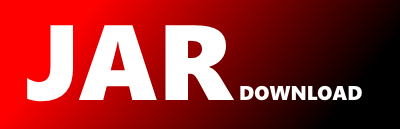
cognitect.aws.iotevents.specs.clj Maven / Gradle / Ivy
;; Copyright (c) Cognitect, Inc.
;; All rights reserved.
(ns cognitect.aws.iotevents.specs
(:require [clojure.spec.alpha :as s] [clojure.spec.gen.alpha :as gen]))
(s/def :cognitect.aws/client map?)
(s/def :core.async/channel any?)
(s/def :cognitect.aws.iotevents/EvaluationMethod (s/spec string? :gen #(s/gen #{"SERIAL" "BATCH"})))
(s/def
:cognitect.aws.iotevents/InputDescription
(s/spec
(s/and string? #(>= 128 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 128) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.iotevents/SetTimerAction
(s/keys
:req-un
[:cognitect.aws.iotevents.SetTimerAction/timerName
:cognitect.aws.iotevents.SetTimerAction/seconds]))
(s/def
:cognitect.aws.iotevents/DescribeLoggingOptionsResponse
(s/keys :opt-un [:cognitect.aws.iotevents.DescribeLoggingOptionsResponse/loggingOptions]))
(s/def
:cognitect.aws.iotevents/TagResourceRequest
(s/keys
:req-un
[:cognitect.aws.iotevents.TagResourceRequest/resourceArn
:cognitect.aws.iotevents.TagResourceRequest/tags]))
(s/def
:cognitect.aws.iotevents/InputSummary
(s/keys
:opt-un
[:cognitect.aws.iotevents.InputSummary/status
:cognitect.aws.iotevents.InputSummary/inputArn
:cognitect.aws.iotevents.InputSummary/inputDescription
:cognitect.aws.iotevents.InputSummary/creationTime
:cognitect.aws.iotevents.InputSummary/lastUpdateTime
:cognitect.aws.iotevents.InputSummary/inputName]))
(s/def
:cognitect.aws.iotevents/OnInputLifecycle
(s/keys
:opt-un
[:cognitect.aws.iotevents.OnInputLifecycle/transitionEvents
:cognitect.aws.iotevents.OnInputLifecycle/events]))
(s/def :cognitect.aws.iotevents/DescribeLoggingOptionsRequest (s/keys))
(s/def :cognitect.aws.iotevents/Actions (s/coll-of :cognitect.aws.iotevents/Action))
(s/def
:cognitect.aws.iotevents/DetectorModelDescription
(s/spec
(s/and string? #(>= 128 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 128) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.iotevents/DetectorModel
(s/keys
:opt-un
[:cognitect.aws.iotevents.DetectorModel/detectorModelConfiguration
:cognitect.aws.iotevents.DetectorModel/detectorModelDefinition]))
(s/def
:cognitect.aws.iotevents/Tag
(s/keys :req-un [:cognitect.aws.iotevents.Tag/key :cognitect.aws.iotevents.Tag/value]))
(s/def
:cognitect.aws.iotevents/DetectorModelVersionSummaries
(s/coll-of :cognitect.aws.iotevents/DetectorModelVersionSummary))
(s/def :cognitect.aws.iotevents/UseBase64 boolean?)
(s/def :cognitect.aws.iotevents/TagResourceResponse (s/keys))
(s/def
:cognitect.aws.iotevents/DetectorDebugOption
(s/keys
:req-un
[:cognitect.aws.iotevents.DetectorDebugOption/detectorModelName]
:opt-un
[:cognitect.aws.iotevents.DetectorDebugOption/keyValue]))
(s/def
:cognitect.aws.iotevents/EventName
(s/spec
(s/and string? #(>= 128 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 128) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.iotevents/InputDefinition
(s/keys :req-un [:cognitect.aws.iotevents.InputDefinition/attributes]))
(s/def
:cognitect.aws.iotevents/OnExitLifecycle
(s/keys :opt-un [:cognitect.aws.iotevents.OnExitLifecycle/events]))
(s/def
:cognitect.aws.iotevents/UpdateInputResponse
(s/keys :opt-un [:cognitect.aws.iotevents.UpdateInputResponse/inputConfiguration]))
(s/def :cognitect.aws.iotevents/Events (s/coll-of :cognitect.aws.iotevents/Event))
(s/def :cognitect.aws.iotevents/DeliveryStreamName string?)
(s/def
:cognitect.aws.iotevents/ResetTimerAction
(s/keys :req-un [:cognitect.aws.iotevents.ResetTimerAction/timerName]))
(s/def
:cognitect.aws.iotevents/InputName
(s/spec #(re-matches (re-pattern "^[a-zA-Z][a-zA-Z0-9_]*$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.iotevents/LoggingOptions
(s/keys
:req-un
[:cognitect.aws.iotevents.LoggingOptions/roleArn
:cognitect.aws.iotevents.LoggingOptions/level
:cognitect.aws.iotevents.LoggingOptions/enabled]
:opt-un
[:cognitect.aws.iotevents.LoggingOptions/detectorDebugOptions]))
(s/def
:cognitect.aws.iotevents/ListDetectorModelsRequest
(s/keys
:opt-un
[:cognitect.aws.iotevents.ListDetectorModelsRequest/maxResults
:cognitect.aws.iotevents.ListDetectorModelsRequest/nextToken]))
(s/def
:cognitect.aws.iotevents/DetectorModelSummary
(s/keys
:opt-un
[:cognitect.aws.iotevents.DetectorModelSummary/detectorModelDescription
:cognitect.aws.iotevents.DetectorModelSummary/creationTime
:cognitect.aws.iotevents.DetectorModelSummary/detectorModelName]))
(s/def :cognitect.aws.iotevents/LoggingEnabled boolean?)
(s/def
:cognitect.aws.iotevents/Input
(s/keys
:opt-un
[:cognitect.aws.iotevents.Input/inputDefinition
:cognitect.aws.iotevents.Input/inputConfiguration]))
(s/def
:cognitect.aws.iotevents/VariableValue
(s/spec
(s/and string? #(<= 1 (count %) 1024))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 1024) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.iotevents/DetectorModelVersionStatus
(s/spec
string?
:gen
#(s/gen #{"DEPRECATED" "ACTIVATING" "DRAFT" "INACTIVE" "ACTIVE" "FAILED" "PAUSED"})))
(s/def :cognitect.aws.iotevents/Tags (s/coll-of :cognitect.aws.iotevents/Tag))
(s/def
:cognitect.aws.iotevents/InputStatus
(s/spec string? :gen #(s/gen #{"UPDATING" "DELETING" "CREATING" "ACTIVE"})))
(s/def
:cognitect.aws.iotevents/KeyValue
(s/spec #(re-matches (re-pattern "^[a-zA-Z0-9\\-_:]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.iotevents/DetectorDebugOptions
(s/coll-of :cognitect.aws.iotevents/DetectorDebugOption :min-count 1))
(s/def
:cognitect.aws.iotevents/SetVariableAction
(s/keys
:req-un
[:cognitect.aws.iotevents.SetVariableAction/variableName
:cognitect.aws.iotevents.SetVariableAction/value]))
(s/def
:cognitect.aws.iotevents/OnEnterLifecycle
(s/keys :opt-un [:cognitect.aws.iotevents.OnEnterLifecycle/events]))
(s/def
:cognitect.aws.iotevents/CreateInputRequest
(s/keys
:req-un
[:cognitect.aws.iotevents.CreateInputRequest/inputName
:cognitect.aws.iotevents.CreateInputRequest/inputDefinition]
:opt-un
[:cognitect.aws.iotevents.CreateInputRequest/tags
:cognitect.aws.iotevents.CreateInputRequest/inputDescription]))
(s/def
:cognitect.aws.iotevents/Event
(s/keys
:req-un
[:cognitect.aws.iotevents.Event/eventName]
:opt-un
[:cognitect.aws.iotevents.Event/condition :cognitect.aws.iotevents.Event/actions]))
(s/def
:cognitect.aws.iotevents/IotTopicPublishAction
(s/keys :req-un [:cognitect.aws.iotevents.IotTopicPublishAction/mqttTopic]))
(s/def
:cognitect.aws.iotevents/AmazonResourceName
(s/spec
(s/and string? #(<= 1 (count %) 2048))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 2048) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.iotevents/TransitionEvents
(s/coll-of :cognitect.aws.iotevents/TransitionEvent))
(s/def
:cognitect.aws.iotevents/AttributeJsonPath
(s/spec
#(re-matches (re-pattern "^((`[\\w\\- ]+`)|([\\w\\-]+))(\\.((`[\\w- ]+`)|([\\w\\-]+)))*$") %)
:gen
#(gen/string)))
(s/def :cognitect.aws.iotevents/NextToken string?)
(s/def
:cognitect.aws.iotevents/UpdateDetectorModelResponse
(s/keys
:opt-un
[:cognitect.aws.iotevents.UpdateDetectorModelResponse/detectorModelConfiguration]))
(s/def
:cognitect.aws.iotevents/DescribeInputResponse
(s/keys :opt-un [:cognitect.aws.iotevents.DescribeInputResponse/input]))
(s/def
:cognitect.aws.iotevents/SNSTopicPublishAction
(s/keys :req-un [:cognitect.aws.iotevents.SNSTopicPublishAction/targetArn]))
(s/def
:cognitect.aws.iotevents/StateName
(s/spec
(s/and string? #(<= 1 (count %) 128))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 128) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.iotevents/DeleteDetectorModelRequest
(s/keys :req-un [:cognitect.aws.iotevents.DeleteDetectorModelRequest/detectorModelName]))
(s/def
:cognitect.aws.iotevents/LoggingLevel
(s/spec string? :gen #(s/gen #{"ERROR" "DEBUG" "INFO"})))
(s/def
:cognitect.aws.iotevents/TagValue
(s/spec
(s/and string? #(<= 0 (count %) 256))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 256) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.iotevents/IotEventsAction
(s/keys :req-un [:cognitect.aws.iotevents.IotEventsAction/inputName]))
(s/def
:cognitect.aws.iotevents/ListTagsForResourceRequest
(s/keys :req-un [:cognitect.aws.iotevents.ListTagsForResourceRequest/resourceArn]))
(s/def
:cognitect.aws.iotevents/DetectorModelSummaries
(s/coll-of :cognitect.aws.iotevents/DetectorModelSummary))
(s/def :cognitect.aws.iotevents/UntagResourceResponse (s/keys))
(s/def
:cognitect.aws.iotevents/MQTTTopic
(s/spec
(s/and string? #(<= 1 (count %) 128))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 128) #(gen/vector (gen/char-alpha) %))))))
(s/def :cognitect.aws.iotevents/InputSummaries (s/coll-of :cognitect.aws.iotevents/InputSummary))
(s/def
:cognitect.aws.iotevents/DetectorModelVersion
(s/spec
(s/and string? #(<= 1 (count %) 128))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 128) #(gen/vector (gen/char-alpha) %))))))
(s/def :cognitect.aws.iotevents/InputArn string?)
(s/def
:cognitect.aws.iotevents/DetectorModelVersionSummary
(s/keys
:opt-un
[:cognitect.aws.iotevents.DetectorModelVersionSummary/evaluationMethod
:cognitect.aws.iotevents.DetectorModelVersionSummary/roleArn
:cognitect.aws.iotevents.DetectorModelVersionSummary/detectorModelArn
:cognitect.aws.iotevents.DetectorModelVersionSummary/status
:cognitect.aws.iotevents.DetectorModelVersionSummary/creationTime
:cognitect.aws.iotevents.DetectorModelVersionSummary/lastUpdateTime
:cognitect.aws.iotevents.DetectorModelVersionSummary/detectorModelVersion
:cognitect.aws.iotevents.DetectorModelVersionSummary/detectorModelName]))
(s/def :cognitect.aws.iotevents/States (s/coll-of :cognitect.aws.iotevents/State :min-count 1))
(s/def
:cognitect.aws.iotevents/UpdateInputRequest
(s/keys
:req-un
[:cognitect.aws.iotevents.UpdateInputRequest/inputName
:cognitect.aws.iotevents.UpdateInputRequest/inputDefinition]
:opt-un
[:cognitect.aws.iotevents.UpdateInputRequest/inputDescription]))
(s/def :cognitect.aws.iotevents/QueueUrl string?)
(s/def
:cognitect.aws.iotevents/ListDetectorModelVersionsResponse
(s/keys
:opt-un
[:cognitect.aws.iotevents.ListDetectorModelVersionsResponse/detectorModelVersionSummaries
:cognitect.aws.iotevents.ListDetectorModelVersionsResponse/nextToken]))
(s/def
:cognitect.aws.iotevents/UpdateDetectorModelRequest
(s/keys
:req-un
[:cognitect.aws.iotevents.UpdateDetectorModelRequest/detectorModelName
:cognitect.aws.iotevents.UpdateDetectorModelRequest/detectorModelDefinition
:cognitect.aws.iotevents.UpdateDetectorModelRequest/roleArn]
:opt-un
[:cognitect.aws.iotevents.UpdateDetectorModelRequest/evaluationMethod
:cognitect.aws.iotevents.UpdateDetectorModelRequest/detectorModelDescription]))
(s/def
:cognitect.aws.iotevents/DescribeDetectorModelResponse
(s/keys :opt-un [:cognitect.aws.iotevents.DescribeDetectorModelResponse/detectorModel]))
(s/def :cognitect.aws.iotevents/DeleteInputResponse (s/keys))
(s/def
:cognitect.aws.iotevents/TransitionEvent
(s/keys
:req-un
[:cognitect.aws.iotevents.TransitionEvent/eventName
:cognitect.aws.iotevents.TransitionEvent/condition
:cognitect.aws.iotevents.TransitionEvent/nextState]
:opt-un
[:cognitect.aws.iotevents.TransitionEvent/actions]))
(s/def
:cognitect.aws.iotevents/Action
(s/keys
:opt-un
[:cognitect.aws.iotevents.Action/sns
:cognitect.aws.iotevents.Action/iotTopicPublish
:cognitect.aws.iotevents.Action/clearTimer
:cognitect.aws.iotevents.Action/resetTimer
:cognitect.aws.iotevents.Action/setVariable
:cognitect.aws.iotevents.Action/sqs
:cognitect.aws.iotevents.Action/firehose
:cognitect.aws.iotevents.Action/setTimer
:cognitect.aws.iotevents.Action/lambda
:cognitect.aws.iotevents.Action/iotEvents]))
(s/def
:cognitect.aws.iotevents/DetectorModelConfiguration
(s/keys
:opt-un
[:cognitect.aws.iotevents.DetectorModelConfiguration/evaluationMethod
:cognitect.aws.iotevents.DetectorModelConfiguration/key
:cognitect.aws.iotevents.DetectorModelConfiguration/roleArn
:cognitect.aws.iotevents.DetectorModelConfiguration/detectorModelArn
:cognitect.aws.iotevents.DetectorModelConfiguration/status
:cognitect.aws.iotevents.DetectorModelConfiguration/detectorModelDescription
:cognitect.aws.iotevents.DetectorModelConfiguration/creationTime
:cognitect.aws.iotevents.DetectorModelConfiguration/lastUpdateTime
:cognitect.aws.iotevents.DetectorModelConfiguration/detectorModelVersion
:cognitect.aws.iotevents.DetectorModelConfiguration/detectorModelName]))
(s/def
:cognitect.aws.iotevents/DeleteInputRequest
(s/keys :req-un [:cognitect.aws.iotevents.DeleteInputRequest/inputName]))
(s/def
:cognitect.aws.iotevents/LambdaAction
(s/keys :req-un [:cognitect.aws.iotevents.LambdaAction/functionArn]))
(s/def
:cognitect.aws.iotevents/ListInputsResponse
(s/keys
:opt-un
[:cognitect.aws.iotevents.ListInputsResponse/nextToken
:cognitect.aws.iotevents.ListInputsResponse/inputSummaries]))
(s/def
:cognitect.aws.iotevents/CreateDetectorModelRequest
(s/keys
:req-un
[:cognitect.aws.iotevents.CreateDetectorModelRequest/detectorModelName
:cognitect.aws.iotevents.CreateDetectorModelRequest/detectorModelDefinition
:cognitect.aws.iotevents.CreateDetectorModelRequest/roleArn]
:opt-un
[:cognitect.aws.iotevents.CreateDetectorModelRequest/evaluationMethod
:cognitect.aws.iotevents.CreateDetectorModelRequest/key
:cognitect.aws.iotevents.CreateDetectorModelRequest/tags
:cognitect.aws.iotevents.CreateDetectorModelRequest/detectorModelDescription]))
(s/def
:cognitect.aws.iotevents/CreateDetectorModelResponse
(s/keys
:opt-un
[:cognitect.aws.iotevents.CreateDetectorModelResponse/detectorModelConfiguration]))
(s/def
:cognitect.aws.iotevents/PutLoggingOptionsRequest
(s/keys :req-un [:cognitect.aws.iotevents.PutLoggingOptionsRequest/loggingOptions]))
(s/def
:cognitect.aws.iotevents/MaxResults
(s/spec (s/and int? #(<= 1 % 250)) :gen #(gen/choose 1 250)))
(s/def
:cognitect.aws.iotevents/UntagResourceRequest
(s/keys
:req-un
[:cognitect.aws.iotevents.UntagResourceRequest/resourceArn
:cognitect.aws.iotevents.UntagResourceRequest/tagKeys]))
(s/def
:cognitect.aws.iotevents/TagKey
(s/spec
(s/and string? #(<= 1 (count %) 128))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 128) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.iotevents/FirehoseAction
(s/keys
:req-un
[:cognitect.aws.iotevents.FirehoseAction/deliveryStreamName]
:opt-un
[:cognitect.aws.iotevents.FirehoseAction/separator]))
(s/def
:cognitect.aws.iotevents/DetectorModelDefinition
(s/keys
:req-un
[:cognitect.aws.iotevents.DetectorModelDefinition/states
:cognitect.aws.iotevents.DetectorModelDefinition/initialStateName]))
(s/def
:cognitect.aws.iotevents/ListDetectorModelsResponse
(s/keys
:opt-un
[:cognitect.aws.iotevents.ListDetectorModelsResponse/detectorModelSummaries
:cognitect.aws.iotevents.ListDetectorModelsResponse/nextToken]))
(s/def :cognitect.aws.iotevents/Timestamp inst?)
(s/def
:cognitect.aws.iotevents/State
(s/keys
:req-un
[:cognitect.aws.iotevents.State/stateName]
:opt-un
[:cognitect.aws.iotevents.State/onInput
:cognitect.aws.iotevents.State/onExit
:cognitect.aws.iotevents.State/onEnter]))
(s/def
:cognitect.aws.iotevents/DetectorModelName
(s/spec #(re-matches (re-pattern "^[a-zA-Z0-9_-]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.iotevents/ClearTimerAction
(s/keys :req-un [:cognitect.aws.iotevents.ClearTimerAction/timerName]))
(s/def
:cognitect.aws.iotevents/Attributes
(s/coll-of :cognitect.aws.iotevents/Attribute :min-count 1 :max-count 200))
(s/def
:cognitect.aws.iotevents/Condition
(s/spec
(s/and string? #(>= 512 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 512) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.iotevents/DescribeInputRequest
(s/keys :req-un [:cognitect.aws.iotevents.DescribeInputRequest/inputName]))
(s/def
:cognitect.aws.iotevents/Attribute
(s/keys :req-un [:cognitect.aws.iotevents.Attribute/jsonPath]))
(s/def :cognitect.aws.iotevents/TagKeys (s/coll-of :cognitect.aws.iotevents/TagKey))
(s/def :cognitect.aws.iotevents/DeleteDetectorModelResponse (s/keys))
(s/def
:cognitect.aws.iotevents/InputConfiguration
(s/keys
:req-un
[:cognitect.aws.iotevents.InputConfiguration/inputName
:cognitect.aws.iotevents.InputConfiguration/inputArn
:cognitect.aws.iotevents.InputConfiguration/creationTime
:cognitect.aws.iotevents.InputConfiguration/lastUpdateTime
:cognitect.aws.iotevents.InputConfiguration/status]
:opt-un
[:cognitect.aws.iotevents.InputConfiguration/inputDescription]))
(s/def
:cognitect.aws.iotevents/ListInputsRequest
(s/keys
:opt-un
[:cognitect.aws.iotevents.ListInputsRequest/maxResults
:cognitect.aws.iotevents.ListInputsRequest/nextToken]))
(s/def :cognitect.aws.iotevents/DetectorModelArn string?)
(s/def
:cognitect.aws.iotevents/Seconds
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.iotevents/ListTagsForResourceResponse
(s/keys :opt-un [:cognitect.aws.iotevents.ListTagsForResourceResponse/tags]))
(s/def
:cognitect.aws.iotevents/VariableName
(s/spec #(re-matches (re-pattern "^[a-zA-Z][a-zA-Z0-9_]*$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.iotevents/DescribeDetectorModelRequest
(s/keys
:req-un
[:cognitect.aws.iotevents.DescribeDetectorModelRequest/detectorModelName]
:opt-un
[:cognitect.aws.iotevents.DescribeDetectorModelRequest/detectorModelVersion]))
(s/def
:cognitect.aws.iotevents/ListDetectorModelVersionsRequest
(s/keys
:req-un
[:cognitect.aws.iotevents.ListDetectorModelVersionsRequest/detectorModelName]
:opt-un
[:cognitect.aws.iotevents.ListDetectorModelVersionsRequest/maxResults
:cognitect.aws.iotevents.ListDetectorModelVersionsRequest/nextToken]))
(s/def
:cognitect.aws.iotevents/TimerName
(s/spec
(s/and string? #(<= 1 (count %) 128))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 1 128) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.iotevents/CreateInputResponse
(s/keys :opt-un [:cognitect.aws.iotevents.CreateInputResponse/inputConfiguration]))
(s/def
:cognitect.aws.iotevents/SqsAction
(s/keys
:req-un
[:cognitect.aws.iotevents.SqsAction/queueUrl]
:opt-un
[:cognitect.aws.iotevents.SqsAction/useBase64]))
(s/def
:cognitect.aws.iotevents/FirehoseSeparator
(s/spec #(re-matches (re-pattern "([\\n\\t])|(\\r\\n)|(,)") %) :gen #(gen/string)))
(s/def :cognitect.aws.iotevents.SetTimerAction/timerName :cognitect.aws.iotevents/TimerName)
(s/def :cognitect.aws.iotevents.SetTimerAction/seconds :cognitect.aws.iotevents/Seconds)
(s/def
:cognitect.aws.iotevents.DescribeLoggingOptionsResponse/loggingOptions
:cognitect.aws.iotevents/LoggingOptions)
(s/def
:cognitect.aws.iotevents.TagResourceRequest/resourceArn
:cognitect.aws.iotevents/AmazonResourceName)
(s/def :cognitect.aws.iotevents.TagResourceRequest/tags :cognitect.aws.iotevents/Tags)
(s/def :cognitect.aws.iotevents.InputSummary/inputName :cognitect.aws.iotevents/InputName)
(s/def
:cognitect.aws.iotevents.InputSummary/inputDescription
:cognitect.aws.iotevents/InputDescription)
(s/def :cognitect.aws.iotevents.InputSummary/inputArn :cognitect.aws.iotevents/InputArn)
(s/def :cognitect.aws.iotevents.InputSummary/creationTime :cognitect.aws.iotevents/Timestamp)
(s/def :cognitect.aws.iotevents.InputSummary/lastUpdateTime :cognitect.aws.iotevents/Timestamp)
(s/def :cognitect.aws.iotevents.InputSummary/status :cognitect.aws.iotevents/InputStatus)
(s/def :cognitect.aws.iotevents.OnInputLifecycle/events :cognitect.aws.iotevents/Events)
(s/def
:cognitect.aws.iotevents.OnInputLifecycle/transitionEvents
:cognitect.aws.iotevents/TransitionEvents)
(s/def
:cognitect.aws.iotevents.DetectorModel/detectorModelDefinition
:cognitect.aws.iotevents/DetectorModelDefinition)
(s/def
:cognitect.aws.iotevents.DetectorModel/detectorModelConfiguration
:cognitect.aws.iotevents/DetectorModelConfiguration)
(s/def :cognitect.aws.iotevents.Tag/key :cognitect.aws.iotevents/TagKey)
(s/def :cognitect.aws.iotevents.Tag/value :cognitect.aws.iotevents/TagValue)
(s/def
:cognitect.aws.iotevents.DetectorDebugOption/detectorModelName
:cognitect.aws.iotevents/DetectorModelName)
(s/def :cognitect.aws.iotevents.DetectorDebugOption/keyValue :cognitect.aws.iotevents/KeyValue)
(s/def :cognitect.aws.iotevents.InputDefinition/attributes :cognitect.aws.iotevents/Attributes)
(s/def :cognitect.aws.iotevents.OnExitLifecycle/events :cognitect.aws.iotevents/Events)
(s/def
:cognitect.aws.iotevents.UpdateInputResponse/inputConfiguration
:cognitect.aws.iotevents/InputConfiguration)
(s/def :cognitect.aws.iotevents.ResetTimerAction/timerName :cognitect.aws.iotevents/TimerName)
(s/def :cognitect.aws.iotevents.LoggingOptions/roleArn :cognitect.aws.iotevents/AmazonResourceName)
(s/def :cognitect.aws.iotevents.LoggingOptions/level :cognitect.aws.iotevents/LoggingLevel)
(s/def :cognitect.aws.iotevents.LoggingOptions/enabled :cognitect.aws.iotevents/LoggingEnabled)
(s/def
:cognitect.aws.iotevents.LoggingOptions/detectorDebugOptions
:cognitect.aws.iotevents/DetectorDebugOptions)
(s/def
:cognitect.aws.iotevents.ListDetectorModelsRequest/nextToken
:cognitect.aws.iotevents/NextToken)
(s/def
:cognitect.aws.iotevents.ListDetectorModelsRequest/maxResults
:cognitect.aws.iotevents/MaxResults)
(s/def
:cognitect.aws.iotevents.DetectorModelSummary/detectorModelName
:cognitect.aws.iotevents/DetectorModelName)
(s/def
:cognitect.aws.iotevents.DetectorModelSummary/detectorModelDescription
:cognitect.aws.iotevents/DetectorModelDescription)
(s/def
:cognitect.aws.iotevents.DetectorModelSummary/creationTime
:cognitect.aws.iotevents/Timestamp)
(s/def
:cognitect.aws.iotevents.Input/inputConfiguration
:cognitect.aws.iotevents/InputConfiguration)
(s/def :cognitect.aws.iotevents.Input/inputDefinition :cognitect.aws.iotevents/InputDefinition)
(s/def
:cognitect.aws.iotevents.SetVariableAction/variableName
:cognitect.aws.iotevents/VariableName)
(s/def :cognitect.aws.iotevents.SetVariableAction/value :cognitect.aws.iotevents/VariableValue)
(s/def :cognitect.aws.iotevents.OnEnterLifecycle/events :cognitect.aws.iotevents/Events)
(s/def :cognitect.aws.iotevents.CreateInputRequest/inputName :cognitect.aws.iotevents/InputName)
(s/def
:cognitect.aws.iotevents.CreateInputRequest/inputDescription
:cognitect.aws.iotevents/InputDescription)
(s/def
:cognitect.aws.iotevents.CreateInputRequest/inputDefinition
:cognitect.aws.iotevents/InputDefinition)
(s/def :cognitect.aws.iotevents.CreateInputRequest/tags :cognitect.aws.iotevents/Tags)
(s/def :cognitect.aws.iotevents.Event/eventName :cognitect.aws.iotevents/EventName)
(s/def :cognitect.aws.iotevents.Event/condition :cognitect.aws.iotevents/Condition)
(s/def :cognitect.aws.iotevents.Event/actions :cognitect.aws.iotevents/Actions)
(s/def :cognitect.aws.iotevents.IotTopicPublishAction/mqttTopic :cognitect.aws.iotevents/MQTTTopic)
(s/def
:cognitect.aws.iotevents.UpdateDetectorModelResponse/detectorModelConfiguration
:cognitect.aws.iotevents/DetectorModelConfiguration)
(s/def :cognitect.aws.iotevents.DescribeInputResponse/input :cognitect.aws.iotevents/Input)
(s/def
:cognitect.aws.iotevents.SNSTopicPublishAction/targetArn
:cognitect.aws.iotevents/AmazonResourceName)
(s/def
:cognitect.aws.iotevents.DeleteDetectorModelRequest/detectorModelName
:cognitect.aws.iotevents/DetectorModelName)
(s/def :cognitect.aws.iotevents.IotEventsAction/inputName :cognitect.aws.iotevents/InputName)
(s/def
:cognitect.aws.iotevents.ListTagsForResourceRequest/resourceArn
:cognitect.aws.iotevents/AmazonResourceName)
(s/def
:cognitect.aws.iotevents.DetectorModelVersionSummary/detectorModelName
:cognitect.aws.iotevents/DetectorModelName)
(s/def
:cognitect.aws.iotevents.DetectorModelVersionSummary/detectorModelVersion
:cognitect.aws.iotevents/DetectorModelVersion)
(s/def
:cognitect.aws.iotevents.DetectorModelVersionSummary/detectorModelArn
:cognitect.aws.iotevents/DetectorModelArn)
(s/def
:cognitect.aws.iotevents.DetectorModelVersionSummary/roleArn
:cognitect.aws.iotevents/AmazonResourceName)
(s/def
:cognitect.aws.iotevents.DetectorModelVersionSummary/creationTime
:cognitect.aws.iotevents/Timestamp)
(s/def
:cognitect.aws.iotevents.DetectorModelVersionSummary/lastUpdateTime
:cognitect.aws.iotevents/Timestamp)
(s/def
:cognitect.aws.iotevents.DetectorModelVersionSummary/status
:cognitect.aws.iotevents/DetectorModelVersionStatus)
(s/def
:cognitect.aws.iotevents.DetectorModelVersionSummary/evaluationMethod
:cognitect.aws.iotevents/EvaluationMethod)
(s/def :cognitect.aws.iotevents.UpdateInputRequest/inputName :cognitect.aws.iotevents/InputName)
(s/def
:cognitect.aws.iotevents.UpdateInputRequest/inputDescription
:cognitect.aws.iotevents/InputDescription)
(s/def
:cognitect.aws.iotevents.UpdateInputRequest/inputDefinition
:cognitect.aws.iotevents/InputDefinition)
(s/def
:cognitect.aws.iotevents.ListDetectorModelVersionsResponse/detectorModelVersionSummaries
:cognitect.aws.iotevents/DetectorModelVersionSummaries)
(s/def
:cognitect.aws.iotevents.ListDetectorModelVersionsResponse/nextToken
:cognitect.aws.iotevents/NextToken)
(s/def
:cognitect.aws.iotevents.UpdateDetectorModelRequest/detectorModelName
:cognitect.aws.iotevents/DetectorModelName)
(s/def
:cognitect.aws.iotevents.UpdateDetectorModelRequest/detectorModelDefinition
:cognitect.aws.iotevents/DetectorModelDefinition)
(s/def
:cognitect.aws.iotevents.UpdateDetectorModelRequest/detectorModelDescription
:cognitect.aws.iotevents/DetectorModelDescription)
(s/def
:cognitect.aws.iotevents.UpdateDetectorModelRequest/roleArn
:cognitect.aws.iotevents/AmazonResourceName)
(s/def
:cognitect.aws.iotevents.UpdateDetectorModelRequest/evaluationMethod
:cognitect.aws.iotevents/EvaluationMethod)
(s/def
:cognitect.aws.iotevents.DescribeDetectorModelResponse/detectorModel
:cognitect.aws.iotevents/DetectorModel)
(s/def :cognitect.aws.iotevents.TransitionEvent/eventName :cognitect.aws.iotevents/EventName)
(s/def :cognitect.aws.iotevents.TransitionEvent/condition :cognitect.aws.iotevents/Condition)
(s/def :cognitect.aws.iotevents.TransitionEvent/actions :cognitect.aws.iotevents/Actions)
(s/def :cognitect.aws.iotevents.TransitionEvent/nextState :cognitect.aws.iotevents/StateName)
(s/def :cognitect.aws.iotevents.Action/sns :cognitect.aws.iotevents/SNSTopicPublishAction)
(s/def :cognitect.aws.iotevents.Action/lambda :cognitect.aws.iotevents/LambdaAction)
(s/def :cognitect.aws.iotevents.Action/sqs :cognitect.aws.iotevents/SqsAction)
(s/def :cognitect.aws.iotevents.Action/resetTimer :cognitect.aws.iotevents/ResetTimerAction)
(s/def :cognitect.aws.iotevents.Action/clearTimer :cognitect.aws.iotevents/ClearTimerAction)
(s/def :cognitect.aws.iotevents.Action/iotEvents :cognitect.aws.iotevents/IotEventsAction)
(s/def :cognitect.aws.iotevents.Action/setVariable :cognitect.aws.iotevents/SetVariableAction)
(s/def :cognitect.aws.iotevents.Action/firehose :cognitect.aws.iotevents/FirehoseAction)
(s/def
:cognitect.aws.iotevents.Action/iotTopicPublish
:cognitect.aws.iotevents/IotTopicPublishAction)
(s/def :cognitect.aws.iotevents.Action/setTimer :cognitect.aws.iotevents/SetTimerAction)
(s/def
:cognitect.aws.iotevents.DetectorModelConfiguration/detectorModelName
:cognitect.aws.iotevents/DetectorModelName)
(s/def
:cognitect.aws.iotevents.DetectorModelConfiguration/creationTime
:cognitect.aws.iotevents/Timestamp)
(s/def
:cognitect.aws.iotevents.DetectorModelConfiguration/detectorModelArn
:cognitect.aws.iotevents/DetectorModelArn)
(s/def
:cognitect.aws.iotevents.DetectorModelConfiguration/key
:cognitect.aws.iotevents/AttributeJsonPath)
(s/def
:cognitect.aws.iotevents.DetectorModelConfiguration/detectorModelDescription
:cognitect.aws.iotevents/DetectorModelDescription)
(s/def
:cognitect.aws.iotevents.DetectorModelConfiguration/status
:cognitect.aws.iotevents/DetectorModelVersionStatus)
(s/def
:cognitect.aws.iotevents.DetectorModelConfiguration/lastUpdateTime
:cognitect.aws.iotevents/Timestamp)
(s/def
:cognitect.aws.iotevents.DetectorModelConfiguration/evaluationMethod
:cognitect.aws.iotevents/EvaluationMethod)
(s/def
:cognitect.aws.iotevents.DetectorModelConfiguration/detectorModelVersion
:cognitect.aws.iotevents/DetectorModelVersion)
(s/def
:cognitect.aws.iotevents.DetectorModelConfiguration/roleArn
:cognitect.aws.iotevents/AmazonResourceName)
(s/def :cognitect.aws.iotevents.DeleteInputRequest/inputName :cognitect.aws.iotevents/InputName)
(s/def
:cognitect.aws.iotevents.LambdaAction/functionArn
:cognitect.aws.iotevents/AmazonResourceName)
(s/def
:cognitect.aws.iotevents.ListInputsResponse/inputSummaries
:cognitect.aws.iotevents/InputSummaries)
(s/def :cognitect.aws.iotevents.ListInputsResponse/nextToken :cognitect.aws.iotevents/NextToken)
(s/def
:cognitect.aws.iotevents.CreateDetectorModelRequest/detectorModelName
:cognitect.aws.iotevents/DetectorModelName)
(s/def
:cognitect.aws.iotevents.CreateDetectorModelRequest/detectorModelDefinition
:cognitect.aws.iotevents/DetectorModelDefinition)
(s/def
:cognitect.aws.iotevents.CreateDetectorModelRequest/detectorModelDescription
:cognitect.aws.iotevents/DetectorModelDescription)
(s/def
:cognitect.aws.iotevents.CreateDetectorModelRequest/key
:cognitect.aws.iotevents/AttributeJsonPath)
(s/def
:cognitect.aws.iotevents.CreateDetectorModelRequest/roleArn
:cognitect.aws.iotevents/AmazonResourceName)
(s/def :cognitect.aws.iotevents.CreateDetectorModelRequest/tags :cognitect.aws.iotevents/Tags)
(s/def
:cognitect.aws.iotevents.CreateDetectorModelRequest/evaluationMethod
:cognitect.aws.iotevents/EvaluationMethod)
(s/def
:cognitect.aws.iotevents.CreateDetectorModelResponse/detectorModelConfiguration
:cognitect.aws.iotevents/DetectorModelConfiguration)
(s/def
:cognitect.aws.iotevents.PutLoggingOptionsRequest/loggingOptions
:cognitect.aws.iotevents/LoggingOptions)
(s/def
:cognitect.aws.iotevents.UntagResourceRequest/resourceArn
:cognitect.aws.iotevents/AmazonResourceName)
(s/def :cognitect.aws.iotevents.UntagResourceRequest/tagKeys :cognitect.aws.iotevents/TagKeys)
(s/def
:cognitect.aws.iotevents.FirehoseAction/deliveryStreamName
:cognitect.aws.iotevents/DeliveryStreamName)
(s/def :cognitect.aws.iotevents.FirehoseAction/separator :cognitect.aws.iotevents/FirehoseSeparator)
(s/def :cognitect.aws.iotevents.DetectorModelDefinition/states :cognitect.aws.iotevents/States)
(s/def
:cognitect.aws.iotevents.DetectorModelDefinition/initialStateName
:cognitect.aws.iotevents/StateName)
(s/def
:cognitect.aws.iotevents.ListDetectorModelsResponse/detectorModelSummaries
:cognitect.aws.iotevents/DetectorModelSummaries)
(s/def
:cognitect.aws.iotevents.ListDetectorModelsResponse/nextToken
:cognitect.aws.iotevents/NextToken)
(s/def :cognitect.aws.iotevents.State/stateName :cognitect.aws.iotevents/StateName)
(s/def :cognitect.aws.iotevents.State/onInput :cognitect.aws.iotevents/OnInputLifecycle)
(s/def :cognitect.aws.iotevents.State/onEnter :cognitect.aws.iotevents/OnEnterLifecycle)
(s/def :cognitect.aws.iotevents.State/onExit :cognitect.aws.iotevents/OnExitLifecycle)
(s/def :cognitect.aws.iotevents.ClearTimerAction/timerName :cognitect.aws.iotevents/TimerName)
(s/def :cognitect.aws.iotevents.DescribeInputRequest/inputName :cognitect.aws.iotevents/InputName)
(s/def :cognitect.aws.iotevents.Attribute/jsonPath :cognitect.aws.iotevents/AttributeJsonPath)
(s/def :cognitect.aws.iotevents.InputConfiguration/inputName :cognitect.aws.iotevents/InputName)
(s/def
:cognitect.aws.iotevents.InputConfiguration/inputDescription
:cognitect.aws.iotevents/InputDescription)
(s/def :cognitect.aws.iotevents.InputConfiguration/inputArn :cognitect.aws.iotevents/InputArn)
(s/def :cognitect.aws.iotevents.InputConfiguration/creationTime :cognitect.aws.iotevents/Timestamp)
(s/def
:cognitect.aws.iotevents.InputConfiguration/lastUpdateTime
:cognitect.aws.iotevents/Timestamp)
(s/def :cognitect.aws.iotevents.InputConfiguration/status :cognitect.aws.iotevents/InputStatus)
(s/def :cognitect.aws.iotevents.ListInputsRequest/nextToken :cognitect.aws.iotevents/NextToken)
(s/def :cognitect.aws.iotevents.ListInputsRequest/maxResults :cognitect.aws.iotevents/MaxResults)
(s/def :cognitect.aws.iotevents.ListTagsForResourceResponse/tags :cognitect.aws.iotevents/Tags)
(s/def
:cognitect.aws.iotevents.DescribeDetectorModelRequest/detectorModelName
:cognitect.aws.iotevents/DetectorModelName)
(s/def
:cognitect.aws.iotevents.DescribeDetectorModelRequest/detectorModelVersion
:cognitect.aws.iotevents/DetectorModelVersion)
(s/def
:cognitect.aws.iotevents.ListDetectorModelVersionsRequest/detectorModelName
:cognitect.aws.iotevents/DetectorModelName)
(s/def
:cognitect.aws.iotevents.ListDetectorModelVersionsRequest/nextToken
:cognitect.aws.iotevents/NextToken)
(s/def
:cognitect.aws.iotevents.ListDetectorModelVersionsRequest/maxResults
:cognitect.aws.iotevents/MaxResults)
(s/def
:cognitect.aws.iotevents.CreateInputResponse/inputConfiguration
:cognitect.aws.iotevents/InputConfiguration)
(s/def :cognitect.aws.iotevents.SqsAction/queueUrl :cognitect.aws.iotevents/QueueUrl)
(s/def :cognitect.aws.iotevents.SqsAction/useBase64 :cognitect.aws.iotevents/UseBase64)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy