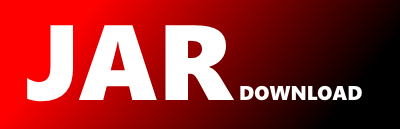
com.cohere.api.requests.EmbedRequest Maven / Gradle / Ivy
Show all versions of cohere-java Show documentation
/**
* This file was auto-generated by Fern from our API Definition.
*/
package com.cohere.api.requests;
import com.cohere.api.core.ObjectMappers;
import com.cohere.api.types.EmbedInputType;
import com.cohere.api.types.EmbedRequestTruncate;
import com.cohere.api.types.EmbeddingType;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSetter;
import com.fasterxml.jackson.annotation.Nulls;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@JsonDeserialize(builder = EmbedRequest.Builder.class)
public final class EmbedRequest {
private final Optional> texts;
private final Optional> images;
private final Optional model;
private final Optional inputType;
private final Optional> embeddingTypes;
private final Optional truncate;
private final Map additionalProperties;
private EmbedRequest(
Optional> texts,
Optional> images,
Optional model,
Optional inputType,
Optional> embeddingTypes,
Optional truncate,
Map additionalProperties) {
this.texts = texts;
this.images = images;
this.model = model;
this.inputType = inputType;
this.embeddingTypes = embeddingTypes;
this.truncate = truncate;
this.additionalProperties = additionalProperties;
}
/**
* @return An array of strings for the model to embed. Maximum number of texts per call is 96
. We recommend reducing the length of each text to be under 512
tokens for optimal quality.
*/
@JsonProperty("texts")
public Optional> getTexts() {
return texts;
}
/**
* @return An array of image data URIs for the model to embed. Maximum number of images per call is 1
.
* The image must be a valid data URI. The image must be in either image/jpeg
or image/png
format and has a maximum size of 5MB.
*/
@JsonProperty("images")
public Optional> getImages() {
return images;
}
/**
* @return Defaults to embed-english-v2.0
* The identifier of the model. Smaller "light" models are faster, while larger models will perform better. Custom models can also be supplied with their full ID.
* Available models and corresponding embedding dimensions:
*
* -
*
embed-english-v3.0
1024
*
* -
*
embed-multilingual-v3.0
1024
*
* -
*
embed-english-light-v3.0
384
*
* -
*
embed-multilingual-light-v3.0
384
*
* -
*
embed-english-v2.0
4096
*
* -
*
embed-english-light-v2.0
1024
*
* -
*
embed-multilingual-v2.0
768
*
*
*/
@JsonProperty("model")
public Optional getModel() {
return model;
}
@JsonProperty("input_type")
public Optional getInputType() {
return inputType;
}
/**
* @return Specifies the types of embeddings you want to get back. Not required and default is None, which returns the Embed Floats response type. Can be one or more of the following types.
*
* "float"
: Use this when you want to get back the default float embeddings. Valid for all models.
* "int8"
: Use this when you want to get back signed int8 embeddings. Valid for only v3 models.
* "uint8"
: Use this when you want to get back unsigned int8 embeddings. Valid for only v3 models.
* "binary"
: Use this when you want to get back signed binary embeddings. Valid for only v3 models.
* "ubinary"
: Use this when you want to get back unsigned binary embeddings. Valid for only v3 models.
*
*/
@JsonProperty("embedding_types")
public Optional> getEmbeddingTypes() {
return embeddingTypes;
}
/**
* @return One of NONE|START|END
to specify how the API will handle inputs longer than the maximum token length.
* Passing START
will discard the start of the input. END
will discard the end of the input. In both cases, input is discarded until the remaining input is exactly the maximum input token length for the model.
* If NONE
is selected, when the input exceeds the maximum input token length an error will be returned.
*/
@JsonProperty("truncate")
public Optional getTruncate() {
return truncate;
}
@java.lang.Override
public boolean equals(Object other) {
if (this == other) return true;
return other instanceof EmbedRequest && equalTo((EmbedRequest) other);
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
private boolean equalTo(EmbedRequest other) {
return texts.equals(other.texts)
&& images.equals(other.images)
&& model.equals(other.model)
&& inputType.equals(other.inputType)
&& embeddingTypes.equals(other.embeddingTypes)
&& truncate.equals(other.truncate);
}
@java.lang.Override
public int hashCode() {
return Objects.hash(this.texts, this.images, this.model, this.inputType, this.embeddingTypes, this.truncate);
}
@java.lang.Override
public String toString() {
return ObjectMappers.stringify(this);
}
public static Builder builder() {
return new Builder();
}
@JsonIgnoreProperties(ignoreUnknown = true)
public static final class Builder {
private Optional> texts = Optional.empty();
private Optional> images = Optional.empty();
private Optional model = Optional.empty();
private Optional inputType = Optional.empty();
private Optional> embeddingTypes = Optional.empty();
private Optional truncate = Optional.empty();
@JsonAnySetter
private Map additionalProperties = new HashMap<>();
private Builder() {}
public Builder from(EmbedRequest other) {
texts(other.getTexts());
images(other.getImages());
model(other.getModel());
inputType(other.getInputType());
embeddingTypes(other.getEmbeddingTypes());
truncate(other.getTruncate());
return this;
}
@JsonSetter(value = "texts", nulls = Nulls.SKIP)
public Builder texts(Optional> texts) {
this.texts = texts;
return this;
}
public Builder texts(List texts) {
this.texts = Optional.of(texts);
return this;
}
@JsonSetter(value = "images", nulls = Nulls.SKIP)
public Builder images(Optional> images) {
this.images = images;
return this;
}
public Builder images(List images) {
this.images = Optional.of(images);
return this;
}
@JsonSetter(value = "model", nulls = Nulls.SKIP)
public Builder model(Optional model) {
this.model = model;
return this;
}
public Builder model(String model) {
this.model = Optional.of(model);
return this;
}
@JsonSetter(value = "input_type", nulls = Nulls.SKIP)
public Builder inputType(Optional inputType) {
this.inputType = inputType;
return this;
}
public Builder inputType(EmbedInputType inputType) {
this.inputType = Optional.of(inputType);
return this;
}
@JsonSetter(value = "embedding_types", nulls = Nulls.SKIP)
public Builder embeddingTypes(Optional> embeddingTypes) {
this.embeddingTypes = embeddingTypes;
return this;
}
public Builder embeddingTypes(List embeddingTypes) {
this.embeddingTypes = Optional.of(embeddingTypes);
return this;
}
@JsonSetter(value = "truncate", nulls = Nulls.SKIP)
public Builder truncate(Optional truncate) {
this.truncate = truncate;
return this;
}
public Builder truncate(EmbedRequestTruncate truncate) {
this.truncate = Optional.of(truncate);
return this;
}
public EmbedRequest build() {
return new EmbedRequest(texts, images, model, inputType, embeddingTypes, truncate, additionalProperties);
}
}
}