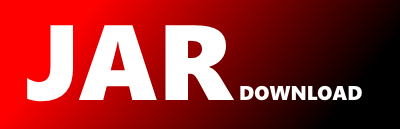
cognitect.aws.sagemaker_featurestore_runtime.specs.clj Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sagemaker-featurestore-runtime
Show all versions of sagemaker-featurestore-runtime
sagemaker-featurestore-runtime resources for com.cognitect.aws/api
;; Copyright (c) Cognitect, Inc.
;; All rights reserved.
(ns cognitect.aws.sagemaker-featurestore-runtime.specs
(:require [clojure.spec.alpha :as s] [clojure.spec.gen.alpha :as gen]))
(s/def :cognitect.aws/client map?)
(s/def :core.async/channel any?)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/Record
(s/coll-of :cognitect.aws.sagemaker-featurestore-runtime/FeatureValue :min-count 1))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordRequest
(s/keys
:req-un
[:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordRequest/Identifiers]))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/Message
(s/spec
(s/and string? #(>= 2048 (count %)))
:gen
(fn []
(gen/fmap #(apply str %) (gen/bind (gen/choose 0 2048) #(gen/vector (gen/char-alpha) %))))))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/GetRecordRequest
(s/keys
:req-un
[:cognitect.aws.sagemaker-featurestore-runtime.GetRecordRequest/FeatureGroupName
:cognitect.aws.sagemaker-featurestore-runtime.GetRecordRequest/RecordIdentifierValueAsString]
:opt-un
[:cognitect.aws.sagemaker-featurestore-runtime.GetRecordRequest/FeatureNames]))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/FeatureGroupName
(s/spec #(re-matches (re-pattern "^[a-zA-Z0-9](-*[a-zA-Z0-9])*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/PutRecordRequest
(s/keys
:req-un
[:cognitect.aws.sagemaker-featurestore-runtime.PutRecordRequest/FeatureGroupName
:cognitect.aws.sagemaker-featurestore-runtime.PutRecordRequest/Record]))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordResultDetail
(s/keys
:req-un
[:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordResultDetail/FeatureGroupName
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordResultDetail/RecordIdentifierValueAsString
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordResultDetail/Record]))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/GetRecordResponse
(s/keys :opt-un [:cognitect.aws.sagemaker-featurestore-runtime.GetRecordResponse/Record]))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordIdentifiers
(s/coll-of
:cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordIdentifier
:min-count
1
:max-count
10))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/UnprocessedIdentifiers
(s/coll-of :cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordIdentifier :min-count 0))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordIdentifier
(s/keys
:req-un
[:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordIdentifier/FeatureGroupName
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordIdentifier/RecordIdentifiersValueAsString]
:opt-un
[:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordIdentifier/FeatureNames]))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordErrors
(s/coll-of :cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordError :min-count 0))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordError
(s/keys
:req-un
[:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordError/FeatureGroupName
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordError/RecordIdentifierValueAsString
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordError/ErrorCode
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordError/ErrorMessage]))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordResponse
(s/keys
:req-un
[:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordResponse/Records
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordResponse/Errors
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordResponse/UnprocessedIdentifiers]))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/FeatureName
(s/spec #(re-matches (re-pattern "^[a-zA-Z0-9]([-_]*[a-zA-Z0-9])*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/FeatureNames
(s/coll-of :cognitect.aws.sagemaker-featurestore-runtime/FeatureName :min-count 1))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/ValueAsString
(s/spec #(re-matches (re-pattern ".*") %) :gen #(gen/string)))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/DeleteRecordRequest
(s/keys
:req-un
[:cognitect.aws.sagemaker-featurestore-runtime.DeleteRecordRequest/FeatureGroupName
:cognitect.aws.sagemaker-featurestore-runtime.DeleteRecordRequest/RecordIdentifierValueAsString
:cognitect.aws.sagemaker-featurestore-runtime.DeleteRecordRequest/EventTime]))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/FeatureValue
(s/keys
:req-un
[:cognitect.aws.sagemaker-featurestore-runtime.FeatureValue/FeatureName
:cognitect.aws.sagemaker-featurestore-runtime.FeatureValue/ValueAsString]))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordResultDetails
(s/coll-of :cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordResultDetail :min-count 0))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime/RecordIdentifiers
(s/coll-of
:cognitect.aws.sagemaker-featurestore-runtime/ValueAsString
:min-count
1
:max-count
100))
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordRequest/Identifiers
:cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordIdentifiers)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.GetRecordRequest/FeatureGroupName
:cognitect.aws.sagemaker-featurestore-runtime/FeatureGroupName)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.GetRecordRequest/RecordIdentifierValueAsString
:cognitect.aws.sagemaker-featurestore-runtime/ValueAsString)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.GetRecordRequest/FeatureNames
:cognitect.aws.sagemaker-featurestore-runtime/FeatureNames)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.PutRecordRequest/FeatureGroupName
:cognitect.aws.sagemaker-featurestore-runtime/FeatureGroupName)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.PutRecordRequest/Record
:cognitect.aws.sagemaker-featurestore-runtime/Record)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordResultDetail/FeatureGroupName
:cognitect.aws.sagemaker-featurestore-runtime/ValueAsString)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordResultDetail/RecordIdentifierValueAsString
:cognitect.aws.sagemaker-featurestore-runtime/ValueAsString)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordResultDetail/Record
:cognitect.aws.sagemaker-featurestore-runtime/Record)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.GetRecordResponse/Record
:cognitect.aws.sagemaker-featurestore-runtime/Record)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordIdentifier/FeatureGroupName
:cognitect.aws.sagemaker-featurestore-runtime/FeatureGroupName)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordIdentifier/RecordIdentifiersValueAsString
:cognitect.aws.sagemaker-featurestore-runtime/RecordIdentifiers)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordIdentifier/FeatureNames
:cognitect.aws.sagemaker-featurestore-runtime/FeatureNames)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordError/FeatureGroupName
:cognitect.aws.sagemaker-featurestore-runtime/ValueAsString)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordError/RecordIdentifierValueAsString
:cognitect.aws.sagemaker-featurestore-runtime/ValueAsString)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordError/ErrorCode
:cognitect.aws.sagemaker-featurestore-runtime/ValueAsString)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordError/ErrorMessage
:cognitect.aws.sagemaker-featurestore-runtime/Message)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordResponse/Records
:cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordResultDetails)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordResponse/Errors
:cognitect.aws.sagemaker-featurestore-runtime/BatchGetRecordErrors)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.BatchGetRecordResponse/UnprocessedIdentifiers
:cognitect.aws.sagemaker-featurestore-runtime/UnprocessedIdentifiers)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.DeleteRecordRequest/FeatureGroupName
:cognitect.aws.sagemaker-featurestore-runtime/FeatureGroupName)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.DeleteRecordRequest/RecordIdentifierValueAsString
:cognitect.aws.sagemaker-featurestore-runtime/ValueAsString)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.DeleteRecordRequest/EventTime
:cognitect.aws.sagemaker-featurestore-runtime/ValueAsString)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.FeatureValue/FeatureName
:cognitect.aws.sagemaker-featurestore-runtime/FeatureName)
(s/def
:cognitect.aws.sagemaker-featurestore-runtime.FeatureValue/ValueAsString
:cognitect.aws.sagemaker-featurestore-runtime/ValueAsString)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy