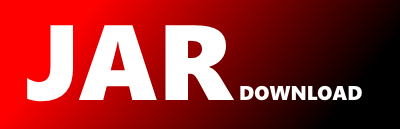
cognitect.aws.fms.specs.clj Maven / Gradle / Ivy
;; Copyright (c) Cognitect, Inc.
;; All rights reserved.
(ns cognitect.aws.fms.specs
(:require [clojure.spec.alpha :as s] [clojure.spec.gen.alpha :as gen]))
(s/def :cognitect.aws/client map?)
(s/def :core.async/channel any?)
(s/def
:cognitect.aws.fms/PolicyUpdateToken
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/ComplianceViolator
(s/keys
:opt-un
[:cognitect.aws.fms.ComplianceViolator/ViolationReason
:cognitect.aws.fms.ComplianceViolator/ResourceId
:cognitect.aws.fms.ComplianceViolator/ResourceType]))
(s/def
:cognitect.aws.fms/ResourceType
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/GetProtectionStatusRequest
(s/keys
:req-un
[:cognitect.aws.fms.GetProtectionStatusRequest/PolicyId]
:opt-un
[:cognitect.aws.fms.GetProtectionStatusRequest/EndTime
:cognitect.aws.fms.GetProtectionStatusRequest/StartTime
:cognitect.aws.fms.GetProtectionStatusRequest/NextToken
:cognitect.aws.fms.GetProtectionStatusRequest/MaxResults
:cognitect.aws.fms.GetProtectionStatusRequest/MemberAccountId]))
(s/def :cognitect.aws.fms/ResourceTypeList (s/coll-of :cognitect.aws.fms/ResourceType))
(s/def
:cognitect.aws.fms/ListMemberAccountsRequest
(s/keys
:opt-un
[:cognitect.aws.fms.ListMemberAccountsRequest/NextToken
:cognitect.aws.fms.ListMemberAccountsRequest/MaxResults]))
(s/def :cognitect.aws.fms/DependentServiceName string?)
(s/def :cognitect.aws.fms/TimeStamp inst?)
(s/def :cognitect.aws.fms/GetAdminAccountRequest (s/keys))
(s/def
:cognitect.aws.fms/PolicyComplianceStatus
(s/keys
:opt-un
[:cognitect.aws.fms.PolicyComplianceStatus/PolicyName
:cognitect.aws.fms.PolicyComplianceStatus/PolicyOwner
:cognitect.aws.fms.PolicyComplianceStatus/EvaluationResults
:cognitect.aws.fms.PolicyComplianceStatus/IssueInfoMap
:cognitect.aws.fms.PolicyComplianceStatus/PolicyId
:cognitect.aws.fms.PolicyComplianceStatus/MemberAccount
:cognitect.aws.fms.PolicyComplianceStatus/LastUpdated]))
(s/def
:cognitect.aws.fms/GetProtectionStatusResponse
(s/keys
:opt-un
[:cognitect.aws.fms.GetProtectionStatusResponse/ServiceType
:cognitect.aws.fms.GetProtectionStatusResponse/NextToken
:cognitect.aws.fms.GetProtectionStatusResponse/Data
:cognitect.aws.fms.GetProtectionStatusResponse/AdminAccountId]))
(s/def
:cognitect.aws.fms/IssueInfoMap
(s/map-of :cognitect.aws.fms/DependentServiceName :cognitect.aws.fms/DetailedInfo))
(s/def
:cognitect.aws.fms/PolicySummary
(s/keys
:opt-un
[:cognitect.aws.fms.PolicySummary/PolicyName
:cognitect.aws.fms.PolicySummary/RemediationEnabled
:cognitect.aws.fms.PolicySummary/PolicyId
:cognitect.aws.fms.PolicySummary/PolicyArn
:cognitect.aws.fms.PolicySummary/ResourceType
:cognitect.aws.fms.PolicySummary/SecurityServiceType]))
(s/def
:cognitect.aws.fms/ResourceTag
(s/keys
:req-un
[:cognitect.aws.fms.ResourceTag/Key]
:opt-un
[:cognitect.aws.fms.ResourceTag/Value]))
(s/def :cognitect.aws.fms/EvaluationResults (s/coll-of :cognitect.aws.fms/EvaluationResult))
(s/def
:cognitect.aws.fms/GetComplianceDetailResponse
(s/keys :opt-un [:cognitect.aws.fms.GetComplianceDetailResponse/PolicyComplianceDetail]))
(s/def
:cognitect.aws.fms/SecurityServicePolicyData
(s/keys
:req-un
[:cognitect.aws.fms.SecurityServicePolicyData/Type]
:opt-un
[:cognitect.aws.fms.SecurityServicePolicyData/ManagedServiceData]))
(s/def
:cognitect.aws.fms/GetNotificationChannelResponse
(s/keys
:opt-un
[:cognitect.aws.fms.GetNotificationChannelResponse/SnsRoleName
:cognitect.aws.fms.GetNotificationChannelResponse/SnsTopicArn]))
(s/def :cognitect.aws.fms/ComplianceViolators (s/coll-of :cognitect.aws.fms/ComplianceViolator))
(s/def
:cognitect.aws.fms/ResourceId
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/ListComplianceStatusResponse
(s/keys
:opt-un
[:cognitect.aws.fms.ListComplianceStatusResponse/NextToken
:cognitect.aws.fms.ListComplianceStatusResponse/PolicyComplianceStatusList]))
(s/def
:cognitect.aws.fms/DetailedInfo
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def :cognitect.aws.fms/Boolean boolean?)
(s/def :cognitect.aws.fms/ViolationReason string?)
(s/def
:cognitect.aws.fms/GetAdminAccountResponse
(s/keys
:opt-un
[:cognitect.aws.fms.GetAdminAccountResponse/RoleStatus
:cognitect.aws.fms.GetAdminAccountResponse/AdminAccount]))
(s/def
:cognitect.aws.fms/CustomerPolicyScopeId
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/TagValue
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/ListPoliciesResponse
(s/keys
:opt-un
[:cognitect.aws.fms.ListPoliciesResponse/NextToken
:cognitect.aws.fms.ListPoliciesResponse/PolicyList]))
(s/def
:cognitect.aws.fms/EvaluationResult
(s/keys
:opt-un
[:cognitect.aws.fms.EvaluationResult/ComplianceStatus
:cognitect.aws.fms.EvaluationResult/ViolatorCount
:cognitect.aws.fms.EvaluationResult/EvaluationLimitExceeded]))
(s/def
:cognitect.aws.fms/PolicyComplianceDetail
(s/keys
:opt-un
[:cognitect.aws.fms.PolicyComplianceDetail/PolicyOwner
:cognitect.aws.fms.PolicyComplianceDetail/ExpiredAt
:cognitect.aws.fms.PolicyComplianceDetail/IssueInfoMap
:cognitect.aws.fms.PolicyComplianceDetail/PolicyId
:cognitect.aws.fms.PolicyComplianceDetail/Violators
:cognitect.aws.fms.PolicyComplianceDetail/MemberAccount
:cognitect.aws.fms.PolicyComplianceDetail/EvaluationLimitExceeded]))
(s/def :cognitect.aws.fms/PolicyComplianceStatusType string?)
(s/def
:cognitect.aws.fms/AssociateAdminAccountRequest
(s/keys :req-un [:cognitect.aws.fms.AssociateAdminAccountRequest/AdminAccount]))
(s/def :cognitect.aws.fms/SecurityServiceType string?)
(s/def :cognitect.aws.fms/ProtectionData string?)
(s/def
:cognitect.aws.fms/ListComplianceStatusRequest
(s/keys
:req-un
[:cognitect.aws.fms.ListComplianceStatusRequest/PolicyId]
:opt-un
[:cognitect.aws.fms.ListComplianceStatusRequest/NextToken
:cognitect.aws.fms.ListComplianceStatusRequest/MaxResults]))
(s/def
:cognitect.aws.fms/CustomerPolicyScopeIdList
(s/coll-of :cognitect.aws.fms/CustomerPolicyScopeId))
(s/def :cognitect.aws.fms/MemberAccounts (s/coll-of :cognitect.aws.fms/AWSAccountId))
(s/def
:cognitect.aws.fms/GetComplianceDetailRequest
(s/keys
:req-un
[:cognitect.aws.fms.GetComplianceDetailRequest/PolicyId
:cognitect.aws.fms.GetComplianceDetailRequest/MemberAccount]))
(s/def
:cognitect.aws.fms/ManagedServiceData
(s/spec
(s/and string? #(<= 1 (count %) 1024))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 1024) #(gen/vector (gen/char-alpha) %))))))
(s/def :cognitect.aws.fms/PolicySummaryList (s/coll-of :cognitect.aws.fms/PolicySummary))
(s/def
:cognitect.aws.fms/ListPoliciesRequest
(s/keys
:opt-un
[:cognitect.aws.fms.ListPoliciesRequest/NextToken
:cognitect.aws.fms.ListPoliciesRequest/MaxResults]))
(s/def
:cognitect.aws.fms/CustomerPolicyScopeMap
(s/map-of
:cognitect.aws.fms/CustomerPolicyScopeIdType
:cognitect.aws.fms/CustomerPolicyScopeIdList))
(s/def
:cognitect.aws.fms/PolicyComplianceStatusList
(s/coll-of :cognitect.aws.fms/PolicyComplianceStatus))
(s/def
:cognitect.aws.fms/PutPolicyRequest
(s/keys :req-un [:cognitect.aws.fms.PutPolicyRequest/Policy]))
(s/def
:cognitect.aws.fms/GetPolicyResponse
(s/keys
:opt-un
[:cognitect.aws.fms.GetPolicyResponse/PolicyArn :cognitect.aws.fms.GetPolicyResponse/Policy]))
(s/def
:cognitect.aws.fms/PutNotificationChannelRequest
(s/keys
:req-un
[:cognitect.aws.fms.PutNotificationChannelRequest/SnsTopicArn
:cognitect.aws.fms.PutNotificationChannelRequest/SnsRoleName]))
(s/def
:cognitect.aws.fms/TagKey
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/PolicyId
(s/spec #(re-matches (re-pattern "^[a-z0-9A-Z-]{36}$") %) :gen #(gen/string)))
(s/def :cognitect.aws.fms/GetNotificationChannelRequest (s/keys))
(s/def
:cognitect.aws.fms/ResourceCount
(s/spec (s/and int? #(<= 0 %)) :gen #(gen/choose 0 Long/MAX_VALUE)))
(s/def
:cognitect.aws.fms/DeletePolicyRequest
(s/keys
:req-un
[:cognitect.aws.fms.DeletePolicyRequest/PolicyId]
:opt-un
[:cognitect.aws.fms.DeletePolicyRequest/DeleteAllPolicyResources]))
(s/def
:cognitect.aws.fms/ResourceArn
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def :cognitect.aws.fms/AccountRoleStatus string?)
(s/def :cognitect.aws.fms/CustomerPolicyScopeIdType string?)
(s/def
:cognitect.aws.fms/ListMemberAccountsResponse
(s/keys
:opt-un
[:cognitect.aws.fms.ListMemberAccountsResponse/NextToken
:cognitect.aws.fms.ListMemberAccountsResponse/MemberAccounts]))
(s/def
:cognitect.aws.fms/PaginationMaxResults
(s/spec (s/and int? #(<= 1 % 100)) :gen #(gen/choose 1 100)))
(s/def :cognitect.aws.fms/DisassociateAdminAccountRequest (s/keys))
(s/def
:cognitect.aws.fms/AWSAccountId
(s/spec #(re-matches (re-pattern "^[0-9]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/ResourceName
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/PaginationToken
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/ResourceTags
(s/coll-of :cognitect.aws.fms/ResourceTag :min-count 0 :max-count 8))
(s/def
:cognitect.aws.fms/GetPolicyRequest
(s/keys :req-un [:cognitect.aws.fms.GetPolicyRequest/PolicyId]))
(s/def :cognitect.aws.fms/DeleteNotificationChannelRequest (s/keys))
(s/def
:cognitect.aws.fms/PutPolicyResponse
(s/keys
:opt-un
[:cognitect.aws.fms.PutPolicyResponse/PolicyArn :cognitect.aws.fms.PutPolicyResponse/Policy]))
(s/def
:cognitect.aws.fms/Policy
(s/keys
:req-un
[:cognitect.aws.fms.Policy/PolicyName
:cognitect.aws.fms.Policy/SecurityServicePolicyData
:cognitect.aws.fms.Policy/ResourceType
:cognitect.aws.fms.Policy/ExcludeResourceTags
:cognitect.aws.fms.Policy/RemediationEnabled]
:opt-un
[:cognitect.aws.fms.Policy/ResourceTags
:cognitect.aws.fms.Policy/IncludeMap
:cognitect.aws.fms.Policy/PolicyId
:cognitect.aws.fms.Policy/ResourceTypeList
:cognitect.aws.fms.Policy/ExcludeMap
:cognitect.aws.fms.Policy/PolicyUpdateToken]))
(s/def :cognitect.aws.fms.ComplianceViolator/ResourceId :cognitect.aws.fms/ResourceId)
(s/def :cognitect.aws.fms.ComplianceViolator/ViolationReason :cognitect.aws.fms/ViolationReason)
(s/def :cognitect.aws.fms.ComplianceViolator/ResourceType :cognitect.aws.fms/ResourceType)
(s/def :cognitect.aws.fms.GetProtectionStatusRequest/PolicyId :cognitect.aws.fms/PolicyId)
(s/def
:cognitect.aws.fms.GetProtectionStatusRequest/MemberAccountId
:cognitect.aws.fms/AWSAccountId)
(s/def :cognitect.aws.fms.GetProtectionStatusRequest/StartTime :cognitect.aws.fms/TimeStamp)
(s/def :cognitect.aws.fms.GetProtectionStatusRequest/EndTime :cognitect.aws.fms/TimeStamp)
(s/def :cognitect.aws.fms.GetProtectionStatusRequest/NextToken :cognitect.aws.fms/PaginationToken)
(s/def
:cognitect.aws.fms.GetProtectionStatusRequest/MaxResults
:cognitect.aws.fms/PaginationMaxResults)
(s/def :cognitect.aws.fms.ListMemberAccountsRequest/NextToken :cognitect.aws.fms/PaginationToken)
(s/def
:cognitect.aws.fms.ListMemberAccountsRequest/MaxResults
:cognitect.aws.fms/PaginationMaxResults)
(s/def :cognitect.aws.fms.PolicyComplianceStatus/PolicyOwner :cognitect.aws.fms/AWSAccountId)
(s/def :cognitect.aws.fms.PolicyComplianceStatus/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.PolicyComplianceStatus/PolicyName :cognitect.aws.fms/ResourceName)
(s/def :cognitect.aws.fms.PolicyComplianceStatus/MemberAccount :cognitect.aws.fms/AWSAccountId)
(s/def
:cognitect.aws.fms.PolicyComplianceStatus/EvaluationResults
:cognitect.aws.fms/EvaluationResults)
(s/def :cognitect.aws.fms.PolicyComplianceStatus/LastUpdated :cognitect.aws.fms/TimeStamp)
(s/def :cognitect.aws.fms.PolicyComplianceStatus/IssueInfoMap :cognitect.aws.fms/IssueInfoMap)
(s/def
:cognitect.aws.fms.GetProtectionStatusResponse/AdminAccountId
:cognitect.aws.fms/AWSAccountId)
(s/def
:cognitect.aws.fms.GetProtectionStatusResponse/ServiceType
:cognitect.aws.fms/SecurityServiceType)
(s/def :cognitect.aws.fms.GetProtectionStatusResponse/Data :cognitect.aws.fms/ProtectionData)
(s/def :cognitect.aws.fms.GetProtectionStatusResponse/NextToken :cognitect.aws.fms/PaginationToken)
(s/def :cognitect.aws.fms.PolicySummary/PolicyArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.PolicySummary/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.PolicySummary/PolicyName :cognitect.aws.fms/ResourceName)
(s/def :cognitect.aws.fms.PolicySummary/ResourceType :cognitect.aws.fms/ResourceType)
(s/def :cognitect.aws.fms.PolicySummary/SecurityServiceType :cognitect.aws.fms/SecurityServiceType)
(s/def :cognitect.aws.fms.PolicySummary/RemediationEnabled :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.ResourceTag/Key :cognitect.aws.fms/TagKey)
(s/def :cognitect.aws.fms.ResourceTag/Value :cognitect.aws.fms/TagValue)
(s/def
:cognitect.aws.fms.GetComplianceDetailResponse/PolicyComplianceDetail
:cognitect.aws.fms/PolicyComplianceDetail)
(s/def :cognitect.aws.fms.SecurityServicePolicyData/Type :cognitect.aws.fms/SecurityServiceType)
(s/def
:cognitect.aws.fms.SecurityServicePolicyData/ManagedServiceData
:cognitect.aws.fms/ManagedServiceData)
(s/def :cognitect.aws.fms.GetNotificationChannelResponse/SnsTopicArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.GetNotificationChannelResponse/SnsRoleName :cognitect.aws.fms/ResourceArn)
(s/def
:cognitect.aws.fms.ListComplianceStatusResponse/PolicyComplianceStatusList
:cognitect.aws.fms/PolicyComplianceStatusList)
(s/def :cognitect.aws.fms.ListComplianceStatusResponse/NextToken :cognitect.aws.fms/PaginationToken)
(s/def :cognitect.aws.fms.GetAdminAccountResponse/AdminAccount :cognitect.aws.fms/AWSAccountId)
(s/def :cognitect.aws.fms.GetAdminAccountResponse/RoleStatus :cognitect.aws.fms/AccountRoleStatus)
(s/def :cognitect.aws.fms.ListPoliciesResponse/PolicyList :cognitect.aws.fms/PolicySummaryList)
(s/def :cognitect.aws.fms.ListPoliciesResponse/NextToken :cognitect.aws.fms/PaginationToken)
(s/def
:cognitect.aws.fms.EvaluationResult/ComplianceStatus
:cognitect.aws.fms/PolicyComplianceStatusType)
(s/def :cognitect.aws.fms.EvaluationResult/ViolatorCount :cognitect.aws.fms/ResourceCount)
(s/def :cognitect.aws.fms.EvaluationResult/EvaluationLimitExceeded :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.PolicyComplianceDetail/PolicyOwner :cognitect.aws.fms/AWSAccountId)
(s/def :cognitect.aws.fms.PolicyComplianceDetail/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.PolicyComplianceDetail/MemberAccount :cognitect.aws.fms/AWSAccountId)
(s/def :cognitect.aws.fms.PolicyComplianceDetail/Violators :cognitect.aws.fms/ComplianceViolators)
(s/def :cognitect.aws.fms.PolicyComplianceDetail/EvaluationLimitExceeded :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.PolicyComplianceDetail/ExpiredAt :cognitect.aws.fms/TimeStamp)
(s/def :cognitect.aws.fms.PolicyComplianceDetail/IssueInfoMap :cognitect.aws.fms/IssueInfoMap)
(s/def :cognitect.aws.fms.AssociateAdminAccountRequest/AdminAccount :cognitect.aws.fms/AWSAccountId)
(s/def :cognitect.aws.fms.ListComplianceStatusRequest/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.ListComplianceStatusRequest/NextToken :cognitect.aws.fms/PaginationToken)
(s/def
:cognitect.aws.fms.ListComplianceStatusRequest/MaxResults
:cognitect.aws.fms/PaginationMaxResults)
(s/def :cognitect.aws.fms.GetComplianceDetailRequest/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.GetComplianceDetailRequest/MemberAccount :cognitect.aws.fms/AWSAccountId)
(s/def :cognitect.aws.fms.ListPoliciesRequest/NextToken :cognitect.aws.fms/PaginationToken)
(s/def :cognitect.aws.fms.ListPoliciesRequest/MaxResults :cognitect.aws.fms/PaginationMaxResults)
(s/def :cognitect.aws.fms.PutPolicyRequest/Policy :cognitect.aws.fms/Policy)
(s/def :cognitect.aws.fms.GetPolicyResponse/Policy :cognitect.aws.fms/Policy)
(s/def :cognitect.aws.fms.GetPolicyResponse/PolicyArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.PutNotificationChannelRequest/SnsTopicArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.PutNotificationChannelRequest/SnsRoleName :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.DeletePolicyRequest/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.DeletePolicyRequest/DeleteAllPolicyResources :cognitect.aws.fms/Boolean)
(s/def
:cognitect.aws.fms.ListMemberAccountsResponse/MemberAccounts
:cognitect.aws.fms/MemberAccounts)
(s/def :cognitect.aws.fms.ListMemberAccountsResponse/NextToken :cognitect.aws.fms/PaginationToken)
(s/def :cognitect.aws.fms.GetPolicyRequest/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.PutPolicyResponse/Policy :cognitect.aws.fms/Policy)
(s/def :cognitect.aws.fms.PutPolicyResponse/PolicyArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.Policy/PolicyUpdateToken :cognitect.aws.fms/PolicyUpdateToken)
(s/def :cognitect.aws.fms.Policy/ResourceType :cognitect.aws.fms/ResourceType)
(s/def :cognitect.aws.fms.Policy/ResourceTypeList :cognitect.aws.fms/ResourceTypeList)
(s/def :cognitect.aws.fms.Policy/ExcludeResourceTags :cognitect.aws.fms/Boolean)
(s/def
:cognitect.aws.fms.Policy/SecurityServicePolicyData
:cognitect.aws.fms/SecurityServicePolicyData)
(s/def :cognitect.aws.fms.Policy/IncludeMap :cognitect.aws.fms/CustomerPolicyScopeMap)
(s/def :cognitect.aws.fms.Policy/PolicyName :cognitect.aws.fms/ResourceName)
(s/def :cognitect.aws.fms.Policy/RemediationEnabled :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.Policy/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.Policy/ExcludeMap :cognitect.aws.fms/CustomerPolicyScopeMap)
(s/def :cognitect.aws.fms.Policy/ResourceTags :cognitect.aws.fms/ResourceTags)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy