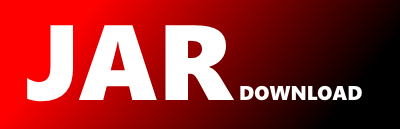
cognitect.aws.fms.specs.clj Maven / Gradle / Ivy
;; Copyright (c) Cognitect, Inc.
;; All rights reserved.
(ns cognitect.aws.fms.specs
(:require [clojure.spec.alpha :as s] [clojure.spec.gen.alpha :as gen]))
(s/def :cognitect.aws/client map?)
(s/def :core.async/channel any?)
(s/def
:cognitect.aws.fms/EC2CreateRouteTableAction
(s/keys
:req-un
[:cognitect.aws.fms.EC2CreateRouteTableAction/VpcId]
:opt-un
[:cognitect.aws.fms.EC2CreateRouteTableAction/Description]))
(s/def
:cognitect.aws.fms/ProtocolsListDataSummary
(s/keys
:opt-un
[:cognitect.aws.fms.ProtocolsListDataSummary/ListName
:cognitect.aws.fms.ProtocolsListDataSummary/ListId
:cognitect.aws.fms.ProtocolsListDataSummary/ProtocolsList
:cognitect.aws.fms.ProtocolsListDataSummary/ListArn]))
(s/def
:cognitect.aws.fms/PolicyUpdateToken
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/AwsEc2NetworkInterfaceViolation
(s/keys
:opt-un
[:cognitect.aws.fms.AwsEc2NetworkInterfaceViolation/ViolationTarget
:cognitect.aws.fms.AwsEc2NetworkInterfaceViolation/ViolatingSecurityGroups]))
(s/def
:cognitect.aws.fms/ListId
(s/spec #(re-matches (re-pattern "^[a-z0-9A-Z-]{36}$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/AppsListDataSummary
(s/keys
:opt-un
[:cognitect.aws.fms.AppsListDataSummary/ListName
:cognitect.aws.fms.AppsListDataSummary/ListId
:cognitect.aws.fms.AppsListDataSummary/AppsList
:cognitect.aws.fms.AppsListDataSummary/ListArn]))
(s/def
:cognitect.aws.fms/TagResourceRequest
(s/keys
:req-un
[:cognitect.aws.fms.TagResourceRequest/ResourceArn
:cognitect.aws.fms.TagResourceRequest/TagList]))
(s/def
:cognitect.aws.fms/ComplianceViolator
(s/keys
:opt-un
[:cognitect.aws.fms.ComplianceViolator/ViolationReason
:cognitect.aws.fms.ComplianceViolator/ResourceId
:cognitect.aws.fms.ComplianceViolator/ResourceType
:cognitect.aws.fms.ComplianceViolator/Metadata]))
(s/def
:cognitect.aws.fms/ResourceType
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/GetProtectionStatusRequest
(s/keys
:req-un
[:cognitect.aws.fms.GetProtectionStatusRequest/PolicyId]
:opt-un
[:cognitect.aws.fms.GetProtectionStatusRequest/EndTime
:cognitect.aws.fms.GetProtectionStatusRequest/StartTime
:cognitect.aws.fms.GetProtectionStatusRequest/NextToken
:cognitect.aws.fms.GetProtectionStatusRequest/MaxResults
:cognitect.aws.fms.GetProtectionStatusRequest/MemberAccountId]))
(s/def
:cognitect.aws.fms/PutAppsListResponse
(s/keys
:opt-un
[:cognitect.aws.fms.PutAppsListResponse/AppsListArn
:cognitect.aws.fms.PutAppsListResponse/AppsList]))
(s/def :cognitect.aws.fms/ResourceTypeList (s/coll-of :cognitect.aws.fms/ResourceType))
(s/def
:cognitect.aws.fms/ListMemberAccountsRequest
(s/keys
:opt-un
[:cognitect.aws.fms.ListMemberAccountsRequest/NextToken
:cognitect.aws.fms.ListMemberAccountsRequest/MaxResults]))
(s/def
:cognitect.aws.fms/EC2CopyRouteTableAction
(s/keys
:req-un
[:cognitect.aws.fms.EC2CopyRouteTableAction/VpcId
:cognitect.aws.fms.EC2CopyRouteTableAction/RouteTableId]
:opt-un
[:cognitect.aws.fms.EC2CopyRouteTableAction/Description]))
(s/def
:cognitect.aws.fms/FMSPolicyUpdateFirewallCreationConfigAction
(s/keys
:opt-un
[:cognitect.aws.fms.FMSPolicyUpdateFirewallCreationConfigAction/Description
:cognitect.aws.fms.FMSPolicyUpdateFirewallCreationConfigAction/FirewallCreationConfig]))
(s/def
:cognitect.aws.fms/NetworkFirewallActionList
(s/coll-of :cognitect.aws.fms/NetworkFirewallAction))
(s/def
:cognitect.aws.fms/DependentServiceName
(s/spec string? :gen #(s/gen #{"AWSSHIELD_ADVANCED" "AWSWAF" "AWSCONFIG" "AWSVPC"})))
(s/def
:cognitect.aws.fms/NetworkFirewallMissingFirewallViolation
(s/keys
:opt-un
[:cognitect.aws.fms.NetworkFirewallMissingFirewallViolation/AvailabilityZone
:cognitect.aws.fms.NetworkFirewallMissingFirewallViolation/TargetViolationReason
:cognitect.aws.fms.NetworkFirewallMissingFirewallViolation/ViolationTarget
:cognitect.aws.fms.NetworkFirewallMissingFirewallViolation/VPC]))
(s/def :cognitect.aws.fms/TimeStamp inst?)
(s/def
:cognitect.aws.fms/GetProtocolsListResponse
(s/keys
:opt-un
[:cognitect.aws.fms.GetProtocolsListResponse/ProtocolsListArn
:cognitect.aws.fms.GetProtocolsListResponse/ProtocolsList]))
(s/def
:cognitect.aws.fms/Tag
(s/keys :req-un [:cognitect.aws.fms.Tag/Key :cognitect.aws.fms.Tag/Value]))
(s/def
:cognitect.aws.fms/ListProtocolsListsResponse
(s/keys
:opt-un
[:cognitect.aws.fms.ListProtocolsListsResponse/NextToken
:cognitect.aws.fms.ListProtocolsListsResponse/ProtocolsLists]))
(s/def
:cognitect.aws.fms/NetworkFirewallUnexpectedFirewallRoutesViolation
(s/keys
:opt-un
[:cognitect.aws.fms.NetworkFirewallUnexpectedFirewallRoutesViolation/FirewallSubnetId
:cognitect.aws.fms.NetworkFirewallUnexpectedFirewallRoutesViolation/ViolatingRoutes
:cognitect.aws.fms.NetworkFirewallUnexpectedFirewallRoutesViolation/VpcId
:cognitect.aws.fms.NetworkFirewallUnexpectedFirewallRoutesViolation/FirewallEndpoint
:cognitect.aws.fms.NetworkFirewallUnexpectedFirewallRoutesViolation/RouteTableId]))
(s/def
:cognitect.aws.fms/PreviousAppsList
(s/map-of :cognitect.aws.fms/PreviousListVersion :cognitect.aws.fms/AppsList))
(s/def
:cognitect.aws.fms/NetworkFirewallPolicy
(s/keys :opt-un [:cognitect.aws.fms.NetworkFirewallPolicy/FirewallDeploymentModel]))
(s/def :cognitect.aws.fms/GetAdminAccountRequest (s/keys))
(s/def
:cognitect.aws.fms/OrderedRemediationActions
(s/coll-of :cognitect.aws.fms/RemediationActionWithOrder))
(s/def
:cognitect.aws.fms/RouteHasOutOfScopeEndpointViolation
(s/keys
:opt-un
[:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/FirewallSubnetId
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/SubnetId
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/CurrentInternetGatewayRouteTable
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/ViolatingRoutes
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/SubnetAvailabilityZoneId
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/InternetGatewayId
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/FirewallSubnetRoutes
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/VpcId
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/InternetGatewayRoutes
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/CurrentFirewallSubnetRouteTable
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/SubnetAvailabilityZone
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/RouteTableId]))
(s/def
:cognitect.aws.fms/GetAppsListRequest
(s/keys
:req-un
[:cognitect.aws.fms.GetAppsListRequest/ListId]
:opt-un
[:cognitect.aws.fms.GetAppsListRequest/DefaultList]))
(s/def :cognitect.aws.fms/TagResourceResponse (s/keys))
(s/def
:cognitect.aws.fms/PolicyComplianceStatus
(s/keys
:opt-un
[:cognitect.aws.fms.PolicyComplianceStatus/PolicyName
:cognitect.aws.fms.PolicyComplianceStatus/PolicyOwner
:cognitect.aws.fms.PolicyComplianceStatus/EvaluationResults
:cognitect.aws.fms.PolicyComplianceStatus/IssueInfoMap
:cognitect.aws.fms.PolicyComplianceStatus/PolicyId
:cognitect.aws.fms.PolicyComplianceStatus/MemberAccount
:cognitect.aws.fms.PolicyComplianceStatus/LastUpdated]))
(s/def
:cognitect.aws.fms/LengthBoundedStringList
(s/coll-of :cognitect.aws.fms/LengthBoundedString))
(s/def
:cognitect.aws.fms/IPPortNumber
(s/spec (s/and int? #(<= 0 % 65535)) :gen #(gen/choose 0 65535)))
(s/def
:cognitect.aws.fms/PossibleRemediationAction
(s/keys
:req-un
[:cognitect.aws.fms.PossibleRemediationAction/OrderedRemediationActions]
:opt-un
[:cognitect.aws.fms.PossibleRemediationAction/Description
:cognitect.aws.fms.PossibleRemediationAction/IsDefaultAction]))
(s/def
:cognitect.aws.fms/DnsRuleGroupPriority
(s/spec (s/and int? #(<= 0 % 10000)) :gen #(gen/choose 0 10000)))
(s/def
:cognitect.aws.fms/ComplianceViolatorMetadata
(s/map-of :cognitect.aws.fms/LengthBoundedString :cognitect.aws.fms/LengthBoundedString))
(s/def
:cognitect.aws.fms/GetProtectionStatusResponse
(s/keys
:opt-un
[:cognitect.aws.fms.GetProtectionStatusResponse/ServiceType
:cognitect.aws.fms.GetProtectionStatusResponse/NextToken
:cognitect.aws.fms.GetProtectionStatusResponse/Data
:cognitect.aws.fms.GetProtectionStatusResponse/AdminAccountId]))
(s/def
:cognitect.aws.fms/TargetViolationReason
(s/spec #(re-matches (re-pattern "\\w+") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/IssueInfoMap
(s/map-of :cognitect.aws.fms/DependentServiceName :cognitect.aws.fms/DetailedInfo))
(s/def :cognitect.aws.fms/TagList (s/coll-of :cognitect.aws.fms/Tag :min-count 0 :max-count 200))
(s/def
:cognitect.aws.fms/PolicySummary
(s/keys
:opt-un
[:cognitect.aws.fms.PolicySummary/DeleteUnusedFMManagedResources
:cognitect.aws.fms.PolicySummary/PolicyName
:cognitect.aws.fms.PolicySummary/RemediationEnabled
:cognitect.aws.fms.PolicySummary/PolicyId
:cognitect.aws.fms.PolicySummary/PolicyArn
:cognitect.aws.fms.PolicySummary/ResourceType
:cognitect.aws.fms.PolicySummary/SecurityServiceType]))
(s/def
:cognitect.aws.fms/ResourceTag
(s/keys
:req-un
[:cognitect.aws.fms.ResourceTag/Key]
:opt-un
[:cognitect.aws.fms.ResourceTag/Value]))
(s/def :cognitect.aws.fms/EvaluationResults (s/coll-of :cognitect.aws.fms/EvaluationResult))
(s/def
:cognitect.aws.fms/GetComplianceDetailResponse
(s/keys :opt-un [:cognitect.aws.fms.GetComplianceDetailResponse/PolicyComplianceDetail]))
(s/def
:cognitect.aws.fms/PolicyOption
(s/keys :opt-un [:cognitect.aws.fms.PolicyOption/NetworkFirewallPolicy]))
(s/def
:cognitect.aws.fms/EC2CreateRouteAction
(s/keys
:req-un
[:cognitect.aws.fms.EC2CreateRouteAction/RouteTableId]
:opt-un
[:cognitect.aws.fms.EC2CreateRouteAction/VpcEndpointId
:cognitect.aws.fms.EC2CreateRouteAction/DestinationIpv6CidrBlock
:cognitect.aws.fms.EC2CreateRouteAction/DestinationCidrBlock
:cognitect.aws.fms.EC2CreateRouteAction/Description
:cognitect.aws.fms.EC2CreateRouteAction/DestinationPrefixListId
:cognitect.aws.fms.EC2CreateRouteAction/GatewayId]))
(s/def :cognitect.aws.fms/RemediationActionType (s/spec string? :gen #(s/gen #{"MODIFY" "REMOVE"})))
(s/def
:cognitect.aws.fms/SecurityServicePolicyData
(s/keys
:req-un
[:cognitect.aws.fms.SecurityServicePolicyData/Type]
:opt-un
[:cognitect.aws.fms.SecurityServicePolicyData/PolicyOption
:cognitect.aws.fms.SecurityServicePolicyData/ManagedServiceData]))
(s/def
:cognitect.aws.fms/EC2ReplaceRouteTableAssociationAction
(s/keys
:req-un
[:cognitect.aws.fms.EC2ReplaceRouteTableAssociationAction/AssociationId
:cognitect.aws.fms.EC2ReplaceRouteTableAssociationAction/RouteTableId]
:opt-un
[:cognitect.aws.fms.EC2ReplaceRouteTableAssociationAction/Description]))
(s/def
:cognitect.aws.fms/Route
(s/keys
:opt-un
[:cognitect.aws.fms.Route/TargetType
:cognitect.aws.fms.Route/Destination
:cognitect.aws.fms.Route/Target
:cognitect.aws.fms.Route/DestinationType]))
(s/def
:cognitect.aws.fms/GetNotificationChannelResponse
(s/keys
:opt-un
[:cognitect.aws.fms.GetNotificationChannelResponse/SnsRoleName
:cognitect.aws.fms.GetNotificationChannelResponse/SnsTopicArn]))
(s/def :cognitect.aws.fms/ComplianceViolators (s/coll-of :cognitect.aws.fms/ComplianceViolator))
(s/def
:cognitect.aws.fms/DnsDuplicateRuleGroupViolation
(s/keys
:opt-un
[:cognitect.aws.fms.DnsDuplicateRuleGroupViolation/ViolationTargetDescription
:cognitect.aws.fms.DnsDuplicateRuleGroupViolation/ViolationTarget]))
(s/def :cognitect.aws.fms/ExpectedRoutes (s/coll-of :cognitect.aws.fms/ExpectedRoute))
(s/def
:cognitect.aws.fms/ResourceId
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/ResourceTagKey
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def :cognitect.aws.fms/StatefulRuleGroupList (s/coll-of :cognitect.aws.fms/StatefulRuleGroup))
(s/def
:cognitect.aws.fms/ListComplianceStatusResponse
(s/keys
:opt-un
[:cognitect.aws.fms.ListComplianceStatusResponse/NextToken
:cognitect.aws.fms.ListComplianceStatusResponse/PolicyComplianceStatusList]))
(s/def
:cognitect.aws.fms/ListAppsListsRequest
(s/keys
:req-un
[:cognitect.aws.fms.ListAppsListsRequest/MaxResults]
:opt-un
[:cognitect.aws.fms.ListAppsListsRequest/DefaultLists
:cognitect.aws.fms.ListAppsListsRequest/NextToken]))
(s/def
:cognitect.aws.fms/DnsRuleGroupPriorityConflictViolation
(s/keys
:opt-un
[:cognitect.aws.fms.DnsRuleGroupPriorityConflictViolation/UnavailablePriorities
:cognitect.aws.fms.DnsRuleGroupPriorityConflictViolation/ViolationTargetDescription
:cognitect.aws.fms.DnsRuleGroupPriorityConflictViolation/ConflictingPolicyId
:cognitect.aws.fms.DnsRuleGroupPriorityConflictViolation/ViolationTarget
:cognitect.aws.fms.DnsRuleGroupPriorityConflictViolation/ConflictingPriority]))
(s/def
:cognitect.aws.fms/DestinationType
(s/spec string? :gen #(s/gen #{"IPV4" "PREFIX_LIST" "IPV6"})))
(s/def
:cognitect.aws.fms/ListAppsListsResponse
(s/keys
:opt-un
[:cognitect.aws.fms.ListAppsListsResponse/NextToken
:cognitect.aws.fms.ListAppsListsResponse/AppsLists]))
(s/def
:cognitect.aws.fms/EC2DeleteRouteAction
(s/keys
:req-un
[:cognitect.aws.fms.EC2DeleteRouteAction/RouteTableId]
:opt-un
[:cognitect.aws.fms.EC2DeleteRouteAction/DestinationIpv6CidrBlock
:cognitect.aws.fms.EC2DeleteRouteAction/DestinationCidrBlock
:cognitect.aws.fms.EC2DeleteRouteAction/Description
:cognitect.aws.fms.EC2DeleteRouteAction/DestinationPrefixListId]))
(s/def
:cognitect.aws.fms/PossibleRemediationActions
(s/keys
:opt-un
[:cognitect.aws.fms.PossibleRemediationActions/Actions
:cognitect.aws.fms.PossibleRemediationActions/Description]))
(s/def
:cognitect.aws.fms/DetailedInfo
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=,+\\-@]*)$") %) :gen #(gen/string)))
(s/def :cognitect.aws.fms/Boolean boolean?)
(s/def
:cognitect.aws.fms/NetworkFirewallResourceName
(s/spec #(re-matches (re-pattern "^[a-zA-Z0-9-]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/ExpectedRoute
(s/keys
:opt-un
[:cognitect.aws.fms.ExpectedRoute/IpV4Cidr
:cognitect.aws.fms.ExpectedRoute/PrefixListId
:cognitect.aws.fms.ExpectedRoute/AllowedTargets
:cognitect.aws.fms.ExpectedRoute/ContributingSubnets
:cognitect.aws.fms.ExpectedRoute/RouteTableId
:cognitect.aws.fms.ExpectedRoute/IpV6Cidr]))
(s/def
:cognitect.aws.fms/NetworkFirewallInternetTrafficNotInspectedViolation
(s/keys
:opt-un
[:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/FirewallSubnetId
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/SubnetId
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/CurrentInternetGatewayRouteTable
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/ViolatingRoutes
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/ActualFirewallSubnetRoutes
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/InternetGatewayId
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/VpcId
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/ExpectedFirewallSubnetRoutes
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/CurrentFirewallSubnetRouteTable
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/SubnetAvailabilityZone
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/ExpectedInternetGatewayRoutes
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/RouteTableId
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/IsRouteTableUsedInDifferentAZ
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/ExpectedFirewallEndpoint
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/ActualInternetGatewayRoutes]))
(s/def
:cognitect.aws.fms/ResourceViolation
(s/keys
:opt-un
[:cognitect.aws.fms.ResourceViolation/AwsEc2NetworkInterfaceViolation
:cognitect.aws.fms.ResourceViolation/NetworkFirewallPolicyModifiedViolation
:cognitect.aws.fms.ResourceViolation/AwsEc2InstanceViolation
:cognitect.aws.fms.ResourceViolation/DnsRuleGroupLimitExceededViolation
:cognitect.aws.fms.ResourceViolation/NetworkFirewallUnexpectedFirewallRoutesViolation
:cognitect.aws.fms.ResourceViolation/AwsVPCSecurityGroupViolation
:cognitect.aws.fms.ResourceViolation/NetworkFirewallUnexpectedGatewayRoutesViolation
:cognitect.aws.fms.ResourceViolation/PossibleRemediationActions
:cognitect.aws.fms.ResourceViolation/NetworkFirewallMissingSubnetViolation
:cognitect.aws.fms.ResourceViolation/NetworkFirewallInternetTrafficNotInspectedViolation
:cognitect.aws.fms.ResourceViolation/RouteHasOutOfScopeEndpointViolation
:cognitect.aws.fms.ResourceViolation/DnsRuleGroupPriorityConflictViolation
:cognitect.aws.fms.ResourceViolation/NetworkFirewallMissingFirewallViolation
:cognitect.aws.fms.ResourceViolation/DnsDuplicateRuleGroupViolation
:cognitect.aws.fms.ResourceViolation/NetworkFirewallMissingExpectedRTViolation
:cognitect.aws.fms.ResourceViolation/NetworkFirewallInvalidRouteConfigurationViolation
:cognitect.aws.fms.ResourceViolation/NetworkFirewallBlackHoleRouteDetectedViolation
:cognitect.aws.fms.ResourceViolation/FirewallSubnetIsOutOfScopeViolation
:cognitect.aws.fms.ResourceViolation/NetworkFirewallMissingExpectedRoutesViolation]))
(s/def :cognitect.aws.fms/StatelessRuleGroupList (s/coll-of :cognitect.aws.fms/StatelessRuleGroup))
(s/def
:cognitect.aws.fms/ResourceTagValue
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/ViolationReason
(s/spec
string?
:gen
#(s/gen
#{"INVALID_ROUTE_CONFIGURATION" "FIREWALL_SUBNET_MISSING_EXPECTED_ROUTE"
"BLACK_HOLE_ROUTE_DETECTED" "RESOURCE_INCORRECT_WEB_ACL" "WEB_ACL_MISSING_RULE_GROUP"
"MISSING_FIREWALL_SUBNET_IN_AZ" "FIREWALL_SUBNET_IS_OUT_OF_SCOPE"
"RESOURCE_MISSING_WEB_ACL" "INTERNET_GATEWAY_MISSING_EXPECTED_ROUTE"
"UNEXPECTED_FIREWALL_ROUTES" "RESOURCE_MISSING_WEB_ACL_OR_SHIELD_PROTECTION"
"MISSING_TARGET_GATEWAY" "RESOURCE_MISSING_DNS_FIREWALL"
"RESOURCE_MISSING_SHIELD_PROTECTION" "SECURITY_GROUP_REDUNDANT"
"TRAFFIC_INSPECTION_CROSSES_AZ_BOUNDARY" "UNEXPECTED_TARGET_GATEWAY_ROUTES"
"FMS_CREATED_SECURITY_GROUP_EDITED" "ROUTE_HAS_OUT_OF_SCOPE_ENDPOINT"
"BLACK_HOLE_ROUTE_DETECTED_IN_FIREWALL_SUBNET" "NETWORK_FIREWALL_POLICY_MODIFIED"
"MISSING_FIREWALL" "MISSING_EXPECTED_ROUTE_TABLE" "SECURITY_GROUP_UNUSED"
"RESOURCE_MISSING_SECURITY_GROUP" "RESOURCE_VIOLATES_AUDIT_SECURITY_GROUP"
"INTERNET_TRAFFIC_NOT_INSPECTED"})))
(s/def
:cognitect.aws.fms/TargetType
(s/spec
string?
:gen
#(s/gen
#{"INSTANCE" "LOCAL_GATEWAY" "CARRIER_GATEWAY" "VPC_PEERING_CONNECTION" "VPC_ENDPOINT"
"NETWORK_INTERFACE" "GATEWAY" "EGRESS_ONLY_INTERNET_GATEWAY" "TRANSIT_GATEWAY"
"NAT_GATEWAY"})))
(s/def
:cognitect.aws.fms/ProtocolsListsData
(s/coll-of :cognitect.aws.fms/ProtocolsListDataSummary))
(s/def
:cognitect.aws.fms/AwsEc2InstanceViolation
(s/keys
:opt-un
[:cognitect.aws.fms.AwsEc2InstanceViolation/ViolationTarget
:cognitect.aws.fms.AwsEc2InstanceViolation/AwsEc2NetworkInterfaceViolations]))
(s/def
:cognitect.aws.fms/GetAdminAccountResponse
(s/keys
:opt-un
[:cognitect.aws.fms.GetAdminAccountResponse/RoleStatus
:cognitect.aws.fms.GetAdminAccountResponse/AdminAccount]))
(s/def :cognitect.aws.fms/Routes (s/coll-of :cognitect.aws.fms/Route))
(s/def
:cognitect.aws.fms/NetworkFirewallPolicyDescription
(s/keys
:opt-un
[:cognitect.aws.fms.NetworkFirewallPolicyDescription/StatelessRuleGroups
:cognitect.aws.fms.NetworkFirewallPolicyDescription/StatelessCustomActions
:cognitect.aws.fms.NetworkFirewallPolicyDescription/StatefulRuleGroups
:cognitect.aws.fms.NetworkFirewallPolicyDescription/StatelessDefaultActions
:cognitect.aws.fms.NetworkFirewallPolicyDescription/StatelessFragmentDefaultActions]))
(s/def :cognitect.aws.fms/PartialMatches (s/coll-of :cognitect.aws.fms/PartialMatch))
(s/def :cognitect.aws.fms/ResourceIdList (s/coll-of :cognitect.aws.fms/ResourceId))
(s/def
:cognitect.aws.fms/GetViolationDetailsRequest
(s/keys
:req-un
[:cognitect.aws.fms.GetViolationDetailsRequest/PolicyId
:cognitect.aws.fms.GetViolationDetailsRequest/MemberAccount
:cognitect.aws.fms.GetViolationDetailsRequest/ResourceId
:cognitect.aws.fms.GetViolationDetailsRequest/ResourceType]))
(s/def
:cognitect.aws.fms/CustomerPolicyScopeId
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/ListProtocolsListsRequest
(s/keys
:req-un
[:cognitect.aws.fms.ListProtocolsListsRequest/MaxResults]
:opt-un
[:cognitect.aws.fms.ListProtocolsListsRequest/DefaultLists
:cognitect.aws.fms.ListProtocolsListsRequest/NextToken]))
(s/def
:cognitect.aws.fms/RemediationActionDescription
(s/spec #(re-matches (re-pattern ".*") %) :gen #(gen/string)))
(s/def :cognitect.aws.fms/ResourceViolations (s/coll-of :cognitect.aws.fms/ResourceViolation))
(s/def
:cognitect.aws.fms/TagValue
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/RemediationActionWithOrder
(s/keys
:opt-un
[:cognitect.aws.fms.RemediationActionWithOrder/Order
:cognitect.aws.fms.RemediationActionWithOrder/RemediationAction]))
(s/def
:cognitect.aws.fms/ListPoliciesResponse
(s/keys
:opt-un
[:cognitect.aws.fms.ListPoliciesResponse/NextToken
:cognitect.aws.fms.ListPoliciesResponse/PolicyList]))
(s/def
:cognitect.aws.fms/EvaluationResult
(s/keys
:opt-un
[:cognitect.aws.fms.EvaluationResult/ComplianceStatus
:cognitect.aws.fms.EvaluationResult/ViolatorCount
:cognitect.aws.fms.EvaluationResult/EvaluationLimitExceeded]))
(s/def
:cognitect.aws.fms/ListTagsForResourceRequest
(s/keys :req-un [:cognitect.aws.fms.ListTagsForResourceRequest/ResourceArn]))
(s/def
:cognitect.aws.fms/PolicyComplianceDetail
(s/keys
:opt-un
[:cognitect.aws.fms.PolicyComplianceDetail/PolicyOwner
:cognitect.aws.fms.PolicyComplianceDetail/ExpiredAt
:cognitect.aws.fms.PolicyComplianceDetail/IssueInfoMap
:cognitect.aws.fms.PolicyComplianceDetail/PolicyId
:cognitect.aws.fms.PolicyComplianceDetail/Violators
:cognitect.aws.fms.PolicyComplianceDetail/MemberAccount
:cognitect.aws.fms.PolicyComplianceDetail/EvaluationLimitExceeded]))
(s/def
:cognitect.aws.fms/StatefulRuleGroup
(s/keys
:opt-un
[:cognitect.aws.fms.StatefulRuleGroup/ResourceId
:cognitect.aws.fms.StatefulRuleGroup/RuleGroupName]))
(s/def
:cognitect.aws.fms/PolicyComplianceStatusType
(s/spec string? :gen #(s/gen #{"NON_COMPLIANT" "COMPLIANT"})))
(s/def
:cognitect.aws.fms/NetworkFirewallInvalidRouteConfigurationViolation
(s/keys
:opt-un
[:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/CurrentInternetGatewayRouteTable
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/AffectedSubnets
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ViolatingRoute
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ActualFirewallSubnetId
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ActualFirewallSubnetRoutes
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ExpectedFirewallSubnetId
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/InternetGatewayId
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/VpcId
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ExpectedFirewallSubnetRoutes
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/CurrentFirewallSubnetRouteTable
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ExpectedInternetGatewayRoutes
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ActualFirewallEndpoint
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/RouteTableId
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/IsRouteTableUsedInDifferentAZ
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ExpectedFirewallEndpoint
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ActualInternetGatewayRoutes]))
(s/def :cognitect.aws.fms/AppsList (s/coll-of :cognitect.aws.fms/App))
(s/def
:cognitect.aws.fms/AssociateAdminAccountRequest
(s/keys :req-un [:cognitect.aws.fms.AssociateAdminAccountRequest/AdminAccount]))
(s/def :cognitect.aws.fms/UntagResourceResponse (s/keys))
(s/def
:cognitect.aws.fms/PreviousProtocolsList
(s/map-of :cognitect.aws.fms/PreviousListVersion :cognitect.aws.fms/ProtocolsList))
(s/def
:cognitect.aws.fms/SecurityGroupRemediationAction
(s/keys
:opt-un
[:cognitect.aws.fms.SecurityGroupRemediationAction/RemediationResult
:cognitect.aws.fms.SecurityGroupRemediationAction/Description
:cognitect.aws.fms.SecurityGroupRemediationAction/RemediationActionType
:cognitect.aws.fms.SecurityGroupRemediationAction/IsDefaultAction]))
(s/def
:cognitect.aws.fms/SecurityServiceType
(s/spec
string?
:gen
#(s/gen
#{"DNS_FIREWALL" "WAF" "SHIELD_ADVANCED" "SECURITY_GROUPS_USAGE_AUDIT" "WAFV2"
"NETWORK_FIREWALL" "SECURITY_GROUPS_COMMON" "SECURITY_GROUPS_CONTENT_AUDIT"})))
(s/def :cognitect.aws.fms/ProtectionData string?)
(s/def
:cognitect.aws.fms/NetworkFirewallMissingExpectedRTViolation
(s/keys
:opt-un
[:cognitect.aws.fms.NetworkFirewallMissingExpectedRTViolation/AvailabilityZone
:cognitect.aws.fms.NetworkFirewallMissingExpectedRTViolation/ExpectedRouteTable
:cognitect.aws.fms.NetworkFirewallMissingExpectedRTViolation/CurrentRouteTable
:cognitect.aws.fms.NetworkFirewallMissingExpectedRTViolation/ViolationTarget
:cognitect.aws.fms.NetworkFirewallMissingExpectedRTViolation/VPC]))
(s/def
:cognitect.aws.fms/ListComplianceStatusRequest
(s/keys
:req-un
[:cognitect.aws.fms.ListComplianceStatusRequest/PolicyId]
:opt-un
[:cognitect.aws.fms.ListComplianceStatusRequest/NextToken
:cognitect.aws.fms.ListComplianceStatusRequest/MaxResults]))
(s/def
:cognitect.aws.fms/PutProtocolsListResponse
(s/keys
:opt-un
[:cognitect.aws.fms.PutProtocolsListResponse/ProtocolsListArn
:cognitect.aws.fms.PutProtocolsListResponse/ProtocolsList]))
(s/def
:cognitect.aws.fms/GetViolationDetailsResponse
(s/keys :opt-un [:cognitect.aws.fms.GetViolationDetailsResponse/ViolationDetail]))
(s/def :cognitect.aws.fms/ReferenceRule string?)
(s/def
:cognitect.aws.fms/NetworkFirewallMissingExpectedRoutesViolation
(s/keys
:opt-un
[:cognitect.aws.fms.NetworkFirewallMissingExpectedRoutesViolation/VpcId
:cognitect.aws.fms.NetworkFirewallMissingExpectedRoutesViolation/ExpectedRoutes
:cognitect.aws.fms.NetworkFirewallMissingExpectedRoutesViolation/ViolationTarget]))
(s/def
:cognitect.aws.fms/ProtocolsListData
(s/keys
:req-un
[:cognitect.aws.fms.ProtocolsListData/ListName
:cognitect.aws.fms.ProtocolsListData/ProtocolsList]
:opt-un
[:cognitect.aws.fms.ProtocolsListData/ListId
:cognitect.aws.fms.ProtocolsListData/LastUpdateTime
:cognitect.aws.fms.ProtocolsListData/CreateTime
:cognitect.aws.fms.ProtocolsListData/PreviousProtocolsList
:cognitect.aws.fms.ProtocolsListData/ListUpdateToken]))
(s/def
:cognitect.aws.fms/CustomerPolicyScopeIdList
(s/coll-of :cognitect.aws.fms/CustomerPolicyScopeId))
(s/def
:cognitect.aws.fms/FirewallSubnetIsOutOfScopeViolation
(s/keys
:opt-un
[:cognitect.aws.fms.FirewallSubnetIsOutOfScopeViolation/FirewallSubnetId
:cognitect.aws.fms.FirewallSubnetIsOutOfScopeViolation/VpcEndpointId
:cognitect.aws.fms.FirewallSubnetIsOutOfScopeViolation/SubnetAvailabilityZoneId
:cognitect.aws.fms.FirewallSubnetIsOutOfScopeViolation/VpcId
:cognitect.aws.fms.FirewallSubnetIsOutOfScopeViolation/SubnetAvailabilityZone]))
(s/def
:cognitect.aws.fms/PutProtocolsListRequest
(s/keys
:req-un
[:cognitect.aws.fms.PutProtocolsListRequest/ProtocolsList]
:opt-un
[:cognitect.aws.fms.PutProtocolsListRequest/TagList]))
(s/def :cognitect.aws.fms/MemberAccounts (s/coll-of :cognitect.aws.fms/AWSAccountId))
(s/def
:cognitect.aws.fms/PreviousListVersion
(s/spec #(re-matches (re-pattern "^\\d{1,2}$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/PutAppsListRequest
(s/keys
:req-un
[:cognitect.aws.fms.PutAppsListRequest/AppsList]
:opt-un
[:cognitect.aws.fms.PutAppsListRequest/TagList]))
(s/def
:cognitect.aws.fms/GetAppsListResponse
(s/keys
:opt-un
[:cognitect.aws.fms.GetAppsListResponse/AppsListArn
:cognitect.aws.fms.GetAppsListResponse/AppsList]))
(s/def
:cognitect.aws.fms/GetComplianceDetailRequest
(s/keys
:req-un
[:cognitect.aws.fms.GetComplianceDetailRequest/PolicyId
:cognitect.aws.fms.GetComplianceDetailRequest/MemberAccount]))
(s/def
:cognitect.aws.fms/NetworkFirewallPolicyModifiedViolation
(s/keys
:opt-un
[:cognitect.aws.fms.NetworkFirewallPolicyModifiedViolation/CurrentPolicyDescription
:cognitect.aws.fms.NetworkFirewallPolicyModifiedViolation/ExpectedPolicyDescription
:cognitect.aws.fms.NetworkFirewallPolicyModifiedViolation/ViolationTarget]))
(s/def
:cognitect.aws.fms/ViolationTarget
(s/spec #(re-matches (re-pattern ".*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/ManagedServiceData
(s/spec #(re-matches (re-pattern "^((?!\\\\[nr]).)+") %) :gen #(gen/string)))
(s/def :cognitect.aws.fms/PolicySummaryList (s/coll-of :cognitect.aws.fms/PolicySummary))
(s/def
:cognitect.aws.fms/CIDR
(s/spec #(re-matches (re-pattern "[a-f0-9:./]+") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/ListPoliciesRequest
(s/keys
:opt-un
[:cognitect.aws.fms.ListPoliciesRequest/NextToken
:cognitect.aws.fms.ListPoliciesRequest/MaxResults]))
(s/def
:cognitect.aws.fms/CustomerPolicyScopeMap
(s/map-of
:cognitect.aws.fms/CustomerPolicyScopeIdType
:cognitect.aws.fms/CustomerPolicyScopeIdList))
(s/def
:cognitect.aws.fms/RemediationAction
(s/keys
:opt-un
[:cognitect.aws.fms.RemediationAction/EC2ReplaceRouteAction
:cognitect.aws.fms.RemediationAction/EC2CopyRouteTableAction
:cognitect.aws.fms.RemediationAction/EC2CreateRouteTableAction
:cognitect.aws.fms.RemediationAction/FMSPolicyUpdateFirewallCreationConfigAction
:cognitect.aws.fms.RemediationAction/Description
:cognitect.aws.fms.RemediationAction/EC2AssociateRouteTableAction
:cognitect.aws.fms.RemediationAction/EC2DeleteRouteAction
:cognitect.aws.fms.RemediationAction/EC2CreateRouteAction
:cognitect.aws.fms.RemediationAction/EC2ReplaceRouteTableAssociationAction]))
(s/def
:cognitect.aws.fms/PolicyComplianceStatusList
(s/coll-of :cognitect.aws.fms/PolicyComplianceStatus))
(s/def
:cognitect.aws.fms/ActionTarget
(s/keys
:opt-un
[:cognitect.aws.fms.ActionTarget/ResourceId :cognitect.aws.fms.ActionTarget/Description]))
(s/def
:cognitect.aws.fms/TargetViolationReasons
(s/coll-of :cognitect.aws.fms/TargetViolationReason))
(s/def
:cognitect.aws.fms/PutPolicyRequest
(s/keys
:req-un
[:cognitect.aws.fms.PutPolicyRequest/Policy]
:opt-un
[:cognitect.aws.fms.PutPolicyRequest/TagList]))
(s/def
:cognitect.aws.fms/PossibleRemediationActionList
(s/coll-of :cognitect.aws.fms/PossibleRemediationAction))
(s/def
:cognitect.aws.fms/GetPolicyResponse
(s/keys
:opt-un
[:cognitect.aws.fms.GetPolicyResponse/PolicyArn :cognitect.aws.fms.GetPolicyResponse/Policy]))
(s/def
:cognitect.aws.fms/AwsEc2NetworkInterfaceViolations
(s/coll-of :cognitect.aws.fms/AwsEc2NetworkInterfaceViolation))
(s/def
:cognitect.aws.fms/AppsListData
(s/keys
:req-un
[:cognitect.aws.fms.AppsListData/ListName :cognitect.aws.fms.AppsListData/AppsList]
:opt-un
[:cognitect.aws.fms.AppsListData/ListId
:cognitect.aws.fms.AppsListData/LastUpdateTime
:cognitect.aws.fms.AppsListData/CreateTime
:cognitect.aws.fms.AppsListData/PreviousAppsList
:cognitect.aws.fms.AppsListData/ListUpdateToken]))
(s/def
:cognitect.aws.fms/PutNotificationChannelRequest
(s/keys
:req-un
[:cognitect.aws.fms.PutNotificationChannelRequest/SnsTopicArn
:cognitect.aws.fms.PutNotificationChannelRequest/SnsRoleName]))
(s/def
:cognitect.aws.fms/GetProtocolsListRequest
(s/keys
:req-un
[:cognitect.aws.fms.GetProtocolsListRequest/ListId]
:opt-un
[:cognitect.aws.fms.GetProtocolsListRequest/DefaultList]))
(s/def
:cognitect.aws.fms/UntagResourceRequest
(s/keys
:req-un
[:cognitect.aws.fms.UntagResourceRequest/ResourceArn
:cognitect.aws.fms.UntagResourceRequest/TagKeys]))
(s/def
:cognitect.aws.fms/TagKey
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/StatelessRuleGroupPriority
(s/spec (s/and int? #(<= 1 % 65535)) :gen #(gen/choose 1 65535)))
(s/def :cognitect.aws.fms/FirewallDeploymentModel (s/spec string? :gen #(s/gen #{"CENTRALIZED"})))
(s/def
:cognitect.aws.fms/NetworkFirewallBlackHoleRouteDetectedViolation
(s/keys
:opt-un
[:cognitect.aws.fms.NetworkFirewallBlackHoleRouteDetectedViolation/ViolatingRoutes
:cognitect.aws.fms.NetworkFirewallBlackHoleRouteDetectedViolation/VpcId
:cognitect.aws.fms.NetworkFirewallBlackHoleRouteDetectedViolation/ViolationTarget
:cognitect.aws.fms.NetworkFirewallBlackHoleRouteDetectedViolation/RouteTableId]))
(s/def
:cognitect.aws.fms/SecurityGroupRuleDescription
(s/keys
:opt-un
[:cognitect.aws.fms.SecurityGroupRuleDescription/ToPort
:cognitect.aws.fms.SecurityGroupRuleDescription/PrefixListId
:cognitect.aws.fms.SecurityGroupRuleDescription/FromPort
:cognitect.aws.fms.SecurityGroupRuleDescription/Protocol
:cognitect.aws.fms.SecurityGroupRuleDescription/IPV4Range
:cognitect.aws.fms.SecurityGroupRuleDescription/IPV6Range]))
(s/def
:cognitect.aws.fms/BasicInteger
(s/spec (s/and int? #(<= -2147483648 % 2147483647)) :gen #(gen/choose -2147483648 2147483647)))
(s/def
:cognitect.aws.fms/PolicyId
(s/spec #(re-matches (re-pattern "^[a-z0-9A-Z-]{36}$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/EC2AssociateRouteTableAction
(s/keys
:req-un
[:cognitect.aws.fms.EC2AssociateRouteTableAction/RouteTableId]
:opt-un
[:cognitect.aws.fms.EC2AssociateRouteTableAction/SubnetId
:cognitect.aws.fms.EC2AssociateRouteTableAction/Description
:cognitect.aws.fms.EC2AssociateRouteTableAction/GatewayId]))
(s/def :cognitect.aws.fms/GetNotificationChannelRequest (s/keys))
(s/def
:cognitect.aws.fms/Protocol
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/ResourceCount
(s/spec (s/and int? #(<= 0 %)) :gen #(gen/choose 0 Long/MAX_VALUE)))
(s/def
:cognitect.aws.fms/DnsRuleGroupLimitExceededViolation
(s/keys
:opt-un
[:cognitect.aws.fms.DnsRuleGroupLimitExceededViolation/ViolationTargetDescription
:cognitect.aws.fms.DnsRuleGroupLimitExceededViolation/ViolationTarget
:cognitect.aws.fms.DnsRuleGroupLimitExceededViolation/NumberOfRuleGroupsAlreadyAssociated]))
(s/def
:cognitect.aws.fms/DeletePolicyRequest
(s/keys
:req-un
[:cognitect.aws.fms.DeletePolicyRequest/PolicyId]
:opt-un
[:cognitect.aws.fms.DeletePolicyRequest/DeleteAllPolicyResources]))
(s/def
:cognitect.aws.fms/DnsRuleGroupPriorities
(s/coll-of :cognitect.aws.fms/DnsRuleGroupPriority))
(s/def
:cognitect.aws.fms/SecurityGroupRemediationActions
(s/coll-of :cognitect.aws.fms/SecurityGroupRemediationAction))
(s/def
:cognitect.aws.fms/ResourceArn
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/EC2ReplaceRouteAction
(s/keys
:req-un
[:cognitect.aws.fms.EC2ReplaceRouteAction/RouteTableId]
:opt-un
[:cognitect.aws.fms.EC2ReplaceRouteAction/DestinationIpv6CidrBlock
:cognitect.aws.fms.EC2ReplaceRouteAction/DestinationCidrBlock
:cognitect.aws.fms.EC2ReplaceRouteAction/Description
:cognitect.aws.fms.EC2ReplaceRouteAction/DestinationPrefixListId
:cognitect.aws.fms.EC2ReplaceRouteAction/GatewayId]))
(s/def
:cognitect.aws.fms/AccountRoleStatus
(s/spec string? :gen #(s/gen #{"READY" "DELETING" "CREATING" "PENDING_DELETION" "DELETED"})))
(s/def
:cognitect.aws.fms/AwsVPCSecurityGroupViolation
(s/keys
:opt-un
[:cognitect.aws.fms.AwsVPCSecurityGroupViolation/PossibleSecurityGroupRemediationActions
:cognitect.aws.fms.AwsVPCSecurityGroupViolation/ViolationTargetDescription
:cognitect.aws.fms.AwsVPCSecurityGroupViolation/PartialMatches
:cognitect.aws.fms.AwsVPCSecurityGroupViolation/ViolationTarget]))
(s/def
:cognitect.aws.fms/NetworkFirewallUnexpectedGatewayRoutesViolation
(s/keys
:opt-un
[:cognitect.aws.fms.NetworkFirewallUnexpectedGatewayRoutesViolation/ViolatingRoutes
:cognitect.aws.fms.NetworkFirewallUnexpectedGatewayRoutesViolation/VpcId
:cognitect.aws.fms.NetworkFirewallUnexpectedGatewayRoutesViolation/GatewayId
:cognitect.aws.fms.NetworkFirewallUnexpectedGatewayRoutesViolation/RouteTableId]))
(s/def
:cognitect.aws.fms/CustomerPolicyScopeIdType
(s/spec string? :gen #(s/gen #{"ACCOUNT" "ORG_UNIT"})))
(s/def
:cognitect.aws.fms/LengthBoundedString
(s/spec
(s/and string? #(<= 0 (count %) 1024))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 1024) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.fms/ListMemberAccountsResponse
(s/keys
:opt-un
[:cognitect.aws.fms.ListMemberAccountsResponse/NextToken
:cognitect.aws.fms.ListMemberAccountsResponse/MemberAccounts]))
(s/def :cognitect.aws.fms/AppsListsData (s/coll-of :cognitect.aws.fms/AppsListDataSummary))
(s/def
:cognitect.aws.fms/PaginationMaxResults
(s/spec (s/and int? #(<= 1 % 100)) :gen #(gen/choose 1 100)))
(s/def :cognitect.aws.fms/DisassociateAdminAccountRequest (s/keys))
(s/def
:cognitect.aws.fms/NetworkFirewallMissingSubnetViolation
(s/keys
:opt-un
[:cognitect.aws.fms.NetworkFirewallMissingSubnetViolation/AvailabilityZone
:cognitect.aws.fms.NetworkFirewallMissingSubnetViolation/TargetViolationReason
:cognitect.aws.fms.NetworkFirewallMissingSubnetViolation/ViolationTarget
:cognitect.aws.fms.NetworkFirewallMissingSubnetViolation/VPC]))
(s/def
:cognitect.aws.fms/App
(s/keys
:req-un
[:cognitect.aws.fms.App/AppName :cognitect.aws.fms.App/Protocol :cognitect.aws.fms.App/Port]))
(s/def
:cognitect.aws.fms/ListTagsForResourceResponse
(s/keys :opt-un [:cognitect.aws.fms.ListTagsForResourceResponse/TagList]))
(s/def
:cognitect.aws.fms/ViolationDetail
(s/keys
:req-un
[:cognitect.aws.fms.ViolationDetail/PolicyId
:cognitect.aws.fms.ViolationDetail/MemberAccount
:cognitect.aws.fms.ViolationDetail/ResourceId
:cognitect.aws.fms.ViolationDetail/ResourceType
:cognitect.aws.fms.ViolationDetail/ResourceViolations]
:opt-un
[:cognitect.aws.fms.ViolationDetail/ResourceDescription
:cognitect.aws.fms.ViolationDetail/ResourceTags]))
(s/def
:cognitect.aws.fms/AWSAccountId
(s/spec #(re-matches (re-pattern "^[0-9]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/ResourceName
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/PaginationToken
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/ResourceTags
(s/coll-of :cognitect.aws.fms/ResourceTag :min-count 0 :max-count 8))
(s/def
:cognitect.aws.fms/DeleteAppsListRequest
(s/keys :req-un [:cognitect.aws.fms.DeleteAppsListRequest/ListId]))
(s/def
:cognitect.aws.fms/DeleteProtocolsListRequest
(s/keys :req-un [:cognitect.aws.fms.DeleteProtocolsListRequest/ListId]))
(s/def
:cognitect.aws.fms/NetworkFirewallAction
(s/spec #(re-matches (re-pattern "^[a-zA-Z0-9]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/GetPolicyRequest
(s/keys :req-un [:cognitect.aws.fms.GetPolicyRequest/PolicyId]))
(s/def :cognitect.aws.fms/DeleteNotificationChannelRequest (s/keys))
(s/def
:cognitect.aws.fms/PutPolicyResponse
(s/keys
:opt-un
[:cognitect.aws.fms.PutPolicyResponse/PolicyArn :cognitect.aws.fms.PutPolicyResponse/Policy]))
(s/def
:cognitect.aws.fms/StatelessRuleGroup
(s/keys
:opt-un
[:cognitect.aws.fms.StatelessRuleGroup/Priority
:cognitect.aws.fms.StatelessRuleGroup/ResourceId
:cognitect.aws.fms.StatelessRuleGroup/RuleGroupName]))
(s/def
:cognitect.aws.fms/PartialMatch
(s/keys
:opt-un
[:cognitect.aws.fms.PartialMatch/TargetViolationReasons
:cognitect.aws.fms.PartialMatch/Reference]))
(s/def :cognitect.aws.fms/ProtocolsList (s/coll-of :cognitect.aws.fms/Protocol))
(s/def
:cognitect.aws.fms/Policy
(s/keys
:req-un
[:cognitect.aws.fms.Policy/PolicyName
:cognitect.aws.fms.Policy/SecurityServicePolicyData
:cognitect.aws.fms.Policy/ResourceType
:cognitect.aws.fms.Policy/ExcludeResourceTags
:cognitect.aws.fms.Policy/RemediationEnabled]
:opt-un
[:cognitect.aws.fms.Policy/DeleteUnusedFMManagedResources
:cognitect.aws.fms.Policy/ResourceTags
:cognitect.aws.fms.Policy/IncludeMap
:cognitect.aws.fms.Policy/PolicyId
:cognitect.aws.fms.Policy/ResourceTypeList
:cognitect.aws.fms.Policy/ExcludeMap
:cognitect.aws.fms.Policy/PolicyUpdateToken]))
(s/def
:cognitect.aws.fms/UpdateToken
(s/spec #(re-matches (re-pattern "^([\\p{L}\\p{Z}\\p{N}_.:/=+\\-@]*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.fms/TagKeyList
(s/coll-of :cognitect.aws.fms/TagKey :min-count 0 :max-count 200))
(s/def
:cognitect.aws.fms.EC2CreateRouteTableAction/Description
:cognitect.aws.fms/LengthBoundedString)
(s/def :cognitect.aws.fms.EC2CreateRouteTableAction/VpcId :cognitect.aws.fms/ActionTarget)
(s/def :cognitect.aws.fms.ProtocolsListDataSummary/ListArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.ProtocolsListDataSummary/ListId :cognitect.aws.fms/ListId)
(s/def :cognitect.aws.fms.ProtocolsListDataSummary/ListName :cognitect.aws.fms/ResourceName)
(s/def :cognitect.aws.fms.ProtocolsListDataSummary/ProtocolsList :cognitect.aws.fms/ProtocolsList)
(s/def
:cognitect.aws.fms.AwsEc2NetworkInterfaceViolation/ViolationTarget
:cognitect.aws.fms/ViolationTarget)
(s/def
:cognitect.aws.fms.AwsEc2NetworkInterfaceViolation/ViolatingSecurityGroups
:cognitect.aws.fms/ResourceIdList)
(s/def :cognitect.aws.fms.AppsListDataSummary/ListArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.AppsListDataSummary/ListId :cognitect.aws.fms/ListId)
(s/def :cognitect.aws.fms.AppsListDataSummary/ListName :cognitect.aws.fms/ResourceName)
(s/def :cognitect.aws.fms.AppsListDataSummary/AppsList :cognitect.aws.fms/AppsList)
(s/def :cognitect.aws.fms.TagResourceRequest/ResourceArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.TagResourceRequest/TagList :cognitect.aws.fms/TagList)
(s/def :cognitect.aws.fms.ComplianceViolator/ResourceId :cognitect.aws.fms/ResourceId)
(s/def :cognitect.aws.fms.ComplianceViolator/ViolationReason :cognitect.aws.fms/ViolationReason)
(s/def :cognitect.aws.fms.ComplianceViolator/ResourceType :cognitect.aws.fms/ResourceType)
(s/def :cognitect.aws.fms.ComplianceViolator/Metadata :cognitect.aws.fms/ComplianceViolatorMetadata)
(s/def :cognitect.aws.fms.GetProtectionStatusRequest/PolicyId :cognitect.aws.fms/PolicyId)
(s/def
:cognitect.aws.fms.GetProtectionStatusRequest/MemberAccountId
:cognitect.aws.fms/AWSAccountId)
(s/def :cognitect.aws.fms.GetProtectionStatusRequest/StartTime :cognitect.aws.fms/TimeStamp)
(s/def :cognitect.aws.fms.GetProtectionStatusRequest/EndTime :cognitect.aws.fms/TimeStamp)
(s/def :cognitect.aws.fms.GetProtectionStatusRequest/NextToken :cognitect.aws.fms/PaginationToken)
(s/def
:cognitect.aws.fms.GetProtectionStatusRequest/MaxResults
:cognitect.aws.fms/PaginationMaxResults)
(s/def :cognitect.aws.fms.PutAppsListResponse/AppsList :cognitect.aws.fms/AppsListData)
(s/def :cognitect.aws.fms.PutAppsListResponse/AppsListArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.ListMemberAccountsRequest/NextToken :cognitect.aws.fms/PaginationToken)
(s/def
:cognitect.aws.fms.ListMemberAccountsRequest/MaxResults
:cognitect.aws.fms/PaginationMaxResults)
(s/def
:cognitect.aws.fms.EC2CopyRouteTableAction/Description
:cognitect.aws.fms/LengthBoundedString)
(s/def :cognitect.aws.fms.EC2CopyRouteTableAction/VpcId :cognitect.aws.fms/ActionTarget)
(s/def :cognitect.aws.fms.EC2CopyRouteTableAction/RouteTableId :cognitect.aws.fms/ActionTarget)
(s/def
:cognitect.aws.fms.FMSPolicyUpdateFirewallCreationConfigAction/Description
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.FMSPolicyUpdateFirewallCreationConfigAction/FirewallCreationConfig
:cognitect.aws.fms/ManagedServiceData)
(s/def
:cognitect.aws.fms.NetworkFirewallMissingFirewallViolation/ViolationTarget
:cognitect.aws.fms/ViolationTarget)
(s/def :cognitect.aws.fms.NetworkFirewallMissingFirewallViolation/VPC :cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallMissingFirewallViolation/AvailabilityZone
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.NetworkFirewallMissingFirewallViolation/TargetViolationReason
:cognitect.aws.fms/TargetViolationReason)
(s/def
:cognitect.aws.fms.GetProtocolsListResponse/ProtocolsList
:cognitect.aws.fms/ProtocolsListData)
(s/def :cognitect.aws.fms.GetProtocolsListResponse/ProtocolsListArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.Tag/Key :cognitect.aws.fms/TagKey)
(s/def :cognitect.aws.fms.Tag/Value :cognitect.aws.fms/TagValue)
(s/def
:cognitect.aws.fms.ListProtocolsListsResponse/ProtocolsLists
:cognitect.aws.fms/ProtocolsListsData)
(s/def :cognitect.aws.fms.ListProtocolsListsResponse/NextToken :cognitect.aws.fms/PaginationToken)
(s/def
:cognitect.aws.fms.NetworkFirewallUnexpectedFirewallRoutesViolation/FirewallSubnetId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallUnexpectedFirewallRoutesViolation/ViolatingRoutes
:cognitect.aws.fms/Routes)
(s/def
:cognitect.aws.fms.NetworkFirewallUnexpectedFirewallRoutesViolation/RouteTableId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallUnexpectedFirewallRoutesViolation/FirewallEndpoint
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallUnexpectedFirewallRoutesViolation/VpcId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallPolicy/FirewallDeploymentModel
:cognitect.aws.fms/FirewallDeploymentModel)
(s/def
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/ViolatingRoutes
:cognitect.aws.fms/Routes)
(s/def
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/InternetGatewayRoutes
:cognitect.aws.fms/Routes)
(s/def
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/CurrentInternetGatewayRouteTable
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/RouteTableId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/FirewallSubnetId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/SubnetAvailabilityZone
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/CurrentFirewallSubnetRouteTable
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/SubnetId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/FirewallSubnetRoutes
:cognitect.aws.fms/Routes)
(s/def
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/SubnetAvailabilityZoneId
:cognitect.aws.fms/LengthBoundedString)
(s/def :cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/VpcId :cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.RouteHasOutOfScopeEndpointViolation/InternetGatewayId
:cognitect.aws.fms/ResourceId)
(s/def :cognitect.aws.fms.GetAppsListRequest/ListId :cognitect.aws.fms/ListId)
(s/def :cognitect.aws.fms.GetAppsListRequest/DefaultList :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.PolicyComplianceStatus/PolicyOwner :cognitect.aws.fms/AWSAccountId)
(s/def :cognitect.aws.fms.PolicyComplianceStatus/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.PolicyComplianceStatus/PolicyName :cognitect.aws.fms/ResourceName)
(s/def :cognitect.aws.fms.PolicyComplianceStatus/MemberAccount :cognitect.aws.fms/AWSAccountId)
(s/def
:cognitect.aws.fms.PolicyComplianceStatus/EvaluationResults
:cognitect.aws.fms/EvaluationResults)
(s/def :cognitect.aws.fms.PolicyComplianceStatus/LastUpdated :cognitect.aws.fms/TimeStamp)
(s/def :cognitect.aws.fms.PolicyComplianceStatus/IssueInfoMap :cognitect.aws.fms/IssueInfoMap)
(s/def
:cognitect.aws.fms.PossibleRemediationAction/Description
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.PossibleRemediationAction/OrderedRemediationActions
:cognitect.aws.fms/OrderedRemediationActions)
(s/def :cognitect.aws.fms.PossibleRemediationAction/IsDefaultAction :cognitect.aws.fms/Boolean)
(s/def
:cognitect.aws.fms.GetProtectionStatusResponse/AdminAccountId
:cognitect.aws.fms/AWSAccountId)
(s/def
:cognitect.aws.fms.GetProtectionStatusResponse/ServiceType
:cognitect.aws.fms/SecurityServiceType)
(s/def :cognitect.aws.fms.GetProtectionStatusResponse/Data :cognitect.aws.fms/ProtectionData)
(s/def :cognitect.aws.fms.GetProtectionStatusResponse/NextToken :cognitect.aws.fms/PaginationToken)
(s/def :cognitect.aws.fms.PolicySummary/PolicyArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.PolicySummary/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.PolicySummary/PolicyName :cognitect.aws.fms/ResourceName)
(s/def :cognitect.aws.fms.PolicySummary/ResourceType :cognitect.aws.fms/ResourceType)
(s/def :cognitect.aws.fms.PolicySummary/SecurityServiceType :cognitect.aws.fms/SecurityServiceType)
(s/def :cognitect.aws.fms.PolicySummary/RemediationEnabled :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.PolicySummary/DeleteUnusedFMManagedResources :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.ResourceTag/Key :cognitect.aws.fms/ResourceTagKey)
(s/def :cognitect.aws.fms.ResourceTag/Value :cognitect.aws.fms/ResourceTagValue)
(s/def
:cognitect.aws.fms.GetComplianceDetailResponse/PolicyComplianceDetail
:cognitect.aws.fms/PolicyComplianceDetail)
(s/def
:cognitect.aws.fms.PolicyOption/NetworkFirewallPolicy
:cognitect.aws.fms/NetworkFirewallPolicy)
(s/def :cognitect.aws.fms.EC2CreateRouteAction/Description :cognitect.aws.fms/LengthBoundedString)
(s/def :cognitect.aws.fms.EC2CreateRouteAction/DestinationCidrBlock :cognitect.aws.fms/CIDR)
(s/def
:cognitect.aws.fms.EC2CreateRouteAction/DestinationPrefixListId
:cognitect.aws.fms/ResourceId)
(s/def :cognitect.aws.fms.EC2CreateRouteAction/DestinationIpv6CidrBlock :cognitect.aws.fms/CIDR)
(s/def :cognitect.aws.fms.EC2CreateRouteAction/VpcEndpointId :cognitect.aws.fms/ActionTarget)
(s/def :cognitect.aws.fms.EC2CreateRouteAction/GatewayId :cognitect.aws.fms/ActionTarget)
(s/def :cognitect.aws.fms.EC2CreateRouteAction/RouteTableId :cognitect.aws.fms/ActionTarget)
(s/def :cognitect.aws.fms.SecurityServicePolicyData/Type :cognitect.aws.fms/SecurityServiceType)
(s/def
:cognitect.aws.fms.SecurityServicePolicyData/ManagedServiceData
:cognitect.aws.fms/ManagedServiceData)
(s/def :cognitect.aws.fms.SecurityServicePolicyData/PolicyOption :cognitect.aws.fms/PolicyOption)
(s/def
:cognitect.aws.fms.EC2ReplaceRouteTableAssociationAction/Description
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.EC2ReplaceRouteTableAssociationAction/AssociationId
:cognitect.aws.fms/ActionTarget)
(s/def
:cognitect.aws.fms.EC2ReplaceRouteTableAssociationAction/RouteTableId
:cognitect.aws.fms/ActionTarget)
(s/def :cognitect.aws.fms.Route/DestinationType :cognitect.aws.fms/DestinationType)
(s/def :cognitect.aws.fms.Route/TargetType :cognitect.aws.fms/TargetType)
(s/def :cognitect.aws.fms.Route/Destination :cognitect.aws.fms/LengthBoundedString)
(s/def :cognitect.aws.fms.Route/Target :cognitect.aws.fms/LengthBoundedString)
(s/def :cognitect.aws.fms.GetNotificationChannelResponse/SnsTopicArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.GetNotificationChannelResponse/SnsRoleName :cognitect.aws.fms/ResourceArn)
(s/def
:cognitect.aws.fms.DnsDuplicateRuleGroupViolation/ViolationTarget
:cognitect.aws.fms/ViolationTarget)
(s/def
:cognitect.aws.fms.DnsDuplicateRuleGroupViolation/ViolationTargetDescription
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.ListComplianceStatusResponse/PolicyComplianceStatusList
:cognitect.aws.fms/PolicyComplianceStatusList)
(s/def :cognitect.aws.fms.ListComplianceStatusResponse/NextToken :cognitect.aws.fms/PaginationToken)
(s/def :cognitect.aws.fms.ListAppsListsRequest/DefaultLists :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.ListAppsListsRequest/NextToken :cognitect.aws.fms/PaginationToken)
(s/def :cognitect.aws.fms.ListAppsListsRequest/MaxResults :cognitect.aws.fms/PaginationMaxResults)
(s/def
:cognitect.aws.fms.DnsRuleGroupPriorityConflictViolation/ViolationTarget
:cognitect.aws.fms/ViolationTarget)
(s/def
:cognitect.aws.fms.DnsRuleGroupPriorityConflictViolation/ViolationTargetDescription
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.DnsRuleGroupPriorityConflictViolation/ConflictingPriority
:cognitect.aws.fms/DnsRuleGroupPriority)
(s/def
:cognitect.aws.fms.DnsRuleGroupPriorityConflictViolation/ConflictingPolicyId
:cognitect.aws.fms/PolicyId)
(s/def
:cognitect.aws.fms.DnsRuleGroupPriorityConflictViolation/UnavailablePriorities
:cognitect.aws.fms/DnsRuleGroupPriorities)
(s/def :cognitect.aws.fms.ListAppsListsResponse/AppsLists :cognitect.aws.fms/AppsListsData)
(s/def :cognitect.aws.fms.ListAppsListsResponse/NextToken :cognitect.aws.fms/PaginationToken)
(s/def :cognitect.aws.fms.EC2DeleteRouteAction/Description :cognitect.aws.fms/LengthBoundedString)
(s/def :cognitect.aws.fms.EC2DeleteRouteAction/DestinationCidrBlock :cognitect.aws.fms/CIDR)
(s/def
:cognitect.aws.fms.EC2DeleteRouteAction/DestinationPrefixListId
:cognitect.aws.fms/ResourceId)
(s/def :cognitect.aws.fms.EC2DeleteRouteAction/DestinationIpv6CidrBlock :cognitect.aws.fms/CIDR)
(s/def :cognitect.aws.fms.EC2DeleteRouteAction/RouteTableId :cognitect.aws.fms/ActionTarget)
(s/def
:cognitect.aws.fms.PossibleRemediationActions/Description
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.PossibleRemediationActions/Actions
:cognitect.aws.fms/PossibleRemediationActionList)
(s/def :cognitect.aws.fms.ExpectedRoute/IpV4Cidr :cognitect.aws.fms/CIDR)
(s/def :cognitect.aws.fms.ExpectedRoute/PrefixListId :cognitect.aws.fms/CIDR)
(s/def :cognitect.aws.fms.ExpectedRoute/IpV6Cidr :cognitect.aws.fms/CIDR)
(s/def :cognitect.aws.fms.ExpectedRoute/ContributingSubnets :cognitect.aws.fms/ResourceIdList)
(s/def :cognitect.aws.fms.ExpectedRoute/AllowedTargets :cognitect.aws.fms/LengthBoundedStringList)
(s/def :cognitect.aws.fms.ExpectedRoute/RouteTableId :cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/ViolatingRoutes
:cognitect.aws.fms/Routes)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/ActualFirewallSubnetRoutes
:cognitect.aws.fms/Routes)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/CurrentInternetGatewayRouteTable
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/ExpectedInternetGatewayRoutes
:cognitect.aws.fms/ExpectedRoutes)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/RouteTableId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/FirewallSubnetId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/ExpectedFirewallEndpoint
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/IsRouteTableUsedInDifferentAZ
:cognitect.aws.fms/Boolean)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/SubnetAvailabilityZone
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/ExpectedFirewallSubnetRoutes
:cognitect.aws.fms/ExpectedRoutes)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/CurrentFirewallSubnetRouteTable
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/SubnetId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/ActualInternetGatewayRoutes
:cognitect.aws.fms/Routes)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/VpcId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInternetTrafficNotInspectedViolation/InternetGatewayId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.ResourceViolation/AwsEc2NetworkInterfaceViolation
:cognitect.aws.fms/AwsEc2NetworkInterfaceViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/NetworkFirewallMissingFirewallViolation
:cognitect.aws.fms/NetworkFirewallMissingFirewallViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/NetworkFirewallUnexpectedFirewallRoutesViolation
:cognitect.aws.fms/NetworkFirewallUnexpectedFirewallRoutesViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/RouteHasOutOfScopeEndpointViolation
:cognitect.aws.fms/RouteHasOutOfScopeEndpointViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/DnsDuplicateRuleGroupViolation
:cognitect.aws.fms/DnsDuplicateRuleGroupViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/DnsRuleGroupPriorityConflictViolation
:cognitect.aws.fms/DnsRuleGroupPriorityConflictViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/PossibleRemediationActions
:cognitect.aws.fms/PossibleRemediationActions)
(s/def
:cognitect.aws.fms.ResourceViolation/NetworkFirewallInternetTrafficNotInspectedViolation
:cognitect.aws.fms/NetworkFirewallInternetTrafficNotInspectedViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/AwsEc2InstanceViolation
:cognitect.aws.fms/AwsEc2InstanceViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/NetworkFirewallInvalidRouteConfigurationViolation
:cognitect.aws.fms/NetworkFirewallInvalidRouteConfigurationViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/NetworkFirewallMissingExpectedRTViolation
:cognitect.aws.fms/NetworkFirewallMissingExpectedRTViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/NetworkFirewallMissingExpectedRoutesViolation
:cognitect.aws.fms/NetworkFirewallMissingExpectedRoutesViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/FirewallSubnetIsOutOfScopeViolation
:cognitect.aws.fms/FirewallSubnetIsOutOfScopeViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/NetworkFirewallPolicyModifiedViolation
:cognitect.aws.fms/NetworkFirewallPolicyModifiedViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/NetworkFirewallBlackHoleRouteDetectedViolation
:cognitect.aws.fms/NetworkFirewallBlackHoleRouteDetectedViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/DnsRuleGroupLimitExceededViolation
:cognitect.aws.fms/DnsRuleGroupLimitExceededViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/AwsVPCSecurityGroupViolation
:cognitect.aws.fms/AwsVPCSecurityGroupViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/NetworkFirewallUnexpectedGatewayRoutesViolation
:cognitect.aws.fms/NetworkFirewallUnexpectedGatewayRoutesViolation)
(s/def
:cognitect.aws.fms.ResourceViolation/NetworkFirewallMissingSubnetViolation
:cognitect.aws.fms/NetworkFirewallMissingSubnetViolation)
(s/def
:cognitect.aws.fms.AwsEc2InstanceViolation/ViolationTarget
:cognitect.aws.fms/ViolationTarget)
(s/def
:cognitect.aws.fms.AwsEc2InstanceViolation/AwsEc2NetworkInterfaceViolations
:cognitect.aws.fms/AwsEc2NetworkInterfaceViolations)
(s/def :cognitect.aws.fms.GetAdminAccountResponse/AdminAccount :cognitect.aws.fms/AWSAccountId)
(s/def :cognitect.aws.fms.GetAdminAccountResponse/RoleStatus :cognitect.aws.fms/AccountRoleStatus)
(s/def
:cognitect.aws.fms.NetworkFirewallPolicyDescription/StatelessRuleGroups
:cognitect.aws.fms/StatelessRuleGroupList)
(s/def
:cognitect.aws.fms.NetworkFirewallPolicyDescription/StatelessDefaultActions
:cognitect.aws.fms/NetworkFirewallActionList)
(s/def
:cognitect.aws.fms.NetworkFirewallPolicyDescription/StatelessFragmentDefaultActions
:cognitect.aws.fms/NetworkFirewallActionList)
(s/def
:cognitect.aws.fms.NetworkFirewallPolicyDescription/StatelessCustomActions
:cognitect.aws.fms/NetworkFirewallActionList)
(s/def
:cognitect.aws.fms.NetworkFirewallPolicyDescription/StatefulRuleGroups
:cognitect.aws.fms/StatefulRuleGroupList)
(s/def :cognitect.aws.fms.GetViolationDetailsRequest/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.GetViolationDetailsRequest/MemberAccount :cognitect.aws.fms/AWSAccountId)
(s/def :cognitect.aws.fms.GetViolationDetailsRequest/ResourceId :cognitect.aws.fms/ResourceId)
(s/def :cognitect.aws.fms.GetViolationDetailsRequest/ResourceType :cognitect.aws.fms/ResourceType)
(s/def :cognitect.aws.fms.ListProtocolsListsRequest/DefaultLists :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.ListProtocolsListsRequest/NextToken :cognitect.aws.fms/PaginationToken)
(s/def
:cognitect.aws.fms.ListProtocolsListsRequest/MaxResults
:cognitect.aws.fms/PaginationMaxResults)
(s/def
:cognitect.aws.fms.RemediationActionWithOrder/RemediationAction
:cognitect.aws.fms/RemediationAction)
(s/def :cognitect.aws.fms.RemediationActionWithOrder/Order :cognitect.aws.fms/BasicInteger)
(s/def :cognitect.aws.fms.ListPoliciesResponse/PolicyList :cognitect.aws.fms/PolicySummaryList)
(s/def :cognitect.aws.fms.ListPoliciesResponse/NextToken :cognitect.aws.fms/PaginationToken)
(s/def
:cognitect.aws.fms.EvaluationResult/ComplianceStatus
:cognitect.aws.fms/PolicyComplianceStatusType)
(s/def :cognitect.aws.fms.EvaluationResult/ViolatorCount :cognitect.aws.fms/ResourceCount)
(s/def :cognitect.aws.fms.EvaluationResult/EvaluationLimitExceeded :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.ListTagsForResourceRequest/ResourceArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.PolicyComplianceDetail/PolicyOwner :cognitect.aws.fms/AWSAccountId)
(s/def :cognitect.aws.fms.PolicyComplianceDetail/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.PolicyComplianceDetail/MemberAccount :cognitect.aws.fms/AWSAccountId)
(s/def :cognitect.aws.fms.PolicyComplianceDetail/Violators :cognitect.aws.fms/ComplianceViolators)
(s/def :cognitect.aws.fms.PolicyComplianceDetail/EvaluationLimitExceeded :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.PolicyComplianceDetail/ExpiredAt :cognitect.aws.fms/TimeStamp)
(s/def :cognitect.aws.fms.PolicyComplianceDetail/IssueInfoMap :cognitect.aws.fms/IssueInfoMap)
(s/def
:cognitect.aws.fms.StatefulRuleGroup/RuleGroupName
:cognitect.aws.fms/NetworkFirewallResourceName)
(s/def :cognitect.aws.fms.StatefulRuleGroup/ResourceId :cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ActualFirewallSubnetId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ActualFirewallSubnetRoutes
:cognitect.aws.fms/Routes)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/CurrentInternetGatewayRouteTable
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ExpectedInternetGatewayRoutes
:cognitect.aws.fms/ExpectedRoutes)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/RouteTableId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ExpectedFirewallEndpoint
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/IsRouteTableUsedInDifferentAZ
:cognitect.aws.fms/Boolean)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ExpectedFirewallSubnetRoutes
:cognitect.aws.fms/ExpectedRoutes)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/CurrentFirewallSubnetRouteTable
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ActualInternetGatewayRoutes
:cognitect.aws.fms/Routes)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ViolatingRoute
:cognitect.aws.fms/Route)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ExpectedFirewallSubnetId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/ActualFirewallEndpoint
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/VpcId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/InternetGatewayId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallInvalidRouteConfigurationViolation/AffectedSubnets
:cognitect.aws.fms/ResourceIdList)
(s/def :cognitect.aws.fms.AssociateAdminAccountRequest/AdminAccount :cognitect.aws.fms/AWSAccountId)
(s/def
:cognitect.aws.fms.SecurityGroupRemediationAction/RemediationActionType
:cognitect.aws.fms/RemediationActionType)
(s/def
:cognitect.aws.fms.SecurityGroupRemediationAction/Description
:cognitect.aws.fms/RemediationActionDescription)
(s/def
:cognitect.aws.fms.SecurityGroupRemediationAction/RemediationResult
:cognitect.aws.fms/SecurityGroupRuleDescription)
(s/def :cognitect.aws.fms.SecurityGroupRemediationAction/IsDefaultAction :cognitect.aws.fms/Boolean)
(s/def
:cognitect.aws.fms.NetworkFirewallMissingExpectedRTViolation/ViolationTarget
:cognitect.aws.fms/ViolationTarget)
(s/def
:cognitect.aws.fms.NetworkFirewallMissingExpectedRTViolation/VPC
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallMissingExpectedRTViolation/AvailabilityZone
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.NetworkFirewallMissingExpectedRTViolation/CurrentRouteTable
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallMissingExpectedRTViolation/ExpectedRouteTable
:cognitect.aws.fms/ResourceId)
(s/def :cognitect.aws.fms.ListComplianceStatusRequest/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.ListComplianceStatusRequest/NextToken :cognitect.aws.fms/PaginationToken)
(s/def
:cognitect.aws.fms.ListComplianceStatusRequest/MaxResults
:cognitect.aws.fms/PaginationMaxResults)
(s/def
:cognitect.aws.fms.PutProtocolsListResponse/ProtocolsList
:cognitect.aws.fms/ProtocolsListData)
(s/def :cognitect.aws.fms.PutProtocolsListResponse/ProtocolsListArn :cognitect.aws.fms/ResourceArn)
(s/def
:cognitect.aws.fms.GetViolationDetailsResponse/ViolationDetail
:cognitect.aws.fms/ViolationDetail)
(s/def
:cognitect.aws.fms.NetworkFirewallMissingExpectedRoutesViolation/ViolationTarget
:cognitect.aws.fms/ViolationTarget)
(s/def
:cognitect.aws.fms.NetworkFirewallMissingExpectedRoutesViolation/ExpectedRoutes
:cognitect.aws.fms/ExpectedRoutes)
(s/def
:cognitect.aws.fms.NetworkFirewallMissingExpectedRoutesViolation/VpcId
:cognitect.aws.fms/ResourceId)
(s/def :cognitect.aws.fms.ProtocolsListData/ListId :cognitect.aws.fms/ListId)
(s/def :cognitect.aws.fms.ProtocolsListData/ListName :cognitect.aws.fms/ResourceName)
(s/def :cognitect.aws.fms.ProtocolsListData/ListUpdateToken :cognitect.aws.fms/UpdateToken)
(s/def :cognitect.aws.fms.ProtocolsListData/CreateTime :cognitect.aws.fms/TimeStamp)
(s/def :cognitect.aws.fms.ProtocolsListData/LastUpdateTime :cognitect.aws.fms/TimeStamp)
(s/def :cognitect.aws.fms.ProtocolsListData/ProtocolsList :cognitect.aws.fms/ProtocolsList)
(s/def
:cognitect.aws.fms.ProtocolsListData/PreviousProtocolsList
:cognitect.aws.fms/PreviousProtocolsList)
(s/def
:cognitect.aws.fms.FirewallSubnetIsOutOfScopeViolation/FirewallSubnetId
:cognitect.aws.fms/ResourceId)
(s/def :cognitect.aws.fms.FirewallSubnetIsOutOfScopeViolation/VpcId :cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.FirewallSubnetIsOutOfScopeViolation/SubnetAvailabilityZone
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.FirewallSubnetIsOutOfScopeViolation/SubnetAvailabilityZoneId
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.FirewallSubnetIsOutOfScopeViolation/VpcEndpointId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.PutProtocolsListRequest/ProtocolsList
:cognitect.aws.fms/ProtocolsListData)
(s/def :cognitect.aws.fms.PutProtocolsListRequest/TagList :cognitect.aws.fms/TagList)
(s/def :cognitect.aws.fms.PutAppsListRequest/AppsList :cognitect.aws.fms/AppsListData)
(s/def :cognitect.aws.fms.PutAppsListRequest/TagList :cognitect.aws.fms/TagList)
(s/def :cognitect.aws.fms.GetAppsListResponse/AppsList :cognitect.aws.fms/AppsListData)
(s/def :cognitect.aws.fms.GetAppsListResponse/AppsListArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.GetComplianceDetailRequest/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.GetComplianceDetailRequest/MemberAccount :cognitect.aws.fms/AWSAccountId)
(s/def
:cognitect.aws.fms.NetworkFirewallPolicyModifiedViolation/ViolationTarget
:cognitect.aws.fms/ViolationTarget)
(s/def
:cognitect.aws.fms.NetworkFirewallPolicyModifiedViolation/CurrentPolicyDescription
:cognitect.aws.fms/NetworkFirewallPolicyDescription)
(s/def
:cognitect.aws.fms.NetworkFirewallPolicyModifiedViolation/ExpectedPolicyDescription
:cognitect.aws.fms/NetworkFirewallPolicyDescription)
(s/def :cognitect.aws.fms.ListPoliciesRequest/NextToken :cognitect.aws.fms/PaginationToken)
(s/def :cognitect.aws.fms.ListPoliciesRequest/MaxResults :cognitect.aws.fms/PaginationMaxResults)
(s/def
:cognitect.aws.fms.RemediationAction/EC2CreateRouteTableAction
:cognitect.aws.fms/EC2CreateRouteTableAction)
(s/def
:cognitect.aws.fms.RemediationAction/EC2CopyRouteTableAction
:cognitect.aws.fms/EC2CopyRouteTableAction)
(s/def
:cognitect.aws.fms.RemediationAction/FMSPolicyUpdateFirewallCreationConfigAction
:cognitect.aws.fms/FMSPolicyUpdateFirewallCreationConfigAction)
(s/def
:cognitect.aws.fms.RemediationAction/EC2CreateRouteAction
:cognitect.aws.fms/EC2CreateRouteAction)
(s/def
:cognitect.aws.fms.RemediationAction/EC2ReplaceRouteTableAssociationAction
:cognitect.aws.fms/EC2ReplaceRouteTableAssociationAction)
(s/def
:cognitect.aws.fms.RemediationAction/EC2DeleteRouteAction
:cognitect.aws.fms/EC2DeleteRouteAction)
(s/def :cognitect.aws.fms.RemediationAction/Description :cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.RemediationAction/EC2AssociateRouteTableAction
:cognitect.aws.fms/EC2AssociateRouteTableAction)
(s/def
:cognitect.aws.fms.RemediationAction/EC2ReplaceRouteAction
:cognitect.aws.fms/EC2ReplaceRouteAction)
(s/def :cognitect.aws.fms.ActionTarget/ResourceId :cognitect.aws.fms/ResourceId)
(s/def :cognitect.aws.fms.ActionTarget/Description :cognitect.aws.fms/LengthBoundedString)
(s/def :cognitect.aws.fms.PutPolicyRequest/Policy :cognitect.aws.fms/Policy)
(s/def :cognitect.aws.fms.PutPolicyRequest/TagList :cognitect.aws.fms/TagList)
(s/def :cognitect.aws.fms.GetPolicyResponse/Policy :cognitect.aws.fms/Policy)
(s/def :cognitect.aws.fms.GetPolicyResponse/PolicyArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.AppsListData/ListId :cognitect.aws.fms/ListId)
(s/def :cognitect.aws.fms.AppsListData/ListName :cognitect.aws.fms/ResourceName)
(s/def :cognitect.aws.fms.AppsListData/ListUpdateToken :cognitect.aws.fms/UpdateToken)
(s/def :cognitect.aws.fms.AppsListData/CreateTime :cognitect.aws.fms/TimeStamp)
(s/def :cognitect.aws.fms.AppsListData/LastUpdateTime :cognitect.aws.fms/TimeStamp)
(s/def :cognitect.aws.fms.AppsListData/AppsList :cognitect.aws.fms/AppsList)
(s/def :cognitect.aws.fms.AppsListData/PreviousAppsList :cognitect.aws.fms/PreviousAppsList)
(s/def :cognitect.aws.fms.PutNotificationChannelRequest/SnsTopicArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.PutNotificationChannelRequest/SnsRoleName :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.GetProtocolsListRequest/ListId :cognitect.aws.fms/ListId)
(s/def :cognitect.aws.fms.GetProtocolsListRequest/DefaultList :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.UntagResourceRequest/ResourceArn :cognitect.aws.fms/ResourceArn)
(s/def :cognitect.aws.fms.UntagResourceRequest/TagKeys :cognitect.aws.fms/TagKeyList)
(s/def
:cognitect.aws.fms.NetworkFirewallBlackHoleRouteDetectedViolation/ViolationTarget
:cognitect.aws.fms/ViolationTarget)
(s/def
:cognitect.aws.fms.NetworkFirewallBlackHoleRouteDetectedViolation/RouteTableId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallBlackHoleRouteDetectedViolation/VpcId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallBlackHoleRouteDetectedViolation/ViolatingRoutes
:cognitect.aws.fms/Routes)
(s/def :cognitect.aws.fms.SecurityGroupRuleDescription/IPV4Range :cognitect.aws.fms/CIDR)
(s/def :cognitect.aws.fms.SecurityGroupRuleDescription/IPV6Range :cognitect.aws.fms/CIDR)
(s/def :cognitect.aws.fms.SecurityGroupRuleDescription/PrefixListId :cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.SecurityGroupRuleDescription/Protocol
:cognitect.aws.fms/LengthBoundedString)
(s/def :cognitect.aws.fms.SecurityGroupRuleDescription/FromPort :cognitect.aws.fms/IPPortNumber)
(s/def :cognitect.aws.fms.SecurityGroupRuleDescription/ToPort :cognitect.aws.fms/IPPortNumber)
(s/def
:cognitect.aws.fms.EC2AssociateRouteTableAction/Description
:cognitect.aws.fms/LengthBoundedString)
(s/def :cognitect.aws.fms.EC2AssociateRouteTableAction/RouteTableId :cognitect.aws.fms/ActionTarget)
(s/def :cognitect.aws.fms.EC2AssociateRouteTableAction/SubnetId :cognitect.aws.fms/ActionTarget)
(s/def :cognitect.aws.fms.EC2AssociateRouteTableAction/GatewayId :cognitect.aws.fms/ActionTarget)
(s/def
:cognitect.aws.fms.DnsRuleGroupLimitExceededViolation/ViolationTarget
:cognitect.aws.fms/ViolationTarget)
(s/def
:cognitect.aws.fms.DnsRuleGroupLimitExceededViolation/ViolationTargetDescription
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.DnsRuleGroupLimitExceededViolation/NumberOfRuleGroupsAlreadyAssociated
:cognitect.aws.fms/BasicInteger)
(s/def :cognitect.aws.fms.DeletePolicyRequest/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.DeletePolicyRequest/DeleteAllPolicyResources :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.EC2ReplaceRouteAction/Description :cognitect.aws.fms/LengthBoundedString)
(s/def :cognitect.aws.fms.EC2ReplaceRouteAction/DestinationCidrBlock :cognitect.aws.fms/CIDR)
(s/def
:cognitect.aws.fms.EC2ReplaceRouteAction/DestinationPrefixListId
:cognitect.aws.fms/ResourceId)
(s/def :cognitect.aws.fms.EC2ReplaceRouteAction/DestinationIpv6CidrBlock :cognitect.aws.fms/CIDR)
(s/def :cognitect.aws.fms.EC2ReplaceRouteAction/GatewayId :cognitect.aws.fms/ActionTarget)
(s/def :cognitect.aws.fms.EC2ReplaceRouteAction/RouteTableId :cognitect.aws.fms/ActionTarget)
(s/def
:cognitect.aws.fms.AwsVPCSecurityGroupViolation/ViolationTarget
:cognitect.aws.fms/ViolationTarget)
(s/def
:cognitect.aws.fms.AwsVPCSecurityGroupViolation/ViolationTargetDescription
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.AwsVPCSecurityGroupViolation/PartialMatches
:cognitect.aws.fms/PartialMatches)
(s/def
:cognitect.aws.fms.AwsVPCSecurityGroupViolation/PossibleSecurityGroupRemediationActions
:cognitect.aws.fms/SecurityGroupRemediationActions)
(s/def
:cognitect.aws.fms.NetworkFirewallUnexpectedGatewayRoutesViolation/GatewayId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallUnexpectedGatewayRoutesViolation/ViolatingRoutes
:cognitect.aws.fms/Routes)
(s/def
:cognitect.aws.fms.NetworkFirewallUnexpectedGatewayRoutesViolation/RouteTableId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallUnexpectedGatewayRoutesViolation/VpcId
:cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.ListMemberAccountsResponse/MemberAccounts
:cognitect.aws.fms/MemberAccounts)
(s/def :cognitect.aws.fms.ListMemberAccountsResponse/NextToken :cognitect.aws.fms/PaginationToken)
(s/def
:cognitect.aws.fms.NetworkFirewallMissingSubnetViolation/ViolationTarget
:cognitect.aws.fms/ViolationTarget)
(s/def :cognitect.aws.fms.NetworkFirewallMissingSubnetViolation/VPC :cognitect.aws.fms/ResourceId)
(s/def
:cognitect.aws.fms.NetworkFirewallMissingSubnetViolation/AvailabilityZone
:cognitect.aws.fms/LengthBoundedString)
(s/def
:cognitect.aws.fms.NetworkFirewallMissingSubnetViolation/TargetViolationReason
:cognitect.aws.fms/TargetViolationReason)
(s/def :cognitect.aws.fms.App/AppName :cognitect.aws.fms/ResourceName)
(s/def :cognitect.aws.fms.App/Protocol :cognitect.aws.fms/Protocol)
(s/def :cognitect.aws.fms.App/Port :cognitect.aws.fms/IPPortNumber)
(s/def :cognitect.aws.fms.ListTagsForResourceResponse/TagList :cognitect.aws.fms/TagList)
(s/def :cognitect.aws.fms.ViolationDetail/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.ViolationDetail/MemberAccount :cognitect.aws.fms/AWSAccountId)
(s/def :cognitect.aws.fms.ViolationDetail/ResourceId :cognitect.aws.fms/ResourceId)
(s/def :cognitect.aws.fms.ViolationDetail/ResourceType :cognitect.aws.fms/ResourceType)
(s/def :cognitect.aws.fms.ViolationDetail/ResourceViolations :cognitect.aws.fms/ResourceViolations)
(s/def :cognitect.aws.fms.ViolationDetail/ResourceTags :cognitect.aws.fms/TagList)
(s/def
:cognitect.aws.fms.ViolationDetail/ResourceDescription
:cognitect.aws.fms/LengthBoundedString)
(s/def :cognitect.aws.fms.DeleteAppsListRequest/ListId :cognitect.aws.fms/ListId)
(s/def :cognitect.aws.fms.DeleteProtocolsListRequest/ListId :cognitect.aws.fms/ListId)
(s/def :cognitect.aws.fms.GetPolicyRequest/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.PutPolicyResponse/Policy :cognitect.aws.fms/Policy)
(s/def :cognitect.aws.fms.PutPolicyResponse/PolicyArn :cognitect.aws.fms/ResourceArn)
(s/def
:cognitect.aws.fms.StatelessRuleGroup/RuleGroupName
:cognitect.aws.fms/NetworkFirewallResourceName)
(s/def :cognitect.aws.fms.StatelessRuleGroup/ResourceId :cognitect.aws.fms/ResourceId)
(s/def :cognitect.aws.fms.StatelessRuleGroup/Priority :cognitect.aws.fms/StatelessRuleGroupPriority)
(s/def :cognitect.aws.fms.PartialMatch/Reference :cognitect.aws.fms/ReferenceRule)
(s/def
:cognitect.aws.fms.PartialMatch/TargetViolationReasons
:cognitect.aws.fms/TargetViolationReasons)
(s/def :cognitect.aws.fms.Policy/PolicyUpdateToken :cognitect.aws.fms/PolicyUpdateToken)
(s/def :cognitect.aws.fms.Policy/ResourceType :cognitect.aws.fms/ResourceType)
(s/def :cognitect.aws.fms.Policy/ResourceTypeList :cognitect.aws.fms/ResourceTypeList)
(s/def :cognitect.aws.fms.Policy/ExcludeResourceTags :cognitect.aws.fms/Boolean)
(s/def
:cognitect.aws.fms.Policy/SecurityServicePolicyData
:cognitect.aws.fms/SecurityServicePolicyData)
(s/def :cognitect.aws.fms.Policy/IncludeMap :cognitect.aws.fms/CustomerPolicyScopeMap)
(s/def :cognitect.aws.fms.Policy/PolicyName :cognitect.aws.fms/ResourceName)
(s/def :cognitect.aws.fms.Policy/DeleteUnusedFMManagedResources :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.Policy/RemediationEnabled :cognitect.aws.fms/Boolean)
(s/def :cognitect.aws.fms.Policy/PolicyId :cognitect.aws.fms/PolicyId)
(s/def :cognitect.aws.fms.Policy/ExcludeMap :cognitect.aws.fms/CustomerPolicyScopeMap)
(s/def :cognitect.aws.fms.Policy/ResourceTags :cognitect.aws.fms/ResourceTags)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy