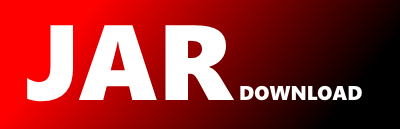
cognitect.aws.sagemaker_a2i_runtime.specs.clj Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sagemaker-a2i-runtime
Show all versions of sagemaker-a2i-runtime
sagemaker-a2i-runtime resources for com.cognitect.aws/api
;; Copyright (c) Cognitect, Inc.
;; All rights reserved.
(ns cognitect.aws.sagemaker-a2i-runtime.specs
(:require [clojure.spec.alpha :as s] [clojure.spec.gen.alpha :as gen]))
(s/def :cognitect.aws/client map?)
(s/def :core.async/channel any?)
(s/def
:cognitect.aws.sagemaker-a2i-runtime/ListHumanLoopsRequest
(s/keys
:opt-un
[:cognitect.aws.sagemaker-a2i-runtime.ListHumanLoopsRequest/CreationTimeAfter
:cognitect.aws.sagemaker-a2i-runtime.ListHumanLoopsRequest/CreationTimeBefore
:cognitect.aws.sagemaker-a2i-runtime.ListHumanLoopsRequest/SortOrder
:cognitect.aws.sagemaker-a2i-runtime.ListHumanLoopsRequest/NextToken
:cognitect.aws.sagemaker-a2i-runtime.ListHumanLoopsRequest/MaxResults]))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/ContentClassifier
(s/spec string? :gen #(s/gen #{"FreeOfAdultContent" "FreeOfPersonallyIdentifiableInformation"})))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/StopHumanLoopRequest
(s/keys :req-un [:cognitect.aws.sagemaker-a2i-runtime.StopHumanLoopRequest/HumanLoopName]))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/StartHumanLoopResponse
(s/keys
:opt-un
[:cognitect.aws.sagemaker-a2i-runtime.StartHumanLoopResponse/HumanLoopArn
:cognitect.aws.sagemaker-a2i-runtime.StartHumanLoopResponse/HumanLoopActivationResults]))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/InputContent
(s/spec
(s/and string? #(>= 4194304 (count %)))
:gen
(fn []
(gen/fmap
#(apply str %)
(gen/bind (gen/choose 0 4194304) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/ListHumanLoopsResponse
(s/keys
:req-un
[:cognitect.aws.sagemaker-a2i-runtime.ListHumanLoopsResponse/HumanLoopSummaries]
:opt-un
[:cognitect.aws.sagemaker-a2i-runtime.ListHumanLoopsResponse/NextToken]))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/DescribeHumanLoopRequest
(s/keys :req-un [:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopRequest/HumanLoopName]))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopInputContent
(s/keys :req-un [:cognitect.aws.sagemaker-a2i-runtime.HumanLoopInputContent/InputContent]))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopActivationReason
(s/keys
:opt-un
[:cognitect.aws.sagemaker-a2i-runtime.HumanLoopActivationReason/ConditionsMatched]))
(s/def :cognitect.aws.sagemaker-a2i-runtime/Boolean boolean?)
(s/def
:cognitect.aws.sagemaker-a2i-runtime/HumanReviewDataAttributes
(s/keys
:req-un
[:cognitect.aws.sagemaker-a2i-runtime.HumanReviewDataAttributes/ContentClassifiers]))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/NextToken
(s/spec #(re-matches (re-pattern ".*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopActivationResults
(s/keys
:opt-un
[:cognitect.aws.sagemaker-a2i-runtime.HumanLoopActivationResults/HumanLoopActivationConditionsEvaluationResults
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopActivationResults/HumanLoopActivationReason]))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/DeleteHumanLoopRequest
(s/keys :req-un [:cognitect.aws.sagemaker-a2i-runtime.DeleteHumanLoopRequest/HumanLoopName]))
(s/def :cognitect.aws.sagemaker-a2i-runtime/DeleteHumanLoopResponse (s/keys))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopStatus
(s/spec string? :gen #(s/gen #{"Failed" "InProgress" "Stopping" "Stopped" "Completed"})))
(s/def :cognitect.aws.sagemaker-a2i-runtime/StopHumanLoopResponse (s/keys))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopSummary
(s/keys
:opt-un
[:cognitect.aws.sagemaker-a2i-runtime.HumanLoopSummary/FlowDefinitionArn
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopSummary/FailureReason
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopSummary/CreationTime
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopSummary/HumanLoopName
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopSummary/HumanLoopStatus]))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopSummaries
(s/coll-of :cognitect.aws.sagemaker-a2i-runtime/HumanLoopSummary))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/SortOrder
(s/spec string? :gen #(s/gen #{"Descending" "Ascending"})))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/ContentClassifiers
(s/coll-of :cognitect.aws.sagemaker-a2i-runtime/ContentClassifier :max-count 256))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/DescribeHumanLoopResponse
(s/keys
:req-un
[:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/CreationTimestamp
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/HumanLoopStatus
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/HumanLoopName
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/HumanLoopArn
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/FlowDefinitionArn
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/HumanLoopInput]
:opt-un
[:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/HumanLoopOutput
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/FailureReason
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/FailureCode]))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/MaxResults
(s/spec (s/and int? #(<= 1 % 100)) :gen #(gen/choose 1 100)))
(s/def :cognitect.aws.sagemaker-a2i-runtime/Timestamp inst?)
(s/def
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopOutputContent
(s/keys :req-un [:cognitect.aws.sagemaker-a2i-runtime.HumanLoopOutputContent/OutputS3Uri]))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopArn
(s/spec
#(re-matches (re-pattern "arn:aws[a-z\\-]*:sagemaker:[a-z0-9\\-]*:[0-9]{12}:human-loop/.*") %)
:gen
#(gen/string)))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/FlowDefinitionArn
(s/spec
#(re-matches
(re-pattern "arn:aws[a-z\\-]*:sagemaker:[a-z0-9\\-]*:[0-9]{12}:flow-definition/.*")
%)
:gen
#(gen/string)))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopName
(s/spec #(re-matches (re-pattern "^[a-z0-9](-*[a-z0-9])*$") %) :gen #(gen/string)))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/FailureReason
(s/spec
(s/and string? #(>= 1024 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 1024) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.sagemaker-a2i-runtime/StartHumanLoopRequest
(s/keys
:req-un
[:cognitect.aws.sagemaker-a2i-runtime.StartHumanLoopRequest/HumanLoopName
:cognitect.aws.sagemaker-a2i-runtime.StartHumanLoopRequest/FlowDefinitionArn
:cognitect.aws.sagemaker-a2i-runtime.StartHumanLoopRequest/HumanLoopInput]
:opt-un
[:cognitect.aws.sagemaker-a2i-runtime.StartHumanLoopRequest/DataAttributes]))
(s/def :cognitect.aws.sagemaker-a2i-runtime/String string?)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.ListHumanLoopsRequest/CreationTimeAfter
:cognitect.aws.sagemaker-a2i-runtime/Timestamp)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.ListHumanLoopsRequest/CreationTimeBefore
:cognitect.aws.sagemaker-a2i-runtime/Timestamp)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.ListHumanLoopsRequest/SortOrder
:cognitect.aws.sagemaker-a2i-runtime/SortOrder)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.ListHumanLoopsRequest/NextToken
:cognitect.aws.sagemaker-a2i-runtime/NextToken)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.ListHumanLoopsRequest/MaxResults
:cognitect.aws.sagemaker-a2i-runtime/MaxResults)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.StopHumanLoopRequest/HumanLoopName
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopName)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.StartHumanLoopResponse/HumanLoopArn
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopArn)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.StartHumanLoopResponse/HumanLoopActivationResults
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopActivationResults)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.ListHumanLoopsResponse/HumanLoopSummaries
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopSummaries)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.ListHumanLoopsResponse/NextToken
:cognitect.aws.sagemaker-a2i-runtime/NextToken)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopRequest/HumanLoopName
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopName)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopInputContent/InputContent
:cognitect.aws.sagemaker-a2i-runtime/InputContent)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopActivationReason/ConditionsMatched
:cognitect.aws.sagemaker-a2i-runtime/Boolean)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.HumanReviewDataAttributes/ContentClassifiers
:cognitect.aws.sagemaker-a2i-runtime/ContentClassifiers)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopActivationResults/HumanLoopActivationReason
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopActivationReason)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopActivationResults/HumanLoopActivationConditionsEvaluationResults
:cognitect.aws.sagemaker-a2i-runtime/String)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.DeleteHumanLoopRequest/HumanLoopName
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopName)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopSummary/HumanLoopName
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopName)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopSummary/HumanLoopStatus
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopStatus)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopSummary/CreationTime
:cognitect.aws.sagemaker-a2i-runtime/Timestamp)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopSummary/FailureReason
:cognitect.aws.sagemaker-a2i-runtime/FailureReason)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopSummary/FlowDefinitionArn
:cognitect.aws.sagemaker-a2i-runtime/FlowDefinitionArn)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/CreationTimestamp
:cognitect.aws.sagemaker-a2i-runtime/Timestamp)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/HumanLoopOutput
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopOutputContent)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/HumanLoopStatus
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopStatus)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/FailureCode
:cognitect.aws.sagemaker-a2i-runtime/String)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/HumanLoopArn
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopArn)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/FlowDefinitionArn
:cognitect.aws.sagemaker-a2i-runtime/FlowDefinitionArn)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/HumanLoopName
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopName)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/FailureReason
:cognitect.aws.sagemaker-a2i-runtime/String)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.DescribeHumanLoopResponse/HumanLoopInput
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopInputContent)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.HumanLoopOutputContent/OutputS3Uri
:cognitect.aws.sagemaker-a2i-runtime/String)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.StartHumanLoopRequest/HumanLoopName
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopName)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.StartHumanLoopRequest/FlowDefinitionArn
:cognitect.aws.sagemaker-a2i-runtime/FlowDefinitionArn)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.StartHumanLoopRequest/HumanLoopInput
:cognitect.aws.sagemaker-a2i-runtime/HumanLoopInputContent)
(s/def
:cognitect.aws.sagemaker-a2i-runtime.StartHumanLoopRequest/DataAttributes
:cognitect.aws.sagemaker-a2i-runtime/HumanReviewDataAttributes)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy