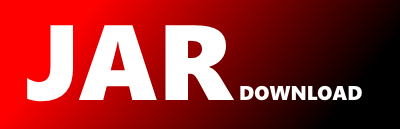
cognitect.aws.glue.specs.clj Maven / Gradle / Ivy
;; Copyright (c) Cognitect, Inc.
;; All rights reserved.
(ns cognitect.aws.glue.specs
(:require [clojure.spec.alpha :as s] [clojure.spec.gen.alpha :as gen]))
(s/def :cognitect.aws/client map?)
(s/def :core.async/channel any?)
(s/def
:cognitect.aws.glue/GetWorkflowRunsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetWorkflowRunsResponse/NextToken
:cognitect.aws.glue.GetWorkflowRunsResponse/Runs]))
(s/def :cognitect.aws.glue/SchemaListDefinition (s/coll-of :cognitect.aws.glue/SchemaListItem))
(s/def
:cognitect.aws.glue/TypeString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/GetTriggerRequest
(s/keys :req-un [:cognitect.aws.glue.GetTriggerRequest/Name]))
(s/def :cognitect.aws.glue/DynamoDBTargetList (s/coll-of :cognitect.aws.glue/DynamoDBTarget))
(s/def
:cognitect.aws.glue/BatchGetDevEndpointsRequest
(s/keys :req-un [:cognitect.aws.glue.BatchGetDevEndpointsRequest/DevEndpointNames]))
(s/def
:cognitect.aws.glue/StopWorkflowRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.StopWorkflowRunRequest/Name
:cognitect.aws.glue.StopWorkflowRunRequest/RunId]))
(s/def
:cognitect.aws.glue/UpdateColumnStatisticsForTableRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateColumnStatisticsForTableRequest/DatabaseName
:cognitect.aws.glue.UpdateColumnStatisticsForTableRequest/TableName
:cognitect.aws.glue.UpdateColumnStatisticsForTableRequest/ColumnStatisticsList]
:opt-un
[:cognitect.aws.glue.UpdateColumnStatisticsForTableRequest/CatalogId]))
(s/def
:cognitect.aws.glue/GetBlueprintResponse
(s/keys :opt-un [:cognitect.aws.glue.GetBlueprintResponse/Blueprint]))
(s/def
:cognitect.aws.glue/LocationString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\r\\n\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/DeleteResourcePolicyRequest
(s/keys
:opt-un
[:cognitect.aws.glue.DeleteResourcePolicyRequest/PolicyHashCondition
:cognitect.aws.glue.DeleteResourcePolicyRequest/ResourceArn]))
(s/def
:cognitect.aws.glue/RegisterSchemaVersionInput
(s/keys
:req-un
[:cognitect.aws.glue.RegisterSchemaVersionInput/SchemaId
:cognitect.aws.glue.RegisterSchemaVersionInput/SchemaDefinition]))
(s/def
:cognitect.aws.glue/DeleteColumnStatisticsForPartitionRequest
(s/keys
:req-un
[:cognitect.aws.glue.DeleteColumnStatisticsForPartitionRequest/DatabaseName
:cognitect.aws.glue.DeleteColumnStatisticsForPartitionRequest/TableName
:cognitect.aws.glue.DeleteColumnStatisticsForPartitionRequest/PartitionValues
:cognitect.aws.glue.DeleteColumnStatisticsForPartitionRequest/ColumnName]
:opt-un
[:cognitect.aws.glue.DeleteColumnStatisticsForPartitionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/UpdateMLTransformResponse
(s/keys :opt-un [:cognitect.aws.glue.UpdateMLTransformResponse/TransformId]))
(s/def :cognitect.aws.glue/CodeGenArgName string?)
(s/def
:cognitect.aws.glue/NodeName
(s/spec
#(re-matches
(re-pattern "([\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF]|[^\\r\\n])*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/DevEndpointNames
(s/coll-of :cognitect.aws.glue/GenericString :min-count 1 :max-count 25))
(s/def
:cognitect.aws.glue/CreateXMLClassifierRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateXMLClassifierRequest/Classification
:cognitect.aws.glue.CreateXMLClassifierRequest/Name]
:opt-un
[:cognitect.aws.glue.CreateXMLClassifierRequest/RowTag]))
(s/def
:cognitect.aws.glue/GetDataQualityRulesetResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetDataQualityRulesetResponse/TargetTable
:cognitect.aws.glue.GetDataQualityRulesetResponse/LastModifiedOn
:cognitect.aws.glue.GetDataQualityRulesetResponse/Description
:cognitect.aws.glue.GetDataQualityRulesetResponse/Ruleset
:cognitect.aws.glue.GetDataQualityRulesetResponse/RecommendationRunId
:cognitect.aws.glue.GetDataQualityRulesetResponse/Name
:cognitect.aws.glue.GetDataQualityRulesetResponse/CreatedOn]))
(s/def
:cognitect.aws.glue/UpdateJobFromSourceControlResponse
(s/keys :opt-un [:cognitect.aws.glue.UpdateJobFromSourceControlResponse/JobName]))
(s/def
:cognitect.aws.glue/RegisterSchemaVersionResponse
(s/keys
:opt-un
[:cognitect.aws.glue.RegisterSchemaVersionResponse/Status
:cognitect.aws.glue.RegisterSchemaVersionResponse/VersionNumber
:cognitect.aws.glue.RegisterSchemaVersionResponse/SchemaVersionId]))
(s/def :cognitect.aws.glue/BatchSize (s/spec (s/and int? #(<= 1 % 100)) :gen #(gen/choose 1 100)))
(s/def
:cognitect.aws.glue/StopSessionResponse
(s/keys :opt-un [:cognitect.aws.glue.StopSessionResponse/Id]))
(s/def
:cognitect.aws.glue/RemoveSchemaVersionMetadataResponse
(s/keys
:opt-un
[:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/MetadataKey
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/MetadataValue
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/LatestVersion
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/RegistryName
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/VersionNumber
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/SchemaName
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/SchemaArn
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/SchemaVersionId]))
(s/def
:cognitect.aws.glue/ListCustomEntityTypesRequest
(s/keys
:opt-un
[:cognitect.aws.glue.ListCustomEntityTypesRequest/Tags
:cognitect.aws.glue.ListCustomEntityTypesRequest/NextToken
:cognitect.aws.glue.ListCustomEntityTypesRequest/MaxResults]))
(s/def
:cognitect.aws.glue/CatalogKafkaSource
(s/keys
:req-un
[:cognitect.aws.glue.CatalogKafkaSource/Name
:cognitect.aws.glue.CatalogKafkaSource/Table
:cognitect.aws.glue.CatalogKafkaSource/Database]
:opt-un
[:cognitect.aws.glue.CatalogKafkaSource/StreamingOptions
:cognitect.aws.glue.CatalogKafkaSource/DetectSchema
:cognitect.aws.glue.CatalogKafkaSource/WindowSize
:cognitect.aws.glue.CatalogKafkaSource/DataPreviewOptions]))
(s/def :cognitect.aws.glue/JobName string?)
(s/def
:cognitect.aws.glue/BatchUpdatePartitionRequestEntry
(s/keys
:req-un
[:cognitect.aws.glue.BatchUpdatePartitionRequestEntry/PartitionValueList
:cognitect.aws.glue.BatchUpdatePartitionRequestEntry/PartitionInput]))
(s/def
:cognitect.aws.glue/ParquetCompressionType
(s/spec string? :gen #(s/gen #{"snappy" "none" "gzip" "uncompressed" "lzo"})))
(s/def :cognitect.aws.glue/ErrorString string?)
(s/def
:cognitect.aws.glue/ExportLabelsTaskRunProperties
(s/keys :opt-un [:cognitect.aws.glue.ExportLabelsTaskRunProperties/OutputS3Path]))
(s/def
:cognitect.aws.glue/CodeGenConfigurationNode
(s/keys
:opt-un
[:cognitect.aws.glue.CodeGenConfigurationNode/JDBCConnectorTarget
:cognitect.aws.glue.CodeGenConfigurationNode/RedshiftTarget
:cognitect.aws.glue.CodeGenConfigurationNode/S3CatalogSource
:cognitect.aws.glue.CodeGenConfigurationNode/Union
:cognitect.aws.glue.CodeGenConfigurationNode/S3GlueParquetTarget
:cognitect.aws.glue.CodeGenConfigurationNode/S3CatalogHudiSource
:cognitect.aws.glue.CodeGenConfigurationNode/SparkConnectorSource
:cognitect.aws.glue.CodeGenConfigurationNode/FillMissingValues
:cognitect.aws.glue.CodeGenConfigurationNode/Filter
:cognitect.aws.glue.CodeGenConfigurationNode/SplitFields
:cognitect.aws.glue.CodeGenConfigurationNode/PostgreSQLCatalogSource
:cognitect.aws.glue.CodeGenConfigurationNode/GovernedCatalogSource
:cognitect.aws.glue.CodeGenConfigurationNode/S3ParquetSource
:cognitect.aws.glue.CodeGenConfigurationNode/GovernedCatalogTarget
:cognitect.aws.glue.CodeGenConfigurationNode/S3DirectTarget
:cognitect.aws.glue.CodeGenConfigurationNode/AmazonRedshiftSource
:cognitect.aws.glue.CodeGenConfigurationNode/OracleSQLCatalogSource
:cognitect.aws.glue.CodeGenConfigurationNode/S3DeltaDirectTarget
:cognitect.aws.glue.CodeGenConfigurationNode/DirectKafkaSource
:cognitect.aws.glue.CodeGenConfigurationNode/JDBCConnectorSource
:cognitect.aws.glue.CodeGenConfigurationNode/DropNullFields
:cognitect.aws.glue.CodeGenConfigurationNode/S3JsonSource
:cognitect.aws.glue.CodeGenConfigurationNode/SparkConnectorTarget
:cognitect.aws.glue.CodeGenConfigurationNode/SelectFromCollection
:cognitect.aws.glue.CodeGenConfigurationNode/Spigot
:cognitect.aws.glue.CodeGenConfigurationNode/Aggregate
:cognitect.aws.glue.CodeGenConfigurationNode/S3CatalogTarget
:cognitect.aws.glue.CodeGenConfigurationNode/DynamoDBCatalogSource
:cognitect.aws.glue.CodeGenConfigurationNode/CatalogHudiSource
:cognitect.aws.glue.CodeGenConfigurationNode/S3HudiDirectTarget
:cognitect.aws.glue.CodeGenConfigurationNode/Merge
:cognitect.aws.glue.CodeGenConfigurationNode/PIIDetection
:cognitect.aws.glue.CodeGenConfigurationNode/RenameField
:cognitect.aws.glue.CodeGenConfigurationNode/EvaluateDataQuality
:cognitect.aws.glue.CodeGenConfigurationNode/DirectKinesisSource
:cognitect.aws.glue.CodeGenConfigurationNode/SparkSQL
:cognitect.aws.glue.CodeGenConfigurationNode/S3DeltaSource
:cognitect.aws.glue.CodeGenConfigurationNode/CatalogTarget
:cognitect.aws.glue.CodeGenConfigurationNode/SelectFields
:cognitect.aws.glue.CodeGenConfigurationNode/CustomCode
:cognitect.aws.glue.CodeGenConfigurationNode/S3CsvSource
:cognitect.aws.glue.CodeGenConfigurationNode/AthenaConnectorSource
:cognitect.aws.glue.CodeGenConfigurationNode/PostgreSQLCatalogTarget
:cognitect.aws.glue.CodeGenConfigurationNode/ApplyMapping
:cognitect.aws.glue.CodeGenConfigurationNode/OracleSQLCatalogTarget
:cognitect.aws.glue.CodeGenConfigurationNode/AmazonRedshiftTarget
:cognitect.aws.glue.CodeGenConfigurationNode/S3HudiCatalogTarget
:cognitect.aws.glue.CodeGenConfigurationNode/CatalogKinesisSource
:cognitect.aws.glue.CodeGenConfigurationNode/EvaluateDataQualityMultiFrame
:cognitect.aws.glue.CodeGenConfigurationNode/MySQLCatalogTarget
:cognitect.aws.glue.CodeGenConfigurationNode/MicrosoftSQLServerCatalogTarget
:cognitect.aws.glue.CodeGenConfigurationNode/MySQLCatalogSource
:cognitect.aws.glue.CodeGenConfigurationNode/RelationalCatalogSource
:cognitect.aws.glue.CodeGenConfigurationNode/DynamicTransform
:cognitect.aws.glue.CodeGenConfigurationNode/S3HudiSource
:cognitect.aws.glue.CodeGenConfigurationNode/CatalogDeltaSource
:cognitect.aws.glue.CodeGenConfigurationNode/RedshiftSource
:cognitect.aws.glue.CodeGenConfigurationNode/MicrosoftSQLServerCatalogSource
:cognitect.aws.glue.CodeGenConfigurationNode/DropDuplicates
:cognitect.aws.glue.CodeGenConfigurationNode/DirectJDBCSource
:cognitect.aws.glue.CodeGenConfigurationNode/Join
:cognitect.aws.glue.CodeGenConfigurationNode/DropFields
:cognitect.aws.glue.CodeGenConfigurationNode/S3DeltaCatalogTarget
:cognitect.aws.glue.CodeGenConfigurationNode/CatalogSource
:cognitect.aws.glue.CodeGenConfigurationNode/S3CatalogDeltaSource
:cognitect.aws.glue.CodeGenConfigurationNode/CatalogKafkaSource]))
(s/def
:cognitect.aws.glue/TagResourceRequest
(s/keys
:req-un
[:cognitect.aws.glue.TagResourceRequest/ResourceArn
:cognitect.aws.glue.TagResourceRequest/TagsToAdd]))
(s/def :cognitect.aws.glue/OptionList (s/coll-of :cognitect.aws.glue/Option))
(s/def
:cognitect.aws.glue/GetCrawlerResponse
(s/keys :opt-un [:cognitect.aws.glue.GetCrawlerResponse/Crawler]))
(s/def
:cognitect.aws.glue/LastCrawlStatus
(s/spec string? :gen #(s/gen #{"SUCCEEDED" "FAILED" "CANCELLED"})))
(s/def
:cognitect.aws.glue/FindMatchesMetrics
(s/keys
:opt-un
[:cognitect.aws.glue.FindMatchesMetrics/Precision
:cognitect.aws.glue.FindMatchesMetrics/ConfusionMatrix
:cognitect.aws.glue.FindMatchesMetrics/Recall
:cognitect.aws.glue.FindMatchesMetrics/ColumnImportances
:cognitect.aws.glue.FindMatchesMetrics/F1
:cognitect.aws.glue.FindMatchesMetrics/AreaUnderPRCurve]))
(s/def :cognitect.aws.glue/CrawlerHistoryList (s/coll-of :cognitect.aws.glue/CrawlerHistory))
(s/def :cognitect.aws.glue/OrchestrationStringList (s/coll-of :cognitect.aws.glue/GenericString))
(s/def :cognitect.aws.glue/CompressionType (s/spec string? :gen #(s/gen #{"gzip" "bzip2"})))
(s/def
:cognitect.aws.glue/JobBookmarkEntry
(s/keys
:opt-un
[:cognitect.aws.glue.JobBookmarkEntry/Run
:cognitect.aws.glue.JobBookmarkEntry/RunId
:cognitect.aws.glue.JobBookmarkEntry/JobName
:cognitect.aws.glue.JobBookmarkEntry/JobBookmark
:cognitect.aws.glue.JobBookmarkEntry/Attempt
:cognitect.aws.glue.JobBookmarkEntry/PreviousRunId
:cognitect.aws.glue.JobBookmarkEntry/Version]))
(s/def
:cognitect.aws.glue/JobRun
(s/keys
:opt-un
[:cognitect.aws.glue.JobRun/AllocatedCapacity
:cognitect.aws.glue.JobRun/Timeout
:cognitect.aws.glue.JobRun/MaxCapacity
:cognitect.aws.glue.JobRun/ExecutionTime
:cognitect.aws.glue.JobRun/Arguments
:cognitect.aws.glue.JobRun/JobName
:cognitect.aws.glue.JobRun/ExecutionClass
:cognitect.aws.glue.JobRun/NumberOfWorkers
:cognitect.aws.glue.JobRun/WorkerType
:cognitect.aws.glue.JobRun/LastModifiedOn
:cognitect.aws.glue.JobRun/ErrorMessage
:cognitect.aws.glue.JobRun/PredecessorRuns
:cognitect.aws.glue.JobRun/TriggerName
:cognitect.aws.glue.JobRun/GlueVersion
:cognitect.aws.glue.JobRun/Attempt
:cognitect.aws.glue.JobRun/SecurityConfiguration
:cognitect.aws.glue.JobRun/LogGroupName
:cognitect.aws.glue.JobRun/PreviousRunId
:cognitect.aws.glue.JobRun/StartedOn
:cognitect.aws.glue.JobRun/NotificationProperty
:cognitect.aws.glue.JobRun/DPUSeconds
:cognitect.aws.glue.JobRun/CompletedOn
:cognitect.aws.glue.JobRun/Id
:cognitect.aws.glue.JobRun/JobRunState]))
(s/def
:cognitect.aws.glue/EvaluateDataQuality
(s/keys
:req-un
[:cognitect.aws.glue.EvaluateDataQuality/Name
:cognitect.aws.glue.EvaluateDataQuality/Inputs
:cognitect.aws.glue.EvaluateDataQuality/Ruleset]
:opt-un
[:cognitect.aws.glue.EvaluateDataQuality/PublishingOptions
:cognitect.aws.glue.EvaluateDataQuality/StopJobOnFailureOptions
:cognitect.aws.glue.EvaluateDataQuality/Output]))
(s/def
:cognitect.aws.glue/DataLakePrincipal
(s/keys :opt-un [:cognitect.aws.glue.DataLakePrincipal/DataLakePrincipalIdentifier]))
(s/def :cognitect.aws.glue/ResourceType (s/spec string? :gen #(s/gen #{"FILE" "JAR" "ARCHIVE"})))
(s/def
:cognitect.aws.glue/BatchGetWorkflowsRequest
(s/keys
:req-un
[:cognitect.aws.glue.BatchGetWorkflowsRequest/Names]
:opt-un
[:cognitect.aws.glue.BatchGetWorkflowsRequest/IncludeGraph]))
(s/def
:cognitect.aws.glue/DirectKafkaSource
(s/keys
:req-un
[:cognitect.aws.glue.DirectKafkaSource/Name]
:opt-un
[:cognitect.aws.glue.DirectKafkaSource/StreamingOptions
:cognitect.aws.glue.DirectKafkaSource/DetectSchema
:cognitect.aws.glue.DirectKafkaSource/WindowSize
:cognitect.aws.glue.DirectKafkaSource/DataPreviewOptions]))
(s/def
:cognitect.aws.glue/BatchDeletePartitionValueList
(s/coll-of :cognitect.aws.glue/PartitionValueList :min-count 0 :max-count 25))
(s/def
:cognitect.aws.glue/SchemaDefinitionDiff
(s/spec #(re-matches (re-pattern ".*\\S.*") %) :gen #(gen/string)))
(s/def :cognitect.aws.glue/Classification string?)
(s/def :cognitect.aws.glue/TransformList (s/coll-of :cognitect.aws.glue/MLTransform))
(s/def :cognitect.aws.glue/ValueStringList (s/coll-of :cognitect.aws.glue/ValueString))
(s/def
:cognitect.aws.glue/KeySchemaElementList
(s/coll-of :cognitect.aws.glue/KeySchemaElement :min-count 1))
(s/def
:cognitect.aws.glue/UpdateTableRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateTableRequest/DatabaseName
:cognitect.aws.glue.UpdateTableRequest/TableInput]
:opt-un
[:cognitect.aws.glue.UpdateTableRequest/TransactionId
:cognitect.aws.glue.UpdateTableRequest/VersionId
:cognitect.aws.glue.UpdateTableRequest/SkipArchive
:cognitect.aws.glue.UpdateTableRequest/CatalogId]))
(s/def
:cognitect.aws.glue/CreateBlueprintRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateBlueprintRequest/Name
:cognitect.aws.glue.CreateBlueprintRequest/BlueprintLocation]
:opt-un
[:cognitect.aws.glue.CreateBlueprintRequest/Tags
:cognitect.aws.glue.CreateBlueprintRequest/Description]))
(s/def
:cognitect.aws.glue/StartCrawlerRequest
(s/keys :req-un [:cognitect.aws.glue.StartCrawlerRequest/Name]))
(s/def
:cognitect.aws.glue/CatalogDeltaSource
(s/keys
:req-un
[:cognitect.aws.glue.CatalogDeltaSource/Name
:cognitect.aws.glue.CatalogDeltaSource/Database
:cognitect.aws.glue.CatalogDeltaSource/Table]
:opt-un
[:cognitect.aws.glue.CatalogDeltaSource/OutputSchemas
:cognitect.aws.glue.CatalogDeltaSource/AdditionalDeltaOptions]))
(s/def
:cognitect.aws.glue/UpdateSchemaInput
(s/keys
:req-un
[:cognitect.aws.glue.UpdateSchemaInput/SchemaId]
:opt-un
[:cognitect.aws.glue.UpdateSchemaInput/SchemaVersionNumber
:cognitect.aws.glue.UpdateSchemaInput/Compatibility
:cognitect.aws.glue.UpdateSchemaInput/Description]))
(s/def
:cognitect.aws.glue/ListDevEndpointsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.ListDevEndpointsRequest/Tags
:cognitect.aws.glue.ListDevEndpointsRequest/NextToken
:cognitect.aws.glue.ListDevEndpointsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/ListTriggersRequest
(s/keys
:opt-un
[:cognitect.aws.glue.ListTriggersRequest/Tags
:cognitect.aws.glue.ListTriggersRequest/NextToken
:cognitect.aws.glue.ListTriggersRequest/DependentJobName
:cognitect.aws.glue.ListTriggersRequest/MaxResults]))
(s/def
:cognitect.aws.glue/GetClassifierRequest
(s/keys :req-un [:cognitect.aws.glue.GetClassifierRequest/Name]))
(s/def
:cognitect.aws.glue/CreateSchemaInput
(s/keys
:req-un
[:cognitect.aws.glue.CreateSchemaInput/SchemaName
:cognitect.aws.glue.CreateSchemaInput/DataFormat]
:opt-un
[:cognitect.aws.glue.CreateSchemaInput/Tags
:cognitect.aws.glue.CreateSchemaInput/Compatibility
:cognitect.aws.glue.CreateSchemaInput/Description
:cognitect.aws.glue.CreateSchemaInput/RegistryId
:cognitect.aws.glue.CreateSchemaInput/SchemaDefinition]))
(s/def
:cognitect.aws.glue/CodeGenNodeArgs
(s/coll-of :cognitect.aws.glue/CodeGenNodeArg :min-count 0 :max-count 50))
(s/def :cognitect.aws.glue/IsVersionValid boolean?)
(s/def
:cognitect.aws.glue/MicrosoftSQLServerCatalogTarget
(s/keys
:req-un
[:cognitect.aws.glue.MicrosoftSQLServerCatalogTarget/Name
:cognitect.aws.glue.MicrosoftSQLServerCatalogTarget/Inputs
:cognitect.aws.glue.MicrosoftSQLServerCatalogTarget/Database
:cognitect.aws.glue.MicrosoftSQLServerCatalogTarget/Table]))
(s/def :cognitect.aws.glue/BackfillErrors (s/coll-of :cognitect.aws.glue/BackfillError))
(s/def :cognitect.aws.glue/UpdateConnectionResponse (s/keys))
(s/def
:cognitect.aws.glue/OrchestrationArgumentsValue
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\r\\n\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/DeleteSessionResponse
(s/keys :opt-un [:cognitect.aws.glue.DeleteSessionResponse/Id]))
(s/def
:cognitect.aws.glue/OrchestrationS3Location
(s/spec #(re-matches (re-pattern "^s3://([^/]+)/([^/]+/)*([^/]+)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/CrawlerState
(s/spec string? :gen #(s/gen #{"READY" "STOPPING" "RUNNING"})))
(s/def
:cognitect.aws.glue/TaskRunFilterCriteria
(s/keys
:opt-un
[:cognitect.aws.glue.TaskRunFilterCriteria/StartedBefore
:cognitect.aws.glue.TaskRunFilterCriteria/Status
:cognitect.aws.glue.TaskRunFilterCriteria/TaskRunType
:cognitect.aws.glue.TaskRunFilterCriteria/StartedAfter]))
(s/def
:cognitect.aws.glue/ResetJobBookmarkRequest
(s/keys
:req-un
[:cognitect.aws.glue.ResetJobBookmarkRequest/JobName]
:opt-un
[:cognitect.aws.glue.ResetJobBookmarkRequest/RunId]))
(s/def
:cognitect.aws.glue/MaxRetries
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def :cognitect.aws.glue/SchemaPathString string?)
(s/def
:cognitect.aws.glue/WorkflowGraph
(s/keys
:opt-un
[:cognitect.aws.glue.WorkflowGraph/Nodes :cognitect.aws.glue.WorkflowGraph/Edges]))
(s/def
:cognitect.aws.glue/UpdateCrawlerScheduleRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateCrawlerScheduleRequest/CrawlerName]
:opt-un
[:cognitect.aws.glue.UpdateCrawlerScheduleRequest/Schedule]))
(s/def
:cognitect.aws.glue/NullCheckBoxList
(s/keys
:opt-un
[:cognitect.aws.glue.NullCheckBoxList/IsNegOne
:cognitect.aws.glue.NullCheckBoxList/IsEmpty
:cognitect.aws.glue.NullCheckBoxList/IsNullString]))
(s/def
:cognitect.aws.glue/FillMissingValues
(s/keys
:req-un
[:cognitect.aws.glue.FillMissingValues/Name
:cognitect.aws.glue.FillMissingValues/Inputs
:cognitect.aws.glue.FillMissingValues/ImputedPath]
:opt-un
[:cognitect.aws.glue.FillMissingValues/FilledPath]))
(s/def
:cognitect.aws.glue/SecurityConfigurationList
(s/coll-of :cognitect.aws.glue/SecurityConfiguration))
(s/def :cognitect.aws.glue/CrawlsFilterList (s/coll-of :cognitect.aws.glue/CrawlsFilter))
(s/def
:cognitect.aws.glue/SplitFields
(s/keys
:req-un
[:cognitect.aws.glue.SplitFields/Name
:cognitect.aws.glue.SplitFields/Inputs
:cognitect.aws.glue.SplitFields/Paths]))
(s/def
:cognitect.aws.glue/PublicKeysList
(s/coll-of :cognitect.aws.glue/GenericString :max-count 5))
(s/def
:cognitect.aws.glue/StartTriggerRequest
(s/keys :req-un [:cognitect.aws.glue.StartTriggerRequest/Name]))
(s/def
:cognitect.aws.glue/GetConnectionsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.GetConnectionsRequest/Filter
:cognitect.aws.glue.GetConnectionsRequest/NextToken
:cognitect.aws.glue.GetConnectionsRequest/HidePassword
:cognitect.aws.glue.GetConnectionsRequest/CatalogId
:cognitect.aws.glue.GetConnectionsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/GetSecurityConfigurationResponse
(s/keys :opt-un [:cognitect.aws.glue.GetSecurityConfigurationResponse/SecurityConfiguration]))
(s/def :cognitect.aws.glue/CreateCrawlerResponse (s/keys))
(s/def
:cognitect.aws.glue/ListCustomEntityTypesResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListCustomEntityTypesResponse/NextToken
:cognitect.aws.glue.ListCustomEntityTypesResponse/CustomEntityTypes]))
(s/def
:cognitect.aws.glue/JobBookmarksEncryptionMode
(s/spec string? :gen #(s/gen #{"DISABLED" "CSE-KMS"})))
(s/def
:cognitect.aws.glue/BackfillError
(s/keys
:opt-un
[:cognitect.aws.glue.BackfillError/Partitions :cognitect.aws.glue.BackfillError/Code]))
(s/def
:cognitect.aws.glue/EventBatchingCondition
(s/keys
:req-un
[:cognitect.aws.glue.EventBatchingCondition/BatchSize]
:opt-un
[:cognitect.aws.glue.EventBatchingCondition/BatchWindow]))
(s/def
:cognitect.aws.glue/StartingEventBatchCondition
(s/keys
:opt-un
[:cognitect.aws.glue.StartingEventBatchCondition/BatchSize
:cognitect.aws.glue.StartingEventBatchCondition/BatchWindow]))
(s/def
:cognitect.aws.glue/AggFunction
(s/spec
string?
:gen
#(s/gen
#{"var_pop"
"countDistinct"
"min"
"sum"
"max"
"count"
"sumDistinct"
"stddev_pop"
"var_samp"
"last"
"avg"
"kurtosis"
"stddev_samp"
"skewness"
"first"})))
(s/def
:cognitect.aws.glue/BatchStopJobRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.BatchStopJobRunRequest/JobName
:cognitect.aws.glue.BatchStopJobRunRequest/JobRunIds]))
(s/def
:cognitect.aws.glue/GetPartitionIndexesResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetPartitionIndexesResponse/NextToken
:cognitect.aws.glue.GetPartitionIndexesResponse/PartitionIndexDescriptorList]))
(s/def
:cognitect.aws.glue/GetCatalogImportStatusRequest
(s/keys :opt-un [:cognitect.aws.glue.GetCatalogImportStatusRequest/CatalogId]))
(s/def
:cognitect.aws.glue/DeleteTriggerRequest
(s/keys :req-un [:cognitect.aws.glue.DeleteTriggerRequest/Name]))
(s/def
:cognitect.aws.glue/CancelMLTaskRunResponse
(s/keys
:opt-un
[:cognitect.aws.glue.CancelMLTaskRunResponse/Status
:cognitect.aws.glue.CancelMLTaskRunResponse/TransformId
:cognitect.aws.glue.CancelMLTaskRunResponse/TaskRunId]))
(s/def
:cognitect.aws.glue/MongoDBTarget
(s/keys
:opt-un
[:cognitect.aws.glue.MongoDBTarget/Path
:cognitect.aws.glue.MongoDBTarget/ConnectionName
:cognitect.aws.glue.MongoDBTarget/ScanAll]))
(s/def
:cognitect.aws.glue/DeltaTarget
(s/keys
:opt-un
[:cognitect.aws.glue.DeltaTarget/ConnectionName
:cognitect.aws.glue.DeltaTarget/CreateNativeDeltaTable
:cognitect.aws.glue.DeltaTarget/DeltaTables
:cognitect.aws.glue.DeltaTarget/WriteManifest]))
(s/def
:cognitect.aws.glue/ListSchemasResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListSchemasResponse/Schemas
:cognitect.aws.glue.ListSchemasResponse/NextToken]))
(s/def
:cognitect.aws.glue/SecurityGroupIdList
(s/coll-of :cognitect.aws.glue/NameString :min-count 0 :max-count 50))
(s/def
:cognitect.aws.glue/JDBCConnectorTarget
(s/keys
:req-un
[:cognitect.aws.glue.JDBCConnectorTarget/Name
:cognitect.aws.glue.JDBCConnectorTarget/Inputs
:cognitect.aws.glue.JDBCConnectorTarget/ConnectionName
:cognitect.aws.glue.JDBCConnectorTarget/ConnectionTable
:cognitect.aws.glue.JDBCConnectorTarget/ConnectorName
:cognitect.aws.glue.JDBCConnectorTarget/ConnectionType]
:opt-un
[:cognitect.aws.glue.JDBCConnectorTarget/OutputSchemas
:cognitect.aws.glue.JDBCConnectorTarget/AdditionalOptions]))
(s/def
:cognitect.aws.glue/RuntimeNameString
(s/spec #(re-matches (re-pattern ".*") %) :gen #(gen/string)))
(s/def :cognitect.aws.glue/JsonValue string?)
(s/def :cognitect.aws.glue/ColumnStatisticsList (s/coll-of :cognitect.aws.glue/ColumnStatistics))
(s/def
:cognitect.aws.glue/LineageConfiguration
(s/keys :opt-un [:cognitect.aws.glue.LineageConfiguration/CrawlerLineageSettings]))
(s/def :cognitect.aws.glue/CreatePartitionIndexResponse (s/keys))
(s/def
:cognitect.aws.glue/UpdateDatabaseRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateDatabaseRequest/Name
:cognitect.aws.glue.UpdateDatabaseRequest/DatabaseInput]
:opt-un
[:cognitect.aws.glue.UpdateDatabaseRequest/CatalogId]))
(s/def
:cognitect.aws.glue/CrawlerSecurityConfiguration
(s/spec
(s/and string? #(<= 0 (count %) 128))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 128) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/ErrorDetail
(s/keys
:opt-un
[:cognitect.aws.glue.ErrorDetail/ErrorMessage :cognitect.aws.glue.ErrorDetail/ErrorCode]))
(s/def
:cognitect.aws.glue/CreateRegistryResponse
(s/keys
:opt-un
[:cognitect.aws.glue.CreateRegistryResponse/RegistryArn
:cognitect.aws.glue.CreateRegistryResponse/Tags
:cognitect.aws.glue.CreateRegistryResponse/Description
:cognitect.aws.glue.CreateRegistryResponse/RegistryName]))
(s/def
:cognitect.aws.glue/ValueString
(s/spec
(s/and string? #(>= 1024 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 1024) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/DateColumnStatisticsData
(s/keys
:req-un
[:cognitect.aws.glue.DateColumnStatisticsData/NumberOfNulls
:cognitect.aws.glue.DateColumnStatisticsData/NumberOfDistinctValues]
:opt-un
[:cognitect.aws.glue.DateColumnStatisticsData/MaximumValue
:cognitect.aws.glue.DateColumnStatisticsData/MinimumValue]))
(s/def
:cognitect.aws.glue/CreatePartitionRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreatePartitionRequest/DatabaseName
:cognitect.aws.glue.CreatePartitionRequest/TableName
:cognitect.aws.glue.CreatePartitionRequest/PartitionInput]
:opt-un
[:cognitect.aws.glue.CreatePartitionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/Timeout
(s/spec (s/and int? #(<= 1 %)) :gen #(gen/choose 1 Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/UpdateJobFromSourceControlRequest
(s/keys
:opt-un
[:cognitect.aws.glue.UpdateJobFromSourceControlRequest/RepositoryOwner
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/AuthToken
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/AuthStrategy
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/RepositoryName
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/Folder
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/BranchName
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/JobName
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/CommitId
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/Provider]))
(s/def
:cognitect.aws.glue/GetMLTaskRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetMLTaskRunRequest/TransformId
:cognitect.aws.glue.GetMLTaskRunRequest/TaskRunId]))
(s/def
:cognitect.aws.glue/GetResourcePoliciesResponseList
(s/coll-of :cognitect.aws.glue/GluePolicy))
(s/def
:cognitect.aws.glue/CreateDevEndpointResponse
(s/keys
:opt-un
[:cognitect.aws.glue.CreateDevEndpointResponse/ZeppelinRemoteSparkInterpreterPort
:cognitect.aws.glue.CreateDevEndpointResponse/CreatedTimestamp
:cognitect.aws.glue.CreateDevEndpointResponse/SecurityGroupIds
:cognitect.aws.glue.CreateDevEndpointResponse/YarnEndpointAddress
:cognitect.aws.glue.CreateDevEndpointResponse/SubnetId
:cognitect.aws.glue.CreateDevEndpointResponse/Arguments
:cognitect.aws.glue.CreateDevEndpointResponse/ExtraJarsS3Path
:cognitect.aws.glue.CreateDevEndpointResponse/RoleArn
:cognitect.aws.glue.CreateDevEndpointResponse/NumberOfNodes
:cognitect.aws.glue.CreateDevEndpointResponse/NumberOfWorkers
:cognitect.aws.glue.CreateDevEndpointResponse/WorkerType
:cognitect.aws.glue.CreateDevEndpointResponse/AvailabilityZone
:cognitect.aws.glue.CreateDevEndpointResponse/Status
:cognitect.aws.glue.CreateDevEndpointResponse/EndpointName
:cognitect.aws.glue.CreateDevEndpointResponse/GlueVersion
:cognitect.aws.glue.CreateDevEndpointResponse/VpcId
:cognitect.aws.glue.CreateDevEndpointResponse/FailureReason
:cognitect.aws.glue.CreateDevEndpointResponse/SecurityConfiguration
:cognitect.aws.glue.CreateDevEndpointResponse/ExtraPythonLibsS3Path]))
(s/def
:cognitect.aws.glue/ColumnRowFilter
(s/keys
:opt-un
[:cognitect.aws.glue.ColumnRowFilter/ColumnName
:cognitect.aws.glue.ColumnRowFilter/RowFilterExpression]))
(s/def
:cognitect.aws.glue/GetWorkflowRunResponse
(s/keys :opt-un [:cognitect.aws.glue.GetWorkflowRunResponse/Run]))
(s/def
:cognitect.aws.glue/StartDataQualityRulesetEvaluationRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/DataSource
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/Role
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/RulesetNames]
:opt-un
[:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/Timeout
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/AdditionalRunOptions
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/AdditionalDataSources
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/NumberOfWorkers
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/ClientToken]))
(s/def
:cognitect.aws.glue/TableVersion
(s/keys
:opt-un
[:cognitect.aws.glue.TableVersion/VersionId :cognitect.aws.glue.TableVersion/Table]))
(s/def
:cognitect.aws.glue/GetWorkflowRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetWorkflowRequest/Name]
:opt-un
[:cognitect.aws.glue.GetWorkflowRequest/IncludeGraph]))
(s/def
:cognitect.aws.glue/Comparator
(s/spec
string?
:gen
#(s/gen #{"GREATER_THAN" "GREATER_THAN_EQUALS" "LESS_THAN" "EQUALS" "LESS_THAN_EQUALS"})))
(s/def
:cognitect.aws.glue/GetStatementRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetStatementRequest/SessionId :cognitect.aws.glue.GetStatementRequest/Id]
:opt-un
[:cognitect.aws.glue.GetStatementRequest/RequestOrigin]))
(s/def
:cognitect.aws.glue/GetDataQualityRulesetEvaluationRunRequest
(s/keys :req-un [:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunRequest/RunId]))
(s/def :cognitect.aws.glue/RunId string?)
(s/def
:cognitect.aws.glue/GetJobsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.GetJobsRequest/NextToken :cognitect.aws.glue.GetJobsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/ListJobsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListJobsResponse/NextToken :cognitect.aws.glue.ListJobsResponse/JobNames]))
(s/def
:cognitect.aws.glue/CsvQuoteSymbol
(s/spec #(re-matches (re-pattern "[^\\r\\n]") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/GetTriggerResponse
(s/keys :opt-un [:cognitect.aws.glue.GetTriggerResponse/Trigger]))
(s/def
:cognitect.aws.glue/BatchStopJobRunSuccessfulSubmissionList
(s/coll-of :cognitect.aws.glue/BatchStopJobRunSuccessfulSubmission))
(s/def :cognitect.aws.glue/Path string?)
(s/def
:cognitect.aws.glue/DeleteClassifierRequest
(s/keys :req-un [:cognitect.aws.glue.DeleteClassifierRequest/Name]))
(s/def
:cognitect.aws.glue/StartBlueprintRunResponse
(s/keys :opt-un [:cognitect.aws.glue.StartBlueprintRunResponse/RunId]))
(s/def
:cognitect.aws.glue/GetRegistryResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetRegistryResponse/RegistryArn
:cognitect.aws.glue.GetRegistryResponse/Status
:cognitect.aws.glue.GetRegistryResponse/CreatedTime
:cognitect.aws.glue.GetRegistryResponse/Description
:cognitect.aws.glue.GetRegistryResponse/UpdatedTime
:cognitect.aws.glue.GetRegistryResponse/RegistryName]))
(s/def
:cognitect.aws.glue/FederatedTable
(s/keys
:opt-un
[:cognitect.aws.glue.FederatedTable/DatabaseIdentifier
:cognitect.aws.glue.FederatedTable/ConnectionName
:cognitect.aws.glue.FederatedTable/Identifier]))
(s/def
:cognitect.aws.glue/BatchGetDataQualityResultResponse
(s/keys
:req-un
[:cognitect.aws.glue.BatchGetDataQualityResultResponse/Results]
:opt-un
[:cognitect.aws.glue.BatchGetDataQualityResultResponse/ResultsNotFound]))
(s/def :cognitect.aws.glue/PathList (s/coll-of :cognitect.aws.glue/Path))
(s/def
:cognitect.aws.glue/DoubleValue
(s/spec double? :gen #(gen/double* {:infinite? false, :NaN? false})))
(s/def :cognitect.aws.glue/LimitedStringList (s/coll-of :cognitect.aws.glue/GenericLimitedString))
(s/def
:cognitect.aws.glue/FilterExpression
(s/keys
:req-un
[:cognitect.aws.glue.FilterExpression/Operation :cognitect.aws.glue.FilterExpression/Values]
:opt-un
[:cognitect.aws.glue.FilterExpression/Negated]))
(s/def :cognitect.aws.glue/DeleteCrawlerResponse (s/keys))
(s/def
:cognitect.aws.glue/GetPartitionRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetPartitionRequest/DatabaseName
:cognitect.aws.glue.GetPartitionRequest/TableName
:cognitect.aws.glue.GetPartitionRequest/PartitionValues]
:opt-un
[:cognitect.aws.glue.GetPartitionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/GetWorkflowRunPropertiesRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetWorkflowRunPropertiesRequest/Name
:cognitect.aws.glue.GetWorkflowRunPropertiesRequest/RunId]))
(s/def
:cognitect.aws.glue/TargetFormat
(s/spec string? :gen #(s/gen #{"json" "orc" "avro" "parquet" "delta" "csv" "hudi"})))
(s/def
:cognitect.aws.glue/JoinColumns
(s/coll-of :cognitect.aws.glue/JoinColumn :min-count 2 :max-count 2))
(s/def
:cognitect.aws.glue/MLUserDataEncryptionModeString
(s/spec string? :gen #(s/gen #{"DISABLED" "SSE-KMS"})))
(s/def
:cognitect.aws.glue/DataQualityResultIds
(s/coll-of :cognitect.aws.glue/HashString :min-count 1 :max-count 100))
(s/def
:cognitect.aws.glue/GetClassifiersRequest
(s/keys
:opt-un
[:cognitect.aws.glue.GetClassifiersRequest/NextToken
:cognitect.aws.glue.GetClassifiersRequest/MaxResults]))
(s/def
:cognitect.aws.glue/SchemaChangePolicy
(s/keys
:opt-un
[:cognitect.aws.glue.SchemaChangePolicy/DeleteBehavior
:cognitect.aws.glue.SchemaChangePolicy/UpdateBehavior]))
(s/def
:cognitect.aws.glue/ListBlueprintsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListBlueprintsResponse/NextToken
:cognitect.aws.glue.ListBlueprintsResponse/Blueprints]))
(s/def
:cognitect.aws.glue/TransformSortColumnType
(s/spec string? :gen #(s/gen #{"LAST_MODIFIED" "NAME" "STATUS" "TRANSFORM_TYPE" "CREATED"})))
(s/def
:cognitect.aws.glue/PhysicalConnectionRequirements
(s/keys
:opt-un
[:cognitect.aws.glue.PhysicalConnectionRequirements/SubnetId
:cognitect.aws.glue.PhysicalConnectionRequirements/AvailabilityZone
:cognitect.aws.glue.PhysicalConnectionRequirements/SecurityGroupIdList]))
(s/def
:cognitect.aws.glue/ColumnStatisticsError
(s/keys
:opt-un
[:cognitect.aws.glue.ColumnStatisticsError/Error
:cognitect.aws.glue.ColumnStatisticsError/ColumnStatistics]))
(s/def
:cognitect.aws.glue/Table
(s/keys
:req-un
[:cognitect.aws.glue.Table/Name]
:opt-un
[:cognitect.aws.glue.Table/ViewExpandedText
:cognitect.aws.glue.Table/FederatedTable
:cognitect.aws.glue.Table/UpdateTime
:cognitect.aws.glue.Table/DatabaseName
:cognitect.aws.glue.Table/TargetTable
:cognitect.aws.glue.Table/VersionId
:cognitect.aws.glue.Table/Parameters
:cognitect.aws.glue.Table/StorageDescriptor
:cognitect.aws.glue.Table/CreatedBy
:cognitect.aws.glue.Table/PartitionKeys
:cognitect.aws.glue.Table/Description
:cognitect.aws.glue.Table/Owner
:cognitect.aws.glue.Table/TableType
:cognitect.aws.glue.Table/CreateTime
:cognitect.aws.glue.Table/LastAccessTime
:cognitect.aws.glue.Table/CatalogId
:cognitect.aws.glue.Table/Retention
:cognitect.aws.glue.Table/IsRegisteredWithLakeFormation
:cognitect.aws.glue.Table/LastAnalyzedTime
:cognitect.aws.glue.Table/ViewOriginalText]))
(s/def
:cognitect.aws.glue/UpdateDevEndpointRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateDevEndpointRequest/EndpointName]
:opt-un
[:cognitect.aws.glue.UpdateDevEndpointRequest/DeleteArguments
:cognitect.aws.glue.UpdateDevEndpointRequest/AddArguments
:cognitect.aws.glue.UpdateDevEndpointRequest/UpdateEtlLibraries
:cognitect.aws.glue.UpdateDevEndpointRequest/AddPublicKeys
:cognitect.aws.glue.UpdateDevEndpointRequest/CustomLibraries
:cognitect.aws.glue.UpdateDevEndpointRequest/DeletePublicKeys
:cognitect.aws.glue.UpdateDevEndpointRequest/PublicKey]))
(s/def :cognitect.aws.glue/TagResourceResponse (s/keys))
(s/def :cognitect.aws.glue/MetadataOperation (s/spec string? :gen #(s/gen #{"CREATE"})))
(s/def :cognitect.aws.glue/UpdateCrawlerScheduleResponse (s/keys))
(s/def :cognitect.aws.glue/LatestSchemaVersionBoolean boolean?)
(s/def
:cognitect.aws.glue/BatchGetCrawlersResponse
(s/keys
:opt-un
[:cognitect.aws.glue.BatchGetCrawlersResponse/Crawlers
:cognitect.aws.glue.BatchGetCrawlersResponse/CrawlersNotFound]))
(s/def
:cognitect.aws.glue/SparkConnectorTarget
(s/keys
:req-un
[:cognitect.aws.glue.SparkConnectorTarget/Name
:cognitect.aws.glue.SparkConnectorTarget/Inputs
:cognitect.aws.glue.SparkConnectorTarget/ConnectionName
:cognitect.aws.glue.SparkConnectorTarget/ConnectorName
:cognitect.aws.glue.SparkConnectorTarget/ConnectionType]
:opt-un
[:cognitect.aws.glue.SparkConnectorTarget/OutputSchemas
:cognitect.aws.glue.SparkConnectorTarget/AdditionalOptions]))
(s/def
:cognitect.aws.glue/BatchStopJobRunResponse
(s/keys
:opt-un
[:cognitect.aws.glue.BatchStopJobRunResponse/SuccessfulSubmissions
:cognitect.aws.glue.BatchStopJobRunResponse/Errors]))
(s/def :cognitect.aws.glue/PutWorkflowRunPropertiesResponse (s/keys))
(s/def
:cognitect.aws.glue/TaskRun
(s/keys
:opt-un
[:cognitect.aws.glue.TaskRun/ExecutionTime
:cognitect.aws.glue.TaskRun/LastModifiedOn
:cognitect.aws.glue.TaskRun/Status
:cognitect.aws.glue.TaskRun/TransformId
:cognitect.aws.glue.TaskRun/Properties
:cognitect.aws.glue.TaskRun/TaskRunId
:cognitect.aws.glue.TaskRun/ErrorString
:cognitect.aws.glue.TaskRun/LogGroupName
:cognitect.aws.glue.TaskRun/StartedOn
:cognitect.aws.glue.TaskRun/CompletedOn]))
(s/def
:cognitect.aws.glue/TriggerType
(s/spec string? :gen #(s/gen #{"CONDITIONAL" "ON_DEMAND" "EVENT" "SCHEDULED"})))
(s/def
:cognitect.aws.glue/UpdateColumnStatisticsForTableResponse
(s/keys :opt-un [:cognitect.aws.glue.UpdateColumnStatisticsForTableResponse/Errors]))
(s/def
:cognitect.aws.glue/UserDefinedFunction
(s/keys
:opt-un
[:cognitect.aws.glue.UserDefinedFunction/OwnerName
:cognitect.aws.glue.UserDefinedFunction/FunctionName
:cognitect.aws.glue.UserDefinedFunction/DatabaseName
:cognitect.aws.glue.UserDefinedFunction/ResourceUris
:cognitect.aws.glue.UserDefinedFunction/OwnerType
:cognitect.aws.glue.UserDefinedFunction/ClassName
:cognitect.aws.glue.UserDefinedFunction/CreateTime
:cognitect.aws.glue.UserDefinedFunction/CatalogId]))
(s/def
:cognitect.aws.glue/OpenTableFormatInput
(s/keys :opt-un [:cognitect.aws.glue.OpenTableFormatInput/IcebergInput]))
(s/def
:cognitect.aws.glue/UpdateColumnStatisticsList
(s/coll-of :cognitect.aws.glue/ColumnStatistics :min-count 0 :max-count 25))
(s/def :cognitect.aws.glue/BoxedLong (s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/JobUpdate
(s/keys
:opt-un
[:cognitect.aws.glue.JobUpdate/AllocatedCapacity
:cognitect.aws.glue.JobUpdate/Timeout
:cognitect.aws.glue.JobUpdate/MaxCapacity
:cognitect.aws.glue.JobUpdate/LogUri
:cognitect.aws.glue.JobUpdate/ExecutionClass
:cognitect.aws.glue.JobUpdate/DefaultArguments
:cognitect.aws.glue.JobUpdate/NumberOfWorkers
:cognitect.aws.glue.JobUpdate/WorkerType
:cognitect.aws.glue.JobUpdate/NonOverridableArguments
:cognitect.aws.glue.JobUpdate/Connections
:cognitect.aws.glue.JobUpdate/Role
:cognitect.aws.glue.JobUpdate/SourceControlDetails
:cognitect.aws.glue.JobUpdate/GlueVersion
:cognitect.aws.glue.JobUpdate/Description
:cognitect.aws.glue.JobUpdate/SecurityConfiguration
:cognitect.aws.glue.JobUpdate/Command
:cognitect.aws.glue.JobUpdate/MaxRetries
:cognitect.aws.glue.JobUpdate/ExecutionProperty
:cognitect.aws.glue.JobUpdate/NotificationProperty
:cognitect.aws.glue.JobUpdate/CodeGenConfigurationNodes]))
(s/def
:cognitect.aws.glue/UpdateSourceControlFromJobResponse
(s/keys :opt-un [:cognitect.aws.glue.UpdateSourceControlFromJobResponse/JobName]))
(s/def :cognitect.aws.glue/FilterValues (s/coll-of :cognitect.aws.glue/FilterValue))
(s/def
:cognitect.aws.glue/GetDataCatalogEncryptionSettingsRequest
(s/keys :opt-un [:cognitect.aws.glue.GetDataCatalogEncryptionSettingsRequest/CatalogId]))
(s/def :cognitect.aws.glue/CreateUserDefinedFunctionResponse (s/keys))
(s/def
:cognitect.aws.glue/DeltaTargetCompressionType
(s/spec string? :gen #(s/gen #{"snappy" "uncompressed"})))
(s/def
:cognitect.aws.glue/PutSchemaVersionMetadataResponse
(s/keys
:opt-un
[:cognitect.aws.glue.PutSchemaVersionMetadataResponse/MetadataKey
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/MetadataValue
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/LatestVersion
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/RegistryName
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/VersionNumber
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/SchemaName
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/SchemaArn
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/SchemaVersionId]))
(s/def :cognitect.aws.glue/Logical (s/spec string? :gen #(s/gen #{"AND" "ANY"})))
(s/def
:cognitect.aws.glue/UpdateConnectionRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateConnectionRequest/Name
:cognitect.aws.glue.UpdateConnectionRequest/ConnectionInput]
:opt-un
[:cognitect.aws.glue.UpdateConnectionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/HashString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/UpdateJsonClassifierRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateJsonClassifierRequest/Name]
:opt-un
[:cognitect.aws.glue.UpdateJsonClassifierRequest/JsonPath]))
(s/def
:cognitect.aws.glue/CreateDevEndpointRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateDevEndpointRequest/EndpointName
:cognitect.aws.glue.CreateDevEndpointRequest/RoleArn]
:opt-un
[:cognitect.aws.glue.CreateDevEndpointRequest/SecurityGroupIds
:cognitect.aws.glue.CreateDevEndpointRequest/SubnetId
:cognitect.aws.glue.CreateDevEndpointRequest/Arguments
:cognitect.aws.glue.CreateDevEndpointRequest/ExtraJarsS3Path
:cognitect.aws.glue.CreateDevEndpointRequest/NumberOfNodes
:cognitect.aws.glue.CreateDevEndpointRequest/Tags
:cognitect.aws.glue.CreateDevEndpointRequest/NumberOfWorkers
:cognitect.aws.glue.CreateDevEndpointRequest/WorkerType
:cognitect.aws.glue.CreateDevEndpointRequest/PublicKeys
:cognitect.aws.glue.CreateDevEndpointRequest/GlueVersion
:cognitect.aws.glue.CreateDevEndpointRequest/SecurityConfiguration
:cognitect.aws.glue.CreateDevEndpointRequest/ExtraPythonLibsS3Path
:cognitect.aws.glue.CreateDevEndpointRequest/PublicKey]))
(s/def
:cognitect.aws.glue/ConnectionInput
(s/keys
:req-un
[:cognitect.aws.glue.ConnectionInput/Name
:cognitect.aws.glue.ConnectionInput/ConnectionType
:cognitect.aws.glue.ConnectionInput/ConnectionProperties]
:opt-un
[:cognitect.aws.glue.ConnectionInput/PhysicalConnectionRequirements
:cognitect.aws.glue.ConnectionInput/Description
:cognitect.aws.glue.ConnectionInput/MatchCriteria]))
(s/def
:cognitect.aws.glue/ParametersMapValue
(s/spec
(s/and string? #(>= 512000 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 512000) #(gen/vector (gen/char-alpha) %))))))
(s/def :cognitect.aws.glue/CancelStatementResponse (s/keys))
(s/def
:cognitect.aws.glue/ColumnStatisticsType
(s/spec string? :gen #(s/gen #{"DATE" "DOUBLE" "STRING" "DECIMAL" "LONG" "BOOLEAN" "BINARY"})))
(s/def
:cognitect.aws.glue/DeleteSchemaInput
(s/keys :req-un [:cognitect.aws.glue.DeleteSchemaInput/SchemaId]))
(s/def
:cognitect.aws.glue/DeleteCustomEntityTypeRequest
(s/keys :req-un [:cognitect.aws.glue.DeleteCustomEntityTypeRequest/Name]))
(s/def
:cognitect.aws.glue/GetColumnStatisticsForTableResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetColumnStatisticsForTableResponse/ColumnStatisticsList
:cognitect.aws.glue.GetColumnStatisticsForTableResponse/Errors]))
(s/def
:cognitect.aws.glue/ParametersMap
(s/map-of :cognitect.aws.glue/KeyString :cognitect.aws.glue/ParametersMapValue))
(s/def
:cognitect.aws.glue/AmazonRedshiftSource
(s/keys
:opt-un
[:cognitect.aws.glue.AmazonRedshiftSource/Data :cognitect.aws.glue.AmazonRedshiftSource/Name]))
(s/def
:cognitect.aws.glue/GetMappingRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetMappingRequest/Source]
:opt-un
[:cognitect.aws.glue.GetMappingRequest/Sinks :cognitect.aws.glue.GetMappingRequest/Location]))
(s/def :cognitect.aws.glue/MongoDBTargetList (s/coll-of :cognitect.aws.glue/MongoDBTarget))
(s/def
:cognitect.aws.glue/ExecutionTime
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/DeleteSchemaVersionsInput
(s/keys
:req-un
[:cognitect.aws.glue.DeleteSchemaVersionsInput/SchemaId
:cognitect.aws.glue.DeleteSchemaVersionsInput/Versions]))
(s/def
:cognitect.aws.glue/MatchCriteria
(s/coll-of :cognitect.aws.glue/NameString :min-count 0 :max-count 10))
(s/def :cognitect.aws.glue/DeleteSecurityConfigurationResponse (s/keys))
(s/def
:cognitect.aws.glue/Filter
(s/keys
:req-un
[:cognitect.aws.glue.Filter/Name
:cognitect.aws.glue.Filter/Inputs
:cognitect.aws.glue.Filter/LogicalOperator
:cognitect.aws.glue.Filter/Filters]))
(s/def
:cognitect.aws.glue/GetResourcePolicyRequest
(s/keys :opt-un [:cognitect.aws.glue.GetResourcePolicyRequest/ResourceArn]))
(s/def
:cognitect.aws.glue/GetClassifiersResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetClassifiersResponse/Classifiers
:cognitect.aws.glue.GetClassifiersResponse/NextToken]))
(s/def
:cognitect.aws.glue/BatchGetDevEndpointsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.BatchGetDevEndpointsResponse/DevEndpointsNotFound
:cognitect.aws.glue.BatchGetDevEndpointsResponse/DevEndpoints]))
(s/def
:cognitect.aws.glue/UpdateColumnStatisticsForPartitionResponse
(s/keys :opt-un [:cognitect.aws.glue.UpdateColumnStatisticsForPartitionResponse/Errors]))
(s/def
:cognitect.aws.glue/AuthTokenString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/GovernedCatalogSource
(s/keys
:req-un
[:cognitect.aws.glue.GovernedCatalogSource/Name
:cognitect.aws.glue.GovernedCatalogSource/Database
:cognitect.aws.glue.GovernedCatalogSource/Table]
:opt-un
[:cognitect.aws.glue.GovernedCatalogSource/AdditionalOptions
:cognitect.aws.glue.GovernedCatalogSource/PartitionPredicate]))
(s/def
:cognitect.aws.glue/CreateWorkflowRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateWorkflowRequest/Name]
:opt-un
[:cognitect.aws.glue.CreateWorkflowRequest/Tags
:cognitect.aws.glue.CreateWorkflowRequest/Description
:cognitect.aws.glue.CreateWorkflowRequest/MaxConcurrentRuns
:cognitect.aws.glue.CreateWorkflowRequest/DefaultRunProperties]))
(s/def
:cognitect.aws.glue/CatalogTarget
(s/keys
:req-un
[:cognitect.aws.glue.CatalogTarget/DatabaseName :cognitect.aws.glue.CatalogTarget/Tables]
:opt-un
[:cognitect.aws.glue.CatalogTarget/EventQueueArn
:cognitect.aws.glue.CatalogTarget/ConnectionName
:cognitect.aws.glue.CatalogTarget/DlqEventQueueArn]))
(s/def
:cognitect.aws.glue/SchemaVersionStatus
(s/spec string? :gen #(s/gen #{"AVAILABLE" "PENDING" "FAILURE" "DELETING"})))
(s/def
:cognitect.aws.glue/CreateJobRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateJobRequest/Name
:cognitect.aws.glue.CreateJobRequest/Role
:cognitect.aws.glue.CreateJobRequest/Command]
:opt-un
[:cognitect.aws.glue.CreateJobRequest/AllocatedCapacity
:cognitect.aws.glue.CreateJobRequest/Timeout
:cognitect.aws.glue.CreateJobRequest/MaxCapacity
:cognitect.aws.glue.CreateJobRequest/LogUri
:cognitect.aws.glue.CreateJobRequest/ExecutionClass
:cognitect.aws.glue.CreateJobRequest/Tags
:cognitect.aws.glue.CreateJobRequest/DefaultArguments
:cognitect.aws.glue.CreateJobRequest/NumberOfWorkers
:cognitect.aws.glue.CreateJobRequest/WorkerType
:cognitect.aws.glue.CreateJobRequest/NonOverridableArguments
:cognitect.aws.glue.CreateJobRequest/Connections
:cognitect.aws.glue.CreateJobRequest/SourceControlDetails
:cognitect.aws.glue.CreateJobRequest/GlueVersion
:cognitect.aws.glue.CreateJobRequest/Description
:cognitect.aws.glue.CreateJobRequest/SecurityConfiguration
:cognitect.aws.glue.CreateJobRequest/MaxRetries
:cognitect.aws.glue.CreateJobRequest/ExecutionProperty
:cognitect.aws.glue.CreateJobRequest/NotificationProperty
:cognitect.aws.glue.CreateJobRequest/CodeGenConfigurationNodes]))
(s/def :cognitect.aws.glue/DeleteClassifierResponse (s/keys))
(s/def
:cognitect.aws.glue/SchemaDefinitionString
(s/spec #(re-matches (re-pattern ".*\\S.*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/DeleteSecurityConfigurationRequest
(s/keys :req-un [:cognitect.aws.glue.DeleteSecurityConfigurationRequest/Name]))
(s/def
:cognitect.aws.glue/HudiTargetCompressionType
(s/spec string? :gen #(s/gen #{"snappy" "gzip" "uncompressed" "lzo"})))
(s/def
:cognitect.aws.glue/GetColumnStatisticsForTableRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetColumnStatisticsForTableRequest/DatabaseName
:cognitect.aws.glue.GetColumnStatisticsForTableRequest/TableName
:cognitect.aws.glue.GetColumnStatisticsForTableRequest/ColumnNames]
:opt-un
[:cognitect.aws.glue.GetColumnStatisticsForTableRequest/CatalogId]))
(s/def
:cognitect.aws.glue/TaskType
(s/spec
string?
:gen
#(s/gen
#{"LABELING_SET_GENERATION" "IMPORT_LABELS" "FIND_MATCHES" "EXPORT_LABELS" "EVALUATION"})))
(s/def
:cognitect.aws.glue/GetTableRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetTableRequest/DatabaseName :cognitect.aws.glue.GetTableRequest/Name]
:opt-un
[:cognitect.aws.glue.GetTableRequest/TransactionId
:cognitect.aws.glue.GetTableRequest/QueryAsOfTime
:cognitect.aws.glue.GetTableRequest/CatalogId]))
(s/def :cognitect.aws.glue/RegistryListDefinition (s/coll-of :cognitect.aws.glue/RegistryListItem))
(s/def :cognitect.aws.glue/TimestampValue inst?)
(s/def
:cognitect.aws.glue/TriggerState
(s/spec
string?
:gen
#(s/gen
#{"DEACTIVATING"
"UPDATING"
"ACTIVATING"
"DELETING"
"CREATING"
"DEACTIVATED"
"ACTIVATED"
"CREATED"})))
(s/def
:cognitect.aws.glue/ListSchemaVersionsInput
(s/keys
:req-un
[:cognitect.aws.glue.ListSchemaVersionsInput/SchemaId]
:opt-un
[:cognitect.aws.glue.ListSchemaVersionsInput/NextToken
:cognitect.aws.glue.ListSchemaVersionsInput/MaxResults]))
(s/def :cognitect.aws.glue/DagNodes (s/coll-of :cognitect.aws.glue/CodeGenNode))
(s/def
:cognitect.aws.glue/QuoteChar
(s/spec string? :gen #(s/gen #{"quote" "disabled" "single_quote" "quillemet"})))
(s/def
:cognitect.aws.glue/DataQualityRulesetString
(s/spec
(s/and string? #(<= 1 (count %) 65536))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 65536) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/PositiveLong
(s/spec (s/and int? #(<= 1 %)) :gen #(gen/choose 1 Long/MAX_VALUE)))
(s/def :cognitect.aws.glue/LimitedPathList (s/coll-of :cognitect.aws.glue/LimitedStringList))
(s/def
:cognitect.aws.glue/GovernedCatalogTarget
(s/keys
:req-un
[:cognitect.aws.glue.GovernedCatalogTarget/Name
:cognitect.aws.glue.GovernedCatalogTarget/Inputs
:cognitect.aws.glue.GovernedCatalogTarget/Table
:cognitect.aws.glue.GovernedCatalogTarget/Database]
:opt-un
[:cognitect.aws.glue.GovernedCatalogTarget/PartitionKeys
:cognitect.aws.glue.GovernedCatalogTarget/SchemaChangePolicy]))
(s/def
:cognitect.aws.glue/Option
(s/keys
:opt-un
[:cognitect.aws.glue.Option/Value
:cognitect.aws.glue.Option/Description
:cognitect.aws.glue.Option/Label]))
(s/def
:cognitect.aws.glue/DescriptionStringRemovable
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\r\\n\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/ListDataQualityRulesetsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.ListDataQualityRulesetsRequest/Filter
:cognitect.aws.glue.ListDataQualityRulesetsRequest/Tags
:cognitect.aws.glue.ListDataQualityRulesetsRequest/NextToken
:cognitect.aws.glue.ListDataQualityRulesetsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/CreateUserDefinedFunctionRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateUserDefinedFunctionRequest/DatabaseName
:cognitect.aws.glue.CreateUserDefinedFunctionRequest/FunctionInput]
:opt-un
[:cognitect.aws.glue.CreateUserDefinedFunctionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/Permission
(s/spec
string?
:gen
#(s/gen
#{"CREATE_TABLE"
"DELETE"
"DATA_LOCATION_ACCESS"
"CREATE_DATABASE"
"INSERT"
"SELECT"
"ALTER"
"ALL"
"DROP"})))
(s/def
:cognitect.aws.glue/GetSecurityConfigurationsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.GetSecurityConfigurationsRequest/NextToken
:cognitect.aws.glue.GetSecurityConfigurationsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/AmazonRedshiftNodeData
(s/keys
:opt-un
[:cognitect.aws.glue.AmazonRedshiftNodeData/MergeWhenMatched
:cognitect.aws.glue.AmazonRedshiftNodeData/Schema
:cognitect.aws.glue.AmazonRedshiftNodeData/StagingTable
:cognitect.aws.glue.AmazonRedshiftNodeData/CatalogRedshiftTable
:cognitect.aws.glue.AmazonRedshiftNodeData/Upsert
:cognitect.aws.glue.AmazonRedshiftNodeData/AccessType
:cognitect.aws.glue.AmazonRedshiftNodeData/IamRole
:cognitect.aws.glue.AmazonRedshiftNodeData/CatalogRedshiftSchema
:cognitect.aws.glue.AmazonRedshiftNodeData/SourceType
:cognitect.aws.glue.AmazonRedshiftNodeData/TablePrefix
:cognitect.aws.glue.AmazonRedshiftNodeData/CatalogTable
:cognitect.aws.glue.AmazonRedshiftNodeData/MergeWhenNotMatched
:cognitect.aws.glue.AmazonRedshiftNodeData/CatalogDatabase
:cognitect.aws.glue.AmazonRedshiftNodeData/TempDir
:cognitect.aws.glue.AmazonRedshiftNodeData/MergeAction
:cognitect.aws.glue.AmazonRedshiftNodeData/TableSchema
:cognitect.aws.glue.AmazonRedshiftNodeData/PreAction
:cognitect.aws.glue.AmazonRedshiftNodeData/AdvancedOptions
:cognitect.aws.glue.AmazonRedshiftNodeData/CrawlerConnection
:cognitect.aws.glue.AmazonRedshiftNodeData/Connection
:cognitect.aws.glue.AmazonRedshiftNodeData/SampleQuery
:cognitect.aws.glue.AmazonRedshiftNodeData/Table
:cognitect.aws.glue.AmazonRedshiftNodeData/MergeClause
:cognitect.aws.glue.AmazonRedshiftNodeData/Action
:cognitect.aws.glue.AmazonRedshiftNodeData/PostAction
:cognitect.aws.glue.AmazonRedshiftNodeData/SelectedColumns]))
(s/def :cognitect.aws.glue/NodeType (s/spec string? :gen #(s/gen #{"TRIGGER" "JOB" "CRAWLER"})))
(s/def
:cognitect.aws.glue/GetSchemaInput
(s/keys :req-un [:cognitect.aws.glue.GetSchemaInput/SchemaId]))
(s/def
:cognitect.aws.glue/BatchGetWorkflowsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.BatchGetWorkflowsResponse/Workflows
:cognitect.aws.glue.BatchGetWorkflowsResponse/MissingWorkflows]))
(s/def
:cognitect.aws.glue/CreateCustomEntityTypeResponse
(s/keys :opt-un [:cognitect.aws.glue.CreateCustomEntityTypeResponse/Name]))
(s/def
:cognitect.aws.glue/Edge
(s/keys :opt-un [:cognitect.aws.glue.Edge/DestinationId :cognitect.aws.glue.Edge/SourceId]))
(s/def
:cognitect.aws.glue/DeleteWorkflowRequest
(s/keys :req-un [:cognitect.aws.glue.DeleteWorkflowRequest/Name]))
(s/def
:cognitect.aws.glue/BatchStopJobRunErrorList
(s/coll-of :cognitect.aws.glue/BatchStopJobRunError))
(s/def
:cognitect.aws.glue/GetPartitionIndexesRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetPartitionIndexesRequest/DatabaseName
:cognitect.aws.glue.GetPartitionIndexesRequest/TableName]
:opt-un
[:cognitect.aws.glue.GetPartitionIndexesRequest/NextToken
:cognitect.aws.glue.GetPartitionIndexesRequest/CatalogId]))
(s/def
:cognitect.aws.glue/CreateScriptResponse
(s/keys
:opt-un
[:cognitect.aws.glue.CreateScriptResponse/PythonScript
:cognitect.aws.glue.CreateScriptResponse/ScalaCode]))
(s/def
:cognitect.aws.glue/SchemaValidationError
(s/spec
(s/and string? #(<= 1 (count %) 5000))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 5000) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/SchemaListItem
(s/keys
:opt-un
[:cognitect.aws.glue.SchemaListItem/SchemaStatus
:cognitect.aws.glue.SchemaListItem/CreatedTime
:cognitect.aws.glue.SchemaListItem/Description
:cognitect.aws.glue.SchemaListItem/UpdatedTime
:cognitect.aws.glue.SchemaListItem/RegistryName
:cognitect.aws.glue.SchemaListItem/SchemaName
:cognitect.aws.glue.SchemaListItem/SchemaArn]))
(s/def
:cognitect.aws.glue/ResourceShareType
(s/spec string? :gen #(s/gen #{"FEDERATED" "FOREIGN" "ALL"})))
(s/def :cognitect.aws.glue/UpdateDevEndpointResponse (s/keys))
(s/def :cognitect.aws.glue/Token string?)
(s/def
:cognitect.aws.glue/CreateDataQualityRulesetResponse
(s/keys :opt-un [:cognitect.aws.glue.CreateDataQualityRulesetResponse/Name]))
(s/def
:cognitect.aws.glue/ColumnImportance
(s/keys
:opt-un
[:cognitect.aws.glue.ColumnImportance/Importance
:cognitect.aws.glue.ColumnImportance/ColumnName]))
(s/def :cognitect.aws.glue/TableVersionErrors (s/coll-of :cognitect.aws.glue/TableVersionError))
(s/def
:cognitect.aws.glue/CreateWorkflowResponse
(s/keys :opt-un [:cognitect.aws.glue.CreateWorkflowResponse/Name]))
(s/def
:cognitect.aws.glue/GetDataQualityRuleRecommendationRunResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/Timeout
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/RunId
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/ExecutionTime
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/RecommendedRuleset
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/CreatedRulesetName
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/NumberOfWorkers
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/LastModifiedOn
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/Status
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/Role
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/DataSource
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/ErrorString
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/StartedOn
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/CompletedOn]))
(s/def
:cognitect.aws.glue/Schedule
(s/keys
:opt-un
[:cognitect.aws.glue.Schedule/ScheduleExpression :cognitect.aws.glue.Schedule/State]))
(s/def
:cognitect.aws.glue/Predicate
(s/keys :opt-un [:cognitect.aws.glue.Predicate/Logical :cognitect.aws.glue.Predicate/Conditions]))
(s/def
:cognitect.aws.glue/Prob
(s/spec
(s/and double? #(<= 0 % 1))
:gen
#(gen/double* {:infinite? false, :NaN? false, :min 0, :max 1})))
(s/def
:cognitect.aws.glue/PolicyJsonString
(s/spec
(s/and string? #(<= 2 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 2 100) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/AthenaConnectorSource
(s/keys
:req-un
[:cognitect.aws.glue.AthenaConnectorSource/Name
:cognitect.aws.glue.AthenaConnectorSource/ConnectionName
:cognitect.aws.glue.AthenaConnectorSource/ConnectorName
:cognitect.aws.glue.AthenaConnectorSource/ConnectionType
:cognitect.aws.glue.AthenaConnectorSource/SchemaName]
:opt-un
[:cognitect.aws.glue.AthenaConnectorSource/OutputSchemas
:cognitect.aws.glue.AthenaConnectorSource/ConnectionTable]))
(s/def
:cognitect.aws.glue/BatchGetPartitionValueList
(s/coll-of :cognitect.aws.glue/PartitionValueList :min-count 0 :max-count 1000))
(s/def
:cognitect.aws.glue/CrawlerTargets
(s/keys
:opt-un
[:cognitect.aws.glue.CrawlerTargets/S3Targets
:cognitect.aws.glue.CrawlerTargets/CatalogTargets
:cognitect.aws.glue.CrawlerTargets/MongoDBTargets
:cognitect.aws.glue.CrawlerTargets/DynamoDBTargets
:cognitect.aws.glue.CrawlerTargets/JdbcTargets
:cognitect.aws.glue.CrawlerTargets/DeltaTargets
:cognitect.aws.glue.CrawlerTargets/IcebergTargets]))
(s/def
:cognitect.aws.glue/MetadataKeyString
(s/spec #(re-matches (re-pattern "[a-zA-Z0-9+-=._./@]+") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/CustomEntityType
(s/keys
:req-un
[:cognitect.aws.glue.CustomEntityType/Name :cognitect.aws.glue.CustomEntityType/RegexString]
:opt-un
[:cognitect.aws.glue.CustomEntityType/ContextWords]))
(s/def
:cognitect.aws.glue/SqlAlias
(s/keys :req-un [:cognitect.aws.glue.SqlAlias/From :cognitect.aws.glue.SqlAlias/Alias]))
(s/def
:cognitect.aws.glue/AccountId
(s/spec
(s/and string? #(<= 0 (count %) 12))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 12) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/StartMLLabelingSetGenerationTaskRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.StartMLLabelingSetGenerationTaskRunRequest/TransformId
:cognitect.aws.glue.StartMLLabelingSetGenerationTaskRunRequest/OutputS3Path]))
(s/def :cognitect.aws.glue/TaskRunList (s/coll-of :cognitect.aws.glue/TaskRun))
(s/def
:cognitect.aws.glue/UserDefinedFunctionList
(s/coll-of :cognitect.aws.glue/UserDefinedFunction))
(s/def
:cognitect.aws.glue/DeleteTableRequest
(s/keys
:req-un
[:cognitect.aws.glue.DeleteTableRequest/DatabaseName
:cognitect.aws.glue.DeleteTableRequest/Name]
:opt-un
[:cognitect.aws.glue.DeleteTableRequest/TransactionId
:cognitect.aws.glue.DeleteTableRequest/CatalogId]))
(s/def
:cognitect.aws.glue/GetTableVersionsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetTableVersionsResponse/TableVersions
:cognitect.aws.glue.GetTableVersionsResponse/NextToken]))
(s/def
:cognitect.aws.glue/Workflows
(s/coll-of :cognitect.aws.glue/Workflow :min-count 1 :max-count 25))
(s/def
:cognitect.aws.glue/SecurityConfiguration
(s/keys
:opt-un
[:cognitect.aws.glue.SecurityConfiguration/EncryptionConfiguration
:cognitect.aws.glue.SecurityConfiguration/CreatedTimeStamp
:cognitect.aws.glue.SecurityConfiguration/Name]))
(s/def
:cognitect.aws.glue/OracleSQLCatalogSource
(s/keys
:req-un
[:cognitect.aws.glue.OracleSQLCatalogSource/Name
:cognitect.aws.glue.OracleSQLCatalogSource/Database
:cognitect.aws.glue.OracleSQLCatalogSource/Table]))
(s/def
:cognitect.aws.glue/FilterValueType
(s/spec string? :gen #(s/gen #{"COLUMNEXTRACTED" "CONSTANT"})))
(s/def
:cognitect.aws.glue/JobCommand
(s/keys
:opt-un
[:cognitect.aws.glue.JobCommand/ScriptLocation
:cognitect.aws.glue.JobCommand/Runtime
:cognitect.aws.glue.JobCommand/PythonVersion
:cognitect.aws.glue.JobCommand/Name]))
(s/def :cognitect.aws.glue/CatalogEntries (s/coll-of :cognitect.aws.glue/CatalogEntry))
(s/def
:cognitect.aws.glue/DataQualityRulesetFilterCriteria
(s/keys
:opt-un
[:cognitect.aws.glue.DataQualityRulesetFilterCriteria/TargetTable
:cognitect.aws.glue.DataQualityRulesetFilterCriteria/CreatedAfter
:cognitect.aws.glue.DataQualityRulesetFilterCriteria/Description
:cognitect.aws.glue.DataQualityRulesetFilterCriteria/CreatedBefore
:cognitect.aws.glue.DataQualityRulesetFilterCriteria/LastModifiedAfter
:cognitect.aws.glue.DataQualityRulesetFilterCriteria/LastModifiedBefore
:cognitect.aws.glue.DataQualityRulesetFilterCriteria/Name]))
(s/def :cognitect.aws.glue/Blueprints (s/coll-of :cognitect.aws.glue/Blueprint))
(s/def :cognitect.aws.glue/DeleteColumnStatisticsForPartitionResponse (s/keys))
(s/def
:cognitect.aws.glue/StatementOutputData
(s/keys :opt-un [:cognitect.aws.glue.StatementOutputData/TextPlain]))
(s/def :cognitect.aws.glue/CronExpression string?)
(s/def
:cognitect.aws.glue/CsvColumnDelimiter
(s/spec #(re-matches (re-pattern "[^\\r\\n]") %) :gen #(gen/string)))
(s/def :cognitect.aws.glue/GlueSchema (s/keys :opt-un [:cognitect.aws.glue.GlueSchema/Columns]))
(s/def
:cognitect.aws.glue/GetUnfilteredPartitionMetadataResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetUnfilteredPartitionMetadataResponse/Partition
:cognitect.aws.glue.GetUnfilteredPartitionMetadataResponse/IsRegisteredWithLakeFormation
:cognitect.aws.glue.GetUnfilteredPartitionMetadataResponse/AuthorizedColumns]))
(s/def
:cognitect.aws.glue/S3CatalogSource
(s/keys
:req-un
[:cognitect.aws.glue.S3CatalogSource/Name
:cognitect.aws.glue.S3CatalogSource/Database
:cognitect.aws.glue.S3CatalogSource/Table]
:opt-un
[:cognitect.aws.glue.S3CatalogSource/AdditionalOptions
:cognitect.aws.glue.S3CatalogSource/PartitionPredicate]))
(s/def
:cognitect.aws.glue/CatalogGetterPageSize
(s/spec (s/and int? #(<= 1 % 100)) :gen #(gen/choose 1 100)))
(s/def
:cognitect.aws.glue/CancelDataQualityRulesetEvaluationRunRequest
(s/keys :req-un [:cognitect.aws.glue.CancelDataQualityRulesetEvaluationRunRequest/RunId]))
(s/def
:cognitect.aws.glue/ListSchemaVersionsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListSchemaVersionsResponse/Schemas
:cognitect.aws.glue.ListSchemaVersionsResponse/NextToken]))
(s/def
:cognitect.aws.glue/GlueTables
(s/coll-of :cognitect.aws.glue/GlueTable :min-count 0 :max-count 10))
(s/def
:cognitect.aws.glue/GetUnfilteredTableMetadataResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetUnfilteredTableMetadataResponse/CellFilters
:cognitect.aws.glue.GetUnfilteredTableMetadataResponse/IsRegisteredWithLakeFormation
:cognitect.aws.glue.GetUnfilteredTableMetadataResponse/AuthorizedColumns
:cognitect.aws.glue.GetUnfilteredTableMetadataResponse/Table]))
(s/def
:cognitect.aws.glue/DataCatalogEncryptionSettings
(s/keys
:opt-un
[:cognitect.aws.glue.DataCatalogEncryptionSettings/EncryptionAtRest
:cognitect.aws.glue.DataCatalogEncryptionSettings/ConnectionPasswordEncryption]))
(s/def
:cognitect.aws.glue/PostgreSQLCatalogSource
(s/keys
:req-un
[:cognitect.aws.glue.PostgreSQLCatalogSource/Name
:cognitect.aws.glue.PostgreSQLCatalogSource/Database
:cognitect.aws.glue.PostgreSQLCatalogSource/Table]))
(s/def :cognitect.aws.glue/Mappings (s/coll-of :cognitect.aws.glue/Mapping))
(s/def
:cognitect.aws.glue/CrawlsFilter
(s/keys
:opt-un
[:cognitect.aws.glue.CrawlsFilter/FilterOperator
:cognitect.aws.glue.CrawlsFilter/FieldName
:cognitect.aws.glue.CrawlsFilter/FieldValue]))
(s/def :cognitect.aws.glue/PredecessorList (s/coll-of :cognitect.aws.glue/Predecessor))
(s/def
:cognitect.aws.glue/UpdateMLTransformRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateMLTransformRequest/TransformId]
:opt-un
[:cognitect.aws.glue.UpdateMLTransformRequest/Timeout
:cognitect.aws.glue.UpdateMLTransformRequest/MaxCapacity
:cognitect.aws.glue.UpdateMLTransformRequest/Parameters
:cognitect.aws.glue.UpdateMLTransformRequest/NumberOfWorkers
:cognitect.aws.glue.UpdateMLTransformRequest/WorkerType
:cognitect.aws.glue.UpdateMLTransformRequest/Role
:cognitect.aws.glue.UpdateMLTransformRequest/GlueVersion
:cognitect.aws.glue.UpdateMLTransformRequest/Description
:cognitect.aws.glue.UpdateMLTransformRequest/MaxRetries
:cognitect.aws.glue.UpdateMLTransformRequest/Name]))
(s/def
:cognitect.aws.glue/GetTableVersionResponse
(s/keys :opt-un [:cognitect.aws.glue.GetTableVersionResponse/TableVersion]))
(s/def
:cognitect.aws.glue/Predecessor
(s/keys :opt-un [:cognitect.aws.glue.Predecessor/RunId :cognitect.aws.glue.Predecessor/JobName]))
(s/def
:cognitect.aws.glue/GlueResourceArn
(s/spec #(re-matches (re-pattern "arn:(aws|aws-us-gov|aws-cn):glue:.*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/ListBlueprintsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.ListBlueprintsRequest/Tags
:cognitect.aws.glue.ListBlueprintsRequest/NextToken
:cognitect.aws.glue.ListBlueprintsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/ConnectionProperties
(s/map-of
:cognitect.aws.glue/ConnectionPropertyKey
:cognitect.aws.glue/ValueString
:min-count
0
:max-count
100))
(s/def :cognitect.aws.glue/CsvHeader (s/coll-of :cognitect.aws.glue/NameString))
(s/def :cognitect.aws.glue/FilterExpressions (s/coll-of :cognitect.aws.glue/FilterExpression))
(s/def
:cognitect.aws.glue/DropFields
(s/keys
:req-un
[:cognitect.aws.glue.DropFields/Name
:cognitect.aws.glue.DropFields/Inputs
:cognitect.aws.glue.DropFields/Paths]))
(s/def
:cognitect.aws.glue/GetColumnNamesList
(s/coll-of :cognitect.aws.glue/NameString :min-count 0 :max-count 100))
(s/def
:cognitect.aws.glue/StartCrawlerScheduleRequest
(s/keys :req-un [:cognitect.aws.glue.StartCrawlerScheduleRequest/CrawlerName]))
(s/def
:cognitect.aws.glue/LastCrawlInfo
(s/keys
:opt-un
[:cognitect.aws.glue.LastCrawlInfo/MessagePrefix
:cognitect.aws.glue.LastCrawlInfo/StartTime
:cognitect.aws.glue.LastCrawlInfo/Status
:cognitect.aws.glue.LastCrawlInfo/ErrorMessage
:cognitect.aws.glue.LastCrawlInfo/LogGroup
:cognitect.aws.glue.LastCrawlInfo/LogStream]))
(s/def
:cognitect.aws.glue/Datatype
(s/keys :req-un [:cognitect.aws.glue.Datatype/Id :cognitect.aws.glue.Datatype/Label]))
(s/def :cognitect.aws.glue/CrawlerConfiguration string?)
(s/def
:cognitect.aws.glue/NonNegativeInt
(s/spec (s/and int? #(<= 0 %)) :gen #(gen/choose 0 Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/EvaluatedMetricsMap
(s/map-of :cognitect.aws.glue/NameString :cognitect.aws.glue/NullableDouble))
(s/def
:cognitect.aws.glue/GetTablesRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetTablesRequest/DatabaseName]
:opt-un
[:cognitect.aws.glue.GetTablesRequest/TransactionId
:cognitect.aws.glue.GetTablesRequest/Expression
:cognitect.aws.glue.GetTablesRequest/NextToken
:cognitect.aws.glue.GetTablesRequest/QueryAsOfTime
:cognitect.aws.glue.GetTablesRequest/CatalogId
:cognitect.aws.glue.GetTablesRequest/MaxResults]))
(s/def :cognitect.aws.glue/SchemaRegistryTokenString string?)
(s/def
:cognitect.aws.glue/GetDatabasesResponse
(s/keys
:req-un
[:cognitect.aws.glue.GetDatabasesResponse/DatabaseList]
:opt-un
[:cognitect.aws.glue.GetDatabasesResponse/NextToken]))
(s/def
:cognitect.aws.glue/BatchUpdatePartitionRequestEntryList
(s/coll-of :cognitect.aws.glue/BatchUpdatePartitionRequestEntry :min-count 1 :max-count 100))
(s/def
:cognitect.aws.glue/GetSchemaResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetSchemaResponse/RegistryArn
:cognitect.aws.glue.GetSchemaResponse/SchemaStatus
:cognitect.aws.glue.GetSchemaResponse/DataFormat
:cognitect.aws.glue.GetSchemaResponse/NextSchemaVersion
:cognitect.aws.glue.GetSchemaResponse/Compatibility
:cognitect.aws.glue.GetSchemaResponse/CreatedTime
:cognitect.aws.glue.GetSchemaResponse/Description
:cognitect.aws.glue.GetSchemaResponse/UpdatedTime
:cognitect.aws.glue.GetSchemaResponse/RegistryName
:cognitect.aws.glue.GetSchemaResponse/LatestSchemaVersion
:cognitect.aws.glue.GetSchemaResponse/SchemaName
:cognitect.aws.glue.GetSchemaResponse/SchemaArn
:cognitect.aws.glue.GetSchemaResponse/SchemaCheckpoint]))
(s/def
:cognitect.aws.glue/TransformParameters
(s/keys
:req-un
[:cognitect.aws.glue.TransformParameters/TransformType]
:opt-un
[:cognitect.aws.glue.TransformParameters/FindMatchesParameters]))
(s/def
:cognitect.aws.glue/UnfilteredPartition
(s/keys
:opt-un
[:cognitect.aws.glue.UnfilteredPartition/Partition
:cognitect.aws.glue.UnfilteredPartition/IsRegisteredWithLakeFormation
:cognitect.aws.glue.UnfilteredPartition/AuthorizedColumns]))
(s/def
:cognitect.aws.glue/CatalogHudiSource
(s/keys
:req-un
[:cognitect.aws.glue.CatalogHudiSource/Name
:cognitect.aws.glue.CatalogHudiSource/Database
:cognitect.aws.glue.CatalogHudiSource/Table]
:opt-un
[:cognitect.aws.glue.CatalogHudiSource/OutputSchemas
:cognitect.aws.glue.CatalogHudiSource/AdditionalHudiOptions]))
(s/def
:cognitect.aws.glue/UpdateXMLClassifierRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateXMLClassifierRequest/Name]
:opt-un
[:cognitect.aws.glue.UpdateXMLClassifierRequest/RowTag
:cognitect.aws.glue.UpdateXMLClassifierRequest/Classification]))
(s/def
:cognitect.aws.glue/DoubleColumnStatisticsData
(s/keys
:req-un
[:cognitect.aws.glue.DoubleColumnStatisticsData/NumberOfNulls
:cognitect.aws.glue.DoubleColumnStatisticsData/NumberOfDistinctValues]
:opt-un
[:cognitect.aws.glue.DoubleColumnStatisticsData/MaximumValue
:cognitect.aws.glue.DoubleColumnStatisticsData/MinimumValue]))
(s/def
:cognitect.aws.glue/UpdateBehavior
(s/spec string? :gen #(s/gen #{"UPDATE_IN_DATABASE" "LOG"})))
(s/def :cognitect.aws.glue/ScalaCode string?)
(s/def
:cognitect.aws.glue/GetMappingResponse
(s/keys :req-un [:cognitect.aws.glue.GetMappingResponse/Mapping]))
(s/def
:cognitect.aws.glue/GetUnfilteredTableMetadataRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetUnfilteredTableMetadataRequest/CatalogId
:cognitect.aws.glue.GetUnfilteredTableMetadataRequest/DatabaseName
:cognitect.aws.glue.GetUnfilteredTableMetadataRequest/Name
:cognitect.aws.glue.GetUnfilteredTableMetadataRequest/SupportedPermissionTypes]
:opt-un
[:cognitect.aws.glue.GetUnfilteredTableMetadataRequest/AuditContext]))
(s/def
:cognitect.aws.glue/ResumeWorkflowRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.ResumeWorkflowRunRequest/Name
:cognitect.aws.glue.ResumeWorkflowRunRequest/RunId
:cognitect.aws.glue.ResumeWorkflowRunRequest/NodeIds]))
(s/def
:cognitect.aws.glue/GlueVersionString
(s/spec #(re-matches (re-pattern "^\\w+\\.\\w+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/GlueTable
(s/keys
:req-un
[:cognitect.aws.glue.GlueTable/DatabaseName :cognitect.aws.glue.GlueTable/TableName]
:opt-un
[:cognitect.aws.glue.GlueTable/AdditionalOptions
:cognitect.aws.glue.GlueTable/ConnectionName
:cognitect.aws.glue.GlueTable/CatalogId]))
(s/def
:cognitect.aws.glue/GlueStudioSchemaColumn
(s/keys
:req-un
[:cognitect.aws.glue.GlueStudioSchemaColumn/Name]
:opt-un
[:cognitect.aws.glue.GlueStudioSchemaColumn/Type]))
(s/def
:cognitect.aws.glue/GetDataflowGraphRequest
(s/keys :opt-un [:cognitect.aws.glue.GetDataflowGraphRequest/PythonScript]))
(s/def
:cognitect.aws.glue/ColumnStatisticsErrors
(s/coll-of :cognitect.aws.glue/ColumnStatisticsError))
(s/def :cognitect.aws.glue/TableErrors (s/coll-of :cognitect.aws.glue/TableError))
(s/def
:cognitect.aws.glue/CatalogSchemaChangePolicy
(s/keys
:opt-un
[:cognitect.aws.glue.CatalogSchemaChangePolicy/EnableUpdateCatalog
:cognitect.aws.glue.CatalogSchemaChangePolicy/UpdateBehavior]))
(s/def
:cognitect.aws.glue/ResetJobBookmarkResponse
(s/keys :opt-un [:cognitect.aws.glue.ResetJobBookmarkResponse/JobBookmarkEntry]))
(s/def
:cognitect.aws.glue/DataQualityRuleResult
(s/keys
:opt-un
[:cognitect.aws.glue.DataQualityRuleResult/Result
:cognitect.aws.glue.DataQualityRuleResult/Description
:cognitect.aws.glue.DataQualityRuleResult/Name
:cognitect.aws.glue.DataQualityRuleResult/EvaluationMessage
:cognitect.aws.glue.DataQualityRuleResult/EvaluatedMetrics]))
(s/def :cognitect.aws.glue/SessionIdList (s/coll-of :cognitect.aws.glue/NameString))
(s/def
:cognitect.aws.glue/SqlQuery
(s/spec
#(re-matches
(re-pattern "([\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\s])*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/DataQualityRuleResultStatus
(s/spec string? :gen #(s/gen #{"PASS" "FAIL" "ERROR"})))
(s/def
:cognitect.aws.glue/CatalogKinesisSource
(s/keys
:req-un
[:cognitect.aws.glue.CatalogKinesisSource/Name
:cognitect.aws.glue.CatalogKinesisSource/Table
:cognitect.aws.glue.CatalogKinesisSource/Database]
:opt-un
[:cognitect.aws.glue.CatalogKinesisSource/StreamingOptions
:cognitect.aws.glue.CatalogKinesisSource/DetectSchema
:cognitect.aws.glue.CatalogKinesisSource/WindowSize
:cognitect.aws.glue.CatalogKinesisSource/DataPreviewOptions]))
(s/def
:cognitect.aws.glue/MetadataInfo
(s/keys
:opt-un
[:cognitect.aws.glue.MetadataInfo/MetadataValue
:cognitect.aws.glue.MetadataInfo/CreatedTime
:cognitect.aws.glue.MetadataInfo/OtherMetadataValueList]))
(s/def
:cognitect.aws.glue/S3Encryption
(s/keys
:opt-un
[:cognitect.aws.glue.S3Encryption/KmsKeyArn :cognitect.aws.glue.S3Encryption/S3EncryptionMode]))
(s/def
:cognitect.aws.glue/S3HudiDirectTarget
(s/keys
:req-un
[:cognitect.aws.glue.S3HudiDirectTarget/Name
:cognitect.aws.glue.S3HudiDirectTarget/Inputs
:cognitect.aws.glue.S3HudiDirectTarget/Path
:cognitect.aws.glue.S3HudiDirectTarget/Compression
:cognitect.aws.glue.S3HudiDirectTarget/Format
:cognitect.aws.glue.S3HudiDirectTarget/AdditionalOptions]
:opt-un
[:cognitect.aws.glue.S3HudiDirectTarget/PartitionKeys
:cognitect.aws.glue.S3HudiDirectTarget/SchemaChangePolicy]))
(s/def :cognitect.aws.glue/Sort (s/spec string? :gen #(s/gen #{"DESC" "ASC"})))
(s/def :cognitect.aws.glue/EventQueueArn string?)
(s/def
:cognitect.aws.glue/GetTagsResponse
(s/keys :opt-un [:cognitect.aws.glue.GetTagsResponse/Tags]))
(s/def :cognitect.aws.glue/SessionList (s/coll-of :cognitect.aws.glue/Session))
(s/def :cognitect.aws.glue/NodeList (s/coll-of :cognitect.aws.glue/Node))
(s/def
:cognitect.aws.glue/GetDevEndpointRequest
(s/keys :req-un [:cognitect.aws.glue.GetDevEndpointRequest/EndpointName]))
(s/def
:cognitect.aws.glue/DQDLString
(s/spec #(re-matches (re-pattern "([\\u0020-\\u007E\\r\\s\\n])*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/OrchestrationStatementCodeString
(s/spec
(s/and string? #(>= 68000 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 68000) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/ListCrawlersRequest
(s/keys
:opt-un
[:cognitect.aws.glue.ListCrawlersRequest/Tags
:cognitect.aws.glue.ListCrawlersRequest/NextToken
:cognitect.aws.glue.ListCrawlersRequest/MaxResults]))
(s/def
:cognitect.aws.glue/NonNegativeInteger
(s/spec (s/and int? #(<= 0 %)) :gen #(gen/choose 0 Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/CreateMLTransformRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateMLTransformRequest/Name
:cognitect.aws.glue.CreateMLTransformRequest/InputRecordTables
:cognitect.aws.glue.CreateMLTransformRequest/Parameters
:cognitect.aws.glue.CreateMLTransformRequest/Role]
:opt-un
[:cognitect.aws.glue.CreateMLTransformRequest/Timeout
:cognitect.aws.glue.CreateMLTransformRequest/MaxCapacity
:cognitect.aws.glue.CreateMLTransformRequest/Tags
:cognitect.aws.glue.CreateMLTransformRequest/NumberOfWorkers
:cognitect.aws.glue.CreateMLTransformRequest/WorkerType
:cognitect.aws.glue.CreateMLTransformRequest/GlueVersion
:cognitect.aws.glue.CreateMLTransformRequest/Description
:cognitect.aws.glue.CreateMLTransformRequest/TransformEncryption
:cognitect.aws.glue.CreateMLTransformRequest/MaxRetries]))
(s/def
:cognitect.aws.glue/PutDataCatalogEncryptionSettingsRequest
(s/keys
:req-un
[:cognitect.aws.glue.PutDataCatalogEncryptionSettingsRequest/DataCatalogEncryptionSettings]
:opt-un
[:cognitect.aws.glue.PutDataCatalogEncryptionSettingsRequest/CatalogId]))
(s/def :cognitect.aws.glue/ActionList (s/coll-of :cognitect.aws.glue/Action))
(s/def
:cognitect.aws.glue/FindMatchesTaskRunProperties
(s/keys
:opt-un
[:cognitect.aws.glue.FindMatchesTaskRunProperties/JobName
:cognitect.aws.glue.FindMatchesTaskRunProperties/JobRunId
:cognitect.aws.glue.FindMatchesTaskRunProperties/JobId]))
(s/def
:cognitect.aws.glue/ContextWords
(s/coll-of :cognitect.aws.glue/NameString :min-count 1 :max-count 20))
(s/def :cognitect.aws.glue/ColumnValueStringList (s/coll-of :cognitect.aws.glue/ColumnValuesString))
(s/def
:cognitect.aws.glue/ListJobsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.ListJobsRequest/Tags
:cognitect.aws.glue.ListJobsRequest/NextToken
:cognitect.aws.glue.ListJobsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/DeleteBlueprintRequest
(s/keys :req-un [:cognitect.aws.glue.DeleteBlueprintRequest/Name]))
(s/def
:cognitect.aws.glue/S3GlueParquetTarget
(s/keys
:req-un
[:cognitect.aws.glue.S3GlueParquetTarget/Name
:cognitect.aws.glue.S3GlueParquetTarget/Inputs
:cognitect.aws.glue.S3GlueParquetTarget/Path]
:opt-un
[:cognitect.aws.glue.S3GlueParquetTarget/PartitionKeys
:cognitect.aws.glue.S3GlueParquetTarget/SchemaChangePolicy
:cognitect.aws.glue.S3GlueParquetTarget/Compression]))
(s/def
:cognitect.aws.glue/AmazonRedshiftAdvancedOption
(s/keys
:opt-un
[:cognitect.aws.glue.AmazonRedshiftAdvancedOption/Key
:cognitect.aws.glue.AmazonRedshiftAdvancedOption/Value]))
(s/def
:cognitect.aws.glue/SparkConnectorSource
(s/keys
:req-un
[:cognitect.aws.glue.SparkConnectorSource/Name
:cognitect.aws.glue.SparkConnectorSource/ConnectionName
:cognitect.aws.glue.SparkConnectorSource/ConnectorName
:cognitect.aws.glue.SparkConnectorSource/ConnectionType]
:opt-un
[:cognitect.aws.glue.SparkConnectorSource/OutputSchemas
:cognitect.aws.glue.SparkConnectorSource/AdditionalOptions]))
(s/def
:cognitect.aws.glue/DeleteWorkflowResponse
(s/keys :opt-un [:cognitect.aws.glue.DeleteWorkflowResponse/Name]))
(s/def :cognitect.aws.glue/ColumnErrors (s/coll-of :cognitect.aws.glue/ColumnError))
(s/def
:cognitect.aws.glue/UpdateGrokClassifierRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateGrokClassifierRequest/Name]
:opt-un
[:cognitect.aws.glue.UpdateGrokClassifierRequest/CustomPatterns
:cognitect.aws.glue.UpdateGrokClassifierRequest/Classification
:cognitect.aws.glue.UpdateGrokClassifierRequest/GrokPattern]))
(s/def :cognitect.aws.glue/TransformType (s/spec string? :gen #(s/gen #{"FIND_MATCHES"})))
(s/def
:cognitect.aws.glue/NotificationProperty
(s/keys :opt-un [:cognitect.aws.glue.NotificationProperty/NotifyDelayAfter]))
(s/def
:cognitect.aws.glue/ListMLTransformsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.ListMLTransformsRequest/Sort
:cognitect.aws.glue.ListMLTransformsRequest/Filter
:cognitect.aws.glue.ListMLTransformsRequest/Tags
:cognitect.aws.glue.ListMLTransformsRequest/NextToken
:cognitect.aws.glue.ListMLTransformsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/DeleteConnectionRequest
(s/keys
:req-un
[:cognitect.aws.glue.DeleteConnectionRequest/ConnectionName]
:opt-un
[:cognitect.aws.glue.DeleteConnectionRequest/CatalogId]))
(s/def :cognitect.aws.glue/BlueprintNames (s/coll-of :cognitect.aws.glue/OrchestrationNameString))
(s/def
:cognitect.aws.glue/CancelStatementRequest
(s/keys
:req-un
[:cognitect.aws.glue.CancelStatementRequest/SessionId
:cognitect.aws.glue.CancelStatementRequest/Id]
:opt-un
[:cognitect.aws.glue.CancelStatementRequest/RequestOrigin]))
(s/def
:cognitect.aws.glue/GetPlanRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetPlanRequest/Mapping :cognitect.aws.glue.GetPlanRequest/Source]
:opt-un
[:cognitect.aws.glue.GetPlanRequest/Sinks
:cognitect.aws.glue.GetPlanRequest/AdditionalPlanOptionsMap
:cognitect.aws.glue.GetPlanRequest/Location
:cognitect.aws.glue.GetPlanRequest/Language]))
(s/def
:cognitect.aws.glue/NullableDouble
(s/spec double? :gen #(gen/double* {:infinite? false, :NaN? false})))
(s/def
:cognitect.aws.glue/DirectJDBCSource
(s/keys
:req-un
[:cognitect.aws.glue.DirectJDBCSource/Name
:cognitect.aws.glue.DirectJDBCSource/Database
:cognitect.aws.glue.DirectJDBCSource/Table
:cognitect.aws.glue.DirectJDBCSource/ConnectionName
:cognitect.aws.glue.DirectJDBCSource/ConnectionType]
:opt-un
[:cognitect.aws.glue.DirectJDBCSource/RedshiftTmpDir]))
(s/def :cognitect.aws.glue/CodeGenArgValue string?)
(s/def
:cognitect.aws.glue/TriggerUpdate
(s/keys
:opt-un
[:cognitect.aws.glue.TriggerUpdate/Actions
:cognitect.aws.glue.TriggerUpdate/EventBatchingCondition
:cognitect.aws.glue.TriggerUpdate/Description
:cognitect.aws.glue.TriggerUpdate/Schedule
:cognitect.aws.glue.TriggerUpdate/Name
:cognitect.aws.glue.TriggerUpdate/Predicate]))
(s/def
:cognitect.aws.glue/ErrorDetails
(s/keys
:opt-un
[:cognitect.aws.glue.ErrorDetails/ErrorMessage :cognitect.aws.glue.ErrorDetails/ErrorCode]))
(s/def
:cognitect.aws.glue/LocationMap
(s/map-of :cognitect.aws.glue/ColumnValuesString :cognitect.aws.glue/ColumnValuesString))
(s/def
:cognitect.aws.glue/XMLClassifier
(s/keys
:req-un
[:cognitect.aws.glue.XMLClassifier/Name :cognitect.aws.glue.XMLClassifier/Classification]
:opt-un
[:cognitect.aws.glue.XMLClassifier/RowTag
:cognitect.aws.glue.XMLClassifier/CreationTime
:cognitect.aws.glue.XMLClassifier/Version
:cognitect.aws.glue.XMLClassifier/LastUpdated]))
(s/def
:cognitect.aws.glue/AmazonRedshiftAdvancedOptions
(s/coll-of :cognitect.aws.glue/AmazonRedshiftAdvancedOption))
(s/def
:cognitect.aws.glue/FindMatchesParameters
(s/keys
:opt-un
[:cognitect.aws.glue.FindMatchesParameters/PrecisionRecallTradeoff
:cognitect.aws.glue.FindMatchesParameters/PrimaryKeyColumnName
:cognitect.aws.glue.FindMatchesParameters/AccuracyCostTradeoff
:cognitect.aws.glue.FindMatchesParameters/EnforceProvidedLabels]))
(s/def
:cognitect.aws.glue/SchemaRegistryNameString
(s/spec #(re-matches (re-pattern "[a-zA-Z0-9-_$#.]+") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/PartitionIndexStatus
(s/spec string? :gen #(s/gen #{"DELETING" "CREATING" "ACTIVE" "FAILED"})))
(s/def
:cognitect.aws.glue/PutResourcePolicyRequest
(s/keys
:req-un
[:cognitect.aws.glue.PutResourcePolicyRequest/PolicyInJson]
:opt-un
[:cognitect.aws.glue.PutResourcePolicyRequest/PolicyExistsCondition
:cognitect.aws.glue.PutResourcePolicyRequest/PolicyHashCondition
:cognitect.aws.glue.PutResourcePolicyRequest/EnableHybrid
:cognitect.aws.glue.PutResourcePolicyRequest/ResourceArn]))
(s/def :cognitect.aws.glue/S3TargetList (s/coll-of :cognitect.aws.glue/S3Target))
(s/def :cognitect.aws.glue/OrderList (s/coll-of :cognitect.aws.glue/Order))
(s/def
:cognitect.aws.glue/WorkflowRuns
(s/coll-of :cognitect.aws.glue/WorkflowRun :min-count 1 :max-count 1000))
(s/def
:cognitect.aws.glue/RedshiftTarget
(s/keys
:req-un
[:cognitect.aws.glue.RedshiftTarget/Name
:cognitect.aws.glue.RedshiftTarget/Inputs
:cognitect.aws.glue.RedshiftTarget/Database
:cognitect.aws.glue.RedshiftTarget/Table]
:opt-un
[:cognitect.aws.glue.RedshiftTarget/TmpDirIAMRole
:cognitect.aws.glue.RedshiftTarget/RedshiftTmpDir
:cognitect.aws.glue.RedshiftTarget/UpsertRedshiftOptions]))
(s/def
:cognitect.aws.glue/TableInput
(s/keys
:req-un
[:cognitect.aws.glue.TableInput/Name]
:opt-un
[:cognitect.aws.glue.TableInput/ViewExpandedText
:cognitect.aws.glue.TableInput/TargetTable
:cognitect.aws.glue.TableInput/Parameters
:cognitect.aws.glue.TableInput/StorageDescriptor
:cognitect.aws.glue.TableInput/PartitionKeys
:cognitect.aws.glue.TableInput/Description
:cognitect.aws.glue.TableInput/Owner
:cognitect.aws.glue.TableInput/TableType
:cognitect.aws.glue.TableInput/LastAccessTime
:cognitect.aws.glue.TableInput/Retention
:cognitect.aws.glue.TableInput/LastAnalyzedTime
:cognitect.aws.glue.TableInput/ViewOriginalText]))
(s/def
:cognitect.aws.glue/LabelCount
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/ListRegistriesInput
(s/keys
:opt-un
[:cognitect.aws.glue.ListRegistriesInput/NextToken
:cognitect.aws.glue.ListRegistriesInput/MaxResults]))
(s/def
:cognitect.aws.glue/JDBCConnectionType
(s/spec string? :gen #(s/gen #{"mysql" "oracle" "postgresql" "redshift" "sqlserver"})))
(s/def
:cognitect.aws.glue/UpdateWorkflowResponse
(s/keys :opt-un [:cognitect.aws.glue.UpdateWorkflowResponse/Name]))
(s/def
:cognitect.aws.glue/UpdateRegistryResponse
(s/keys
:opt-un
[:cognitect.aws.glue.UpdateRegistryResponse/RegistryArn
:cognitect.aws.glue.UpdateRegistryResponse/RegistryName]))
(s/def
:cognitect.aws.glue/GetUnfilteredPartitionsMetadataResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetUnfilteredPartitionsMetadataResponse/NextToken
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataResponse/UnfilteredPartitions]))
(s/def
:cognitect.aws.glue/DataQualityRulesetEvaluationRunFilter
(s/keys
:req-un
[:cognitect.aws.glue.DataQualityRulesetEvaluationRunFilter/DataSource]
:opt-un
[:cognitect.aws.glue.DataQualityRulesetEvaluationRunFilter/StartedBefore
:cognitect.aws.glue.DataQualityRulesetEvaluationRunFilter/StartedAfter]))
(s/def
:cognitect.aws.glue/BatchGetBlueprintsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.BatchGetBlueprintsResponse/Blueprints
:cognitect.aws.glue.BatchGetBlueprintsResponse/MissingBlueprints]))
(s/def :cognitect.aws.glue/BooleanValue boolean?)
(s/def
:cognitect.aws.glue/GetPlanResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetPlanResponse/PythonScript
:cognitect.aws.glue.GetPlanResponse/ScalaCode]))
(s/def
:cognitect.aws.glue/BackfillErroredPartitionsList
(s/coll-of :cognitect.aws.glue/PartitionValueList))
(s/def
:cognitect.aws.glue/ResourceUriList
(s/coll-of :cognitect.aws.glue/ResourceUri :min-count 0 :max-count 1000))
(s/def
:cognitect.aws.glue/StartExportLabelsTaskRunResponse
(s/keys :opt-un [:cognitect.aws.glue.StartExportLabelsTaskRunResponse/TaskRunId]))
(s/def :cognitect.aws.glue/UpdateCrawlerResponse (s/keys))
(s/def
:cognitect.aws.glue/SchemaStatus
(s/spec string? :gen #(s/gen #{"AVAILABLE" "PENDING" "DELETING"})))
(s/def
:cognitect.aws.glue/SchemaVersionNumber
(s/keys
:opt-un
[:cognitect.aws.glue.SchemaVersionNumber/LatestVersion
:cognitect.aws.glue.SchemaVersionNumber/VersionNumber]))
(s/def
:cognitect.aws.glue/GetDataQualityResultResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetDataQualityResultResponse/RulesetName
:cognitect.aws.glue.GetDataQualityResultResponse/EvaluationContext
:cognitect.aws.glue.GetDataQualityResultResponse/RuleResults
:cognitect.aws.glue.GetDataQualityResultResponse/JobName
:cognitect.aws.glue.GetDataQualityResultResponse/RulesetEvaluationRunId
:cognitect.aws.glue.GetDataQualityResultResponse/Score
:cognitect.aws.glue.GetDataQualityResultResponse/JobRunId
:cognitect.aws.glue.GetDataQualityResultResponse/DataSource
:cognitect.aws.glue.GetDataQualityResultResponse/StartedOn
:cognitect.aws.glue.GetDataQualityResultResponse/CompletedOn
:cognitect.aws.glue.GetDataQualityResultResponse/ResultId]))
(s/def
:cognitect.aws.glue/SearchPropertyPredicates
(s/coll-of :cognitect.aws.glue/PropertyPredicate))
(s/def
:cognitect.aws.glue/WorkflowNames
(s/coll-of :cognitect.aws.glue/NameString :min-count 1 :max-count 25))
(s/def
:cognitect.aws.glue/KmsKeyArn
(s/spec #(re-matches (re-pattern "arn:aws:kms:.*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/IdString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/DeleteDataQualityRulesetRequest
(s/keys :req-un [:cognitect.aws.glue.DeleteDataQualityRulesetRequest/Name]))
(s/def
:cognitect.aws.glue/IcebergTarget
(s/keys
:opt-un
[:cognitect.aws.glue.IcebergTarget/ConnectionName
:cognitect.aws.glue.IcebergTarget/MaximumTraversalDepth
:cognitect.aws.glue.IcebergTarget/Paths
:cognitect.aws.glue.IcebergTarget/Exclusions]))
(s/def
:cognitect.aws.glue/GetMLTransformsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.GetMLTransformsRequest/Sort
:cognitect.aws.glue.GetMLTransformsRequest/Filter
:cognitect.aws.glue.GetMLTransformsRequest/NextToken
:cognitect.aws.glue.GetMLTransformsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/NameString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def :cognitect.aws.glue/DatabaseList (s/coll-of :cognitect.aws.glue/Database))
(s/def
:cognitect.aws.glue/GetBlueprintRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetBlueprintRunRequest/BlueprintName
:cognitect.aws.glue.GetBlueprintRunRequest/RunId]))
(s/def
:cognitect.aws.glue/GlueStudioSchemaColumnList
(s/coll-of :cognitect.aws.glue/GlueStudioSchemaColumn))
(s/def
:cognitect.aws.glue/RunStatementRequest
(s/keys
:req-un
[:cognitect.aws.glue.RunStatementRequest/SessionId
:cognitect.aws.glue.RunStatementRequest/Code]
:opt-un
[:cognitect.aws.glue.RunStatementRequest/RequestOrigin]))
(s/def
:cognitect.aws.glue/GetConnectionsFilter
(s/keys
:opt-un
[:cognitect.aws.glue.GetConnectionsFilter/ConnectionType
:cognitect.aws.glue.GetConnectionsFilter/MatchCriteria]))
(s/def
:cognitect.aws.glue/JDBCDataTypeMapping
(s/map-of :cognitect.aws.glue/JDBCDataType :cognitect.aws.glue/GlueRecordType))
(s/def :cognitect.aws.glue/Boolean boolean?)
(s/def
:cognitect.aws.glue/PartitionIndex
(s/keys
:req-un
[:cognitect.aws.glue.PartitionIndex/Keys :cognitect.aws.glue.PartitionIndex/IndexName]))
(s/def
:cognitect.aws.glue/StopCrawlerRequest
(s/keys :req-un [:cognitect.aws.glue.StopCrawlerRequest/Name]))
(s/def :cognitect.aws.glue/CatalogTargetList (s/coll-of :cognitect.aws.glue/CatalogTarget))
(s/def
:cognitect.aws.glue/JoinColumn
(s/keys :req-un [:cognitect.aws.glue.JoinColumn/From :cognitect.aws.glue.JoinColumn/Keys]))
(s/def
:cognitect.aws.glue/DirectKinesisSource
(s/keys
:req-un
[:cognitect.aws.glue.DirectKinesisSource/Name]
:opt-un
[:cognitect.aws.glue.DirectKinesisSource/StreamingOptions
:cognitect.aws.glue.DirectKinesisSource/DetectSchema
:cognitect.aws.glue.DirectKinesisSource/WindowSize
:cognitect.aws.glue.DirectKinesisSource/DataPreviewOptions]))
(s/def
:cognitect.aws.glue/DeletePartitionIndexRequest
(s/keys
:req-un
[:cognitect.aws.glue.DeletePartitionIndexRequest/DatabaseName
:cognitect.aws.glue.DeletePartitionIndexRequest/TableName
:cognitect.aws.glue.DeletePartitionIndexRequest/IndexName]
:opt-un
[:cognitect.aws.glue.DeletePartitionIndexRequest/CatalogId]))
(s/def
:cognitect.aws.glue/StartMLEvaluationTaskRunRequest
(s/keys :req-un [:cognitect.aws.glue.StartMLEvaluationTaskRunRequest/TransformId]))
(s/def
:cognitect.aws.glue/RunStatementResponse
(s/keys :opt-un [:cognitect.aws.glue.RunStatementResponse/Id]))
(s/def
:cognitect.aws.glue/QuerySchemaVersionMetadataInput
(s/keys
:opt-un
[:cognitect.aws.glue.QuerySchemaVersionMetadataInput/SchemaVersionNumber
:cognitect.aws.glue.QuerySchemaVersionMetadataInput/NextToken
:cognitect.aws.glue.QuerySchemaVersionMetadataInput/MetadataList
:cognitect.aws.glue.QuerySchemaVersionMetadataInput/SchemaId
:cognitect.aws.glue.QuerySchemaVersionMetadataInput/MaxResults
:cognitect.aws.glue.QuerySchemaVersionMetadataInput/SchemaVersionId]))
(s/def
:cognitect.aws.glue/GetClassifierResponse
(s/keys :opt-un [:cognitect.aws.glue.GetClassifierResponse/Classifier]))
(s/def
:cognitect.aws.glue/GenericMap
(s/map-of :cognitect.aws.glue/GenericString :cognitect.aws.glue/GenericString))
(s/def
:cognitect.aws.glue/DevEndpoint
(s/keys
:opt-un
[:cognitect.aws.glue.DevEndpoint/ZeppelinRemoteSparkInterpreterPort
:cognitect.aws.glue.DevEndpoint/CreatedTimestamp
:cognitect.aws.glue.DevEndpoint/SecurityGroupIds
:cognitect.aws.glue.DevEndpoint/YarnEndpointAddress
:cognitect.aws.glue.DevEndpoint/SubnetId
:cognitect.aws.glue.DevEndpoint/PrivateAddress
:cognitect.aws.glue.DevEndpoint/Arguments
:cognitect.aws.glue.DevEndpoint/ExtraJarsS3Path
:cognitect.aws.glue.DevEndpoint/RoleArn
:cognitect.aws.glue.DevEndpoint/NumberOfNodes
:cognitect.aws.glue.DevEndpoint/LastUpdateStatus
:cognitect.aws.glue.DevEndpoint/NumberOfWorkers
:cognitect.aws.glue.DevEndpoint/WorkerType
:cognitect.aws.glue.DevEndpoint/AvailabilityZone
:cognitect.aws.glue.DevEndpoint/Status
:cognitect.aws.glue.DevEndpoint/EndpointName
:cognitect.aws.glue.DevEndpoint/PublicKeys
:cognitect.aws.glue.DevEndpoint/GlueVersion
:cognitect.aws.glue.DevEndpoint/LastModifiedTimestamp
:cognitect.aws.glue.DevEndpoint/VpcId
:cognitect.aws.glue.DevEndpoint/FailureReason
:cognitect.aws.glue.DevEndpoint/SecurityConfiguration
:cognitect.aws.glue.DevEndpoint/PublicAddress
:cognitect.aws.glue.DevEndpoint/ExtraPythonLibsS3Path
:cognitect.aws.glue.DevEndpoint/PublicKey]))
(s/def
:cognitect.aws.glue/VersionString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/TransformEncryption
(s/keys
:opt-un
[:cognitect.aws.glue.TransformEncryption/TaskRunSecurityConfigurationName
:cognitect.aws.glue.TransformEncryption/MlUserDataEncryption]))
(s/def
:cognitect.aws.glue/BatchDeleteConnectionRequest
(s/keys
:req-un
[:cognitect.aws.glue.BatchDeleteConnectionRequest/ConnectionNameList]
:opt-un
[:cognitect.aws.glue.BatchDeleteConnectionRequest/CatalogId]))
(s/def :cognitect.aws.glue/NullableBoolean boolean?)
(s/def :cognitect.aws.glue/PutDataCatalogEncryptionSettingsResponse (s/keys))
(s/def
:cognitect.aws.glue/Database
(s/keys
:req-un
[:cognitect.aws.glue.Database/Name]
:opt-un
[:cognitect.aws.glue.Database/FederatedDatabase
:cognitect.aws.glue.Database/Parameters
:cognitect.aws.glue.Database/Description
:cognitect.aws.glue.Database/TargetDatabase
:cognitect.aws.glue.Database/CreateTime
:cognitect.aws.glue.Database/CatalogId
:cognitect.aws.glue.Database/LocationUri
:cognitect.aws.glue.Database/CreateTableDefaultPermissions]))
(s/def
:cognitect.aws.glue/VersionsString
(s/spec #(re-matches (re-pattern "[1-9][0-9]*|[1-9][0-9]*-[1-9][0-9]*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/PiiType
(s/spec string? :gen #(s/gen #{"RowMasking" "ColumnMasking" "ColumnAudit" "RowAudit"})))
(s/def
:cognitect.aws.glue/EncryptionAtRest
(s/keys
:req-un
[:cognitect.aws.glue.EncryptionAtRest/CatalogEncryptionMode]
:opt-un
[:cognitect.aws.glue.EncryptionAtRest/SseAwsKmsKeyId]))
(s/def
:cognitect.aws.glue/QuerySchemaVersionMetadataMaxResults
(s/spec (s/and int? #(<= 1 % 50)) :gen #(gen/choose 1 50)))
(s/def
:cognitect.aws.glue/AmazonRedshiftTarget
(s/keys
:opt-un
[:cognitect.aws.glue.AmazonRedshiftTarget/Inputs
:cognitect.aws.glue.AmazonRedshiftTarget/Data
:cognitect.aws.glue.AmazonRedshiftTarget/Name]))
(s/def
:cognitect.aws.glue/GetWorkflowResponse
(s/keys :opt-un [:cognitect.aws.glue.GetWorkflowResponse/Workflow]))
(s/def
:cognitect.aws.glue/ScriptLocationString
(s/spec
(s/and string? #(>= 400000 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 400000) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/BatchUpdatePartitionResponse
(s/keys :opt-un [:cognitect.aws.glue.BatchUpdatePartitionResponse/Errors]))
(s/def :cognitect.aws.glue/SchemaDiffType (s/spec string? :gen #(s/gen #{"SYNTAX_DIFF"})))
(s/def :cognitect.aws.glue/ClassifierNameList (s/coll-of :cognitect.aws.glue/NameString))
(s/def
:cognitect.aws.glue/VersionLongNumber
(s/spec (s/and int? #(<= 1 % 100000)) :gen #(gen/choose 1 100000)))
(s/def
:cognitect.aws.glue/CancelDataQualityRuleRecommendationRunRequest
(s/keys :req-un [:cognitect.aws.glue.CancelDataQualityRuleRecommendationRunRequest/RunId]))
(s/def
:cognitect.aws.glue/NotifyDelayAfter
(s/spec (s/and int? #(<= 1 %)) :gen #(gen/choose 1 Long/MAX_VALUE)))
(s/def :cognitect.aws.glue/UnionType (s/spec string? :gen #(s/gen #{"DISTINCT" "ALL"})))
(s/def
:cognitect.aws.glue/GetUnfilteredPartitionsMetadataRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/CatalogId
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/DatabaseName
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/TableName
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/SupportedPermissionTypes]
:opt-un
[:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/AuditContext
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/Expression
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/NextToken
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/Segment
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/MaxResults]))
(s/def
:cognitect.aws.glue/GluePolicy
(s/keys
:opt-un
[:cognitect.aws.glue.GluePolicy/PolicyInJson
:cognitect.aws.glue.GluePolicy/UpdateTime
:cognitect.aws.glue.GluePolicy/CreateTime
:cognitect.aws.glue.GluePolicy/PolicyHash]))
(s/def
:cognitect.aws.glue/CreateSessionResponse
(s/keys :opt-un [:cognitect.aws.glue.CreateSessionResponse/Session]))
(s/def
:cognitect.aws.glue/EvaluateDataQualityMultiFrame
(s/keys
:req-un
[:cognitect.aws.glue.EvaluateDataQualityMultiFrame/Name
:cognitect.aws.glue.EvaluateDataQualityMultiFrame/Inputs
:cognitect.aws.glue.EvaluateDataQualityMultiFrame/Ruleset]
:opt-un
[:cognitect.aws.glue.EvaluateDataQualityMultiFrame/AdditionalDataSources
:cognitect.aws.glue.EvaluateDataQualityMultiFrame/AdditionalOptions
:cognitect.aws.glue.EvaluateDataQualityMultiFrame/PublishingOptions
:cognitect.aws.glue.EvaluateDataQualityMultiFrame/StopJobOnFailureOptions]))
(s/def
:cognitect.aws.glue/WorkflowRunStatus
(s/spec string? :gen #(s/gen #{"COMPLETED" "ERROR" "STOPPED" "STOPPING" "RUNNING"})))
(s/def
:cognitect.aws.glue/GetPartitionsRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetPartitionsRequest/DatabaseName
:cognitect.aws.glue.GetPartitionsRequest/TableName]
:opt-un
[:cognitect.aws.glue.GetPartitionsRequest/TransactionId
:cognitect.aws.glue.GetPartitionsRequest/Expression
:cognitect.aws.glue.GetPartitionsRequest/NextToken
:cognitect.aws.glue.GetPartitionsRequest/QueryAsOfTime
:cognitect.aws.glue.GetPartitionsRequest/Segment
:cognitect.aws.glue.GetPartitionsRequest/CatalogId
:cognitect.aws.glue.GetPartitionsRequest/MaxResults
:cognitect.aws.glue.GetPartitionsRequest/ExcludeColumnSchema]))
(s/def
:cognitect.aws.glue/BlueprintStatus
(s/spec string? :gen #(s/gen #{"UPDATING" "CREATING" "ACTIVE" "FAILED"})))
(s/def
:cognitect.aws.glue/OtherMetadataValueList
(s/coll-of :cognitect.aws.glue/OtherMetadataValueListItem))
(s/def
:cognitect.aws.glue/GetCatalogImportStatusResponse
(s/keys :opt-un [:cognitect.aws.glue.GetCatalogImportStatusResponse/ImportStatus]))
(s/def
:cognitect.aws.glue/DQStopJobOnFailureTiming
(s/spec string? :gen #(s/gen #{"AfterDataLoad" "Immediate"})))
(s/def :cognitect.aws.glue/JobNameList (s/coll-of :cognitect.aws.glue/NameString))
(s/def :cognitect.aws.glue/BlueprintRuns (s/coll-of :cognitect.aws.glue/BlueprintRun))
(s/def
:cognitect.aws.glue/DatabaseIdentifier
(s/keys
:opt-un
[:cognitect.aws.glue.DatabaseIdentifier/DatabaseName
:cognitect.aws.glue.DatabaseIdentifier/CatalogId
:cognitect.aws.glue.DatabaseIdentifier/Region]))
(s/def
:cognitect.aws.glue/ConnectionPasswordEncryption
(s/keys
:req-un
[:cognitect.aws.glue.ConnectionPasswordEncryption/ReturnConnectionPasswordEncrypted]
:opt-un
[:cognitect.aws.glue.ConnectionPasswordEncryption/AwsKmsKeyId]))
(s/def
:cognitect.aws.glue/TaskRunSortCriteria
(s/keys
:req-un
[:cognitect.aws.glue.TaskRunSortCriteria/Column
:cognitect.aws.glue.TaskRunSortCriteria/SortDirection]))
(s/def
:cognitect.aws.glue/StringColumnStatisticsData
(s/keys
:req-un
[:cognitect.aws.glue.StringColumnStatisticsData/MaximumLength
:cognitect.aws.glue.StringColumnStatisticsData/AverageLength
:cognitect.aws.glue.StringColumnStatisticsData/NumberOfNulls
:cognitect.aws.glue.StringColumnStatisticsData/NumberOfDistinctValues]))
(s/def
:cognitect.aws.glue/Aggregate
(s/keys
:req-un
[:cognitect.aws.glue.Aggregate/Name
:cognitect.aws.glue.Aggregate/Inputs
:cognitect.aws.glue.Aggregate/Groups
:cognitect.aws.glue.Aggregate/Aggs]))
(s/def
:cognitect.aws.glue/GetJobRunsRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetJobRunsRequest/JobName]
:opt-un
[:cognitect.aws.glue.GetJobRunsRequest/NextToken
:cognitect.aws.glue.GetJobRunsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/PythonVersionString
(s/spec #(re-matches (re-pattern "^([2-3]|3[.]9)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/GetDataQualityRulesetEvaluationRunResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/RulesetNames
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/Timeout
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/RunId
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/AdditionalRunOptions
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/ExecutionTime
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/AdditionalDataSources
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/NumberOfWorkers
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/LastModifiedOn
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/Status
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/Role
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/DataSource
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/ErrorString
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/StartedOn
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/ResultIds
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/CompletedOn]))
(s/def
:cognitect.aws.glue/KafkaStreamingSourceOptions
(s/keys
:opt-un
[:cognitect.aws.glue.KafkaStreamingSourceOptions/AddRecordTimestamp
:cognitect.aws.glue.KafkaStreamingSourceOptions/TopicName
:cognitect.aws.glue.KafkaStreamingSourceOptions/ConnectionName
:cognitect.aws.glue.KafkaStreamingSourceOptions/MinPartitions
:cognitect.aws.glue.KafkaStreamingSourceOptions/StartingOffsets
:cognitect.aws.glue.KafkaStreamingSourceOptions/EndingOffsets
:cognitect.aws.glue.KafkaStreamingSourceOptions/NumRetries
:cognitect.aws.glue.KafkaStreamingSourceOptions/IncludeHeaders
:cognitect.aws.glue.KafkaStreamingSourceOptions/SecurityProtocol
:cognitect.aws.glue.KafkaStreamingSourceOptions/SubscribePattern
:cognitect.aws.glue.KafkaStreamingSourceOptions/Classification
:cognitect.aws.glue.KafkaStreamingSourceOptions/Assign
:cognitect.aws.glue.KafkaStreamingSourceOptions/PollTimeoutMs
:cognitect.aws.glue.KafkaStreamingSourceOptions/EmitConsumerLagMetrics
:cognitect.aws.glue.KafkaStreamingSourceOptions/Delimiter
:cognitect.aws.glue.KafkaStreamingSourceOptions/RetryIntervalMs
:cognitect.aws.glue.KafkaStreamingSourceOptions/StartingTimestamp
:cognitect.aws.glue.KafkaStreamingSourceOptions/BootstrapServers
:cognitect.aws.glue.KafkaStreamingSourceOptions/MaxOffsetsPerTrigger]))
(s/def
:cognitect.aws.glue/WorkflowRun
(s/keys
:opt-un
[:cognitect.aws.glue.WorkflowRun/Status
:cognitect.aws.glue.WorkflowRun/WorkflowRunProperties
:cognitect.aws.glue.WorkflowRun/Graph
:cognitect.aws.glue.WorkflowRun/WorkflowRunId
:cognitect.aws.glue.WorkflowRun/ErrorMessage
:cognitect.aws.glue.WorkflowRun/StartingEventBatchCondition
:cognitect.aws.glue.WorkflowRun/PreviousRunId
:cognitect.aws.glue.WorkflowRun/StartedOn
:cognitect.aws.glue.WorkflowRun/Statistics
:cognitect.aws.glue.WorkflowRun/Name
:cognitect.aws.glue.WorkflowRun/CompletedOn]))
(s/def
:cognitect.aws.glue/RecrawlBehavior
(s/spec string? :gen #(s/gen #{"CRAWL_EVENT_MODE" "CRAWL_EVERYTHING" "CRAWL_NEW_FOLDERS_ONLY"})))
(s/def
:cognitect.aws.glue/MetadataValueString
(s/spec #(re-matches (re-pattern "[a-zA-Z0-9+-=._./@]+") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/PermissionTypeList
(s/coll-of :cognitect.aws.glue/PermissionType :min-count 1 :max-count 255))
(s/def
:cognitect.aws.glue/GetWorkflowRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetWorkflowRunRequest/Name
:cognitect.aws.glue.GetWorkflowRunRequest/RunId]
:opt-un
[:cognitect.aws.glue.GetWorkflowRunRequest/IncludeGraph]))
(s/def
:cognitect.aws.glue/ConnectionsList
(s/keys :opt-un [:cognitect.aws.glue.ConnectionsList/Connections]))
(s/def
:cognitect.aws.glue/S3HudiCatalogTarget
(s/keys
:req-un
[:cognitect.aws.glue.S3HudiCatalogTarget/Name
:cognitect.aws.glue.S3HudiCatalogTarget/Inputs
:cognitect.aws.glue.S3HudiCatalogTarget/Table
:cognitect.aws.glue.S3HudiCatalogTarget/Database
:cognitect.aws.glue.S3HudiCatalogTarget/AdditionalOptions]
:opt-un
[:cognitect.aws.glue.S3HudiCatalogTarget/PartitionKeys
:cognitect.aws.glue.S3HudiCatalogTarget/SchemaChangePolicy]))
(s/def
:cognitect.aws.glue/GlueStudioPathList
(s/coll-of :cognitect.aws.glue/EnclosedInStringProperties))
(s/def
:cognitect.aws.glue/GetJobRunResponse
(s/keys :opt-un [:cognitect.aws.glue.GetJobRunResponse/JobRun]))
(s/def
:cognitect.aws.glue/StorageDescriptor
(s/keys
:opt-un
[:cognitect.aws.glue.StorageDescriptor/NumberOfBuckets
:cognitect.aws.glue.StorageDescriptor/Parameters
:cognitect.aws.glue.StorageDescriptor/Location
:cognitect.aws.glue.StorageDescriptor/SchemaReference
:cognitect.aws.glue.StorageDescriptor/Compressed
:cognitect.aws.glue.StorageDescriptor/SerdeInfo
:cognitect.aws.glue.StorageDescriptor/OutputFormat
:cognitect.aws.glue.StorageDescriptor/SkewedInfo
:cognitect.aws.glue.StorageDescriptor/StoredAsSubDirectories
:cognitect.aws.glue.StorageDescriptor/BucketColumns
:cognitect.aws.glue.StorageDescriptor/InputFormat
:cognitect.aws.glue.StorageDescriptor/Columns
:cognitect.aws.glue.StorageDescriptor/AdditionalLocations
:cognitect.aws.glue.StorageDescriptor/SortColumns]))
(s/def
:cognitect.aws.glue/StatementState
(s/spec
string?
:gen
#(s/gen #{"CANCELLING" "AVAILABLE" "ERROR" "WAITING" "CANCELLED" "RUNNING"})))
(s/def
:cognitect.aws.glue/StreamingDataPreviewOptions
(s/keys
:opt-un
[:cognitect.aws.glue.StreamingDataPreviewOptions/RecordPollingLimit
:cognitect.aws.glue.StreamingDataPreviewOptions/PollingTime]))
(s/def
:cognitect.aws.glue/WorkflowRunProperties
(s/map-of :cognitect.aws.glue/IdString :cognitect.aws.glue/GenericString))
(s/def
:cognitect.aws.glue/GetConnectionsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetConnectionsResponse/ConnectionList
:cognitect.aws.glue.GetConnectionsResponse/NextToken]))
(s/def
:cognitect.aws.glue/StartDataQualityRulesetEvaluationRunResponse
(s/keys :opt-un [:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunResponse/RunId]))
(s/def
:cognitect.aws.glue/CrawlerHistoryState
(s/spec string? :gen #(s/gen #{"COMPLETED" "STOPPED" "FAILED" "RUNNING"})))
(s/def :cognitect.aws.glue/BatchWindow (s/spec (s/and int? #(<= 1 % 900)) :gen #(gen/choose 1 900)))
(s/def :cognitect.aws.glue/CustomDatatypes (s/coll-of :cognitect.aws.glue/NameString))
(s/def
:cognitect.aws.glue/DescriptionString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\r\\n\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/UpdateDataQualityRulesetRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateDataQualityRulesetRequest/Name]
:opt-un
[:cognitect.aws.glue.UpdateDataQualityRulesetRequest/Description
:cognitect.aws.glue.UpdateDataQualityRulesetRequest/Ruleset]))
(s/def
:cognitect.aws.glue/MLTransform
(s/keys
:opt-un
[:cognitect.aws.glue.MLTransform/Schema
:cognitect.aws.glue.MLTransform/InputRecordTables
:cognitect.aws.glue.MLTransform/Timeout
:cognitect.aws.glue.MLTransform/MaxCapacity
:cognitect.aws.glue.MLTransform/Parameters
:cognitect.aws.glue.MLTransform/EvaluationMetrics
:cognitect.aws.glue.MLTransform/NumberOfWorkers
:cognitect.aws.glue.MLTransform/WorkerType
:cognitect.aws.glue.MLTransform/LastModifiedOn
:cognitect.aws.glue.MLTransform/Status
:cognitect.aws.glue.MLTransform/TransformId
:cognitect.aws.glue.MLTransform/Role
:cognitect.aws.glue.MLTransform/GlueVersion
:cognitect.aws.glue.MLTransform/Description
:cognitect.aws.glue.MLTransform/TransformEncryption
:cognitect.aws.glue.MLTransform/LabelCount
:cognitect.aws.glue.MLTransform/MaxRetries
:cognitect.aws.glue.MLTransform/Name
:cognitect.aws.glue.MLTransform/CreatedOn]))
(s/def
:cognitect.aws.glue/TagKeysList
(s/coll-of :cognitect.aws.glue/TagKey :min-count 0 :max-count 50))
(s/def
:cognitect.aws.glue/GetUserDefinedFunctionsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetUserDefinedFunctionsResponse/NextToken
:cognitect.aws.glue.GetUserDefinedFunctionsResponse/UserDefinedFunctions]))
(s/def
:cognitect.aws.glue/DeleteSessionRequest
(s/keys
:req-un
[:cognitect.aws.glue.DeleteSessionRequest/Id]
:opt-un
[:cognitect.aws.glue.DeleteSessionRequest/RequestOrigin]))
(s/def
:cognitect.aws.glue/BatchDeletePartitionRequest
(s/keys
:req-un
[:cognitect.aws.glue.BatchDeletePartitionRequest/DatabaseName
:cognitect.aws.glue.BatchDeletePartitionRequest/TableName
:cognitect.aws.glue.BatchDeletePartitionRequest/PartitionsToDelete]
:opt-un
[:cognitect.aws.glue.BatchDeletePartitionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/CreateSchemaResponse
(s/keys
:opt-un
[:cognitect.aws.glue.CreateSchemaResponse/RegistryArn
:cognitect.aws.glue.CreateSchemaResponse/SchemaStatus
:cognitect.aws.glue.CreateSchemaResponse/DataFormat
:cognitect.aws.glue.CreateSchemaResponse/Tags
:cognitect.aws.glue.CreateSchemaResponse/NextSchemaVersion
:cognitect.aws.glue.CreateSchemaResponse/Compatibility
:cognitect.aws.glue.CreateSchemaResponse/Description
:cognitect.aws.glue.CreateSchemaResponse/SchemaVersionStatus
:cognitect.aws.glue.CreateSchemaResponse/RegistryName
:cognitect.aws.glue.CreateSchemaResponse/LatestSchemaVersion
:cognitect.aws.glue.CreateSchemaResponse/SchemaName
:cognitect.aws.glue.CreateSchemaResponse/SchemaArn
:cognitect.aws.glue.CreateSchemaResponse/SchemaCheckpoint
:cognitect.aws.glue.CreateSchemaResponse/SchemaVersionId]))
(s/def :cognitect.aws.glue/UpdatePartitionResponse (s/keys))
(s/def
:cognitect.aws.glue/ListWorkflowsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.ListWorkflowsRequest/NextToken
:cognitect.aws.glue.ListWorkflowsRequest/MaxResults]))
(s/def :cognitect.aws.glue/UpdateClassifierResponse (s/keys))
(s/def
:cognitect.aws.glue/PartitionError
(s/keys
:opt-un
[:cognitect.aws.glue.PartitionError/ErrorDetail
:cognitect.aws.glue.PartitionError/PartitionValues]))
(s/def
:cognitect.aws.glue/Separator
(s/spec string? :gen #(s/gen #{"tab" "comma" "semicolon" "pipe" "ctrla"})))
(s/def
:cognitect.aws.glue/CreateClassifierRequest
(s/keys
:opt-un
[:cognitect.aws.glue.CreateClassifierRequest/GrokClassifier
:cognitect.aws.glue.CreateClassifierRequest/XMLClassifier
:cognitect.aws.glue.CreateClassifierRequest/JsonClassifier
:cognitect.aws.glue.CreateClassifierRequest/CsvClassifier]))
(s/def
:cognitect.aws.glue/NonNegativeLong
(s/spec (s/and int? #(<= 0 %)) :gen #(gen/choose 0 Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/SortDirectionType
(s/spec string? :gen #(s/gen #{"ASCENDING" "DESCENDING"})))
(s/def
:cognitect.aws.glue/DataQualityRuleResults
(s/coll-of :cognitect.aws.glue/DataQualityRuleResult :min-count 1 :max-count 2000))
(s/def
:cognitect.aws.glue/DataQualityTargetTable
(s/keys
:req-un
[:cognitect.aws.glue.DataQualityTargetTable/TableName
:cognitect.aws.glue.DataQualityTargetTable/DatabaseName]
:opt-un
[:cognitect.aws.glue.DataQualityTargetTable/CatalogId]))
(s/def :cognitect.aws.glue/LocationStringList (s/coll-of :cognitect.aws.glue/LocationString))
(s/def
:cognitect.aws.glue/MaxConcurrentRuns
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/GetMLTransformRequest
(s/keys :req-un [:cognitect.aws.glue.GetMLTransformRequest/TransformId]))
(s/def
:cognitect.aws.glue/UnfilteredPartitionList
(s/coll-of :cognitect.aws.glue/UnfilteredPartition))
(s/def
:cognitect.aws.glue/StartDataQualityRuleRecommendationRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.StartDataQualityRuleRecommendationRunRequest/DataSource
:cognitect.aws.glue.StartDataQualityRuleRecommendationRunRequest/Role]
:opt-un
[:cognitect.aws.glue.StartDataQualityRuleRecommendationRunRequest/Timeout
:cognitect.aws.glue.StartDataQualityRuleRecommendationRunRequest/CreatedRulesetName
:cognitect.aws.glue.StartDataQualityRuleRecommendationRunRequest/NumberOfWorkers
:cognitect.aws.glue.StartDataQualityRuleRecommendationRunRequest/ClientToken]))
(s/def
:cognitect.aws.glue/ConfusionMatrix
(s/keys
:opt-un
[:cognitect.aws.glue.ConfusionMatrix/NumTrueNegatives
:cognitect.aws.glue.ConfusionMatrix/NumFalsePositives
:cognitect.aws.glue.ConfusionMatrix/NumTruePositives
:cognitect.aws.glue.ConfusionMatrix/NumFalseNegatives]))
(s/def
:cognitect.aws.glue/CatalogSource
(s/keys
:req-un
[:cognitect.aws.glue.CatalogSource/Name
:cognitect.aws.glue.CatalogSource/Database
:cognitect.aws.glue.CatalogSource/Table]))
(s/def :cognitect.aws.glue/UpdateDatabaseResponse (s/keys))
(s/def :cognitect.aws.glue/Integer (s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/CrawlerLineageSettings
(s/spec string? :gen #(s/gen #{"DISABLE" "ENABLE"})))
(s/def
:cognitect.aws.glue/StopTriggerRequest
(s/keys :req-un [:cognitect.aws.glue.StopTriggerRequest/Name]))
(s/def
:cognitect.aws.glue/S3DeltaDirectTarget
(s/keys
:req-un
[:cognitect.aws.glue.S3DeltaDirectTarget/Name
:cognitect.aws.glue.S3DeltaDirectTarget/Inputs
:cognitect.aws.glue.S3DeltaDirectTarget/Path
:cognitect.aws.glue.S3DeltaDirectTarget/Compression
:cognitect.aws.glue.S3DeltaDirectTarget/Format]
:opt-un
[:cognitect.aws.glue.S3DeltaDirectTarget/AdditionalOptions
:cognitect.aws.glue.S3DeltaDirectTarget/PartitionKeys
:cognitect.aws.glue.S3DeltaDirectTarget/SchemaChangePolicy]))
(s/def
:cognitect.aws.glue/CheckSchemaVersionValidityInput
(s/keys
:req-un
[:cognitect.aws.glue.CheckSchemaVersionValidityInput/DataFormat
:cognitect.aws.glue.CheckSchemaVersionValidityInput/SchemaDefinition]))
(s/def
:cognitect.aws.glue/CustomEntityTypeNames
(s/coll-of :cognitect.aws.glue/NameString :min-count 1 :max-count 50))
(s/def
:cognitect.aws.glue/RecordsCount
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/AdditionalOptions
(s/map-of
:cognitect.aws.glue/EnclosedInStringProperty
:cognitect.aws.glue/EnclosedInStringProperty))
(s/def
:cognitect.aws.glue/ListCrawlsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListCrawlsResponse/NextToken
:cognitect.aws.glue.ListCrawlsResponse/Crawls]))
(s/def
:cognitect.aws.glue/BatchStopJobRunSuccessfulSubmission
(s/keys
:opt-un
[:cognitect.aws.glue.BatchStopJobRunSuccessfulSubmission/JobName
:cognitect.aws.glue.BatchStopJobRunSuccessfulSubmission/JobRunId]))
(s/def
:cognitect.aws.glue/FormatString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/SearchTablesResponse
(s/keys
:opt-un
[:cognitect.aws.glue.SearchTablesResponse/TableList
:cognitect.aws.glue.SearchTablesResponse/NextToken]))
(s/def :cognitect.aws.glue/PrincipalType (s/spec string? :gen #(s/gen #{"USER" "ROLE" "GROUP"})))
(s/def
:cognitect.aws.glue/DirectSchemaChangePolicy
(s/keys
:opt-un
[:cognitect.aws.glue.DirectSchemaChangePolicy/EnableUpdateCatalog
:cognitect.aws.glue.DirectSchemaChangePolicy/UpdateBehavior
:cognitect.aws.glue.DirectSchemaChangePolicy/Table
:cognitect.aws.glue.DirectSchemaChangePolicy/Database]))
(s/def
:cognitect.aws.glue/ListDataQualityRulesetEvaluationRunsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListDataQualityRulesetEvaluationRunsResponse/NextToken
:cognitect.aws.glue.ListDataQualityRulesetEvaluationRunsResponse/Runs]))
(s/def
:cognitect.aws.glue/BatchDeleteTableResponse
(s/keys :opt-un [:cognitect.aws.glue.BatchDeleteTableResponse/Errors]))
(s/def
:cognitect.aws.glue/CrawlState
(s/spec string? :gen #(s/gen #{"CANCELLING" "ERROR" "SUCCEEDED" "FAILED" "CANCELLED" "RUNNING"})))
(s/def :cognitect.aws.glue/UriString string?)
(s/def
:cognitect.aws.glue/BoxedDoubleFraction
(s/spec
(s/and double? #(<= 0 % 1))
:gen
#(gen/double* {:infinite? false, :NaN? false, :min 0, :max 1})))
(s/def
:cognitect.aws.glue/CatalogImportStatus
(s/keys
:opt-un
[:cognitect.aws.glue.CatalogImportStatus/ImportCompleted
:cognitect.aws.glue.CatalogImportStatus/ImportTime
:cognitect.aws.glue.CatalogImportStatus/ImportedBy]))
(s/def :cognitect.aws.glue/DeleteTableResponse (s/keys))
(s/def
:cognitect.aws.glue/JDBCDataType
(s/spec
string?
:gen
#(s/gen
#{"NULL"
"NVARCHAR"
"BIGINT"
"SQLXML"
"LONGNVARCHAR"
"TIMESTAMP"
"VARCHAR"
"REF_CURSOR"
"ARRAY"
"DATE"
"TINYINT"
"NCHAR"
"NCLOB"
"DISTINCT"
"DOUBLE"
"STRUCT"
"LONGVARCHAR"
"LONGVARBINARY"
"JAVA_OBJECT"
"REF"
"OTHER"
"TIME"
"DECIMAL"
"INTEGER"
"TIMESTAMP_WITH_TIMEZONE"
"VARBINARY"
"TIME_WITH_TIMEZONE"
"BIT"
"CLOB"
"SMALLINT"
"BLOB"
"FLOAT"
"ROWID"
"CHAR"
"REAL"
"NUMERIC"
"BOOLEAN"
"DATALINK"
"BINARY"})))
(s/def
:cognitect.aws.glue/GetResourcePoliciesRequest
(s/keys
:opt-un
[:cognitect.aws.glue.GetResourcePoliciesRequest/NextToken
:cognitect.aws.glue.GetResourcePoliciesRequest/MaxResults]))
(s/def
:cognitect.aws.glue/TransformSchema
(s/coll-of :cognitect.aws.glue/SchemaColumn :max-count 100))
(s/def
:cognitect.aws.glue/TagValue
(s/spec
(s/and string? #(<= 0 (count %) 256))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 256) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/GetCrawlerRequest
(s/keys :req-un [:cognitect.aws.glue.GetCrawlerRequest/Name]))
(s/def
:cognitect.aws.glue/BlueprintRun
(s/keys
:opt-un
[:cognitect.aws.glue.BlueprintRun/RunId
:cognitect.aws.glue.BlueprintRun/Parameters
:cognitect.aws.glue.BlueprintRun/WorkflowName
:cognitect.aws.glue.BlueprintRun/RoleArn
:cognitect.aws.glue.BlueprintRun/RollbackErrorMessage
:cognitect.aws.glue.BlueprintRun/BlueprintName
:cognitect.aws.glue.BlueprintRun/ErrorMessage
:cognitect.aws.glue.BlueprintRun/StartedOn
:cognitect.aws.glue.BlueprintRun/State
:cognitect.aws.glue.BlueprintRun/CompletedOn]))
(s/def :cognitect.aws.glue/StartCrawlerScheduleResponse (s/keys))
(s/def
:cognitect.aws.glue/SparkSQL
(s/keys
:req-un
[:cognitect.aws.glue.SparkSQL/Name
:cognitect.aws.glue.SparkSQL/Inputs
:cognitect.aws.glue.SparkSQL/SqlQuery
:cognitect.aws.glue.SparkSQL/SqlAliases]
:opt-un
[:cognitect.aws.glue.SparkSQL/OutputSchemas]))
(s/def
:cognitect.aws.glue/GlueTableAdditionalOptions
(s/map-of
:cognitect.aws.glue/NameString
:cognitect.aws.glue/DescriptionString
:min-count
1
:max-count
10))
(s/def
:cognitect.aws.glue/CodeGenIdentifier
(s/spec #(re-matches (re-pattern "[A-Za-z_][A-Za-z0-9_]*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/ListCrawlsRequest
(s/keys
:req-un
[:cognitect.aws.glue.ListCrawlsRequest/CrawlerName]
:opt-un
[:cognitect.aws.glue.ListCrawlsRequest/NextToken
:cognitect.aws.glue.ListCrawlsRequest/Filters
:cognitect.aws.glue.ListCrawlsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/BatchDeleteConnectionResponse
(s/keys
:opt-un
[:cognitect.aws.glue.BatchDeleteConnectionResponse/Succeeded
:cognitect.aws.glue.BatchDeleteConnectionResponse/Errors]))
(s/def
:cognitect.aws.glue/S3DeltaSource
(s/keys
:req-un
[:cognitect.aws.glue.S3DeltaSource/Name :cognitect.aws.glue.S3DeltaSource/Paths]
:opt-un
[:cognitect.aws.glue.S3DeltaSource/OutputSchemas
:cognitect.aws.glue.S3DeltaSource/AdditionalOptions
:cognitect.aws.glue.S3DeltaSource/AdditionalDeltaOptions]))
(s/def
:cognitect.aws.glue/SchemaCheckpointNumber
(s/spec (s/and int? #(<= 1 % 100000)) :gen #(gen/choose 1 100000)))
(s/def
:cognitect.aws.glue/Blueprint
(s/keys
:opt-un
[:cognitect.aws.glue.Blueprint/BlueprintLocation
:cognitect.aws.glue.Blueprint/LastModifiedOn
:cognitect.aws.glue.Blueprint/Status
:cognitect.aws.glue.Blueprint/ErrorMessage
:cognitect.aws.glue.Blueprint/Description
:cognitect.aws.glue.Blueprint/ParameterSpec
:cognitect.aws.glue.Blueprint/BlueprintServiceLocation
:cognitect.aws.glue.Blueprint/Name
:cognitect.aws.glue.Blueprint/CreatedOn
:cognitect.aws.glue.Blueprint/LastActiveDefinition]))
(s/def
:cognitect.aws.glue/PermissionType
(s/spec string? :gen #(s/gen #{"COLUMN_PERMISSION" "CELL_FILTER_PERMISSION"})))
(s/def :cognitect.aws.glue/ConnectionName string?)
(s/def
:cognitect.aws.glue/GetDatabasesRequest
(s/keys
:opt-un
[:cognitect.aws.glue.GetDatabasesRequest/NextToken
:cognitect.aws.glue.GetDatabasesRequest/CatalogId
:cognitect.aws.glue.GetDatabasesRequest/ResourceShareType
:cognitect.aws.glue.GetDatabasesRequest/MaxResults]))
(s/def
:cognitect.aws.glue/TransformConfigParameter
(s/keys
:req-un
[:cognitect.aws.glue.TransformConfigParameter/Name
:cognitect.aws.glue.TransformConfigParameter/Type]
:opt-un
[:cognitect.aws.glue.TransformConfigParameter/ListType
:cognitect.aws.glue.TransformConfigParameter/IsOptional
:cognitect.aws.glue.TransformConfigParameter/ValidationMessage
:cognitect.aws.glue.TransformConfigParameter/Value
:cognitect.aws.glue.TransformConfigParameter/ValidationRule]))
(s/def
:cognitect.aws.glue/ListDataQualityResultsResponse
(s/keys
:req-un
[:cognitect.aws.glue.ListDataQualityResultsResponse/Results]
:opt-un
[:cognitect.aws.glue.ListDataQualityResultsResponse/NextToken]))
(s/def
:cognitect.aws.glue/SessionStatus
(s/spec string? :gen #(s/gen #{"TIMEOUT" "PROVISIONING" "READY" "STOPPED" "FAILED" "STOPPING"})))
(s/def
:cognitect.aws.glue/DataQualityResultDescription
(s/keys
:opt-un
[:cognitect.aws.glue.DataQualityResultDescription/JobName
:cognitect.aws.glue.DataQualityResultDescription/JobRunId
:cognitect.aws.glue.DataQualityResultDescription/DataSource
:cognitect.aws.glue.DataQualityResultDescription/StartedOn
:cognitect.aws.glue.DataQualityResultDescription/ResultId]))
(s/def
:cognitect.aws.glue/EnclosedInStringPropertyWithQuote
(s/spec
#(re-matches
(re-pattern "([\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF]|[^\\S\\r\\n])*")
%)
:gen
#(gen/string)))
(s/def :cognitect.aws.glue/DeleteConnectionResponse (s/keys))
(s/def :cognitect.aws.glue/SqlAliases (s/coll-of :cognitect.aws.glue/SqlAlias))
(s/def
:cognitect.aws.glue/S3CsvSource
(s/keys
:req-un
[:cognitect.aws.glue.S3CsvSource/Name
:cognitect.aws.glue.S3CsvSource/Paths
:cognitect.aws.glue.S3CsvSource/Separator
:cognitect.aws.glue.S3CsvSource/QuoteChar]
:opt-un
[:cognitect.aws.glue.S3CsvSource/Multiline
:cognitect.aws.glue.S3CsvSource/GroupSize
:cognitect.aws.glue.S3CsvSource/CompressionType
:cognitect.aws.glue.S3CsvSource/OutputSchemas
:cognitect.aws.glue.S3CsvSource/Recurse
:cognitect.aws.glue.S3CsvSource/AdditionalOptions
:cognitect.aws.glue.S3CsvSource/WithHeader
:cognitect.aws.glue.S3CsvSource/MaxFilesInBand
:cognitect.aws.glue.S3CsvSource/WriteHeader
:cognitect.aws.glue.S3CsvSource/Escaper
:cognitect.aws.glue.S3CsvSource/Exclusions
:cognitect.aws.glue.S3CsvSource/OptimizePerformance
:cognitect.aws.glue.S3CsvSource/MaxBand
:cognitect.aws.glue.S3CsvSource/GroupFiles
:cognitect.aws.glue.S3CsvSource/SkipFirst]))
(s/def
:cognitect.aws.glue/NullableInteger
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/S3HudiSource
(s/keys
:req-un
[:cognitect.aws.glue.S3HudiSource/Name :cognitect.aws.glue.S3HudiSource/Paths]
:opt-un
[:cognitect.aws.glue.S3HudiSource/OutputSchemas
:cognitect.aws.glue.S3HudiSource/AdditionalHudiOptions
:cognitect.aws.glue.S3HudiSource/AdditionalOptions]))
(s/def
:cognitect.aws.glue/CreateSecurityConfigurationRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateSecurityConfigurationRequest/Name
:cognitect.aws.glue.CreateSecurityConfigurationRequest/EncryptionConfiguration]))
(s/def :cognitect.aws.glue/PartitionErrors (s/coll-of :cognitect.aws.glue/PartitionError))
(s/def
:cognitect.aws.glue/SearchTablesRequest
(s/keys
:opt-un
[:cognitect.aws.glue.SearchTablesRequest/SearchText
:cognitect.aws.glue.SearchTablesRequest/NextToken
:cognitect.aws.glue.SearchTablesRequest/Filters
:cognitect.aws.glue.SearchTablesRequest/CatalogId
:cognitect.aws.glue.SearchTablesRequest/ResourceShareType
:cognitect.aws.glue.SearchTablesRequest/MaxResults
:cognitect.aws.glue.SearchTablesRequest/SortCriteria]))
(s/def
:cognitect.aws.glue/ParamType
(s/spec string? :gen #(s/gen #{"float" "int" "list" "str" "null" "bool" "complex"})))
(s/def
:cognitect.aws.glue/MillisecondsCount
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/WorkflowRunStatistics
(s/keys
:opt-un
[:cognitect.aws.glue.WorkflowRunStatistics/ErroredActions
:cognitect.aws.glue.WorkflowRunStatistics/RunningActions
:cognitect.aws.glue.WorkflowRunStatistics/TimeoutActions
:cognitect.aws.glue.WorkflowRunStatistics/WaitingActions
:cognitect.aws.glue.WorkflowRunStatistics/TotalActions
:cognitect.aws.glue.WorkflowRunStatistics/StoppedActions
:cognitect.aws.glue.WorkflowRunStatistics/SucceededActions
:cognitect.aws.glue.WorkflowRunStatistics/FailedActions]))
(s/def
:cognitect.aws.glue/CrawlerNodeDetails
(s/keys :opt-un [:cognitect.aws.glue.CrawlerNodeDetails/Crawls]))
(s/def
:cognitect.aws.glue/DevEndpointCustomLibraries
(s/keys
:opt-un
[:cognitect.aws.glue.DevEndpointCustomLibraries/ExtraJarsS3Path
:cognitect.aws.glue.DevEndpointCustomLibraries/ExtraPythonLibsS3Path]))
(s/def
:cognitect.aws.glue/CreateTriggerRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateTriggerRequest/Name
:cognitect.aws.glue.CreateTriggerRequest/Type
:cognitect.aws.glue.CreateTriggerRequest/Actions]
:opt-un
[:cognitect.aws.glue.CreateTriggerRequest/WorkflowName
:cognitect.aws.glue.CreateTriggerRequest/Tags
:cognitect.aws.glue.CreateTriggerRequest/StartOnCreation
:cognitect.aws.glue.CreateTriggerRequest/EventBatchingCondition
:cognitect.aws.glue.CreateTriggerRequest/Description
:cognitect.aws.glue.CreateTriggerRequest/Schedule
:cognitect.aws.glue.CreateTriggerRequest/Predicate]))
(s/def
:cognitect.aws.glue/DynamoDBTarget
(s/keys
:opt-un
[:cognitect.aws.glue.DynamoDBTarget/Path
:cognitect.aws.glue.DynamoDBTarget/scanAll
:cognitect.aws.glue.DynamoDBTarget/scanRate]))
(s/def :cognitect.aws.glue/DataQualityResultsList (s/coll-of :cognitect.aws.glue/DataQualityResult))
(s/def
:cognitect.aws.glue/TableError
(s/keys
:opt-un
[:cognitect.aws.glue.TableError/ErrorDetail :cognitect.aws.glue.TableError/TableName]))
(s/def
:cognitect.aws.glue/EnclosedInStringProperty
(s/spec
#(re-matches
(re-pattern
"([\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF]|[^\\S\\r\\n\"'])*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/BatchGetCrawlersRequest
(s/keys :req-un [:cognitect.aws.glue.BatchGetCrawlersRequest/CrawlerNames]))
(s/def
:cognitect.aws.glue/EvaluationMetrics
(s/keys
:req-un
[:cognitect.aws.glue.EvaluationMetrics/TransformType]
:opt-un
[:cognitect.aws.glue.EvaluationMetrics/FindMatchesMetrics]))
(s/def :cognitect.aws.glue/CreatePartitionResponse (s/keys))
(s/def
:cognitect.aws.glue/TransactionIdString
(s/spec #(re-matches (re-pattern "[\\p{L}\\p{N}\\p{P}]*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/BatchGetJobsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.BatchGetJobsResponse/JobsNotFound
:cognitect.aws.glue.BatchGetJobsResponse/Jobs]))
(s/def
:cognitect.aws.glue/SchemaVersionListItem
(s/keys
:opt-un
[:cognitect.aws.glue.SchemaVersionListItem/Status
:cognitect.aws.glue.SchemaVersionListItem/CreatedTime
:cognitect.aws.glue.SchemaVersionListItem/VersionNumber
:cognitect.aws.glue.SchemaVersionListItem/SchemaArn
:cognitect.aws.glue.SchemaVersionListItem/SchemaVersionId]))
(s/def
:cognitect.aws.glue/CodeGenConfigurationNodes
(s/map-of :cognitect.aws.glue/NodeId :cognitect.aws.glue/CodeGenConfigurationNode))
(s/def
:cognitect.aws.glue/CommitIdString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/ResourceUri
(s/keys
:opt-un
[:cognitect.aws.glue.ResourceUri/Uri :cognitect.aws.glue.ResourceUri/ResourceType]))
(s/def
:cognitect.aws.glue/GetPartitionsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetPartitionsResponse/NextToken
:cognitect.aws.glue.GetPartitionsResponse/Partitions]))
(s/def
:cognitect.aws.glue/GetDevEndpointResponse
(s/keys :opt-un [:cognitect.aws.glue.GetDevEndpointResponse/DevEndpoint]))
(s/def
:cognitect.aws.glue/WorkerType
(s/spec string? :gen #(s/gen #{"G.1X" "G.4X" "Z.2X" "G.8X" "Standard" "G.025X" "G.2X"})))
(s/def :cognitect.aws.glue/ColumnValuesString string?)
(s/def :cognitect.aws.glue/StartCrawlerResponse (s/keys))
(s/def
:cognitect.aws.glue/Mapping
(s/keys
:opt-un
[:cognitect.aws.glue.Mapping/Dropped
:cognitect.aws.glue.Mapping/FromPath
:cognitect.aws.glue.Mapping/Children
:cognitect.aws.glue.Mapping/ToType
:cognitect.aws.glue.Mapping/FromType
:cognitect.aws.glue.Mapping/ToKey]))
(s/def
:cognitect.aws.glue/Job
(s/keys
:opt-un
[:cognitect.aws.glue.Job/AllocatedCapacity
:cognitect.aws.glue.Job/Timeout
:cognitect.aws.glue.Job/MaxCapacity
:cognitect.aws.glue.Job/LogUri
:cognitect.aws.glue.Job/ExecutionClass
:cognitect.aws.glue.Job/DefaultArguments
:cognitect.aws.glue.Job/NumberOfWorkers
:cognitect.aws.glue.Job/WorkerType
:cognitect.aws.glue.Job/LastModifiedOn
:cognitect.aws.glue.Job/NonOverridableArguments
:cognitect.aws.glue.Job/Connections
:cognitect.aws.glue.Job/Role
:cognitect.aws.glue.Job/SourceControlDetails
:cognitect.aws.glue.Job/GlueVersion
:cognitect.aws.glue.Job/Description
:cognitect.aws.glue.Job/SecurityConfiguration
:cognitect.aws.glue.Job/Command
:cognitect.aws.glue.Job/MaxRetries
:cognitect.aws.glue.Job/ExecutionProperty
:cognitect.aws.glue.Job/Name
:cognitect.aws.glue.Job/CreatedOn
:cognitect.aws.glue.Job/NotificationProperty
:cognitect.aws.glue.Job/CodeGenConfigurationNodes]))
(s/def
:cognitect.aws.glue/SchemaColumn
(s/keys
:opt-un
[:cognitect.aws.glue.SchemaColumn/DataType :cognitect.aws.glue.SchemaColumn/Name]))
(s/def
:cognitect.aws.glue/PutResourcePolicyResponse
(s/keys :opt-un [:cognitect.aws.glue.PutResourcePolicyResponse/PolicyHash]))
(s/def
:cognitect.aws.glue/DynamoDBCatalogSource
(s/keys
:req-un
[:cognitect.aws.glue.DynamoDBCatalogSource/Name
:cognitect.aws.glue.DynamoDBCatalogSource/Database
:cognitect.aws.glue.DynamoDBCatalogSource/Table]))
(s/def
:cognitect.aws.glue/PartitionInputList
(s/coll-of :cognitect.aws.glue/PartitionInput :min-count 0 :max-count 100))
(s/def
:cognitect.aws.glue/ColumnTypeString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/CreateCrawlerRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateCrawlerRequest/Name
:cognitect.aws.glue.CreateCrawlerRequest/Role
:cognitect.aws.glue.CreateCrawlerRequest/Targets]
:opt-un
[:cognitect.aws.glue.CreateCrawlerRequest/Classifiers
:cognitect.aws.glue.CreateCrawlerRequest/DatabaseName
:cognitect.aws.glue.CreateCrawlerRequest/LineageConfiguration
:cognitect.aws.glue.CreateCrawlerRequest/LakeFormationConfiguration
:cognitect.aws.glue.CreateCrawlerRequest/RecrawlPolicy
:cognitect.aws.glue.CreateCrawlerRequest/Tags
:cognitect.aws.glue.CreateCrawlerRequest/CrawlerSecurityConfiguration
:cognitect.aws.glue.CreateCrawlerRequest/TablePrefix
:cognitect.aws.glue.CreateCrawlerRequest/SchemaChangePolicy
:cognitect.aws.glue.CreateCrawlerRequest/Description
:cognitect.aws.glue.CreateCrawlerRequest/Schedule
:cognitect.aws.glue.CreateCrawlerRequest/Configuration]))
(s/def
:cognitect.aws.glue/ConnectionPropertyKey
(s/spec
string?
:gen
#(s/gen
#{"CUSTOM_JDBC_CERT"
"KAFKA_CLIENT_KEYSTORE"
"KAFKA_CLIENT_KEYSTORE_PASSWORD"
"JDBC_CONNECTION_URL"
"CONNECTOR_CLASS_NAME"
"ENCRYPTED_PASSWORD"
"CONNECTOR_URL"
"USERNAME"
"ENCRYPTED_KAFKA_CLIENT_KEYSTORE_PASSWORD"
"SECRET_ID"
"INSTANCE_ID"
"JDBC_DRIVER_JAR_URI"
"KAFKA_BOOTSTRAP_SERVERS"
"CONFIG_FILES"
"JDBC_ENGINE_VERSION"
"PASSWORD"
"KAFKA_CLIENT_KEY_PASSWORD"
"KAFKA_SKIP_CUSTOM_CERT_VALIDATION"
"HOST"
"JDBC_ENGINE"
"CONNECTION_URL"
"CUSTOM_JDBC_CERT_STRING"
"KAFKA_SSL_ENABLED"
"JDBC_ENFORCE_SSL"
"PORT"
"CONNECTOR_TYPE"
"KAFKA_CUSTOM_CERT"
"ENCRYPTED_KAFKA_CLIENT_KEY_PASSWORD"
"SKIP_CUSTOM_JDBC_CERT_VALIDATION"
"JDBC_DRIVER_CLASS_NAME"})))
(s/def
:cognitect.aws.glue/DataQualityResultFilterCriteria
(s/keys
:opt-un
[:cognitect.aws.glue.DataQualityResultFilterCriteria/StartedBefore
:cognitect.aws.glue.DataQualityResultFilterCriteria/JobName
:cognitect.aws.glue.DataQualityResultFilterCriteria/JobRunId
:cognitect.aws.glue.DataQualityResultFilterCriteria/DataSource
:cognitect.aws.glue.DataQualityResultFilterCriteria/StartedAfter]))
(s/def
:cognitect.aws.glue/ConnectionType
(s/spec
string?
:gen
#(s/gen #{"CUSTOM" "KAFKA" "NETWORK" "JDBC" "SFTP" "MONGODB" "MARKETPLACE"})))
(s/def
:cognitect.aws.glue/ListWorkflowsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListWorkflowsResponse/NextToken
:cognitect.aws.glue.ListWorkflowsResponse/Workflows]))
(s/def
:cognitect.aws.glue/FilterString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/LabelingSetGenerationTaskRunProperties
(s/keys :opt-un [:cognitect.aws.glue.LabelingSetGenerationTaskRunProperties/OutputS3Path]))
(s/def :cognitect.aws.glue/ErrorMessageString string?)
(s/def
:cognitect.aws.glue/DeleteDevEndpointRequest
(s/keys :req-un [:cognitect.aws.glue.DeleteDevEndpointRequest/EndpointName]))
(s/def
:cognitect.aws.glue/RoleArn
(s/spec #(re-matches (re-pattern "arn:aws:iam::\\d{12}:role/.*") %) :gen #(gen/string)))
(s/def :cognitect.aws.glue/FilterLogicalOperator (s/spec string? :gen #(s/gen #{"AND" "OR"})))
(s/def
:cognitect.aws.glue/ErrorByName
(s/map-of :cognitect.aws.glue/NameString :cognitect.aws.glue/ErrorDetail))
(s/def :cognitect.aws.glue/UntagResourceResponse (s/keys))
(s/def
:cognitect.aws.glue/GetDataCatalogEncryptionSettingsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetDataCatalogEncryptionSettingsResponse/DataCatalogEncryptionSettings]))
(s/def
:cognitect.aws.glue/TriggerNodeDetails
(s/keys :opt-un [:cognitect.aws.glue.TriggerNodeDetails/Trigger]))
(s/def
:cognitect.aws.glue/TableTypeString
(s/spec
(s/and string? #(>= 255 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 255) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/UserDefinedFunctionInput
(s/keys
:opt-un
[:cognitect.aws.glue.UserDefinedFunctionInput/OwnerName
:cognitect.aws.glue.UserDefinedFunctionInput/FunctionName
:cognitect.aws.glue.UserDefinedFunctionInput/ResourceUris
:cognitect.aws.glue.UserDefinedFunctionInput/OwnerType
:cognitect.aws.glue.UserDefinedFunctionInput/ClassName]))
(s/def
:cognitect.aws.glue/S3EncryptionMode
(s/spec string? :gen #(s/gen #{"DISABLED" "SSE-KMS" "SSE-S3"})))
(s/def
:cognitect.aws.glue/GetJobRequest
(s/keys :req-un [:cognitect.aws.glue.GetJobRequest/JobName]))
(s/def
:cognitect.aws.glue/StopCrawlerScheduleRequest
(s/keys :req-un [:cognitect.aws.glue.StopCrawlerScheduleRequest/CrawlerName]))
(s/def
:cognitect.aws.glue/DataQualityRuleRecommendationRunList
(s/coll-of :cognitect.aws.glue/DataQualityRuleRecommendationRunDescription))
(s/def :cognitect.aws.glue/EdgeList (s/coll-of :cognitect.aws.glue/Edge))
(s/def :cognitect.aws.glue/PermissionList (s/coll-of :cognitect.aws.glue/Permission))
(s/def :cognitect.aws.glue/CreateConnectionResponse (s/keys))
(s/def
:cognitect.aws.glue/IntegerValue
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/TableVersionError
(s/keys
:opt-un
[:cognitect.aws.glue.TableVersionError/VersionId
:cognitect.aws.glue.TableVersionError/ErrorDetail
:cognitect.aws.glue.TableVersionError/TableName]))
(s/def
:cognitect.aws.glue/GetMLTransformsResponse
(s/keys
:req-un
[:cognitect.aws.glue.GetMLTransformsResponse/Transforms]
:opt-un
[:cognitect.aws.glue.GetMLTransformsResponse/NextToken]))
(s/def
:cognitect.aws.glue/StartBlueprintRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.StartBlueprintRunRequest/BlueprintName
:cognitect.aws.glue.StartBlueprintRunRequest/RoleArn]
:opt-un
[:cognitect.aws.glue.StartBlueprintRunRequest/Parameters]))
(s/def
:cognitect.aws.glue/CreateCustomEntityTypeRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateCustomEntityTypeRequest/Name
:cognitect.aws.glue.CreateCustomEntityTypeRequest/RegexString]
:opt-un
[:cognitect.aws.glue.CreateCustomEntityTypeRequest/Tags
:cognitect.aws.glue.CreateCustomEntityTypeRequest/ContextWords]))
(s/def
:cognitect.aws.glue/GetResourcePoliciesResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetResourcePoliciesResponse/NextToken
:cognitect.aws.glue.GetResourcePoliciesResponse/GetResourcePoliciesResponseList]))
(s/def :cognitect.aws.glue/CreateTableResponse (s/keys))
(s/def
:cognitect.aws.glue/ResumeWorkflowRunResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ResumeWorkflowRunResponse/RunId
:cognitect.aws.glue.ResumeWorkflowRunResponse/NodeIds]))
(s/def :cognitect.aws.glue/RegistryStatus (s/spec string? :gen #(s/gen #{"AVAILABLE" "DELETING"})))
(s/def
:cognitect.aws.glue/FilterOperation
(s/spec string? :gen #(s/gen #{"LTE" "ISNULL" "GTE" "LT" "EQ" "REGEX" "GT"})))
(s/def
:cognitect.aws.glue/GetUnfilteredPartitionMetadataRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetUnfilteredPartitionMetadataRequest/CatalogId
:cognitect.aws.glue.GetUnfilteredPartitionMetadataRequest/DatabaseName
:cognitect.aws.glue.GetUnfilteredPartitionMetadataRequest/TableName
:cognitect.aws.glue.GetUnfilteredPartitionMetadataRequest/PartitionValues
:cognitect.aws.glue.GetUnfilteredPartitionMetadataRequest/SupportedPermissionTypes]
:opt-un
[:cognitect.aws.glue.GetUnfilteredPartitionMetadataRequest/AuditContext]))
(s/def :cognitect.aws.glue/DataFormat (s/spec string? :gen #(s/gen #{"AVRO" "JSON" "PROTOBUF"})))
(s/def
:cognitect.aws.glue/BasicCatalogTarget
(s/keys
:req-un
[:cognitect.aws.glue.BasicCatalogTarget/Name
:cognitect.aws.glue.BasicCatalogTarget/Inputs
:cognitect.aws.glue.BasicCatalogTarget/Database
:cognitect.aws.glue.BasicCatalogTarget/Table]))
(s/def
:cognitect.aws.glue/ColumnStatistics
(s/keys
:req-un
[:cognitect.aws.glue.ColumnStatistics/ColumnName
:cognitect.aws.glue.ColumnStatistics/ColumnType
:cognitect.aws.glue.ColumnStatistics/AnalyzedTime
:cognitect.aws.glue.ColumnStatistics/StatisticsData]))
(s/def
:cognitect.aws.glue/JobBookmarksEncryption
(s/keys
:opt-un
[:cognitect.aws.glue.JobBookmarksEncryption/KmsKeyArn
:cognitect.aws.glue.JobBookmarksEncryption/JobBookmarksEncryptionMode]))
(s/def
:cognitect.aws.glue/Workflow
(s/keys
:opt-un
[:cognitect.aws.glue.Workflow/LastRun
:cognitect.aws.glue.Workflow/LastModifiedOn
:cognitect.aws.glue.Workflow/Graph
:cognitect.aws.glue.Workflow/Description
:cognitect.aws.glue.Workflow/MaxConcurrentRuns
:cognitect.aws.glue.Workflow/BlueprintDetails
:cognitect.aws.glue.Workflow/Name
:cognitect.aws.glue.Workflow/CreatedOn
:cognitect.aws.glue.Workflow/DefaultRunProperties]))
(s/def
:cognitect.aws.glue/LogGroup
(s/spec #(re-matches (re-pattern "[\\.\\-_/#A-Za-z0-9]+") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/Trigger
(s/keys
:opt-un
[:cognitect.aws.glue.Trigger/Type
:cognitect.aws.glue.Trigger/WorkflowName
:cognitect.aws.glue.Trigger/Actions
:cognitect.aws.glue.Trigger/EventBatchingCondition
:cognitect.aws.glue.Trigger/Description
:cognitect.aws.glue.Trigger/State
:cognitect.aws.glue.Trigger/Schedule
:cognitect.aws.glue.Trigger/Name
:cognitect.aws.glue.Trigger/Predicate
:cognitect.aws.glue.Trigger/Id]))
(s/def
:cognitect.aws.glue/KeyString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/SerDeInfo
(s/keys
:opt-un
[:cognitect.aws.glue.SerDeInfo/SerializationLibrary
:cognitect.aws.glue.SerDeInfo/Parameters
:cognitect.aws.glue.SerDeInfo/Name]))
(s/def
:cognitect.aws.glue/GlueRecordType
(s/spec
string?
:gen
#(s/gen
#{"TIMESTAMP" "BIGDECIMAL" "DATE" "DOUBLE" "SHORT" "STRING" "INT" "LONG" "FLOAT" "BYTE"})))
(s/def :cognitect.aws.glue/DeleteColumnStatisticsForTableResponse (s/keys))
(s/def
:cognitect.aws.glue/PrincipalPermissionsList
(s/coll-of :cognitect.aws.glue/PrincipalPermissions))
(s/def
:cognitect.aws.glue/ListDataQualityRulesetsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListDataQualityRulesetsResponse/NextToken
:cognitect.aws.glue.ListDataQualityRulesetsResponse/Rulesets]))
(s/def
:cognitect.aws.glue/UpdateSourceControlFromJobRequest
(s/keys
:opt-un
[:cognitect.aws.glue.UpdateSourceControlFromJobRequest/RepositoryOwner
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/AuthToken
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/AuthStrategy
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/RepositoryName
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/Folder
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/BranchName
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/JobName
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/CommitId
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/Provider]))
(s/def
:cognitect.aws.glue/StartImportLabelsTaskRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.StartImportLabelsTaskRunRequest/TransformId
:cognitect.aws.glue.StartImportLabelsTaskRunRequest/InputS3Path]
:opt-un
[:cognitect.aws.glue.StartImportLabelsTaskRunRequest/ReplaceAllLabels]))
(s/def
:cognitect.aws.glue/Generic512CharString
(s/spec
(s/and string? #(<= 1 (count %) 512))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 512) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/ListCrawlersResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListCrawlersResponse/NextToken
:cognitect.aws.glue.ListCrawlersResponse/CrawlerNames]))
(s/def
:cognitect.aws.glue/DataQualityRulesetEvaluationRunList
(s/coll-of :cognitect.aws.glue/DataQualityRulesetEvaluationRunDescription))
(s/def
:cognitect.aws.glue/EnclosedInStringPropertiesMinOne
(s/coll-of :cognitect.aws.glue/EnclosedInStringProperty))
(s/def :cognitect.aws.glue/DeletePartitionIndexResponse (s/keys))
(s/def
:cognitect.aws.glue/StartJobRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.StartJobRunRequest/JobName]
:opt-un
[:cognitect.aws.glue.StartJobRunRequest/AllocatedCapacity
:cognitect.aws.glue.StartJobRunRequest/Timeout
:cognitect.aws.glue.StartJobRunRequest/MaxCapacity
:cognitect.aws.glue.StartJobRunRequest/Arguments
:cognitect.aws.glue.StartJobRunRequest/ExecutionClass
:cognitect.aws.glue.StartJobRunRequest/NumberOfWorkers
:cognitect.aws.glue.StartJobRunRequest/WorkerType
:cognitect.aws.glue.StartJobRunRequest/JobRunId
:cognitect.aws.glue.StartJobRunRequest/SecurityConfiguration
:cognitect.aws.glue.StartJobRunRequest/NotificationProperty]))
(s/def :cognitect.aws.glue/GlueSchemas (s/coll-of :cognitect.aws.glue/GlueSchema))
(s/def
:cognitect.aws.glue/GetTagsRequest
(s/keys :req-un [:cognitect.aws.glue.GetTagsRequest/ResourceArn]))
(s/def
:cognitect.aws.glue/BatchStopJobRunJobRunIdList
(s/coll-of :cognitect.aws.glue/IdString :min-count 1 :max-count 25))
(s/def
:cognitect.aws.glue/S3CatalogHudiSource
(s/keys
:req-un
[:cognitect.aws.glue.S3CatalogHudiSource/Name
:cognitect.aws.glue.S3CatalogHudiSource/Database
:cognitect.aws.glue.S3CatalogHudiSource/Table]
:opt-un
[:cognitect.aws.glue.S3CatalogHudiSource/OutputSchemas
:cognitect.aws.glue.S3CatalogHudiSource/AdditionalHudiOptions]))
(s/def :cognitect.aws.glue/ManyInputs (s/coll-of :cognitect.aws.glue/NodeId :min-count 1))
(s/def
:cognitect.aws.glue/ImportLabelsTaskRunProperties
(s/keys
:opt-un
[:cognitect.aws.glue.ImportLabelsTaskRunProperties/InputS3Path
:cognitect.aws.glue.ImportLabelsTaskRunProperties/Replace]))
(s/def
:cognitect.aws.glue/JdbcTarget
(s/keys
:opt-un
[:cognitect.aws.glue.JdbcTarget/Path
:cognitect.aws.glue.JdbcTarget/ConnectionName
:cognitect.aws.glue.JdbcTarget/EnableAdditionalMetadata
:cognitect.aws.glue.JdbcTarget/Exclusions]))
(s/def
:cognitect.aws.glue/TaskRunProperties
(s/keys
:opt-un
[:cognitect.aws.glue.TaskRunProperties/FindMatchesTaskRunProperties
:cognitect.aws.glue.TaskRunProperties/ImportLabelsTaskRunProperties
:cognitect.aws.glue.TaskRunProperties/TaskType
:cognitect.aws.glue.TaskRunProperties/LabelingSetGenerationTaskRunProperties
:cognitect.aws.glue.TaskRunProperties/ExportLabelsTaskRunProperties]))
(s/def
:cognitect.aws.glue/PostgreSQLCatalogTarget
(s/keys
:req-un
[:cognitect.aws.glue.PostgreSQLCatalogTarget/Name
:cognitect.aws.glue.PostgreSQLCatalogTarget/Inputs
:cognitect.aws.glue.PostgreSQLCatalogTarget/Database
:cognitect.aws.glue.PostgreSQLCatalogTarget/Table]))
(s/def
:cognitect.aws.glue/MySQLCatalogSource
(s/keys
:req-un
[:cognitect.aws.glue.MySQLCatalogSource/Name
:cognitect.aws.glue.MySQLCatalogSource/Database
:cognitect.aws.glue.MySQLCatalogSource/Table]))
(s/def
:cognitect.aws.glue/StartImportLabelsTaskRunResponse
(s/keys :opt-un [:cognitect.aws.glue.StartImportLabelsTaskRunResponse/TaskRunId]))
(s/def
:cognitect.aws.glue/UpdateJobResponse
(s/keys :opt-un [:cognitect.aws.glue.UpdateJobResponse/JobName]))
(s/def
:cognitect.aws.glue/CsvHeaderOption
(s/spec string? :gen #(s/gen #{"UNKNOWN" "ABSENT" "PRESENT"})))
(s/def
:cognitect.aws.glue/ViewTextString
(s/spec
(s/and string? #(>= 409600 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 409600) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/UpdateColumnStatisticsForPartitionRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateColumnStatisticsForPartitionRequest/DatabaseName
:cognitect.aws.glue.UpdateColumnStatisticsForPartitionRequest/TableName
:cognitect.aws.glue.UpdateColumnStatisticsForPartitionRequest/PartitionValues
:cognitect.aws.glue.UpdateColumnStatisticsForPartitionRequest/ColumnStatisticsList]
:opt-un
[:cognitect.aws.glue.UpdateColumnStatisticsForPartitionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/FilterOperator
(s/spec string? :gen #(s/gen #{"NE" "LT" "GE" "LE" "EQ" "GT"})))
(s/def
:cognitect.aws.glue/URI
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\r\\n\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/Spigot
(s/keys
:req-un
[:cognitect.aws.glue.Spigot/Name
:cognitect.aws.glue.Spigot/Inputs
:cognitect.aws.glue.Spigot/Path]
:opt-un
[:cognitect.aws.glue.Spigot/Prob :cognitect.aws.glue.Spigot/Topk]))
(s/def
:cognitect.aws.glue/S3Target
(s/keys
:opt-un
[:cognitect.aws.glue.S3Target/EventQueueArn
:cognitect.aws.glue.S3Target/Path
:cognitect.aws.glue.S3Target/ConnectionName
:cognitect.aws.glue.S3Target/SampleSize
:cognitect.aws.glue.S3Target/DlqEventQueueArn
:cognitect.aws.glue.S3Target/Exclusions]))
(s/def
:cognitect.aws.glue/DeleteColumnStatisticsForTableRequest
(s/keys
:req-un
[:cognitect.aws.glue.DeleteColumnStatisticsForTableRequest/DatabaseName
:cognitect.aws.glue.DeleteColumnStatisticsForTableRequest/TableName
:cognitect.aws.glue.DeleteColumnStatisticsForTableRequest/ColumnName]
:opt-un
[:cognitect.aws.glue.DeleteColumnStatisticsForTableRequest/CatalogId]))
(s/def
:cognitect.aws.glue/OneInput
(s/coll-of :cognitect.aws.glue/NodeId :min-count 1 :max-count 1))
(s/def :cognitect.aws.glue/ExecutionClass (s/spec string? :gen #(s/gen #{"FLEX" "STANDARD"})))
(s/def
:cognitect.aws.glue/CreateGrokClassifierRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateGrokClassifierRequest/Classification
:cognitect.aws.glue.CreateGrokClassifierRequest/Name
:cognitect.aws.glue.CreateGrokClassifierRequest/GrokPattern]
:opt-un
[:cognitect.aws.glue.CreateGrokClassifierRequest/CustomPatterns]))
(s/def
:cognitect.aws.glue/JsonClassifier
(s/keys
:req-un
[:cognitect.aws.glue.JsonClassifier/Name :cognitect.aws.glue.JsonClassifier/JsonPath]
:opt-un
[:cognitect.aws.glue.JsonClassifier/CreationTime
:cognitect.aws.glue.JsonClassifier/Version
:cognitect.aws.glue.JsonClassifier/LastUpdated]))
(s/def
:cognitect.aws.glue/DeleteRegistryInput
(s/keys :req-un [:cognitect.aws.glue.DeleteRegistryInput/RegistryId]))
(s/def
:cognitect.aws.glue/ColumnError
(s/keys
:opt-un
[:cognitect.aws.glue.ColumnError/Error :cognitect.aws.glue.ColumnError/ColumnName]))
(s/def
:cognitect.aws.glue/GetBlueprintRunResponse
(s/keys :opt-un [:cognitect.aws.glue.GetBlueprintRunResponse/BlueprintRun]))
(s/def
:cognitect.aws.glue/UpdateBlueprintResponse
(s/keys :opt-un [:cognitect.aws.glue.UpdateBlueprintResponse/Name]))
(s/def
:cognitect.aws.glue/OrchestrationIAMRoleArn
(s/spec #(re-matches (re-pattern "arn:aws[^:]*:iam::[0-9]*:role/.+") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/GetCrawlerMetricsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.GetCrawlerMetricsRequest/NextToken
:cognitect.aws.glue.GetCrawlerMetricsRequest/CrawlerNameList
:cognitect.aws.glue.GetCrawlerMetricsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/GetDatabaseResponse
(s/keys :opt-un [:cognitect.aws.glue.GetDatabaseResponse/Database]))
(s/def
:cognitect.aws.glue/CreateSecurityConfigurationResponse
(s/keys
:opt-un
[:cognitect.aws.glue.CreateSecurityConfigurationResponse/CreatedTimestamp
:cognitect.aws.glue.CreateSecurityConfigurationResponse/Name]))
(s/def
:cognitect.aws.glue/BatchDeleteTableVersionResponse
(s/keys :opt-un [:cognitect.aws.glue.BatchDeleteTableVersionResponse/Errors]))
(s/def
:cognitect.aws.glue/CreateConnectionRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateConnectionRequest/ConnectionInput]
:opt-un
[:cognitect.aws.glue.CreateConnectionRequest/Tags
:cognitect.aws.glue.CreateConnectionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/CsvClassifier
(s/keys
:req-un
[:cognitect.aws.glue.CsvClassifier/Name]
:opt-un
[:cognitect.aws.glue.CsvClassifier/Header
:cognitect.aws.glue.CsvClassifier/CustomDatatypeConfigured
:cognitect.aws.glue.CsvClassifier/CustomDatatypes
:cognitect.aws.glue.CsvClassifier/CreationTime
:cognitect.aws.glue.CsvClassifier/Delimiter
:cognitect.aws.glue.CsvClassifier/QuoteSymbol
:cognitect.aws.glue.CsvClassifier/Version
:cognitect.aws.glue.CsvClassifier/AllowSingleColumn
:cognitect.aws.glue.CsvClassifier/DisableValueTrimming
:cognitect.aws.glue.CsvClassifier/ContainsHeader
:cognitect.aws.glue.CsvClassifier/LastUpdated]))
(s/def
:cognitect.aws.glue/BatchGetTriggersResponse
(s/keys
:opt-un
[:cognitect.aws.glue.BatchGetTriggersResponse/TriggersNotFound
:cognitect.aws.glue.BatchGetTriggersResponse/Triggers]))
(s/def
:cognitect.aws.glue/BatchCreatePartitionRequest
(s/keys
:req-un
[:cognitect.aws.glue.BatchCreatePartitionRequest/DatabaseName
:cognitect.aws.glue.BatchCreatePartitionRequest/TableName
:cognitect.aws.glue.BatchCreatePartitionRequest/PartitionInputList]
:opt-un
[:cognitect.aws.glue.BatchCreatePartitionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/SchemaId
(s/keys
:opt-un
[:cognitect.aws.glue.SchemaId/RegistryName
:cognitect.aws.glue.SchemaId/SchemaName
:cognitect.aws.glue.SchemaId/SchemaArn]))
(s/def
:cognitect.aws.glue/CheckSchemaVersionValidityResponse
(s/keys
:opt-un
[:cognitect.aws.glue.CheckSchemaVersionValidityResponse/Error
:cognitect.aws.glue.CheckSchemaVersionValidityResponse/Valid]))
(s/def
:cognitect.aws.glue/DataQualityRulesetListDetails
(s/keys
:opt-un
[:cognitect.aws.glue.DataQualityRulesetListDetails/TargetTable
:cognitect.aws.glue.DataQualityRulesetListDetails/LastModifiedOn
:cognitect.aws.glue.DataQualityRulesetListDetails/RuleCount
:cognitect.aws.glue.DataQualityRulesetListDetails/Description
:cognitect.aws.glue.DataQualityRulesetListDetails/RecommendationRunId
:cognitect.aws.glue.DataQualityRulesetListDetails/Name
:cognitect.aws.glue.DataQualityRulesetListDetails/CreatedOn]))
(s/def
:cognitect.aws.glue/BlueprintParameters
(s/spec
(s/and string? #(<= 1 (count %) 131072))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 131072) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/BatchUpdatePartitionRequest
(s/keys
:req-un
[:cognitect.aws.glue.BatchUpdatePartitionRequest/DatabaseName
:cognitect.aws.glue.BatchUpdatePartitionRequest/TableName
:cognitect.aws.glue.BatchUpdatePartitionRequest/Entries]
:opt-un
[:cognitect.aws.glue.BatchUpdatePartitionRequest/CatalogId]))
(s/def :cognitect.aws.glue/CrawlList (s/coll-of :cognitect.aws.glue/Crawl))
(s/def
:cognitect.aws.glue/BlueprintRunState
(s/spec string? :gen #(s/gen #{"ROLLING_BACK" "SUCCEEDED" "FAILED" "RUNNING"})))
(s/def
:cognitect.aws.glue/FederationIdentifier
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def :cognitect.aws.glue/S3EncryptionList (s/coll-of :cognitect.aws.glue/S3Encryption))
(s/def
:cognitect.aws.glue/CreateTableRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateTableRequest/DatabaseName
:cognitect.aws.glue.CreateTableRequest/TableInput]
:opt-un
[:cognitect.aws.glue.CreateTableRequest/OpenTableFormatInput
:cognitect.aws.glue.CreateTableRequest/TransactionId
:cognitect.aws.glue.CreateTableRequest/PartitionIndexes
:cognitect.aws.glue.CreateTableRequest/CatalogId]))
(s/def
:cognitect.aws.glue/GetCustomEntityTypeResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetCustomEntityTypeResponse/RegexString
:cognitect.aws.glue.GetCustomEntityTypeResponse/ContextWords
:cognitect.aws.glue.GetCustomEntityTypeResponse/Name]))
(s/def :cognitect.aws.glue/MetadataList (s/coll-of :cognitect.aws.glue/MetadataKeyValuePair))
(s/def
:cognitect.aws.glue/BatchUpdatePartitionFailureEntry
(s/keys
:opt-un
[:cognitect.aws.glue.BatchUpdatePartitionFailureEntry/ErrorDetail
:cognitect.aws.glue.BatchUpdatePartitionFailureEntry/PartitionValueList]))
(s/def
:cognitect.aws.glue/BatchDeletePartitionResponse
(s/keys :opt-un [:cognitect.aws.glue.BatchDeletePartitionResponse/Errors]))
(s/def :cognitect.aws.glue/DevEndpointNameList (s/coll-of :cognitect.aws.glue/NameString))
(s/def
:cognitect.aws.glue/PartitionIndexList
(s/coll-of :cognitect.aws.glue/PartitionIndex :max-count 3))
(s/def
:cognitect.aws.glue/GetDataQualityRuleRecommendationRunRequest
(s/keys :req-un [:cognitect.aws.glue.GetDataQualityRuleRecommendationRunRequest/RunId]))
(s/def :cognitect.aws.glue/RoleString string?)
(s/def
:cognitect.aws.glue/TransformStatusType
(s/spec string? :gen #(s/gen #{"READY" "DELETING" "NOT_READY"})))
(s/def :cognitect.aws.glue/PythonScript string?)
(s/def :cognitect.aws.glue/TableList (s/coll-of :cognitect.aws.glue/Table))
(s/def
:cognitect.aws.glue/UpdateDataQualityRulesetResponse
(s/keys
:opt-un
[:cognitect.aws.glue.UpdateDataQualityRulesetResponse/Description
:cognitect.aws.glue.UpdateDataQualityRulesetResponse/Ruleset
:cognitect.aws.glue.UpdateDataQualityRulesetResponse/Name]))
(s/def
:cognitect.aws.glue/PropertyPredicate
(s/keys
:opt-un
[:cognitect.aws.glue.PropertyPredicate/Comparator
:cognitect.aws.glue.PropertyPredicate/Key
:cognitect.aws.glue.PropertyPredicate/Value]))
(s/def
:cognitect.aws.glue/DropDuplicates
(s/keys
:req-un
[:cognitect.aws.glue.DropDuplicates/Name :cognitect.aws.glue.DropDuplicates/Inputs]
:opt-un
[:cognitect.aws.glue.DropDuplicates/Columns]))
(s/def
:cognitect.aws.glue/StartTriggerResponse
(s/keys :opt-un [:cognitect.aws.glue.StartTriggerResponse/Name]))
(s/def :cognitect.aws.glue/EnableHybridValues (s/spec string? :gen #(s/gen #{"TRUE" "FALSE"})))
(s/def
:cognitect.aws.glue/UpdateTriggerRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateTriggerRequest/Name
:cognitect.aws.glue.UpdateTriggerRequest/TriggerUpdate]))
(s/def
:cognitect.aws.glue/Join
(s/keys
:req-un
[:cognitect.aws.glue.Join/Name
:cognitect.aws.glue.Join/Inputs
:cognitect.aws.glue.Join/JoinType
:cognitect.aws.glue.Join/Columns]))
(s/def
:cognitect.aws.glue/GetBlueprintRunsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetBlueprintRunsResponse/NextToken
:cognitect.aws.glue.GetBlueprintRunsResponse/BlueprintRuns]))
(s/def
:cognitect.aws.glue/SelectFields
(s/keys
:req-un
[:cognitect.aws.glue.SelectFields/Name
:cognitect.aws.glue.SelectFields/Inputs
:cognitect.aws.glue.SelectFields/Paths]))
(s/def
:cognitect.aws.glue/CloudWatchEncryption
(s/keys
:opt-un
[:cognitect.aws.glue.CloudWatchEncryption/KmsKeyArn
:cognitect.aws.glue.CloudWatchEncryption/CloudWatchEncryptionMode]))
(s/def
:cognitect.aws.glue/DQDLAliases
(s/map-of :cognitect.aws.glue/NodeName :cognitect.aws.glue/EnclosedInStringProperty))
(s/def :cognitect.aws.glue/BooleanNullable boolean?)
(s/def
:cognitect.aws.glue/ListStatementsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListStatementsResponse/NextToken
:cognitect.aws.glue.ListStatementsResponse/Statements]))
(s/def
:cognitect.aws.glue/ApplyMapping
(s/keys
:req-un
[:cognitect.aws.glue.ApplyMapping/Name
:cognitect.aws.glue.ApplyMapping/Inputs
:cognitect.aws.glue.ApplyMapping/Mapping]))
(s/def :cognitect.aws.glue/UpdatedTimestamp string?)
(s/def
:cognitect.aws.glue/RelationalCatalogSource
(s/keys
:req-un
[:cognitect.aws.glue.RelationalCatalogSource/Name
:cognitect.aws.glue.RelationalCatalogSource/Database
:cognitect.aws.glue.RelationalCatalogSource/Table]))
(s/def
:cognitect.aws.glue/NullValueField
(s/keys
:req-un
[:cognitect.aws.glue.NullValueField/Value :cognitect.aws.glue.NullValueField/Datatype]))
(s/def :cognitect.aws.glue/DeleteDevEndpointResponse (s/keys))
(s/def
:cognitect.aws.glue/MappingEntry
(s/keys
:opt-un
[:cognitect.aws.glue.MappingEntry/TargetTable
:cognitect.aws.glue.MappingEntry/TargetType
:cognitect.aws.glue.MappingEntry/SourceType
:cognitect.aws.glue.MappingEntry/SourceTable
:cognitect.aws.glue.MappingEntry/TargetPath
:cognitect.aws.glue.MappingEntry/SourcePath]))
(s/def
:cognitect.aws.glue/GetDataQualityResultRequest
(s/keys :req-un [:cognitect.aws.glue.GetDataQualityResultRequest/ResultId]))
(s/def
:cognitect.aws.glue/DQAdditionalOptions
(s/map-of :cognitect.aws.glue/AdditionalOptionKeys :cognitect.aws.glue/GenericString))
(s/def
:cognitect.aws.glue/GetMLTaskRunsRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetMLTaskRunsRequest/TransformId]
:opt-un
[:cognitect.aws.glue.GetMLTaskRunsRequest/Sort
:cognitect.aws.glue.GetMLTaskRunsRequest/Filter
:cognitect.aws.glue.GetMLTaskRunsRequest/NextToken
:cognitect.aws.glue.GetMLTaskRunsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/GetSchemaVersionInput
(s/keys
:opt-un
[:cognitect.aws.glue.GetSchemaVersionInput/SchemaVersionNumber
:cognitect.aws.glue.GetSchemaVersionInput/SchemaId
:cognitect.aws.glue.GetSchemaVersionInput/SchemaVersionId]))
(s/def
:cognitect.aws.glue/DataQualityRuleRecommendationRunFilter
(s/keys
:req-un
[:cognitect.aws.glue.DataQualityRuleRecommendationRunFilter/DataSource]
:opt-un
[:cognitect.aws.glue.DataQualityRuleRecommendationRunFilter/StartedBefore
:cognitect.aws.glue.DataQualityRuleRecommendationRunFilter/StartedAfter]))
(s/def
:cognitect.aws.glue/BlueprintParameterSpec
(s/spec
(s/and string? #(<= 1 (count %) 131072))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 131072) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/Union
(s/keys
:req-un
[:cognitect.aws.glue.Union/Name
:cognitect.aws.glue.Union/Inputs
:cognitect.aws.glue.Union/UnionType]))
(s/def
:cognitect.aws.glue/CatalogEncryptionMode
(s/spec string? :gen #(s/gen #{"DISABLED" "SSE-KMS"})))
(s/def
:cognitect.aws.glue/GetColumnStatisticsForPartitionRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetColumnStatisticsForPartitionRequest/DatabaseName
:cognitect.aws.glue.GetColumnStatisticsForPartitionRequest/TableName
:cognitect.aws.glue.GetColumnStatisticsForPartitionRequest/PartitionValues
:cognitect.aws.glue.GetColumnStatisticsForPartitionRequest/ColumnNames]
:opt-un
[:cognitect.aws.glue.GetColumnStatisticsForPartitionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/CloudWatchEncryptionMode
(s/spec string? :gen #(s/gen #{"DISABLED" "SSE-KMS"})))
(s/def
:cognitect.aws.glue/DataQualityRuleRecommendationRunDescription
(s/keys
:opt-un
[:cognitect.aws.glue.DataQualityRuleRecommendationRunDescription/RunId
:cognitect.aws.glue.DataQualityRuleRecommendationRunDescription/Status
:cognitect.aws.glue.DataQualityRuleRecommendationRunDescription/DataSource
:cognitect.aws.glue.DataQualityRuleRecommendationRunDescription/StartedOn]))
(s/def
:cognitect.aws.glue/RegistryListItem
(s/keys
:opt-un
[:cognitect.aws.glue.RegistryListItem/RegistryArn
:cognitect.aws.glue.RegistryListItem/Status
:cognitect.aws.glue.RegistryListItem/CreatedTime
:cognitect.aws.glue.RegistryListItem/Description
:cognitect.aws.glue.RegistryListItem/UpdatedTime
:cognitect.aws.glue.RegistryListItem/RegistryName]))
(s/def
:cognitect.aws.glue/EnclosedInStringProperties
(s/coll-of :cognitect.aws.glue/EnclosedInStringProperty))
(s/def
:cognitect.aws.glue/MessagePrefix
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/ListDataQualityRuleRecommendationRunsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.ListDataQualityRuleRecommendationRunsRequest/Filter
:cognitect.aws.glue.ListDataQualityRuleRecommendationRunsRequest/NextToken
:cognitect.aws.glue.ListDataQualityRuleRecommendationRunsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/CrawlerMetrics
(s/keys
:opt-un
[:cognitect.aws.glue.CrawlerMetrics/TablesCreated
:cognitect.aws.glue.CrawlerMetrics/MedianRuntimeSeconds
:cognitect.aws.glue.CrawlerMetrics/CrawlerName
:cognitect.aws.glue.CrawlerMetrics/LastRuntimeSeconds
:cognitect.aws.glue.CrawlerMetrics/TablesDeleted
:cognitect.aws.glue.CrawlerMetrics/TimeLeftSeconds
:cognitect.aws.glue.CrawlerMetrics/TablesUpdated
:cognitect.aws.glue.CrawlerMetrics/StillEstimating]))
(s/def
:cognitect.aws.glue/Segment
(s/keys
:req-un
[:cognitect.aws.glue.Segment/SegmentNumber :cognitect.aws.glue.Segment/TotalSegments]))
(s/def
:cognitect.aws.glue/JobRunState
(s/spec
string?
:gen
#(s/gen
#{"TIMEOUT"
"ERROR"
"SUCCEEDED"
"STOPPED"
"STARTING"
"WAITING"
"FAILED"
"STOPPING"
"RUNNING"})))
(s/def
:cognitect.aws.glue/PartitionIndexDescriptorList
(s/coll-of :cognitect.aws.glue/PartitionIndexDescriptor))
(s/def
:cognitect.aws.glue/ListStatementsRequest
(s/keys
:req-un
[:cognitect.aws.glue.ListStatementsRequest/SessionId]
:opt-un
[:cognitect.aws.glue.ListStatementsRequest/NextToken
:cognitect.aws.glue.ListStatementsRequest/RequestOrigin]))
(s/def :cognitect.aws.glue/ConnectionList (s/coll-of :cognitect.aws.glue/Connection))
(s/def
:cognitect.aws.glue/GetPartitionResponse
(s/keys :opt-un [:cognitect.aws.glue.GetPartitionResponse/Partition]))
(s/def
:cognitect.aws.glue/UpdateWorkflowRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateWorkflowRequest/Name]
:opt-un
[:cognitect.aws.glue.UpdateWorkflowRequest/Description
:cognitect.aws.glue.UpdateWorkflowRequest/MaxConcurrentRuns
:cognitect.aws.glue.UpdateWorkflowRequest/DefaultRunProperties]))
(s/def
:cognitect.aws.glue/BatchGetBlueprintsRequest
(s/keys
:req-un
[:cognitect.aws.glue.BatchGetBlueprintsRequest/Names]
:opt-un
[:cognitect.aws.glue.BatchGetBlueprintsRequest/IncludeParameterSpec
:cognitect.aws.glue.BatchGetBlueprintsRequest/IncludeBlueprint]))
(s/def
:cognitect.aws.glue/PutWorkflowRunPropertiesRequest
(s/keys
:req-un
[:cognitect.aws.glue.PutWorkflowRunPropertiesRequest/Name
:cognitect.aws.glue.PutWorkflowRunPropertiesRequest/RunId
:cognitect.aws.glue.PutWorkflowRunPropertiesRequest/RunProperties]))
(s/def
:cognitect.aws.glue/DynamicTransform
(s/keys
:req-un
[:cognitect.aws.glue.DynamicTransform/Name
:cognitect.aws.glue.DynamicTransform/TransformName
:cognitect.aws.glue.DynamicTransform/Inputs
:cognitect.aws.glue.DynamicTransform/FunctionName
:cognitect.aws.glue.DynamicTransform/Path]
:opt-un
[:cognitect.aws.glue.DynamicTransform/OutputSchemas
:cognitect.aws.glue.DynamicTransform/Parameters
:cognitect.aws.glue.DynamicTransform/Version]))
(s/def
:cognitect.aws.glue/UpdatePartitionRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdatePartitionRequest/DatabaseName
:cognitect.aws.glue.UpdatePartitionRequest/TableName
:cognitect.aws.glue.UpdatePartitionRequest/PartitionValueList
:cognitect.aws.glue.UpdatePartitionRequest/PartitionInput]
:opt-un
[:cognitect.aws.glue.UpdatePartitionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/DeletePartitionRequest
(s/keys
:req-un
[:cognitect.aws.glue.DeletePartitionRequest/DatabaseName
:cognitect.aws.glue.DeletePartitionRequest/TableName
:cognitect.aws.glue.DeletePartitionRequest/PartitionValues]
:opt-un
[:cognitect.aws.glue.DeletePartitionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/Action
(s/keys
:opt-un
[:cognitect.aws.glue.Action/Timeout
:cognitect.aws.glue.Action/Arguments
:cognitect.aws.glue.Action/JobName
:cognitect.aws.glue.Action/CrawlerName
:cognitect.aws.glue.Action/SecurityConfiguration
:cognitect.aws.glue.Action/NotificationProperty]))
(s/def
:cognitect.aws.glue/GetUserDefinedFunctionsRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetUserDefinedFunctionsRequest/Pattern]
:opt-un
[:cognitect.aws.glue.GetUserDefinedFunctionsRequest/DatabaseName
:cognitect.aws.glue.GetUserDefinedFunctionsRequest/NextToken
:cognitect.aws.glue.GetUserDefinedFunctionsRequest/CatalogId
:cognitect.aws.glue.GetUserDefinedFunctionsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/DataSourceMap
(s/map-of :cognitect.aws.glue/NameString :cognitect.aws.glue/DataSource))
(s/def
:cognitect.aws.glue/EncryptionConfiguration
(s/keys
:opt-un
[:cognitect.aws.glue.EncryptionConfiguration/S3Encryption
:cognitect.aws.glue.EncryptionConfiguration/JobBookmarksEncryption
:cognitect.aws.glue.EncryptionConfiguration/CloudWatchEncryption]))
(s/def :cognitect.aws.glue/Long (s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/ListDevEndpointsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListDevEndpointsResponse/NextToken
:cognitect.aws.glue.ListDevEndpointsResponse/DevEndpointNames]))
(s/def :cognitect.aws.glue/NodeIdList (s/coll-of :cognitect.aws.glue/NameString))
(s/def
:cognitect.aws.glue/DeleteCustomEntityTypeResponse
(s/keys :opt-un [:cognitect.aws.glue.DeleteCustomEntityTypeResponse/Name]))
(s/def
:cognitect.aws.glue/GetBlueprintRunsRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetBlueprintRunsRequest/BlueprintName]
:opt-un
[:cognitect.aws.glue.GetBlueprintRunsRequest/NextToken
:cognitect.aws.glue.GetBlueprintRunsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/ListRegistriesResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListRegistriesResponse/Registries
:cognitect.aws.glue.ListRegistriesResponse/NextToken]))
(s/def
:cognitect.aws.glue/BatchGetCustomEntityTypesResponse
(s/keys
:opt-un
[:cognitect.aws.glue.BatchGetCustomEntityTypesResponse/CustomEntityTypesNotFound
:cognitect.aws.glue.BatchGetCustomEntityTypesResponse/CustomEntityTypes]))
(s/def
:cognitect.aws.glue/PartitionInput
(s/keys
:opt-un
[:cognitect.aws.glue.PartitionInput/Parameters
:cognitect.aws.glue.PartitionInput/StorageDescriptor
:cognitect.aws.glue.PartitionInput/Values
:cognitect.aws.glue.PartitionInput/LastAccessTime
:cognitect.aws.glue.PartitionInput/LastAnalyzedTime]))
(s/def
:cognitect.aws.glue/AuditContextString
(s/spec
(s/and string? #(<= 0 (count %) 2048))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 2048) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/CreateBlueprintResponse
(s/keys :opt-un [:cognitect.aws.glue.CreateBlueprintResponse/Name]))
(s/def
:cognitect.aws.glue/CreateJsonClassifierRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateJsonClassifierRequest/Name
:cognitect.aws.glue.CreateJsonClassifierRequest/JsonPath]))
(s/def
:cognitect.aws.glue/DeleteBlueprintResponse
(s/keys :opt-un [:cognitect.aws.glue.DeleteBlueprintResponse/Name]))
(s/def
:cognitect.aws.glue/GetConnectionResponse
(s/keys :opt-un [:cognitect.aws.glue.GetConnectionResponse/Connection]))
(s/def :cognitect.aws.glue/GenericString string?)
(s/def :cognitect.aws.glue/AuditColumnNamesList (s/coll-of :cognitect.aws.glue/ColumnNameString))
(s/def
:cognitect.aws.glue/PrincipalPermissions
(s/keys
:opt-un
[:cognitect.aws.glue.PrincipalPermissions/Permissions
:cognitect.aws.glue.PrincipalPermissions/Principal]))
(s/def
:cognitect.aws.glue/CustomPatterns
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\r\\n\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/ExecutionProperty
(s/keys :opt-un [:cognitect.aws.glue.ExecutionProperty/MaxConcurrentRuns]))
(s/def
:cognitect.aws.glue/UpdateClassifierRequest
(s/keys
:opt-un
[:cognitect.aws.glue.UpdateClassifierRequest/GrokClassifier
:cognitect.aws.glue.UpdateClassifierRequest/XMLClassifier
:cognitect.aws.glue.UpdateClassifierRequest/JsonClassifier
:cognitect.aws.glue.UpdateClassifierRequest/CsvClassifier]))
(s/def :cognitect.aws.glue/ErrorCodeString string?)
(s/def :cognitect.aws.glue/BoxedBoolean boolean?)
(s/def
:cognitect.aws.glue/AggregateOperation
(s/keys
:req-un
[:cognitect.aws.glue.AggregateOperation/Column :cognitect.aws.glue.AggregateOperation/AggFunc]))
(s/def :cognitect.aws.glue/StopCrawlerResponse (s/keys))
(s/def
:cognitect.aws.glue/MLUserDataEncryption
(s/keys
:req-un
[:cognitect.aws.glue.MLUserDataEncryption/MlUserDataEncryptionMode]
:opt-un
[:cognitect.aws.glue.MLUserDataEncryption/KmsKeyId]))
(s/def :cognitect.aws.glue/CreateDatabaseResponse (s/keys))
(s/def
:cognitect.aws.glue/RedshiftSource
(s/keys
:req-un
[:cognitect.aws.glue.RedshiftSource/Name
:cognitect.aws.glue.RedshiftSource/Database
:cognitect.aws.glue.RedshiftSource/Table]
:opt-un
[:cognitect.aws.glue.RedshiftSource/TmpDirIAMRole
:cognitect.aws.glue.RedshiftSource/RedshiftTmpDir]))
(s/def :cognitect.aws.glue/CreatedTimestamp string?)
(s/def
:cognitect.aws.glue/Column
(s/keys
:req-un
[:cognitect.aws.glue.Column/Name]
:opt-un
[:cognitect.aws.glue.Column/Comment
:cognitect.aws.glue.Column/Type
:cognitect.aws.glue.Column/Parameters]))
(s/def
:cognitect.aws.glue/S3ParquetSource
(s/keys
:req-un
[:cognitect.aws.glue.S3ParquetSource/Name :cognitect.aws.glue.S3ParquetSource/Paths]
:opt-un
[:cognitect.aws.glue.S3ParquetSource/GroupSize
:cognitect.aws.glue.S3ParquetSource/CompressionType
:cognitect.aws.glue.S3ParquetSource/OutputSchemas
:cognitect.aws.glue.S3ParquetSource/Recurse
:cognitect.aws.glue.S3ParquetSource/AdditionalOptions
:cognitect.aws.glue.S3ParquetSource/MaxFilesInBand
:cognitect.aws.glue.S3ParquetSource/Exclusions
:cognitect.aws.glue.S3ParquetSource/MaxBand
:cognitect.aws.glue.S3ParquetSource/GroupFiles]))
(s/def
:cognitect.aws.glue/GetSecurityConfigurationsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetSecurityConfigurationsResponse/NextToken
:cognitect.aws.glue.GetSecurityConfigurationsResponse/SecurityConfigurations]))
(s/def
:cognitect.aws.glue/BoxedNonNegativeLong
(s/spec (s/and int? #(<= 0 %)) :gen #(gen/choose 0 Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/PIIDetection
(s/keys
:req-un
[:cognitect.aws.glue.PIIDetection/Name
:cognitect.aws.glue.PIIDetection/Inputs
:cognitect.aws.glue.PIIDetection/PiiType
:cognitect.aws.glue.PIIDetection/EntityTypesToDetect]
:opt-un
[:cognitect.aws.glue.PIIDetection/ThresholdFraction
:cognitect.aws.glue.PIIDetection/MaskValue
:cognitect.aws.glue.PIIDetection/SampleFraction
:cognitect.aws.glue.PIIDetection/OutputColumnName]))
(s/def
:cognitect.aws.glue/DatabaseInput
(s/keys
:req-un
[:cognitect.aws.glue.DatabaseInput/Name]
:opt-un
[:cognitect.aws.glue.DatabaseInput/FederatedDatabase
:cognitect.aws.glue.DatabaseInput/Parameters
:cognitect.aws.glue.DatabaseInput/Description
:cognitect.aws.glue.DatabaseInput/TargetDatabase
:cognitect.aws.glue.DatabaseInput/LocationUri
:cognitect.aws.glue.DatabaseInput/CreateTableDefaultPermissions]))
(s/def
:cognitect.aws.glue/JDBCConnectorOptions
(s/keys
:opt-un
[:cognitect.aws.glue.JDBCConnectorOptions/NumPartitions
:cognitect.aws.glue.JDBCConnectorOptions/JobBookmarkKeysSortOrder
:cognitect.aws.glue.JDBCConnectorOptions/DataTypeMapping
:cognitect.aws.glue.JDBCConnectorOptions/FilterPredicate
:cognitect.aws.glue.JDBCConnectorOptions/LowerBound
:cognitect.aws.glue.JDBCConnectorOptions/UpperBound
:cognitect.aws.glue.JDBCConnectorOptions/JobBookmarkKeys
:cognitect.aws.glue.JDBCConnectorOptions/PartitionColumn]))
(s/def
:cognitect.aws.glue/UpsertRedshiftTargetOptions
(s/keys
:opt-un
[:cognitect.aws.glue.UpsertRedshiftTargetOptions/UpsertKeys
:cognitect.aws.glue.UpsertRedshiftTargetOptions/ConnectionName
:cognitect.aws.glue.UpsertRedshiftTargetOptions/TableLocation]))
(s/def
:cognitect.aws.glue/SelectFromCollection
(s/keys
:req-un
[:cognitect.aws.glue.SelectFromCollection/Name
:cognitect.aws.glue.SelectFromCollection/Inputs
:cognitect.aws.glue.SelectFromCollection/Index]))
(s/def
:cognitect.aws.glue/S3CatalogTarget
(s/keys
:req-un
[:cognitect.aws.glue.S3CatalogTarget/Name
:cognitect.aws.glue.S3CatalogTarget/Inputs
:cognitect.aws.glue.S3CatalogTarget/Table
:cognitect.aws.glue.S3CatalogTarget/Database]
:opt-un
[:cognitect.aws.glue.S3CatalogTarget/PartitionKeys
:cognitect.aws.glue.S3CatalogTarget/SchemaChangePolicy]))
(s/def
:cognitect.aws.glue/BatchGetPartitionResponse
(s/keys
:opt-un
[:cognitect.aws.glue.BatchGetPartitionResponse/UnprocessedKeys
:cognitect.aws.glue.BatchGetPartitionResponse/Partitions]))
(s/def
:cognitect.aws.glue/OracleSQLCatalogTarget
(s/keys
:req-un
[:cognitect.aws.glue.OracleSQLCatalogTarget/Name
:cognitect.aws.glue.OracleSQLCatalogTarget/Inputs
:cognitect.aws.glue.OracleSQLCatalogTarget/Database
:cognitect.aws.glue.OracleSQLCatalogTarget/Table]))
(s/def :cognitect.aws.glue/JobRunList (s/coll-of :cognitect.aws.glue/JobRun))
(s/def
:cognitect.aws.glue/GetWorkflowRunPropertiesResponse
(s/keys :opt-un [:cognitect.aws.glue.GetWorkflowRunPropertiesResponse/RunProperties]))
(s/def
:cognitect.aws.glue/TablePrefix
(s/spec
(s/and string? #(<= 0 (count %) 128))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 128) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/ImportCatalogToGlueRequest
(s/keys :opt-un [:cognitect.aws.glue.ImportCatalogToGlueRequest/CatalogId]))
(s/def
:cognitect.aws.glue/StartWorkflowRunResponse
(s/keys :opt-un [:cognitect.aws.glue.StartWorkflowRunResponse/RunId]))
(s/def
:cognitect.aws.glue/Merge
(s/keys
:req-un
[:cognitect.aws.glue.Merge/Name
:cognitect.aws.glue.Merge/Inputs
:cognitect.aws.glue.Merge/Source
:cognitect.aws.glue.Merge/PrimaryKeys]))
(s/def :cognitect.aws.glue/TableName string?)
(s/def
:cognitect.aws.glue/DeleteSchemaResponse
(s/keys
:opt-un
[:cognitect.aws.glue.DeleteSchemaResponse/Status
:cognitect.aws.glue.DeleteSchemaResponse/SchemaName
:cognitect.aws.glue.DeleteSchemaResponse/SchemaArn]))
(s/def
:cognitect.aws.glue/S3JsonSource
(s/keys
:req-un
[:cognitect.aws.glue.S3JsonSource/Name :cognitect.aws.glue.S3JsonSource/Paths]
:opt-un
[:cognitect.aws.glue.S3JsonSource/Multiline
:cognitect.aws.glue.S3JsonSource/GroupSize
:cognitect.aws.glue.S3JsonSource/CompressionType
:cognitect.aws.glue.S3JsonSource/OutputSchemas
:cognitect.aws.glue.S3JsonSource/Recurse
:cognitect.aws.glue.S3JsonSource/JsonPath
:cognitect.aws.glue.S3JsonSource/AdditionalOptions
:cognitect.aws.glue.S3JsonSource/MaxFilesInBand
:cognitect.aws.glue.S3JsonSource/Exclusions
:cognitect.aws.glue.S3JsonSource/MaxBand
:cognitect.aws.glue.S3JsonSource/GroupFiles]))
(s/def :cognitect.aws.glue/KeyList (s/coll-of :cognitect.aws.glue/NameString :min-count 1))
(s/def
:cognitect.aws.glue/GetSessionResponse
(s/keys :opt-un [:cognitect.aws.glue.GetSessionResponse/Session]))
(s/def :cognitect.aws.glue/MappingList (s/coll-of :cognitect.aws.glue/MappingEntry))
(s/def
:cognitect.aws.glue/S3DeltaCatalogTarget
(s/keys
:req-un
[:cognitect.aws.glue.S3DeltaCatalogTarget/Name
:cognitect.aws.glue.S3DeltaCatalogTarget/Inputs
:cognitect.aws.glue.S3DeltaCatalogTarget/Table
:cognitect.aws.glue.S3DeltaCatalogTarget/Database]
:opt-un
[:cognitect.aws.glue.S3DeltaCatalogTarget/AdditionalOptions
:cognitect.aws.glue.S3DeltaCatalogTarget/PartitionKeys
:cognitect.aws.glue.S3DeltaCatalogTarget/SchemaChangePolicy]))
(s/def
:cognitect.aws.glue/BatchUpdatePartitionFailureList
(s/coll-of :cognitect.aws.glue/BatchUpdatePartitionFailureEntry))
(s/def
:cognitect.aws.glue/GetDevEndpointsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetDevEndpointsResponse/DevEndpoints
:cognitect.aws.glue.GetDevEndpointsResponse/NextToken]))
(s/def
:cognitect.aws.glue/GetDevEndpointsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.GetDevEndpointsRequest/NextToken
:cognitect.aws.glue.GetDevEndpointsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/GetSchemaByDefinitionResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetSchemaByDefinitionResponse/DataFormat
:cognitect.aws.glue.GetSchemaByDefinitionResponse/Status
:cognitect.aws.glue.GetSchemaByDefinitionResponse/CreatedTime
:cognitect.aws.glue.GetSchemaByDefinitionResponse/SchemaArn
:cognitect.aws.glue.GetSchemaByDefinitionResponse/SchemaVersionId]))
(s/def :cognitect.aws.glue/RowTag string?)
(s/def :cognitect.aws.glue/PageSize (s/spec (s/and int? #(<= 1 % 1000)) :gen #(gen/choose 1 1000)))
(s/def
:cognitect.aws.glue/DeleteTableVersionRequest
(s/keys
:req-un
[:cognitect.aws.glue.DeleteTableVersionRequest/DatabaseName
:cognitect.aws.glue.DeleteTableVersionRequest/TableName
:cognitect.aws.glue.DeleteTableVersionRequest/VersionId]
:opt-un
[:cognitect.aws.glue.DeleteTableVersionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/UntagResourceRequest
(s/keys
:req-un
[:cognitect.aws.glue.UntagResourceRequest/ResourceArn
:cognitect.aws.glue.UntagResourceRequest/TagsToRemove]))
(s/def
:cognitect.aws.glue/BinaryColumnStatisticsData
(s/keys
:req-un
[:cognitect.aws.glue.BinaryColumnStatisticsData/MaximumLength
:cognitect.aws.glue.BinaryColumnStatisticsData/AverageLength
:cognitect.aws.glue.BinaryColumnStatisticsData/NumberOfNulls]))
(s/def
:cognitect.aws.glue/GetTriggersRequest
(s/keys
:opt-un
[:cognitect.aws.glue.GetTriggersRequest/NextToken
:cognitect.aws.glue.GetTriggersRequest/DependentJobName
:cognitect.aws.glue.GetTriggersRequest/MaxResults]))
(s/def
:cognitect.aws.glue/TagKey
(s/spec
(s/and string? #(<= 1 (count %) 128))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 128) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/CrawlerNameList
(s/coll-of :cognitect.aws.glue/NameString :min-count 0 :max-count 100))
(s/def :cognitect.aws.glue/DeleteDataQualityRulesetResponse (s/keys))
(s/def
:cognitect.aws.glue/PartitionValueList
(s/keys :req-un [:cognitect.aws.glue.PartitionValueList/Values]))
(s/def :cognitect.aws.glue/GetJobResponse (s/keys :opt-un [:cognitect.aws.glue.GetJobResponse/Job]))
(s/def
:cognitect.aws.glue/ListSessionsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListSessionsResponse/Ids
:cognitect.aws.glue.ListSessionsResponse/NextToken
:cognitect.aws.glue.ListSessionsResponse/Sessions]))
(s/def :cognitect.aws.glue/NameStringList (s/coll-of :cognitect.aws.glue/NameString))
(s/def
:cognitect.aws.glue/GetSchemaVersionsDiffResponse
(s/keys :opt-un [:cognitect.aws.glue.GetSchemaVersionsDiffResponse/Diff]))
(s/def
:cognitect.aws.glue/PartitionIndexDescriptor
(s/keys
:req-un
[:cognitect.aws.glue.PartitionIndexDescriptor/IndexName
:cognitect.aws.glue.PartitionIndexDescriptor/Keys
:cognitect.aws.glue.PartitionIndexDescriptor/IndexStatus]
:opt-un
[:cognitect.aws.glue.PartitionIndexDescriptor/BackfillErrors]))
(s/def
:cognitect.aws.glue/BooleanColumnStatisticsData
(s/keys
:req-un
[:cognitect.aws.glue.BooleanColumnStatisticsData/NumberOfTrues
:cognitect.aws.glue.BooleanColumnStatisticsData/NumberOfFalses
:cognitect.aws.glue.BooleanColumnStatisticsData/NumberOfNulls]))
(s/def
:cognitect.aws.glue/Blob
(s/or :byte-array bytes? :input-stream #(instance? java.io.InputStream %)))
(s/def
:cognitect.aws.glue/SortCriteria
(s/coll-of :cognitect.aws.glue/SortCriterion :min-count 0 :max-count 1))
(s/def :cognitect.aws.glue/PartitionList (s/coll-of :cognitect.aws.glue/Partition))
(s/def
:cognitect.aws.glue/CodeGenEdge
(s/keys
:req-un
[:cognitect.aws.glue.CodeGenEdge/Source :cognitect.aws.glue.CodeGenEdge/Target]
:opt-un
[:cognitect.aws.glue.CodeGenEdge/TargetParameter]))
(s/def
:cognitect.aws.glue/Node
(s/keys
:opt-un
[:cognitect.aws.glue.Node/JobDetails
:cognitect.aws.glue.Node/Type
:cognitect.aws.glue.Node/UniqueId
:cognitect.aws.glue.Node/Name
:cognitect.aws.glue.Node/CrawlerDetails
:cognitect.aws.glue.Node/TriggerDetails]))
(s/def
:cognitect.aws.glue/BatchDeleteTableVersionList
(s/coll-of :cognitect.aws.glue/VersionString :min-count 0 :max-count 100))
(s/def :cognitect.aws.glue/ColumnList (s/coll-of :cognitect.aws.glue/Column))
(s/def
:cognitect.aws.glue/ListTriggersResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListTriggersResponse/NextToken
:cognitect.aws.glue.ListTriggersResponse/TriggerNames]))
(s/def
:cognitect.aws.glue/AdditionalPlanOptionsMap
(s/map-of :cognitect.aws.glue/GenericString :cognitect.aws.glue/GenericString))
(s/def
:cognitect.aws.glue/CreateTriggerResponse
(s/keys :opt-un [:cognitect.aws.glue.CreateTriggerResponse/Name]))
(s/def
:cognitect.aws.glue/DataQualityResultIdList
(s/coll-of :cognitect.aws.glue/HashString :min-count 1 :max-count 10))
(s/def
:cognitect.aws.glue/DQStopJobOnFailureOptions
(s/keys :opt-un [:cognitect.aws.glue.DQStopJobOnFailureOptions/StopJobOnFailureTiming]))
(s/def :cognitect.aws.glue/MessageString string?)
(s/def
:cognitect.aws.glue/S3CatalogDeltaSource
(s/keys
:req-un
[:cognitect.aws.glue.S3CatalogDeltaSource/Name
:cognitect.aws.glue.S3CatalogDeltaSource/Database
:cognitect.aws.glue.S3CatalogDeltaSource/Table]
:opt-un
[:cognitect.aws.glue.S3CatalogDeltaSource/OutputSchemas
:cognitect.aws.glue.S3CatalogDeltaSource/AdditionalDeltaOptions]))
(s/def
:cognitect.aws.glue/GetSecurityConfigurationRequest
(s/keys :req-un [:cognitect.aws.glue.GetSecurityConfigurationRequest/Name]))
(s/def
:cognitect.aws.glue/DataQualityEvaluationRunAdditionalRunOptions
(s/keys
:opt-un
[:cognitect.aws.glue.DataQualityEvaluationRunAdditionalRunOptions/ResultsS3Prefix
:cognitect.aws.glue.DataQualityEvaluationRunAdditionalRunOptions/CloudWatchMetricsEnabled]))
(s/def
:cognitect.aws.glue/GetDatabaseRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetDatabaseRequest/Name]
:opt-un
[:cognitect.aws.glue.GetDatabaseRequest/CatalogId]))
(s/def
:cognitect.aws.glue/StartMLLabelingSetGenerationTaskRunResponse
(s/keys :opt-un [:cognitect.aws.glue.StartMLLabelingSetGenerationTaskRunResponse/TaskRunId]))
(s/def
:cognitect.aws.glue/OrchestrationNameString
(s/spec #(re-matches (re-pattern "[\\.\\-_A-Za-z0-9]+") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/GetRegistryInput
(s/keys :req-un [:cognitect.aws.glue.GetRegistryInput/RegistryId]))
(s/def
:cognitect.aws.glue/UpdateSchemaResponse
(s/keys
:opt-un
[:cognitect.aws.glue.UpdateSchemaResponse/RegistryName
:cognitect.aws.glue.UpdateSchemaResponse/SchemaName
:cognitect.aws.glue.UpdateSchemaResponse/SchemaArn]))
(s/def :cognitect.aws.glue/Iso8601DateTime inst?)
(s/def
:cognitect.aws.glue/LongColumnStatisticsData
(s/keys
:req-un
[:cognitect.aws.glue.LongColumnStatisticsData/NumberOfNulls
:cognitect.aws.glue.LongColumnStatisticsData/NumberOfDistinctValues]
:opt-un
[:cognitect.aws.glue.LongColumnStatisticsData/MaximumValue
:cognitect.aws.glue.LongColumnStatisticsData/MinimumValue]))
(s/def
:cognitect.aws.glue/GetJobRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetJobRunRequest/JobName :cognitect.aws.glue.GetJobRunRequest/RunId]
:opt-un
[:cognitect.aws.glue.GetJobRunRequest/PredecessorsIncluded]))
(s/def
:cognitect.aws.glue/GetTableVersionRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetTableVersionRequest/DatabaseName
:cognitect.aws.glue.GetTableVersionRequest/TableName]
:opt-un
[:cognitect.aws.glue.GetTableVersionRequest/VersionId
:cognitect.aws.glue.GetTableVersionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/UpdateBlueprintRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateBlueprintRequest/Name
:cognitect.aws.glue.UpdateBlueprintRequest/BlueprintLocation]
:opt-un
[:cognitect.aws.glue.UpdateBlueprintRequest/Description]))
(s/def
:cognitect.aws.glue/UpdateCrawlerRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateCrawlerRequest/Name]
:opt-un
[:cognitect.aws.glue.UpdateCrawlerRequest/Classifiers
:cognitect.aws.glue.UpdateCrawlerRequest/DatabaseName
:cognitect.aws.glue.UpdateCrawlerRequest/LineageConfiguration
:cognitect.aws.glue.UpdateCrawlerRequest/LakeFormationConfiguration
:cognitect.aws.glue.UpdateCrawlerRequest/RecrawlPolicy
:cognitect.aws.glue.UpdateCrawlerRequest/CrawlerSecurityConfiguration
:cognitect.aws.glue.UpdateCrawlerRequest/Role
:cognitect.aws.glue.UpdateCrawlerRequest/TablePrefix
:cognitect.aws.glue.UpdateCrawlerRequest/SchemaChangePolicy
:cognitect.aws.glue.UpdateCrawlerRequest/Description
:cognitect.aws.glue.UpdateCrawlerRequest/Targets
:cognitect.aws.glue.UpdateCrawlerRequest/Schedule
:cognitect.aws.glue.UpdateCrawlerRequest/Configuration]))
(s/def
:cognitect.aws.glue/DataQualityRulesetList
(s/coll-of :cognitect.aws.glue/DataQualityRulesetListDetails))
(s/def
:cognitect.aws.glue/SortCriterion
(s/keys
:opt-un
[:cognitect.aws.glue.SortCriterion/Sort :cognitect.aws.glue.SortCriterion/FieldName]))
(s/def
:cognitect.aws.glue/MaxResultsNumber
(s/spec (s/and int? #(<= 1 % 100)) :gen #(gen/choose 1 100)))
(s/def :cognitect.aws.glue/CreateClassifierResponse (s/keys))
(s/def
:cognitect.aws.glue/ListDataQualityRulesetEvaluationRunsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.ListDataQualityRulesetEvaluationRunsRequest/Filter
:cognitect.aws.glue.ListDataQualityRulesetEvaluationRunsRequest/NextToken
:cognitect.aws.glue.ListDataQualityRulesetEvaluationRunsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/FederatedDatabase
(s/keys
:opt-un
[:cognitect.aws.glue.FederatedDatabase/ConnectionName
:cognitect.aws.glue.FederatedDatabase/Identifier]))
(s/def
:cognitect.aws.glue/DeleteJobResponse
(s/keys :opt-un [:cognitect.aws.glue.DeleteJobResponse/JobName]))
(s/def
:cognitect.aws.glue/DataQualityResultDescriptionList
(s/coll-of :cognitect.aws.glue/DataQualityResultDescription))
(s/def
:cognitect.aws.glue/EnableAdditionalMetadata
(s/coll-of :cognitect.aws.glue/JdbcMetadataEntry))
(s/def
:cognitect.aws.glue/MaskValue
(s/spec #(re-matches (re-pattern "[*A-Za-z0-9_-]*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/SourceControlAuthStrategy
(s/spec string? :gen #(s/gen #{"PERSONAL_ACCESS_TOKEN" "AWS_SECRETS_MANAGER"})))
(s/def
:cognitect.aws.glue/PredicateString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\r\\n\\t]*")
%)
:gen
#(gen/string)))
(s/def :cognitect.aws.glue/DeleteDatabaseResponse (s/keys))
(s/def :cognitect.aws.glue/ConditionList (s/coll-of :cognitect.aws.glue/Condition))
(s/def
:cognitect.aws.glue/ListDataQualityResultsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.ListDataQualityResultsRequest/Filter
:cognitect.aws.glue.ListDataQualityResultsRequest/NextToken
:cognitect.aws.glue.ListDataQualityResultsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/Compatibility
(s/spec
string?
:gen
#(s/gen
#{"DISABLED" "FULL_ALL" "BACKWARD_ALL" "FULL" "NONE" "FORWARD_ALL" "BACKWARD" "FORWARD"})))
(s/def :cognitect.aws.glue/DevEndpointList (s/coll-of :cognitect.aws.glue/DevEndpoint))
(s/def
:cognitect.aws.glue/DataQualityResult
(s/keys
:opt-un
[:cognitect.aws.glue.DataQualityResult/RulesetName
:cognitect.aws.glue.DataQualityResult/EvaluationContext
:cognitect.aws.glue.DataQualityResult/RuleResults
:cognitect.aws.glue.DataQualityResult/JobName
:cognitect.aws.glue.DataQualityResult/RulesetEvaluationRunId
:cognitect.aws.glue.DataQualityResult/Score
:cognitect.aws.glue.DataQualityResult/JobRunId
:cognitect.aws.glue.DataQualityResult/DataSource
:cognitect.aws.glue.DataQualityResult/StartedOn
:cognitect.aws.glue.DataQualityResult/CompletedOn
:cognitect.aws.glue.DataQualityResult/ResultId]))
(s/def
:cognitect.aws.glue/BoxedPositiveInt
(s/spec (s/and int? #(<= 0 %)) :gen #(gen/choose 0 Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/JDBCConnectorSource
(s/keys
:req-un
[:cognitect.aws.glue.JDBCConnectorSource/Name
:cognitect.aws.glue.JDBCConnectorSource/ConnectionName
:cognitect.aws.glue.JDBCConnectorSource/ConnectorName
:cognitect.aws.glue.JDBCConnectorSource/ConnectionType]
:opt-un
[:cognitect.aws.glue.JDBCConnectorSource/OutputSchemas
:cognitect.aws.glue.JDBCConnectorSource/AdditionalOptions
:cognitect.aws.glue.JDBCConnectorSource/Query
:cognitect.aws.glue.JDBCConnectorSource/ConnectionTable]))
(s/def
:cognitect.aws.glue/GetSchemaVersionsDiffInput
(s/keys
:req-un
[:cognitect.aws.glue.GetSchemaVersionsDiffInput/SchemaId
:cognitect.aws.glue.GetSchemaVersionsDiffInput/FirstSchemaVersionNumber
:cognitect.aws.glue.GetSchemaVersionsDiffInput/SecondSchemaVersionNumber
:cognitect.aws.glue.GetSchemaVersionsDiffInput/SchemaDiffType]))
(s/def
:cognitect.aws.glue/SessionCommand
(s/keys
:opt-un
[:cognitect.aws.glue.SessionCommand/PythonVersion :cognitect.aws.glue.SessionCommand/Name]))
(s/def
:cognitect.aws.glue/ListSchemasInput
(s/keys
:opt-un
[:cognitect.aws.glue.ListSchemasInput/NextToken
:cognitect.aws.glue.ListSchemasInput/RegistryId
:cognitect.aws.glue.ListSchemasInput/MaxResults]))
(s/def :cognitect.aws.glue/JobList (s/coll-of :cognitect.aws.glue/Job))
(s/def
:cognitect.aws.glue/StartingPosition
(s/spec string? :gen #(s/gen #{"earliest" "timestamp" "trim_horizon" "latest"})))
(s/def :cognitect.aws.glue/Timestamp inst?)
(s/def :cognitect.aws.glue/JdbcTargetList (s/coll-of :cognitect.aws.glue/JdbcTarget))
(s/def
:cognitect.aws.glue/CommentString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/NonNegativeDouble
(s/spec (s/and double? #(<= 0 %)) :gen #(gen/double* {:infinite? false, :NaN? false, :min 0})))
(s/def
:cognitect.aws.glue/CreateSessionRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateSessionRequest/Id
:cognitect.aws.glue.CreateSessionRequest/Role
:cognitect.aws.glue.CreateSessionRequest/Command]
:opt-un
[:cognitect.aws.glue.CreateSessionRequest/Timeout
:cognitect.aws.glue.CreateSessionRequest/MaxCapacity
:cognitect.aws.glue.CreateSessionRequest/Tags
:cognitect.aws.glue.CreateSessionRequest/DefaultArguments
:cognitect.aws.glue.CreateSessionRequest/NumberOfWorkers
:cognitect.aws.glue.CreateSessionRequest/WorkerType
:cognitect.aws.glue.CreateSessionRequest/Connections
:cognitect.aws.glue.CreateSessionRequest/IdleTimeout
:cognitect.aws.glue.CreateSessionRequest/GlueVersion
:cognitect.aws.glue.CreateSessionRequest/Description
:cognitect.aws.glue.CreateSessionRequest/SecurityConfiguration
:cognitect.aws.glue.CreateSessionRequest/RequestOrigin]))
(s/def :cognitect.aws.glue/JsonPath string?)
(s/def
:cognitect.aws.glue/TwoInputs
(s/coll-of :cognitect.aws.glue/NodeId :min-count 2 :max-count 2))
(s/def
:cognitect.aws.glue/RulesetNames
(s/coll-of :cognitect.aws.glue/NameString :min-count 1 :max-count 10))
(s/def
:cognitect.aws.glue/StartExportLabelsTaskRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.StartExportLabelsTaskRunRequest/TransformId
:cognitect.aws.glue.StartExportLabelsTaskRunRequest/OutputS3Path]))
(s/def
:cognitect.aws.glue/TagsMap
(s/map-of :cognitect.aws.glue/TagKey :cognitect.aws.glue/TagValue :min-count 0 :max-count 50))
(s/def :cognitect.aws.glue/StringList (s/coll-of :cognitect.aws.glue/GenericString))
(s/def
:cognitect.aws.glue/GrokClassifier
(s/keys
:req-un
[:cognitect.aws.glue.GrokClassifier/Name
:cognitect.aws.glue.GrokClassifier/Classification
:cognitect.aws.glue.GrokClassifier/GrokPattern]
:opt-un
[:cognitect.aws.glue.GrokClassifier/CustomPatterns
:cognitect.aws.glue.GrokClassifier/CreationTime
:cognitect.aws.glue.GrokClassifier/Version
:cognitect.aws.glue.GrokClassifier/LastUpdated]))
(s/def
:cognitect.aws.glue/GetTablesResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetTablesResponse/TableList
:cognitect.aws.glue.GetTablesResponse/NextToken]))
(s/def
:cognitect.aws.glue/OtherMetadataValueListItem
(s/keys
:opt-un
[:cognitect.aws.glue.OtherMetadataValueListItem/MetadataValue
:cognitect.aws.glue.OtherMetadataValueListItem/CreatedTime]))
(s/def
:cognitect.aws.glue/KinesisStreamingSourceOptions
(s/keys
:opt-un
[:cognitect.aws.glue.KinesisStreamingSourceOptions/AddRecordTimestamp
:cognitect.aws.glue.KinesisStreamingSourceOptions/StreamName
:cognitect.aws.glue.KinesisStreamingSourceOptions/AvoidEmptyBatches
:cognitect.aws.glue.KinesisStreamingSourceOptions/MaxFetchTimeInMs
:cognitect.aws.glue.KinesisStreamingSourceOptions/RoleArn
:cognitect.aws.glue.KinesisStreamingSourceOptions/RoleSessionName
:cognitect.aws.glue.KinesisStreamingSourceOptions/DescribeShardInterval
:cognitect.aws.glue.KinesisStreamingSourceOptions/NumRetries
:cognitect.aws.glue.KinesisStreamingSourceOptions/StreamArn
:cognitect.aws.glue.KinesisStreamingSourceOptions/IdleTimeBetweenReadsInMs
:cognitect.aws.glue.KinesisStreamingSourceOptions/StartingPosition
:cognitect.aws.glue.KinesisStreamingSourceOptions/EndpointUrl
:cognitect.aws.glue.KinesisStreamingSourceOptions/Classification
:cognitect.aws.glue.KinesisStreamingSourceOptions/MaxRecordPerRead
:cognitect.aws.glue.KinesisStreamingSourceOptions/EmitConsumerLagMetrics
:cognitect.aws.glue.KinesisStreamingSourceOptions/Delimiter
:cognitect.aws.glue.KinesisStreamingSourceOptions/RetryIntervalMs
:cognitect.aws.glue.KinesisStreamingSourceOptions/MaxFetchRecordsPerShard
:cognitect.aws.glue.KinesisStreamingSourceOptions/StartingTimestamp
:cognitect.aws.glue.KinesisStreamingSourceOptions/MaxRetryIntervalMs
:cognitect.aws.glue.KinesisStreamingSourceOptions/AddIdleTimeBetweenReads]))
(s/def
:cognitect.aws.glue/GetSchemaVersionResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetSchemaVersionResponse/DataFormat
:cognitect.aws.glue.GetSchemaVersionResponse/Status
:cognitect.aws.glue.GetSchemaVersionResponse/CreatedTime
:cognitect.aws.glue.GetSchemaVersionResponse/VersionNumber
:cognitect.aws.glue.GetSchemaVersionResponse/SchemaArn
:cognitect.aws.glue.GetSchemaVersionResponse/SchemaDefinition
:cognitect.aws.glue.GetSchemaVersionResponse/SchemaVersionId]))
(s/def
:cognitect.aws.glue/TransformConfigParameterList
(s/coll-of :cognitect.aws.glue/TransformConfigParameter))
(s/def
:cognitect.aws.glue/GetSchemaByDefinitionInput
(s/keys
:req-un
[:cognitect.aws.glue.GetSchemaByDefinitionInput/SchemaId
:cognitect.aws.glue.GetSchemaByDefinitionInput/SchemaDefinition]))
(s/def
:cognitect.aws.glue/Crawler
(s/keys
:opt-un
[:cognitect.aws.glue.Crawler/LastCrawl
:cognitect.aws.glue.Crawler/Classifiers
:cognitect.aws.glue.Crawler/DatabaseName
:cognitect.aws.glue.Crawler/LineageConfiguration
:cognitect.aws.glue.Crawler/LakeFormationConfiguration
:cognitect.aws.glue.Crawler/RecrawlPolicy
:cognitect.aws.glue.Crawler/CrawlerSecurityConfiguration
:cognitect.aws.glue.Crawler/Role
:cognitect.aws.glue.Crawler/TablePrefix
:cognitect.aws.glue.Crawler/CrawlElapsedTime
:cognitect.aws.glue.Crawler/SchemaChangePolicy
:cognitect.aws.glue.Crawler/Description
:cognitect.aws.glue.Crawler/Targets
:cognitect.aws.glue.Crawler/CreationTime
:cognitect.aws.glue.Crawler/State
:cognitect.aws.glue.Crawler/Version
:cognitect.aws.glue.Crawler/Schedule
:cognitect.aws.glue.Crawler/Name
:cognitect.aws.glue.Crawler/Configuration
:cognitect.aws.glue.Crawler/LastUpdated]))
(s/def :cognitect.aws.glue/FieldType string?)
(s/def
:cognitect.aws.glue/BoundedPartitionValueList
(s/coll-of :cognitect.aws.glue/ValueString :min-count 0 :max-count 100))
(s/def
:cognitect.aws.glue/BatchGetJobsRequest
(s/keys :req-un [:cognitect.aws.glue.BatchGetJobsRequest/JobNames]))
(s/def :cognitect.aws.glue/LongValue (s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/ListSessionsRequest
(s/keys
:opt-un
[:cognitect.aws.glue.ListSessionsRequest/Tags
:cognitect.aws.glue.ListSessionsRequest/NextToken
:cognitect.aws.glue.ListSessionsRequest/MaxResults
:cognitect.aws.glue.ListSessionsRequest/RequestOrigin]))
(s/def
:cognitect.aws.glue/GenericBoundedDouble
(s/spec
(s/and double? #(<= 0 % 1))
:gen
#(gen/double* {:infinite? false, :NaN? false, :min 0, :max 1})))
(s/def
:cognitect.aws.glue/GetSessionRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetSessionRequest/Id]
:opt-un
[:cognitect.aws.glue.GetSessionRequest/RequestOrigin]))
(s/def
:cognitect.aws.glue/UpdateCatalogBehavior
(s/spec string? :gen #(s/gen #{"UPDATE_IN_DATABASE" "LOG"})))
(s/def
:cognitect.aws.glue/FieldName
(s/spec string? :gen #(s/gen #{"DPU_HOUR" "STATE" "START_TIME" "END_TIME" "CRAWL_ID"})))
(s/def
:cognitect.aws.glue/S3DirectTarget
(s/keys
:req-un
[:cognitect.aws.glue.S3DirectTarget/Name
:cognitect.aws.glue.S3DirectTarget/Inputs
:cognitect.aws.glue.S3DirectTarget/Path
:cognitect.aws.glue.S3DirectTarget/Format]
:opt-un
[:cognitect.aws.glue.S3DirectTarget/PartitionKeys
:cognitect.aws.glue.S3DirectTarget/SchemaChangePolicy
:cognitect.aws.glue.S3DirectTarget/Compression]))
(s/def
:cognitect.aws.glue/Classifier
(s/keys
:opt-un
[:cognitect.aws.glue.Classifier/GrokClassifier
:cognitect.aws.glue.Classifier/XMLClassifier
:cognitect.aws.glue.Classifier/JsonClassifier
:cognitect.aws.glue.Classifier/CsvClassifier]))
(s/def
:cognitect.aws.glue/ScheduleState
(s/spec string? :gen #(s/gen #{"SCHEDULED" "TRANSITIONING" "NOT_SCHEDULED"})))
(s/def
:cognitect.aws.glue/GetJobBookmarkResponse
(s/keys :opt-un [:cognitect.aws.glue.GetJobBookmarkResponse/JobBookmarkEntry]))
(s/def
:cognitect.aws.glue/CatalogIdString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/TransformSortCriteria
(s/keys
:req-un
[:cognitect.aws.glue.TransformSortCriteria/Column
:cognitect.aws.glue.TransformSortCriteria/SortDirection]))
(s/def
:cognitect.aws.glue/UpdateRegistryInput
(s/keys
:req-un
[:cognitect.aws.glue.UpdateRegistryInput/RegistryId
:cognitect.aws.glue.UpdateRegistryInput/Description]))
(s/def
:cognitect.aws.glue/Condition
(s/keys
:opt-un
[:cognitect.aws.glue.Condition/JobName
:cognitect.aws.glue.Condition/CrawlerName
:cognitect.aws.glue.Condition/State
:cognitect.aws.glue.Condition/LogicalOperator
:cognitect.aws.glue.Condition/CrawlState]))
(s/def :cognitect.aws.glue/LogicalOperator (s/spec string? :gen #(s/gen #{"EQUALS"})))
(s/def :cognitect.aws.glue/Role string?)
(s/def
:cognitect.aws.glue/OrchestrationRoleArn
(s/spec #(re-matches (re-pattern "arn:aws[^:]*:iam::[0-9]*:role/.+") %) :gen #(gen/string)))
(s/def :cognitect.aws.glue/TransformIdList (s/coll-of :cognitect.aws.glue/HashString))
(s/def
:cognitect.aws.glue/GetMLTaskRunResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetMLTaskRunResponse/ExecutionTime
:cognitect.aws.glue.GetMLTaskRunResponse/LastModifiedOn
:cognitect.aws.glue.GetMLTaskRunResponse/Status
:cognitect.aws.glue.GetMLTaskRunResponse/TransformId
:cognitect.aws.glue.GetMLTaskRunResponse/Properties
:cognitect.aws.glue.GetMLTaskRunResponse/TaskRunId
:cognitect.aws.glue.GetMLTaskRunResponse/ErrorString
:cognitect.aws.glue.GetMLTaskRunResponse/LogGroupName
:cognitect.aws.glue.GetMLTaskRunResponse/StartedOn
:cognitect.aws.glue.GetMLTaskRunResponse/CompletedOn]))
(s/def :cognitect.aws.glue/ColumnRowFilterList (s/coll-of :cognitect.aws.glue/ColumnRowFilter))
(s/def :cognitect.aws.glue/TriggerList (s/coll-of :cognitect.aws.glue/Trigger))
(s/def
:cognitect.aws.glue/BlueprintDetails
(s/keys
:opt-un
[:cognitect.aws.glue.BlueprintDetails/RunId
:cognitect.aws.glue.BlueprintDetails/BlueprintName]))
(s/def
:cognitect.aws.glue/KeySchemaElement
(s/keys
:req-un
[:cognitect.aws.glue.KeySchemaElement/Name :cognitect.aws.glue.KeySchemaElement/Type]))
(s/def
:cognitect.aws.glue/Partition
(s/keys
:opt-un
[:cognitect.aws.glue.Partition/DatabaseName
:cognitect.aws.glue.Partition/Parameters
:cognitect.aws.glue.Partition/StorageDescriptor
:cognitect.aws.glue.Partition/Values
:cognitect.aws.glue.Partition/CreationTime
:cognitect.aws.glue.Partition/TableName
:cognitect.aws.glue.Partition/LastAccessTime
:cognitect.aws.glue.Partition/CatalogId
:cognitect.aws.glue.Partition/LastAnalyzedTime]))
(s/def
:cognitect.aws.glue/DeleteCrawlerRequest
(s/keys :req-un [:cognitect.aws.glue.DeleteCrawlerRequest/Name]))
(s/def :cognitect.aws.glue/Language (s/spec string? :gen #(s/gen #{"PYTHON" "SCALA"})))
(s/def
:cognitect.aws.glue/DeleteMLTransformRequest
(s/keys :req-un [:cognitect.aws.glue.DeleteMLTransformRequest/TransformId]))
(s/def
:cognitect.aws.glue/PollingTime
(s/spec (s/and int? #(<= 10 %)) :gen #(gen/choose 10 Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/Crawl
(s/keys
:opt-un
[:cognitect.aws.glue.Crawl/ErrorMessage
:cognitect.aws.glue.Crawl/LogGroup
:cognitect.aws.glue.Crawl/LogStream
:cognitect.aws.glue.Crawl/StartedOn
:cognitect.aws.glue.Crawl/State
:cognitect.aws.glue.Crawl/CompletedOn]))
(s/def
:cognitect.aws.glue/GetBlueprintRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetBlueprintRequest/Name]
:opt-un
[:cognitect.aws.glue.GetBlueprintRequest/IncludeParameterSpec
:cognitect.aws.glue.GetBlueprintRequest/IncludeBlueprint]))
(s/def :cognitect.aws.glue/CancelDataQualityRuleRecommendationRunResponse (s/keys))
(s/def :cognitect.aws.glue/IntegerFlag (s/spec (s/and int? #(<= 0 % 1)) :gen #(gen/choose 0 1)))
(s/def
:cognitect.aws.glue/GetWorkflowRunsRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetWorkflowRunsRequest/Name]
:opt-un
[:cognitect.aws.glue.GetWorkflowRunsRequest/NextToken
:cognitect.aws.glue.GetWorkflowRunsRequest/IncludeGraph
:cognitect.aws.glue.GetWorkflowRunsRequest/MaxResults]))
(s/def :cognitect.aws.glue/ReplaceBoolean boolean?)
(s/def
:cognitect.aws.glue/DeleteBehavior
(s/spec string? :gen #(s/gen #{"DELETE_FROM_DATABASE" "DEPRECATE_IN_DATABASE" "LOG"})))
(s/def
:cognitect.aws.glue/CatalogEntry
(s/keys
:req-un
[:cognitect.aws.glue.CatalogEntry/DatabaseName :cognitect.aws.glue.CatalogEntry/TableName]))
(s/def
:cognitect.aws.glue/LastActiveDefinition
(s/keys
:opt-un
[:cognitect.aws.glue.LastActiveDefinition/BlueprintLocation
:cognitect.aws.glue.LastActiveDefinition/LastModifiedOn
:cognitect.aws.glue.LastActiveDefinition/Description
:cognitect.aws.glue.LastActiveDefinition/ParameterSpec
:cognitect.aws.glue.LastActiveDefinition/BlueprintServiceLocation]))
(s/def
:cognitect.aws.glue/JoinType
(s/spec string? :gen #(s/gen #{"leftsemi" "right" "equijoin" "outer" "leftanti" "left"})))
(s/def :cognitect.aws.glue/DataSource (s/keys :req-un [:cognitect.aws.glue.DataSource/GlueTable]))
(s/def
:cognitect.aws.glue/OrchestrationToken
(s/spec
(s/and string? #(>= 400000 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 400000) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/TableIdentifier
(s/keys
:opt-un
[:cognitect.aws.glue.TableIdentifier/DatabaseName
:cognitect.aws.glue.TableIdentifier/CatalogId
:cognitect.aws.glue.TableIdentifier/Name
:cognitect.aws.glue.TableIdentifier/Region]))
(s/def
:cognitect.aws.glue/GlueStudioColumnNameString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/UpdateJobRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateJobRequest/JobName :cognitect.aws.glue.UpdateJobRequest/JobUpdate]))
(s/def
:cognitect.aws.glue/SkewedInfo
(s/keys
:opt-un
[:cognitect.aws.glue.SkewedInfo/SkewedColumnValues
:cognitect.aws.glue.SkewedInfo/SkewedColumnNames
:cognitect.aws.glue.SkewedInfo/SkewedColumnValueLocationMaps]))
(s/def
:cognitect.aws.glue/StartWorkflowRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.StartWorkflowRunRequest/Name]
:opt-un
[:cognitect.aws.glue.StartWorkflowRunRequest/RunProperties]))
(s/def
:cognitect.aws.glue/DeleteRegistryResponse
(s/keys
:opt-un
[:cognitect.aws.glue.DeleteRegistryResponse/RegistryArn
:cognitect.aws.glue.DeleteRegistryResponse/Status
:cognitect.aws.glue.DeleteRegistryResponse/RegistryName]))
(s/def
:cognitect.aws.glue/LogStream
(s/spec #(re-matches (re-pattern "[^:*]*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/RemoveSchemaVersionMetadataInput
(s/keys
:req-un
[:cognitect.aws.glue.RemoveSchemaVersionMetadataInput/MetadataKeyValue]
:opt-un
[:cognitect.aws.glue.RemoveSchemaVersionMetadataInput/SchemaVersionNumber
:cognitect.aws.glue.RemoveSchemaVersionMetadataInput/SchemaId
:cognitect.aws.glue.RemoveSchemaVersionMetadataInput/SchemaVersionId]))
(s/def
:cognitect.aws.glue/S3SourceAdditionalOptions
(s/keys
:opt-un
[:cognitect.aws.glue.S3SourceAdditionalOptions/BoundedFiles
:cognitect.aws.glue.S3SourceAdditionalOptions/BoundedSize]))
(s/def
:cognitect.aws.glue/CatalogTablesList
(s/coll-of :cognitect.aws.glue/NameString :min-count 1))
(s/def :cognitect.aws.glue/DeleteUserDefinedFunctionResponse (s/keys))
(s/def
:cognitect.aws.glue/SourceControlDetails
(s/keys
:opt-un
[:cognitect.aws.glue.SourceControlDetails/AuthToken
:cognitect.aws.glue.SourceControlDetails/AuthStrategy
:cognitect.aws.glue.SourceControlDetails/Branch
:cognitect.aws.glue.SourceControlDetails/Folder
:cognitect.aws.glue.SourceControlDetails/Repository
:cognitect.aws.glue.SourceControlDetails/LastCommitId
:cognitect.aws.glue.SourceControlDetails/Provider
:cognitect.aws.glue.SourceControlDetails/Owner]))
(s/def
:cognitect.aws.glue/CodeGenNode
(s/keys
:req-un
[:cognitect.aws.glue.CodeGenNode/Id
:cognitect.aws.glue.CodeGenNode/NodeType
:cognitect.aws.glue.CodeGenNode/Args]
:opt-un
[:cognitect.aws.glue.CodeGenNode/LineNumber]))
(s/def
:cognitect.aws.glue/GetUserDefinedFunctionRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetUserDefinedFunctionRequest/DatabaseName
:cognitect.aws.glue.GetUserDefinedFunctionRequest/FunctionName]
:opt-un
[:cognitect.aws.glue.GetUserDefinedFunctionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/DeleteUserDefinedFunctionRequest
(s/keys
:req-un
[:cognitect.aws.glue.DeleteUserDefinedFunctionRequest/DatabaseName
:cognitect.aws.glue.DeleteUserDefinedFunctionRequest/FunctionName]
:opt-un
[:cognitect.aws.glue.DeleteUserDefinedFunctionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/LakeFormationConfiguration
(s/keys
:opt-un
[:cognitect.aws.glue.LakeFormationConfiguration/UseLakeFormationCredentials
:cognitect.aws.glue.LakeFormationConfiguration/AccountId]))
(s/def
:cognitect.aws.glue/GetCustomEntityTypeRequest
(s/keys :req-un [:cognitect.aws.glue.GetCustomEntityTypeRequest/Name]))
(s/def
:cognitect.aws.glue/GetTableResponse
(s/keys :opt-un [:cognitect.aws.glue.GetTableResponse/Table]))
(s/def
:cognitect.aws.glue/Double
(s/spec double? :gen #(gen/double* {:infinite? false, :NaN? false})))
(s/def
:cognitect.aws.glue/DQResultsPublishingOptions
(s/keys
:opt-un
[:cognitect.aws.glue.DQResultsPublishingOptions/EvaluationContext
:cognitect.aws.glue.DQResultsPublishingOptions/ResultsS3Prefix
:cognitect.aws.glue.DQResultsPublishingOptions/CloudWatchMetricsEnabled
:cognitect.aws.glue.DQResultsPublishingOptions/ResultsPublishingEnabled]))
(s/def
:cognitect.aws.glue/SchemaVersionErrorList
(s/coll-of :cognitect.aws.glue/SchemaVersionErrorItem))
(s/def
:cognitect.aws.glue/StartMLEvaluationTaskRunResponse
(s/keys :opt-un [:cognitect.aws.glue.StartMLEvaluationTaskRunResponse/TaskRunId]))
(s/def
:cognitect.aws.glue/RecrawlPolicy
(s/keys :opt-un [:cognitect.aws.glue.RecrawlPolicy/RecrawlBehavior]))
(s/def
:cognitect.aws.glue/Location
(s/keys
:opt-un
[:cognitect.aws.glue.Location/Jdbc
:cognitect.aws.glue.Location/DynamoDB
:cognitect.aws.glue.Location/S3]))
(s/def
:cognitect.aws.glue/BatchDeleteTableRequest
(s/keys
:req-un
[:cognitect.aws.glue.BatchDeleteTableRequest/DatabaseName
:cognitect.aws.glue.BatchDeleteTableRequest/TablesToDelete]
:opt-un
[:cognitect.aws.glue.BatchDeleteTableRequest/TransactionId
:cognitect.aws.glue.BatchDeleteTableRequest/CatalogId]))
(s/def
:cognitect.aws.glue/Statement
(s/keys
:opt-un
[:cognitect.aws.glue.Statement/Progress
:cognitect.aws.glue.Statement/StartedOn
:cognitect.aws.glue.Statement/State
:cognitect.aws.glue.Statement/Output
:cognitect.aws.glue.Statement/Code
:cognitect.aws.glue.Statement/CompletedOn
:cognitect.aws.glue.Statement/Id]))
(s/def :cognitect.aws.glue/CrawlerMetricsList (s/coll-of :cognitect.aws.glue/CrawlerMetrics))
(s/def
:cognitect.aws.glue/AuditContext
(s/keys
:opt-un
[:cognitect.aws.glue.AuditContext/RequestedColumns
:cognitect.aws.glue.AuditContext/AdditionalAuditContext
:cognitect.aws.glue.AuditContext/AllColumnsRequested]))
(s/def :cognitect.aws.glue/CrawlerList (s/coll-of :cognitect.aws.glue/Crawler))
(s/def
:cognitect.aws.glue/Session
(s/keys
:opt-un
[:cognitect.aws.glue.Session/MaxCapacity
:cognitect.aws.glue.Session/DefaultArguments
:cognitect.aws.glue.Session/Status
:cognitect.aws.glue.Session/Progress
:cognitect.aws.glue.Session/ErrorMessage
:cognitect.aws.glue.Session/Connections
:cognitect.aws.glue.Session/Role
:cognitect.aws.glue.Session/GlueVersion
:cognitect.aws.glue.Session/Description
:cognitect.aws.glue.Session/SecurityConfiguration
:cognitect.aws.glue.Session/Command
:cognitect.aws.glue.Session/CreatedOn
:cognitect.aws.glue.Session/Id]))
(s/def
:cognitect.aws.glue/GrokPattern
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\r\\t]*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.glue/GetMLTransformResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetMLTransformResponse/Schema
:cognitect.aws.glue.GetMLTransformResponse/InputRecordTables
:cognitect.aws.glue.GetMLTransformResponse/Timeout
:cognitect.aws.glue.GetMLTransformResponse/MaxCapacity
:cognitect.aws.glue.GetMLTransformResponse/Parameters
:cognitect.aws.glue.GetMLTransformResponse/EvaluationMetrics
:cognitect.aws.glue.GetMLTransformResponse/NumberOfWorkers
:cognitect.aws.glue.GetMLTransformResponse/WorkerType
:cognitect.aws.glue.GetMLTransformResponse/LastModifiedOn
:cognitect.aws.glue.GetMLTransformResponse/Status
:cognitect.aws.glue.GetMLTransformResponse/TransformId
:cognitect.aws.glue.GetMLTransformResponse/Role
:cognitect.aws.glue.GetMLTransformResponse/GlueVersion
:cognitect.aws.glue.GetMLTransformResponse/Description
:cognitect.aws.glue.GetMLTransformResponse/TransformEncryption
:cognitect.aws.glue.GetMLTransformResponse/LabelCount
:cognitect.aws.glue.GetMLTransformResponse/MaxRetries
:cognitect.aws.glue.GetMLTransformResponse/Name
:cognitect.aws.glue.GetMLTransformResponse/CreatedOn]))
(s/def
:cognitect.aws.glue/UpdateCsvClassifierRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateCsvClassifierRequest/Name]
:opt-un
[:cognitect.aws.glue.UpdateCsvClassifierRequest/Header
:cognitect.aws.glue.UpdateCsvClassifierRequest/CustomDatatypeConfigured
:cognitect.aws.glue.UpdateCsvClassifierRequest/CustomDatatypes
:cognitect.aws.glue.UpdateCsvClassifierRequest/Delimiter
:cognitect.aws.glue.UpdateCsvClassifierRequest/QuoteSymbol
:cognitect.aws.glue.UpdateCsvClassifierRequest/AllowSingleColumn
:cognitect.aws.glue.UpdateCsvClassifierRequest/DisableValueTrimming
:cognitect.aws.glue.UpdateCsvClassifierRequest/ContainsHeader]))
(s/def
:cognitect.aws.glue/ExtendedString
(s/spec #(re-matches (re-pattern "[\\s\\S]*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/GetCrawlerMetricsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetCrawlerMetricsResponse/CrawlerMetricsList
:cognitect.aws.glue.GetCrawlerMetricsResponse/NextToken]))
(s/def
:cognitect.aws.glue/GetJobBookmarkRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetJobBookmarkRequest/JobName]
:opt-un
[:cognitect.aws.glue.GetJobBookmarkRequest/RunId]))
(s/def
:cognitect.aws.glue/DeleteMLTransformResponse
(s/keys :opt-un [:cognitect.aws.glue.DeleteMLTransformResponse/TransformId]))
(s/def
:cognitect.aws.glue/GetJobRunsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetJobRunsResponse/NextToken
:cognitect.aws.glue.GetJobRunsResponse/JobRuns]))
(s/def
:cognitect.aws.glue/SchemaVersionErrorItem
(s/keys
:opt-un
[:cognitect.aws.glue.SchemaVersionErrorItem/ErrorDetails
:cognitect.aws.glue.SchemaVersionErrorItem/VersionNumber]))
(s/def
:cognitect.aws.glue/GetTableVersionsRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetTableVersionsRequest/DatabaseName
:cognitect.aws.glue.GetTableVersionsRequest/TableName]
:opt-un
[:cognitect.aws.glue.GetTableVersionsRequest/NextToken
:cognitect.aws.glue.GetTableVersionsRequest/CatalogId
:cognitect.aws.glue.GetTableVersionsRequest/MaxResults]))
(s/def
:cognitect.aws.glue/StartDataQualityRuleRecommendationRunResponse
(s/keys :opt-un [:cognitect.aws.glue.StartDataQualityRuleRecommendationRunResponse/RunId]))
(s/def
:cognitect.aws.glue/Connection
(s/keys
:opt-un
[:cognitect.aws.glue.Connection/PhysicalConnectionRequirements
:cognitect.aws.glue.Connection/Description
:cognitect.aws.glue.Connection/ConnectionProperties
:cognitect.aws.glue.Connection/LastUpdatedTime
:cognitect.aws.glue.Connection/ConnectionType
:cognitect.aws.glue.Connection/CreationTime
:cognitect.aws.glue.Connection/Name
:cognitect.aws.glue.Connection/MatchCriteria
:cognitect.aws.glue.Connection/LastUpdatedBy]))
(s/def
:cognitect.aws.glue/CreateDatabaseRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateDatabaseRequest/DatabaseInput]
:opt-un
[:cognitect.aws.glue.CreateDatabaseRequest/Tags
:cognitect.aws.glue.CreateDatabaseRequest/CatalogId]))
(s/def :cognitect.aws.glue/DeleteTableVersionResponse (s/keys))
(s/def
:cognitect.aws.glue/TransformFilterCriteria
(s/keys
:opt-un
[:cognitect.aws.glue.TransformFilterCriteria/Schema
:cognitect.aws.glue.TransformFilterCriteria/Status
:cognitect.aws.glue.TransformFilterCriteria/CreatedAfter
:cognitect.aws.glue.TransformFilterCriteria/GlueVersion
:cognitect.aws.glue.TransformFilterCriteria/CreatedBefore
:cognitect.aws.glue.TransformFilterCriteria/LastModifiedAfter
:cognitect.aws.glue.TransformFilterCriteria/LastModifiedBefore
:cognitect.aws.glue.TransformFilterCriteria/TransformType
:cognitect.aws.glue.TransformFilterCriteria/Name]))
(s/def
:cognitect.aws.glue/CreatePartitionIndexRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreatePartitionIndexRequest/DatabaseName
:cognitect.aws.glue.CreatePartitionIndexRequest/TableName
:cognitect.aws.glue.CreatePartitionIndexRequest/PartitionIndex]
:opt-un
[:cognitect.aws.glue.CreatePartitionIndexRequest/CatalogId]))
(s/def
:cognitect.aws.glue/JdbcMetadataEntry
(s/spec string? :gen #(s/gen #{"RAWTYPES" "COMMENTS"})))
(s/def
:cognitect.aws.glue/TaskRunSortColumnType
(s/spec string? :gen #(s/gen #{"STATUS" "TASK_RUN_TYPE" "STARTED"})))
(s/def :cognitect.aws.glue/GetTableVersionsList (s/coll-of :cognitect.aws.glue/TableVersion))
(s/def
:cognitect.aws.glue/SchemaReference
(s/keys
:opt-un
[:cognitect.aws.glue.SchemaReference/SchemaVersionNumber
:cognitect.aws.glue.SchemaReference/SchemaId
:cognitect.aws.glue.SchemaReference/SchemaVersionId]))
(s/def
:cognitect.aws.glue/BatchDeleteTableVersionRequest
(s/keys
:req-un
[:cognitect.aws.glue.BatchDeleteTableVersionRequest/DatabaseName
:cognitect.aws.glue.BatchDeleteTableVersionRequest/TableName
:cognitect.aws.glue.BatchDeleteTableVersionRequest/VersionIds]
:opt-un
[:cognitect.aws.glue.BatchDeleteTableVersionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/CreateRegistryInput
(s/keys
:req-un
[:cognitect.aws.glue.CreateRegistryInput/RegistryName]
:opt-un
[:cognitect.aws.glue.CreateRegistryInput/Tags
:cognitect.aws.glue.CreateRegistryInput/Description]))
(s/def
:cognitect.aws.glue/ColumnStatisticsData
(s/keys
:req-un
[:cognitect.aws.glue.ColumnStatisticsData/Type]
:opt-un
[:cognitect.aws.glue.ColumnStatisticsData/DateColumnStatisticsData
:cognitect.aws.glue.ColumnStatisticsData/StringColumnStatisticsData
:cognitect.aws.glue.ColumnStatisticsData/DoubleColumnStatisticsData
:cognitect.aws.glue.ColumnStatisticsData/BooleanColumnStatisticsData
:cognitect.aws.glue.ColumnStatisticsData/LongColumnStatisticsData
:cognitect.aws.glue.ColumnStatisticsData/DecimalColumnStatisticsData
:cognitect.aws.glue.ColumnStatisticsData/BinaryColumnStatisticsData]))
(s/def
:cognitect.aws.glue/FilterValue
(s/keys :req-un [:cognitect.aws.glue.FilterValue/Type :cognitect.aws.glue.FilterValue/Value]))
(s/def
:cognitect.aws.glue/ExistCondition
(s/spec string? :gen #(s/gen #{"MUST_EXIST" "NONE" "NOT_EXIST"})))
(s/def :cognitect.aws.glue/DatabaseName string?)
(s/def :cognitect.aws.glue/DeltaTargetList (s/coll-of :cognitect.aws.glue/DeltaTarget))
(s/def
:cognitect.aws.glue/BatchGetDataQualityResultRequest
(s/keys :req-un [:cognitect.aws.glue.BatchGetDataQualityResultRequest/ResultIds]))
(s/def :cognitect.aws.glue/DeletePartitionResponse (s/keys))
(s/def
:cognitect.aws.glue/CreateDataQualityRulesetRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateDataQualityRulesetRequest/Name
:cognitect.aws.glue.CreateDataQualityRulesetRequest/Ruleset]
:opt-un
[:cognitect.aws.glue.CreateDataQualityRulesetRequest/TargetTable
:cognitect.aws.glue.CreateDataQualityRulesetRequest/Tags
:cognitect.aws.glue.CreateDataQualityRulesetRequest/Description
:cognitect.aws.glue.CreateDataQualityRulesetRequest/ClientToken]))
(s/def
:cognitect.aws.glue/MicrosoftSQLServerCatalogSource
(s/keys
:req-un
[:cognitect.aws.glue.MicrosoftSQLServerCatalogSource/Name
:cognitect.aws.glue.MicrosoftSQLServerCatalogSource/Database
:cognitect.aws.glue.MicrosoftSQLServerCatalogSource/Table]))
(s/def
:cognitect.aws.glue/GetJobsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetJobsResponse/NextToken :cognitect.aws.glue.GetJobsResponse/Jobs]))
(s/def
:cognitect.aws.glue/BatchGetCustomEntityTypesRequest
(s/keys :req-un [:cognitect.aws.glue.BatchGetCustomEntityTypesRequest/Names]))
(s/def :cognitect.aws.glue/DagEdges (s/coll-of :cognitect.aws.glue/CodeGenEdge))
(s/def
:cognitect.aws.glue/ColumnImportanceList
(s/coll-of :cognitect.aws.glue/ColumnImportance :min-count 0 :max-count 100))
(s/def
:cognitect.aws.glue/DecimalColumnStatisticsData
(s/keys
:req-un
[:cognitect.aws.glue.DecimalColumnStatisticsData/NumberOfNulls
:cognitect.aws.glue.DecimalColumnStatisticsData/NumberOfDistinctValues]
:opt-un
[:cognitect.aws.glue.DecimalColumnStatisticsData/MaximumValue
:cognitect.aws.glue.DecimalColumnStatisticsData/MinimumValue]))
(s/def
:cognitect.aws.glue/IcebergInput
(s/keys
:req-un
[:cognitect.aws.glue.IcebergInput/MetadataOperation]
:opt-un
[:cognitect.aws.glue.IcebergInput/Version]))
(s/def
:cognitect.aws.glue/SourceControlProvider
(s/spec string? :gen #(s/gen #{"AWS_CODE_COMMIT" "GITHUB"})))
(s/def
:cognitect.aws.glue/GetCrawlersRequest
(s/keys
:opt-un
[:cognitect.aws.glue.GetCrawlersRequest/NextToken
:cognitect.aws.glue.GetCrawlersRequest/MaxResults]))
(s/def :cognitect.aws.glue/StopCrawlerScheduleResponse (s/keys))
(s/def
:cognitect.aws.glue/DQTransformOutput
(s/spec string? :gen #(s/gen #{"EvaluationResults" "PrimaryInput"})))
(s/def :cognitect.aws.glue/PaginationToken string?)
(s/def :cognitect.aws.glue/ClassifierList (s/coll-of :cognitect.aws.glue/Classifier))
(s/def :cognitect.aws.glue/StopWorkflowRunResponse (s/keys))
(s/def
:cognitect.aws.glue/GetDataflowGraphResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetDataflowGraphResponse/DagNodes
:cognitect.aws.glue.GetDataflowGraphResponse/DagEdges]))
(s/def
:cognitect.aws.glue/GetStatementResponse
(s/keys :opt-un [:cognitect.aws.glue.GetStatementResponse/Statement]))
(s/def
:cognitect.aws.glue/RenameField
(s/keys
:req-un
[:cognitect.aws.glue.RenameField/Name
:cognitect.aws.glue.RenameField/Inputs
:cognitect.aws.glue.RenameField/SourcePath
:cognitect.aws.glue.RenameField/TargetPath]))
(s/def
:cognitect.aws.glue/DecimalNumber
(s/keys
:req-un
[:cognitect.aws.glue.DecimalNumber/UnscaledValue :cognitect.aws.glue.DecimalNumber/Scale]))
(s/def
:cognitect.aws.glue/RegistryId
(s/keys
:opt-un
[:cognitect.aws.glue.RegistryId/RegistryArn :cognitect.aws.glue.RegistryId/RegistryName]))
(s/def
:cognitect.aws.glue/AdditionalOptionKeys
(s/spec string? :gen #(s/gen #{"performanceTuning.caching"})))
(s/def :cognitect.aws.glue/ImportCatalogToGlueResponse (s/keys))
(s/def
:cognitect.aws.glue/StopSessionRequest
(s/keys
:req-un
[:cognitect.aws.glue.StopSessionRequest/Id]
:opt-un
[:cognitect.aws.glue.StopSessionRequest/RequestOrigin]))
(s/def
:cognitect.aws.glue/S3DirectSourceAdditionalOptions
(s/keys
:opt-un
[:cognitect.aws.glue.S3DirectSourceAdditionalOptions/SamplePath
:cognitect.aws.glue.S3DirectSourceAdditionalOptions/BoundedFiles
:cognitect.aws.glue.S3DirectSourceAdditionalOptions/BoundedSize
:cognitect.aws.glue.S3DirectSourceAdditionalOptions/EnableSamplePath]))
(s/def
:cognitect.aws.glue/MySQLCatalogTarget
(s/keys
:req-un
[:cognitect.aws.glue.MySQLCatalogTarget/Name
:cognitect.aws.glue.MySQLCatalogTarget/Inputs
:cognitect.aws.glue.MySQLCatalogTarget/Database
:cognitect.aws.glue.MySQLCatalogTarget/Table]))
(s/def
:cognitect.aws.glue/GetTriggersResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetTriggersResponse/NextToken
:cognitect.aws.glue.GetTriggersResponse/Triggers]))
(s/def
:cognitect.aws.glue/AggregateOperations
(s/coll-of :cognitect.aws.glue/AggregateOperation :min-count 1 :max-count 30))
(s/def
:cognitect.aws.glue/CancelMLTaskRunRequest
(s/keys
:req-un
[:cognitect.aws.glue.CancelMLTaskRunRequest/TransformId
:cognitect.aws.glue.CancelMLTaskRunRequest/TaskRunId]))
(s/def :cognitect.aws.glue/TriggerNameList (s/coll-of :cognitect.aws.glue/NameString))
(s/def
:cognitect.aws.glue/GenericLimitedString
(s/spec #(re-matches (re-pattern "[A-Za-z0-9_-]*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/DeleteTriggerResponse
(s/keys :opt-un [:cognitect.aws.glue.DeleteTriggerResponse/Name]))
(s/def
:cognitect.aws.glue/GetDataQualityRulesetRequest
(s/keys :req-un [:cognitect.aws.glue.GetDataQualityRulesetRequest/Name]))
(s/def :cognitect.aws.glue/StatementList (s/coll-of :cognitect.aws.glue/Statement))
(s/def
:cognitect.aws.glue/PutSchemaVersionMetadataInput
(s/keys
:req-un
[:cognitect.aws.glue.PutSchemaVersionMetadataInput/MetadataKeyValue]
:opt-un
[:cognitect.aws.glue.PutSchemaVersionMetadataInput/SchemaVersionNumber
:cognitect.aws.glue.PutSchemaVersionMetadataInput/SchemaId
:cognitect.aws.glue.PutSchemaVersionMetadataInput/SchemaVersionId]))
(s/def :cognitect.aws.glue/IcebergTargetList (s/coll-of :cognitect.aws.glue/IcebergTarget))
(s/def
:cognitect.aws.glue/UpdateUserDefinedFunctionRequest
(s/keys
:req-un
[:cognitect.aws.glue.UpdateUserDefinedFunctionRequest/DatabaseName
:cognitect.aws.glue.UpdateUserDefinedFunctionRequest/FunctionName
:cognitect.aws.glue.UpdateUserDefinedFunctionRequest/FunctionInput]
:opt-un
[:cognitect.aws.glue.UpdateUserDefinedFunctionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/GetResourcePolicyResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetResourcePolicyResponse/PolicyInJson
:cognitect.aws.glue.GetResourcePolicyResponse/UpdateTime
:cognitect.aws.glue.GetResourcePolicyResponse/CreateTime
:cognitect.aws.glue.GetResourcePolicyResponse/PolicyHash]))
(s/def
:cognitect.aws.glue/SchemaVersionIdString
(s/spec
#(re-matches (re-pattern "[a-f0-9]{8}-[a-f0-9]{4}-[a-f0-9]{4}-[a-f0-9]{4}-[a-f0-9]{12}") %)
:gen
#(gen/string)))
(s/def :cognitect.aws.glue/CancelDataQualityRulesetEvaluationRunResponse (s/keys))
(s/def
:cognitect.aws.glue/CreateScriptRequest
(s/keys
:opt-un
[:cognitect.aws.glue.CreateScriptRequest/DagNodes
:cognitect.aws.glue.CreateScriptRequest/DagEdges
:cognitect.aws.glue.CreateScriptRequest/Language]))
(s/def
:cognitect.aws.glue/DataLakePrincipalString
(s/spec
(s/and string? #(<= 1 (count %) 255))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 255) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.glue/BackfillErrorCode
(s/spec
string?
:gen
#(s/gen
#{"MISSING_PARTITION_VALUE_ERROR"
"INTERNAL_ERROR"
"UNSUPPORTED_PARTITION_CHARACTER_ERROR"
"INVALID_PARTITION_TYPE_DATA_ERROR"
"ENCRYPTED_PARTITION_ERROR"})))
(s/def
:cognitect.aws.glue/NullValueFields
(s/coll-of :cognitect.aws.glue/NullValueField :min-count 0 :max-count 50))
(s/def
:cognitect.aws.glue/UpdateTriggerResponse
(s/keys :opt-un [:cognitect.aws.glue.UpdateTriggerResponse/Trigger]))
(s/def
:cognitect.aws.glue/GetMLTaskRunsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetMLTaskRunsResponse/NextToken
:cognitect.aws.glue.GetMLTaskRunsResponse/TaskRuns]))
(s/def
:cognitect.aws.glue/CrawlerHistory
(s/keys
:opt-un
[:cognitect.aws.glue.CrawlerHistory/MessagePrefix
:cognitect.aws.glue.CrawlerHistory/EndTime
:cognitect.aws.glue.CrawlerHistory/StartTime
:cognitect.aws.glue.CrawlerHistory/ErrorMessage
:cognitect.aws.glue.CrawlerHistory/DPUHour
:cognitect.aws.glue.CrawlerHistory/CrawlId
:cognitect.aws.glue.CrawlerHistory/LogGroup
:cognitect.aws.glue.CrawlerHistory/LogStream
:cognitect.aws.glue.CrawlerHistory/Summary
:cognitect.aws.glue.CrawlerHistory/State]))
(s/def
:cognitect.aws.glue/BatchCreatePartitionResponse
(s/keys :opt-un [:cognitect.aws.glue.BatchCreatePartitionResponse/Errors]))
(s/def
:cognitect.aws.glue/QuerySchemaVersionMetadataResponse
(s/keys
:opt-un
[:cognitect.aws.glue.QuerySchemaVersionMetadataResponse/NextToken
:cognitect.aws.glue.QuerySchemaVersionMetadataResponse/MetadataInfoMap
:cognitect.aws.glue.QuerySchemaVersionMetadataResponse/SchemaVersionId]))
(s/def :cognitect.aws.glue/Topk (s/spec (s/and int? #(<= 0 % 100)) :gen #(gen/choose 0 100)))
(s/def
:cognitect.aws.glue/MapValue
(s/map-of
:cognitect.aws.glue/GenericString
:cognitect.aws.glue/GenericString
:min-count
0
:max-count
100))
(s/def :cognitect.aws.glue/SchemaVersionList (s/coll-of :cognitect.aws.glue/SchemaVersionListItem))
(s/def
:cognitect.aws.glue/NodeId
(s/spec #(re-matches (re-pattern "[A-Za-z0-9_-]*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.glue/DropNullFields
(s/keys
:req-un
[:cognitect.aws.glue.DropNullFields/Name :cognitect.aws.glue.DropNullFields/Inputs]
:opt-un
[:cognitect.aws.glue.DropNullFields/NullCheckBoxList
:cognitect.aws.glue.DropNullFields/NullTextList]))
(s/def
:cognitect.aws.glue/CreateCsvClassifierRequest
(s/keys
:req-un
[:cognitect.aws.glue.CreateCsvClassifierRequest/Name]
:opt-un
[:cognitect.aws.glue.CreateCsvClassifierRequest/Header
:cognitect.aws.glue.CreateCsvClassifierRequest/CustomDatatypeConfigured
:cognitect.aws.glue.CreateCsvClassifierRequest/CustomDatatypes
:cognitect.aws.glue.CreateCsvClassifierRequest/Delimiter
:cognitect.aws.glue.CreateCsvClassifierRequest/QuoteSymbol
:cognitect.aws.glue.CreateCsvClassifierRequest/AllowSingleColumn
:cognitect.aws.glue.CreateCsvClassifierRequest/DisableValueTrimming
:cognitect.aws.glue.CreateCsvClassifierRequest/ContainsHeader]))
(s/def
:cognitect.aws.glue/StartJobRunResponse
(s/keys :opt-un [:cognitect.aws.glue.StartJobRunResponse/JobRunId]))
(s/def
:cognitect.aws.glue/DeleteDatabaseRequest
(s/keys
:req-un
[:cognitect.aws.glue.DeleteDatabaseRequest/Name]
:opt-un
[:cognitect.aws.glue.DeleteDatabaseRequest/CatalogId]))
(s/def
:cognitect.aws.glue/ColumnNameString
(s/spec
#(re-matches
(re-pattern "[\\u0020-\\uD7FF\\uE000-\\uFFFD\\uD800\\uDC00-\\uDBFF\\uDFFF\\t]*")
%)
:gen
#(gen/string)))
(s/def :cognitect.aws.glue/CrawlId string?)
(s/def :cognitect.aws.glue/UpdateTableResponse (s/keys))
(s/def
:cognitect.aws.glue/ListDataQualityRuleRecommendationRunsResponse
(s/keys
:opt-un
[:cognitect.aws.glue.ListDataQualityRuleRecommendationRunsResponse/NextToken
:cognitect.aws.glue.ListDataQualityRuleRecommendationRunsResponse/Runs]))
(s/def
:cognitect.aws.glue/BatchGetTriggersRequest
(s/keys :req-un [:cognitect.aws.glue.BatchGetTriggersRequest/TriggerNames]))
(s/def :cognitect.aws.glue/CustomEntityTypes (s/coll-of :cognitect.aws.glue/CustomEntityType))
(s/def
:cognitect.aws.glue/CreateJobResponse
(s/keys :opt-un [:cognitect.aws.glue.CreateJobResponse/Name]))
(s/def
:cognitect.aws.glue/BatchGetPartitionRequest
(s/keys
:req-un
[:cognitect.aws.glue.BatchGetPartitionRequest/DatabaseName
:cognitect.aws.glue.BatchGetPartitionRequest/TableName
:cognitect.aws.glue.BatchGetPartitionRequest/PartitionsToGet]
:opt-un
[:cognitect.aws.glue.BatchGetPartitionRequest/CatalogId]))
(s/def :cognitect.aws.glue/VersionId (s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def :cognitect.aws.glue/DeleteResourcePolicyResponse (s/keys))
(s/def
:cognitect.aws.glue/CreateMLTransformResponse
(s/keys :opt-un [:cognitect.aws.glue.CreateMLTransformResponse/TransformId]))
(s/def
:cognitect.aws.glue/TaskStatusType
(s/spec
string?
:gen
#(s/gen #{"TIMEOUT" "SUCCEEDED" "STOPPED" "STARTING" "FAILED" "STOPPING" "RUNNING"})))
(s/def :cognitect.aws.glue/UpdateUserDefinedFunctionResponse (s/keys))
(s/def
:cognitect.aws.glue/DataQualityRulesetEvaluationRunDescription
(s/keys
:opt-un
[:cognitect.aws.glue.DataQualityRulesetEvaluationRunDescription/RunId
:cognitect.aws.glue.DataQualityRulesetEvaluationRunDescription/Status
:cognitect.aws.glue.DataQualityRulesetEvaluationRunDescription/DataSource
:cognitect.aws.glue.DataQualityRulesetEvaluationRunDescription/StartedOn]))
(s/def
:cognitect.aws.glue/BatchDeleteTableNameList
(s/coll-of :cognitect.aws.glue/NameString :min-count 0 :max-count 100))
(s/def
:cognitect.aws.glue/GetColumnStatisticsForPartitionResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetColumnStatisticsForPartitionResponse/ColumnStatisticsList
:cognitect.aws.glue.GetColumnStatisticsForPartitionResponse/Errors]))
(s/def
:cognitect.aws.glue/GetUserDefinedFunctionResponse
(s/keys :opt-un [:cognitect.aws.glue.GetUserDefinedFunctionResponse/UserDefinedFunction]))
(s/def
:cognitect.aws.glue/StopTriggerResponse
(s/keys :opt-un [:cognitect.aws.glue.StopTriggerResponse/Name]))
(s/def
:cognitect.aws.glue/BatchGetBlueprintNames
(s/coll-of :cognitect.aws.glue/OrchestrationNameString :min-count 1 :max-count 25))
(s/def
:cognitect.aws.glue/DeleteSchemaVersionsResponse
(s/keys :opt-un [:cognitect.aws.glue.DeleteSchemaVersionsResponse/SchemaVersionErrors]))
(s/def
:cognitect.aws.glue/MetadataInfoMap
(s/map-of :cognitect.aws.glue/MetadataKeyString :cognitect.aws.glue/MetadataInfo))
(s/def
:cognitect.aws.glue/OrchestrationArgumentsMap
(s/map-of
:cognitect.aws.glue/OrchestrationNameString
:cognitect.aws.glue/OrchestrationArgumentsValue
:min-count
0
:max-count
75))
(s/def :cognitect.aws.glue/CodeGenNodeType string?)
(s/def
:cognitect.aws.glue/DeleteConnectionNameList
(s/coll-of :cognitect.aws.glue/NameString :min-count 0 :max-count 25))
(s/def
:cognitect.aws.glue/BoxedNonNegativeInt
(s/spec (s/and int? #(<= 0 %)) :gen #(gen/choose 0 Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/TotalSegmentsInteger
(s/spec (s/and int? #(<= 1 % 10)) :gen #(gen/choose 1 10)))
(s/def
:cognitect.aws.glue/BatchStopJobRunError
(s/keys
:opt-un
[:cognitect.aws.glue.BatchStopJobRunError/JobName
:cognitect.aws.glue.BatchStopJobRunError/JobRunId
:cognitect.aws.glue.BatchStopJobRunError/ErrorDetail]))
(s/def
:cognitect.aws.glue/AttemptCount
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.glue/JobNodeDetails
(s/keys :opt-un [:cognitect.aws.glue.JobNodeDetails/JobRuns]))
(s/def
:cognitect.aws.glue/StatementOutput
(s/keys
:opt-un
[:cognitect.aws.glue.StatementOutput/ExecutionCount
:cognitect.aws.glue.StatementOutput/Status
:cognitect.aws.glue.StatementOutput/ErrorName
:cognitect.aws.glue.StatementOutput/Traceback
:cognitect.aws.glue.StatementOutput/Data
:cognitect.aws.glue.StatementOutput/ErrorValue]))
(s/def
:cognitect.aws.glue/GetCrawlersResponse
(s/keys
:opt-un
[:cognitect.aws.glue.GetCrawlersResponse/Crawlers
:cognitect.aws.glue.GetCrawlersResponse/NextToken]))
(s/def
:cognitect.aws.glue/CustomCode
(s/keys
:req-un
[:cognitect.aws.glue.CustomCode/Name
:cognitect.aws.glue.CustomCode/Inputs
:cognitect.aws.glue.CustomCode/Code
:cognitect.aws.glue.CustomCode/ClassName]
:opt-un
[:cognitect.aws.glue.CustomCode/OutputSchemas]))
(s/def
:cognitect.aws.glue/MetadataKeyValuePair
(s/keys
:opt-un
[:cognitect.aws.glue.MetadataKeyValuePair/MetadataKey
:cognitect.aws.glue.MetadataKeyValuePair/MetadataValue]))
(s/def
:cognitect.aws.glue/DeleteJobRequest
(s/keys :req-un [:cognitect.aws.glue.DeleteJobRequest/JobName]))
(s/def
:cognitect.aws.glue/GetConnectionRequest
(s/keys
:req-un
[:cognitect.aws.glue.GetConnectionRequest/Name]
:opt-un
[:cognitect.aws.glue.GetConnectionRequest/HidePassword
:cognitect.aws.glue.GetConnectionRequest/CatalogId]))
(s/def
:cognitect.aws.glue/Order
(s/keys :req-un [:cognitect.aws.glue.Order/Column :cognitect.aws.glue.Order/SortOrder]))
(s/def
:cognitect.aws.glue/CodeGenNodeArg
(s/keys
:req-un
[:cognitect.aws.glue.CodeGenNodeArg/Name :cognitect.aws.glue.CodeGenNodeArg/Value]
:opt-un
[:cognitect.aws.glue.CodeGenNodeArg/Param]))
(s/def
:cognitect.aws.glue/ListMLTransformsResponse
(s/keys
:req-un
[:cognitect.aws.glue.ListMLTransformsResponse/TransformIds]
:opt-un
[:cognitect.aws.glue.ListMLTransformsResponse/NextToken]))
(s/def :cognitect.aws.glue.GetWorkflowRunsResponse/Runs :cognitect.aws.glue/WorkflowRuns)
(s/def :cognitect.aws.glue.GetWorkflowRunsResponse/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.GetTriggerRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.BatchGetDevEndpointsRequest/DevEndpointNames
:cognitect.aws.glue/DevEndpointNames)
(s/def :cognitect.aws.glue.StopWorkflowRunRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.StopWorkflowRunRequest/RunId :cognitect.aws.glue/IdString)
(s/def
:cognitect.aws.glue.UpdateColumnStatisticsForTableRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.UpdateColumnStatisticsForTableRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateColumnStatisticsForTableRequest/TableName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateColumnStatisticsForTableRequest/ColumnStatisticsList
:cognitect.aws.glue/UpdateColumnStatisticsList)
(s/def :cognitect.aws.glue.GetBlueprintResponse/Blueprint :cognitect.aws.glue/Blueprint)
(s/def
:cognitect.aws.glue.DeleteResourcePolicyRequest/PolicyHashCondition
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.DeleteResourcePolicyRequest/ResourceArn
:cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.RegisterSchemaVersionInput/SchemaId :cognitect.aws.glue/SchemaId)
(s/def
:cognitect.aws.glue.RegisterSchemaVersionInput/SchemaDefinition
:cognitect.aws.glue/SchemaDefinitionString)
(s/def
:cognitect.aws.glue.DeleteColumnStatisticsForPartitionRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.DeleteColumnStatisticsForPartitionRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.DeleteColumnStatisticsForPartitionRequest/TableName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.DeleteColumnStatisticsForPartitionRequest/PartitionValues
:cognitect.aws.glue/ValueStringList)
(s/def
:cognitect.aws.glue.DeleteColumnStatisticsForPartitionRequest/ColumnName
:cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UpdateMLTransformResponse/TransformId :cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.CreateXMLClassifierRequest/Classification
:cognitect.aws.glue/Classification)
(s/def :cognitect.aws.glue.CreateXMLClassifierRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateXMLClassifierRequest/RowTag :cognitect.aws.glue/RowTag)
(s/def :cognitect.aws.glue.GetDataQualityRulesetResponse/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetResponse/Description
:cognitect.aws.glue/DescriptionString)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetResponse/Ruleset
:cognitect.aws.glue/DataQualityRulesetString)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetResponse/TargetTable
:cognitect.aws.glue/DataQualityTargetTable)
(s/def :cognitect.aws.glue.GetDataQualityRulesetResponse/CreatedOn :cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetResponse/LastModifiedOn
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetResponse/RecommendationRunId
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.UpdateJobFromSourceControlResponse/JobName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.RegisterSchemaVersionResponse/SchemaVersionId
:cognitect.aws.glue/SchemaVersionIdString)
(s/def
:cognitect.aws.glue.RegisterSchemaVersionResponse/VersionNumber
:cognitect.aws.glue/VersionLongNumber)
(s/def
:cognitect.aws.glue.RegisterSchemaVersionResponse/Status
:cognitect.aws.glue/SchemaVersionStatus)
(s/def :cognitect.aws.glue.StopSessionResponse/Id :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/SchemaArn
:cognitect.aws.glue/GlueResourceArn)
(s/def
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/SchemaName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/RegistryName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/LatestVersion
:cognitect.aws.glue/LatestSchemaVersionBoolean)
(s/def
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/VersionNumber
:cognitect.aws.glue/VersionLongNumber)
(s/def
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/SchemaVersionId
:cognitect.aws.glue/SchemaVersionIdString)
(s/def
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/MetadataKey
:cognitect.aws.glue/MetadataKeyString)
(s/def
:cognitect.aws.glue.RemoveSchemaVersionMetadataResponse/MetadataValue
:cognitect.aws.glue/MetadataValueString)
(s/def
:cognitect.aws.glue.ListCustomEntityTypesRequest/NextToken
:cognitect.aws.glue/PaginationToken)
(s/def :cognitect.aws.glue.ListCustomEntityTypesRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.ListCustomEntityTypesRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.CatalogKafkaSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.CatalogKafkaSource/WindowSize :cognitect.aws.glue/BoxedPositiveInt)
(s/def :cognitect.aws.glue.CatalogKafkaSource/DetectSchema :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.CatalogKafkaSource/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.CatalogKafkaSource/Database :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.CatalogKafkaSource/StreamingOptions
:cognitect.aws.glue/KafkaStreamingSourceOptions)
(s/def
:cognitect.aws.glue.CatalogKafkaSource/DataPreviewOptions
:cognitect.aws.glue/StreamingDataPreviewOptions)
(s/def
:cognitect.aws.glue.BatchUpdatePartitionRequestEntry/PartitionValueList
:cognitect.aws.glue/BoundedPartitionValueList)
(s/def
:cognitect.aws.glue.BatchUpdatePartitionRequestEntry/PartitionInput
:cognitect.aws.glue/PartitionInput)
(s/def :cognitect.aws.glue.ExportLabelsTaskRunProperties/OutputS3Path :cognitect.aws.glue/UriString)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/CatalogKafkaSource
:cognitect.aws.glue/CatalogKafkaSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/EvaluateDataQuality
:cognitect.aws.glue/EvaluateDataQuality)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/DirectKafkaSource
:cognitect.aws.glue/DirectKafkaSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/CatalogDeltaSource
:cognitect.aws.glue/CatalogDeltaSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/MicrosoftSQLServerCatalogTarget
:cognitect.aws.glue/MicrosoftSQLServerCatalogTarget)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/FillMissingValues
:cognitect.aws.glue/FillMissingValues)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/SplitFields :cognitect.aws.glue/SplitFields)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/JDBCConnectorTarget
:cognitect.aws.glue/JDBCConnectorTarget)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/SparkConnectorTarget
:cognitect.aws.glue/SparkConnectorTarget)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/AmazonRedshiftSource
:cognitect.aws.glue/AmazonRedshiftSource)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/Filter :cognitect.aws.glue/Filter)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/GovernedCatalogSource
:cognitect.aws.glue/GovernedCatalogSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/CatalogTarget
:cognitect.aws.glue/BasicCatalogTarget)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/GovernedCatalogTarget
:cognitect.aws.glue/GovernedCatalogTarget)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/AthenaConnectorSource
:cognitect.aws.glue/AthenaConnectorSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/OracleSQLCatalogSource
:cognitect.aws.glue/OracleSQLCatalogSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/S3CatalogSource
:cognitect.aws.glue/S3CatalogSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/PostgreSQLCatalogSource
:cognitect.aws.glue/PostgreSQLCatalogSource)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/DropFields :cognitect.aws.glue/DropFields)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/CatalogHudiSource
:cognitect.aws.glue/CatalogHudiSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/CatalogKinesisSource
:cognitect.aws.glue/CatalogKinesisSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/S3HudiDirectTarget
:cognitect.aws.glue/S3HudiDirectTarget)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/S3GlueParquetTarget
:cognitect.aws.glue/S3GlueParquetTarget)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/SparkConnectorSource
:cognitect.aws.glue/SparkConnectorSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/DirectJDBCSource
:cognitect.aws.glue/DirectJDBCSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/RedshiftTarget
:cognitect.aws.glue/RedshiftTarget)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/DirectKinesisSource
:cognitect.aws.glue/DirectKinesisSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/AmazonRedshiftTarget
:cognitect.aws.glue/AmazonRedshiftTarget)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/EvaluateDataQualityMultiFrame
:cognitect.aws.glue/EvaluateDataQualityMultiFrame)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/Aggregate :cognitect.aws.glue/Aggregate)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/S3HudiCatalogTarget
:cognitect.aws.glue/S3HudiCatalogTarget)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/CatalogSource :cognitect.aws.glue/CatalogSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/S3DeltaDirectTarget
:cognitect.aws.glue/S3DeltaDirectTarget)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/SparkSQL :cognitect.aws.glue/SparkSQL)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/S3DeltaSource :cognitect.aws.glue/S3DeltaSource)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/S3CsvSource :cognitect.aws.glue/S3CsvSource)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/S3HudiSource :cognitect.aws.glue/S3HudiSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/DynamoDBCatalogSource
:cognitect.aws.glue/DynamoDBCatalogSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/S3CatalogHudiSource
:cognitect.aws.glue/S3CatalogHudiSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/PostgreSQLCatalogTarget
:cognitect.aws.glue/PostgreSQLCatalogTarget)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/MySQLCatalogSource
:cognitect.aws.glue/MySQLCatalogSource)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/Spigot :cognitect.aws.glue/Spigot)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/DropDuplicates
:cognitect.aws.glue/DropDuplicates)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/Join :cognitect.aws.glue/Join)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/SelectFields :cognitect.aws.glue/SelectFields)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/ApplyMapping :cognitect.aws.glue/ApplyMapping)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/RelationalCatalogSource
:cognitect.aws.glue/RelationalCatalogSource)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/Union :cognitect.aws.glue/Union)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/DynamicTransform
:cognitect.aws.glue/DynamicTransform)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/RedshiftSource
:cognitect.aws.glue/RedshiftSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/S3ParquetSource
:cognitect.aws.glue/S3ParquetSource)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/PIIDetection :cognitect.aws.glue/PIIDetection)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/SelectFromCollection
:cognitect.aws.glue/SelectFromCollection)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/S3CatalogTarget
:cognitect.aws.glue/S3CatalogTarget)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/OracleSQLCatalogTarget
:cognitect.aws.glue/OracleSQLCatalogTarget)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/Merge :cognitect.aws.glue/Merge)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/S3JsonSource :cognitect.aws.glue/S3JsonSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/S3DeltaCatalogTarget
:cognitect.aws.glue/S3DeltaCatalogTarget)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/S3CatalogDeltaSource
:cognitect.aws.glue/S3CatalogDeltaSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/JDBCConnectorSource
:cognitect.aws.glue/JDBCConnectorSource)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/S3DirectTarget
:cognitect.aws.glue/S3DirectTarget)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/MicrosoftSQLServerCatalogSource
:cognitect.aws.glue/MicrosoftSQLServerCatalogSource)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/RenameField :cognitect.aws.glue/RenameField)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/MySQLCatalogTarget
:cognitect.aws.glue/MySQLCatalogTarget)
(s/def
:cognitect.aws.glue.CodeGenConfigurationNode/DropNullFields
:cognitect.aws.glue/DropNullFields)
(s/def :cognitect.aws.glue.CodeGenConfigurationNode/CustomCode :cognitect.aws.glue/CustomCode)
(s/def :cognitect.aws.glue.TagResourceRequest/ResourceArn :cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.TagResourceRequest/TagsToAdd :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.GetCrawlerResponse/Crawler :cognitect.aws.glue/Crawler)
(s/def
:cognitect.aws.glue.FindMatchesMetrics/AreaUnderPRCurve
:cognitect.aws.glue/GenericBoundedDouble)
(s/def :cognitect.aws.glue.FindMatchesMetrics/Precision :cognitect.aws.glue/GenericBoundedDouble)
(s/def :cognitect.aws.glue.FindMatchesMetrics/Recall :cognitect.aws.glue/GenericBoundedDouble)
(s/def :cognitect.aws.glue.FindMatchesMetrics/F1 :cognitect.aws.glue/GenericBoundedDouble)
(s/def :cognitect.aws.glue.FindMatchesMetrics/ConfusionMatrix :cognitect.aws.glue/ConfusionMatrix)
(s/def
:cognitect.aws.glue.FindMatchesMetrics/ColumnImportances
:cognitect.aws.glue/ColumnImportanceList)
(s/def :cognitect.aws.glue.JobBookmarkEntry/JobName :cognitect.aws.glue/JobName)
(s/def :cognitect.aws.glue.JobBookmarkEntry/Version :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.JobBookmarkEntry/Run :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.JobBookmarkEntry/Attempt :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.JobBookmarkEntry/PreviousRunId :cognitect.aws.glue/RunId)
(s/def :cognitect.aws.glue.JobBookmarkEntry/RunId :cognitect.aws.glue/RunId)
(s/def :cognitect.aws.glue.JobBookmarkEntry/JobBookmark :cognitect.aws.glue/JsonValue)
(s/def :cognitect.aws.glue.JobRun/PreviousRunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.JobRun/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.JobRun/AllocatedCapacity :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.JobRun/Timeout :cognitect.aws.glue/Timeout)
(s/def :cognitect.aws.glue.JobRun/ExecutionTime :cognitect.aws.glue/ExecutionTime)
(s/def :cognitect.aws.glue.JobRun/NumberOfWorkers :cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.JobRun/ErrorMessage :cognitect.aws.glue/ErrorString)
(s/def :cognitect.aws.glue.JobRun/SecurityConfiguration :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.JobRun/Id :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.JobRun/MaxCapacity :cognitect.aws.glue/NullableDouble)
(s/def :cognitect.aws.glue.JobRun/DPUSeconds :cognitect.aws.glue/NullableDouble)
(s/def :cognitect.aws.glue.JobRun/CompletedOn :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.JobRun/NotificationProperty :cognitect.aws.glue/NotificationProperty)
(s/def :cognitect.aws.glue.JobRun/LogGroupName :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.JobRun/LastModifiedOn :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.JobRun/StartedOn :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.JobRun/Arguments :cognitect.aws.glue/GenericMap)
(s/def :cognitect.aws.glue.JobRun/WorkerType :cognitect.aws.glue/WorkerType)
(s/def :cognitect.aws.glue.JobRun/Attempt :cognitect.aws.glue/AttemptCount)
(s/def :cognitect.aws.glue.JobRun/ExecutionClass :cognitect.aws.glue/ExecutionClass)
(s/def :cognitect.aws.glue.JobRun/JobRunState :cognitect.aws.glue/JobRunState)
(s/def :cognitect.aws.glue.JobRun/TriggerName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.JobRun/PredecessorRuns :cognitect.aws.glue/PredecessorList)
(s/def :cognitect.aws.glue.JobRun/GlueVersion :cognitect.aws.glue/GlueVersionString)
(s/def :cognitect.aws.glue.EvaluateDataQuality/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.EvaluateDataQuality/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.EvaluateDataQuality/Ruleset :cognitect.aws.glue/DQDLString)
(s/def :cognitect.aws.glue.EvaluateDataQuality/Output :cognitect.aws.glue/DQTransformOutput)
(s/def
:cognitect.aws.glue.EvaluateDataQuality/PublishingOptions
:cognitect.aws.glue/DQResultsPublishingOptions)
(s/def
:cognitect.aws.glue.EvaluateDataQuality/StopJobOnFailureOptions
:cognitect.aws.glue/DQStopJobOnFailureOptions)
(s/def
:cognitect.aws.glue.DataLakePrincipal/DataLakePrincipalIdentifier
:cognitect.aws.glue/DataLakePrincipalString)
(s/def :cognitect.aws.glue.BatchGetWorkflowsRequest/Names :cognitect.aws.glue/WorkflowNames)
(s/def
:cognitect.aws.glue.BatchGetWorkflowsRequest/IncludeGraph
:cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.DirectKafkaSource/Name :cognitect.aws.glue/NodeName)
(s/def
:cognitect.aws.glue.DirectKafkaSource/StreamingOptions
:cognitect.aws.glue/KafkaStreamingSourceOptions)
(s/def :cognitect.aws.glue.DirectKafkaSource/WindowSize :cognitect.aws.glue/BoxedPositiveInt)
(s/def :cognitect.aws.glue.DirectKafkaSource/DetectSchema :cognitect.aws.glue/BoxedBoolean)
(s/def
:cognitect.aws.glue.DirectKafkaSource/DataPreviewOptions
:cognitect.aws.glue/StreamingDataPreviewOptions)
(s/def :cognitect.aws.glue.UpdateTableRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.UpdateTableRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UpdateTableRequest/TableInput :cognitect.aws.glue/TableInput)
(s/def :cognitect.aws.glue.UpdateTableRequest/SkipArchive :cognitect.aws.glue/BooleanNullable)
(s/def :cognitect.aws.glue.UpdateTableRequest/TransactionId :cognitect.aws.glue/TransactionIdString)
(s/def :cognitect.aws.glue.UpdateTableRequest/VersionId :cognitect.aws.glue/VersionString)
(s/def :cognitect.aws.glue.CreateBlueprintRequest/Name :cognitect.aws.glue/OrchestrationNameString)
(s/def
:cognitect.aws.glue.CreateBlueprintRequest/Description
:cognitect.aws.glue/Generic512CharString)
(s/def
:cognitect.aws.glue.CreateBlueprintRequest/BlueprintLocation
:cognitect.aws.glue/OrchestrationS3Location)
(s/def :cognitect.aws.glue.CreateBlueprintRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.StartCrawlerRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CatalogDeltaSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.CatalogDeltaSource/Database :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.CatalogDeltaSource/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.CatalogDeltaSource/AdditionalDeltaOptions
:cognitect.aws.glue/AdditionalOptions)
(s/def :cognitect.aws.glue.CatalogDeltaSource/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def :cognitect.aws.glue.UpdateSchemaInput/SchemaId :cognitect.aws.glue/SchemaId)
(s/def
:cognitect.aws.glue.UpdateSchemaInput/SchemaVersionNumber
:cognitect.aws.glue/SchemaVersionNumber)
(s/def :cognitect.aws.glue.UpdateSchemaInput/Compatibility :cognitect.aws.glue/Compatibility)
(s/def :cognitect.aws.glue.UpdateSchemaInput/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.ListDevEndpointsRequest/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.ListDevEndpointsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.ListDevEndpointsRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.ListTriggersRequest/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.ListTriggersRequest/DependentJobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.ListTriggersRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.ListTriggersRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.GetClassifierRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateSchemaInput/RegistryId :cognitect.aws.glue/RegistryId)
(s/def
:cognitect.aws.glue.CreateSchemaInput/SchemaName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.CreateSchemaInput/DataFormat :cognitect.aws.glue/DataFormat)
(s/def :cognitect.aws.glue.CreateSchemaInput/Compatibility :cognitect.aws.glue/Compatibility)
(s/def :cognitect.aws.glue.CreateSchemaInput/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.CreateSchemaInput/Tags :cognitect.aws.glue/TagsMap)
(s/def
:cognitect.aws.glue.CreateSchemaInput/SchemaDefinition
:cognitect.aws.glue/SchemaDefinitionString)
(s/def :cognitect.aws.glue.MicrosoftSQLServerCatalogTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.MicrosoftSQLServerCatalogTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def
:cognitect.aws.glue.MicrosoftSQLServerCatalogTarget/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.MicrosoftSQLServerCatalogTarget/Table
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.DeleteSessionResponse/Id :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.TaskRunFilterCriteria/TaskRunType :cognitect.aws.glue/TaskType)
(s/def :cognitect.aws.glue.TaskRunFilterCriteria/Status :cognitect.aws.glue/TaskStatusType)
(s/def :cognitect.aws.glue.TaskRunFilterCriteria/StartedBefore :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.TaskRunFilterCriteria/StartedAfter :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.ResetJobBookmarkRequest/JobName :cognitect.aws.glue/JobName)
(s/def :cognitect.aws.glue.ResetJobBookmarkRequest/RunId :cognitect.aws.glue/RunId)
(s/def :cognitect.aws.glue.WorkflowGraph/Nodes :cognitect.aws.glue/NodeList)
(s/def :cognitect.aws.glue.WorkflowGraph/Edges :cognitect.aws.glue/EdgeList)
(s/def :cognitect.aws.glue.UpdateCrawlerScheduleRequest/CrawlerName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UpdateCrawlerScheduleRequest/Schedule :cognitect.aws.glue/CronExpression)
(s/def :cognitect.aws.glue.NullCheckBoxList/IsEmpty :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.NullCheckBoxList/IsNullString :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.NullCheckBoxList/IsNegOne :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.FillMissingValues/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.FillMissingValues/Inputs :cognitect.aws.glue/OneInput)
(s/def
:cognitect.aws.glue.FillMissingValues/ImputedPath
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.FillMissingValues/FilledPath
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.SplitFields/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.SplitFields/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.SplitFields/Paths :cognitect.aws.glue/GlueStudioPathList)
(s/def :cognitect.aws.glue.StartTriggerRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetConnectionsRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.GetConnectionsRequest/Filter :cognitect.aws.glue/GetConnectionsFilter)
(s/def :cognitect.aws.glue.GetConnectionsRequest/HidePassword :cognitect.aws.glue/Boolean)
(s/def :cognitect.aws.glue.GetConnectionsRequest/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.GetConnectionsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def
:cognitect.aws.glue.GetSecurityConfigurationResponse/SecurityConfiguration
:cognitect.aws.glue/SecurityConfiguration)
(s/def
:cognitect.aws.glue.ListCustomEntityTypesResponse/CustomEntityTypes
:cognitect.aws.glue/CustomEntityTypes)
(s/def
:cognitect.aws.glue.ListCustomEntityTypesResponse/NextToken
:cognitect.aws.glue/PaginationToken)
(s/def :cognitect.aws.glue.BackfillError/Code :cognitect.aws.glue/BackfillErrorCode)
(s/def
:cognitect.aws.glue.BackfillError/Partitions
:cognitect.aws.glue/BackfillErroredPartitionsList)
(s/def :cognitect.aws.glue.EventBatchingCondition/BatchSize :cognitect.aws.glue/BatchSize)
(s/def :cognitect.aws.glue.EventBatchingCondition/BatchWindow :cognitect.aws.glue/BatchWindow)
(s/def
:cognitect.aws.glue.StartingEventBatchCondition/BatchSize
:cognitect.aws.glue/NullableInteger)
(s/def
:cognitect.aws.glue.StartingEventBatchCondition/BatchWindow
:cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.BatchStopJobRunRequest/JobName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.BatchStopJobRunRequest/JobRunIds
:cognitect.aws.glue/BatchStopJobRunJobRunIdList)
(s/def
:cognitect.aws.glue.GetPartitionIndexesResponse/PartitionIndexDescriptorList
:cognitect.aws.glue/PartitionIndexDescriptorList)
(s/def :cognitect.aws.glue.GetPartitionIndexesResponse/NextToken :cognitect.aws.glue/Token)
(s/def
:cognitect.aws.glue.GetCatalogImportStatusRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.DeleteTriggerRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CancelMLTaskRunResponse/TransformId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.CancelMLTaskRunResponse/TaskRunId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.CancelMLTaskRunResponse/Status :cognitect.aws.glue/TaskStatusType)
(s/def :cognitect.aws.glue.MongoDBTarget/ConnectionName :cognitect.aws.glue/ConnectionName)
(s/def :cognitect.aws.glue.MongoDBTarget/Path :cognitect.aws.glue/Path)
(s/def :cognitect.aws.glue.MongoDBTarget/ScanAll :cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.DeltaTarget/DeltaTables :cognitect.aws.glue/PathList)
(s/def :cognitect.aws.glue.DeltaTarget/ConnectionName :cognitect.aws.glue/ConnectionName)
(s/def :cognitect.aws.glue.DeltaTarget/WriteManifest :cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.DeltaTarget/CreateNativeDeltaTable :cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.ListSchemasResponse/Schemas :cognitect.aws.glue/SchemaListDefinition)
(s/def
:cognitect.aws.glue.ListSchemasResponse/NextToken
:cognitect.aws.glue/SchemaRegistryTokenString)
(s/def :cognitect.aws.glue.JDBCConnectorTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.JDBCConnectorTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def
:cognitect.aws.glue.JDBCConnectorTarget/ConnectionName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.JDBCConnectorTarget/ConnectionTable
:cognitect.aws.glue/EnclosedInStringPropertyWithQuote)
(s/def
:cognitect.aws.glue.JDBCConnectorTarget/ConnectorName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.JDBCConnectorTarget/ConnectionType
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.JDBCConnectorTarget/AdditionalOptions
:cognitect.aws.glue/AdditionalOptions)
(s/def :cognitect.aws.glue.JDBCConnectorTarget/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def
:cognitect.aws.glue.LineageConfiguration/CrawlerLineageSettings
:cognitect.aws.glue/CrawlerLineageSettings)
(s/def :cognitect.aws.glue.UpdateDatabaseRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.UpdateDatabaseRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UpdateDatabaseRequest/DatabaseInput :cognitect.aws.glue/DatabaseInput)
(s/def :cognitect.aws.glue.ErrorDetail/ErrorCode :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.ErrorDetail/ErrorMessage :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.CreateRegistryResponse/RegistryArn :cognitect.aws.glue/GlueResourceArn)
(s/def
:cognitect.aws.glue.CreateRegistryResponse/RegistryName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.CreateRegistryResponse/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.CreateRegistryResponse/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.DateColumnStatisticsData/MinimumValue :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.DateColumnStatisticsData/MaximumValue :cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.DateColumnStatisticsData/NumberOfNulls
:cognitect.aws.glue/NonNegativeLong)
(s/def
:cognitect.aws.glue.DateColumnStatisticsData/NumberOfDistinctValues
:cognitect.aws.glue/NonNegativeLong)
(s/def :cognitect.aws.glue.CreatePartitionRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.CreatePartitionRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreatePartitionRequest/TableName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreatePartitionRequest/PartitionInput :cognitect.aws.glue/PartitionInput)
(s/def :cognitect.aws.glue.UpdateJobFromSourceControlRequest/JobName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/AuthStrategy
:cognitect.aws.glue/SourceControlAuthStrategy)
(s/def
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/RepositoryName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/BranchName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/AuthToken
:cognitect.aws.glue/AuthTokenString)
(s/def
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/CommitId
:cognitect.aws.glue/CommitIdString)
(s/def :cognitect.aws.glue.UpdateJobFromSourceControlRequest/Folder :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/Provider
:cognitect.aws.glue/SourceControlProvider)
(s/def
:cognitect.aws.glue.UpdateJobFromSourceControlRequest/RepositoryOwner
:cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetMLTaskRunRequest/TransformId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.GetMLTaskRunRequest/TaskRunId :cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.CreateDevEndpointResponse/AvailabilityZone
:cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.CreateDevEndpointResponse/EndpointName :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.CreateDevEndpointResponse/NumberOfNodes :cognitect.aws.glue/IntegerValue)
(s/def
:cognitect.aws.glue.CreateDevEndpointResponse/NumberOfWorkers
:cognitect.aws.glue/NullableInteger)
(s/def
:cognitect.aws.glue.CreateDevEndpointResponse/YarnEndpointAddress
:cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.CreateDevEndpointResponse/SecurityConfiguration
:cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateDevEndpointResponse/Arguments :cognitect.aws.glue/MapValue)
(s/def :cognitect.aws.glue.CreateDevEndpointResponse/WorkerType :cognitect.aws.glue/WorkerType)
(s/def :cognitect.aws.glue.CreateDevEndpointResponse/RoleArn :cognitect.aws.glue/RoleArn)
(s/def :cognitect.aws.glue.CreateDevEndpointResponse/SubnetId :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.CreateDevEndpointResponse/ExtraJarsS3Path
:cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.CreateDevEndpointResponse/ZeppelinRemoteSparkInterpreterPort
:cognitect.aws.glue/IntegerValue)
(s/def
:cognitect.aws.glue.CreateDevEndpointResponse/CreatedTimestamp
:cognitect.aws.glue/TimestampValue)
(s/def
:cognitect.aws.glue.CreateDevEndpointResponse/ExtraPythonLibsS3Path
:cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.CreateDevEndpointResponse/GlueVersion
:cognitect.aws.glue/GlueVersionString)
(s/def
:cognitect.aws.glue.CreateDevEndpointResponse/SecurityGroupIds
:cognitect.aws.glue/StringList)
(s/def
:cognitect.aws.glue.CreateDevEndpointResponse/FailureReason
:cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.CreateDevEndpointResponse/VpcId :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.CreateDevEndpointResponse/Status :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.ColumnRowFilter/ColumnName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.ColumnRowFilter/RowFilterExpression :cognitect.aws.glue/PredicateString)
(s/def :cognitect.aws.glue.GetWorkflowRunResponse/Run :cognitect.aws.glue/WorkflowRun)
(s/def
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/DataSource
:cognitect.aws.glue/DataSource)
(s/def
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/Role
:cognitect.aws.glue/RoleString)
(s/def
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/NumberOfWorkers
:cognitect.aws.glue/NullableInteger)
(s/def
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/Timeout
:cognitect.aws.glue/Timeout)
(s/def
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/ClientToken
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/AdditionalRunOptions
:cognitect.aws.glue/DataQualityEvaluationRunAdditionalRunOptions)
(s/def
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/RulesetNames
:cognitect.aws.glue/RulesetNames)
(s/def
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunRequest/AdditionalDataSources
:cognitect.aws.glue/DataSourceMap)
(s/def :cognitect.aws.glue.TableVersion/Table :cognitect.aws.glue/Table)
(s/def :cognitect.aws.glue.TableVersion/VersionId :cognitect.aws.glue/VersionString)
(s/def :cognitect.aws.glue.GetWorkflowRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetWorkflowRequest/IncludeGraph :cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.GetStatementRequest/SessionId :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetStatementRequest/Id :cognitect.aws.glue/IntegerValue)
(s/def
:cognitect.aws.glue.GetStatementRequest/RequestOrigin
:cognitect.aws.glue/OrchestrationNameString)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunRequest/RunId
:cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.GetJobsRequest/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.GetJobsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.ListJobsResponse/JobNames :cognitect.aws.glue/JobNameList)
(s/def :cognitect.aws.glue.ListJobsResponse/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.GetTriggerResponse/Trigger :cognitect.aws.glue/Trigger)
(s/def :cognitect.aws.glue.DeleteClassifierRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.StartBlueprintRunResponse/RunId :cognitect.aws.glue/IdString)
(s/def
:cognitect.aws.glue.GetRegistryResponse/RegistryName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.GetRegistryResponse/RegistryArn :cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.GetRegistryResponse/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.GetRegistryResponse/Status :cognitect.aws.glue/RegistryStatus)
(s/def :cognitect.aws.glue.GetRegistryResponse/CreatedTime :cognitect.aws.glue/CreatedTimestamp)
(s/def :cognitect.aws.glue.GetRegistryResponse/UpdatedTime :cognitect.aws.glue/UpdatedTimestamp)
(s/def :cognitect.aws.glue.FederatedTable/Identifier :cognitect.aws.glue/FederationIdentifier)
(s/def
:cognitect.aws.glue.FederatedTable/DatabaseIdentifier
:cognitect.aws.glue/FederationIdentifier)
(s/def :cognitect.aws.glue.FederatedTable/ConnectionName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.BatchGetDataQualityResultResponse/Results
:cognitect.aws.glue/DataQualityResultsList)
(s/def
:cognitect.aws.glue.BatchGetDataQualityResultResponse/ResultsNotFound
:cognitect.aws.glue/DataQualityResultIds)
(s/def :cognitect.aws.glue.FilterExpression/Operation :cognitect.aws.glue/FilterOperation)
(s/def :cognitect.aws.glue.FilterExpression/Negated :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.FilterExpression/Values :cognitect.aws.glue/FilterValues)
(s/def :cognitect.aws.glue.GetPartitionRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.GetPartitionRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetPartitionRequest/TableName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetPartitionRequest/PartitionValues :cognitect.aws.glue/ValueStringList)
(s/def :cognitect.aws.glue.GetWorkflowRunPropertiesRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetWorkflowRunPropertiesRequest/RunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.GetClassifiersRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.GetClassifiersRequest/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.SchemaChangePolicy/UpdateBehavior :cognitect.aws.glue/UpdateBehavior)
(s/def :cognitect.aws.glue.SchemaChangePolicy/DeleteBehavior :cognitect.aws.glue/DeleteBehavior)
(s/def :cognitect.aws.glue.ListBlueprintsResponse/Blueprints :cognitect.aws.glue/BlueprintNames)
(s/def :cognitect.aws.glue.ListBlueprintsResponse/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.PhysicalConnectionRequirements/SubnetId :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.PhysicalConnectionRequirements/SecurityGroupIdList
:cognitect.aws.glue/SecurityGroupIdList)
(s/def
:cognitect.aws.glue.PhysicalConnectionRequirements/AvailabilityZone
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.ColumnStatisticsError/ColumnStatistics
:cognitect.aws.glue/ColumnStatistics)
(s/def :cognitect.aws.glue.ColumnStatisticsError/Error :cognitect.aws.glue/ErrorDetail)
(s/def :cognitect.aws.glue.Table/IsRegisteredWithLakeFormation :cognitect.aws.glue/Boolean)
(s/def :cognitect.aws.glue.Table/FederatedTable :cognitect.aws.glue/FederatedTable)
(s/def :cognitect.aws.glue.Table/UpdateTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.Table/LastAnalyzedTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.Table/LastAccessTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.Table/Owner :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Table/PartitionKeys :cognitect.aws.glue/ColumnList)
(s/def :cognitect.aws.glue.Table/Parameters :cognitect.aws.glue/ParametersMap)
(s/def :cognitect.aws.glue.Table/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.Table/StorageDescriptor :cognitect.aws.glue/StorageDescriptor)
(s/def :cognitect.aws.glue.Table/ViewOriginalText :cognitect.aws.glue/ViewTextString)
(s/def :cognitect.aws.glue.Table/ViewExpandedText :cognitect.aws.glue/ViewTextString)
(s/def :cognitect.aws.glue.Table/CreatedBy :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Table/Retention :cognitect.aws.glue/NonNegativeInteger)
(s/def :cognitect.aws.glue.Table/TableType :cognitect.aws.glue/TableTypeString)
(s/def :cognitect.aws.glue.Table/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Table/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.Table/CreateTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.Table/TargetTable :cognitect.aws.glue/TableIdentifier)
(s/def :cognitect.aws.glue.Table/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Table/VersionId :cognitect.aws.glue/VersionString)
(s/def :cognitect.aws.glue.UpdateDevEndpointRequest/EndpointName :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.UpdateDevEndpointRequest/PublicKey :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.UpdateDevEndpointRequest/AddPublicKeys
:cognitect.aws.glue/PublicKeysList)
(s/def
:cognitect.aws.glue.UpdateDevEndpointRequest/DeletePublicKeys
:cognitect.aws.glue/PublicKeysList)
(s/def
:cognitect.aws.glue.UpdateDevEndpointRequest/CustomLibraries
:cognitect.aws.glue/DevEndpointCustomLibraries)
(s/def
:cognitect.aws.glue.UpdateDevEndpointRequest/UpdateEtlLibraries
:cognitect.aws.glue/BooleanValue)
(s/def :cognitect.aws.glue.UpdateDevEndpointRequest/DeleteArguments :cognitect.aws.glue/StringList)
(s/def :cognitect.aws.glue.UpdateDevEndpointRequest/AddArguments :cognitect.aws.glue/MapValue)
(s/def :cognitect.aws.glue.BatchGetCrawlersResponse/Crawlers :cognitect.aws.glue/CrawlerList)
(s/def
:cognitect.aws.glue.BatchGetCrawlersResponse/CrawlersNotFound
:cognitect.aws.glue/CrawlerNameList)
(s/def :cognitect.aws.glue.SparkConnectorTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.SparkConnectorTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def
:cognitect.aws.glue.SparkConnectorTarget/ConnectionName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.SparkConnectorTarget/ConnectorName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.SparkConnectorTarget/ConnectionType
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.SparkConnectorTarget/AdditionalOptions
:cognitect.aws.glue/AdditionalOptions)
(s/def :cognitect.aws.glue.SparkConnectorTarget/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def
:cognitect.aws.glue.BatchStopJobRunResponse/SuccessfulSubmissions
:cognitect.aws.glue/BatchStopJobRunSuccessfulSubmissionList)
(s/def
:cognitect.aws.glue.BatchStopJobRunResponse/Errors
:cognitect.aws.glue/BatchStopJobRunErrorList)
(s/def :cognitect.aws.glue.TaskRun/ErrorString :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.TaskRun/TaskRunId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.TaskRun/ExecutionTime :cognitect.aws.glue/ExecutionTime)
(s/def :cognitect.aws.glue.TaskRun/TransformId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.TaskRun/CompletedOn :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.TaskRun/LogGroupName :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.TaskRun/LastModifiedOn :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.TaskRun/StartedOn :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.TaskRun/Properties :cognitect.aws.glue/TaskRunProperties)
(s/def :cognitect.aws.glue.TaskRun/Status :cognitect.aws.glue/TaskStatusType)
(s/def
:cognitect.aws.glue.UpdateColumnStatisticsForTableResponse/Errors
:cognitect.aws.glue/ColumnStatisticsErrors)
(s/def :cognitect.aws.glue.UserDefinedFunction/FunctionName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UserDefinedFunction/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UserDefinedFunction/ClassName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UserDefinedFunction/OwnerName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UserDefinedFunction/OwnerType :cognitect.aws.glue/PrincipalType)
(s/def :cognitect.aws.glue.UserDefinedFunction/CreateTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.UserDefinedFunction/ResourceUris :cognitect.aws.glue/ResourceUriList)
(s/def :cognitect.aws.glue.UserDefinedFunction/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.OpenTableFormatInput/IcebergInput :cognitect.aws.glue/IcebergInput)
(s/def :cognitect.aws.glue.JobUpdate/MaxRetries :cognitect.aws.glue/MaxRetries)
(s/def :cognitect.aws.glue.JobUpdate/AllocatedCapacity :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.JobUpdate/Timeout :cognitect.aws.glue/Timeout)
(s/def :cognitect.aws.glue.JobUpdate/NumberOfWorkers :cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.JobUpdate/SecurityConfiguration :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.JobUpdate/MaxCapacity :cognitect.aws.glue/NullableDouble)
(s/def :cognitect.aws.glue.JobUpdate/NotificationProperty :cognitect.aws.glue/NotificationProperty)
(s/def :cognitect.aws.glue.JobUpdate/LogUri :cognitect.aws.glue/UriString)
(s/def
:cognitect.aws.glue.JobUpdate/CodeGenConfigurationNodes
:cognitect.aws.glue/CodeGenConfigurationNodes)
(s/def :cognitect.aws.glue.JobUpdate/WorkerType :cognitect.aws.glue/WorkerType)
(s/def :cognitect.aws.glue.JobUpdate/DefaultArguments :cognitect.aws.glue/GenericMap)
(s/def :cognitect.aws.glue.JobUpdate/Command :cognitect.aws.glue/JobCommand)
(s/def :cognitect.aws.glue.JobUpdate/ExecutionClass :cognitect.aws.glue/ExecutionClass)
(s/def :cognitect.aws.glue.JobUpdate/NonOverridableArguments :cognitect.aws.glue/GenericMap)
(s/def :cognitect.aws.glue.JobUpdate/ExecutionProperty :cognitect.aws.glue/ExecutionProperty)
(s/def :cognitect.aws.glue.JobUpdate/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.JobUpdate/GlueVersion :cognitect.aws.glue/GlueVersionString)
(s/def :cognitect.aws.glue.JobUpdate/Connections :cognitect.aws.glue/ConnectionsList)
(s/def :cognitect.aws.glue.JobUpdate/Role :cognitect.aws.glue/RoleString)
(s/def :cognitect.aws.glue.JobUpdate/SourceControlDetails :cognitect.aws.glue/SourceControlDetails)
(s/def
:cognitect.aws.glue.UpdateSourceControlFromJobResponse/JobName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetDataCatalogEncryptionSettingsRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/SchemaArn
:cognitect.aws.glue/GlueResourceArn)
(s/def
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/SchemaName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/RegistryName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/LatestVersion
:cognitect.aws.glue/LatestSchemaVersionBoolean)
(s/def
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/VersionNumber
:cognitect.aws.glue/VersionLongNumber)
(s/def
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/SchemaVersionId
:cognitect.aws.glue/SchemaVersionIdString)
(s/def
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/MetadataKey
:cognitect.aws.glue/MetadataKeyString)
(s/def
:cognitect.aws.glue.PutSchemaVersionMetadataResponse/MetadataValue
:cognitect.aws.glue/MetadataValueString)
(s/def :cognitect.aws.glue.UpdateConnectionRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.UpdateConnectionRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateConnectionRequest/ConnectionInput
:cognitect.aws.glue/ConnectionInput)
(s/def :cognitect.aws.glue.UpdateJsonClassifierRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UpdateJsonClassifierRequest/JsonPath :cognitect.aws.glue/JsonPath)
(s/def :cognitect.aws.glue.CreateDevEndpointRequest/EndpointName :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.CreateDevEndpointRequest/NumberOfNodes :cognitect.aws.glue/IntegerValue)
(s/def
:cognitect.aws.glue.CreateDevEndpointRequest/NumberOfWorkers
:cognitect.aws.glue/NullableInteger)
(s/def
:cognitect.aws.glue.CreateDevEndpointRequest/SecurityConfiguration
:cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateDevEndpointRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.CreateDevEndpointRequest/Arguments :cognitect.aws.glue/MapValue)
(s/def :cognitect.aws.glue.CreateDevEndpointRequest/PublicKeys :cognitect.aws.glue/PublicKeysList)
(s/def :cognitect.aws.glue.CreateDevEndpointRequest/PublicKey :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.CreateDevEndpointRequest/WorkerType :cognitect.aws.glue/WorkerType)
(s/def :cognitect.aws.glue.CreateDevEndpointRequest/RoleArn :cognitect.aws.glue/RoleArn)
(s/def :cognitect.aws.glue.CreateDevEndpointRequest/SubnetId :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.CreateDevEndpointRequest/ExtraJarsS3Path
:cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.CreateDevEndpointRequest/ExtraPythonLibsS3Path
:cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.CreateDevEndpointRequest/GlueVersion
:cognitect.aws.glue/GlueVersionString)
(s/def :cognitect.aws.glue.CreateDevEndpointRequest/SecurityGroupIds :cognitect.aws.glue/StringList)
(s/def :cognitect.aws.glue.ConnectionInput/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.ConnectionInput/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.ConnectionInput/ConnectionType :cognitect.aws.glue/ConnectionType)
(s/def :cognitect.aws.glue.ConnectionInput/MatchCriteria :cognitect.aws.glue/MatchCriteria)
(s/def
:cognitect.aws.glue.ConnectionInput/ConnectionProperties
:cognitect.aws.glue/ConnectionProperties)
(s/def
:cognitect.aws.glue.ConnectionInput/PhysicalConnectionRequirements
:cognitect.aws.glue/PhysicalConnectionRequirements)
(s/def :cognitect.aws.glue.DeleteSchemaInput/SchemaId :cognitect.aws.glue/SchemaId)
(s/def :cognitect.aws.glue.DeleteCustomEntityTypeRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetColumnStatisticsForTableResponse/ColumnStatisticsList
:cognitect.aws.glue/ColumnStatisticsList)
(s/def
:cognitect.aws.glue.GetColumnStatisticsForTableResponse/Errors
:cognitect.aws.glue/ColumnErrors)
(s/def :cognitect.aws.glue.AmazonRedshiftSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.AmazonRedshiftSource/Data :cognitect.aws.glue/AmazonRedshiftNodeData)
(s/def :cognitect.aws.glue.GetMappingRequest/Source :cognitect.aws.glue/CatalogEntry)
(s/def :cognitect.aws.glue.GetMappingRequest/Sinks :cognitect.aws.glue/CatalogEntries)
(s/def :cognitect.aws.glue.GetMappingRequest/Location :cognitect.aws.glue/Location)
(s/def :cognitect.aws.glue.DeleteSchemaVersionsInput/SchemaId :cognitect.aws.glue/SchemaId)
(s/def :cognitect.aws.glue.DeleteSchemaVersionsInput/Versions :cognitect.aws.glue/VersionsString)
(s/def :cognitect.aws.glue.Filter/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.Filter/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.Filter/LogicalOperator :cognitect.aws.glue/FilterLogicalOperator)
(s/def :cognitect.aws.glue.Filter/Filters :cognitect.aws.glue/FilterExpressions)
(s/def :cognitect.aws.glue.GetResourcePolicyRequest/ResourceArn :cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.GetClassifiersResponse/Classifiers :cognitect.aws.glue/ClassifierList)
(s/def :cognitect.aws.glue.GetClassifiersResponse/NextToken :cognitect.aws.glue/Token)
(s/def
:cognitect.aws.glue.BatchGetDevEndpointsResponse/DevEndpoints
:cognitect.aws.glue/DevEndpointList)
(s/def
:cognitect.aws.glue.BatchGetDevEndpointsResponse/DevEndpointsNotFound
:cognitect.aws.glue/DevEndpointNames)
(s/def
:cognitect.aws.glue.UpdateColumnStatisticsForPartitionResponse/Errors
:cognitect.aws.glue/ColumnStatisticsErrors)
(s/def :cognitect.aws.glue.GovernedCatalogSource/Name :cognitect.aws.glue/NodeName)
(s/def
:cognitect.aws.glue.GovernedCatalogSource/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.GovernedCatalogSource/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.GovernedCatalogSource/PartitionPredicate
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.GovernedCatalogSource/AdditionalOptions
:cognitect.aws.glue/S3SourceAdditionalOptions)
(s/def :cognitect.aws.glue.CreateWorkflowRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateWorkflowRequest/Description :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.CreateWorkflowRequest/DefaultRunProperties
:cognitect.aws.glue/WorkflowRunProperties)
(s/def :cognitect.aws.glue.CreateWorkflowRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def
:cognitect.aws.glue.CreateWorkflowRequest/MaxConcurrentRuns
:cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.CatalogTarget/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CatalogTarget/Tables :cognitect.aws.glue/CatalogTablesList)
(s/def :cognitect.aws.glue.CatalogTarget/ConnectionName :cognitect.aws.glue/ConnectionName)
(s/def :cognitect.aws.glue.CatalogTarget/EventQueueArn :cognitect.aws.glue/EventQueueArn)
(s/def :cognitect.aws.glue.CatalogTarget/DlqEventQueueArn :cognitect.aws.glue/EventQueueArn)
(s/def :cognitect.aws.glue.CreateJobRequest/MaxRetries :cognitect.aws.glue/MaxRetries)
(s/def :cognitect.aws.glue.CreateJobRequest/AllocatedCapacity :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.CreateJobRequest/Timeout :cognitect.aws.glue/Timeout)
(s/def :cognitect.aws.glue.CreateJobRequest/NumberOfWorkers :cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.CreateJobRequest/SecurityConfiguration :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateJobRequest/MaxCapacity :cognitect.aws.glue/NullableDouble)
(s/def :cognitect.aws.glue.CreateJobRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def
:cognitect.aws.glue.CreateJobRequest/NotificationProperty
:cognitect.aws.glue/NotificationProperty)
(s/def :cognitect.aws.glue.CreateJobRequest/LogUri :cognitect.aws.glue/UriString)
(s/def
:cognitect.aws.glue.CreateJobRequest/CodeGenConfigurationNodes
:cognitect.aws.glue/CodeGenConfigurationNodes)
(s/def :cognitect.aws.glue.CreateJobRequest/WorkerType :cognitect.aws.glue/WorkerType)
(s/def :cognitect.aws.glue.CreateJobRequest/DefaultArguments :cognitect.aws.glue/GenericMap)
(s/def :cognitect.aws.glue.CreateJobRequest/Command :cognitect.aws.glue/JobCommand)
(s/def :cognitect.aws.glue.CreateJobRequest/ExecutionClass :cognitect.aws.glue/ExecutionClass)
(s/def :cognitect.aws.glue.CreateJobRequest/NonOverridableArguments :cognitect.aws.glue/GenericMap)
(s/def :cognitect.aws.glue.CreateJobRequest/ExecutionProperty :cognitect.aws.glue/ExecutionProperty)
(s/def :cognitect.aws.glue.CreateJobRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateJobRequest/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.CreateJobRequest/GlueVersion :cognitect.aws.glue/GlueVersionString)
(s/def :cognitect.aws.glue.CreateJobRequest/Connections :cognitect.aws.glue/ConnectionsList)
(s/def :cognitect.aws.glue.CreateJobRequest/Role :cognitect.aws.glue/RoleString)
(s/def
:cognitect.aws.glue.CreateJobRequest/SourceControlDetails
:cognitect.aws.glue/SourceControlDetails)
(s/def :cognitect.aws.glue.DeleteSecurityConfigurationRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetColumnStatisticsForTableRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.GetColumnStatisticsForTableRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetColumnStatisticsForTableRequest/TableName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetColumnStatisticsForTableRequest/ColumnNames
:cognitect.aws.glue/GetColumnNamesList)
(s/def :cognitect.aws.glue.GetTableRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.GetTableRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetTableRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetTableRequest/TransactionId :cognitect.aws.glue/TransactionIdString)
(s/def :cognitect.aws.glue.GetTableRequest/QueryAsOfTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.ListSchemaVersionsInput/SchemaId :cognitect.aws.glue/SchemaId)
(s/def :cognitect.aws.glue.ListSchemaVersionsInput/MaxResults :cognitect.aws.glue/MaxResultsNumber)
(s/def
:cognitect.aws.glue.ListSchemaVersionsInput/NextToken
:cognitect.aws.glue/SchemaRegistryTokenString)
(s/def :cognitect.aws.glue.GovernedCatalogTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.GovernedCatalogTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def
:cognitect.aws.glue.GovernedCatalogTarget/PartitionKeys
:cognitect.aws.glue/GlueStudioPathList)
(s/def :cognitect.aws.glue.GovernedCatalogTarget/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.GovernedCatalogTarget/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.GovernedCatalogTarget/SchemaChangePolicy
:cognitect.aws.glue/CatalogSchemaChangePolicy)
(s/def :cognitect.aws.glue.Option/Value :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.Option/Label :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.Option/Description :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.ListDataQualityRulesetsRequest/NextToken
:cognitect.aws.glue/PaginationToken)
(s/def :cognitect.aws.glue.ListDataQualityRulesetsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def
:cognitect.aws.glue.ListDataQualityRulesetsRequest/Filter
:cognitect.aws.glue/DataQualityRulesetFilterCriteria)
(s/def :cognitect.aws.glue.ListDataQualityRulesetsRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def
:cognitect.aws.glue.CreateUserDefinedFunctionRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.CreateUserDefinedFunctionRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.CreateUserDefinedFunctionRequest/FunctionInput
:cognitect.aws.glue/UserDefinedFunctionInput)
(s/def :cognitect.aws.glue.GetSecurityConfigurationsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def
:cognitect.aws.glue.GetSecurityConfigurationsRequest/NextToken
:cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/SampleQuery :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.AmazonRedshiftNodeData/CatalogRedshiftTable
:cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.AmazonRedshiftNodeData/CatalogRedshiftSchema
:cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/Table :cognitect.aws.glue/Option)
(s/def
:cognitect.aws.glue.AmazonRedshiftNodeData/AdvancedOptions
:cognitect.aws.glue/AmazonRedshiftAdvancedOptions)
(s/def
:cognitect.aws.glue.AmazonRedshiftNodeData/MergeAction
:cognitect.aws.glue/GenericLimitedString)
(s/def
:cognitect.aws.glue.AmazonRedshiftNodeData/MergeWhenMatched
:cognitect.aws.glue/GenericLimitedString)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/Upsert :cognitect.aws.glue/BooleanValue)
(s/def
:cognitect.aws.glue.AmazonRedshiftNodeData/TempDir
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/CatalogDatabase :cognitect.aws.glue/Option)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/PreAction :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/TableSchema :cognitect.aws.glue/OptionList)
(s/def
:cognitect.aws.glue.AmazonRedshiftNodeData/MergeWhenNotMatched
:cognitect.aws.glue/GenericLimitedString)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/IamRole :cognitect.aws.glue/Option)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/PostAction :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.AmazonRedshiftNodeData/AccessType
:cognitect.aws.glue/GenericLimitedString)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/Action :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/MergeClause :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/CatalogTable :cognitect.aws.glue/Option)
(s/def
:cognitect.aws.glue.AmazonRedshiftNodeData/TablePrefix
:cognitect.aws.glue/GenericLimitedString)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/Schema :cognitect.aws.glue/Option)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/StagingTable :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/SelectedColumns :cognitect.aws.glue/OptionList)
(s/def :cognitect.aws.glue.AmazonRedshiftNodeData/Connection :cognitect.aws.glue/Option)
(s/def
:cognitect.aws.glue.AmazonRedshiftNodeData/CrawlerConnection
:cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.AmazonRedshiftNodeData/SourceType
:cognitect.aws.glue/GenericLimitedString)
(s/def :cognitect.aws.glue.GetSchemaInput/SchemaId :cognitect.aws.glue/SchemaId)
(s/def :cognitect.aws.glue.BatchGetWorkflowsResponse/Workflows :cognitect.aws.glue/Workflows)
(s/def
:cognitect.aws.glue.BatchGetWorkflowsResponse/MissingWorkflows
:cognitect.aws.glue/WorkflowNames)
(s/def :cognitect.aws.glue.CreateCustomEntityTypeResponse/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Edge/SourceId :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Edge/DestinationId :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DeleteWorkflowRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetPartitionIndexesRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.GetPartitionIndexesRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetPartitionIndexesRequest/TableName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetPartitionIndexesRequest/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.CreateScriptResponse/PythonScript :cognitect.aws.glue/PythonScript)
(s/def :cognitect.aws.glue.CreateScriptResponse/ScalaCode :cognitect.aws.glue/ScalaCode)
(s/def :cognitect.aws.glue.SchemaListItem/RegistryName :cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.SchemaListItem/SchemaName :cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.SchemaListItem/SchemaArn :cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.SchemaListItem/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.SchemaListItem/SchemaStatus :cognitect.aws.glue/SchemaStatus)
(s/def :cognitect.aws.glue.SchemaListItem/CreatedTime :cognitect.aws.glue/CreatedTimestamp)
(s/def :cognitect.aws.glue.SchemaListItem/UpdatedTime :cognitect.aws.glue/UpdatedTimestamp)
(s/def :cognitect.aws.glue.CreateDataQualityRulesetResponse/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.ColumnImportance/ColumnName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.ColumnImportance/Importance :cognitect.aws.glue/GenericBoundedDouble)
(s/def :cognitect.aws.glue.CreateWorkflowResponse/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/ErrorString
:cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/Timeout
:cognitect.aws.glue/Timeout)
(s/def
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/RunId
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/ExecutionTime
:cognitect.aws.glue/ExecutionTime)
(s/def
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/NumberOfWorkers
:cognitect.aws.glue/NullableInteger)
(s/def
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/CreatedRulesetName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/CompletedOn
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/LastModifiedOn
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/StartedOn
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/RecommendedRuleset
:cognitect.aws.glue/DataQualityRulesetString)
(s/def
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/Role
:cognitect.aws.glue/RoleString)
(s/def
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/DataSource
:cognitect.aws.glue/DataSource)
(s/def
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunResponse/Status
:cognitect.aws.glue/TaskStatusType)
(s/def :cognitect.aws.glue.Schedule/ScheduleExpression :cognitect.aws.glue/CronExpression)
(s/def :cognitect.aws.glue.Schedule/State :cognitect.aws.glue/ScheduleState)
(s/def :cognitect.aws.glue.Predicate/Logical :cognitect.aws.glue/Logical)
(s/def :cognitect.aws.glue.Predicate/Conditions :cognitect.aws.glue/ConditionList)
(s/def :cognitect.aws.glue.AthenaConnectorSource/Name :cognitect.aws.glue/NodeName)
(s/def
:cognitect.aws.glue.AthenaConnectorSource/ConnectionName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.AthenaConnectorSource/ConnectorName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.AthenaConnectorSource/ConnectionType
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.AthenaConnectorSource/ConnectionTable
:cognitect.aws.glue/EnclosedInStringPropertyWithQuote)
(s/def
:cognitect.aws.glue.AthenaConnectorSource/SchemaName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.AthenaConnectorSource/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def :cognitect.aws.glue.CrawlerTargets/S3Targets :cognitect.aws.glue/S3TargetList)
(s/def :cognitect.aws.glue.CrawlerTargets/JdbcTargets :cognitect.aws.glue/JdbcTargetList)
(s/def :cognitect.aws.glue.CrawlerTargets/MongoDBTargets :cognitect.aws.glue/MongoDBTargetList)
(s/def :cognitect.aws.glue.CrawlerTargets/DynamoDBTargets :cognitect.aws.glue/DynamoDBTargetList)
(s/def :cognitect.aws.glue.CrawlerTargets/CatalogTargets :cognitect.aws.glue/CatalogTargetList)
(s/def :cognitect.aws.glue.CrawlerTargets/DeltaTargets :cognitect.aws.glue/DeltaTargetList)
(s/def :cognitect.aws.glue.CrawlerTargets/IcebergTargets :cognitect.aws.glue/IcebergTargetList)
(s/def :cognitect.aws.glue.CustomEntityType/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CustomEntityType/RegexString :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CustomEntityType/ContextWords :cognitect.aws.glue/ContextWords)
(s/def :cognitect.aws.glue.SqlAlias/From :cognitect.aws.glue/NodeId)
(s/def :cognitect.aws.glue.SqlAlias/Alias :cognitect.aws.glue/EnclosedInStringPropertyWithQuote)
(s/def
:cognitect.aws.glue.StartMLLabelingSetGenerationTaskRunRequest/TransformId
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.StartMLLabelingSetGenerationTaskRunRequest/OutputS3Path
:cognitect.aws.glue/UriString)
(s/def :cognitect.aws.glue.DeleteTableRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.DeleteTableRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DeleteTableRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DeleteTableRequest/TransactionId :cognitect.aws.glue/TransactionIdString)
(s/def
:cognitect.aws.glue.GetTableVersionsResponse/TableVersions
:cognitect.aws.glue/GetTableVersionsList)
(s/def :cognitect.aws.glue.GetTableVersionsResponse/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.SecurityConfiguration/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.SecurityConfiguration/CreatedTimeStamp
:cognitect.aws.glue/TimestampValue)
(s/def
:cognitect.aws.glue.SecurityConfiguration/EncryptionConfiguration
:cognitect.aws.glue/EncryptionConfiguration)
(s/def :cognitect.aws.glue.OracleSQLCatalogSource/Name :cognitect.aws.glue/NodeName)
(s/def
:cognitect.aws.glue.OracleSQLCatalogSource/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.OracleSQLCatalogSource/Table
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.JobCommand/Name :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.JobCommand/ScriptLocation :cognitect.aws.glue/ScriptLocationString)
(s/def :cognitect.aws.glue.JobCommand/PythonVersion :cognitect.aws.glue/PythonVersionString)
(s/def :cognitect.aws.glue.JobCommand/Runtime :cognitect.aws.glue/RuntimeNameString)
(s/def :cognitect.aws.glue.DataQualityRulesetFilterCriteria/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.DataQualityRulesetFilterCriteria/Description
:cognitect.aws.glue/DescriptionString)
(s/def
:cognitect.aws.glue.DataQualityRulesetFilterCriteria/CreatedBefore
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.DataQualityRulesetFilterCriteria/CreatedAfter
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.DataQualityRulesetFilterCriteria/LastModifiedBefore
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.DataQualityRulesetFilterCriteria/LastModifiedAfter
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.DataQualityRulesetFilterCriteria/TargetTable
:cognitect.aws.glue/DataQualityTargetTable)
(s/def :cognitect.aws.glue.StatementOutputData/TextPlain :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.GlueSchema/Columns :cognitect.aws.glue/GlueStudioSchemaColumnList)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionMetadataResponse/Partition
:cognitect.aws.glue/Partition)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionMetadataResponse/AuthorizedColumns
:cognitect.aws.glue/NameStringList)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionMetadataResponse/IsRegisteredWithLakeFormation
:cognitect.aws.glue/Boolean)
(s/def :cognitect.aws.glue.S3CatalogSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.S3CatalogSource/Database :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.S3CatalogSource/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.S3CatalogSource/PartitionPredicate
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.S3CatalogSource/AdditionalOptions
:cognitect.aws.glue/S3SourceAdditionalOptions)
(s/def
:cognitect.aws.glue.CancelDataQualityRulesetEvaluationRunRequest/RunId
:cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.ListSchemaVersionsResponse/Schemas :cognitect.aws.glue/SchemaVersionList)
(s/def
:cognitect.aws.glue.ListSchemaVersionsResponse/NextToken
:cognitect.aws.glue/SchemaRegistryTokenString)
(s/def :cognitect.aws.glue.GetUnfilteredTableMetadataResponse/Table :cognitect.aws.glue/Table)
(s/def
:cognitect.aws.glue.GetUnfilteredTableMetadataResponse/AuthorizedColumns
:cognitect.aws.glue/NameStringList)
(s/def
:cognitect.aws.glue.GetUnfilteredTableMetadataResponse/IsRegisteredWithLakeFormation
:cognitect.aws.glue/Boolean)
(s/def
:cognitect.aws.glue.GetUnfilteredTableMetadataResponse/CellFilters
:cognitect.aws.glue/ColumnRowFilterList)
(s/def
:cognitect.aws.glue.DataCatalogEncryptionSettings/EncryptionAtRest
:cognitect.aws.glue/EncryptionAtRest)
(s/def
:cognitect.aws.glue.DataCatalogEncryptionSettings/ConnectionPasswordEncryption
:cognitect.aws.glue/ConnectionPasswordEncryption)
(s/def :cognitect.aws.glue.PostgreSQLCatalogSource/Name :cognitect.aws.glue/NodeName)
(s/def
:cognitect.aws.glue.PostgreSQLCatalogSource/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.PostgreSQLCatalogSource/Table
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.CrawlsFilter/FieldName :cognitect.aws.glue/FieldName)
(s/def :cognitect.aws.glue.CrawlsFilter/FilterOperator :cognitect.aws.glue/FilterOperator)
(s/def :cognitect.aws.glue.CrawlsFilter/FieldValue :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.UpdateMLTransformRequest/MaxRetries :cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.UpdateMLTransformRequest/Timeout :cognitect.aws.glue/Timeout)
(s/def
:cognitect.aws.glue.UpdateMLTransformRequest/NumberOfWorkers
:cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.UpdateMLTransformRequest/TransformId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.UpdateMLTransformRequest/MaxCapacity :cognitect.aws.glue/NullableDouble)
(s/def
:cognitect.aws.glue.UpdateMLTransformRequest/Parameters
:cognitect.aws.glue/TransformParameters)
(s/def :cognitect.aws.glue.UpdateMLTransformRequest/WorkerType :cognitect.aws.glue/WorkerType)
(s/def :cognitect.aws.glue.UpdateMLTransformRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateMLTransformRequest/Description
:cognitect.aws.glue/DescriptionString)
(s/def
:cognitect.aws.glue.UpdateMLTransformRequest/GlueVersion
:cognitect.aws.glue/GlueVersionString)
(s/def :cognitect.aws.glue.UpdateMLTransformRequest/Role :cognitect.aws.glue/RoleString)
(s/def :cognitect.aws.glue.GetTableVersionResponse/TableVersion :cognitect.aws.glue/TableVersion)
(s/def :cognitect.aws.glue.Predecessor/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Predecessor/RunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.ListBlueprintsRequest/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.ListBlueprintsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.ListBlueprintsRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.DropFields/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.DropFields/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.DropFields/Paths :cognitect.aws.glue/GlueStudioPathList)
(s/def :cognitect.aws.glue.StartCrawlerScheduleRequest/CrawlerName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.LastCrawlInfo/Status :cognitect.aws.glue/LastCrawlStatus)
(s/def :cognitect.aws.glue.LastCrawlInfo/ErrorMessage :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.LastCrawlInfo/LogGroup :cognitect.aws.glue/LogGroup)
(s/def :cognitect.aws.glue.LastCrawlInfo/LogStream :cognitect.aws.glue/LogStream)
(s/def :cognitect.aws.glue.LastCrawlInfo/MessagePrefix :cognitect.aws.glue/MessagePrefix)
(s/def :cognitect.aws.glue.LastCrawlInfo/StartTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.Datatype/Id :cognitect.aws.glue/GenericLimitedString)
(s/def :cognitect.aws.glue.Datatype/Label :cognitect.aws.glue/GenericLimitedString)
(s/def :cognitect.aws.glue.GetTablesRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.GetTablesRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetTablesRequest/Expression :cognitect.aws.glue/FilterString)
(s/def :cognitect.aws.glue.GetTablesRequest/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.GetTablesRequest/MaxResults :cognitect.aws.glue/CatalogGetterPageSize)
(s/def :cognitect.aws.glue.GetTablesRequest/TransactionId :cognitect.aws.glue/TransactionIdString)
(s/def :cognitect.aws.glue.GetTablesRequest/QueryAsOfTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.GetDatabasesResponse/DatabaseList :cognitect.aws.glue/DatabaseList)
(s/def :cognitect.aws.glue.GetDatabasesResponse/NextToken :cognitect.aws.glue/Token)
(s/def
:cognitect.aws.glue.GetSchemaResponse/SchemaName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def
:cognitect.aws.glue.GetSchemaResponse/SchemaCheckpoint
:cognitect.aws.glue/SchemaCheckpointNumber)
(s/def :cognitect.aws.glue.GetSchemaResponse/SchemaArn :cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.GetSchemaResponse/SchemaStatus :cognitect.aws.glue/SchemaStatus)
(s/def :cognitect.aws.glue.GetSchemaResponse/RegistryArn :cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.GetSchemaResponse/DataFormat :cognitect.aws.glue/DataFormat)
(s/def
:cognitect.aws.glue.GetSchemaResponse/NextSchemaVersion
:cognitect.aws.glue/VersionLongNumber)
(s/def :cognitect.aws.glue.GetSchemaResponse/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.GetSchemaResponse/Compatibility :cognitect.aws.glue/Compatibility)
(s/def :cognitect.aws.glue.GetSchemaResponse/CreatedTime :cognitect.aws.glue/CreatedTimestamp)
(s/def :cognitect.aws.glue.GetSchemaResponse/UpdatedTime :cognitect.aws.glue/UpdatedTimestamp)
(s/def
:cognitect.aws.glue.GetSchemaResponse/LatestSchemaVersion
:cognitect.aws.glue/VersionLongNumber)
(s/def
:cognitect.aws.glue.GetSchemaResponse/RegistryName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.TransformParameters/TransformType :cognitect.aws.glue/TransformType)
(s/def
:cognitect.aws.glue.TransformParameters/FindMatchesParameters
:cognitect.aws.glue/FindMatchesParameters)
(s/def :cognitect.aws.glue.UnfilteredPartition/Partition :cognitect.aws.glue/Partition)
(s/def :cognitect.aws.glue.UnfilteredPartition/AuthorizedColumns :cognitect.aws.glue/NameStringList)
(s/def
:cognitect.aws.glue.UnfilteredPartition/IsRegisteredWithLakeFormation
:cognitect.aws.glue/Boolean)
(s/def :cognitect.aws.glue.CatalogHudiSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.CatalogHudiSource/Database :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.CatalogHudiSource/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.CatalogHudiSource/AdditionalHudiOptions
:cognitect.aws.glue/AdditionalOptions)
(s/def :cognitect.aws.glue.CatalogHudiSource/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def :cognitect.aws.glue.UpdateXMLClassifierRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateXMLClassifierRequest/Classification
:cognitect.aws.glue/Classification)
(s/def :cognitect.aws.glue.UpdateXMLClassifierRequest/RowTag :cognitect.aws.glue/RowTag)
(s/def :cognitect.aws.glue.DoubleColumnStatisticsData/MinimumValue :cognitect.aws.glue/Double)
(s/def :cognitect.aws.glue.DoubleColumnStatisticsData/MaximumValue :cognitect.aws.glue/Double)
(s/def
:cognitect.aws.glue.DoubleColumnStatisticsData/NumberOfNulls
:cognitect.aws.glue/NonNegativeLong)
(s/def
:cognitect.aws.glue.DoubleColumnStatisticsData/NumberOfDistinctValues
:cognitect.aws.glue/NonNegativeLong)
(s/def :cognitect.aws.glue.GetMappingResponse/Mapping :cognitect.aws.glue/MappingList)
(s/def
:cognitect.aws.glue.GetUnfilteredTableMetadataRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.GetUnfilteredTableMetadataRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetUnfilteredTableMetadataRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetUnfilteredTableMetadataRequest/AuditContext
:cognitect.aws.glue/AuditContext)
(s/def
:cognitect.aws.glue.GetUnfilteredTableMetadataRequest/SupportedPermissionTypes
:cognitect.aws.glue/PermissionTypeList)
(s/def :cognitect.aws.glue.ResumeWorkflowRunRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.ResumeWorkflowRunRequest/RunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.ResumeWorkflowRunRequest/NodeIds :cognitect.aws.glue/NodeIdList)
(s/def :cognitect.aws.glue.GlueTable/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GlueTable/TableName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GlueTable/CatalogId :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GlueTable/ConnectionName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GlueTable/AdditionalOptions
:cognitect.aws.glue/GlueTableAdditionalOptions)
(s/def
:cognitect.aws.glue.GlueStudioSchemaColumn/Name
:cognitect.aws.glue/GlueStudioColumnNameString)
(s/def :cognitect.aws.glue.GlueStudioSchemaColumn/Type :cognitect.aws.glue/ColumnTypeString)
(s/def :cognitect.aws.glue.GetDataflowGraphRequest/PythonScript :cognitect.aws.glue/PythonScript)
(s/def
:cognitect.aws.glue.CatalogSchemaChangePolicy/EnableUpdateCatalog
:cognitect.aws.glue/BoxedBoolean)
(s/def
:cognitect.aws.glue.CatalogSchemaChangePolicy/UpdateBehavior
:cognitect.aws.glue/UpdateCatalogBehavior)
(s/def
:cognitect.aws.glue.ResetJobBookmarkResponse/JobBookmarkEntry
:cognitect.aws.glue/JobBookmarkEntry)
(s/def :cognitect.aws.glue.DataQualityRuleResult/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DataQualityRuleResult/Description :cognitect.aws.glue/DescriptionString)
(s/def
:cognitect.aws.glue.DataQualityRuleResult/EvaluationMessage
:cognitect.aws.glue/DescriptionString)
(s/def
:cognitect.aws.glue.DataQualityRuleResult/Result
:cognitect.aws.glue/DataQualityRuleResultStatus)
(s/def
:cognitect.aws.glue.DataQualityRuleResult/EvaluatedMetrics
:cognitect.aws.glue/EvaluatedMetricsMap)
(s/def :cognitect.aws.glue.CatalogKinesisSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.CatalogKinesisSource/WindowSize :cognitect.aws.glue/BoxedPositiveInt)
(s/def :cognitect.aws.glue.CatalogKinesisSource/DetectSchema :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.CatalogKinesisSource/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.CatalogKinesisSource/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.CatalogKinesisSource/StreamingOptions
:cognitect.aws.glue/KinesisStreamingSourceOptions)
(s/def
:cognitect.aws.glue.CatalogKinesisSource/DataPreviewOptions
:cognitect.aws.glue/StreamingDataPreviewOptions)
(s/def :cognitect.aws.glue.MetadataInfo/MetadataValue :cognitect.aws.glue/MetadataValueString)
(s/def :cognitect.aws.glue.MetadataInfo/CreatedTime :cognitect.aws.glue/CreatedTimestamp)
(s/def
:cognitect.aws.glue.MetadataInfo/OtherMetadataValueList
:cognitect.aws.glue/OtherMetadataValueList)
(s/def :cognitect.aws.glue.S3Encryption/S3EncryptionMode :cognitect.aws.glue/S3EncryptionMode)
(s/def :cognitect.aws.glue.S3Encryption/KmsKeyArn :cognitect.aws.glue/KmsKeyArn)
(s/def :cognitect.aws.glue.S3HudiDirectTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.S3HudiDirectTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.S3HudiDirectTarget/Path :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.S3HudiDirectTarget/Compression
:cognitect.aws.glue/HudiTargetCompressionType)
(s/def :cognitect.aws.glue.S3HudiDirectTarget/PartitionKeys :cognitect.aws.glue/GlueStudioPathList)
(s/def :cognitect.aws.glue.S3HudiDirectTarget/Format :cognitect.aws.glue/TargetFormat)
(s/def
:cognitect.aws.glue.S3HudiDirectTarget/AdditionalOptions
:cognitect.aws.glue/AdditionalOptions)
(s/def
:cognitect.aws.glue.S3HudiDirectTarget/SchemaChangePolicy
:cognitect.aws.glue/DirectSchemaChangePolicy)
(s/def :cognitect.aws.glue.GetTagsResponse/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.GetDevEndpointRequest/EndpointName :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.ListCrawlersRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.ListCrawlersRequest/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.ListCrawlersRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.CreateMLTransformRequest/MaxRetries :cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.CreateMLTransformRequest/Timeout :cognitect.aws.glue/Timeout)
(s/def
:cognitect.aws.glue.CreateMLTransformRequest/NumberOfWorkers
:cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.CreateMLTransformRequest/MaxCapacity :cognitect.aws.glue/NullableDouble)
(s/def :cognitect.aws.glue.CreateMLTransformRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def
:cognitect.aws.glue.CreateMLTransformRequest/Parameters
:cognitect.aws.glue/TransformParameters)
(s/def
:cognitect.aws.glue.CreateMLTransformRequest/TransformEncryption
:cognitect.aws.glue/TransformEncryption)
(s/def :cognitect.aws.glue.CreateMLTransformRequest/WorkerType :cognitect.aws.glue/WorkerType)
(s/def :cognitect.aws.glue.CreateMLTransformRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.CreateMLTransformRequest/Description
:cognitect.aws.glue/DescriptionString)
(s/def
:cognitect.aws.glue.CreateMLTransformRequest/GlueVersion
:cognitect.aws.glue/GlueVersionString)
(s/def :cognitect.aws.glue.CreateMLTransformRequest/Role :cognitect.aws.glue/RoleString)
(s/def
:cognitect.aws.glue.CreateMLTransformRequest/InputRecordTables
:cognitect.aws.glue/GlueTables)
(s/def
:cognitect.aws.glue.PutDataCatalogEncryptionSettingsRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.PutDataCatalogEncryptionSettingsRequest/DataCatalogEncryptionSettings
:cognitect.aws.glue/DataCatalogEncryptionSettings)
(s/def :cognitect.aws.glue.FindMatchesTaskRunProperties/JobId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.FindMatchesTaskRunProperties/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.FindMatchesTaskRunProperties/JobRunId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.ListJobsRequest/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.ListJobsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.ListJobsRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.DeleteBlueprintRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.S3GlueParquetTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.S3GlueParquetTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.S3GlueParquetTarget/PartitionKeys :cognitect.aws.glue/GlueStudioPathList)
(s/def :cognitect.aws.glue.S3GlueParquetTarget/Path :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.S3GlueParquetTarget/Compression
:cognitect.aws.glue/ParquetCompressionType)
(s/def
:cognitect.aws.glue.S3GlueParquetTarget/SchemaChangePolicy
:cognitect.aws.glue/DirectSchemaChangePolicy)
(s/def :cognitect.aws.glue.AmazonRedshiftAdvancedOption/Key :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.AmazonRedshiftAdvancedOption/Value :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.SparkConnectorSource/Name :cognitect.aws.glue/NodeName)
(s/def
:cognitect.aws.glue.SparkConnectorSource/ConnectionName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.SparkConnectorSource/ConnectorName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.SparkConnectorSource/ConnectionType
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.SparkConnectorSource/AdditionalOptions
:cognitect.aws.glue/AdditionalOptions)
(s/def :cognitect.aws.glue.SparkConnectorSource/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def :cognitect.aws.glue.DeleteWorkflowResponse/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UpdateGrokClassifierRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateGrokClassifierRequest/Classification
:cognitect.aws.glue/Classification)
(s/def :cognitect.aws.glue.UpdateGrokClassifierRequest/GrokPattern :cognitect.aws.glue/GrokPattern)
(s/def
:cognitect.aws.glue.UpdateGrokClassifierRequest/CustomPatterns
:cognitect.aws.glue/CustomPatterns)
(s/def
:cognitect.aws.glue.NotificationProperty/NotifyDelayAfter
:cognitect.aws.glue/NotifyDelayAfter)
(s/def :cognitect.aws.glue.ListMLTransformsRequest/NextToken :cognitect.aws.glue/PaginationToken)
(s/def :cognitect.aws.glue.ListMLTransformsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def
:cognitect.aws.glue.ListMLTransformsRequest/Filter
:cognitect.aws.glue/TransformFilterCriteria)
(s/def :cognitect.aws.glue.ListMLTransformsRequest/Sort :cognitect.aws.glue/TransformSortCriteria)
(s/def :cognitect.aws.glue.ListMLTransformsRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.DeleteConnectionRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.DeleteConnectionRequest/ConnectionName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CancelStatementRequest/SessionId :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CancelStatementRequest/Id :cognitect.aws.glue/IntegerValue)
(s/def
:cognitect.aws.glue.CancelStatementRequest/RequestOrigin
:cognitect.aws.glue/OrchestrationNameString)
(s/def :cognitect.aws.glue.GetPlanRequest/Mapping :cognitect.aws.glue/MappingList)
(s/def :cognitect.aws.glue.GetPlanRequest/Source :cognitect.aws.glue/CatalogEntry)
(s/def :cognitect.aws.glue.GetPlanRequest/Sinks :cognitect.aws.glue/CatalogEntries)
(s/def :cognitect.aws.glue.GetPlanRequest/Location :cognitect.aws.glue/Location)
(s/def :cognitect.aws.glue.GetPlanRequest/Language :cognitect.aws.glue/Language)
(s/def
:cognitect.aws.glue.GetPlanRequest/AdditionalPlanOptionsMap
:cognitect.aws.glue/AdditionalPlanOptionsMap)
(s/def :cognitect.aws.glue.DirectJDBCSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.DirectJDBCSource/Database :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.DirectJDBCSource/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.DirectJDBCSource/ConnectionName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.DirectJDBCSource/ConnectionType :cognitect.aws.glue/JDBCConnectionType)
(s/def
:cognitect.aws.glue.DirectJDBCSource/RedshiftTmpDir
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.TriggerUpdate/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.TriggerUpdate/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.TriggerUpdate/Schedule :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.TriggerUpdate/Actions :cognitect.aws.glue/ActionList)
(s/def :cognitect.aws.glue.TriggerUpdate/Predicate :cognitect.aws.glue/Predicate)
(s/def
:cognitect.aws.glue.TriggerUpdate/EventBatchingCondition
:cognitect.aws.glue/EventBatchingCondition)
(s/def :cognitect.aws.glue.ErrorDetails/ErrorCode :cognitect.aws.glue/ErrorCodeString)
(s/def :cognitect.aws.glue.ErrorDetails/ErrorMessage :cognitect.aws.glue/ErrorMessageString)
(s/def :cognitect.aws.glue.XMLClassifier/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.XMLClassifier/Classification :cognitect.aws.glue/Classification)
(s/def :cognitect.aws.glue.XMLClassifier/CreationTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.XMLClassifier/LastUpdated :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.XMLClassifier/Version :cognitect.aws.glue/VersionId)
(s/def :cognitect.aws.glue.XMLClassifier/RowTag :cognitect.aws.glue/RowTag)
(s/def
:cognitect.aws.glue.FindMatchesParameters/PrimaryKeyColumnName
:cognitect.aws.glue/ColumnNameString)
(s/def
:cognitect.aws.glue.FindMatchesParameters/PrecisionRecallTradeoff
:cognitect.aws.glue/GenericBoundedDouble)
(s/def
:cognitect.aws.glue.FindMatchesParameters/AccuracyCostTradeoff
:cognitect.aws.glue/GenericBoundedDouble)
(s/def
:cognitect.aws.glue.FindMatchesParameters/EnforceProvidedLabels
:cognitect.aws.glue/NullableBoolean)
(s/def
:cognitect.aws.glue.PutResourcePolicyRequest/PolicyInJson
:cognitect.aws.glue/PolicyJsonString)
(s/def :cognitect.aws.glue.PutResourcePolicyRequest/ResourceArn :cognitect.aws.glue/GlueResourceArn)
(s/def
:cognitect.aws.glue.PutResourcePolicyRequest/PolicyHashCondition
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.PutResourcePolicyRequest/PolicyExistsCondition
:cognitect.aws.glue/ExistCondition)
(s/def
:cognitect.aws.glue.PutResourcePolicyRequest/EnableHybrid
:cognitect.aws.glue/EnableHybridValues)
(s/def :cognitect.aws.glue.RedshiftTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.RedshiftTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.RedshiftTarget/Database :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.RedshiftTarget/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.RedshiftTarget/RedshiftTmpDir
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.RedshiftTarget/TmpDirIAMRole
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.RedshiftTarget/UpsertRedshiftOptions
:cognitect.aws.glue/UpsertRedshiftTargetOptions)
(s/def :cognitect.aws.glue.TableInput/LastAnalyzedTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.TableInput/LastAccessTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.TableInput/Owner :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.TableInput/PartitionKeys :cognitect.aws.glue/ColumnList)
(s/def :cognitect.aws.glue.TableInput/Parameters :cognitect.aws.glue/ParametersMap)
(s/def :cognitect.aws.glue.TableInput/StorageDescriptor :cognitect.aws.glue/StorageDescriptor)
(s/def :cognitect.aws.glue.TableInput/ViewOriginalText :cognitect.aws.glue/ViewTextString)
(s/def :cognitect.aws.glue.TableInput/ViewExpandedText :cognitect.aws.glue/ViewTextString)
(s/def :cognitect.aws.glue.TableInput/Retention :cognitect.aws.glue/NonNegativeInteger)
(s/def :cognitect.aws.glue.TableInput/TableType :cognitect.aws.glue/TableTypeString)
(s/def :cognitect.aws.glue.TableInput/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.TableInput/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.TableInput/TargetTable :cognitect.aws.glue/TableIdentifier)
(s/def :cognitect.aws.glue.ListRegistriesInput/MaxResults :cognitect.aws.glue/MaxResultsNumber)
(s/def
:cognitect.aws.glue.ListRegistriesInput/NextToken
:cognitect.aws.glue/SchemaRegistryTokenString)
(s/def :cognitect.aws.glue.UpdateWorkflowResponse/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateRegistryResponse/RegistryName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.UpdateRegistryResponse/RegistryArn :cognitect.aws.glue/GlueResourceArn)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataResponse/UnfilteredPartitions
:cognitect.aws.glue/UnfilteredPartitionList)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataResponse/NextToken
:cognitect.aws.glue/Token)
(s/def
:cognitect.aws.glue.DataQualityRulesetEvaluationRunFilter/DataSource
:cognitect.aws.glue/DataSource)
(s/def
:cognitect.aws.glue.DataQualityRulesetEvaluationRunFilter/StartedBefore
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.DataQualityRulesetEvaluationRunFilter/StartedAfter
:cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.BatchGetBlueprintsResponse/Blueprints :cognitect.aws.glue/Blueprints)
(s/def
:cognitect.aws.glue.BatchGetBlueprintsResponse/MissingBlueprints
:cognitect.aws.glue/BlueprintNames)
(s/def :cognitect.aws.glue.GetPlanResponse/PythonScript :cognitect.aws.glue/PythonScript)
(s/def :cognitect.aws.glue.GetPlanResponse/ScalaCode :cognitect.aws.glue/ScalaCode)
(s/def
:cognitect.aws.glue.StartExportLabelsTaskRunResponse/TaskRunId
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.SchemaVersionNumber/LatestVersion
:cognitect.aws.glue/LatestSchemaVersionBoolean)
(s/def :cognitect.aws.glue.SchemaVersionNumber/VersionNumber :cognitect.aws.glue/VersionLongNumber)
(s/def
:cognitect.aws.glue.GetDataQualityResultResponse/RulesetEvaluationRunId
:cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.GetDataQualityResultResponse/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetDataQualityResultResponse/ResultId :cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.GetDataQualityResultResponse/Score
:cognitect.aws.glue/GenericBoundedDouble)
(s/def :cognitect.aws.glue.GetDataQualityResultResponse/CompletedOn :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.GetDataQualityResultResponse/StartedOn :cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.GetDataQualityResultResponse/EvaluationContext
:cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.GetDataQualityResultResponse/RulesetName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetDataQualityResultResponse/RuleResults
:cognitect.aws.glue/DataQualityRuleResults)
(s/def :cognitect.aws.glue.GetDataQualityResultResponse/DataSource :cognitect.aws.glue/DataSource)
(s/def :cognitect.aws.glue.GetDataQualityResultResponse/JobRunId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.DeleteDataQualityRulesetRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.IcebergTarget/Paths :cognitect.aws.glue/PathList)
(s/def :cognitect.aws.glue.IcebergTarget/ConnectionName :cognitect.aws.glue/ConnectionName)
(s/def :cognitect.aws.glue.IcebergTarget/Exclusions :cognitect.aws.glue/PathList)
(s/def :cognitect.aws.glue.IcebergTarget/MaximumTraversalDepth :cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.GetMLTransformsRequest/NextToken :cognitect.aws.glue/PaginationToken)
(s/def :cognitect.aws.glue.GetMLTransformsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def
:cognitect.aws.glue.GetMLTransformsRequest/Filter
:cognitect.aws.glue/TransformFilterCriteria)
(s/def :cognitect.aws.glue.GetMLTransformsRequest/Sort :cognitect.aws.glue/TransformSortCriteria)
(s/def
:cognitect.aws.glue.GetBlueprintRunRequest/BlueprintName
:cognitect.aws.glue/OrchestrationNameString)
(s/def :cognitect.aws.glue.GetBlueprintRunRequest/RunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.RunStatementRequest/SessionId :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.RunStatementRequest/Code
:cognitect.aws.glue/OrchestrationStatementCodeString)
(s/def
:cognitect.aws.glue.RunStatementRequest/RequestOrigin
:cognitect.aws.glue/OrchestrationNameString)
(s/def :cognitect.aws.glue.GetConnectionsFilter/MatchCriteria :cognitect.aws.glue/MatchCriteria)
(s/def :cognitect.aws.glue.GetConnectionsFilter/ConnectionType :cognitect.aws.glue/ConnectionType)
(s/def :cognitect.aws.glue.PartitionIndex/Keys :cognitect.aws.glue/KeyList)
(s/def :cognitect.aws.glue.PartitionIndex/IndexName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.StopCrawlerRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.JoinColumn/From :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.JoinColumn/Keys :cognitect.aws.glue/GlueStudioPathList)
(s/def :cognitect.aws.glue.DirectKinesisSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.DirectKinesisSource/WindowSize :cognitect.aws.glue/BoxedPositiveInt)
(s/def :cognitect.aws.glue.DirectKinesisSource/DetectSchema :cognitect.aws.glue/BoxedBoolean)
(s/def
:cognitect.aws.glue.DirectKinesisSource/StreamingOptions
:cognitect.aws.glue/KinesisStreamingSourceOptions)
(s/def
:cognitect.aws.glue.DirectKinesisSource/DataPreviewOptions
:cognitect.aws.glue/StreamingDataPreviewOptions)
(s/def
:cognitect.aws.glue.DeletePartitionIndexRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.DeletePartitionIndexRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DeletePartitionIndexRequest/TableName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DeletePartitionIndexRequest/IndexName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.StartMLEvaluationTaskRunRequest/TransformId
:cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.RunStatementResponse/Id :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.QuerySchemaVersionMetadataInput/SchemaId :cognitect.aws.glue/SchemaId)
(s/def
:cognitect.aws.glue.QuerySchemaVersionMetadataInput/SchemaVersionNumber
:cognitect.aws.glue/SchemaVersionNumber)
(s/def
:cognitect.aws.glue.QuerySchemaVersionMetadataInput/SchemaVersionId
:cognitect.aws.glue/SchemaVersionIdString)
(s/def
:cognitect.aws.glue.QuerySchemaVersionMetadataInput/MetadataList
:cognitect.aws.glue/MetadataList)
(s/def
:cognitect.aws.glue.QuerySchemaVersionMetadataInput/MaxResults
:cognitect.aws.glue/QuerySchemaVersionMetadataMaxResults)
(s/def
:cognitect.aws.glue.QuerySchemaVersionMetadataInput/NextToken
:cognitect.aws.glue/SchemaRegistryTokenString)
(s/def :cognitect.aws.glue.GetClassifierResponse/Classifier :cognitect.aws.glue/Classifier)
(s/def :cognitect.aws.glue.DevEndpoint/AvailabilityZone :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.DevEndpoint/EndpointName :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.DevEndpoint/NumberOfNodes :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.DevEndpoint/PublicAddress :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.DevEndpoint/NumberOfWorkers :cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.DevEndpoint/YarnEndpointAddress :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.DevEndpoint/SecurityConfiguration :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DevEndpoint/LastUpdateStatus :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.DevEndpoint/PrivateAddress :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.DevEndpoint/Arguments :cognitect.aws.glue/MapValue)
(s/def :cognitect.aws.glue.DevEndpoint/PublicKeys :cognitect.aws.glue/PublicKeysList)
(s/def :cognitect.aws.glue.DevEndpoint/PublicKey :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.DevEndpoint/WorkerType :cognitect.aws.glue/WorkerType)
(s/def :cognitect.aws.glue.DevEndpoint/RoleArn :cognitect.aws.glue/RoleArn)
(s/def :cognitect.aws.glue.DevEndpoint/SubnetId :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.DevEndpoint/ExtraJarsS3Path :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.DevEndpoint/ZeppelinRemoteSparkInterpreterPort
:cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.DevEndpoint/CreatedTimestamp :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.DevEndpoint/ExtraPythonLibsS3Path :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.DevEndpoint/GlueVersion :cognitect.aws.glue/GlueVersionString)
(s/def :cognitect.aws.glue.DevEndpoint/SecurityGroupIds :cognitect.aws.glue/StringList)
(s/def :cognitect.aws.glue.DevEndpoint/FailureReason :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.DevEndpoint/LastModifiedTimestamp :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.DevEndpoint/VpcId :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.DevEndpoint/Status :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.TransformEncryption/MlUserDataEncryption
:cognitect.aws.glue/MLUserDataEncryption)
(s/def
:cognitect.aws.glue.TransformEncryption/TaskRunSecurityConfigurationName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.BatchDeleteConnectionRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.BatchDeleteConnectionRequest/ConnectionNameList
:cognitect.aws.glue/DeleteConnectionNameList)
(s/def :cognitect.aws.glue.Database/Parameters :cognitect.aws.glue/ParametersMap)
(s/def :cognitect.aws.glue.Database/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.Database/TargetDatabase :cognitect.aws.glue/DatabaseIdentifier)
(s/def
:cognitect.aws.glue.Database/CreateTableDefaultPermissions
:cognitect.aws.glue/PrincipalPermissionsList)
(s/def :cognitect.aws.glue.Database/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Database/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.Database/FederatedDatabase :cognitect.aws.glue/FederatedDatabase)
(s/def :cognitect.aws.glue.Database/CreateTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.Database/LocationUri :cognitect.aws.glue/URI)
(s/def
:cognitect.aws.glue.EncryptionAtRest/CatalogEncryptionMode
:cognitect.aws.glue/CatalogEncryptionMode)
(s/def :cognitect.aws.glue.EncryptionAtRest/SseAwsKmsKeyId :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.AmazonRedshiftTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.AmazonRedshiftTarget/Data :cognitect.aws.glue/AmazonRedshiftNodeData)
(s/def :cognitect.aws.glue.AmazonRedshiftTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.GetWorkflowResponse/Workflow :cognitect.aws.glue/Workflow)
(s/def
:cognitect.aws.glue.BatchUpdatePartitionResponse/Errors
:cognitect.aws.glue/BatchUpdatePartitionFailureList)
(s/def
:cognitect.aws.glue.CancelDataQualityRuleRecommendationRunRequest/RunId
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/SupportedPermissionTypes
:cognitect.aws.glue/PermissionTypeList)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/NextToken
:cognitect.aws.glue/Token)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/Expression
:cognitect.aws.glue/PredicateString)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/Segment
:cognitect.aws.glue/Segment)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/TableName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/MaxResults
:cognitect.aws.glue/PageSize)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/AuditContext
:cognitect.aws.glue/AuditContext)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionsMetadataRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GluePolicy/PolicyInJson :cognitect.aws.glue/PolicyJsonString)
(s/def :cognitect.aws.glue.GluePolicy/PolicyHash :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.GluePolicy/CreateTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.GluePolicy/UpdateTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.CreateSessionResponse/Session :cognitect.aws.glue/Session)
(s/def :cognitect.aws.glue.EvaluateDataQualityMultiFrame/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.EvaluateDataQualityMultiFrame/Inputs :cognitect.aws.glue/ManyInputs)
(s/def
:cognitect.aws.glue.EvaluateDataQualityMultiFrame/AdditionalDataSources
:cognitect.aws.glue/DQDLAliases)
(s/def :cognitect.aws.glue.EvaluateDataQualityMultiFrame/Ruleset :cognitect.aws.glue/DQDLString)
(s/def
:cognitect.aws.glue.EvaluateDataQualityMultiFrame/PublishingOptions
:cognitect.aws.glue/DQResultsPublishingOptions)
(s/def
:cognitect.aws.glue.EvaluateDataQualityMultiFrame/AdditionalOptions
:cognitect.aws.glue/DQAdditionalOptions)
(s/def
:cognitect.aws.glue.EvaluateDataQualityMultiFrame/StopJobOnFailureOptions
:cognitect.aws.glue/DQStopJobOnFailureOptions)
(s/def :cognitect.aws.glue.GetPartitionsRequest/QueryAsOfTime :cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.GetPartitionsRequest/ExcludeColumnSchema
:cognitect.aws.glue/BooleanNullable)
(s/def :cognitect.aws.glue.GetPartitionsRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.GetPartitionsRequest/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.GetPartitionsRequest/Expression :cognitect.aws.glue/PredicateString)
(s/def :cognitect.aws.glue.GetPartitionsRequest/Segment :cognitect.aws.glue/Segment)
(s/def
:cognitect.aws.glue.GetPartitionsRequest/TransactionId
:cognitect.aws.glue/TransactionIdString)
(s/def :cognitect.aws.glue.GetPartitionsRequest/TableName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetPartitionsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.GetPartitionsRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetCatalogImportStatusResponse/ImportStatus
:cognitect.aws.glue/CatalogImportStatus)
(s/def :cognitect.aws.glue.DatabaseIdentifier/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.DatabaseIdentifier/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DatabaseIdentifier/Region :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.ConnectionPasswordEncryption/ReturnConnectionPasswordEncrypted
:cognitect.aws.glue/Boolean)
(s/def :cognitect.aws.glue.ConnectionPasswordEncryption/AwsKmsKeyId :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.TaskRunSortCriteria/Column :cognitect.aws.glue/TaskRunSortColumnType)
(s/def :cognitect.aws.glue.TaskRunSortCriteria/SortDirection :cognitect.aws.glue/SortDirectionType)
(s/def
:cognitect.aws.glue.StringColumnStatisticsData/MaximumLength
:cognitect.aws.glue/NonNegativeLong)
(s/def
:cognitect.aws.glue.StringColumnStatisticsData/AverageLength
:cognitect.aws.glue/NonNegativeDouble)
(s/def
:cognitect.aws.glue.StringColumnStatisticsData/NumberOfNulls
:cognitect.aws.glue/NonNegativeLong)
(s/def
:cognitect.aws.glue.StringColumnStatisticsData/NumberOfDistinctValues
:cognitect.aws.glue/NonNegativeLong)
(s/def :cognitect.aws.glue.Aggregate/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.Aggregate/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.Aggregate/Groups :cognitect.aws.glue/GlueStudioPathList)
(s/def :cognitect.aws.glue.Aggregate/Aggs :cognitect.aws.glue/AggregateOperations)
(s/def :cognitect.aws.glue.GetJobRunsRequest/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetJobRunsRequest/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.GetJobRunsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/ErrorString
:cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/Timeout
:cognitect.aws.glue/Timeout)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/RunId
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/ExecutionTime
:cognitect.aws.glue/ExecutionTime)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/NumberOfWorkers
:cognitect.aws.glue/NullableInteger)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/CompletedOn
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/LastModifiedOn
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/StartedOn
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/AdditionalRunOptions
:cognitect.aws.glue/DataQualityEvaluationRunAdditionalRunOptions)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/RulesetNames
:cognitect.aws.glue/RulesetNames)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/Role
:cognitect.aws.glue/RoleString)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/DataSource
:cognitect.aws.glue/DataSource)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/AdditionalDataSources
:cognitect.aws.glue/DataSourceMap)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/ResultIds
:cognitect.aws.glue/DataQualityResultIdList)
(s/def
:cognitect.aws.glue.GetDataQualityRulesetEvaluationRunResponse/Status
:cognitect.aws.glue/TaskStatusType)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/Classification
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/EndingOffsets
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/StartingTimestamp
:cognitect.aws.glue/Iso8601DateTime)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/TopicName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/NumRetries
:cognitect.aws.glue/BoxedNonNegativeInt)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/IncludeHeaders
:cognitect.aws.glue/BoxedBoolean)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/StartingOffsets
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/Assign
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/Delimiter
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/SecurityProtocol
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/ConnectionName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/MaxOffsetsPerTrigger
:cognitect.aws.glue/BoxedNonNegativeLong)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/BootstrapServers
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/MinPartitions
:cognitect.aws.glue/BoxedNonNegativeInt)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/AddRecordTimestamp
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/EmitConsumerLagMetrics
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/RetryIntervalMs
:cognitect.aws.glue/BoxedNonNegativeLong)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/PollTimeoutMs
:cognitect.aws.glue/BoxedNonNegativeLong)
(s/def
:cognitect.aws.glue.KafkaStreamingSourceOptions/SubscribePattern
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.WorkflowRun/PreviousRunId :cognitect.aws.glue/IdString)
(s/def
:cognitect.aws.glue.WorkflowRun/StartingEventBatchCondition
:cognitect.aws.glue/StartingEventBatchCondition)
(s/def :cognitect.aws.glue.WorkflowRun/ErrorMessage :cognitect.aws.glue/ErrorString)
(s/def :cognitect.aws.glue.WorkflowRun/CompletedOn :cognitect.aws.glue/TimestampValue)
(s/def
:cognitect.aws.glue.WorkflowRun/WorkflowRunProperties
:cognitect.aws.glue/WorkflowRunProperties)
(s/def :cognitect.aws.glue.WorkflowRun/StartedOn :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.WorkflowRun/Statistics :cognitect.aws.glue/WorkflowRunStatistics)
(s/def :cognitect.aws.glue.WorkflowRun/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.WorkflowRun/Graph :cognitect.aws.glue/WorkflowGraph)
(s/def :cognitect.aws.glue.WorkflowRun/WorkflowRunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.WorkflowRun/Status :cognitect.aws.glue/WorkflowRunStatus)
(s/def :cognitect.aws.glue.GetWorkflowRunRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetWorkflowRunRequest/RunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.GetWorkflowRunRequest/IncludeGraph :cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.ConnectionsList/Connections :cognitect.aws.glue/OrchestrationStringList)
(s/def :cognitect.aws.glue.S3HudiCatalogTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.S3HudiCatalogTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.S3HudiCatalogTarget/PartitionKeys :cognitect.aws.glue/GlueStudioPathList)
(s/def :cognitect.aws.glue.S3HudiCatalogTarget/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.S3HudiCatalogTarget/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.S3HudiCatalogTarget/AdditionalOptions
:cognitect.aws.glue/AdditionalOptions)
(s/def
:cognitect.aws.glue.S3HudiCatalogTarget/SchemaChangePolicy
:cognitect.aws.glue/CatalogSchemaChangePolicy)
(s/def :cognitect.aws.glue.GetJobRunResponse/JobRun :cognitect.aws.glue/JobRun)
(s/def :cognitect.aws.glue.StorageDescriptor/SortColumns :cognitect.aws.glue/OrderList)
(s/def :cognitect.aws.glue.StorageDescriptor/OutputFormat :cognitect.aws.glue/FormatString)
(s/def :cognitect.aws.glue.StorageDescriptor/NumberOfBuckets :cognitect.aws.glue/Integer)
(s/def :cognitect.aws.glue.StorageDescriptor/Parameters :cognitect.aws.glue/ParametersMap)
(s/def :cognitect.aws.glue.StorageDescriptor/Compressed :cognitect.aws.glue/Boolean)
(s/def :cognitect.aws.glue.StorageDescriptor/StoredAsSubDirectories :cognitect.aws.glue/Boolean)
(s/def :cognitect.aws.glue.StorageDescriptor/Columns :cognitect.aws.glue/ColumnList)
(s/def :cognitect.aws.glue.StorageDescriptor/SerdeInfo :cognitect.aws.glue/SerDeInfo)
(s/def
:cognitect.aws.glue.StorageDescriptor/AdditionalLocations
:cognitect.aws.glue/LocationStringList)
(s/def :cognitect.aws.glue.StorageDescriptor/BucketColumns :cognitect.aws.glue/NameStringList)
(s/def :cognitect.aws.glue.StorageDescriptor/InputFormat :cognitect.aws.glue/FormatString)
(s/def :cognitect.aws.glue.StorageDescriptor/SkewedInfo :cognitect.aws.glue/SkewedInfo)
(s/def :cognitect.aws.glue.StorageDescriptor/Location :cognitect.aws.glue/LocationString)
(s/def :cognitect.aws.glue.StorageDescriptor/SchemaReference :cognitect.aws.glue/SchemaReference)
(s/def :cognitect.aws.glue.StreamingDataPreviewOptions/PollingTime :cognitect.aws.glue/PollingTime)
(s/def
:cognitect.aws.glue.StreamingDataPreviewOptions/RecordPollingLimit
:cognitect.aws.glue/PositiveLong)
(s/def :cognitect.aws.glue.GetConnectionsResponse/ConnectionList :cognitect.aws.glue/ConnectionList)
(s/def :cognitect.aws.glue.GetConnectionsResponse/NextToken :cognitect.aws.glue/Token)
(s/def
:cognitect.aws.glue.StartDataQualityRulesetEvaluationRunResponse/RunId
:cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.UpdateDataQualityRulesetRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateDataQualityRulesetRequest/Description
:cognitect.aws.glue/DescriptionString)
(s/def
:cognitect.aws.glue.UpdateDataQualityRulesetRequest/Ruleset
:cognitect.aws.glue/DataQualityRulesetString)
(s/def :cognitect.aws.glue.MLTransform/MaxRetries :cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.MLTransform/Timeout :cognitect.aws.glue/Timeout)
(s/def :cognitect.aws.glue.MLTransform/NumberOfWorkers :cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.MLTransform/TransformId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.MLTransform/MaxCapacity :cognitect.aws.glue/NullableDouble)
(s/def :cognitect.aws.glue.MLTransform/Parameters :cognitect.aws.glue/TransformParameters)
(s/def :cognitect.aws.glue.MLTransform/LabelCount :cognitect.aws.glue/LabelCount)
(s/def :cognitect.aws.glue.MLTransform/TransformEncryption :cognitect.aws.glue/TransformEncryption)
(s/def :cognitect.aws.glue.MLTransform/LastModifiedOn :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.MLTransform/EvaluationMetrics :cognitect.aws.glue/EvaluationMetrics)
(s/def :cognitect.aws.glue.MLTransform/WorkerType :cognitect.aws.glue/WorkerType)
(s/def :cognitect.aws.glue.MLTransform/Schema :cognitect.aws.glue/TransformSchema)
(s/def :cognitect.aws.glue.MLTransform/CreatedOn :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.MLTransform/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.MLTransform/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.MLTransform/GlueVersion :cognitect.aws.glue/GlueVersionString)
(s/def :cognitect.aws.glue.MLTransform/Role :cognitect.aws.glue/RoleString)
(s/def :cognitect.aws.glue.MLTransform/InputRecordTables :cognitect.aws.glue/GlueTables)
(s/def :cognitect.aws.glue.MLTransform/Status :cognitect.aws.glue/TransformStatusType)
(s/def
:cognitect.aws.glue.GetUserDefinedFunctionsResponse/UserDefinedFunctions
:cognitect.aws.glue/UserDefinedFunctionList)
(s/def :cognitect.aws.glue.GetUserDefinedFunctionsResponse/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.DeleteSessionRequest/Id :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.DeleteSessionRequest/RequestOrigin
:cognitect.aws.glue/OrchestrationNameString)
(s/def
:cognitect.aws.glue.BatchDeletePartitionRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.BatchDeletePartitionRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.BatchDeletePartitionRequest/TableName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.BatchDeletePartitionRequest/PartitionsToDelete
:cognitect.aws.glue/BatchDeletePartitionValueList)
(s/def
:cognitect.aws.glue.CreateSchemaResponse/SchemaVersionId
:cognitect.aws.glue/SchemaVersionIdString)
(s/def
:cognitect.aws.glue.CreateSchemaResponse/SchemaName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def
:cognitect.aws.glue.CreateSchemaResponse/SchemaVersionStatus
:cognitect.aws.glue/SchemaVersionStatus)
(s/def
:cognitect.aws.glue.CreateSchemaResponse/SchemaCheckpoint
:cognitect.aws.glue/SchemaCheckpointNumber)
(s/def :cognitect.aws.glue.CreateSchemaResponse/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.CreateSchemaResponse/SchemaArn :cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.CreateSchemaResponse/SchemaStatus :cognitect.aws.glue/SchemaStatus)
(s/def :cognitect.aws.glue.CreateSchemaResponse/RegistryArn :cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.CreateSchemaResponse/DataFormat :cognitect.aws.glue/DataFormat)
(s/def
:cognitect.aws.glue.CreateSchemaResponse/NextSchemaVersion
:cognitect.aws.glue/VersionLongNumber)
(s/def :cognitect.aws.glue.CreateSchemaResponse/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.CreateSchemaResponse/Compatibility :cognitect.aws.glue/Compatibility)
(s/def
:cognitect.aws.glue.CreateSchemaResponse/LatestSchemaVersion
:cognitect.aws.glue/VersionLongNumber)
(s/def
:cognitect.aws.glue.CreateSchemaResponse/RegistryName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.ListWorkflowsRequest/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.ListWorkflowsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.PartitionError/PartitionValues :cognitect.aws.glue/ValueStringList)
(s/def :cognitect.aws.glue.PartitionError/ErrorDetail :cognitect.aws.glue/ErrorDetail)
(s/def
:cognitect.aws.glue.CreateClassifierRequest/GrokClassifier
:cognitect.aws.glue/CreateGrokClassifierRequest)
(s/def
:cognitect.aws.glue.CreateClassifierRequest/XMLClassifier
:cognitect.aws.glue/CreateXMLClassifierRequest)
(s/def
:cognitect.aws.glue.CreateClassifierRequest/JsonClassifier
:cognitect.aws.glue/CreateJsonClassifierRequest)
(s/def
:cognitect.aws.glue.CreateClassifierRequest/CsvClassifier
:cognitect.aws.glue/CreateCsvClassifierRequest)
(s/def :cognitect.aws.glue.DataQualityTargetTable/TableName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DataQualityTargetTable/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DataQualityTargetTable/CatalogId :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetMLTransformRequest/TransformId :cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.StartDataQualityRuleRecommendationRunRequest/DataSource
:cognitect.aws.glue/DataSource)
(s/def
:cognitect.aws.glue.StartDataQualityRuleRecommendationRunRequest/Role
:cognitect.aws.glue/RoleString)
(s/def
:cognitect.aws.glue.StartDataQualityRuleRecommendationRunRequest/NumberOfWorkers
:cognitect.aws.glue/NullableInteger)
(s/def
:cognitect.aws.glue.StartDataQualityRuleRecommendationRunRequest/Timeout
:cognitect.aws.glue/Timeout)
(s/def
:cognitect.aws.glue.StartDataQualityRuleRecommendationRunRequest/CreatedRulesetName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.StartDataQualityRuleRecommendationRunRequest/ClientToken
:cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.ConfusionMatrix/NumTruePositives :cognitect.aws.glue/RecordsCount)
(s/def :cognitect.aws.glue.ConfusionMatrix/NumFalsePositives :cognitect.aws.glue/RecordsCount)
(s/def :cognitect.aws.glue.ConfusionMatrix/NumTrueNegatives :cognitect.aws.glue/RecordsCount)
(s/def :cognitect.aws.glue.ConfusionMatrix/NumFalseNegatives :cognitect.aws.glue/RecordsCount)
(s/def :cognitect.aws.glue.CatalogSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.CatalogSource/Database :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.CatalogSource/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.StopTriggerRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.S3DeltaDirectTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.S3DeltaDirectTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.S3DeltaDirectTarget/PartitionKeys :cognitect.aws.glue/GlueStudioPathList)
(s/def :cognitect.aws.glue.S3DeltaDirectTarget/Path :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.S3DeltaDirectTarget/Compression
:cognitect.aws.glue/DeltaTargetCompressionType)
(s/def :cognitect.aws.glue.S3DeltaDirectTarget/Format :cognitect.aws.glue/TargetFormat)
(s/def
:cognitect.aws.glue.S3DeltaDirectTarget/AdditionalOptions
:cognitect.aws.glue/AdditionalOptions)
(s/def
:cognitect.aws.glue.S3DeltaDirectTarget/SchemaChangePolicy
:cognitect.aws.glue/DirectSchemaChangePolicy)
(s/def
:cognitect.aws.glue.CheckSchemaVersionValidityInput/DataFormat
:cognitect.aws.glue/DataFormat)
(s/def
:cognitect.aws.glue.CheckSchemaVersionValidityInput/SchemaDefinition
:cognitect.aws.glue/SchemaDefinitionString)
(s/def :cognitect.aws.glue.ListCrawlsResponse/Crawls :cognitect.aws.glue/CrawlerHistoryList)
(s/def :cognitect.aws.glue.ListCrawlsResponse/NextToken :cognitect.aws.glue/Token)
(s/def
:cognitect.aws.glue.BatchStopJobRunSuccessfulSubmission/JobName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.BatchStopJobRunSuccessfulSubmission/JobRunId
:cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.SearchTablesResponse/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.SearchTablesResponse/TableList :cognitect.aws.glue/TableList)
(s/def
:cognitect.aws.glue.DirectSchemaChangePolicy/EnableUpdateCatalog
:cognitect.aws.glue/BoxedBoolean)
(s/def
:cognitect.aws.glue.DirectSchemaChangePolicy/UpdateBehavior
:cognitect.aws.glue/UpdateCatalogBehavior)
(s/def
:cognitect.aws.glue.DirectSchemaChangePolicy/Table
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.DirectSchemaChangePolicy/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.ListDataQualityRulesetEvaluationRunsResponse/Runs
:cognitect.aws.glue/DataQualityRulesetEvaluationRunList)
(s/def
:cognitect.aws.glue.ListDataQualityRulesetEvaluationRunsResponse/NextToken
:cognitect.aws.glue/PaginationToken)
(s/def :cognitect.aws.glue.BatchDeleteTableResponse/Errors :cognitect.aws.glue/TableErrors)
(s/def :cognitect.aws.glue.CatalogImportStatus/ImportCompleted :cognitect.aws.glue/Boolean)
(s/def :cognitect.aws.glue.CatalogImportStatus/ImportTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.CatalogImportStatus/ImportedBy :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetResourcePoliciesRequest/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.GetResourcePoliciesRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.GetCrawlerRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.BlueprintRun/BlueprintName :cognitect.aws.glue/OrchestrationNameString)
(s/def :cognitect.aws.glue.BlueprintRun/RunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.BlueprintRun/ErrorMessage :cognitect.aws.glue/MessageString)
(s/def :cognitect.aws.glue.BlueprintRun/CompletedOn :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.BlueprintRun/RollbackErrorMessage :cognitect.aws.glue/MessageString)
(s/def :cognitect.aws.glue.BlueprintRun/Parameters :cognitect.aws.glue/BlueprintParameters)
(s/def :cognitect.aws.glue.BlueprintRun/StartedOn :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.BlueprintRun/WorkflowName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.BlueprintRun/RoleArn :cognitect.aws.glue/OrchestrationIAMRoleArn)
(s/def :cognitect.aws.glue.BlueprintRun/State :cognitect.aws.glue/BlueprintRunState)
(s/def :cognitect.aws.glue.SparkSQL/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.SparkSQL/Inputs :cognitect.aws.glue/ManyInputs)
(s/def :cognitect.aws.glue.SparkSQL/SqlQuery :cognitect.aws.glue/SqlQuery)
(s/def :cognitect.aws.glue.SparkSQL/SqlAliases :cognitect.aws.glue/SqlAliases)
(s/def :cognitect.aws.glue.SparkSQL/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def :cognitect.aws.glue.ListCrawlsRequest/CrawlerName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.ListCrawlsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.ListCrawlsRequest/Filters :cognitect.aws.glue/CrawlsFilterList)
(s/def :cognitect.aws.glue.ListCrawlsRequest/NextToken :cognitect.aws.glue/Token)
(s/def
:cognitect.aws.glue.BatchDeleteConnectionResponse/Succeeded
:cognitect.aws.glue/NameStringList)
(s/def :cognitect.aws.glue.BatchDeleteConnectionResponse/Errors :cognitect.aws.glue/ErrorByName)
(s/def :cognitect.aws.glue.S3DeltaSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.S3DeltaSource/Paths :cognitect.aws.glue/EnclosedInStringProperties)
(s/def
:cognitect.aws.glue.S3DeltaSource/AdditionalDeltaOptions
:cognitect.aws.glue/AdditionalOptions)
(s/def
:cognitect.aws.glue.S3DeltaSource/AdditionalOptions
:cognitect.aws.glue/S3DirectSourceAdditionalOptions)
(s/def :cognitect.aws.glue.S3DeltaSource/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def :cognitect.aws.glue.Blueprint/ErrorMessage :cognitect.aws.glue/ErrorString)
(s/def :cognitect.aws.glue.Blueprint/LastModifiedOn :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.Blueprint/ParameterSpec :cognitect.aws.glue/BlueprintParameterSpec)
(s/def :cognitect.aws.glue.Blueprint/BlueprintLocation :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.Blueprint/CreatedOn :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.Blueprint/Name :cognitect.aws.glue/OrchestrationNameString)
(s/def :cognitect.aws.glue.Blueprint/Description :cognitect.aws.glue/Generic512CharString)
(s/def :cognitect.aws.glue.Blueprint/LastActiveDefinition :cognitect.aws.glue/LastActiveDefinition)
(s/def :cognitect.aws.glue.Blueprint/BlueprintServiceLocation :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.Blueprint/Status :cognitect.aws.glue/BlueprintStatus)
(s/def :cognitect.aws.glue.GetDatabasesRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.GetDatabasesRequest/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.GetDatabasesRequest/MaxResults :cognitect.aws.glue/CatalogGetterPageSize)
(s/def
:cognitect.aws.glue.GetDatabasesRequest/ResourceShareType
:cognitect.aws.glue/ResourceShareType)
(s/def
:cognitect.aws.glue.TransformConfigParameter/Name
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.TransformConfigParameter/Type :cognitect.aws.glue/ParamType)
(s/def
:cognitect.aws.glue.TransformConfigParameter/ValidationRule
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.TransformConfigParameter/ValidationMessage
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.TransformConfigParameter/Value
:cognitect.aws.glue/EnclosedInStringProperties)
(s/def :cognitect.aws.glue.TransformConfigParameter/ListType :cognitect.aws.glue/ParamType)
(s/def :cognitect.aws.glue.TransformConfigParameter/IsOptional :cognitect.aws.glue/BoxedBoolean)
(s/def
:cognitect.aws.glue.ListDataQualityResultsResponse/Results
:cognitect.aws.glue/DataQualityResultDescriptionList)
(s/def
:cognitect.aws.glue.ListDataQualityResultsResponse/NextToken
:cognitect.aws.glue/PaginationToken)
(s/def :cognitect.aws.glue.DataQualityResultDescription/ResultId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.DataQualityResultDescription/DataSource :cognitect.aws.glue/DataSource)
(s/def :cognitect.aws.glue.DataQualityResultDescription/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DataQualityResultDescription/JobRunId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.DataQualityResultDescription/StartedOn :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.S3CsvSource/Multiline :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.S3CsvSource/CompressionType :cognitect.aws.glue/CompressionType)
(s/def :cognitect.aws.glue.S3CsvSource/Recurse :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.S3CsvSource/QuoteChar :cognitect.aws.glue/QuoteChar)
(s/def :cognitect.aws.glue.S3CsvSource/Paths :cognitect.aws.glue/EnclosedInStringProperties)
(s/def :cognitect.aws.glue.S3CsvSource/Separator :cognitect.aws.glue/Separator)
(s/def
:cognitect.aws.glue.S3CsvSource/AdditionalOptions
:cognitect.aws.glue/S3DirectSourceAdditionalOptions)
(s/def :cognitect.aws.glue.S3CsvSource/WithHeader :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.S3CsvSource/GroupSize :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.S3CsvSource/GroupFiles :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.S3CsvSource/WriteHeader :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.S3CsvSource/SkipFirst :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.S3CsvSource/MaxBand :cognitect.aws.glue/BoxedNonNegativeInt)
(s/def
:cognitect.aws.glue.S3CsvSource/Escaper
:cognitect.aws.glue/EnclosedInStringPropertyWithQuote)
(s/def :cognitect.aws.glue.S3CsvSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.S3CsvSource/MaxFilesInBand :cognitect.aws.glue/BoxedNonNegativeInt)
(s/def :cognitect.aws.glue.S3CsvSource/Exclusions :cognitect.aws.glue/EnclosedInStringProperties)
(s/def :cognitect.aws.glue.S3CsvSource/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def :cognitect.aws.glue.S3CsvSource/OptimizePerformance :cognitect.aws.glue/BooleanValue)
(s/def :cognitect.aws.glue.S3HudiSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.S3HudiSource/Paths :cognitect.aws.glue/EnclosedInStringProperties)
(s/def :cognitect.aws.glue.S3HudiSource/AdditionalHudiOptions :cognitect.aws.glue/AdditionalOptions)
(s/def
:cognitect.aws.glue.S3HudiSource/AdditionalOptions
:cognitect.aws.glue/S3DirectSourceAdditionalOptions)
(s/def :cognitect.aws.glue.S3HudiSource/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def :cognitect.aws.glue.CreateSecurityConfigurationRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.CreateSecurityConfigurationRequest/EncryptionConfiguration
:cognitect.aws.glue/EncryptionConfiguration)
(s/def :cognitect.aws.glue.SearchTablesRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.SearchTablesRequest/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.SearchTablesRequest/Filters :cognitect.aws.glue/SearchPropertyPredicates)
(s/def :cognitect.aws.glue.SearchTablesRequest/SearchText :cognitect.aws.glue/ValueString)
(s/def :cognitect.aws.glue.SearchTablesRequest/SortCriteria :cognitect.aws.glue/SortCriteria)
(s/def :cognitect.aws.glue.SearchTablesRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def
:cognitect.aws.glue.SearchTablesRequest/ResourceShareType
:cognitect.aws.glue/ResourceShareType)
(s/def :cognitect.aws.glue.WorkflowRunStatistics/TotalActions :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.WorkflowRunStatistics/TimeoutActions :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.WorkflowRunStatistics/FailedActions :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.WorkflowRunStatistics/StoppedActions :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.WorkflowRunStatistics/SucceededActions :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.WorkflowRunStatistics/RunningActions :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.WorkflowRunStatistics/ErroredActions :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.WorkflowRunStatistics/WaitingActions :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.CrawlerNodeDetails/Crawls :cognitect.aws.glue/CrawlList)
(s/def
:cognitect.aws.glue.DevEndpointCustomLibraries/ExtraPythonLibsS3Path
:cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.DevEndpointCustomLibraries/ExtraJarsS3Path
:cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.CreateTriggerRequest/StartOnCreation :cognitect.aws.glue/BooleanValue)
(s/def :cognitect.aws.glue.CreateTriggerRequest/Actions :cognitect.aws.glue/ActionList)
(s/def
:cognitect.aws.glue.CreateTriggerRequest/EventBatchingCondition
:cognitect.aws.glue/EventBatchingCondition)
(s/def :cognitect.aws.glue.CreateTriggerRequest/Schedule :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.CreateTriggerRequest/Predicate :cognitect.aws.glue/Predicate)
(s/def :cognitect.aws.glue.CreateTriggerRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.CreateTriggerRequest/WorkflowName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateTriggerRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateTriggerRequest/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.CreateTriggerRequest/Type :cognitect.aws.glue/TriggerType)
(s/def :cognitect.aws.glue.DynamoDBTarget/Path :cognitect.aws.glue/Path)
(s/def :cognitect.aws.glue.DynamoDBTarget/scanAll :cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.DynamoDBTarget/scanRate :cognitect.aws.glue/NullableDouble)
(s/def :cognitect.aws.glue.TableError/TableName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.TableError/ErrorDetail :cognitect.aws.glue/ErrorDetail)
(s/def :cognitect.aws.glue.BatchGetCrawlersRequest/CrawlerNames :cognitect.aws.glue/CrawlerNameList)
(s/def :cognitect.aws.glue.EvaluationMetrics/TransformType :cognitect.aws.glue/TransformType)
(s/def
:cognitect.aws.glue.EvaluationMetrics/FindMatchesMetrics
:cognitect.aws.glue/FindMatchesMetrics)
(s/def :cognitect.aws.glue.BatchGetJobsResponse/Jobs :cognitect.aws.glue/JobList)
(s/def :cognitect.aws.glue.BatchGetJobsResponse/JobsNotFound :cognitect.aws.glue/JobNameList)
(s/def :cognitect.aws.glue.SchemaVersionListItem/SchemaArn :cognitect.aws.glue/GlueResourceArn)
(s/def
:cognitect.aws.glue.SchemaVersionListItem/SchemaVersionId
:cognitect.aws.glue/SchemaVersionIdString)
(s/def
:cognitect.aws.glue.SchemaVersionListItem/VersionNumber
:cognitect.aws.glue/VersionLongNumber)
(s/def :cognitect.aws.glue.SchemaVersionListItem/Status :cognitect.aws.glue/SchemaVersionStatus)
(s/def :cognitect.aws.glue.SchemaVersionListItem/CreatedTime :cognitect.aws.glue/CreatedTimestamp)
(s/def :cognitect.aws.glue.ResourceUri/ResourceType :cognitect.aws.glue/ResourceType)
(s/def :cognitect.aws.glue.ResourceUri/Uri :cognitect.aws.glue/URI)
(s/def :cognitect.aws.glue.GetPartitionsResponse/Partitions :cognitect.aws.glue/PartitionList)
(s/def :cognitect.aws.glue.GetPartitionsResponse/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.GetDevEndpointResponse/DevEndpoint :cognitect.aws.glue/DevEndpoint)
(s/def :cognitect.aws.glue.Mapping/ToKey :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.Mapping/FromPath :cognitect.aws.glue/EnclosedInStringProperties)
(s/def :cognitect.aws.glue.Mapping/FromType :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.Mapping/ToType :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.Mapping/Dropped :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.Mapping/Children :cognitect.aws.glue/Mappings)
(s/def :cognitect.aws.glue.Job/MaxRetries :cognitect.aws.glue/MaxRetries)
(s/def :cognitect.aws.glue.Job/AllocatedCapacity :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.Job/Timeout :cognitect.aws.glue/Timeout)
(s/def :cognitect.aws.glue.Job/NumberOfWorkers :cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.Job/SecurityConfiguration :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Job/MaxCapacity :cognitect.aws.glue/NullableDouble)
(s/def :cognitect.aws.glue.Job/NotificationProperty :cognitect.aws.glue/NotificationProperty)
(s/def :cognitect.aws.glue.Job/LogUri :cognitect.aws.glue/UriString)
(s/def :cognitect.aws.glue.Job/LastModifiedOn :cognitect.aws.glue/TimestampValue)
(s/def
:cognitect.aws.glue.Job/CodeGenConfigurationNodes
:cognitect.aws.glue/CodeGenConfigurationNodes)
(s/def :cognitect.aws.glue.Job/WorkerType :cognitect.aws.glue/WorkerType)
(s/def :cognitect.aws.glue.Job/DefaultArguments :cognitect.aws.glue/GenericMap)
(s/def :cognitect.aws.glue.Job/Command :cognitect.aws.glue/JobCommand)
(s/def :cognitect.aws.glue.Job/ExecutionClass :cognitect.aws.glue/ExecutionClass)
(s/def :cognitect.aws.glue.Job/NonOverridableArguments :cognitect.aws.glue/GenericMap)
(s/def :cognitect.aws.glue.Job/ExecutionProperty :cognitect.aws.glue/ExecutionProperty)
(s/def :cognitect.aws.glue.Job/CreatedOn :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.Job/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Job/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.Job/GlueVersion :cognitect.aws.glue/GlueVersionString)
(s/def :cognitect.aws.glue.Job/Connections :cognitect.aws.glue/ConnectionsList)
(s/def :cognitect.aws.glue.Job/Role :cognitect.aws.glue/RoleString)
(s/def :cognitect.aws.glue.Job/SourceControlDetails :cognitect.aws.glue/SourceControlDetails)
(s/def :cognitect.aws.glue.SchemaColumn/Name :cognitect.aws.glue/ColumnNameString)
(s/def :cognitect.aws.glue.SchemaColumn/DataType :cognitect.aws.glue/ColumnTypeString)
(s/def :cognitect.aws.glue.PutResourcePolicyResponse/PolicyHash :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.DynamoDBCatalogSource/Name :cognitect.aws.glue/NodeName)
(s/def
:cognitect.aws.glue.DynamoDBCatalogSource/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.DynamoDBCatalogSource/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.CreateCrawlerRequest/LineageConfiguration
:cognitect.aws.glue/LineageConfiguration)
(s/def
:cognitect.aws.glue.CreateCrawlerRequest/CrawlerSecurityConfiguration
:cognitect.aws.glue/CrawlerSecurityConfiguration)
(s/def
:cognitect.aws.glue.CreateCrawlerRequest/SchemaChangePolicy
:cognitect.aws.glue/SchemaChangePolicy)
(s/def :cognitect.aws.glue.CreateCrawlerRequest/Schedule :cognitect.aws.glue/CronExpression)
(s/def :cognitect.aws.glue.CreateCrawlerRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.CreateCrawlerRequest/Targets :cognitect.aws.glue/CrawlerTargets)
(s/def :cognitect.aws.glue.CreateCrawlerRequest/Classifiers :cognitect.aws.glue/ClassifierNameList)
(s/def :cognitect.aws.glue.CreateCrawlerRequest/TablePrefix :cognitect.aws.glue/TablePrefix)
(s/def :cognitect.aws.glue.CreateCrawlerRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateCrawlerRequest/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.CreateCrawlerRequest/Role :cognitect.aws.glue/Role)
(s/def
:cognitect.aws.glue.CreateCrawlerRequest/Configuration
:cognitect.aws.glue/CrawlerConfiguration)
(s/def
:cognitect.aws.glue.CreateCrawlerRequest/LakeFormationConfiguration
:cognitect.aws.glue/LakeFormationConfiguration)
(s/def :cognitect.aws.glue.CreateCrawlerRequest/RecrawlPolicy :cognitect.aws.glue/RecrawlPolicy)
(s/def :cognitect.aws.glue.CreateCrawlerRequest/DatabaseName :cognitect.aws.glue/DatabaseName)
(s/def
:cognitect.aws.glue.DataQualityResultFilterCriteria/DataSource
:cognitect.aws.glue/DataSource)
(s/def :cognitect.aws.glue.DataQualityResultFilterCriteria/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DataQualityResultFilterCriteria/JobRunId :cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.DataQualityResultFilterCriteria/StartedAfter
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.DataQualityResultFilterCriteria/StartedBefore
:cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.ListWorkflowsResponse/Workflows :cognitect.aws.glue/WorkflowNames)
(s/def :cognitect.aws.glue.ListWorkflowsResponse/NextToken :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.LabelingSetGenerationTaskRunProperties/OutputS3Path
:cognitect.aws.glue/UriString)
(s/def :cognitect.aws.glue.DeleteDevEndpointRequest/EndpointName :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.GetDataCatalogEncryptionSettingsResponse/DataCatalogEncryptionSettings
:cognitect.aws.glue/DataCatalogEncryptionSettings)
(s/def :cognitect.aws.glue.TriggerNodeDetails/Trigger :cognitect.aws.glue/Trigger)
(s/def :cognitect.aws.glue.UserDefinedFunctionInput/FunctionName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UserDefinedFunctionInput/ClassName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UserDefinedFunctionInput/OwnerName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UserDefinedFunctionInput/OwnerType :cognitect.aws.glue/PrincipalType)
(s/def
:cognitect.aws.glue.UserDefinedFunctionInput/ResourceUris
:cognitect.aws.glue/ResourceUriList)
(s/def :cognitect.aws.glue.GetJobRequest/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.StopCrawlerScheduleRequest/CrawlerName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.TableVersionError/TableName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.TableVersionError/VersionId :cognitect.aws.glue/VersionString)
(s/def :cognitect.aws.glue.TableVersionError/ErrorDetail :cognitect.aws.glue/ErrorDetail)
(s/def :cognitect.aws.glue.GetMLTransformsResponse/Transforms :cognitect.aws.glue/TransformList)
(s/def :cognitect.aws.glue.GetMLTransformsResponse/NextToken :cognitect.aws.glue/PaginationToken)
(s/def
:cognitect.aws.glue.StartBlueprintRunRequest/BlueprintName
:cognitect.aws.glue/OrchestrationNameString)
(s/def
:cognitect.aws.glue.StartBlueprintRunRequest/Parameters
:cognitect.aws.glue/BlueprintParameters)
(s/def
:cognitect.aws.glue.StartBlueprintRunRequest/RoleArn
:cognitect.aws.glue/OrchestrationIAMRoleArn)
(s/def :cognitect.aws.glue.CreateCustomEntityTypeRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateCustomEntityTypeRequest/RegexString :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.CreateCustomEntityTypeRequest/ContextWords
:cognitect.aws.glue/ContextWords)
(s/def :cognitect.aws.glue.CreateCustomEntityTypeRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def
:cognitect.aws.glue.GetResourcePoliciesResponse/GetResourcePoliciesResponseList
:cognitect.aws.glue/GetResourcePoliciesResponseList)
(s/def :cognitect.aws.glue.GetResourcePoliciesResponse/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.ResumeWorkflowRunResponse/RunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.ResumeWorkflowRunResponse/NodeIds :cognitect.aws.glue/NodeIdList)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionMetadataRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionMetadataRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionMetadataRequest/TableName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionMetadataRequest/PartitionValues
:cognitect.aws.glue/ValueStringList)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionMetadataRequest/AuditContext
:cognitect.aws.glue/AuditContext)
(s/def
:cognitect.aws.glue.GetUnfilteredPartitionMetadataRequest/SupportedPermissionTypes
:cognitect.aws.glue/PermissionTypeList)
(s/def :cognitect.aws.glue.BasicCatalogTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.BasicCatalogTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.BasicCatalogTarget/Database :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.BasicCatalogTarget/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.ColumnStatistics/ColumnName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.ColumnStatistics/ColumnType :cognitect.aws.glue/TypeString)
(s/def :cognitect.aws.glue.ColumnStatistics/AnalyzedTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.ColumnStatistics/StatisticsData :cognitect.aws.glue/ColumnStatisticsData)
(s/def
:cognitect.aws.glue.JobBookmarksEncryption/JobBookmarksEncryptionMode
:cognitect.aws.glue/JobBookmarksEncryptionMode)
(s/def :cognitect.aws.glue.JobBookmarksEncryption/KmsKeyArn :cognitect.aws.glue/KmsKeyArn)
(s/def :cognitect.aws.glue.Workflow/LastModifiedOn :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.Workflow/MaxConcurrentRuns :cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.Workflow/CreatedOn :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.Workflow/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Workflow/Description :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.Workflow/Graph :cognitect.aws.glue/WorkflowGraph)
(s/def :cognitect.aws.glue.Workflow/DefaultRunProperties :cognitect.aws.glue/WorkflowRunProperties)
(s/def :cognitect.aws.glue.Workflow/BlueprintDetails :cognitect.aws.glue/BlueprintDetails)
(s/def :cognitect.aws.glue.Workflow/LastRun :cognitect.aws.glue/WorkflowRun)
(s/def :cognitect.aws.glue.Trigger/Actions :cognitect.aws.glue/ActionList)
(s/def
:cognitect.aws.glue.Trigger/EventBatchingCondition
:cognitect.aws.glue/EventBatchingCondition)
(s/def :cognitect.aws.glue.Trigger/Schedule :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.Trigger/Predicate :cognitect.aws.glue/Predicate)
(s/def :cognitect.aws.glue.Trigger/Id :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.Trigger/WorkflowName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Trigger/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Trigger/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.Trigger/Type :cognitect.aws.glue/TriggerType)
(s/def :cognitect.aws.glue.Trigger/State :cognitect.aws.glue/TriggerState)
(s/def :cognitect.aws.glue.SerDeInfo/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.SerDeInfo/SerializationLibrary :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.SerDeInfo/Parameters :cognitect.aws.glue/ParametersMap)
(s/def
:cognitect.aws.glue.ListDataQualityRulesetsResponse/Rulesets
:cognitect.aws.glue/DataQualityRulesetList)
(s/def
:cognitect.aws.glue.ListDataQualityRulesetsResponse/NextToken
:cognitect.aws.glue/PaginationToken)
(s/def :cognitect.aws.glue.UpdateSourceControlFromJobRequest/JobName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/AuthStrategy
:cognitect.aws.glue/SourceControlAuthStrategy)
(s/def
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/RepositoryName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/BranchName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/AuthToken
:cognitect.aws.glue/AuthTokenString)
(s/def
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/CommitId
:cognitect.aws.glue/CommitIdString)
(s/def :cognitect.aws.glue.UpdateSourceControlFromJobRequest/Folder :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/Provider
:cognitect.aws.glue/SourceControlProvider)
(s/def
:cognitect.aws.glue.UpdateSourceControlFromJobRequest/RepositoryOwner
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.StartImportLabelsTaskRunRequest/TransformId
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.StartImportLabelsTaskRunRequest/InputS3Path
:cognitect.aws.glue/UriString)
(s/def
:cognitect.aws.glue.StartImportLabelsTaskRunRequest/ReplaceAllLabels
:cognitect.aws.glue/ReplaceBoolean)
(s/def :cognitect.aws.glue.ListCrawlersResponse/CrawlerNames :cognitect.aws.glue/CrawlerNameList)
(s/def :cognitect.aws.glue.ListCrawlersResponse/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.StartJobRunRequest/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.StartJobRunRequest/AllocatedCapacity :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.StartJobRunRequest/Timeout :cognitect.aws.glue/Timeout)
(s/def :cognitect.aws.glue.StartJobRunRequest/NumberOfWorkers :cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.StartJobRunRequest/SecurityConfiguration :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.StartJobRunRequest/MaxCapacity :cognitect.aws.glue/NullableDouble)
(s/def
:cognitect.aws.glue.StartJobRunRequest/NotificationProperty
:cognitect.aws.glue/NotificationProperty)
(s/def :cognitect.aws.glue.StartJobRunRequest/Arguments :cognitect.aws.glue/GenericMap)
(s/def :cognitect.aws.glue.StartJobRunRequest/WorkerType :cognitect.aws.glue/WorkerType)
(s/def :cognitect.aws.glue.StartJobRunRequest/ExecutionClass :cognitect.aws.glue/ExecutionClass)
(s/def :cognitect.aws.glue.StartJobRunRequest/JobRunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.GetTagsRequest/ResourceArn :cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.S3CatalogHudiSource/Name :cognitect.aws.glue/NodeName)
(s/def
:cognitect.aws.glue.S3CatalogHudiSource/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.S3CatalogHudiSource/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.S3CatalogHudiSource/AdditionalHudiOptions
:cognitect.aws.glue/AdditionalOptions)
(s/def :cognitect.aws.glue.S3CatalogHudiSource/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def :cognitect.aws.glue.ImportLabelsTaskRunProperties/InputS3Path :cognitect.aws.glue/UriString)
(s/def :cognitect.aws.glue.ImportLabelsTaskRunProperties/Replace :cognitect.aws.glue/ReplaceBoolean)
(s/def :cognitect.aws.glue.JdbcTarget/ConnectionName :cognitect.aws.glue/ConnectionName)
(s/def :cognitect.aws.glue.JdbcTarget/Path :cognitect.aws.glue/Path)
(s/def :cognitect.aws.glue.JdbcTarget/Exclusions :cognitect.aws.glue/PathList)
(s/def
:cognitect.aws.glue.JdbcTarget/EnableAdditionalMetadata
:cognitect.aws.glue/EnableAdditionalMetadata)
(s/def :cognitect.aws.glue.TaskRunProperties/TaskType :cognitect.aws.glue/TaskType)
(s/def
:cognitect.aws.glue.TaskRunProperties/ImportLabelsTaskRunProperties
:cognitect.aws.glue/ImportLabelsTaskRunProperties)
(s/def
:cognitect.aws.glue.TaskRunProperties/ExportLabelsTaskRunProperties
:cognitect.aws.glue/ExportLabelsTaskRunProperties)
(s/def
:cognitect.aws.glue.TaskRunProperties/LabelingSetGenerationTaskRunProperties
:cognitect.aws.glue/LabelingSetGenerationTaskRunProperties)
(s/def
:cognitect.aws.glue.TaskRunProperties/FindMatchesTaskRunProperties
:cognitect.aws.glue/FindMatchesTaskRunProperties)
(s/def :cognitect.aws.glue.PostgreSQLCatalogTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.PostgreSQLCatalogTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def
:cognitect.aws.glue.PostgreSQLCatalogTarget/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.PostgreSQLCatalogTarget/Table
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.MySQLCatalogSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.MySQLCatalogSource/Database :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.MySQLCatalogSource/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.StartImportLabelsTaskRunResponse/TaskRunId
:cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.UpdateJobResponse/JobName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateColumnStatisticsForPartitionRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.UpdateColumnStatisticsForPartitionRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateColumnStatisticsForPartitionRequest/TableName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateColumnStatisticsForPartitionRequest/PartitionValues
:cognitect.aws.glue/ValueStringList)
(s/def
:cognitect.aws.glue.UpdateColumnStatisticsForPartitionRequest/ColumnStatisticsList
:cognitect.aws.glue/UpdateColumnStatisticsList)
(s/def :cognitect.aws.glue.Spigot/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.Spigot/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.Spigot/Path :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.Spigot/Topk :cognitect.aws.glue/Topk)
(s/def :cognitect.aws.glue.Spigot/Prob :cognitect.aws.glue/Prob)
(s/def :cognitect.aws.glue.S3Target/Path :cognitect.aws.glue/Path)
(s/def :cognitect.aws.glue.S3Target/Exclusions :cognitect.aws.glue/PathList)
(s/def :cognitect.aws.glue.S3Target/ConnectionName :cognitect.aws.glue/ConnectionName)
(s/def :cognitect.aws.glue.S3Target/SampleSize :cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.S3Target/EventQueueArn :cognitect.aws.glue/EventQueueArn)
(s/def :cognitect.aws.glue.S3Target/DlqEventQueueArn :cognitect.aws.glue/EventQueueArn)
(s/def
:cognitect.aws.glue.DeleteColumnStatisticsForTableRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.DeleteColumnStatisticsForTableRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.DeleteColumnStatisticsForTableRequest/TableName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.DeleteColumnStatisticsForTableRequest/ColumnName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.CreateGrokClassifierRequest/Classification
:cognitect.aws.glue/Classification)
(s/def :cognitect.aws.glue.CreateGrokClassifierRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateGrokClassifierRequest/GrokPattern :cognitect.aws.glue/GrokPattern)
(s/def
:cognitect.aws.glue.CreateGrokClassifierRequest/CustomPatterns
:cognitect.aws.glue/CustomPatterns)
(s/def :cognitect.aws.glue.JsonClassifier/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.JsonClassifier/CreationTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.JsonClassifier/LastUpdated :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.JsonClassifier/Version :cognitect.aws.glue/VersionId)
(s/def :cognitect.aws.glue.JsonClassifier/JsonPath :cognitect.aws.glue/JsonPath)
(s/def :cognitect.aws.glue.DeleteRegistryInput/RegistryId :cognitect.aws.glue/RegistryId)
(s/def :cognitect.aws.glue.ColumnError/ColumnName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.ColumnError/Error :cognitect.aws.glue/ErrorDetail)
(s/def :cognitect.aws.glue.GetBlueprintRunResponse/BlueprintRun :cognitect.aws.glue/BlueprintRun)
(s/def :cognitect.aws.glue.UpdateBlueprintResponse/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetCrawlerMetricsRequest/CrawlerNameList
:cognitect.aws.glue/CrawlerNameList)
(s/def :cognitect.aws.glue.GetCrawlerMetricsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.GetCrawlerMetricsRequest/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.GetDatabaseResponse/Database :cognitect.aws.glue/Database)
(s/def :cognitect.aws.glue.CreateSecurityConfigurationResponse/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.CreateSecurityConfigurationResponse/CreatedTimestamp
:cognitect.aws.glue/TimestampValue)
(s/def
:cognitect.aws.glue.BatchDeleteTableVersionResponse/Errors
:cognitect.aws.glue/TableVersionErrors)
(s/def :cognitect.aws.glue.CreateConnectionRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.CreateConnectionRequest/ConnectionInput
:cognitect.aws.glue/ConnectionInput)
(s/def :cognitect.aws.glue.CreateConnectionRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.CsvClassifier/Header :cognitect.aws.glue/CsvHeader)
(s/def :cognitect.aws.glue.CsvClassifier/LastUpdated :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.CsvClassifier/Delimiter :cognitect.aws.glue/CsvColumnDelimiter)
(s/def :cognitect.aws.glue.CsvClassifier/CustomDatatypes :cognitect.aws.glue/CustomDatatypes)
(s/def
:cognitect.aws.glue.CsvClassifier/CustomDatatypeConfigured
:cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.CsvClassifier/QuoteSymbol :cognitect.aws.glue/CsvQuoteSymbol)
(s/def :cognitect.aws.glue.CsvClassifier/CreationTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.CsvClassifier/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CsvClassifier/DisableValueTrimming :cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.CsvClassifier/Version :cognitect.aws.glue/VersionId)
(s/def :cognitect.aws.glue.CsvClassifier/AllowSingleColumn :cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.CsvClassifier/ContainsHeader :cognitect.aws.glue/CsvHeaderOption)
(s/def :cognitect.aws.glue.BatchGetTriggersResponse/Triggers :cognitect.aws.glue/TriggerList)
(s/def
:cognitect.aws.glue.BatchGetTriggersResponse/TriggersNotFound
:cognitect.aws.glue/TriggerNameList)
(s/def
:cognitect.aws.glue.BatchCreatePartitionRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.BatchCreatePartitionRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.BatchCreatePartitionRequest/TableName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.BatchCreatePartitionRequest/PartitionInputList
:cognitect.aws.glue/PartitionInputList)
(s/def :cognitect.aws.glue.SchemaId/SchemaArn :cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.SchemaId/SchemaName :cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.SchemaId/RegistryName :cognitect.aws.glue/SchemaRegistryNameString)
(s/def
:cognitect.aws.glue.CheckSchemaVersionValidityResponse/Valid
:cognitect.aws.glue/IsVersionValid)
(s/def
:cognitect.aws.glue.CheckSchemaVersionValidityResponse/Error
:cognitect.aws.glue/SchemaValidationError)
(s/def :cognitect.aws.glue.DataQualityRulesetListDetails/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.DataQualityRulesetListDetails/Description
:cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.DataQualityRulesetListDetails/CreatedOn :cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.DataQualityRulesetListDetails/LastModifiedOn
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.DataQualityRulesetListDetails/TargetTable
:cognitect.aws.glue/DataQualityTargetTable)
(s/def
:cognitect.aws.glue.DataQualityRulesetListDetails/RecommendationRunId
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.DataQualityRulesetListDetails/RuleCount
:cognitect.aws.glue/NullableInteger)
(s/def
:cognitect.aws.glue.BatchUpdatePartitionRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.BatchUpdatePartitionRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.BatchUpdatePartitionRequest/TableName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.BatchUpdatePartitionRequest/Entries
:cognitect.aws.glue/BatchUpdatePartitionRequestEntryList)
(s/def :cognitect.aws.glue.CreateTableRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.CreateTableRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateTableRequest/TableInput :cognitect.aws.glue/TableInput)
(s/def
:cognitect.aws.glue.CreateTableRequest/PartitionIndexes
:cognitect.aws.glue/PartitionIndexList)
(s/def :cognitect.aws.glue.CreateTableRequest/TransactionId :cognitect.aws.glue/TransactionIdString)
(s/def
:cognitect.aws.glue.CreateTableRequest/OpenTableFormatInput
:cognitect.aws.glue/OpenTableFormatInput)
(s/def :cognitect.aws.glue.GetCustomEntityTypeResponse/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetCustomEntityTypeResponse/RegexString :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetCustomEntityTypeResponse/ContextWords
:cognitect.aws.glue/ContextWords)
(s/def
:cognitect.aws.glue.BatchUpdatePartitionFailureEntry/PartitionValueList
:cognitect.aws.glue/BoundedPartitionValueList)
(s/def
:cognitect.aws.glue.BatchUpdatePartitionFailureEntry/ErrorDetail
:cognitect.aws.glue/ErrorDetail)
(s/def :cognitect.aws.glue.BatchDeletePartitionResponse/Errors :cognitect.aws.glue/PartitionErrors)
(s/def
:cognitect.aws.glue.GetDataQualityRuleRecommendationRunRequest/RunId
:cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.UpdateDataQualityRulesetResponse/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateDataQualityRulesetResponse/Description
:cognitect.aws.glue/DescriptionString)
(s/def
:cognitect.aws.glue.UpdateDataQualityRulesetResponse/Ruleset
:cognitect.aws.glue/DataQualityRulesetString)
(s/def :cognitect.aws.glue.PropertyPredicate/Key :cognitect.aws.glue/ValueString)
(s/def :cognitect.aws.glue.PropertyPredicate/Value :cognitect.aws.glue/ValueString)
(s/def :cognitect.aws.glue.PropertyPredicate/Comparator :cognitect.aws.glue/Comparator)
(s/def :cognitect.aws.glue.DropDuplicates/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.DropDuplicates/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.DropDuplicates/Columns :cognitect.aws.glue/LimitedPathList)
(s/def :cognitect.aws.glue.StartTriggerResponse/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UpdateTriggerRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UpdateTriggerRequest/TriggerUpdate :cognitect.aws.glue/TriggerUpdate)
(s/def :cognitect.aws.glue.Join/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.Join/Inputs :cognitect.aws.glue/TwoInputs)
(s/def :cognitect.aws.glue.Join/JoinType :cognitect.aws.glue/JoinType)
(s/def :cognitect.aws.glue.Join/Columns :cognitect.aws.glue/JoinColumns)
(s/def :cognitect.aws.glue.GetBlueprintRunsResponse/BlueprintRuns :cognitect.aws.glue/BlueprintRuns)
(s/def :cognitect.aws.glue.GetBlueprintRunsResponse/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.SelectFields/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.SelectFields/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.SelectFields/Paths :cognitect.aws.glue/GlueStudioPathList)
(s/def
:cognitect.aws.glue.CloudWatchEncryption/CloudWatchEncryptionMode
:cognitect.aws.glue/CloudWatchEncryptionMode)
(s/def :cognitect.aws.glue.CloudWatchEncryption/KmsKeyArn :cognitect.aws.glue/KmsKeyArn)
(s/def :cognitect.aws.glue.ListStatementsResponse/Statements :cognitect.aws.glue/StatementList)
(s/def :cognitect.aws.glue.ListStatementsResponse/NextToken :cognitect.aws.glue/OrchestrationToken)
(s/def :cognitect.aws.glue.ApplyMapping/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.ApplyMapping/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.ApplyMapping/Mapping :cognitect.aws.glue/Mappings)
(s/def :cognitect.aws.glue.RelationalCatalogSource/Name :cognitect.aws.glue/NodeName)
(s/def
:cognitect.aws.glue.RelationalCatalogSource/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.RelationalCatalogSource/Table
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.NullValueField/Value :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.NullValueField/Datatype :cognitect.aws.glue/Datatype)
(s/def :cognitect.aws.glue.MappingEntry/SourceTable :cognitect.aws.glue/TableName)
(s/def :cognitect.aws.glue.MappingEntry/SourcePath :cognitect.aws.glue/SchemaPathString)
(s/def :cognitect.aws.glue.MappingEntry/SourceType :cognitect.aws.glue/FieldType)
(s/def :cognitect.aws.glue.MappingEntry/TargetTable :cognitect.aws.glue/TableName)
(s/def :cognitect.aws.glue.MappingEntry/TargetPath :cognitect.aws.glue/SchemaPathString)
(s/def :cognitect.aws.glue.MappingEntry/TargetType :cognitect.aws.glue/FieldType)
(s/def :cognitect.aws.glue.GetDataQualityResultRequest/ResultId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.GetMLTaskRunsRequest/TransformId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.GetMLTaskRunsRequest/NextToken :cognitect.aws.glue/PaginationToken)
(s/def :cognitect.aws.glue.GetMLTaskRunsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.GetMLTaskRunsRequest/Filter :cognitect.aws.glue/TaskRunFilterCriteria)
(s/def :cognitect.aws.glue.GetMLTaskRunsRequest/Sort :cognitect.aws.glue/TaskRunSortCriteria)
(s/def :cognitect.aws.glue.GetSchemaVersionInput/SchemaId :cognitect.aws.glue/SchemaId)
(s/def
:cognitect.aws.glue.GetSchemaVersionInput/SchemaVersionId
:cognitect.aws.glue/SchemaVersionIdString)
(s/def
:cognitect.aws.glue.GetSchemaVersionInput/SchemaVersionNumber
:cognitect.aws.glue/SchemaVersionNumber)
(s/def
:cognitect.aws.glue.DataQualityRuleRecommendationRunFilter/DataSource
:cognitect.aws.glue/DataSource)
(s/def
:cognitect.aws.glue.DataQualityRuleRecommendationRunFilter/StartedBefore
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.DataQualityRuleRecommendationRunFilter/StartedAfter
:cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.Union/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.Union/Inputs :cognitect.aws.glue/TwoInputs)
(s/def :cognitect.aws.glue.Union/UnionType :cognitect.aws.glue/UnionType)
(s/def
:cognitect.aws.glue.GetColumnStatisticsForPartitionRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.GetColumnStatisticsForPartitionRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetColumnStatisticsForPartitionRequest/TableName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetColumnStatisticsForPartitionRequest/PartitionValues
:cognitect.aws.glue/ValueStringList)
(s/def
:cognitect.aws.glue.GetColumnStatisticsForPartitionRequest/ColumnNames
:cognitect.aws.glue/GetColumnNamesList)
(s/def
:cognitect.aws.glue.DataQualityRuleRecommendationRunDescription/RunId
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.DataQualityRuleRecommendationRunDescription/Status
:cognitect.aws.glue/TaskStatusType)
(s/def
:cognitect.aws.glue.DataQualityRuleRecommendationRunDescription/StartedOn
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.DataQualityRuleRecommendationRunDescription/DataSource
:cognitect.aws.glue/DataSource)
(s/def
:cognitect.aws.glue.RegistryListItem/RegistryName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.RegistryListItem/RegistryArn :cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.RegistryListItem/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.RegistryListItem/Status :cognitect.aws.glue/RegistryStatus)
(s/def :cognitect.aws.glue.RegistryListItem/CreatedTime :cognitect.aws.glue/CreatedTimestamp)
(s/def :cognitect.aws.glue.RegistryListItem/UpdatedTime :cognitect.aws.glue/UpdatedTimestamp)
(s/def
:cognitect.aws.glue.ListDataQualityRuleRecommendationRunsRequest/Filter
:cognitect.aws.glue/DataQualityRuleRecommendationRunFilter)
(s/def
:cognitect.aws.glue.ListDataQualityRuleRecommendationRunsRequest/NextToken
:cognitect.aws.glue/PaginationToken)
(s/def
:cognitect.aws.glue.ListDataQualityRuleRecommendationRunsRequest/MaxResults
:cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.CrawlerMetrics/CrawlerName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CrawlerMetrics/TimeLeftSeconds :cognitect.aws.glue/NonNegativeDouble)
(s/def :cognitect.aws.glue.CrawlerMetrics/StillEstimating :cognitect.aws.glue/Boolean)
(s/def :cognitect.aws.glue.CrawlerMetrics/LastRuntimeSeconds :cognitect.aws.glue/NonNegativeDouble)
(s/def
:cognitect.aws.glue.CrawlerMetrics/MedianRuntimeSeconds
:cognitect.aws.glue/NonNegativeDouble)
(s/def :cognitect.aws.glue.CrawlerMetrics/TablesCreated :cognitect.aws.glue/NonNegativeInteger)
(s/def :cognitect.aws.glue.CrawlerMetrics/TablesUpdated :cognitect.aws.glue/NonNegativeInteger)
(s/def :cognitect.aws.glue.CrawlerMetrics/TablesDeleted :cognitect.aws.glue/NonNegativeInteger)
(s/def :cognitect.aws.glue.Segment/SegmentNumber :cognitect.aws.glue/NonNegativeInteger)
(s/def :cognitect.aws.glue.Segment/TotalSegments :cognitect.aws.glue/TotalSegmentsInteger)
(s/def :cognitect.aws.glue.ListStatementsRequest/SessionId :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.ListStatementsRequest/RequestOrigin
:cognitect.aws.glue/OrchestrationNameString)
(s/def :cognitect.aws.glue.ListStatementsRequest/NextToken :cognitect.aws.glue/OrchestrationToken)
(s/def :cognitect.aws.glue.GetPartitionResponse/Partition :cognitect.aws.glue/Partition)
(s/def :cognitect.aws.glue.UpdateWorkflowRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UpdateWorkflowRequest/Description :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.UpdateWorkflowRequest/DefaultRunProperties
:cognitect.aws.glue/WorkflowRunProperties)
(s/def
:cognitect.aws.glue.UpdateWorkflowRequest/MaxConcurrentRuns
:cognitect.aws.glue/NullableInteger)
(s/def
:cognitect.aws.glue.BatchGetBlueprintsRequest/Names
:cognitect.aws.glue/BatchGetBlueprintNames)
(s/def
:cognitect.aws.glue.BatchGetBlueprintsRequest/IncludeBlueprint
:cognitect.aws.glue/NullableBoolean)
(s/def
:cognitect.aws.glue.BatchGetBlueprintsRequest/IncludeParameterSpec
:cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.PutWorkflowRunPropertiesRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.PutWorkflowRunPropertiesRequest/RunId :cognitect.aws.glue/IdString)
(s/def
:cognitect.aws.glue.PutWorkflowRunPropertiesRequest/RunProperties
:cognitect.aws.glue/WorkflowRunProperties)
(s/def :cognitect.aws.glue.DynamicTransform/Name :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.DynamicTransform/TransformName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.DynamicTransform/Inputs :cognitect.aws.glue/OneInput)
(s/def
:cognitect.aws.glue.DynamicTransform/Parameters
:cognitect.aws.glue/TransformConfigParameterList)
(s/def
:cognitect.aws.glue.DynamicTransform/FunctionName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.DynamicTransform/Path :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.DynamicTransform/Version :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.DynamicTransform/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def :cognitect.aws.glue.UpdatePartitionRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.UpdatePartitionRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UpdatePartitionRequest/TableName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdatePartitionRequest/PartitionValueList
:cognitect.aws.glue/BoundedPartitionValueList)
(s/def :cognitect.aws.glue.UpdatePartitionRequest/PartitionInput :cognitect.aws.glue/PartitionInput)
(s/def :cognitect.aws.glue.DeletePartitionRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.DeletePartitionRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DeletePartitionRequest/TableName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.DeletePartitionRequest/PartitionValues
:cognitect.aws.glue/ValueStringList)
(s/def :cognitect.aws.glue.Action/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Action/Arguments :cognitect.aws.glue/GenericMap)
(s/def :cognitect.aws.glue.Action/Timeout :cognitect.aws.glue/Timeout)
(s/def :cognitect.aws.glue.Action/SecurityConfiguration :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Action/NotificationProperty :cognitect.aws.glue/NotificationProperty)
(s/def :cognitect.aws.glue.Action/CrawlerName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetUserDefinedFunctionsRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.GetUserDefinedFunctionsRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetUserDefinedFunctionsRequest/Pattern :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetUserDefinedFunctionsRequest/NextToken :cognitect.aws.glue/Token)
(s/def
:cognitect.aws.glue.GetUserDefinedFunctionsRequest/MaxResults
:cognitect.aws.glue/CatalogGetterPageSize)
(s/def
:cognitect.aws.glue.EncryptionConfiguration/S3Encryption
:cognitect.aws.glue/S3EncryptionList)
(s/def
:cognitect.aws.glue.EncryptionConfiguration/CloudWatchEncryption
:cognitect.aws.glue/CloudWatchEncryption)
(s/def
:cognitect.aws.glue.EncryptionConfiguration/JobBookmarksEncryption
:cognitect.aws.glue/JobBookmarksEncryption)
(s/def
:cognitect.aws.glue.ListDevEndpointsResponse/DevEndpointNames
:cognitect.aws.glue/DevEndpointNameList)
(s/def :cognitect.aws.glue.ListDevEndpointsResponse/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.DeleteCustomEntityTypeResponse/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetBlueprintRunsRequest/BlueprintName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetBlueprintRunsRequest/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.GetBlueprintRunsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def
:cognitect.aws.glue.ListRegistriesResponse/Registries
:cognitect.aws.glue/RegistryListDefinition)
(s/def
:cognitect.aws.glue.ListRegistriesResponse/NextToken
:cognitect.aws.glue/SchemaRegistryTokenString)
(s/def
:cognitect.aws.glue.BatchGetCustomEntityTypesResponse/CustomEntityTypes
:cognitect.aws.glue/CustomEntityTypes)
(s/def
:cognitect.aws.glue.BatchGetCustomEntityTypesResponse/CustomEntityTypesNotFound
:cognitect.aws.glue/CustomEntityTypeNames)
(s/def :cognitect.aws.glue.PartitionInput/Values :cognitect.aws.glue/ValueStringList)
(s/def :cognitect.aws.glue.PartitionInput/LastAccessTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.PartitionInput/StorageDescriptor :cognitect.aws.glue/StorageDescriptor)
(s/def :cognitect.aws.glue.PartitionInput/Parameters :cognitect.aws.glue/ParametersMap)
(s/def :cognitect.aws.glue.PartitionInput/LastAnalyzedTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.CreateBlueprintResponse/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateJsonClassifierRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateJsonClassifierRequest/JsonPath :cognitect.aws.glue/JsonPath)
(s/def :cognitect.aws.glue.DeleteBlueprintResponse/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetConnectionResponse/Connection :cognitect.aws.glue/Connection)
(s/def :cognitect.aws.glue.PrincipalPermissions/Principal :cognitect.aws.glue/DataLakePrincipal)
(s/def :cognitect.aws.glue.PrincipalPermissions/Permissions :cognitect.aws.glue/PermissionList)
(s/def
:cognitect.aws.glue.ExecutionProperty/MaxConcurrentRuns
:cognitect.aws.glue/MaxConcurrentRuns)
(s/def
:cognitect.aws.glue.UpdateClassifierRequest/GrokClassifier
:cognitect.aws.glue/UpdateGrokClassifierRequest)
(s/def
:cognitect.aws.glue.UpdateClassifierRequest/XMLClassifier
:cognitect.aws.glue/UpdateXMLClassifierRequest)
(s/def
:cognitect.aws.glue.UpdateClassifierRequest/JsonClassifier
:cognitect.aws.glue/UpdateJsonClassifierRequest)
(s/def
:cognitect.aws.glue.UpdateClassifierRequest/CsvClassifier
:cognitect.aws.glue/UpdateCsvClassifierRequest)
(s/def :cognitect.aws.glue.AggregateOperation/Column :cognitect.aws.glue/EnclosedInStringProperties)
(s/def :cognitect.aws.glue.AggregateOperation/AggFunc :cognitect.aws.glue/AggFunction)
(s/def
:cognitect.aws.glue.MLUserDataEncryption/MlUserDataEncryptionMode
:cognitect.aws.glue/MLUserDataEncryptionModeString)
(s/def :cognitect.aws.glue.MLUserDataEncryption/KmsKeyId :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.RedshiftSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.RedshiftSource/Database :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.RedshiftSource/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.RedshiftSource/RedshiftTmpDir
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.RedshiftSource/TmpDirIAMRole
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.Column/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Column/Type :cognitect.aws.glue/ColumnTypeString)
(s/def :cognitect.aws.glue.Column/Comment :cognitect.aws.glue/CommentString)
(s/def :cognitect.aws.glue.Column/Parameters :cognitect.aws.glue/ParametersMap)
(s/def
:cognitect.aws.glue.S3ParquetSource/CompressionType
:cognitect.aws.glue/ParquetCompressionType)
(s/def :cognitect.aws.glue.S3ParquetSource/Recurse :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.S3ParquetSource/Paths :cognitect.aws.glue/EnclosedInStringProperties)
(s/def
:cognitect.aws.glue.S3ParquetSource/AdditionalOptions
:cognitect.aws.glue/S3DirectSourceAdditionalOptions)
(s/def :cognitect.aws.glue.S3ParquetSource/GroupSize :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.S3ParquetSource/GroupFiles :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.S3ParquetSource/MaxBand :cognitect.aws.glue/BoxedNonNegativeInt)
(s/def :cognitect.aws.glue.S3ParquetSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.S3ParquetSource/MaxFilesInBand :cognitect.aws.glue/BoxedNonNegativeInt)
(s/def
:cognitect.aws.glue.S3ParquetSource/Exclusions
:cognitect.aws.glue/EnclosedInStringProperties)
(s/def :cognitect.aws.glue.S3ParquetSource/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def
:cognitect.aws.glue.GetSecurityConfigurationsResponse/SecurityConfigurations
:cognitect.aws.glue/SecurityConfigurationList)
(s/def
:cognitect.aws.glue.GetSecurityConfigurationsResponse/NextToken
:cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.PIIDetection/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.PIIDetection/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.PIIDetection/PiiType :cognitect.aws.glue/PiiType)
(s/def
:cognitect.aws.glue.PIIDetection/EntityTypesToDetect
:cognitect.aws.glue/EnclosedInStringProperties)
(s/def
:cognitect.aws.glue.PIIDetection/OutputColumnName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.PIIDetection/SampleFraction :cognitect.aws.glue/BoxedDoubleFraction)
(s/def :cognitect.aws.glue.PIIDetection/ThresholdFraction :cognitect.aws.glue/BoxedDoubleFraction)
(s/def :cognitect.aws.glue.PIIDetection/MaskValue :cognitect.aws.glue/MaskValue)
(s/def :cognitect.aws.glue.DatabaseInput/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DatabaseInput/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.DatabaseInput/LocationUri :cognitect.aws.glue/URI)
(s/def :cognitect.aws.glue.DatabaseInput/Parameters :cognitect.aws.glue/ParametersMap)
(s/def
:cognitect.aws.glue.DatabaseInput/CreateTableDefaultPermissions
:cognitect.aws.glue/PrincipalPermissionsList)
(s/def :cognitect.aws.glue.DatabaseInput/TargetDatabase :cognitect.aws.glue/DatabaseIdentifier)
(s/def :cognitect.aws.glue.DatabaseInput/FederatedDatabase :cognitect.aws.glue/FederatedDatabase)
(s/def
:cognitect.aws.glue.JDBCConnectorOptions/FilterPredicate
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.JDBCConnectorOptions/PartitionColumn
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.JDBCConnectorOptions/LowerBound :cognitect.aws.glue/BoxedNonNegativeLong)
(s/def :cognitect.aws.glue.JDBCConnectorOptions/UpperBound :cognitect.aws.glue/BoxedNonNegativeLong)
(s/def
:cognitect.aws.glue.JDBCConnectorOptions/NumPartitions
:cognitect.aws.glue/BoxedNonNegativeLong)
(s/def
:cognitect.aws.glue.JDBCConnectorOptions/JobBookmarkKeys
:cognitect.aws.glue/EnclosedInStringProperties)
(s/def
:cognitect.aws.glue.JDBCConnectorOptions/JobBookmarkKeysSortOrder
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.JDBCConnectorOptions/DataTypeMapping
:cognitect.aws.glue/JDBCDataTypeMapping)
(s/def
:cognitect.aws.glue.UpsertRedshiftTargetOptions/TableLocation
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.UpsertRedshiftTargetOptions/ConnectionName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.UpsertRedshiftTargetOptions/UpsertKeys
:cognitect.aws.glue/EnclosedInStringPropertiesMinOne)
(s/def :cognitect.aws.glue.SelectFromCollection/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.SelectFromCollection/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.SelectFromCollection/Index :cognitect.aws.glue/NonNegativeInt)
(s/def :cognitect.aws.glue.S3CatalogTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.S3CatalogTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.S3CatalogTarget/PartitionKeys :cognitect.aws.glue/GlueStudioPathList)
(s/def :cognitect.aws.glue.S3CatalogTarget/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.S3CatalogTarget/Database :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.S3CatalogTarget/SchemaChangePolicy
:cognitect.aws.glue/CatalogSchemaChangePolicy)
(s/def :cognitect.aws.glue.BatchGetPartitionResponse/Partitions :cognitect.aws.glue/PartitionList)
(s/def
:cognitect.aws.glue.BatchGetPartitionResponse/UnprocessedKeys
:cognitect.aws.glue/BatchGetPartitionValueList)
(s/def :cognitect.aws.glue.OracleSQLCatalogTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.OracleSQLCatalogTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def
:cognitect.aws.glue.OracleSQLCatalogTarget/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.OracleSQLCatalogTarget/Table
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.GetWorkflowRunPropertiesResponse/RunProperties
:cognitect.aws.glue/WorkflowRunProperties)
(s/def :cognitect.aws.glue.ImportCatalogToGlueRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.StartWorkflowRunResponse/RunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.Merge/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.Merge/Inputs :cognitect.aws.glue/TwoInputs)
(s/def :cognitect.aws.glue.Merge/Source :cognitect.aws.glue/NodeId)
(s/def :cognitect.aws.glue.Merge/PrimaryKeys :cognitect.aws.glue/GlueStudioPathList)
(s/def :cognitect.aws.glue.DeleteSchemaResponse/SchemaArn :cognitect.aws.glue/GlueResourceArn)
(s/def
:cognitect.aws.glue.DeleteSchemaResponse/SchemaName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.DeleteSchemaResponse/Status :cognitect.aws.glue/SchemaStatus)
(s/def :cognitect.aws.glue.S3JsonSource/Multiline :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.S3JsonSource/CompressionType :cognitect.aws.glue/CompressionType)
(s/def :cognitect.aws.glue.S3JsonSource/Recurse :cognitect.aws.glue/BoxedBoolean)
(s/def :cognitect.aws.glue.S3JsonSource/Paths :cognitect.aws.glue/EnclosedInStringProperties)
(s/def
:cognitect.aws.glue.S3JsonSource/AdditionalOptions
:cognitect.aws.glue/S3DirectSourceAdditionalOptions)
(s/def :cognitect.aws.glue.S3JsonSource/GroupSize :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.S3JsonSource/GroupFiles :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.S3JsonSource/MaxBand :cognitect.aws.glue/BoxedNonNegativeInt)
(s/def :cognitect.aws.glue.S3JsonSource/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.S3JsonSource/MaxFilesInBand :cognitect.aws.glue/BoxedNonNegativeInt)
(s/def :cognitect.aws.glue.S3JsonSource/JsonPath :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.S3JsonSource/Exclusions :cognitect.aws.glue/EnclosedInStringProperties)
(s/def :cognitect.aws.glue.S3JsonSource/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def :cognitect.aws.glue.GetSessionResponse/Session :cognitect.aws.glue/Session)
(s/def :cognitect.aws.glue.S3DeltaCatalogTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.S3DeltaCatalogTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def
:cognitect.aws.glue.S3DeltaCatalogTarget/PartitionKeys
:cognitect.aws.glue/GlueStudioPathList)
(s/def :cognitect.aws.glue.S3DeltaCatalogTarget/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.S3DeltaCatalogTarget/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.S3DeltaCatalogTarget/AdditionalOptions
:cognitect.aws.glue/AdditionalOptions)
(s/def
:cognitect.aws.glue.S3DeltaCatalogTarget/SchemaChangePolicy
:cognitect.aws.glue/CatalogSchemaChangePolicy)
(s/def :cognitect.aws.glue.GetDevEndpointsResponse/DevEndpoints :cognitect.aws.glue/DevEndpointList)
(s/def :cognitect.aws.glue.GetDevEndpointsResponse/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.GetDevEndpointsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.GetDevEndpointsRequest/NextToken :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.GetSchemaByDefinitionResponse/SchemaVersionId
:cognitect.aws.glue/SchemaVersionIdString)
(s/def
:cognitect.aws.glue.GetSchemaByDefinitionResponse/SchemaArn
:cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.GetSchemaByDefinitionResponse/DataFormat :cognitect.aws.glue/DataFormat)
(s/def
:cognitect.aws.glue.GetSchemaByDefinitionResponse/Status
:cognitect.aws.glue/SchemaVersionStatus)
(s/def
:cognitect.aws.glue.GetSchemaByDefinitionResponse/CreatedTime
:cognitect.aws.glue/CreatedTimestamp)
(s/def :cognitect.aws.glue.DeleteTableVersionRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.DeleteTableVersionRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DeleteTableVersionRequest/TableName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DeleteTableVersionRequest/VersionId :cognitect.aws.glue/VersionString)
(s/def :cognitect.aws.glue.UntagResourceRequest/ResourceArn :cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.UntagResourceRequest/TagsToRemove :cognitect.aws.glue/TagKeysList)
(s/def
:cognitect.aws.glue.BinaryColumnStatisticsData/MaximumLength
:cognitect.aws.glue/NonNegativeLong)
(s/def
:cognitect.aws.glue.BinaryColumnStatisticsData/AverageLength
:cognitect.aws.glue/NonNegativeDouble)
(s/def
:cognitect.aws.glue.BinaryColumnStatisticsData/NumberOfNulls
:cognitect.aws.glue/NonNegativeLong)
(s/def :cognitect.aws.glue.GetTriggersRequest/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.GetTriggersRequest/DependentJobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetTriggersRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.PartitionValueList/Values :cognitect.aws.glue/ValueStringList)
(s/def :cognitect.aws.glue.GetJobResponse/Job :cognitect.aws.glue/Job)
(s/def :cognitect.aws.glue.ListSessionsResponse/Ids :cognitect.aws.glue/SessionIdList)
(s/def :cognitect.aws.glue.ListSessionsResponse/Sessions :cognitect.aws.glue/SessionList)
(s/def :cognitect.aws.glue.ListSessionsResponse/NextToken :cognitect.aws.glue/OrchestrationToken)
(s/def
:cognitect.aws.glue.GetSchemaVersionsDiffResponse/Diff
:cognitect.aws.glue/SchemaDefinitionDiff)
(s/def :cognitect.aws.glue.PartitionIndexDescriptor/IndexName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.PartitionIndexDescriptor/Keys :cognitect.aws.glue/KeySchemaElementList)
(s/def
:cognitect.aws.glue.PartitionIndexDescriptor/IndexStatus
:cognitect.aws.glue/PartitionIndexStatus)
(s/def
:cognitect.aws.glue.PartitionIndexDescriptor/BackfillErrors
:cognitect.aws.glue/BackfillErrors)
(s/def
:cognitect.aws.glue.BooleanColumnStatisticsData/NumberOfTrues
:cognitect.aws.glue/NonNegativeLong)
(s/def
:cognitect.aws.glue.BooleanColumnStatisticsData/NumberOfFalses
:cognitect.aws.glue/NonNegativeLong)
(s/def
:cognitect.aws.glue.BooleanColumnStatisticsData/NumberOfNulls
:cognitect.aws.glue/NonNegativeLong)
(s/def :cognitect.aws.glue.CodeGenEdge/Source :cognitect.aws.glue/CodeGenIdentifier)
(s/def :cognitect.aws.glue.CodeGenEdge/Target :cognitect.aws.glue/CodeGenIdentifier)
(s/def :cognitect.aws.glue.CodeGenEdge/TargetParameter :cognitect.aws.glue/CodeGenArgName)
(s/def :cognitect.aws.glue.Node/Type :cognitect.aws.glue/NodeType)
(s/def :cognitect.aws.glue.Node/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Node/UniqueId :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Node/TriggerDetails :cognitect.aws.glue/TriggerNodeDetails)
(s/def :cognitect.aws.glue.Node/JobDetails :cognitect.aws.glue/JobNodeDetails)
(s/def :cognitect.aws.glue.Node/CrawlerDetails :cognitect.aws.glue/CrawlerNodeDetails)
(s/def :cognitect.aws.glue.ListTriggersResponse/TriggerNames :cognitect.aws.glue/TriggerNameList)
(s/def :cognitect.aws.glue.ListTriggersResponse/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.CreateTriggerResponse/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.DQStopJobOnFailureOptions/StopJobOnFailureTiming
:cognitect.aws.glue/DQStopJobOnFailureTiming)
(s/def :cognitect.aws.glue.S3CatalogDeltaSource/Name :cognitect.aws.glue/NodeName)
(s/def
:cognitect.aws.glue.S3CatalogDeltaSource/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.S3CatalogDeltaSource/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.S3CatalogDeltaSource/AdditionalDeltaOptions
:cognitect.aws.glue/AdditionalOptions)
(s/def :cognitect.aws.glue.S3CatalogDeltaSource/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def :cognitect.aws.glue.GetSecurityConfigurationRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.DataQualityEvaluationRunAdditionalRunOptions/CloudWatchMetricsEnabled
:cognitect.aws.glue/NullableBoolean)
(s/def
:cognitect.aws.glue.DataQualityEvaluationRunAdditionalRunOptions/ResultsS3Prefix
:cognitect.aws.glue/UriString)
(s/def :cognitect.aws.glue.GetDatabaseRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.GetDatabaseRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.StartMLLabelingSetGenerationTaskRunResponse/TaskRunId
:cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.GetRegistryInput/RegistryId :cognitect.aws.glue/RegistryId)
(s/def :cognitect.aws.glue.UpdateSchemaResponse/SchemaArn :cognitect.aws.glue/GlueResourceArn)
(s/def
:cognitect.aws.glue.UpdateSchemaResponse/SchemaName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def
:cognitect.aws.glue.UpdateSchemaResponse/RegistryName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.LongColumnStatisticsData/MinimumValue :cognitect.aws.glue/Long)
(s/def :cognitect.aws.glue.LongColumnStatisticsData/MaximumValue :cognitect.aws.glue/Long)
(s/def
:cognitect.aws.glue.LongColumnStatisticsData/NumberOfNulls
:cognitect.aws.glue/NonNegativeLong)
(s/def
:cognitect.aws.glue.LongColumnStatisticsData/NumberOfDistinctValues
:cognitect.aws.glue/NonNegativeLong)
(s/def :cognitect.aws.glue.GetJobRunRequest/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetJobRunRequest/RunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.GetJobRunRequest/PredecessorsIncluded :cognitect.aws.glue/BooleanValue)
(s/def :cognitect.aws.glue.GetTableVersionRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.GetTableVersionRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetTableVersionRequest/TableName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetTableVersionRequest/VersionId :cognitect.aws.glue/VersionString)
(s/def :cognitect.aws.glue.UpdateBlueprintRequest/Name :cognitect.aws.glue/OrchestrationNameString)
(s/def
:cognitect.aws.glue.UpdateBlueprintRequest/Description
:cognitect.aws.glue/Generic512CharString)
(s/def
:cognitect.aws.glue.UpdateBlueprintRequest/BlueprintLocation
:cognitect.aws.glue/OrchestrationS3Location)
(s/def
:cognitect.aws.glue.UpdateCrawlerRequest/LineageConfiguration
:cognitect.aws.glue/LineageConfiguration)
(s/def
:cognitect.aws.glue.UpdateCrawlerRequest/CrawlerSecurityConfiguration
:cognitect.aws.glue/CrawlerSecurityConfiguration)
(s/def
:cognitect.aws.glue.UpdateCrawlerRequest/SchemaChangePolicy
:cognitect.aws.glue/SchemaChangePolicy)
(s/def :cognitect.aws.glue.UpdateCrawlerRequest/Schedule :cognitect.aws.glue/CronExpression)
(s/def :cognitect.aws.glue.UpdateCrawlerRequest/Targets :cognitect.aws.glue/CrawlerTargets)
(s/def :cognitect.aws.glue.UpdateCrawlerRequest/Classifiers :cognitect.aws.glue/ClassifierNameList)
(s/def :cognitect.aws.glue.UpdateCrawlerRequest/TablePrefix :cognitect.aws.glue/TablePrefix)
(s/def :cognitect.aws.glue.UpdateCrawlerRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateCrawlerRequest/Description
:cognitect.aws.glue/DescriptionStringRemovable)
(s/def :cognitect.aws.glue.UpdateCrawlerRequest/Role :cognitect.aws.glue/Role)
(s/def
:cognitect.aws.glue.UpdateCrawlerRequest/Configuration
:cognitect.aws.glue/CrawlerConfiguration)
(s/def
:cognitect.aws.glue.UpdateCrawlerRequest/LakeFormationConfiguration
:cognitect.aws.glue/LakeFormationConfiguration)
(s/def :cognitect.aws.glue.UpdateCrawlerRequest/RecrawlPolicy :cognitect.aws.glue/RecrawlPolicy)
(s/def :cognitect.aws.glue.UpdateCrawlerRequest/DatabaseName :cognitect.aws.glue/DatabaseName)
(s/def :cognitect.aws.glue.SortCriterion/FieldName :cognitect.aws.glue/ValueString)
(s/def :cognitect.aws.glue.SortCriterion/Sort :cognitect.aws.glue/Sort)
(s/def
:cognitect.aws.glue.ListDataQualityRulesetEvaluationRunsRequest/Filter
:cognitect.aws.glue/DataQualityRulesetEvaluationRunFilter)
(s/def
:cognitect.aws.glue.ListDataQualityRulesetEvaluationRunsRequest/NextToken
:cognitect.aws.glue/PaginationToken)
(s/def
:cognitect.aws.glue.ListDataQualityRulesetEvaluationRunsRequest/MaxResults
:cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.FederatedDatabase/Identifier :cognitect.aws.glue/FederationIdentifier)
(s/def :cognitect.aws.glue.FederatedDatabase/ConnectionName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DeleteJobResponse/JobName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.ListDataQualityResultsRequest/Filter
:cognitect.aws.glue/DataQualityResultFilterCriteria)
(s/def
:cognitect.aws.glue.ListDataQualityResultsRequest/NextToken
:cognitect.aws.glue/PaginationToken)
(s/def :cognitect.aws.glue.ListDataQualityResultsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.DataQualityResult/RulesetEvaluationRunId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.DataQualityResult/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DataQualityResult/ResultId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.DataQualityResult/Score :cognitect.aws.glue/GenericBoundedDouble)
(s/def :cognitect.aws.glue.DataQualityResult/CompletedOn :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.DataQualityResult/StartedOn :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.DataQualityResult/EvaluationContext :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.DataQualityResult/RulesetName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DataQualityResult/RuleResults :cognitect.aws.glue/DataQualityRuleResults)
(s/def :cognitect.aws.glue.DataQualityResult/DataSource :cognitect.aws.glue/DataSource)
(s/def :cognitect.aws.glue.DataQualityResult/JobRunId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.JDBCConnectorSource/Name :cognitect.aws.glue/NodeName)
(s/def
:cognitect.aws.glue.JDBCConnectorSource/ConnectionName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.JDBCConnectorSource/ConnectorName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.JDBCConnectorSource/ConnectionType
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.JDBCConnectorSource/AdditionalOptions
:cognitect.aws.glue/JDBCConnectorOptions)
(s/def
:cognitect.aws.glue.JDBCConnectorSource/ConnectionTable
:cognitect.aws.glue/EnclosedInStringPropertyWithQuote)
(s/def :cognitect.aws.glue.JDBCConnectorSource/Query :cognitect.aws.glue/SqlQuery)
(s/def :cognitect.aws.glue.JDBCConnectorSource/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def :cognitect.aws.glue.GetSchemaVersionsDiffInput/SchemaId :cognitect.aws.glue/SchemaId)
(s/def
:cognitect.aws.glue.GetSchemaVersionsDiffInput/FirstSchemaVersionNumber
:cognitect.aws.glue/SchemaVersionNumber)
(s/def
:cognitect.aws.glue.GetSchemaVersionsDiffInput/SecondSchemaVersionNumber
:cognitect.aws.glue/SchemaVersionNumber)
(s/def
:cognitect.aws.glue.GetSchemaVersionsDiffInput/SchemaDiffType
:cognitect.aws.glue/SchemaDiffType)
(s/def :cognitect.aws.glue.SessionCommand/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.SessionCommand/PythonVersion :cognitect.aws.glue/PythonVersionString)
(s/def :cognitect.aws.glue.ListSchemasInput/RegistryId :cognitect.aws.glue/RegistryId)
(s/def :cognitect.aws.glue.ListSchemasInput/MaxResults :cognitect.aws.glue/MaxResultsNumber)
(s/def :cognitect.aws.glue.ListSchemasInput/NextToken :cognitect.aws.glue/SchemaRegistryTokenString)
(s/def :cognitect.aws.glue.CreateSessionRequest/Timeout :cognitect.aws.glue/Timeout)
(s/def :cognitect.aws.glue.CreateSessionRequest/NumberOfWorkers :cognitect.aws.glue/NullableInteger)
(s/def
:cognitect.aws.glue.CreateSessionRequest/SecurityConfiguration
:cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateSessionRequest/Id :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateSessionRequest/MaxCapacity :cognitect.aws.glue/NullableDouble)
(s/def :cognitect.aws.glue.CreateSessionRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def
:cognitect.aws.glue.CreateSessionRequest/RequestOrigin
:cognitect.aws.glue/OrchestrationNameString)
(s/def :cognitect.aws.glue.CreateSessionRequest/WorkerType :cognitect.aws.glue/WorkerType)
(s/def
:cognitect.aws.glue.CreateSessionRequest/DefaultArguments
:cognitect.aws.glue/OrchestrationArgumentsMap)
(s/def :cognitect.aws.glue.CreateSessionRequest/Command :cognitect.aws.glue/SessionCommand)
(s/def :cognitect.aws.glue.CreateSessionRequest/IdleTimeout :cognitect.aws.glue/Timeout)
(s/def :cognitect.aws.glue.CreateSessionRequest/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.CreateSessionRequest/GlueVersion :cognitect.aws.glue/GlueVersionString)
(s/def :cognitect.aws.glue.CreateSessionRequest/Connections :cognitect.aws.glue/ConnectionsList)
(s/def :cognitect.aws.glue.CreateSessionRequest/Role :cognitect.aws.glue/OrchestrationRoleArn)
(s/def
:cognitect.aws.glue.StartExportLabelsTaskRunRequest/TransformId
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.StartExportLabelsTaskRunRequest/OutputS3Path
:cognitect.aws.glue/UriString)
(s/def :cognitect.aws.glue.GrokClassifier/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GrokClassifier/Classification :cognitect.aws.glue/Classification)
(s/def :cognitect.aws.glue.GrokClassifier/CreationTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.GrokClassifier/LastUpdated :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.GrokClassifier/Version :cognitect.aws.glue/VersionId)
(s/def :cognitect.aws.glue.GrokClassifier/GrokPattern :cognitect.aws.glue/GrokPattern)
(s/def :cognitect.aws.glue.GrokClassifier/CustomPatterns :cognitect.aws.glue/CustomPatterns)
(s/def :cognitect.aws.glue.GetTablesResponse/TableList :cognitect.aws.glue/TableList)
(s/def :cognitect.aws.glue.GetTablesResponse/NextToken :cognitect.aws.glue/Token)
(s/def
:cognitect.aws.glue.OtherMetadataValueListItem/MetadataValue
:cognitect.aws.glue/MetadataValueString)
(s/def
:cognitect.aws.glue.OtherMetadataValueListItem/CreatedTime
:cognitect.aws.glue/CreatedTimestamp)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/Classification
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/AvoidEmptyBatches
:cognitect.aws.glue/BoxedBoolean)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/MaxFetchTimeInMs
:cognitect.aws.glue/BoxedNonNegativeLong)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/MaxFetchRecordsPerShard
:cognitect.aws.glue/BoxedNonNegativeLong)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/IdleTimeBetweenReadsInMs
:cognitect.aws.glue/BoxedNonNegativeLong)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/RoleSessionName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/StartingTimestamp
:cognitect.aws.glue/Iso8601DateTime)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/NumRetries
:cognitect.aws.glue/BoxedNonNegativeInt)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/AddIdleTimeBetweenReads
:cognitect.aws.glue/BoxedBoolean)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/Delimiter
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/MaxRecordPerRead
:cognitect.aws.glue/BoxedNonNegativeLong)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/StreamName
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/MaxRetryIntervalMs
:cognitect.aws.glue/BoxedNonNegativeLong)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/RoleArn
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/StreamArn
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/DescribeShardInterval
:cognitect.aws.glue/BoxedNonNegativeLong)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/AddRecordTimestamp
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/StartingPosition
:cognitect.aws.glue/StartingPosition)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/EmitConsumerLagMetrics
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/RetryIntervalMs
:cognitect.aws.glue/BoxedNonNegativeLong)
(s/def
:cognitect.aws.glue.KinesisStreamingSourceOptions/EndpointUrl
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.GetSchemaVersionResponse/SchemaVersionId
:cognitect.aws.glue/SchemaVersionIdString)
(s/def
:cognitect.aws.glue.GetSchemaVersionResponse/SchemaDefinition
:cognitect.aws.glue/SchemaDefinitionString)
(s/def :cognitect.aws.glue.GetSchemaVersionResponse/DataFormat :cognitect.aws.glue/DataFormat)
(s/def :cognitect.aws.glue.GetSchemaVersionResponse/SchemaArn :cognitect.aws.glue/GlueResourceArn)
(s/def
:cognitect.aws.glue.GetSchemaVersionResponse/VersionNumber
:cognitect.aws.glue/VersionLongNumber)
(s/def :cognitect.aws.glue.GetSchemaVersionResponse/Status :cognitect.aws.glue/SchemaVersionStatus)
(s/def
:cognitect.aws.glue.GetSchemaVersionResponse/CreatedTime
:cognitect.aws.glue/CreatedTimestamp)
(s/def :cognitect.aws.glue.GetSchemaByDefinitionInput/SchemaId :cognitect.aws.glue/SchemaId)
(s/def
:cognitect.aws.glue.GetSchemaByDefinitionInput/SchemaDefinition
:cognitect.aws.glue/SchemaDefinitionString)
(s/def :cognitect.aws.glue.Crawler/LineageConfiguration :cognitect.aws.glue/LineageConfiguration)
(s/def
:cognitect.aws.glue.Crawler/CrawlerSecurityConfiguration
:cognitect.aws.glue/CrawlerSecurityConfiguration)
(s/def :cognitect.aws.glue.Crawler/SchemaChangePolicy :cognitect.aws.glue/SchemaChangePolicy)
(s/def :cognitect.aws.glue.Crawler/LastUpdated :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.Crawler/Schedule :cognitect.aws.glue/Schedule)
(s/def :cognitect.aws.glue.Crawler/Targets :cognitect.aws.glue/CrawlerTargets)
(s/def :cognitect.aws.glue.Crawler/CrawlElapsedTime :cognitect.aws.glue/MillisecondsCount)
(s/def :cognitect.aws.glue.Crawler/Classifiers :cognitect.aws.glue/ClassifierNameList)
(s/def :cognitect.aws.glue.Crawler/CreationTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.Crawler/TablePrefix :cognitect.aws.glue/TablePrefix)
(s/def :cognitect.aws.glue.Crawler/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Crawler/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.Crawler/State :cognitect.aws.glue/CrawlerState)
(s/def :cognitect.aws.glue.Crawler/LastCrawl :cognitect.aws.glue/LastCrawlInfo)
(s/def :cognitect.aws.glue.Crawler/Role :cognitect.aws.glue/Role)
(s/def :cognitect.aws.glue.Crawler/Configuration :cognitect.aws.glue/CrawlerConfiguration)
(s/def
:cognitect.aws.glue.Crawler/LakeFormationConfiguration
:cognitect.aws.glue/LakeFormationConfiguration)
(s/def :cognitect.aws.glue.Crawler/RecrawlPolicy :cognitect.aws.glue/RecrawlPolicy)
(s/def :cognitect.aws.glue.Crawler/Version :cognitect.aws.glue/VersionId)
(s/def :cognitect.aws.glue.Crawler/DatabaseName :cognitect.aws.glue/DatabaseName)
(s/def :cognitect.aws.glue.BatchGetJobsRequest/JobNames :cognitect.aws.glue/JobNameList)
(s/def :cognitect.aws.glue.ListSessionsRequest/NextToken :cognitect.aws.glue/OrchestrationToken)
(s/def :cognitect.aws.glue.ListSessionsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.ListSessionsRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def
:cognitect.aws.glue.ListSessionsRequest/RequestOrigin
:cognitect.aws.glue/OrchestrationNameString)
(s/def :cognitect.aws.glue.GetSessionRequest/Id :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetSessionRequest/RequestOrigin
:cognitect.aws.glue/OrchestrationNameString)
(s/def :cognitect.aws.glue.S3DirectTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.S3DirectTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.S3DirectTarget/PartitionKeys :cognitect.aws.glue/GlueStudioPathList)
(s/def :cognitect.aws.glue.S3DirectTarget/Path :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.S3DirectTarget/Compression :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.S3DirectTarget/Format :cognitect.aws.glue/TargetFormat)
(s/def
:cognitect.aws.glue.S3DirectTarget/SchemaChangePolicy
:cognitect.aws.glue/DirectSchemaChangePolicy)
(s/def :cognitect.aws.glue.Classifier/GrokClassifier :cognitect.aws.glue/GrokClassifier)
(s/def :cognitect.aws.glue.Classifier/XMLClassifier :cognitect.aws.glue/XMLClassifier)
(s/def :cognitect.aws.glue.Classifier/JsonClassifier :cognitect.aws.glue/JsonClassifier)
(s/def :cognitect.aws.glue.Classifier/CsvClassifier :cognitect.aws.glue/CsvClassifier)
(s/def
:cognitect.aws.glue.GetJobBookmarkResponse/JobBookmarkEntry
:cognitect.aws.glue/JobBookmarkEntry)
(s/def :cognitect.aws.glue.TransformSortCriteria/Column :cognitect.aws.glue/TransformSortColumnType)
(s/def
:cognitect.aws.glue.TransformSortCriteria/SortDirection
:cognitect.aws.glue/SortDirectionType)
(s/def :cognitect.aws.glue.UpdateRegistryInput/RegistryId :cognitect.aws.glue/RegistryId)
(s/def :cognitect.aws.glue.UpdateRegistryInput/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.Condition/LogicalOperator :cognitect.aws.glue/LogicalOperator)
(s/def :cognitect.aws.glue.Condition/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Condition/State :cognitect.aws.glue/JobRunState)
(s/def :cognitect.aws.glue.Condition/CrawlerName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Condition/CrawlState :cognitect.aws.glue/CrawlState)
(s/def :cognitect.aws.glue.GetMLTaskRunResponse/ErrorString :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.GetMLTaskRunResponse/TaskRunId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.GetMLTaskRunResponse/ExecutionTime :cognitect.aws.glue/ExecutionTime)
(s/def :cognitect.aws.glue.GetMLTaskRunResponse/TransformId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.GetMLTaskRunResponse/CompletedOn :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.GetMLTaskRunResponse/LogGroupName :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.GetMLTaskRunResponse/LastModifiedOn :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.GetMLTaskRunResponse/StartedOn :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.GetMLTaskRunResponse/Properties :cognitect.aws.glue/TaskRunProperties)
(s/def :cognitect.aws.glue.GetMLTaskRunResponse/Status :cognitect.aws.glue/TaskStatusType)
(s/def
:cognitect.aws.glue.BlueprintDetails/BlueprintName
:cognitect.aws.glue/OrchestrationNameString)
(s/def :cognitect.aws.glue.BlueprintDetails/RunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.KeySchemaElement/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.KeySchemaElement/Type :cognitect.aws.glue/ColumnTypeString)
(s/def :cognitect.aws.glue.Partition/LastAnalyzedTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.Partition/LastAccessTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.Partition/Parameters :cognitect.aws.glue/ParametersMap)
(s/def :cognitect.aws.glue.Partition/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.Partition/StorageDescriptor :cognitect.aws.glue/StorageDescriptor)
(s/def :cognitect.aws.glue.Partition/Values :cognitect.aws.glue/ValueStringList)
(s/def :cognitect.aws.glue.Partition/CreationTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.Partition/TableName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Partition/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DeleteCrawlerRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.DeleteMLTransformRequest/TransformId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.Crawl/State :cognitect.aws.glue/CrawlState)
(s/def :cognitect.aws.glue.Crawl/StartedOn :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.Crawl/CompletedOn :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.Crawl/ErrorMessage :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.Crawl/LogGroup :cognitect.aws.glue/LogGroup)
(s/def :cognitect.aws.glue.Crawl/LogStream :cognitect.aws.glue/LogStream)
(s/def :cognitect.aws.glue.GetBlueprintRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetBlueprintRequest/IncludeBlueprint :cognitect.aws.glue/NullableBoolean)
(s/def
:cognitect.aws.glue.GetBlueprintRequest/IncludeParameterSpec
:cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.GetWorkflowRunsRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetWorkflowRunsRequest/IncludeGraph :cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.GetWorkflowRunsRequest/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.GetWorkflowRunsRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.CatalogEntry/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CatalogEntry/TableName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.LastActiveDefinition/Description
:cognitect.aws.glue/Generic512CharString)
(s/def :cognitect.aws.glue.LastActiveDefinition/LastModifiedOn :cognitect.aws.glue/TimestampValue)
(s/def
:cognitect.aws.glue.LastActiveDefinition/ParameterSpec
:cognitect.aws.glue/BlueprintParameterSpec)
(s/def :cognitect.aws.glue.LastActiveDefinition/BlueprintLocation :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.LastActiveDefinition/BlueprintServiceLocation
:cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.DataSource/GlueTable :cognitect.aws.glue/GlueTable)
(s/def :cognitect.aws.glue.TableIdentifier/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.TableIdentifier/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.TableIdentifier/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.TableIdentifier/Region :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UpdateJobRequest/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.UpdateJobRequest/JobUpdate :cognitect.aws.glue/JobUpdate)
(s/def :cognitect.aws.glue.SkewedInfo/SkewedColumnNames :cognitect.aws.glue/NameStringList)
(s/def :cognitect.aws.glue.SkewedInfo/SkewedColumnValues :cognitect.aws.glue/ColumnValueStringList)
(s/def :cognitect.aws.glue.SkewedInfo/SkewedColumnValueLocationMaps :cognitect.aws.glue/LocationMap)
(s/def :cognitect.aws.glue.StartWorkflowRunRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.StartWorkflowRunRequest/RunProperties
:cognitect.aws.glue/WorkflowRunProperties)
(s/def
:cognitect.aws.glue.DeleteRegistryResponse/RegistryName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.DeleteRegistryResponse/RegistryArn :cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.DeleteRegistryResponse/Status :cognitect.aws.glue/RegistryStatus)
(s/def :cognitect.aws.glue.RemoveSchemaVersionMetadataInput/SchemaId :cognitect.aws.glue/SchemaId)
(s/def
:cognitect.aws.glue.RemoveSchemaVersionMetadataInput/SchemaVersionNumber
:cognitect.aws.glue/SchemaVersionNumber)
(s/def
:cognitect.aws.glue.RemoveSchemaVersionMetadataInput/SchemaVersionId
:cognitect.aws.glue/SchemaVersionIdString)
(s/def
:cognitect.aws.glue.RemoveSchemaVersionMetadataInput/MetadataKeyValue
:cognitect.aws.glue/MetadataKeyValuePair)
(s/def :cognitect.aws.glue.S3SourceAdditionalOptions/BoundedSize :cognitect.aws.glue/BoxedLong)
(s/def :cognitect.aws.glue.S3SourceAdditionalOptions/BoundedFiles :cognitect.aws.glue/BoxedLong)
(s/def :cognitect.aws.glue.SourceControlDetails/Provider :cognitect.aws.glue/SourceControlProvider)
(s/def :cognitect.aws.glue.SourceControlDetails/Repository :cognitect.aws.glue/Generic512CharString)
(s/def :cognitect.aws.glue.SourceControlDetails/Owner :cognitect.aws.glue/Generic512CharString)
(s/def :cognitect.aws.glue.SourceControlDetails/Branch :cognitect.aws.glue/Generic512CharString)
(s/def :cognitect.aws.glue.SourceControlDetails/Folder :cognitect.aws.glue/Generic512CharString)
(s/def
:cognitect.aws.glue.SourceControlDetails/LastCommitId
:cognitect.aws.glue/Generic512CharString)
(s/def
:cognitect.aws.glue.SourceControlDetails/AuthStrategy
:cognitect.aws.glue/SourceControlAuthStrategy)
(s/def :cognitect.aws.glue.SourceControlDetails/AuthToken :cognitect.aws.glue/Generic512CharString)
(s/def :cognitect.aws.glue.CodeGenNode/Id :cognitect.aws.glue/CodeGenIdentifier)
(s/def :cognitect.aws.glue.CodeGenNode/NodeType :cognitect.aws.glue/CodeGenNodeType)
(s/def :cognitect.aws.glue.CodeGenNode/Args :cognitect.aws.glue/CodeGenNodeArgs)
(s/def :cognitect.aws.glue.CodeGenNode/LineNumber :cognitect.aws.glue/Integer)
(s/def
:cognitect.aws.glue.GetUserDefinedFunctionRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.GetUserDefinedFunctionRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.GetUserDefinedFunctionRequest/FunctionName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.DeleteUserDefinedFunctionRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.DeleteUserDefinedFunctionRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.DeleteUserDefinedFunctionRequest/FunctionName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.LakeFormationConfiguration/UseLakeFormationCredentials
:cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.LakeFormationConfiguration/AccountId :cognitect.aws.glue/AccountId)
(s/def :cognitect.aws.glue.GetCustomEntityTypeRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetTableResponse/Table :cognitect.aws.glue/Table)
(s/def
:cognitect.aws.glue.DQResultsPublishingOptions/EvaluationContext
:cognitect.aws.glue/GenericLimitedString)
(s/def
:cognitect.aws.glue.DQResultsPublishingOptions/ResultsS3Prefix
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.DQResultsPublishingOptions/CloudWatchMetricsEnabled
:cognitect.aws.glue/BoxedBoolean)
(s/def
:cognitect.aws.glue.DQResultsPublishingOptions/ResultsPublishingEnabled
:cognitect.aws.glue/BoxedBoolean)
(s/def
:cognitect.aws.glue.StartMLEvaluationTaskRunResponse/TaskRunId
:cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.RecrawlPolicy/RecrawlBehavior :cognitect.aws.glue/RecrawlBehavior)
(s/def :cognitect.aws.glue.Location/Jdbc :cognitect.aws.glue/CodeGenNodeArgs)
(s/def :cognitect.aws.glue.Location/S3 :cognitect.aws.glue/CodeGenNodeArgs)
(s/def :cognitect.aws.glue.Location/DynamoDB :cognitect.aws.glue/CodeGenNodeArgs)
(s/def :cognitect.aws.glue.BatchDeleteTableRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.BatchDeleteTableRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.BatchDeleteTableRequest/TablesToDelete
:cognitect.aws.glue/BatchDeleteTableNameList)
(s/def
:cognitect.aws.glue.BatchDeleteTableRequest/TransactionId
:cognitect.aws.glue/TransactionIdString)
(s/def :cognitect.aws.glue.Statement/Id :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.Statement/Code :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.Statement/State :cognitect.aws.glue/StatementState)
(s/def :cognitect.aws.glue.Statement/Output :cognitect.aws.glue/StatementOutput)
(s/def :cognitect.aws.glue.Statement/Progress :cognitect.aws.glue/DoubleValue)
(s/def :cognitect.aws.glue.Statement/StartedOn :cognitect.aws.glue/LongValue)
(s/def :cognitect.aws.glue.Statement/CompletedOn :cognitect.aws.glue/LongValue)
(s/def
:cognitect.aws.glue.AuditContext/AdditionalAuditContext
:cognitect.aws.glue/AuditContextString)
(s/def :cognitect.aws.glue.AuditContext/RequestedColumns :cognitect.aws.glue/AuditColumnNamesList)
(s/def :cognitect.aws.glue.AuditContext/AllColumnsRequested :cognitect.aws.glue/NullableBoolean)
(s/def :cognitect.aws.glue.Session/ErrorMessage :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.Session/SecurityConfiguration :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Session/Id :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Session/MaxCapacity :cognitect.aws.glue/NullableDouble)
(s/def :cognitect.aws.glue.Session/DefaultArguments :cognitect.aws.glue/OrchestrationArgumentsMap)
(s/def :cognitect.aws.glue.Session/Command :cognitect.aws.glue/SessionCommand)
(s/def :cognitect.aws.glue.Session/CreatedOn :cognitect.aws.glue/TimestampValue)
(s/def :cognitect.aws.glue.Session/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.Session/GlueVersion :cognitect.aws.glue/GlueVersionString)
(s/def :cognitect.aws.glue.Session/Connections :cognitect.aws.glue/ConnectionsList)
(s/def :cognitect.aws.glue.Session/Role :cognitect.aws.glue/OrchestrationRoleArn)
(s/def :cognitect.aws.glue.Session/Progress :cognitect.aws.glue/DoubleValue)
(s/def :cognitect.aws.glue.Session/Status :cognitect.aws.glue/SessionStatus)
(s/def :cognitect.aws.glue.GetMLTransformResponse/MaxRetries :cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.GetMLTransformResponse/Timeout :cognitect.aws.glue/Timeout)
(s/def
:cognitect.aws.glue.GetMLTransformResponse/NumberOfWorkers
:cognitect.aws.glue/NullableInteger)
(s/def :cognitect.aws.glue.GetMLTransformResponse/TransformId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.GetMLTransformResponse/MaxCapacity :cognitect.aws.glue/NullableDouble)
(s/def
:cognitect.aws.glue.GetMLTransformResponse/Parameters
:cognitect.aws.glue/TransformParameters)
(s/def :cognitect.aws.glue.GetMLTransformResponse/LabelCount :cognitect.aws.glue/LabelCount)
(s/def
:cognitect.aws.glue.GetMLTransformResponse/TransformEncryption
:cognitect.aws.glue/TransformEncryption)
(s/def :cognitect.aws.glue.GetMLTransformResponse/LastModifiedOn :cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.GetMLTransformResponse/EvaluationMetrics
:cognitect.aws.glue/EvaluationMetrics)
(s/def :cognitect.aws.glue.GetMLTransformResponse/WorkerType :cognitect.aws.glue/WorkerType)
(s/def :cognitect.aws.glue.GetMLTransformResponse/Schema :cognitect.aws.glue/TransformSchema)
(s/def :cognitect.aws.glue.GetMLTransformResponse/CreatedOn :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.GetMLTransformResponse/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetMLTransformResponse/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.GetMLTransformResponse/GlueVersion :cognitect.aws.glue/GlueVersionString)
(s/def :cognitect.aws.glue.GetMLTransformResponse/Role :cognitect.aws.glue/RoleString)
(s/def :cognitect.aws.glue.GetMLTransformResponse/InputRecordTables :cognitect.aws.glue/GlueTables)
(s/def :cognitect.aws.glue.GetMLTransformResponse/Status :cognitect.aws.glue/TransformStatusType)
(s/def :cognitect.aws.glue.UpdateCsvClassifierRequest/Header :cognitect.aws.glue/CsvHeader)
(s/def
:cognitect.aws.glue.UpdateCsvClassifierRequest/Delimiter
:cognitect.aws.glue/CsvColumnDelimiter)
(s/def
:cognitect.aws.glue.UpdateCsvClassifierRequest/CustomDatatypes
:cognitect.aws.glue/CustomDatatypes)
(s/def
:cognitect.aws.glue.UpdateCsvClassifierRequest/CustomDatatypeConfigured
:cognitect.aws.glue/NullableBoolean)
(s/def
:cognitect.aws.glue.UpdateCsvClassifierRequest/QuoteSymbol
:cognitect.aws.glue/CsvQuoteSymbol)
(s/def :cognitect.aws.glue.UpdateCsvClassifierRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateCsvClassifierRequest/DisableValueTrimming
:cognitect.aws.glue/NullableBoolean)
(s/def
:cognitect.aws.glue.UpdateCsvClassifierRequest/AllowSingleColumn
:cognitect.aws.glue/NullableBoolean)
(s/def
:cognitect.aws.glue.UpdateCsvClassifierRequest/ContainsHeader
:cognitect.aws.glue/CsvHeaderOption)
(s/def
:cognitect.aws.glue.GetCrawlerMetricsResponse/CrawlerMetricsList
:cognitect.aws.glue/CrawlerMetricsList)
(s/def :cognitect.aws.glue.GetCrawlerMetricsResponse/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.GetJobBookmarkRequest/JobName :cognitect.aws.glue/JobName)
(s/def :cognitect.aws.glue.GetJobBookmarkRequest/RunId :cognitect.aws.glue/RunId)
(s/def :cognitect.aws.glue.DeleteMLTransformResponse/TransformId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.GetJobRunsResponse/JobRuns :cognitect.aws.glue/JobRunList)
(s/def :cognitect.aws.glue.GetJobRunsResponse/NextToken :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.SchemaVersionErrorItem/VersionNumber
:cognitect.aws.glue/VersionLongNumber)
(s/def :cognitect.aws.glue.SchemaVersionErrorItem/ErrorDetails :cognitect.aws.glue/ErrorDetails)
(s/def :cognitect.aws.glue.GetTableVersionsRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.GetTableVersionsRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetTableVersionsRequest/TableName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetTableVersionsRequest/NextToken :cognitect.aws.glue/Token)
(s/def
:cognitect.aws.glue.GetTableVersionsRequest/MaxResults
:cognitect.aws.glue/CatalogGetterPageSize)
(s/def
:cognitect.aws.glue.StartDataQualityRuleRecommendationRunResponse/RunId
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.Connection/PhysicalConnectionRequirements
:cognitect.aws.glue/PhysicalConnectionRequirements)
(s/def :cognitect.aws.glue.Connection/MatchCriteria :cognitect.aws.glue/MatchCriteria)
(s/def :cognitect.aws.glue.Connection/LastUpdatedTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.Connection/ConnectionProperties :cognitect.aws.glue/ConnectionProperties)
(s/def :cognitect.aws.glue.Connection/ConnectionType :cognitect.aws.glue/ConnectionType)
(s/def :cognitect.aws.glue.Connection/CreationTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.Connection/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Connection/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.Connection/LastUpdatedBy :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreateDatabaseRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.CreateDatabaseRequest/DatabaseInput :cognitect.aws.glue/DatabaseInput)
(s/def :cognitect.aws.glue.CreateDatabaseRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.TransformFilterCriteria/LastModifiedAfter :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.TransformFilterCriteria/TransformType :cognitect.aws.glue/TransformType)
(s/def :cognitect.aws.glue.TransformFilterCriteria/LastModifiedBefore :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.TransformFilterCriteria/CreatedAfter :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.TransformFilterCriteria/Schema :cognitect.aws.glue/TransformSchema)
(s/def :cognitect.aws.glue.TransformFilterCriteria/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.TransformFilterCriteria/GlueVersion
:cognitect.aws.glue/GlueVersionString)
(s/def :cognitect.aws.glue.TransformFilterCriteria/CreatedBefore :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.TransformFilterCriteria/Status :cognitect.aws.glue/TransformStatusType)
(s/def
:cognitect.aws.glue.CreatePartitionIndexRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.CreatePartitionIndexRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CreatePartitionIndexRequest/TableName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.CreatePartitionIndexRequest/PartitionIndex
:cognitect.aws.glue/PartitionIndex)
(s/def :cognitect.aws.glue.SchemaReference/SchemaId :cognitect.aws.glue/SchemaId)
(s/def
:cognitect.aws.glue.SchemaReference/SchemaVersionId
:cognitect.aws.glue/SchemaVersionIdString)
(s/def
:cognitect.aws.glue.SchemaReference/SchemaVersionNumber
:cognitect.aws.glue/VersionLongNumber)
(s/def
:cognitect.aws.glue.BatchDeleteTableVersionRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.BatchDeleteTableVersionRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.BatchDeleteTableVersionRequest/TableName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.BatchDeleteTableVersionRequest/VersionIds
:cognitect.aws.glue/BatchDeleteTableVersionList)
(s/def
:cognitect.aws.glue.CreateRegistryInput/RegistryName
:cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.CreateRegistryInput/Description :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.CreateRegistryInput/Tags :cognitect.aws.glue/TagsMap)
(s/def :cognitect.aws.glue.ColumnStatisticsData/Type :cognitect.aws.glue/ColumnStatisticsType)
(s/def
:cognitect.aws.glue.ColumnStatisticsData/BooleanColumnStatisticsData
:cognitect.aws.glue/BooleanColumnStatisticsData)
(s/def
:cognitect.aws.glue.ColumnStatisticsData/DateColumnStatisticsData
:cognitect.aws.glue/DateColumnStatisticsData)
(s/def
:cognitect.aws.glue.ColumnStatisticsData/DecimalColumnStatisticsData
:cognitect.aws.glue/DecimalColumnStatisticsData)
(s/def
:cognitect.aws.glue.ColumnStatisticsData/DoubleColumnStatisticsData
:cognitect.aws.glue/DoubleColumnStatisticsData)
(s/def
:cognitect.aws.glue.ColumnStatisticsData/LongColumnStatisticsData
:cognitect.aws.glue/LongColumnStatisticsData)
(s/def
:cognitect.aws.glue.ColumnStatisticsData/StringColumnStatisticsData
:cognitect.aws.glue/StringColumnStatisticsData)
(s/def
:cognitect.aws.glue.ColumnStatisticsData/BinaryColumnStatisticsData
:cognitect.aws.glue/BinaryColumnStatisticsData)
(s/def :cognitect.aws.glue.FilterValue/Type :cognitect.aws.glue/FilterValueType)
(s/def :cognitect.aws.glue.FilterValue/Value :cognitect.aws.glue/EnclosedInStringProperties)
(s/def
:cognitect.aws.glue.BatchGetDataQualityResultRequest/ResultIds
:cognitect.aws.glue/DataQualityResultIds)
(s/def :cognitect.aws.glue.CreateDataQualityRulesetRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.CreateDataQualityRulesetRequest/Description
:cognitect.aws.glue/DescriptionString)
(s/def
:cognitect.aws.glue.CreateDataQualityRulesetRequest/Ruleset
:cognitect.aws.glue/DataQualityRulesetString)
(s/def :cognitect.aws.glue.CreateDataQualityRulesetRequest/Tags :cognitect.aws.glue/TagsMap)
(s/def
:cognitect.aws.glue.CreateDataQualityRulesetRequest/TargetTable
:cognitect.aws.glue/DataQualityTargetTable)
(s/def
:cognitect.aws.glue.CreateDataQualityRulesetRequest/ClientToken
:cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.MicrosoftSQLServerCatalogSource/Name :cognitect.aws.glue/NodeName)
(s/def
:cognitect.aws.glue.MicrosoftSQLServerCatalogSource/Database
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def
:cognitect.aws.glue.MicrosoftSQLServerCatalogSource/Table
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.GetJobsResponse/Jobs :cognitect.aws.glue/JobList)
(s/def :cognitect.aws.glue.GetJobsResponse/NextToken :cognitect.aws.glue/GenericString)
(s/def
:cognitect.aws.glue.BatchGetCustomEntityTypesRequest/Names
:cognitect.aws.glue/CustomEntityTypeNames)
(s/def
:cognitect.aws.glue.DecimalColumnStatisticsData/MinimumValue
:cognitect.aws.glue/DecimalNumber)
(s/def
:cognitect.aws.glue.DecimalColumnStatisticsData/MaximumValue
:cognitect.aws.glue/DecimalNumber)
(s/def
:cognitect.aws.glue.DecimalColumnStatisticsData/NumberOfNulls
:cognitect.aws.glue/NonNegativeLong)
(s/def
:cognitect.aws.glue.DecimalColumnStatisticsData/NumberOfDistinctValues
:cognitect.aws.glue/NonNegativeLong)
(s/def :cognitect.aws.glue.IcebergInput/MetadataOperation :cognitect.aws.glue/MetadataOperation)
(s/def :cognitect.aws.glue.IcebergInput/Version :cognitect.aws.glue/VersionString)
(s/def :cognitect.aws.glue.GetCrawlersRequest/MaxResults :cognitect.aws.glue/PageSize)
(s/def :cognitect.aws.glue.GetCrawlersRequest/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.GetDataflowGraphResponse/DagNodes :cognitect.aws.glue/DagNodes)
(s/def :cognitect.aws.glue.GetDataflowGraphResponse/DagEdges :cognitect.aws.glue/DagEdges)
(s/def :cognitect.aws.glue.GetStatementResponse/Statement :cognitect.aws.glue/Statement)
(s/def :cognitect.aws.glue.RenameField/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.RenameField/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.RenameField/SourcePath :cognitect.aws.glue/EnclosedInStringProperties)
(s/def :cognitect.aws.glue.RenameField/TargetPath :cognitect.aws.glue/EnclosedInStringProperties)
(s/def :cognitect.aws.glue.DecimalNumber/UnscaledValue :cognitect.aws.glue/Blob)
(s/def :cognitect.aws.glue.DecimalNumber/Scale :cognitect.aws.glue/Integer)
(s/def :cognitect.aws.glue.RegistryId/RegistryName :cognitect.aws.glue/SchemaRegistryNameString)
(s/def :cognitect.aws.glue.RegistryId/RegistryArn :cognitect.aws.glue/GlueResourceArn)
(s/def :cognitect.aws.glue.StopSessionRequest/Id :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.StopSessionRequest/RequestOrigin
:cognitect.aws.glue/OrchestrationNameString)
(s/def
:cognitect.aws.glue.S3DirectSourceAdditionalOptions/BoundedSize
:cognitect.aws.glue/BoxedLong)
(s/def
:cognitect.aws.glue.S3DirectSourceAdditionalOptions/BoundedFiles
:cognitect.aws.glue/BoxedLong)
(s/def
:cognitect.aws.glue.S3DirectSourceAdditionalOptions/EnableSamplePath
:cognitect.aws.glue/BoxedBoolean)
(s/def
:cognitect.aws.glue.S3DirectSourceAdditionalOptions/SamplePath
:cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.MySQLCatalogTarget/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.MySQLCatalogTarget/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.MySQLCatalogTarget/Database :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.MySQLCatalogTarget/Table :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.GetTriggersResponse/Triggers :cognitect.aws.glue/TriggerList)
(s/def :cognitect.aws.glue.GetTriggersResponse/NextToken :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.CancelMLTaskRunRequest/TransformId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.CancelMLTaskRunRequest/TaskRunId :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.DeleteTriggerResponse/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetDataQualityRulesetRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.PutSchemaVersionMetadataInput/SchemaId :cognitect.aws.glue/SchemaId)
(s/def
:cognitect.aws.glue.PutSchemaVersionMetadataInput/SchemaVersionNumber
:cognitect.aws.glue/SchemaVersionNumber)
(s/def
:cognitect.aws.glue.PutSchemaVersionMetadataInput/SchemaVersionId
:cognitect.aws.glue/SchemaVersionIdString)
(s/def
:cognitect.aws.glue.PutSchemaVersionMetadataInput/MetadataKeyValue
:cognitect.aws.glue/MetadataKeyValuePair)
(s/def
:cognitect.aws.glue.UpdateUserDefinedFunctionRequest/CatalogId
:cognitect.aws.glue/CatalogIdString)
(s/def
:cognitect.aws.glue.UpdateUserDefinedFunctionRequest/DatabaseName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateUserDefinedFunctionRequest/FunctionName
:cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.UpdateUserDefinedFunctionRequest/FunctionInput
:cognitect.aws.glue/UserDefinedFunctionInput)
(s/def
:cognitect.aws.glue.GetResourcePolicyResponse/PolicyInJson
:cognitect.aws.glue/PolicyJsonString)
(s/def :cognitect.aws.glue.GetResourcePolicyResponse/PolicyHash :cognitect.aws.glue/HashString)
(s/def :cognitect.aws.glue.GetResourcePolicyResponse/CreateTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.GetResourcePolicyResponse/UpdateTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.CreateScriptRequest/DagNodes :cognitect.aws.glue/DagNodes)
(s/def :cognitect.aws.glue.CreateScriptRequest/DagEdges :cognitect.aws.glue/DagEdges)
(s/def :cognitect.aws.glue.CreateScriptRequest/Language :cognitect.aws.glue/Language)
(s/def :cognitect.aws.glue.UpdateTriggerResponse/Trigger :cognitect.aws.glue/Trigger)
(s/def :cognitect.aws.glue.GetMLTaskRunsResponse/TaskRuns :cognitect.aws.glue/TaskRunList)
(s/def :cognitect.aws.glue.GetMLTaskRunsResponse/NextToken :cognitect.aws.glue/PaginationToken)
(s/def :cognitect.aws.glue.CrawlerHistory/EndTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.CrawlerHistory/DPUHour :cognitect.aws.glue/NonNegativeDouble)
(s/def :cognitect.aws.glue.CrawlerHistory/ErrorMessage :cognitect.aws.glue/DescriptionString)
(s/def :cognitect.aws.glue.CrawlerHistory/LogGroup :cognitect.aws.glue/LogGroup)
(s/def :cognitect.aws.glue.CrawlerHistory/StartTime :cognitect.aws.glue/Timestamp)
(s/def :cognitect.aws.glue.CrawlerHistory/MessagePrefix :cognitect.aws.glue/MessagePrefix)
(s/def :cognitect.aws.glue.CrawlerHistory/Summary :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.CrawlerHistory/State :cognitect.aws.glue/CrawlerHistoryState)
(s/def :cognitect.aws.glue.CrawlerHistory/LogStream :cognitect.aws.glue/LogStream)
(s/def :cognitect.aws.glue.CrawlerHistory/CrawlId :cognitect.aws.glue/CrawlId)
(s/def :cognitect.aws.glue.BatchCreatePartitionResponse/Errors :cognitect.aws.glue/PartitionErrors)
(s/def
:cognitect.aws.glue.QuerySchemaVersionMetadataResponse/MetadataInfoMap
:cognitect.aws.glue/MetadataInfoMap)
(s/def
:cognitect.aws.glue.QuerySchemaVersionMetadataResponse/SchemaVersionId
:cognitect.aws.glue/SchemaVersionIdString)
(s/def
:cognitect.aws.glue.QuerySchemaVersionMetadataResponse/NextToken
:cognitect.aws.glue/SchemaRegistryTokenString)
(s/def :cognitect.aws.glue.DropNullFields/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.DropNullFields/Inputs :cognitect.aws.glue/OneInput)
(s/def :cognitect.aws.glue.DropNullFields/NullCheckBoxList :cognitect.aws.glue/NullCheckBoxList)
(s/def :cognitect.aws.glue.DropNullFields/NullTextList :cognitect.aws.glue/NullValueFields)
(s/def :cognitect.aws.glue.CreateCsvClassifierRequest/Header :cognitect.aws.glue/CsvHeader)
(s/def
:cognitect.aws.glue.CreateCsvClassifierRequest/Delimiter
:cognitect.aws.glue/CsvColumnDelimiter)
(s/def
:cognitect.aws.glue.CreateCsvClassifierRequest/CustomDatatypes
:cognitect.aws.glue/CustomDatatypes)
(s/def
:cognitect.aws.glue.CreateCsvClassifierRequest/CustomDatatypeConfigured
:cognitect.aws.glue/NullableBoolean)
(s/def
:cognitect.aws.glue.CreateCsvClassifierRequest/QuoteSymbol
:cognitect.aws.glue/CsvQuoteSymbol)
(s/def :cognitect.aws.glue.CreateCsvClassifierRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.CreateCsvClassifierRequest/DisableValueTrimming
:cognitect.aws.glue/NullableBoolean)
(s/def
:cognitect.aws.glue.CreateCsvClassifierRequest/AllowSingleColumn
:cognitect.aws.glue/NullableBoolean)
(s/def
:cognitect.aws.glue.CreateCsvClassifierRequest/ContainsHeader
:cognitect.aws.glue/CsvHeaderOption)
(s/def :cognitect.aws.glue.StartJobRunResponse/JobRunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.DeleteDatabaseRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.DeleteDatabaseRequest/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.ListDataQualityRuleRecommendationRunsResponse/Runs
:cognitect.aws.glue/DataQualityRuleRecommendationRunList)
(s/def
:cognitect.aws.glue.ListDataQualityRuleRecommendationRunsResponse/NextToken
:cognitect.aws.glue/PaginationToken)
(s/def :cognitect.aws.glue.BatchGetTriggersRequest/TriggerNames :cognitect.aws.glue/TriggerNameList)
(s/def :cognitect.aws.glue.CreateJobResponse/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.BatchGetPartitionRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.BatchGetPartitionRequest/DatabaseName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.BatchGetPartitionRequest/TableName :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.BatchGetPartitionRequest/PartitionsToGet
:cognitect.aws.glue/BatchGetPartitionValueList)
(s/def :cognitect.aws.glue.CreateMLTransformResponse/TransformId :cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.DataQualityRulesetEvaluationRunDescription/RunId
:cognitect.aws.glue/HashString)
(s/def
:cognitect.aws.glue.DataQualityRulesetEvaluationRunDescription/Status
:cognitect.aws.glue/TaskStatusType)
(s/def
:cognitect.aws.glue.DataQualityRulesetEvaluationRunDescription/StartedOn
:cognitect.aws.glue/Timestamp)
(s/def
:cognitect.aws.glue.DataQualityRulesetEvaluationRunDescription/DataSource
:cognitect.aws.glue/DataSource)
(s/def
:cognitect.aws.glue.GetColumnStatisticsForPartitionResponse/ColumnStatisticsList
:cognitect.aws.glue/ColumnStatisticsList)
(s/def
:cognitect.aws.glue.GetColumnStatisticsForPartitionResponse/Errors
:cognitect.aws.glue/ColumnErrors)
(s/def
:cognitect.aws.glue.GetUserDefinedFunctionResponse/UserDefinedFunction
:cognitect.aws.glue/UserDefinedFunction)
(s/def :cognitect.aws.glue.StopTriggerResponse/Name :cognitect.aws.glue/NameString)
(s/def
:cognitect.aws.glue.DeleteSchemaVersionsResponse/SchemaVersionErrors
:cognitect.aws.glue/SchemaVersionErrorList)
(s/def :cognitect.aws.glue.BatchStopJobRunError/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.BatchStopJobRunError/JobRunId :cognitect.aws.glue/IdString)
(s/def :cognitect.aws.glue.BatchStopJobRunError/ErrorDetail :cognitect.aws.glue/ErrorDetail)
(s/def :cognitect.aws.glue.JobNodeDetails/JobRuns :cognitect.aws.glue/JobRunList)
(s/def :cognitect.aws.glue.StatementOutput/Data :cognitect.aws.glue/StatementOutputData)
(s/def :cognitect.aws.glue.StatementOutput/ExecutionCount :cognitect.aws.glue/IntegerValue)
(s/def :cognitect.aws.glue.StatementOutput/Status :cognitect.aws.glue/StatementState)
(s/def :cognitect.aws.glue.StatementOutput/ErrorName :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.StatementOutput/ErrorValue :cognitect.aws.glue/GenericString)
(s/def :cognitect.aws.glue.StatementOutput/Traceback :cognitect.aws.glue/OrchestrationStringList)
(s/def :cognitect.aws.glue.GetCrawlersResponse/Crawlers :cognitect.aws.glue/CrawlerList)
(s/def :cognitect.aws.glue.GetCrawlersResponse/NextToken :cognitect.aws.glue/Token)
(s/def :cognitect.aws.glue.CustomCode/Name :cognitect.aws.glue/NodeName)
(s/def :cognitect.aws.glue.CustomCode/Inputs :cognitect.aws.glue/ManyInputs)
(s/def :cognitect.aws.glue.CustomCode/Code :cognitect.aws.glue/ExtendedString)
(s/def :cognitect.aws.glue.CustomCode/ClassName :cognitect.aws.glue/EnclosedInStringProperty)
(s/def :cognitect.aws.glue.CustomCode/OutputSchemas :cognitect.aws.glue/GlueSchemas)
(s/def :cognitect.aws.glue.MetadataKeyValuePair/MetadataKey :cognitect.aws.glue/MetadataKeyString)
(s/def
:cognitect.aws.glue.MetadataKeyValuePair/MetadataValue
:cognitect.aws.glue/MetadataValueString)
(s/def :cognitect.aws.glue.DeleteJobRequest/JobName :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetConnectionRequest/CatalogId :cognitect.aws.glue/CatalogIdString)
(s/def :cognitect.aws.glue.GetConnectionRequest/Name :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.GetConnectionRequest/HidePassword :cognitect.aws.glue/Boolean)
(s/def :cognitect.aws.glue.Order/Column :cognitect.aws.glue/NameString)
(s/def :cognitect.aws.glue.Order/SortOrder :cognitect.aws.glue/IntegerFlag)
(s/def :cognitect.aws.glue.CodeGenNodeArg/Name :cognitect.aws.glue/CodeGenArgName)
(s/def :cognitect.aws.glue.CodeGenNodeArg/Value :cognitect.aws.glue/CodeGenArgValue)
(s/def :cognitect.aws.glue.CodeGenNodeArg/Param :cognitect.aws.glue/Boolean)
(s/def
:cognitect.aws.glue.ListMLTransformsResponse/TransformIds
:cognitect.aws.glue/TransformIdList)
(s/def :cognitect.aws.glue.ListMLTransformsResponse/NextToken :cognitect.aws.glue/PaginationToken)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy