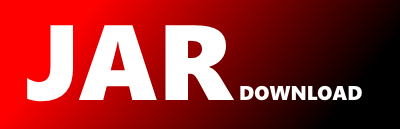
cognitect.aws.outposts.specs.clj Maven / Gradle / Ivy
The newest version!
;; Copyright (c) Cognitect, Inc.
;; All rights reserved.
(ns cognitect.aws.outposts.specs
(:require [clojure.spec.alpha :as s] [clojure.spec.gen.alpha :as gen]))
(s/def :cognitect.aws/client map?)
(s/def :core.async/channel any?)
(s/def
:cognitect.aws.outposts/OutpostIdOnly
(s/spec #(re-matches (re-pattern "^op-[a-f0-9]{17}$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/CreateSiteInput
(s/keys
:req-un
[:cognitect.aws.outposts.CreateSiteInput/Name]
:opt-un
[:cognitect.aws.outposts.CreateSiteInput/ShippingAddress
:cognitect.aws.outposts.CreateSiteInput/Tags
:cognitect.aws.outposts.CreateSiteInput/Description
:cognitect.aws.outposts.CreateSiteInput/OperatingAddress
:cognitect.aws.outposts.CreateSiteInput/RackPhysicalProperties
:cognitect.aws.outposts.CreateSiteInput/Notes]))
(s/def
:cognitect.aws.outposts/AvailabilityZone
(s/spec
#(re-matches (re-pattern "^([a-zA-Z]+-){1,3}([a-zA-Z]+)?(\\d+[a-zA-Z]?)?$") %)
:gen
#(gen/string)))
(s/def
:cognitect.aws.outposts/AssetInstance
(s/keys
:opt-un
[:cognitect.aws.outposts.AssetInstance/InstanceId
:cognitect.aws.outposts.AssetInstance/InstanceType
:cognitect.aws.outposts.AssetInstance/AccountId
:cognitect.aws.outposts.AssetInstance/AwsServiceName
:cognitect.aws.outposts.AssetInstance/AssetId]))
(s/def :cognitect.aws.outposts/AssetInstanceList (s/coll-of :cognitect.aws.outposts/AssetInstance))
(s/def
:cognitect.aws.outposts/CatalogItem
(s/keys
:opt-un
[:cognitect.aws.outposts.CatalogItem/PowerKva
:cognitect.aws.outposts.CatalogItem/SupportedStorage
:cognitect.aws.outposts.CatalogItem/ItemStatus
:cognitect.aws.outposts.CatalogItem/EC2Capacities
:cognitect.aws.outposts.CatalogItem/WeightLbs
:cognitect.aws.outposts.CatalogItem/SupportedUplinkGbps
:cognitect.aws.outposts.CatalogItem/CatalogItemId]))
(s/def
:cognitect.aws.outposts/TagResourceRequest
(s/keys
:req-un
[:cognitect.aws.outposts.TagResourceRequest/ResourceArn
:cognitect.aws.outposts.TagResourceRequest/Tags]))
(s/def
:cognitect.aws.outposts/GetOutpostInstanceTypesOutput
(s/keys
:opt-un
[:cognitect.aws.outposts.GetOutpostInstanceTypesOutput/InstanceTypes
:cognitect.aws.outposts.GetOutpostInstanceTypesOutput/OutpostId
:cognitect.aws.outposts.GetOutpostInstanceTypesOutput/NextToken
:cognitect.aws.outposts.GetOutpostInstanceTypesOutput/OutpostArn]))
(s/def
:cognitect.aws.outposts/ServiceQuotaExceededException
(s/keys :opt-un [:cognitect.aws.outposts.ServiceQuotaExceededException/Message]))
(s/def
:cognitect.aws.outposts/InternalServerException
(s/keys :opt-un [:cognitect.aws.outposts.InternalServerException/Message]))
(s/def :cognitect.aws.outposts/ResourceType (s/spec string? :gen #(s/gen #{"ORDER" "OUTPOST"})))
(s/def
:cognitect.aws.outposts/BlockingInstance
(s/keys
:opt-un
[:cognitect.aws.outposts.BlockingInstance/InstanceId
:cognitect.aws.outposts.BlockingInstance/AccountId
:cognitect.aws.outposts.BlockingInstance/AwsServiceName]))
(s/def
:cognitect.aws.outposts/RackId
(s/spec #(re-matches (re-pattern "^[\\S \\n]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/LifeCycleStatusList
(s/coll-of :cognitect.aws.outposts/LifeCycleStatus :min-count 1 :max-count 5))
(s/def
:cognitect.aws.outposts/AssetId
(s/spec #(re-matches (re-pattern "^(\\w+)$") %) :gen #(gen/string)))
(s/def :cognitect.aws.outposts/siteListDefinition (s/coll-of :cognitect.aws.outposts/Site))
(s/def
:cognitect.aws.outposts/LineItemAssetInformationList
(s/coll-of :cognitect.aws.outposts/LineItemAssetInformation))
(s/def
:cognitect.aws.outposts/ListCapacityTasksInput
(s/keys
:opt-un
[:cognitect.aws.outposts.ListCapacityTasksInput/OutpostIdentifierFilter
:cognitect.aws.outposts.ListCapacityTasksInput/NextToken
:cognitect.aws.outposts.ListCapacityTasksInput/CapacityTaskStatusFilter
:cognitect.aws.outposts.ListCapacityTasksInput/MaxResults]))
(s/def
:cognitect.aws.outposts/CatalogItemWeightLbs
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.outposts/AssetInstanceCapacityList
(s/coll-of :cognitect.aws.outposts/AssetInstanceTypeCapacity))
(s/def
:cognitect.aws.outposts/GetSiteInput
(s/keys :req-un [:cognitect.aws.outposts.GetSiteInput/SiteId]))
(s/def :cognitect.aws.outposts/outpostListDefinition (s/coll-of :cognitect.aws.outposts/Outpost))
(s/def
:cognitect.aws.outposts/AddressLine3
(s/spec #(re-matches (re-pattern "^\\S[\\S ]*$") %) :gen #(gen/string)))
(s/def :cognitect.aws.outposts/CancelCapacityTaskOutput (s/keys))
(s/def
:cognitect.aws.outposts/SiteArn
(s/spec
#(re-matches
(re-pattern "^arn:aws([a-z-]+)?:outposts:[a-z\\d-]+:\\d{12}:site/(os-[a-f0-9]{17})$")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.outposts/Municipality
(s/spec #(re-matches (re-pattern "^\\S[\\S ]*$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/GetCatalogItemOutput
(s/keys :opt-un [:cognitect.aws.outposts.GetCatalogItemOutput/CatalogItem]))
(s/def
:cognitect.aws.outposts/TagMap
(s/map-of
:cognitect.aws.outposts/TagKey
:cognitect.aws.outposts/TagValue
:min-count
1
:max-count
50))
(s/def
:cognitect.aws.outposts/CatalogItemPowerKva
(s/spec double? :gen #(gen/double* {:infinite? false, :NaN? false})))
(s/def :cognitect.aws.outposts/CatalogItemClass (s/spec string? :gen #(s/gen #{"SERVER" "RACK"})))
(s/def
:cognitect.aws.outposts/DistrictOrCounty
(s/spec #(re-matches (re-pattern "^\\S[\\S ]*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/CreateOutpostInput
(s/keys
:req-un
[:cognitect.aws.outposts.CreateOutpostInput/Name
:cognitect.aws.outposts.CreateOutpostInput/SiteId]
:opt-un
[:cognitect.aws.outposts.CreateOutpostInput/SupportedHardwareType
:cognitect.aws.outposts.CreateOutpostInput/Tags
:cognitect.aws.outposts.CreateOutpostInput/AvailabilityZone
:cognitect.aws.outposts.CreateOutpostInput/Description
:cognitect.aws.outposts.CreateOutpostInput/AvailabilityZoneId]))
(s/def :cognitect.aws.outposts/ISO8601Timestamp inst?)
(s/def
:cognitect.aws.outposts/PowerDrawKva
(s/spec string? :gen #(s/gen #{"POWER_15_KVA" "POWER_10_KVA" "POWER_5_KVA" "POWER_30_KVA"})))
(s/def
:cognitect.aws.outposts/AssetLocation
(s/keys :opt-un [:cognitect.aws.outposts.AssetLocation/RackElevation]))
(s/def
:cognitect.aws.outposts/PowerConnector
(s/spec string? :gen #(s/gen #{"L6_30P" "IEC309" "AH532P6W" "CS8365C" "AH530P7W"})))
(s/def :cognitect.aws.outposts/TagResourceResponse (s/keys))
(s/def :cognitect.aws.outposts/CIDRList (s/coll-of :cognitect.aws.outposts/CIDR))
(s/def
:cognitect.aws.outposts/UpdateSiteInput
(s/keys
:req-un
[:cognitect.aws.outposts.UpdateSiteInput/SiteId]
:opt-un
[:cognitect.aws.outposts.UpdateSiteInput/Description
:cognitect.aws.outposts.UpdateSiteInput/Name
:cognitect.aws.outposts.UpdateSiteInput/Notes]))
(s/def
:cognitect.aws.outposts/GetOutpostInstanceTypesInput
(s/keys
:req-un
[:cognitect.aws.outposts.GetOutpostInstanceTypesInput/OutpostId]
:opt-un
[:cognitect.aws.outposts.GetOutpostInstanceTypesInput/NextToken
:cognitect.aws.outposts.GetOutpostInstanceTypesInput/MaxResults]))
(s/def
:cognitect.aws.outposts/MaxResults1000
(s/spec (s/and int? #(<= 1 % 1000)) :gen #(gen/choose 1 1000)))
(s/def :cognitect.aws.outposts/MacAddressList (s/coll-of :cognitect.aws.outposts/MacAddress))
(s/def
:cognitect.aws.outposts/InstanceTypeItem
(s/keys
:opt-un
[:cognitect.aws.outposts.InstanceTypeItem/InstanceType
:cognitect.aws.outposts.InstanceTypeItem/VCPUs]))
(s/def
:cognitect.aws.outposts/TaskActionOnBlockingInstances
(s/spec string? :gen #(s/gen #{"FAIL_TASK" "WAIT_FOR_EVACUATION"})))
(s/def
:cognitect.aws.outposts/InstanceFamilies
(s/coll-of :cognitect.aws.outposts/InstanceFamilyName))
(s/def
:cognitect.aws.outposts/CapacityTaskStatusList
(s/coll-of :cognitect.aws.outposts/CapacityTaskStatus))
(s/def :cognitect.aws.outposts/DeleteOutpostOutput (s/keys))
(s/def
:cognitect.aws.outposts/ListOrdersInput
(s/keys
:opt-un
[:cognitect.aws.outposts.ListOrdersInput/OutpostIdentifierFilter
:cognitect.aws.outposts.ListOrdersInput/NextToken
:cognitect.aws.outposts.ListOrdersInput/MaxResults]))
(s/def
:cognitect.aws.outposts/Outpost
(s/keys
:opt-un
[:cognitect.aws.outposts.Outpost/OutpostId
:cognitect.aws.outposts.Outpost/SupportedHardwareType
:cognitect.aws.outposts.Outpost/OwnerId
:cognitect.aws.outposts.Outpost/Tags
:cognitect.aws.outposts.Outpost/SiteArn
:cognitect.aws.outposts.Outpost/AvailabilityZone
:cognitect.aws.outposts.Outpost/Description
:cognitect.aws.outposts.Outpost/SiteId
:cognitect.aws.outposts.Outpost/Name
:cognitect.aws.outposts.Outpost/OutpostArn
:cognitect.aws.outposts.Outpost/AvailabilityZoneId
:cognitect.aws.outposts.Outpost/LifeCycleStatus]))
(s/def :cognitect.aws.outposts/HostIdList (s/coll-of :cognitect.aws.outposts/HostId))
(s/def
:cognitect.aws.outposts/ListCatalogItemsInput
(s/keys
:opt-un
[:cognitect.aws.outposts.ListCatalogItemsInput/ItemClassFilter
:cognitect.aws.outposts.ListCatalogItemsInput/NextToken
:cognitect.aws.outposts.ListCatalogItemsInput/SupportedStorageFilter
:cognitect.aws.outposts.ListCatalogItemsInput/MaxResults
:cognitect.aws.outposts.ListCatalogItemsInput/EC2FamilyFilter]))
(s/def
:cognitect.aws.outposts/InstanceTypeListDefinition
(s/coll-of :cognitect.aws.outposts/InstanceTypeItem))
(s/def
:cognitect.aws.outposts/ValidationException
(s/keys :opt-un [:cognitect.aws.outposts.ValidationException/Message]))
(s/def
:cognitect.aws.outposts/OrderId
(s/spec #(re-matches (re-pattern "oo-[a-f0-9]{17}$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/AccessDeniedException
(s/keys :opt-un [:cognitect.aws.outposts.AccessDeniedException/Message]))
(s/def
:cognitect.aws.outposts/Token
(s/spec #(re-matches (re-pattern "^(\\d+)##(\\S+)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/ErrorMessage
(s/spec #(re-matches (re-pattern "^[\\S \\n]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/RackPhysicalProperties
(s/keys
:opt-un
[:cognitect.aws.outposts.RackPhysicalProperties/UplinkCount
:cognitect.aws.outposts.RackPhysicalProperties/PowerFeedDrop
:cognitect.aws.outposts.RackPhysicalProperties/PowerDrawKva
:cognitect.aws.outposts.RackPhysicalProperties/PowerPhase
:cognitect.aws.outposts.RackPhysicalProperties/UplinkGbps
:cognitect.aws.outposts.RackPhysicalProperties/MaximumSupportedWeightLbs
:cognitect.aws.outposts.RackPhysicalProperties/PowerConnector
:cognitect.aws.outposts.RackPhysicalProperties/FiberOpticCableType
:cognitect.aws.outposts.RackPhysicalProperties/OpticalStandard]))
(s/def
:cognitect.aws.outposts/AccountId
(s/spec #(re-matches (re-pattern "\\d{12}") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/CancelOrderInput
(s/keys :req-un [:cognitect.aws.outposts.CancelOrderInput/OrderId]))
(s/def
:cognitect.aws.outposts/ComputeAttributes
(s/keys
:opt-un
[:cognitect.aws.outposts.ComputeAttributes/InstanceFamilies
:cognitect.aws.outposts.ComputeAttributes/HostId
:cognitect.aws.outposts.ComputeAttributes/MaxVcpus
:cognitect.aws.outposts.ComputeAttributes/InstanceTypeCapacities
:cognitect.aws.outposts.ComputeAttributes/State]))
(s/def
:cognitect.aws.outposts/CatalogItemListDefinition
(s/coll-of :cognitect.aws.outposts/CatalogItem))
(s/def
:cognitect.aws.outposts/StatusList
(s/coll-of :cognitect.aws.outposts/AssetState :min-count 1 :max-count 3))
(s/def :cognitect.aws.outposts/AssetType (s/spec string? :gen #(s/gen #{"COMPUTE"})))
(s/def
:cognitect.aws.outposts/AvailabilityZoneId
(s/spec #(re-matches (re-pattern "^[a-zA-Z]+\\d-[a-zA-Z]+\\d$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/OrderSummary
(s/keys
:opt-un
[:cognitect.aws.outposts.OrderSummary/OrderSubmissionDate
:cognitect.aws.outposts.OrderSummary/OutpostId
:cognitect.aws.outposts.OrderSummary/OrderFulfilledDate
:cognitect.aws.outposts.OrderSummary/Status
:cognitect.aws.outposts.OrderSummary/LineItemCountsByStatus
:cognitect.aws.outposts.OrderSummary/OrderId
:cognitect.aws.outposts.OrderSummary/OrderType]))
(s/def
:cognitect.aws.outposts/CapacityTaskFailureType
(s/spec
string?
:gen
#(s/gen
#{"RESOURCE_NOT_FOUND"
"INTERNAL_SERVER_ERROR"
"UNSUPPORTED_CAPACITY_CONFIGURATION"
"BLOCKING_INSTANCES_NOT_EVACUATED"
"UNEXPECTED_ASSET_STATE"})))
(s/def
:cognitect.aws.outposts/GetOutpostSupportedInstanceTypesOutput
(s/keys
:opt-un
[:cognitect.aws.outposts.GetOutpostSupportedInstanceTypesOutput/InstanceTypes
:cognitect.aws.outposts.GetOutpostSupportedInstanceTypesOutput/NextToken]))
(s/def
:cognitect.aws.outposts/ListAssetsOutput
(s/keys
:opt-un
[:cognitect.aws.outposts.ListAssetsOutput/NextToken
:cognitect.aws.outposts.ListAssetsOutput/Assets]))
(s/def
:cognitect.aws.outposts/CatalogItemStatus
(s/spec string? :gen #(s/gen #{"AVAILABLE" "DISCONTINUED"})))
(s/def
:cognitect.aws.outposts/CountryCode
(s/spec #(re-matches (re-pattern "^[A-Z]{2}$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/PowerPhase
(s/spec string? :gen #(s/gen #{"SINGLE_PHASE" "THREE_PHASE"})))
(s/def
:cognitect.aws.outposts/InstanceId
(s/spec #(re-matches (re-pattern "^i-[0-9a-z]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/UplinkGbps
(s/spec string? :gen #(s/gen #{"UPLINK_100G" "UPLINK_40G" "UPLINK_1G" "UPLINK_10G"})))
(s/def :cognitect.aws.outposts/InstanceType string?)
(s/def
:cognitect.aws.outposts/AvailabilityZoneIdList
(s/coll-of :cognitect.aws.outposts/AvailabilityZoneId :min-count 1 :max-count 5))
(s/def
:cognitect.aws.outposts/ListBlockingInstancesForCapacityTaskInput
(s/keys
:req-un
[:cognitect.aws.outposts.ListBlockingInstancesForCapacityTaskInput/OutpostIdentifier
:cognitect.aws.outposts.ListBlockingInstancesForCapacityTaskInput/CapacityTaskId]
:opt-un
[:cognitect.aws.outposts.ListBlockingInstancesForCapacityTaskInput/NextToken
:cognitect.aws.outposts.ListBlockingInstancesForCapacityTaskInput/MaxResults]))
(s/def
:cognitect.aws.outposts/OutpostArn
(s/spec
#(re-matches
(re-pattern "^arn:aws([a-z-]+)?:outposts:[a-z\\d-]+:\\d{12}:outpost/op-[a-f0-9]{17}$")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.outposts/LineItemQuantity
(s/spec (s/and int? #(<= 1 %)) :gen #(gen/choose 1 Long/MAX_VALUE)))
(s/def
:cognitect.aws.outposts/AWSServiceName
(s/spec string? :gen #(s/gen #{"RDS" "AWS" "EC2" "ELB" "ROUTE53" "ELASTICACHE"})))
(s/def
:cognitect.aws.outposts/SiteName
(s/spec #(re-matches (re-pattern "^[\\S ]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/CapacityTaskId
(s/spec #(re-matches (re-pattern "^cap-[a-f0-9]{17}$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/UpdateSiteRackPhysicalPropertiesOutput
(s/keys :opt-un [:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesOutput/Site]))
(s/def :cognitect.aws.outposts/CancelOrderOutput (s/keys))
(s/def
:cognitect.aws.outposts/UplinkCount
(s/spec
string?
:gen
#(s/gen
#{"UPLINK_COUNT_1"
"UPLINK_COUNT_5"
"UPLINK_COUNT_7"
"UPLINK_COUNT_8"
"UPLINK_COUNT_4"
"UPLINK_COUNT_6"
"UPLINK_COUNT_3"
"UPLINK_COUNT_12"
"UPLINK_COUNT_16"
"UPLINK_COUNT_2"})))
(s/def
:cognitect.aws.outposts/UpdateSiteAddressInput
(s/keys
:req-un
[:cognitect.aws.outposts.UpdateSiteAddressInput/SiteId
:cognitect.aws.outposts.UpdateSiteAddressInput/AddressType
:cognitect.aws.outposts.UpdateSiteAddressInput/Address]))
(s/def
:cognitect.aws.outposts/City
(s/spec #(re-matches (re-pattern "^\\S[\\S ]*$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/GetCapacityTaskOutput
(s/keys
:opt-un
[:cognitect.aws.outposts.GetCapacityTaskOutput/DryRun
:cognitect.aws.outposts.GetCapacityTaskOutput/Failed
:cognitect.aws.outposts.GetCapacityTaskOutput/OutpostId
:cognitect.aws.outposts.GetCapacityTaskOutput/CompletionDate
:cognitect.aws.outposts.GetCapacityTaskOutput/LastModifiedDate
:cognitect.aws.outposts.GetCapacityTaskOutput/CapacityTaskStatus
:cognitect.aws.outposts.GetCapacityTaskOutput/RequestedInstancePools
:cognitect.aws.outposts.GetCapacityTaskOutput/InstancesToExclude
:cognitect.aws.outposts.GetCapacityTaskOutput/CapacityTaskId
:cognitect.aws.outposts.GetCapacityTaskOutput/OrderId
:cognitect.aws.outposts.GetCapacityTaskOutput/CreationDate
:cognitect.aws.outposts.GetCapacityTaskOutput/TaskActionOnBlockingInstances]))
(s/def
:cognitect.aws.outposts/ListAssetsInput
(s/keys
:req-un
[:cognitect.aws.outposts.ListAssetsInput/OutpostIdentifier]
:opt-un
[:cognitect.aws.outposts.ListAssetsInput/StatusFilter
:cognitect.aws.outposts.ListAssetsInput/NextToken
:cognitect.aws.outposts.ListAssetsInput/HostIdFilter
:cognitect.aws.outposts.ListAssetsInput/MaxResults]))
(s/def
:cognitect.aws.outposts/UpdateSiteAddressOutput
(s/keys
:opt-un
[:cognitect.aws.outposts.UpdateSiteAddressOutput/Address
:cognitect.aws.outposts.UpdateSiteAddressOutput/AddressType]))
(s/def
:cognitect.aws.outposts/FiberOpticCableType
(s/spec string? :gen #(s/gen #{"MULTI_MODE" "SINGLE_MODE"})))
(s/def
:cognitect.aws.outposts/GetSiteOutput
(s/keys :opt-un [:cognitect.aws.outposts.GetSiteOutput/Site]))
(s/def
:cognitect.aws.outposts/NotFoundException
(s/keys :opt-un [:cognitect.aws.outposts.NotFoundException/Message]))
(s/def :cognitect.aws.outposts/DryRun boolean?)
(s/def
:cognitect.aws.outposts/LineItem
(s/keys
:opt-un
[:cognitect.aws.outposts.LineItem/ShipmentInformation
:cognitect.aws.outposts.LineItem/Status
:cognitect.aws.outposts.LineItem/Quantity
:cognitect.aws.outposts.LineItem/PreviousLineItemId
:cognitect.aws.outposts.LineItem/AssetInformationList
:cognitect.aws.outposts.LineItem/PreviousOrderId
:cognitect.aws.outposts.LineItem/CatalogItemId
:cognitect.aws.outposts.LineItem/LineItemId]))
(s/def
:cognitect.aws.outposts/SiteNotes
(s/spec #(re-matches (re-pattern "^[\\S \\n]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/NetworkInterfaceDeviceIndex
(s/spec (s/and int? #(<= 0 % 1)) :gen #(gen/choose 0 1)))
(s/def
:cognitect.aws.outposts/GetOrderInput
(s/keys :req-un [:cognitect.aws.outposts.GetOrderInput/OrderId]))
(s/def :cognitect.aws.outposts/MaxSize string?)
(s/def
:cognitect.aws.outposts/SkuCode
(s/spec #(re-matches (re-pattern "OR-[A-Z0-9]{7}") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/GetOutpostSupportedInstanceTypesInput
(s/keys
:req-un
[:cognitect.aws.outposts.GetOutpostSupportedInstanceTypesInput/OutpostIdentifier]
:opt-un
[:cognitect.aws.outposts.GetOutpostSupportedInstanceTypesInput/NextToken
:cognitect.aws.outposts.GetOutpostSupportedInstanceTypesInput/OrderId
:cognitect.aws.outposts.GetOutpostSupportedInstanceTypesInput/MaxResults]))
(s/def
:cognitect.aws.outposts/OrderStatus
(s/spec
string?
:gen
#(s/gen
#{"COMPLETED"
"IN_PROGRESS"
"PENDING"
"RECEIVED"
"ERROR"
"PREPARING"
"FULFILLED"
"DELIVERED"
"CANCELLED"
"INSTALLING"
"PROCESSING"})))
(s/def
:cognitect.aws.outposts/UnderlayIpAddress
(s/spec #(re-matches (re-pattern "^([0-9]{1,3}\\.){3}[0-9]{1,3}$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/OutpostIdentifier
(s/spec
#(re-matches
(re-pattern "^(arn:aws([a-z-]+)?:outposts:[a-z\\d-]+:\\d{12}:outpost/)?op-[a-f0-9]{17}$")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.outposts/InstanceFamilyName
(s/spec #(re-matches (re-pattern "^(?:.{1,200}/)?(?:[a-z0-9-_A-Z])+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/SupportedHardwareType
(s/spec string? :gen #(s/gen #{"SERVER" "RACK"})))
(s/def
:cognitect.aws.outposts/ContactName
(s/spec #(re-matches (re-pattern "^\\S[\\S ]*$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/UpdateOutpostOutput
(s/keys :opt-un [:cognitect.aws.outposts.UpdateOutpostOutput/Outpost]))
(s/def
:cognitect.aws.outposts/UpdateSiteOutput
(s/keys :opt-un [:cognitect.aws.outposts.UpdateSiteOutput/Site]))
(s/def
:cognitect.aws.outposts/CreateSiteOutput
(s/keys :opt-un [:cognitect.aws.outposts.CreateSiteOutput/Site]))
(s/def
:cognitect.aws.outposts/StartConnectionRequest
(s/keys
:req-un
[:cognitect.aws.outposts.StartConnectionRequest/AssetId
:cognitect.aws.outposts.StartConnectionRequest/ClientPublicKey
:cognitect.aws.outposts.StartConnectionRequest/NetworkInterfaceDeviceIndex]
:opt-un
[:cognitect.aws.outposts.StartConnectionRequest/DeviceSerialNumber]))
(s/def
:cognitect.aws.outposts/Address
(s/keys
:req-un
[:cognitect.aws.outposts.Address/AddressLine1
:cognitect.aws.outposts.Address/City
:cognitect.aws.outposts.Address/StateOrRegion
:cognitect.aws.outposts.Address/PostalCode
:cognitect.aws.outposts.Address/CountryCode]
:opt-un
[:cognitect.aws.outposts.Address/AddressLine3
:cognitect.aws.outposts.Address/ContactPhoneNumber
:cognitect.aws.outposts.Address/ContactName
:cognitect.aws.outposts.Address/DistrictOrCounty
:cognitect.aws.outposts.Address/Municipality
:cognitect.aws.outposts.Address/AddressLine2]))
(s/def
:cognitect.aws.outposts/ListBlockingInstancesForCapacityTaskOutput
(s/keys
:opt-un
[:cognitect.aws.outposts.ListBlockingInstancesForCapacityTaskOutput/BlockingInstances
:cognitect.aws.outposts.ListBlockingInstancesForCapacityTaskOutput/NextToken]))
(s/def
:cognitect.aws.outposts/UpdateSiteRackPhysicalPropertiesInput
(s/keys
:req-un
[:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/SiteId]
:opt-un
[:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/UplinkCount
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/PowerFeedDrop
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/PowerDrawKva
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/PowerPhase
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/UplinkGbps
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/MaximumSupportedWeightLbs
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/PowerConnector
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/FiberOpticCableType
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/OpticalStandard]))
(s/def
:cognitect.aws.outposts/CapacityTaskStatus
(s/spec
string?
:gen
#(s/gen
#{"COMPLETED"
"CANCELLATION_IN_PROGRESS"
"IN_PROGRESS"
"WAITING_FOR_EVACUATION"
"FAILED"
"CANCELLED"
"REQUESTED"})))
(s/def
:cognitect.aws.outposts/CapacityTaskFailure
(s/keys
:req-un
[:cognitect.aws.outposts.CapacityTaskFailure/Reason]
:opt-un
[:cognitect.aws.outposts.CapacityTaskFailure/Type]))
(s/def :cognitect.aws.outposts/SupportedStorageEnum (s/spec string? :gen #(s/gen #{"S3" "EBS"})))
(s/def
:cognitect.aws.outposts/HostId
(s/spec #(re-matches (re-pattern "^[A-Za-z0-9-]*$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/LineItemId
(s/spec #(re-matches (re-pattern "ooi-[a-f0-9]{17}") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/TagValue
(s/spec #(re-matches (re-pattern "^[\\S \\n]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/DeviceSerialNumber
(s/spec #(re-matches (re-pattern "^(\\w+)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/GetOrderOutput
(s/keys :opt-un [:cognitect.aws.outposts.GetOrderOutput/Order]))
(s/def
:cognitect.aws.outposts/AWSServiceNameList
(s/coll-of :cognitect.aws.outposts/AWSServiceName))
(s/def
:cognitect.aws.outposts/OutpostInstanceTypeList
(s/coll-of :cognitect.aws.outposts/OutpostInstanceType))
(s/def
:cognitect.aws.outposts/SupportedUplinkGbpsListDefinition
(s/coll-of :cognitect.aws.outposts/SupportedUplinkGbps))
(s/def
:cognitect.aws.outposts/CatalogItemClassList
(s/coll-of :cognitect.aws.outposts/CatalogItemClass))
(s/def
:cognitect.aws.outposts/RackElevation
(s/spec
(s/and double? #(<= 0 % 99))
:gen
#(gen/double* {:infinite? false, :NaN? false, :min 0, :max 99})))
(s/def
:cognitect.aws.outposts/ListSitesInput
(s/keys
:opt-un
[:cognitect.aws.outposts.ListSitesInput/OperatingAddressCountryCodeFilter
:cognitect.aws.outposts.ListSitesInput/NextToken
:cognitect.aws.outposts.ListSitesInput/OperatingAddressStateOrRegionFilter
:cognitect.aws.outposts.ListSitesInput/MaxResults
:cognitect.aws.outposts.ListSitesInput/OperatingAddressCityFilter]))
(s/def
:cognitect.aws.outposts/ListTagsForResourceRequest
(s/keys :req-un [:cognitect.aws.outposts.ListTagsForResourceRequest/ResourceArn]))
(s/def
:cognitect.aws.outposts/CapacityTaskStatusReason
(s/spec
(s/and string? #(>= 128 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 128) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.outposts/MacAddress
(s/spec
#(re-matches (re-pattern "^([0-9A-Fa-f]{2}[:-]){5}([0-9A-Fa-f]{2})$") %)
:gen
#(gen/string)))
(s/def :cognitect.aws.outposts/CityList (s/coll-of :cognitect.aws.outposts/City))
(s/def
:cognitect.aws.outposts/AddressLine2
(s/spec #(re-matches (re-pattern "^\\S[\\S ]*$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/DeleteOutpostInput
(s/keys :req-un [:cognitect.aws.outposts.DeleteOutpostInput/OutpostId]))
(s/def
:cognitect.aws.outposts/LineItemRequestListDefinition
(s/coll-of :cognitect.aws.outposts/LineItemRequest :min-count 1 :max-count 20))
(s/def
:cognitect.aws.outposts/VCPUCount
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def :cognitect.aws.outposts/InstanceIdList (s/coll-of :cognitect.aws.outposts/InstanceId))
(s/def
:cognitect.aws.outposts/PaymentTerm
(s/spec string? :gen #(s/gen #{"ONE_YEAR" "THREE_YEARS" "FIVE_YEARS"})))
(s/def
:cognitect.aws.outposts/OutpostInstanceType
(s/spec #(re-matches (re-pattern "[a-z0-9\\-\\.]+") %) :gen #(gen/string)))
(s/def :cognitect.aws.outposts/UntagResourceResponse (s/keys))
(s/def
:cognitect.aws.outposts/PaymentOption
(s/spec string? :gen #(s/gen #{"NO_UPFRONT" "PARTIAL_UPFRONT" "ALL_UPFRONT"})))
(s/def
:cognitect.aws.outposts/AssetInstanceTypeCapacity
(s/keys
:req-un
[:cognitect.aws.outposts.AssetInstanceTypeCapacity/InstanceType
:cognitect.aws.outposts.AssetInstanceTypeCapacity/Count]))
(s/def
:cognitect.aws.outposts/LifeCycleStatus
(s/spec #(re-matches (re-pattern "^[ A-Za-z]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/GetOutpostInput
(s/keys :req-un [:cognitect.aws.outposts.GetOutpostInput/OutpostId]))
(s/def
:cognitect.aws.outposts/BlockingInstancesList
(s/coll-of :cognitect.aws.outposts/BlockingInstance))
(s/def
:cognitect.aws.outposts/ComputeAssetState
(s/spec string? :gen #(s/gen #{"ISOLATED" "RETIRING" "ACTIVE"})))
(s/def
:cognitect.aws.outposts/RequestedInstancePools
(s/coll-of :cognitect.aws.outposts/InstanceTypeCapacity))
(s/def
:cognitect.aws.outposts/PowerFeedDrop
(s/spec string? :gen #(s/gen #{"BELOW_RACK" "ABOVE_RACK"})))
(s/def
:cognitect.aws.outposts/SiteId
(s/spec
#(re-matches
(re-pattern "^(arn:aws([a-z-]+)?:outposts:[a-z\\d-]+:\\d{12}:site/)?(os-[a-f0-9]{17})$")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.outposts/ListAssetInstancesInput
(s/keys
:req-un
[:cognitect.aws.outposts.ListAssetInstancesInput/OutpostIdentifier]
:opt-un
[:cognitect.aws.outposts.ListAssetInstancesInput/InstanceTypeFilter
:cognitect.aws.outposts.ListAssetInstancesInput/AssetIdFilter
:cognitect.aws.outposts.ListAssetInstancesInput/NextToken
:cognitect.aws.outposts.ListAssetInstancesInput/AwsServiceFilter
:cognitect.aws.outposts.ListAssetInstancesInput/AccountIdFilter
:cognitect.aws.outposts.ListAssetInstancesInput/MaxResults]))
(s/def
:cognitect.aws.outposts/CreateOrderOutput
(s/keys :opt-un [:cognitect.aws.outposts.CreateOrderOutput/Order]))
(s/def
:cognitect.aws.outposts/ServerEndpoint
(s/spec
#(re-matches (re-pattern "^([0-9]{1,3}\\.){3}[0-9]{1,3}:[0-9]{1,5}$") %)
:gen
#(gen/string)))
(s/def
:cognitect.aws.outposts/TrackingId
(s/spec #(re-matches (re-pattern "^[a-zA-Z0-9]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/OwnerId
(s/spec #(re-matches (re-pattern "\\d{12}") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/AvailabilityZoneList
(s/coll-of :cognitect.aws.outposts/AvailabilityZone :min-count 1 :max-count 5))
(s/def
:cognitect.aws.outposts/ListOrdersOutput
(s/keys
:opt-un
[:cognitect.aws.outposts.ListOrdersOutput/NextToken
:cognitect.aws.outposts.ListOrdersOutput/Orders]))
(s/def
:cognitect.aws.outposts/OutpostName
(s/spec #(re-matches (re-pattern "^[\\S ]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/StartCapacityTaskInput
(s/keys
:req-un
[:cognitect.aws.outposts.StartCapacityTaskInput/OutpostIdentifier
:cognitect.aws.outposts.StartCapacityTaskInput/InstancePools]
:opt-un
[:cognitect.aws.outposts.StartCapacityTaskInput/DryRun
:cognitect.aws.outposts.StartCapacityTaskInput/InstancesToExclude
:cognitect.aws.outposts.StartCapacityTaskInput/OrderId
:cognitect.aws.outposts.StartCapacityTaskInput/TaskActionOnBlockingInstances]))
(s/def :cognitect.aws.outposts/CountryCodeList (s/coll-of :cognitect.aws.outposts/CountryCode))
(s/def
:cognitect.aws.outposts/ConnectionId
(s/spec #(re-matches (re-pattern "^[a-zA-Z0-9+/=]{1,1024}$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/ShipmentInformation
(s/keys
:opt-un
[:cognitect.aws.outposts.ShipmentInformation/ShipmentTrackingNumber
:cognitect.aws.outposts.ShipmentInformation/ShipmentCarrier]))
(s/def
:cognitect.aws.outposts/DeleteSiteInput
(s/keys :req-un [:cognitect.aws.outposts.DeleteSiteInput/SiteId]))
(s/def
:cognitect.aws.outposts/ListOutpostsInput
(s/keys
:opt-un
[:cognitect.aws.outposts.ListOutpostsInput/AvailabilityZoneFilter
:cognitect.aws.outposts.ListOutpostsInput/NextToken
:cognitect.aws.outposts.ListOutpostsInput/LifeCycleStatusFilter
:cognitect.aws.outposts.ListOutpostsInput/AvailabilityZoneIdFilter
:cognitect.aws.outposts.ListOutpostsInput/MaxResults]))
(s/def :cognitect.aws.outposts/AssetListDefinition (s/coll-of :cognitect.aws.outposts/AssetInfo))
(s/def :cognitect.aws.outposts/DeleteSiteOutput (s/keys))
(s/def
:cognitect.aws.outposts/InstanceTypeCount
(s/spec (s/and int? #(<= 0 % 9999)) :gen #(gen/choose 0 9999)))
(s/def
:cognitect.aws.outposts/CIDR
(s/spec
#(re-matches (re-pattern "^([0-9]{1,3}\\.){3}[0-9]{1,3}/[0-9]{1,2}$") %)
:gen
#(gen/string)))
(s/def
:cognitect.aws.outposts/OpticalStandard
(s/spec
string?
:gen
#(s/gen
#{"OPTIC_40GBASE_IR4_LR4L"
"OPTIC_40GBASE_LR4"
"OPTIC_100G_PSM4_MSA"
"OPTIC_10GBASE_SR"
"OPTIC_100GBASE_LR4"
"OPTIC_100GBASE_SR4"
"OPTIC_40GBASE_ESR"
"OPTIC_10GBASE_IR"
"OPTIC_10GBASE_LR"
"OPTIC_1000BASE_LX"
"OPTIC_40GBASE_SR"
"OPTIC_1000BASE_SX"
"OPTIC_100GBASE_CWDM4"})))
(s/def
:cognitect.aws.outposts/ContactPhoneNumber
(s/spec #(re-matches (re-pattern "^[\\S ]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/ConnectionDetails
(s/keys
:opt-un
[:cognitect.aws.outposts.ConnectionDetails/ClientTunnelAddress
:cognitect.aws.outposts.ConnectionDetails/ServerEndpoint
:cognitect.aws.outposts.ConnectionDetails/ClientPublicKey
:cognitect.aws.outposts.ConnectionDetails/ServerPublicKey
:cognitect.aws.outposts.ConnectionDetails/AllowedIps
:cognitect.aws.outposts.ConnectionDetails/ServerTunnelAddress]))
(s/def :cognitect.aws.outposts/AccountIdList (s/coll-of :cognitect.aws.outposts/AccountId))
(s/def
:cognitect.aws.outposts/AssetInfo
(s/keys
:opt-un
[:cognitect.aws.outposts.AssetInfo/RackId
:cognitect.aws.outposts.AssetInfo/ComputeAttributes
:cognitect.aws.outposts.AssetInfo/AssetType
:cognitect.aws.outposts.AssetInfo/AssetLocation
:cognitect.aws.outposts.AssetInfo/AssetId]))
(s/def
:cognitect.aws.outposts/Arn
(s/spec
#(re-matches
(re-pattern
"^(arn:aws([a-z-]+)?:outposts:[a-z\\d-]+:\\d{12}:([a-z\\d-]+)/)[a-z]{2,8}-[a-f0-9]{17}$")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.outposts/GetConnectionResponse
(s/keys
:opt-un
[:cognitect.aws.outposts.GetConnectionResponse/ConnectionId
:cognitect.aws.outposts.GetConnectionResponse/ConnectionDetails]))
(s/def
:cognitect.aws.outposts/Family
(s/spec #(re-matches (re-pattern "[a-z0-9]+") %) :gen #(gen/string)))
(s/def :cognitect.aws.outposts/StateOrRegionList (s/coll-of :cognitect.aws.outposts/StateOrRegion))
(s/def
:cognitect.aws.outposts/StateOrRegion
(s/spec #(re-matches (re-pattern "^\\S[\\S ]*$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/SupportedUplinkGbps
(s/spec int? :gen #(gen/choose Long/MIN_VALUE Long/MAX_VALUE)))
(s/def
:cognitect.aws.outposts/ListCapacityTasksOutput
(s/keys
:opt-un
[:cognitect.aws.outposts.ListCapacityTasksOutput/NextToken
:cognitect.aws.outposts.ListCapacityTasksOutput/CapacityTasks]))
(s/def
:cognitect.aws.outposts/UntagResourceRequest
(s/keys
:req-un
[:cognitect.aws.outposts.UntagResourceRequest/ResourceArn
:cognitect.aws.outposts.UntagResourceRequest/TagKeys]))
(s/def
:cognitect.aws.outposts/TagKey
(s/spec #(re-matches (re-pattern "^(?!aws:)[a-zA-Z+-=._:/]+$") %) :gen #(gen/string)))
(s/def :cognitect.aws.outposts/AssetIdList (s/coll-of :cognitect.aws.outposts/AssetId))
(s/def :cognitect.aws.outposts/OrderType (s/spec string? :gen #(s/gen #{"OUTPOST" "REPLACEMENT"})))
(s/def
:cognitect.aws.outposts/GetCapacityTaskInput
(s/keys
:req-un
[:cognitect.aws.outposts.GetCapacityTaskInput/CapacityTaskId
:cognitect.aws.outposts.GetCapacityTaskInput/OutpostIdentifier]))
(s/def
:cognitect.aws.outposts/ListOutpostsOutput
(s/keys
:opt-un
[:cognitect.aws.outposts.ListOutpostsOutput/NextToken
:cognitect.aws.outposts.ListOutpostsOutput/Outposts]))
(s/def
:cognitect.aws.outposts/MaximumSupportedWeightLbs
(s/spec
string?
:gen
#(s/gen #{"NO_LIMIT" "MAX_1800_LBS" "MAX_1400_LBS" "MAX_2000_LBS" "MAX_1600_LBS"})))
(s/def
:cognitect.aws.outposts/InstanceTypeCapacity
(s/keys
:req-un
[:cognitect.aws.outposts.InstanceTypeCapacity/InstanceType
:cognitect.aws.outposts.InstanceTypeCapacity/Count]))
(s/def
:cognitect.aws.outposts/StartCapacityTaskOutput
(s/keys
:opt-un
[:cognitect.aws.outposts.StartCapacityTaskOutput/DryRun
:cognitect.aws.outposts.StartCapacityTaskOutput/Failed
:cognitect.aws.outposts.StartCapacityTaskOutput/OutpostId
:cognitect.aws.outposts.StartCapacityTaskOutput/CompletionDate
:cognitect.aws.outposts.StartCapacityTaskOutput/LastModifiedDate
:cognitect.aws.outposts.StartCapacityTaskOutput/CapacityTaskStatus
:cognitect.aws.outposts.StartCapacityTaskOutput/RequestedInstancePools
:cognitect.aws.outposts.StartCapacityTaskOutput/InstancesToExclude
:cognitect.aws.outposts.StartCapacityTaskOutput/CapacityTaskId
:cognitect.aws.outposts.StartCapacityTaskOutput/OrderId
:cognitect.aws.outposts.StartCapacityTaskOutput/CreationDate
:cognitect.aws.outposts.StartCapacityTaskOutput/TaskActionOnBlockingInstances]))
(s/def
:cognitect.aws.outposts/ShipmentCarrier
(s/spec string? :gen #(s/gen #{"DHL" "FEDEX" "UPS" "EXPEDITORS" "DBS"})))
(s/def
:cognitect.aws.outposts/CancelCapacityTaskInput
(s/keys
:req-un
[:cognitect.aws.outposts.CancelCapacityTaskInput/CapacityTaskId
:cognitect.aws.outposts.CancelCapacityTaskInput/OutpostIdentifier]))
(s/def
:cognitect.aws.outposts/LineItemStatusCounts
(s/map-of :cognitect.aws.outposts/LineItemStatus :cognitect.aws.outposts/LineItemQuantity))
(s/def
:cognitect.aws.outposts/LineItemStatus
(s/spec
string?
:gen
#(s/gen
#{"REPLACED"
"ERROR"
"PREPARING"
"INSTALLED"
"BUILDING"
"SHIPPED"
"DELIVERED"
"CANCELLED"
"INSTALLING"})))
(s/def :cognitect.aws.outposts/Quantity string?)
(s/def
:cognitect.aws.outposts/UpdateOutpostInput
(s/keys
:req-un
[:cognitect.aws.outposts.UpdateOutpostInput/OutpostId]
:opt-un
[:cognitect.aws.outposts.UpdateOutpostInput/SupportedHardwareType
:cognitect.aws.outposts.UpdateOutpostInput/Description
:cognitect.aws.outposts.UpdateOutpostInput/Name]))
(s/def
:cognitect.aws.outposts/ListSitesOutput
(s/keys
:opt-un
[:cognitect.aws.outposts.ListSitesOutput/Sites
:cognitect.aws.outposts.ListSitesOutput/NextToken]))
(s/def
:cognitect.aws.outposts/InstancesToExclude
(s/keys
:opt-un
[:cognitect.aws.outposts.InstancesToExclude/Services
:cognitect.aws.outposts.InstancesToExclude/AccountIds
:cognitect.aws.outposts.InstancesToExclude/Instances]))
(s/def
:cognitect.aws.outposts/EC2Capacity
(s/keys
:opt-un
[:cognitect.aws.outposts.EC2Capacity/Family
:cognitect.aws.outposts.EC2Capacity/Quantity
:cognitect.aws.outposts.EC2Capacity/MaxSize]))
(s/def
:cognitect.aws.outposts/StartConnectionResponse
(s/keys
:opt-un
[:cognitect.aws.outposts.StartConnectionResponse/ConnectionId
:cognitect.aws.outposts.StartConnectionResponse/UnderlayIpAddress]))
(s/def
:cognitect.aws.outposts/CapacityTaskList
(s/coll-of :cognitect.aws.outposts/CapacityTaskSummary))
(s/def
:cognitect.aws.outposts/AssetState
(s/spec string? :gen #(s/gen #{"ISOLATED" "RETIRING" "ACTIVE"})))
(s/def
:cognitect.aws.outposts/GetSiteAddressOutput
(s/keys
:opt-un
[:cognitect.aws.outposts.GetSiteAddressOutput/Address
:cognitect.aws.outposts.GetSiteAddressOutput/AddressType
:cognitect.aws.outposts.GetSiteAddressOutput/SiteId]))
(s/def
:cognitect.aws.outposts/OutpostId
(s/spec
#(re-matches
(re-pattern "^(arn:aws([a-z-]+)?:outposts:[a-z\\d-]+:\\d{12}:outpost/)?op-[a-f0-9]{17}$")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.outposts/CreateOrderInput
(s/keys
:req-un
[:cognitect.aws.outposts.CreateOrderInput/OutpostIdentifier
:cognitect.aws.outposts.CreateOrderInput/LineItems
:cognitect.aws.outposts.CreateOrderInput/PaymentOption]
:opt-un
[:cognitect.aws.outposts.CreateOrderInput/PaymentTerm]))
(s/def
:cognitect.aws.outposts/SiteDescription
(s/spec #(re-matches (re-pattern "^[\\S ]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/ListTagsForResourceResponse
(s/keys :opt-un [:cognitect.aws.outposts.ListTagsForResourceResponse/Tags]))
(s/def
:cognitect.aws.outposts/OrderSummaryListDefinition
(s/coll-of :cognitect.aws.outposts/OrderSummary))
(s/def :cognitect.aws.outposts/LineItemListDefinition (s/coll-of :cognitect.aws.outposts/LineItem))
(s/def
:cognitect.aws.outposts/ListAssetInstancesOutput
(s/keys
:opt-un
[:cognitect.aws.outposts.ListAssetInstancesOutput/AssetInstances
:cognitect.aws.outposts.ListAssetInstancesOutput/NextToken]))
(s/def
:cognitect.aws.outposts/AddressType
(s/spec string? :gen #(s/gen #{"OPERATING_ADDRESS" "SHIPPING_ADDRESS"})))
(s/def
:cognitect.aws.outposts/Site
(s/keys
:opt-un
[:cognitect.aws.outposts.Site/OperatingAddressCity
:cognitect.aws.outposts.Site/OperatingAddressCountryCode
:cognitect.aws.outposts.Site/Tags
:cognitect.aws.outposts.Site/SiteArn
:cognitect.aws.outposts.Site/Description
:cognitect.aws.outposts.Site/OperatingAddressStateOrRegion
:cognitect.aws.outposts.Site/AccountId
:cognitect.aws.outposts.Site/RackPhysicalProperties
:cognitect.aws.outposts.Site/SiteId
:cognitect.aws.outposts.Site/Name
:cognitect.aws.outposts.Site/Notes]))
(s/def
:cognitect.aws.outposts/GetCatalogItemInput
(s/keys :req-un [:cognitect.aws.outposts.GetCatalogItemInput/CatalogItemId]))
(s/def
:cognitect.aws.outposts/InstanceTypeName
(s/spec #(re-matches (re-pattern "^[a-z0-9\\-]+\\.[a-z0-9\\-]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/CapacityTaskSummary
(s/keys
:opt-un
[:cognitect.aws.outposts.CapacityTaskSummary/OutpostId
:cognitect.aws.outposts.CapacityTaskSummary/CompletionDate
:cognitect.aws.outposts.CapacityTaskSummary/LastModifiedDate
:cognitect.aws.outposts.CapacityTaskSummary/CapacityTaskStatus
:cognitect.aws.outposts.CapacityTaskSummary/CapacityTaskId
:cognitect.aws.outposts.CapacityTaskSummary/OrderId
:cognitect.aws.outposts.CapacityTaskSummary/CreationDate]))
(s/def
:cognitect.aws.outposts/AddressLine1
(s/spec #(re-matches (re-pattern "^\\S[\\S ]*$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/WireGuardPublicKey
(s/spec #(re-matches (re-pattern "^[a-zA-Z0-9/+]{43}=$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/SupportedStorageList
(s/coll-of :cognitect.aws.outposts/SupportedStorageEnum))
(s/def
:cognitect.aws.outposts/GetOutpostOutput
(s/keys :opt-un [:cognitect.aws.outposts.GetOutpostOutput/Outpost]))
(s/def
:cognitect.aws.outposts/CreateOutpostOutput
(s/keys :opt-un [:cognitect.aws.outposts.CreateOutpostOutput/Outpost]))
(s/def
:cognitect.aws.outposts/EC2CapacityListDefinition
(s/coll-of :cognitect.aws.outposts/EC2Capacity))
(s/def
:cognitect.aws.outposts/LineItemAssetInformation
(s/keys
:opt-un
[:cognitect.aws.outposts.LineItemAssetInformation/MacAddressList
:cognitect.aws.outposts.LineItemAssetInformation/AssetId]))
(s/def
:cognitect.aws.outposts/GetSiteAddressInput
(s/keys
:req-un
[:cognitect.aws.outposts.GetSiteAddressInput/SiteId
:cognitect.aws.outposts.GetSiteAddressInput/AddressType]))
(s/def
:cognitect.aws.outposts/OutpostDescription
(s/spec #(re-matches (re-pattern "^[\\S ]*$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/String
(s/spec #(re-matches (re-pattern "^[\\S \\n]+$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.outposts/ListCatalogItemsOutput
(s/keys
:opt-un
[:cognitect.aws.outposts.ListCatalogItemsOutput/NextToken
:cognitect.aws.outposts.ListCatalogItemsOutput/CatalogItems]))
(s/def
:cognitect.aws.outposts/LineItemRequest
(s/keys
:opt-un
[:cognitect.aws.outposts.LineItemRequest/Quantity
:cognitect.aws.outposts.LineItemRequest/CatalogItemId]))
(s/def
:cognitect.aws.outposts/PostalCode
(s/spec #(re-matches (re-pattern "^[a-zA-Z0-9 -]+$") %) :gen #(gen/string)))
(s/def :cognitect.aws.outposts/EC2FamilyList (s/coll-of :cognitect.aws.outposts/Family))
(s/def
:cognitect.aws.outposts/ConflictException
(s/keys
:opt-un
[:cognitect.aws.outposts.ConflictException/ResourceId
:cognitect.aws.outposts.ConflictException/Message
:cognitect.aws.outposts.ConflictException/ResourceType]))
(s/def
:cognitect.aws.outposts/TagKeyList
(s/coll-of :cognitect.aws.outposts/TagKey :min-count 1 :max-count 50))
(s/def
:cognitect.aws.outposts/GetConnectionRequest
(s/keys :req-un [:cognitect.aws.outposts.GetConnectionRequest/ConnectionId]))
(s/def
:cognitect.aws.outposts/Order
(s/keys
:opt-un
[:cognitect.aws.outposts.Order/OrderSubmissionDate
:cognitect.aws.outposts.Order/OutpostId
:cognitect.aws.outposts.Order/OrderFulfilledDate
:cognitect.aws.outposts.Order/PaymentTerm
:cognitect.aws.outposts.Order/PaymentOption
:cognitect.aws.outposts.Order/Status
:cognitect.aws.outposts.Order/OrderId
:cognitect.aws.outposts.Order/OrderType
:cognitect.aws.outposts.Order/LineItems]))
(s/def :cognitect.aws.outposts.CreateSiteInput/Name :cognitect.aws.outposts/SiteName)
(s/def :cognitect.aws.outposts.CreateSiteInput/Description :cognitect.aws.outposts/SiteDescription)
(s/def :cognitect.aws.outposts.CreateSiteInput/Notes :cognitect.aws.outposts/SiteNotes)
(s/def :cognitect.aws.outposts.CreateSiteInput/Tags :cognitect.aws.outposts/TagMap)
(s/def :cognitect.aws.outposts.CreateSiteInput/OperatingAddress :cognitect.aws.outposts/Address)
(s/def :cognitect.aws.outposts.CreateSiteInput/ShippingAddress :cognitect.aws.outposts/Address)
(s/def
:cognitect.aws.outposts.CreateSiteInput/RackPhysicalProperties
:cognitect.aws.outposts/RackPhysicalProperties)
(s/def :cognitect.aws.outposts.AssetInstance/InstanceId :cognitect.aws.outposts/InstanceId)
(s/def
:cognitect.aws.outposts.AssetInstance/InstanceType
:cognitect.aws.outposts/OutpostInstanceType)
(s/def :cognitect.aws.outposts.AssetInstance/AssetId :cognitect.aws.outposts/AssetId)
(s/def :cognitect.aws.outposts.AssetInstance/AccountId :cognitect.aws.outposts/AccountId)
(s/def :cognitect.aws.outposts.AssetInstance/AwsServiceName :cognitect.aws.outposts/AWSServiceName)
(s/def :cognitect.aws.outposts.CatalogItem/CatalogItemId :cognitect.aws.outposts/SkuCode)
(s/def :cognitect.aws.outposts.CatalogItem/ItemStatus :cognitect.aws.outposts/CatalogItemStatus)
(s/def
:cognitect.aws.outposts.CatalogItem/EC2Capacities
:cognitect.aws.outposts/EC2CapacityListDefinition)
(s/def :cognitect.aws.outposts.CatalogItem/PowerKva :cognitect.aws.outposts/CatalogItemPowerKva)
(s/def :cognitect.aws.outposts.CatalogItem/WeightLbs :cognitect.aws.outposts/CatalogItemWeightLbs)
(s/def
:cognitect.aws.outposts.CatalogItem/SupportedUplinkGbps
:cognitect.aws.outposts/SupportedUplinkGbpsListDefinition)
(s/def
:cognitect.aws.outposts.CatalogItem/SupportedStorage
:cognitect.aws.outposts/SupportedStorageList)
(s/def :cognitect.aws.outposts.TagResourceRequest/ResourceArn :cognitect.aws.outposts/Arn)
(s/def :cognitect.aws.outposts.TagResourceRequest/Tags :cognitect.aws.outposts/TagMap)
(s/def
:cognitect.aws.outposts.GetOutpostInstanceTypesOutput/InstanceTypes
:cognitect.aws.outposts/InstanceTypeListDefinition)
(s/def
:cognitect.aws.outposts.GetOutpostInstanceTypesOutput/NextToken
:cognitect.aws.outposts/Token)
(s/def
:cognitect.aws.outposts.GetOutpostInstanceTypesOutput/OutpostId
:cognitect.aws.outposts/OutpostId)
(s/def
:cognitect.aws.outposts.GetOutpostInstanceTypesOutput/OutpostArn
:cognitect.aws.outposts/OutpostArn)
(s/def
:cognitect.aws.outposts.ServiceQuotaExceededException/Message
:cognitect.aws.outposts/ErrorMessage)
(s/def :cognitect.aws.outposts.InternalServerException/Message :cognitect.aws.outposts/ErrorMessage)
(s/def :cognitect.aws.outposts.BlockingInstance/InstanceId :cognitect.aws.outposts/InstanceId)
(s/def :cognitect.aws.outposts.BlockingInstance/AccountId :cognitect.aws.outposts/AccountId)
(s/def
:cognitect.aws.outposts.BlockingInstance/AwsServiceName
:cognitect.aws.outposts/AWSServiceName)
(s/def
:cognitect.aws.outposts.ListCapacityTasksInput/OutpostIdentifierFilter
:cognitect.aws.outposts/OutpostIdentifier)
(s/def
:cognitect.aws.outposts.ListCapacityTasksInput/MaxResults
:cognitect.aws.outposts/MaxResults1000)
(s/def :cognitect.aws.outposts.ListCapacityTasksInput/NextToken :cognitect.aws.outposts/Token)
(s/def
:cognitect.aws.outposts.ListCapacityTasksInput/CapacityTaskStatusFilter
:cognitect.aws.outposts/CapacityTaskStatusList)
(s/def :cognitect.aws.outposts.GetSiteInput/SiteId :cognitect.aws.outposts/SiteId)
(s/def :cognitect.aws.outposts.GetCatalogItemOutput/CatalogItem :cognitect.aws.outposts/CatalogItem)
(s/def :cognitect.aws.outposts.CreateOutpostInput/Name :cognitect.aws.outposts/OutpostName)
(s/def
:cognitect.aws.outposts.CreateOutpostInput/Description
:cognitect.aws.outposts/OutpostDescription)
(s/def :cognitect.aws.outposts.CreateOutpostInput/SiteId :cognitect.aws.outposts/SiteId)
(s/def
:cognitect.aws.outposts.CreateOutpostInput/AvailabilityZone
:cognitect.aws.outposts/AvailabilityZone)
(s/def
:cognitect.aws.outposts.CreateOutpostInput/AvailabilityZoneId
:cognitect.aws.outposts/AvailabilityZoneId)
(s/def :cognitect.aws.outposts.CreateOutpostInput/Tags :cognitect.aws.outposts/TagMap)
(s/def
:cognitect.aws.outposts.CreateOutpostInput/SupportedHardwareType
:cognitect.aws.outposts/SupportedHardwareType)
(s/def :cognitect.aws.outposts.AssetLocation/RackElevation :cognitect.aws.outposts/RackElevation)
(s/def :cognitect.aws.outposts.UpdateSiteInput/SiteId :cognitect.aws.outposts/SiteId)
(s/def :cognitect.aws.outposts.UpdateSiteInput/Name :cognitect.aws.outposts/SiteName)
(s/def :cognitect.aws.outposts.UpdateSiteInput/Description :cognitect.aws.outposts/SiteDescription)
(s/def :cognitect.aws.outposts.UpdateSiteInput/Notes :cognitect.aws.outposts/SiteNotes)
(s/def
:cognitect.aws.outposts.GetOutpostInstanceTypesInput/OutpostId
:cognitect.aws.outposts/OutpostId)
(s/def :cognitect.aws.outposts.GetOutpostInstanceTypesInput/NextToken :cognitect.aws.outposts/Token)
(s/def
:cognitect.aws.outposts.GetOutpostInstanceTypesInput/MaxResults
:cognitect.aws.outposts/MaxResults1000)
(s/def :cognitect.aws.outposts.InstanceTypeItem/InstanceType :cognitect.aws.outposts/InstanceType)
(s/def :cognitect.aws.outposts.InstanceTypeItem/VCPUs :cognitect.aws.outposts/VCPUCount)
(s/def
:cognitect.aws.outposts.ListOrdersInput/OutpostIdentifierFilter
:cognitect.aws.outposts/OutpostIdentifier)
(s/def :cognitect.aws.outposts.ListOrdersInput/NextToken :cognitect.aws.outposts/Token)
(s/def :cognitect.aws.outposts.ListOrdersInput/MaxResults :cognitect.aws.outposts/MaxResults1000)
(s/def :cognitect.aws.outposts.Outpost/AvailabilityZone :cognitect.aws.outposts/AvailabilityZone)
(s/def :cognitect.aws.outposts.Outpost/SiteArn :cognitect.aws.outposts/SiteArn)
(s/def
:cognitect.aws.outposts.Outpost/AvailabilityZoneId
:cognitect.aws.outposts/AvailabilityZoneId)
(s/def :cognitect.aws.outposts.Outpost/Tags :cognitect.aws.outposts/TagMap)
(s/def :cognitect.aws.outposts.Outpost/OutpostArn :cognitect.aws.outposts/OutpostArn)
(s/def
:cognitect.aws.outposts.Outpost/SupportedHardwareType
:cognitect.aws.outposts/SupportedHardwareType)
(s/def :cognitect.aws.outposts.Outpost/LifeCycleStatus :cognitect.aws.outposts/LifeCycleStatus)
(s/def :cognitect.aws.outposts.Outpost/SiteId :cognitect.aws.outposts/SiteId)
(s/def :cognitect.aws.outposts.Outpost/OwnerId :cognitect.aws.outposts/OwnerId)
(s/def :cognitect.aws.outposts.Outpost/Name :cognitect.aws.outposts/OutpostName)
(s/def :cognitect.aws.outposts.Outpost/Description :cognitect.aws.outposts/OutpostDescription)
(s/def :cognitect.aws.outposts.Outpost/OutpostId :cognitect.aws.outposts/OutpostId)
(s/def :cognitect.aws.outposts.ListCatalogItemsInput/NextToken :cognitect.aws.outposts/Token)
(s/def
:cognitect.aws.outposts.ListCatalogItemsInput/MaxResults
:cognitect.aws.outposts/MaxResults1000)
(s/def
:cognitect.aws.outposts.ListCatalogItemsInput/ItemClassFilter
:cognitect.aws.outposts/CatalogItemClassList)
(s/def
:cognitect.aws.outposts.ListCatalogItemsInput/SupportedStorageFilter
:cognitect.aws.outposts/SupportedStorageList)
(s/def
:cognitect.aws.outposts.ListCatalogItemsInput/EC2FamilyFilter
:cognitect.aws.outposts/EC2FamilyList)
(s/def :cognitect.aws.outposts.ValidationException/Message :cognitect.aws.outposts/ErrorMessage)
(s/def :cognitect.aws.outposts.AccessDeniedException/Message :cognitect.aws.outposts/ErrorMessage)
(s/def
:cognitect.aws.outposts.RackPhysicalProperties/PowerDrawKva
:cognitect.aws.outposts/PowerDrawKva)
(s/def
:cognitect.aws.outposts.RackPhysicalProperties/PowerConnector
:cognitect.aws.outposts/PowerConnector)
(s/def :cognitect.aws.outposts.RackPhysicalProperties/PowerPhase :cognitect.aws.outposts/PowerPhase)
(s/def :cognitect.aws.outposts.RackPhysicalProperties/UplinkGbps :cognitect.aws.outposts/UplinkGbps)
(s/def
:cognitect.aws.outposts.RackPhysicalProperties/UplinkCount
:cognitect.aws.outposts/UplinkCount)
(s/def
:cognitect.aws.outposts.RackPhysicalProperties/FiberOpticCableType
:cognitect.aws.outposts/FiberOpticCableType)
(s/def
:cognitect.aws.outposts.RackPhysicalProperties/PowerFeedDrop
:cognitect.aws.outposts/PowerFeedDrop)
(s/def
:cognitect.aws.outposts.RackPhysicalProperties/OpticalStandard
:cognitect.aws.outposts/OpticalStandard)
(s/def
:cognitect.aws.outposts.RackPhysicalProperties/MaximumSupportedWeightLbs
:cognitect.aws.outposts/MaximumSupportedWeightLbs)
(s/def :cognitect.aws.outposts.CancelOrderInput/OrderId :cognitect.aws.outposts/OrderId)
(s/def :cognitect.aws.outposts.ComputeAttributes/HostId :cognitect.aws.outposts/HostId)
(s/def :cognitect.aws.outposts.ComputeAttributes/State :cognitect.aws.outposts/ComputeAssetState)
(s/def
:cognitect.aws.outposts.ComputeAttributes/InstanceFamilies
:cognitect.aws.outposts/InstanceFamilies)
(s/def
:cognitect.aws.outposts.ComputeAttributes/InstanceTypeCapacities
:cognitect.aws.outposts/AssetInstanceCapacityList)
(s/def :cognitect.aws.outposts.ComputeAttributes/MaxVcpus :cognitect.aws.outposts/VCPUCount)
(s/def :cognitect.aws.outposts.OrderSummary/OutpostId :cognitect.aws.outposts/OutpostIdOnly)
(s/def :cognitect.aws.outposts.OrderSummary/OrderId :cognitect.aws.outposts/OrderId)
(s/def :cognitect.aws.outposts.OrderSummary/OrderType :cognitect.aws.outposts/OrderType)
(s/def :cognitect.aws.outposts.OrderSummary/Status :cognitect.aws.outposts/OrderStatus)
(s/def
:cognitect.aws.outposts.OrderSummary/LineItemCountsByStatus
:cognitect.aws.outposts/LineItemStatusCounts)
(s/def
:cognitect.aws.outposts.OrderSummary/OrderSubmissionDate
:cognitect.aws.outposts/ISO8601Timestamp)
(s/def
:cognitect.aws.outposts.OrderSummary/OrderFulfilledDate
:cognitect.aws.outposts/ISO8601Timestamp)
(s/def
:cognitect.aws.outposts.GetOutpostSupportedInstanceTypesOutput/InstanceTypes
:cognitect.aws.outposts/InstanceTypeListDefinition)
(s/def
:cognitect.aws.outposts.GetOutpostSupportedInstanceTypesOutput/NextToken
:cognitect.aws.outposts/Token)
(s/def :cognitect.aws.outposts.ListAssetsOutput/Assets :cognitect.aws.outposts/AssetListDefinition)
(s/def :cognitect.aws.outposts.ListAssetsOutput/NextToken :cognitect.aws.outposts/Token)
(s/def
:cognitect.aws.outposts.ListBlockingInstancesForCapacityTaskInput/OutpostIdentifier
:cognitect.aws.outposts/OutpostIdentifier)
(s/def
:cognitect.aws.outposts.ListBlockingInstancesForCapacityTaskInput/CapacityTaskId
:cognitect.aws.outposts/CapacityTaskId)
(s/def
:cognitect.aws.outposts.ListBlockingInstancesForCapacityTaskInput/MaxResults
:cognitect.aws.outposts/MaxResults1000)
(s/def
:cognitect.aws.outposts.ListBlockingInstancesForCapacityTaskInput/NextToken
:cognitect.aws.outposts/Token)
(s/def
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesOutput/Site
:cognitect.aws.outposts/Site)
(s/def :cognitect.aws.outposts.UpdateSiteAddressInput/SiteId :cognitect.aws.outposts/SiteId)
(s/def
:cognitect.aws.outposts.UpdateSiteAddressInput/AddressType
:cognitect.aws.outposts/AddressType)
(s/def :cognitect.aws.outposts.UpdateSiteAddressInput/Address :cognitect.aws.outposts/Address)
(s/def
:cognitect.aws.outposts.GetCapacityTaskOutput/LastModifiedDate
:cognitect.aws.outposts/ISO8601Timestamp)
(s/def
:cognitect.aws.outposts.GetCapacityTaskOutput/Failed
:cognitect.aws.outposts/CapacityTaskFailure)
(s/def
:cognitect.aws.outposts.GetCapacityTaskOutput/CompletionDate
:cognitect.aws.outposts/ISO8601Timestamp)
(s/def
:cognitect.aws.outposts.GetCapacityTaskOutput/TaskActionOnBlockingInstances
:cognitect.aws.outposts/TaskActionOnBlockingInstances)
(s/def :cognitect.aws.outposts.GetCapacityTaskOutput/OrderId :cognitect.aws.outposts/OrderId)
(s/def
:cognitect.aws.outposts.GetCapacityTaskOutput/CapacityTaskId
:cognitect.aws.outposts/CapacityTaskId)
(s/def :cognitect.aws.outposts.GetCapacityTaskOutput/DryRun :cognitect.aws.outposts/DryRun)
(s/def
:cognitect.aws.outposts.GetCapacityTaskOutput/CapacityTaskStatus
:cognitect.aws.outposts/CapacityTaskStatus)
(s/def
:cognitect.aws.outposts.GetCapacityTaskOutput/RequestedInstancePools
:cognitect.aws.outposts/RequestedInstancePools)
(s/def
:cognitect.aws.outposts.GetCapacityTaskOutput/InstancesToExclude
:cognitect.aws.outposts/InstancesToExclude)
(s/def :cognitect.aws.outposts.GetCapacityTaskOutput/OutpostId :cognitect.aws.outposts/OutpostId)
(s/def
:cognitect.aws.outposts.GetCapacityTaskOutput/CreationDate
:cognitect.aws.outposts/ISO8601Timestamp)
(s/def
:cognitect.aws.outposts.ListAssetsInput/OutpostIdentifier
:cognitect.aws.outposts/OutpostIdentifier)
(s/def :cognitect.aws.outposts.ListAssetsInput/HostIdFilter :cognitect.aws.outposts/HostIdList)
(s/def :cognitect.aws.outposts.ListAssetsInput/MaxResults :cognitect.aws.outposts/MaxResults1000)
(s/def :cognitect.aws.outposts.ListAssetsInput/NextToken :cognitect.aws.outposts/Token)
(s/def :cognitect.aws.outposts.ListAssetsInput/StatusFilter :cognitect.aws.outposts/StatusList)
(s/def
:cognitect.aws.outposts.UpdateSiteAddressOutput/AddressType
:cognitect.aws.outposts/AddressType)
(s/def :cognitect.aws.outposts.UpdateSiteAddressOutput/Address :cognitect.aws.outposts/Address)
(s/def :cognitect.aws.outposts.GetSiteOutput/Site :cognitect.aws.outposts/Site)
(s/def :cognitect.aws.outposts.NotFoundException/Message :cognitect.aws.outposts/ErrorMessage)
(s/def :cognitect.aws.outposts.LineItem/CatalogItemId :cognitect.aws.outposts/SkuCode)
(s/def :cognitect.aws.outposts.LineItem/LineItemId :cognitect.aws.outposts/LineItemId)
(s/def :cognitect.aws.outposts.LineItem/Quantity :cognitect.aws.outposts/LineItemQuantity)
(s/def :cognitect.aws.outposts.LineItem/Status :cognitect.aws.outposts/LineItemStatus)
(s/def
:cognitect.aws.outposts.LineItem/ShipmentInformation
:cognitect.aws.outposts/ShipmentInformation)
(s/def
:cognitect.aws.outposts.LineItem/AssetInformationList
:cognitect.aws.outposts/LineItemAssetInformationList)
(s/def :cognitect.aws.outposts.LineItem/PreviousLineItemId :cognitect.aws.outposts/LineItemId)
(s/def :cognitect.aws.outposts.LineItem/PreviousOrderId :cognitect.aws.outposts/OrderId)
(s/def :cognitect.aws.outposts.GetOrderInput/OrderId :cognitect.aws.outposts/OrderId)
(s/def
:cognitect.aws.outposts.GetOutpostSupportedInstanceTypesInput/OutpostIdentifier
:cognitect.aws.outposts/OutpostIdentifier)
(s/def
:cognitect.aws.outposts.GetOutpostSupportedInstanceTypesInput/OrderId
:cognitect.aws.outposts/OrderId)
(s/def
:cognitect.aws.outposts.GetOutpostSupportedInstanceTypesInput/MaxResults
:cognitect.aws.outposts/MaxResults1000)
(s/def
:cognitect.aws.outposts.GetOutpostSupportedInstanceTypesInput/NextToken
:cognitect.aws.outposts/Token)
(s/def :cognitect.aws.outposts.UpdateOutpostOutput/Outpost :cognitect.aws.outposts/Outpost)
(s/def :cognitect.aws.outposts.UpdateSiteOutput/Site :cognitect.aws.outposts/Site)
(s/def :cognitect.aws.outposts.CreateSiteOutput/Site :cognitect.aws.outposts/Site)
(s/def
:cognitect.aws.outposts.StartConnectionRequest/DeviceSerialNumber
:cognitect.aws.outposts/DeviceSerialNumber)
(s/def :cognitect.aws.outposts.StartConnectionRequest/AssetId :cognitect.aws.outposts/AssetId)
(s/def
:cognitect.aws.outposts.StartConnectionRequest/ClientPublicKey
:cognitect.aws.outposts/WireGuardPublicKey)
(s/def
:cognitect.aws.outposts.StartConnectionRequest/NetworkInterfaceDeviceIndex
:cognitect.aws.outposts/NetworkInterfaceDeviceIndex)
(s/def :cognitect.aws.outposts.Address/AddressLine3 :cognitect.aws.outposts/AddressLine3)
(s/def :cognitect.aws.outposts.Address/Municipality :cognitect.aws.outposts/Municipality)
(s/def :cognitect.aws.outposts.Address/DistrictOrCounty :cognitect.aws.outposts/DistrictOrCounty)
(s/def :cognitect.aws.outposts.Address/CountryCode :cognitect.aws.outposts/CountryCode)
(s/def :cognitect.aws.outposts.Address/City :cognitect.aws.outposts/City)
(s/def :cognitect.aws.outposts.Address/ContactName :cognitect.aws.outposts/ContactName)
(s/def :cognitect.aws.outposts.Address/AddressLine2 :cognitect.aws.outposts/AddressLine2)
(s/def
:cognitect.aws.outposts.Address/ContactPhoneNumber
:cognitect.aws.outposts/ContactPhoneNumber)
(s/def :cognitect.aws.outposts.Address/StateOrRegion :cognitect.aws.outposts/StateOrRegion)
(s/def :cognitect.aws.outposts.Address/AddressLine1 :cognitect.aws.outposts/AddressLine1)
(s/def :cognitect.aws.outposts.Address/PostalCode :cognitect.aws.outposts/PostalCode)
(s/def
:cognitect.aws.outposts.ListBlockingInstancesForCapacityTaskOutput/BlockingInstances
:cognitect.aws.outposts/BlockingInstancesList)
(s/def
:cognitect.aws.outposts.ListBlockingInstancesForCapacityTaskOutput/NextToken
:cognitect.aws.outposts/Token)
(s/def
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/PowerDrawKva
:cognitect.aws.outposts/PowerDrawKva)
(s/def
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/PowerConnector
:cognitect.aws.outposts/PowerConnector)
(s/def
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/PowerPhase
:cognitect.aws.outposts/PowerPhase)
(s/def
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/UplinkGbps
:cognitect.aws.outposts/UplinkGbps)
(s/def
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/UplinkCount
:cognitect.aws.outposts/UplinkCount)
(s/def
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/FiberOpticCableType
:cognitect.aws.outposts/FiberOpticCableType)
(s/def
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/PowerFeedDrop
:cognitect.aws.outposts/PowerFeedDrop)
(s/def
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/SiteId
:cognitect.aws.outposts/SiteId)
(s/def
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/OpticalStandard
:cognitect.aws.outposts/OpticalStandard)
(s/def
:cognitect.aws.outposts.UpdateSiteRackPhysicalPropertiesInput/MaximumSupportedWeightLbs
:cognitect.aws.outposts/MaximumSupportedWeightLbs)
(s/def
:cognitect.aws.outposts.CapacityTaskFailure/Reason
:cognitect.aws.outposts/CapacityTaskStatusReason)
(s/def
:cognitect.aws.outposts.CapacityTaskFailure/Type
:cognitect.aws.outposts/CapacityTaskFailureType)
(s/def :cognitect.aws.outposts.GetOrderOutput/Order :cognitect.aws.outposts/Order)
(s/def :cognitect.aws.outposts.ListSitesInput/NextToken :cognitect.aws.outposts/Token)
(s/def :cognitect.aws.outposts.ListSitesInput/MaxResults :cognitect.aws.outposts/MaxResults1000)
(s/def
:cognitect.aws.outposts.ListSitesInput/OperatingAddressCountryCodeFilter
:cognitect.aws.outposts/CountryCodeList)
(s/def
:cognitect.aws.outposts.ListSitesInput/OperatingAddressStateOrRegionFilter
:cognitect.aws.outposts/StateOrRegionList)
(s/def
:cognitect.aws.outposts.ListSitesInput/OperatingAddressCityFilter
:cognitect.aws.outposts/CityList)
(s/def :cognitect.aws.outposts.ListTagsForResourceRequest/ResourceArn :cognitect.aws.outposts/Arn)
(s/def :cognitect.aws.outposts.DeleteOutpostInput/OutpostId :cognitect.aws.outposts/OutpostId)
(s/def
:cognitect.aws.outposts.AssetInstanceTypeCapacity/InstanceType
:cognitect.aws.outposts/InstanceTypeName)
(s/def
:cognitect.aws.outposts.AssetInstanceTypeCapacity/Count
:cognitect.aws.outposts/InstanceTypeCount)
(s/def :cognitect.aws.outposts.GetOutpostInput/OutpostId :cognitect.aws.outposts/OutpostId)
(s/def
:cognitect.aws.outposts.ListAssetInstancesInput/OutpostIdentifier
:cognitect.aws.outposts/OutpostIdentifier)
(s/def
:cognitect.aws.outposts.ListAssetInstancesInput/AssetIdFilter
:cognitect.aws.outposts/AssetIdList)
(s/def
:cognitect.aws.outposts.ListAssetInstancesInput/InstanceTypeFilter
:cognitect.aws.outposts/OutpostInstanceTypeList)
(s/def
:cognitect.aws.outposts.ListAssetInstancesInput/AccountIdFilter
:cognitect.aws.outposts/AccountIdList)
(s/def
:cognitect.aws.outposts.ListAssetInstancesInput/AwsServiceFilter
:cognitect.aws.outposts/AWSServiceNameList)
(s/def
:cognitect.aws.outposts.ListAssetInstancesInput/MaxResults
:cognitect.aws.outposts/MaxResults1000)
(s/def :cognitect.aws.outposts.ListAssetInstancesInput/NextToken :cognitect.aws.outposts/Token)
(s/def :cognitect.aws.outposts.CreateOrderOutput/Order :cognitect.aws.outposts/Order)
(s/def
:cognitect.aws.outposts.ListOrdersOutput/Orders
:cognitect.aws.outposts/OrderSummaryListDefinition)
(s/def :cognitect.aws.outposts.ListOrdersOutput/NextToken :cognitect.aws.outposts/Token)
(s/def
:cognitect.aws.outposts.StartCapacityTaskInput/OutpostIdentifier
:cognitect.aws.outposts/OutpostIdentifier)
(s/def :cognitect.aws.outposts.StartCapacityTaskInput/OrderId :cognitect.aws.outposts/OrderId)
(s/def
:cognitect.aws.outposts.StartCapacityTaskInput/InstancePools
:cognitect.aws.outposts/RequestedInstancePools)
(s/def
:cognitect.aws.outposts.StartCapacityTaskInput/InstancesToExclude
:cognitect.aws.outposts/InstancesToExclude)
(s/def :cognitect.aws.outposts.StartCapacityTaskInput/DryRun :cognitect.aws.outposts/DryRun)
(s/def
:cognitect.aws.outposts.StartCapacityTaskInput/TaskActionOnBlockingInstances
:cognitect.aws.outposts/TaskActionOnBlockingInstances)
(s/def
:cognitect.aws.outposts.ShipmentInformation/ShipmentTrackingNumber
:cognitect.aws.outposts/TrackingId)
(s/def
:cognitect.aws.outposts.ShipmentInformation/ShipmentCarrier
:cognitect.aws.outposts/ShipmentCarrier)
(s/def :cognitect.aws.outposts.DeleteSiteInput/SiteId :cognitect.aws.outposts/SiteId)
(s/def :cognitect.aws.outposts.ListOutpostsInput/NextToken :cognitect.aws.outposts/Token)
(s/def :cognitect.aws.outposts.ListOutpostsInput/MaxResults :cognitect.aws.outposts/MaxResults1000)
(s/def
:cognitect.aws.outposts.ListOutpostsInput/LifeCycleStatusFilter
:cognitect.aws.outposts/LifeCycleStatusList)
(s/def
:cognitect.aws.outposts.ListOutpostsInput/AvailabilityZoneFilter
:cognitect.aws.outposts/AvailabilityZoneList)
(s/def
:cognitect.aws.outposts.ListOutpostsInput/AvailabilityZoneIdFilter
:cognitect.aws.outposts/AvailabilityZoneIdList)
(s/def
:cognitect.aws.outposts.ConnectionDetails/ClientPublicKey
:cognitect.aws.outposts/WireGuardPublicKey)
(s/def
:cognitect.aws.outposts.ConnectionDetails/ServerPublicKey
:cognitect.aws.outposts/WireGuardPublicKey)
(s/def
:cognitect.aws.outposts.ConnectionDetails/ServerEndpoint
:cognitect.aws.outposts/ServerEndpoint)
(s/def :cognitect.aws.outposts.ConnectionDetails/ClientTunnelAddress :cognitect.aws.outposts/CIDR)
(s/def :cognitect.aws.outposts.ConnectionDetails/ServerTunnelAddress :cognitect.aws.outposts/CIDR)
(s/def :cognitect.aws.outposts.ConnectionDetails/AllowedIps :cognitect.aws.outposts/CIDRList)
(s/def :cognitect.aws.outposts.AssetInfo/AssetId :cognitect.aws.outposts/AssetId)
(s/def :cognitect.aws.outposts.AssetInfo/RackId :cognitect.aws.outposts/RackId)
(s/def :cognitect.aws.outposts.AssetInfo/AssetType :cognitect.aws.outposts/AssetType)
(s/def
:cognitect.aws.outposts.AssetInfo/ComputeAttributes
:cognitect.aws.outposts/ComputeAttributes)
(s/def :cognitect.aws.outposts.AssetInfo/AssetLocation :cognitect.aws.outposts/AssetLocation)
(s/def
:cognitect.aws.outposts.GetConnectionResponse/ConnectionId
:cognitect.aws.outposts/ConnectionId)
(s/def
:cognitect.aws.outposts.GetConnectionResponse/ConnectionDetails
:cognitect.aws.outposts/ConnectionDetails)
(s/def
:cognitect.aws.outposts.ListCapacityTasksOutput/CapacityTasks
:cognitect.aws.outposts/CapacityTaskList)
(s/def :cognitect.aws.outposts.ListCapacityTasksOutput/NextToken :cognitect.aws.outposts/Token)
(s/def :cognitect.aws.outposts.UntagResourceRequest/ResourceArn :cognitect.aws.outposts/Arn)
(s/def :cognitect.aws.outposts.UntagResourceRequest/TagKeys :cognitect.aws.outposts/TagKeyList)
(s/def
:cognitect.aws.outposts.GetCapacityTaskInput/CapacityTaskId
:cognitect.aws.outposts/CapacityTaskId)
(s/def
:cognitect.aws.outposts.GetCapacityTaskInput/OutpostIdentifier
:cognitect.aws.outposts/OutpostIdentifier)
(s/def
:cognitect.aws.outposts.ListOutpostsOutput/Outposts
:cognitect.aws.outposts/outpostListDefinition)
(s/def :cognitect.aws.outposts.ListOutpostsOutput/NextToken :cognitect.aws.outposts/Token)
(s/def
:cognitect.aws.outposts.InstanceTypeCapacity/InstanceType
:cognitect.aws.outposts/InstanceTypeName)
(s/def :cognitect.aws.outposts.InstanceTypeCapacity/Count :cognitect.aws.outposts/InstanceTypeCount)
(s/def
:cognitect.aws.outposts.StartCapacityTaskOutput/LastModifiedDate
:cognitect.aws.outposts/ISO8601Timestamp)
(s/def
:cognitect.aws.outposts.StartCapacityTaskOutput/Failed
:cognitect.aws.outposts/CapacityTaskFailure)
(s/def
:cognitect.aws.outposts.StartCapacityTaskOutput/CompletionDate
:cognitect.aws.outposts/ISO8601Timestamp)
(s/def
:cognitect.aws.outposts.StartCapacityTaskOutput/TaskActionOnBlockingInstances
:cognitect.aws.outposts/TaskActionOnBlockingInstances)
(s/def :cognitect.aws.outposts.StartCapacityTaskOutput/OrderId :cognitect.aws.outposts/OrderId)
(s/def
:cognitect.aws.outposts.StartCapacityTaskOutput/CapacityTaskId
:cognitect.aws.outposts/CapacityTaskId)
(s/def :cognitect.aws.outposts.StartCapacityTaskOutput/DryRun :cognitect.aws.outposts/DryRun)
(s/def
:cognitect.aws.outposts.StartCapacityTaskOutput/CapacityTaskStatus
:cognitect.aws.outposts/CapacityTaskStatus)
(s/def
:cognitect.aws.outposts.StartCapacityTaskOutput/RequestedInstancePools
:cognitect.aws.outposts/RequestedInstancePools)
(s/def
:cognitect.aws.outposts.StartCapacityTaskOutput/InstancesToExclude
:cognitect.aws.outposts/InstancesToExclude)
(s/def :cognitect.aws.outposts.StartCapacityTaskOutput/OutpostId :cognitect.aws.outposts/OutpostId)
(s/def
:cognitect.aws.outposts.StartCapacityTaskOutput/CreationDate
:cognitect.aws.outposts/ISO8601Timestamp)
(s/def
:cognitect.aws.outposts.CancelCapacityTaskInput/CapacityTaskId
:cognitect.aws.outposts/CapacityTaskId)
(s/def
:cognitect.aws.outposts.CancelCapacityTaskInput/OutpostIdentifier
:cognitect.aws.outposts/OutpostIdentifier)
(s/def :cognitect.aws.outposts.UpdateOutpostInput/OutpostId :cognitect.aws.outposts/OutpostId)
(s/def :cognitect.aws.outposts.UpdateOutpostInput/Name :cognitect.aws.outposts/OutpostName)
(s/def
:cognitect.aws.outposts.UpdateOutpostInput/Description
:cognitect.aws.outposts/OutpostDescription)
(s/def
:cognitect.aws.outposts.UpdateOutpostInput/SupportedHardwareType
:cognitect.aws.outposts/SupportedHardwareType)
(s/def :cognitect.aws.outposts.ListSitesOutput/Sites :cognitect.aws.outposts/siteListDefinition)
(s/def :cognitect.aws.outposts.ListSitesOutput/NextToken :cognitect.aws.outposts/Token)
(s/def :cognitect.aws.outposts.InstancesToExclude/Instances :cognitect.aws.outposts/InstanceIdList)
(s/def :cognitect.aws.outposts.InstancesToExclude/AccountIds :cognitect.aws.outposts/AccountIdList)
(s/def
:cognitect.aws.outposts.InstancesToExclude/Services
:cognitect.aws.outposts/AWSServiceNameList)
(s/def :cognitect.aws.outposts.EC2Capacity/Family :cognitect.aws.outposts/Family)
(s/def :cognitect.aws.outposts.EC2Capacity/MaxSize :cognitect.aws.outposts/MaxSize)
(s/def :cognitect.aws.outposts.EC2Capacity/Quantity :cognitect.aws.outposts/Quantity)
(s/def
:cognitect.aws.outposts.StartConnectionResponse/ConnectionId
:cognitect.aws.outposts/ConnectionId)
(s/def
:cognitect.aws.outposts.StartConnectionResponse/UnderlayIpAddress
:cognitect.aws.outposts/UnderlayIpAddress)
(s/def :cognitect.aws.outposts.GetSiteAddressOutput/SiteId :cognitect.aws.outposts/SiteId)
(s/def :cognitect.aws.outposts.GetSiteAddressOutput/AddressType :cognitect.aws.outposts/AddressType)
(s/def :cognitect.aws.outposts.GetSiteAddressOutput/Address :cognitect.aws.outposts/Address)
(s/def
:cognitect.aws.outposts.CreateOrderInput/OutpostIdentifier
:cognitect.aws.outposts/OutpostIdentifier)
(s/def
:cognitect.aws.outposts.CreateOrderInput/LineItems
:cognitect.aws.outposts/LineItemRequestListDefinition)
(s/def :cognitect.aws.outposts.CreateOrderInput/PaymentOption :cognitect.aws.outposts/PaymentOption)
(s/def :cognitect.aws.outposts.CreateOrderInput/PaymentTerm :cognitect.aws.outposts/PaymentTerm)
(s/def :cognitect.aws.outposts.ListTagsForResourceResponse/Tags :cognitect.aws.outposts/TagMap)
(s/def
:cognitect.aws.outposts.ListAssetInstancesOutput/AssetInstances
:cognitect.aws.outposts/AssetInstanceList)
(s/def :cognitect.aws.outposts.ListAssetInstancesOutput/NextToken :cognitect.aws.outposts/Token)
(s/def :cognitect.aws.outposts.Site/SiteArn :cognitect.aws.outposts/SiteArn)
(s/def :cognitect.aws.outposts.Site/OperatingAddressCity :cognitect.aws.outposts/City)
(s/def
:cognitect.aws.outposts.Site/RackPhysicalProperties
:cognitect.aws.outposts/RackPhysicalProperties)
(s/def :cognitect.aws.outposts.Site/AccountId :cognitect.aws.outposts/AccountId)
(s/def :cognitect.aws.outposts.Site/Tags :cognitect.aws.outposts/TagMap)
(s/def
:cognitect.aws.outposts.Site/OperatingAddressStateOrRegion
:cognitect.aws.outposts/StateOrRegion)
(s/def :cognitect.aws.outposts.Site/SiteId :cognitect.aws.outposts/SiteId)
(s/def :cognitect.aws.outposts.Site/OperatingAddressCountryCode :cognitect.aws.outposts/CountryCode)
(s/def :cognitect.aws.outposts.Site/Notes :cognitect.aws.outposts/SiteNotes)
(s/def :cognitect.aws.outposts.Site/Name :cognitect.aws.outposts/SiteName)
(s/def :cognitect.aws.outposts.Site/Description :cognitect.aws.outposts/SiteDescription)
(s/def :cognitect.aws.outposts.GetCatalogItemInput/CatalogItemId :cognitect.aws.outposts/SkuCode)
(s/def
:cognitect.aws.outposts.CapacityTaskSummary/CapacityTaskId
:cognitect.aws.outposts/CapacityTaskId)
(s/def :cognitect.aws.outposts.CapacityTaskSummary/OutpostId :cognitect.aws.outposts/OutpostId)
(s/def :cognitect.aws.outposts.CapacityTaskSummary/OrderId :cognitect.aws.outposts/OrderId)
(s/def
:cognitect.aws.outposts.CapacityTaskSummary/CapacityTaskStatus
:cognitect.aws.outposts/CapacityTaskStatus)
(s/def
:cognitect.aws.outposts.CapacityTaskSummary/CreationDate
:cognitect.aws.outposts/ISO8601Timestamp)
(s/def
:cognitect.aws.outposts.CapacityTaskSummary/CompletionDate
:cognitect.aws.outposts/ISO8601Timestamp)
(s/def
:cognitect.aws.outposts.CapacityTaskSummary/LastModifiedDate
:cognitect.aws.outposts/ISO8601Timestamp)
(s/def :cognitect.aws.outposts.GetOutpostOutput/Outpost :cognitect.aws.outposts/Outpost)
(s/def :cognitect.aws.outposts.CreateOutpostOutput/Outpost :cognitect.aws.outposts/Outpost)
(s/def :cognitect.aws.outposts.LineItemAssetInformation/AssetId :cognitect.aws.outposts/AssetId)
(s/def
:cognitect.aws.outposts.LineItemAssetInformation/MacAddressList
:cognitect.aws.outposts/MacAddressList)
(s/def :cognitect.aws.outposts.GetSiteAddressInput/SiteId :cognitect.aws.outposts/SiteId)
(s/def :cognitect.aws.outposts.GetSiteAddressInput/AddressType :cognitect.aws.outposts/AddressType)
(s/def
:cognitect.aws.outposts.ListCatalogItemsOutput/CatalogItems
:cognitect.aws.outposts/CatalogItemListDefinition)
(s/def :cognitect.aws.outposts.ListCatalogItemsOutput/NextToken :cognitect.aws.outposts/Token)
(s/def :cognitect.aws.outposts.LineItemRequest/CatalogItemId :cognitect.aws.outposts/SkuCode)
(s/def :cognitect.aws.outposts.LineItemRequest/Quantity :cognitect.aws.outposts/LineItemQuantity)
(s/def :cognitect.aws.outposts.ConflictException/Message :cognitect.aws.outposts/ErrorMessage)
(s/def :cognitect.aws.outposts.ConflictException/ResourceId :cognitect.aws.outposts/String)
(s/def :cognitect.aws.outposts.ConflictException/ResourceType :cognitect.aws.outposts/ResourceType)
(s/def
:cognitect.aws.outposts.GetConnectionRequest/ConnectionId
:cognitect.aws.outposts/ConnectionId)
(s/def :cognitect.aws.outposts.Order/OrderSubmissionDate :cognitect.aws.outposts/ISO8601Timestamp)
(s/def :cognitect.aws.outposts.Order/LineItems :cognitect.aws.outposts/LineItemListDefinition)
(s/def :cognitect.aws.outposts.Order/OrderId :cognitect.aws.outposts/OrderId)
(s/def :cognitect.aws.outposts.Order/OrderFulfilledDate :cognitect.aws.outposts/ISO8601Timestamp)
(s/def :cognitect.aws.outposts.Order/PaymentTerm :cognitect.aws.outposts/PaymentTerm)
(s/def :cognitect.aws.outposts.Order/PaymentOption :cognitect.aws.outposts/PaymentOption)
(s/def :cognitect.aws.outposts.Order/OrderType :cognitect.aws.outposts/OrderType)
(s/def :cognitect.aws.outposts.Order/OutpostId :cognitect.aws.outposts/OutpostIdOnly)
(s/def :cognitect.aws.outposts.Order/Status :cognitect.aws.outposts/OrderStatus)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy