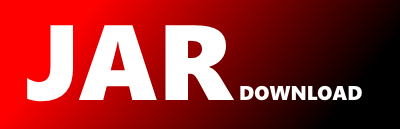
cognitect.aws.runtime_sagemaker.specs.clj Maven / Gradle / Ivy
;; Copyright (c) Cognitect, Inc.
;; All rights reserved.
(ns cognitect.aws.runtime-sagemaker.specs
(:require [clojure.spec.alpha :as s] [clojure.spec.gen.alpha :as gen]))
(s/def :cognitect.aws/client map?)
(s/def :core.async/channel any?)
(s/def
:cognitect.aws.runtime-sagemaker/InvocationTimeoutSecondsHeader
(s/spec (s/and int? #(<= 1 % 3600)) :gen #(gen/choose 1 3600)))
(s/def :cognitect.aws.runtime-sagemaker/InvokeEndpointAsyncInput (s/keys))
(s/def
:cognitect.aws.runtime-sagemaker/EndpointName
(s/spec #(re-matches (re-pattern "^[a-zA-Z0-9](-*[a-zA-Z0-9])*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.runtime-sagemaker/TargetContainerHostnameHeader
(s/spec #(re-matches (re-pattern "^[a-zA-Z0-9](-*[a-zA-Z0-9])*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.runtime-sagemaker/TargetVariantHeader
(s/spec #(re-matches (re-pattern "^[a-zA-Z0-9](-*[a-zA-Z0-9])*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.runtime-sagemaker/Header
(s/spec #(re-matches (re-pattern "\\p{ASCII}*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.runtime-sagemaker/RequestTTLSecondsHeader
(s/spec (s/and int? #(<= 60 % 21600)) :gen #(gen/choose 60 21600)))
(s/def
:cognitect.aws.runtime-sagemaker/EnableExplanationsHeader
(s/spec #(re-matches (re-pattern ".*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.runtime-sagemaker/InferenceId
(s/spec #(re-matches (re-pattern "\\A\\S[\\p{Print}]*\\z") %) :gen #(gen/string)))
(s/def :cognitect.aws.runtime-sagemaker/InvokeEndpointAsyncOutput (s/keys))
(s/def :cognitect.aws.runtime-sagemaker/InvokeEndpointInput (s/keys))
(s/def
:cognitect.aws.runtime-sagemaker/BodyBlob
(s/spec
(s/or
:byte-array
(s/and bytes? #(>= 6291456 (count %)))
:input-stream
#(instance? java.io.InputStream %))
:gen
(fn []
(gen/bind
(gen/choose (or nil 0) (or 6291456 100))
#(gen/return (byte-array % (repeatedly (fn [] (rand-int 256)))))))))
(s/def
:cognitect.aws.runtime-sagemaker/InputLocationHeader
(s/spec #(re-matches (re-pattern "^(https|s3)://([^/]+)/?(.*)$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.runtime-sagemaker/CustomAttributesHeader
(s/spec #(re-matches (re-pattern "\\p{ASCII}*") %) :gen #(gen/string)))
(s/def :cognitect.aws.runtime-sagemaker/InvokeEndpointOutput (s/keys))
(s/def
:cognitect.aws.runtime-sagemaker/TargetModelHeader
(s/spec #(re-matches (re-pattern "\\A\\S[\\p{Print}]*\\z") %) :gen #(gen/string)))
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointAsyncInput/EndpointName
:cognitect.aws.runtime-sagemaker/EndpointName)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointAsyncInput/ContentType
:cognitect.aws.runtime-sagemaker/Header)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointAsyncInput/Accept
:cognitect.aws.runtime-sagemaker/Header)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointAsyncInput/CustomAttributes
:cognitect.aws.runtime-sagemaker/CustomAttributesHeader)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointAsyncInput/InferenceId
:cognitect.aws.runtime-sagemaker/InferenceId)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointAsyncInput/InputLocation
:cognitect.aws.runtime-sagemaker/InputLocationHeader)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointAsyncInput/RequestTTLSeconds
:cognitect.aws.runtime-sagemaker/RequestTTLSecondsHeader)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointAsyncInput/InvocationTimeoutSeconds
:cognitect.aws.runtime-sagemaker/InvocationTimeoutSecondsHeader)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointAsyncOutput/InferenceId
:cognitect.aws.runtime-sagemaker/Header)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointAsyncOutput/OutputLocation
:cognitect.aws.runtime-sagemaker/Header)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointAsyncOutput/FailureLocation
:cognitect.aws.runtime-sagemaker/Header)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointInput/EnableExplanations
:cognitect.aws.runtime-sagemaker/EnableExplanationsHeader)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointInput/EndpointName
:cognitect.aws.runtime-sagemaker/EndpointName)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointInput/TargetVariant
:cognitect.aws.runtime-sagemaker/TargetVariantHeader)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointInput/CustomAttributes
:cognitect.aws.runtime-sagemaker/CustomAttributesHeader)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointInput/InferenceId
:cognitect.aws.runtime-sagemaker/InferenceId)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointInput/TargetModel
:cognitect.aws.runtime-sagemaker/TargetModelHeader)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointInput/Accept
:cognitect.aws.runtime-sagemaker/Header)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointInput/Body
:cognitect.aws.runtime-sagemaker/BodyBlob)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointInput/TargetContainerHostname
:cognitect.aws.runtime-sagemaker/TargetContainerHostnameHeader)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointInput/ContentType
:cognitect.aws.runtime-sagemaker/Header)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointOutput/Body
:cognitect.aws.runtime-sagemaker/BodyBlob)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointOutput/ContentType
:cognitect.aws.runtime-sagemaker/Header)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointOutput/InvokedProductionVariant
:cognitect.aws.runtime-sagemaker/Header)
(s/def
:cognitect.aws.runtime-sagemaker.InvokeEndpointOutput/CustomAttributes
:cognitect.aws.runtime-sagemaker/CustomAttributesHeader)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy