ecr-public.TagResourceRequest/tags :cognitect.aws.ecr-public/TagList)
(s/def
:cognitect.aws.ecr-public.DescribeImageTagsResponse/imageTagDetails
:cognitect.aws.ecr-public/ImageTagDetailList)
(s/def
:cognitect.aws.ecr-public.DescribeImageTagsResponse/nextToken
:cognitect.aws.ecr-public/NextToken)
(s/def
:cognitect.aws.ecr-public.DescribeRepositoriesRequest/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.DescribeRepositoriesRequest/repositoryNames
:cognitect.aws.ecr-public/RepositoryNameList)
(s/def
:cognitect.aws.ecr-public.DescribeRepositoriesRequest/nextToken
:cognitect.aws.ecr-public/NextToken)
(s/def
:cognitect.aws.ecr-public.DescribeRepositoriesRequest/maxResults
:cognitect.aws.ecr-public/MaxResults)
(s/def
:cognitect.aws.ecr-public.RepositoryCatalogDataInput/description
:cognitect.aws.ecr-public/RepositoryDescription)
(s/def
:cognitect.aws.ecr-public.RepositoryCatalogDataInput/architectures
:cognitect.aws.ecr-public/ArchitectureList)
(s/def
:cognitect.aws.ecr-public.RepositoryCatalogDataInput/operatingSystems
:cognitect.aws.ecr-public/OperatingSystemList)
(s/def
:cognitect.aws.ecr-public.RepositoryCatalogDataInput/logoImageBlob
:cognitect.aws.ecr-public/LogoImageBlob)
(s/def
:cognitect.aws.ecr-public.RepositoryCatalogDataInput/aboutText
:cognitect.aws.ecr-public/AboutText)
(s/def
:cognitect.aws.ecr-public.RepositoryCatalogDataInput/usageText
:cognitect.aws.ecr-public/UsageText)
(s/def
:cognitect.aws.ecr-public.GetRepositoryCatalogDataResponse/catalogData
:cognitect.aws.ecr-public/RepositoryCatalogData)
(s/def
:cognitect.aws.ecr-public.RegistryCatalogData/displayName
:cognitect.aws.ecr-public/RegistryDisplayName)
(s/def
:cognitect.aws.ecr-public.UploadLayerPartResponse/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.UploadLayerPartResponse/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.UploadLayerPartResponse/uploadId
:cognitect.aws.ecr-public/UploadId)
(s/def
:cognitect.aws.ecr-public.UploadLayerPartResponse/lastByteReceived
:cognitect.aws.ecr-public/PartSize)
(s/def
:cognitect.aws.ecr-public.DescribeImagesRequest/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.DescribeImagesRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.DescribeImagesRequest/imageIds
:cognitect.aws.ecr-public/ImageIdentifierList)
(s/def
:cognitect.aws.ecr-public.DescribeImagesRequest/nextToken
:cognitect.aws.ecr-public/NextToken)
(s/def
:cognitect.aws.ecr-public.DescribeImagesRequest/maxResults
:cognitect.aws.ecr-public/MaxResults)
(s/def :cognitect.aws.ecr-public.Tag/Key :cognitect.aws.ecr-public/TagKey)
(s/def :cognitect.aws.ecr-public.Tag/Value :cognitect.aws.ecr-public/TagValue)
(s/def
:cognitect.aws.ecr-public.LayerPartTooSmallException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.DeleteRepositoryResponse/repository
:cognitect.aws.ecr-public/Repository)
(s/def
:cognitect.aws.ecr-public.GetRepositoryPolicyResponse/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.GetRepositoryPolicyResponse/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.GetRepositoryPolicyResponse/policyText
:cognitect.aws.ecr-public/RepositoryPolicyText)
(s/def
:cognitect.aws.ecr-public.DescribeRegistriesRequest/nextToken
:cognitect.aws.ecr-public/NextToken)
(s/def
:cognitect.aws.ecr-public.DescribeRegistriesRequest/maxResults
:cognitect.aws.ecr-public/MaxResults)
(s/def
:cognitect.aws.ecr-public.LayersNotFoundException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.UnsupportedCommandException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def :cognitect.aws.ecr-public.Image/registryId :cognitect.aws.ecr-public/RegistryIdOrAlias)
(s/def :cognitect.aws.ecr-public.Image/repositoryName :cognitect.aws.ecr-public/RepositoryName)
(s/def :cognitect.aws.ecr-public.Image/imageId :cognitect.aws.ecr-public/ImageIdentifier)
(s/def :cognitect.aws.ecr-public.Image/imageManifest :cognitect.aws.ecr-public/ImageManifest)
(s/def :cognitect.aws.ecr-public.Image/imageManifestMediaType :cognitect.aws.ecr-public/MediaType)
(s/def
:cognitect.aws.ecr-public.CompleteLayerUploadResponse/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.CompleteLayerUploadResponse/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.CompleteLayerUploadResponse/uploadId
:cognitect.aws.ecr-public/UploadId)
(s/def
:cognitect.aws.ecr-public.CompleteLayerUploadResponse/layerDigest
:cognitect.aws.ecr-public/LayerDigest)
(s/def
:cognitect.aws.ecr-public.ImageAlreadyExistsException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def :cognitect.aws.ecr-public.ImageTagDetail/imageTag :cognitect.aws.ecr-public/ImageTag)
(s/def
:cognitect.aws.ecr-public.ImageTagDetail/createdAt
:cognitect.aws.ecr-public/CreationTimestamp)
(s/def
:cognitect.aws.ecr-public.ImageTagDetail/imageDetail
:cognitect.aws.ecr-public/ReferencedImageDetail)
(s/def
:cognitect.aws.ecr-public.RepositoryNotEmptyException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.ReferencedImageDetail/imageDigest
:cognitect.aws.ecr-public/ImageDigest)
(s/def
:cognitect.aws.ecr-public.ReferencedImageDetail/imageSizeInBytes
:cognitect.aws.ecr-public/ImageSizeInBytes)
(s/def
:cognitect.aws.ecr-public.ReferencedImageDetail/imagePushedAt
:cognitect.aws.ecr-public/PushTimestamp)
(s/def
:cognitect.aws.ecr-public.ReferencedImageDetail/imageManifestMediaType
:cognitect.aws.ecr-public/MediaType)
(s/def
:cognitect.aws.ecr-public.ReferencedImageDetail/artifactMediaType
:cognitect.aws.ecr-public/MediaType)
(s/def
:cognitect.aws.ecr-public.ImageDigestDoesNotMatchException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.RegistryNotFoundException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.PutRepositoryCatalogDataResponse/catalogData
:cognitect.aws.ecr-public/RepositoryCatalogData)
(s/def
:cognitect.aws.ecr-public.LayerFailure/layerDigest
:cognitect.aws.ecr-public/BatchedOperationLayerDigest)
(s/def
:cognitect.aws.ecr-public.LayerFailure/failureCode
:cognitect.aws.ecr-public/LayerFailureCode)
(s/def
:cognitect.aws.ecr-public.LayerFailure/failureReason
:cognitect.aws.ecr-public/LayerFailureReason)
(s/def
:cognitect.aws.ecr-public.RepositoryCatalogDataNotFoundException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.GetRegistryCatalogDataResponse/registryCatalogData
:cognitect.aws.ecr-public/RegistryCatalogData)
(s/def
:cognitect.aws.ecr-public.SetRepositoryPolicyRequest/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.SetRepositoryPolicyRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.SetRepositoryPolicyRequest/policyText
:cognitect.aws.ecr-public/RepositoryPolicyText)
(s/def
:cognitect.aws.ecr-public.SetRepositoryPolicyRequest/force
:cognitect.aws.ecr-public/ForceFlag)
(s/def
:cognitect.aws.ecr-public.RepositoryNotFoundException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.DescribeRegistriesResponse/registries
:cognitect.aws.ecr-public/RegistryList)
(s/def
:cognitect.aws.ecr-public.DescribeRegistriesResponse/nextToken
:cognitect.aws.ecr-public/NextToken)
(s/def
:cognitect.aws.ecr-public.DeleteRepositoryPolicyResponse/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.DeleteRepositoryPolicyResponse/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.DeleteRepositoryPolicyResponse/policyText
:cognitect.aws.ecr-public/RepositoryPolicyText)
(s/def
:cognitect.aws.ecr-public.TooManyTagsException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.PutRepositoryCatalogDataRequest/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.PutRepositoryCatalogDataRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.PutRepositoryCatalogDataRequest/catalogData
:cognitect.aws.ecr-public/RepositoryCatalogDataInput)
(s/def
:cognitect.aws.ecr-public.PutRegistryCatalogDataResponse/registryCatalogData
:cognitect.aws.ecr-public/RegistryCatalogData)
(s/def
:cognitect.aws.ecr-public.UploadLayerPartRequest/registryId
:cognitect.aws.ecr-public/RegistryIdOrAlias)
(s/def
:cognitect.aws.ecr-public.UploadLayerPartRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def :cognitect.aws.ecr-public.UploadLayerPartRequest/uploadId :cognitect.aws.ecr-public/UploadId)
(s/def
:cognitect.aws.ecr-public.UploadLayerPartRequest/partFirstByte
:cognitect.aws.ecr-public/PartSize)
(s/def
:cognitect.aws.ecr-public.UploadLayerPartRequest/partLastByte
:cognitect.aws.ecr-public/PartSize)
(s/def
:cognitect.aws.ecr-public.UploadLayerPartRequest/layerPartBlob
:cognitect.aws.ecr-public/LayerPartBlob)
(s/def
:cognitect.aws.ecr-public.ListTagsForResourceRequest/resourceArn
:cognitect.aws.ecr-public/Arn)
(s/def
:cognitect.aws.ecr-public.ImageNotFoundException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.PutRegistryCatalogDataRequest/displayName
:cognitect.aws.ecr-public/RegistryDisplayName)
(s/def
:cognitect.aws.ecr-public.InvalidParameterException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.EmptyUploadException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.InvalidLayerException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.RepositoryCatalogData/description
:cognitect.aws.ecr-public/RepositoryDescription)
(s/def
:cognitect.aws.ecr-public.RepositoryCatalogData/architectures
:cognitect.aws.ecr-public/ArchitectureList)
(s/def
:cognitect.aws.ecr-public.RepositoryCatalogData/operatingSystems
:cognitect.aws.ecr-public/OperatingSystemList)
(s/def
:cognitect.aws.ecr-public.RepositoryCatalogData/logoUrl
:cognitect.aws.ecr-public/ResourceUrl)
(s/def
:cognitect.aws.ecr-public.RepositoryCatalogData/aboutText
:cognitect.aws.ecr-public/AboutText)
(s/def
:cognitect.aws.ecr-public.RepositoryCatalogData/usageText
:cognitect.aws.ecr-public/UsageText)
(s/def
:cognitect.aws.ecr-public.RepositoryCatalogData/marketplaceCertified
:cognitect.aws.ecr-public/MarketplaceCertified)
(s/def :cognitect.aws.ecr-public.Registry/registryId :cognitect.aws.ecr-public/RegistryId)
(s/def :cognitect.aws.ecr-public.Registry/registryArn :cognitect.aws.ecr-public/Arn)
(s/def :cognitect.aws.ecr-public.Registry/registryUri :cognitect.aws.ecr-public/Url)
(s/def :cognitect.aws.ecr-public.Registry/verified :cognitect.aws.ecr-public/RegistryVerified)
(s/def :cognitect.aws.ecr-public.Registry/aliases :cognitect.aws.ecr-public/RegistryAliasList)
(s/def
:cognitect.aws.ecr-public.CreateRepositoryResponse/repository
:cognitect.aws.ecr-public/Repository)
(s/def
:cognitect.aws.ecr-public.CreateRepositoryResponse/catalogData
:cognitect.aws.ecr-public/RepositoryCatalogData)
(s/def
:cognitect.aws.ecr-public.ReferencedImagesNotFoundException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.UploadNotFoundException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.InvalidTagParameterException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.InvalidLayerPartException/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.InvalidLayerPartException/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.InvalidLayerPartException/uploadId
:cognitect.aws.ecr-public/UploadId)
(s/def
:cognitect.aws.ecr-public.InvalidLayerPartException/lastValidByteReceived
:cognitect.aws.ecr-public/PartSize)
(s/def
:cognitect.aws.ecr-public.InvalidLayerPartException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.CompleteLayerUploadRequest/registryId
:cognitect.aws.ecr-public/RegistryIdOrAlias)
(s/def
:cognitect.aws.ecr-public.CompleteLayerUploadRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.CompleteLayerUploadRequest/uploadId
:cognitect.aws.ecr-public/UploadId)
(s/def
:cognitect.aws.ecr-public.CompleteLayerUploadRequest/layerDigests
:cognitect.aws.ecr-public/LayerDigestList)
(s/def
:cognitect.aws.ecr-public.BatchDeleteImageRequest/registryId
:cognitect.aws.ecr-public/RegistryIdOrAlias)
(s/def
:cognitect.aws.ecr-public.BatchDeleteImageRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.BatchDeleteImageRequest/imageIds
:cognitect.aws.ecr-public/ImageIdentifierList)
(s/def
:cognitect.aws.ecr-public.RepositoryPolicyNotFoundException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.BatchCheckLayerAvailabilityResponse/layers
:cognitect.aws.ecr-public/LayerList)
(s/def
:cognitect.aws.ecr-public.BatchCheckLayerAvailabilityResponse/failures
:cognitect.aws.ecr-public/LayerFailureList)
(s/def
:cognitect.aws.ecr-public.BatchCheckLayerAvailabilityRequest/registryId
:cognitect.aws.ecr-public/RegistryIdOrAlias)
(s/def
:cognitect.aws.ecr-public.BatchCheckLayerAvailabilityRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.BatchCheckLayerAvailabilityRequest/layerDigests
:cognitect.aws.ecr-public/BatchedOperationLayerDigestList)
(s/def
:cognitect.aws.ecr-public.InitiateLayerUploadRequest/registryId
:cognitect.aws.ecr-public/RegistryIdOrAlias)
(s/def
:cognitect.aws.ecr-public.InitiateLayerUploadRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def :cognitect.aws.ecr-public.ServerException/message :cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.GetRepositoryPolicyRequest/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.GetRepositoryPolicyRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.SetRepositoryPolicyResponse/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.SetRepositoryPolicyResponse/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.SetRepositoryPolicyResponse/policyText
:cognitect.aws.ecr-public/RepositoryPolicyText)
(s/def :cognitect.aws.ecr-public.ImageDetail/registryId :cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.ImageDetail/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def :cognitect.aws.ecr-public.ImageDetail/imageDigest :cognitect.aws.ecr-public/ImageDigest)
(s/def :cognitect.aws.ecr-public.ImageDetail/imageTags :cognitect.aws.ecr-public/ImageTagList)
(s/def
:cognitect.aws.ecr-public.ImageDetail/imageSizeInBytes
:cognitect.aws.ecr-public/ImageSizeInBytes)
(s/def :cognitect.aws.ecr-public.ImageDetail/imagePushedAt :cognitect.aws.ecr-public/PushTimestamp)
(s/def
:cognitect.aws.ecr-public.ImageDetail/imageManifestMediaType
:cognitect.aws.ecr-public/MediaType)
(s/def :cognitect.aws.ecr-public.ImageDetail/artifactMediaType :cognitect.aws.ecr-public/MediaType)
(s/def :cognitect.aws.ecr-public.UntagResourceRequest/resourceArn :cognitect.aws.ecr-public/Arn)
(s/def :cognitect.aws.ecr-public.UntagResourceRequest/tagKeys :cognitect.aws.ecr-public/TagKeyList)
(s/def
:cognitect.aws.ecr-public.GetRepositoryCatalogDataRequest/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.GetRepositoryCatalogDataRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.LayerAlreadyExistsException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.RepositoryAlreadyExistsException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def
:cognitect.aws.ecr-public.DescribeRepositoriesResponse/repositories
:cognitect.aws.ecr-public/RepositoryList)
(s/def
:cognitect.aws.ecr-public.DescribeRepositoriesResponse/nextToken
:cognitect.aws.ecr-public/NextToken)
(s/def
:cognitect.aws.ecr-public.GetAuthorizationTokenResponse/authorizationData
:cognitect.aws.ecr-public/AuthorizationData)
(s/def
:cognitect.aws.ecr-public.DescribeImagesResponse/imageDetails
:cognitect.aws.ecr-public/ImageDetailList)
(s/def
:cognitect.aws.ecr-public.DescribeImagesResponse/nextToken
:cognitect.aws.ecr-public/NextToken)
(s/def
:cognitect.aws.ecr-public.DeleteRepositoryRequest/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.DeleteRepositoryRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def :cognitect.aws.ecr-public.DeleteRepositoryRequest/force :cognitect.aws.ecr-public/ForceFlag)
(s/def
:cognitect.aws.ecr-public.DescribeImageTagsRequest/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.DescribeImageTagsRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.DescribeImageTagsRequest/nextToken
:cognitect.aws.ecr-public/NextToken)
(s/def
:cognitect.aws.ecr-public.DescribeImageTagsRequest/maxResults
:cognitect.aws.ecr-public/MaxResults)
(s/def
:cognitect.aws.ecr-public.AuthorizationData/authorizationToken
:cognitect.aws.ecr-public/Base64)
(s/def
:cognitect.aws.ecr-public.AuthorizationData/expiresAt
:cognitect.aws.ecr-public/ExpirationTimestamp)
(s/def :cognitect.aws.ecr-public.PutImageResponse/image :cognitect.aws.ecr-public/Image)
(s/def :cognitect.aws.ecr-public.Repository/repositoryArn :cognitect.aws.ecr-public/Arn)
(s/def :cognitect.aws.ecr-public.Repository/registryId :cognitect.aws.ecr-public/RegistryId)
(s/def :cognitect.aws.ecr-public.Repository/repositoryName :cognitect.aws.ecr-public/RepositoryName)
(s/def :cognitect.aws.ecr-public.Repository/repositoryUri :cognitect.aws.ecr-public/Url)
(s/def :cognitect.aws.ecr-public.Repository/createdAt :cognitect.aws.ecr-public/CreationTimestamp)
(s/def
:cognitect.aws.ecr-public.BatchDeleteImageResponse/imageIds
:cognitect.aws.ecr-public/ImageIdentifierList)
(s/def
:cognitect.aws.ecr-public.BatchDeleteImageResponse/failures
:cognitect.aws.ecr-public/ImageFailureList)
(s/def :cognitect.aws.ecr-public.ImageFailure/imageId :cognitect.aws.ecr-public/ImageIdentifier)
(s/def
:cognitect.aws.ecr-public.ImageFailure/failureCode
:cognitect.aws.ecr-public/ImageFailureCode)
(s/def
:cognitect.aws.ecr-public.ImageFailure/failureReason
:cognitect.aws.ecr-public/ImageFailureReason)
(s/def :cognitect.aws.ecr-public.ImageIdentifier/imageDigest :cognitect.aws.ecr-public/ImageDigest)
(s/def :cognitect.aws.ecr-public.ImageIdentifier/imageTag :cognitect.aws.ecr-public/ImageTag)
(s/def
:cognitect.aws.ecr-public.PutImageRequest/registryId
:cognitect.aws.ecr-public/RegistryIdOrAlias)
(s/def
:cognitect.aws.ecr-public.PutImageRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.PutImageRequest/imageManifest
:cognitect.aws.ecr-public/ImageManifest)
(s/def
:cognitect.aws.ecr-public.PutImageRequest/imageManifestMediaType
:cognitect.aws.ecr-public/MediaType)
(s/def :cognitect.aws.ecr-public.PutImageRequest/imageTag :cognitect.aws.ecr-public/ImageTag)
(s/def :cognitect.aws.ecr-public.PutImageRequest/imageDigest :cognitect.aws.ecr-public/ImageDigest)
(s/def :cognitect.aws.ecr-public.ListTagsForResourceResponse/tags :cognitect.aws.ecr-public/TagList)
(s/def
:cognitect.aws.ecr-public.LimitExceededException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def :cognitect.aws.ecr-public.Layer/layerDigest :cognitect.aws.ecr-public/LayerDigest)
(s/def
:cognitect.aws.ecr-public.Layer/layerAvailability
:cognitect.aws.ecr-public/LayerAvailability)
(s/def :cognitect.aws.ecr-public.Layer/layerSize :cognitect.aws.ecr-public/LayerSizeInBytes)
(s/def :cognitect.aws.ecr-public.Layer/mediaType :cognitect.aws.ecr-public/MediaType)
(s/def
:cognitect.aws.ecr-public.InitiateLayerUploadResponse/uploadId
:cognitect.aws.ecr-public/UploadId)
(s/def
:cognitect.aws.ecr-public.InitiateLayerUploadResponse/partSize
:cognitect.aws.ecr-public/PartSize)
(s/def
:cognitect.aws.ecr-public.CreateRepositoryRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
(s/def
:cognitect.aws.ecr-public.CreateRepositoryRequest/catalogData
:cognitect.aws.ecr-public/RepositoryCatalogDataInput)
(s/def :cognitect.aws.ecr-public.CreateRepositoryRequest/tags :cognitect.aws.ecr-public/TagList)
(s/def
:cognitect.aws.ecr-public.ImageTagAlreadyExistsException/message
:cognitect.aws.ecr-public/ExceptionMessage)
(s/def :cognitect.aws.ecr-public.RegistryAlias/name :cognitect.aws.ecr-public/RegistryAliasName)
(s/def :cognitect.aws.ecr-public.RegistryAlias/status :cognitect.aws.ecr-public/RegistryAliasStatus)
(s/def
:cognitect.aws.ecr-public.RegistryAlias/primaryRegistryAlias
:cognitect.aws.ecr-public/PrimaryRegistryAliasFlag)
(s/def
:cognitect.aws.ecr-public.RegistryAlias/defaultRegistryAlias
:cognitect.aws.ecr-public/DefaultRegistryAliasFlag)
(s/def
:cognitect.aws.ecr-public.DeleteRepositoryPolicyRequest/registryId
:cognitect.aws.ecr-public/RegistryId)
(s/def
:cognitect.aws.ecr-public.DeleteRepositoryPolicyRequest/repositoryName
:cognitect.aws.ecr-public/RepositoryName)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy