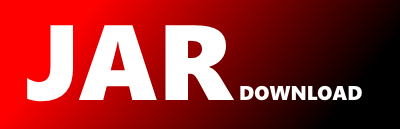
com.cohere.api.requests.SummarizeRequest Maven / Gradle / Ivy
Show all versions of cohere-java Show documentation
/**
* This file was auto-generated by Fern from our API Definition.
*/
package com.cohere.api.requests;
import com.cohere.api.core.ObjectMappers;
import com.cohere.api.types.SummarizeRequestExtractiveness;
import com.cohere.api.types.SummarizeRequestFormat;
import com.cohere.api.types.SummarizeRequestLength;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSetter;
import com.fasterxml.jackson.annotation.Nulls;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@JsonDeserialize(builder = SummarizeRequest.Builder.class)
public final class SummarizeRequest {
private final String text;
private final Optional length;
private final Optional format;
private final Optional model;
private final Optional extractiveness;
private final Optional temperature;
private final Optional additionalCommand;
private final Map additionalProperties;
private SummarizeRequest(
String text,
Optional length,
Optional format,
Optional model,
Optional extractiveness,
Optional temperature,
Optional additionalCommand,
Map additionalProperties) {
this.text = text;
this.length = length;
this.format = format;
this.model = model;
this.extractiveness = extractiveness;
this.temperature = temperature;
this.additionalCommand = additionalCommand;
this.additionalProperties = additionalProperties;
}
/**
* @return The text to generate a summary for. Can be up to 100,000 characters long. Currently the only supported language is English.
*/
@JsonProperty("text")
public String getText() {
return text;
}
/**
* @return One of short
, medium
, long
, or auto
defaults to auto
. Indicates the approximate length of the summary. If auto
is selected, the best option will be picked based on the input text.
*/
@JsonProperty("length")
public Optional getLength() {
return length;
}
/**
* @return One of paragraph
, bullets
, or auto
, defaults to auto
. Indicates the style in which the summary will be delivered - in a free form paragraph or in bullet points. If auto
is selected, the best option will be picked based on the input text.
*/
@JsonProperty("format")
public Optional getFormat() {
return format;
}
/**
* @return The identifier of the model to generate the summary with. Currently available models are command
(default), command-nightly
(experimental), command-light
, and command-light-nightly
(experimental). Smaller, "light" models are faster, while larger models will perform better.
*/
@JsonProperty("model")
public Optional getModel() {
return model;
}
/**
* @return One of low
, medium
, high
, or auto
, defaults to auto
. Controls how close to the original text the summary is. high
extractiveness summaries will lean towards reusing sentences verbatim, while low
extractiveness summaries will tend to paraphrase more. If auto
is selected, the best option will be picked based on the input text.
*/
@JsonProperty("extractiveness")
public Optional getExtractiveness() {
return extractiveness;
}
/**
* @return Ranges from 0 to 5. Controls the randomness of the output. Lower values tend to generate more “predictable” output, while higher values tend to generate more “creative” output. The sweet spot is typically between 0 and 1.
*/
@JsonProperty("temperature")
public Optional getTemperature() {
return temperature;
}
/**
* @return A free-form instruction for modifying how the summaries get generated. Should complete the sentence "Generate a summary _". Eg. "focusing on the next steps" or "written by Yoda"
*/
@JsonProperty("additional_command")
public Optional getAdditionalCommand() {
return additionalCommand;
}
@java.lang.Override
public boolean equals(Object other) {
if (this == other) return true;
return other instanceof SummarizeRequest && equalTo((SummarizeRequest) other);
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
private boolean equalTo(SummarizeRequest other) {
return text.equals(other.text)
&& length.equals(other.length)
&& format.equals(other.format)
&& model.equals(other.model)
&& extractiveness.equals(other.extractiveness)
&& temperature.equals(other.temperature)
&& additionalCommand.equals(other.additionalCommand);
}
@java.lang.Override
public int hashCode() {
return Objects.hash(
this.text,
this.length,
this.format,
this.model,
this.extractiveness,
this.temperature,
this.additionalCommand);
}
@java.lang.Override
public String toString() {
return ObjectMappers.stringify(this);
}
public static TextStage builder() {
return new Builder();
}
public interface TextStage {
_FinalStage text(String text);
Builder from(SummarizeRequest other);
}
public interface _FinalStage {
SummarizeRequest build();
_FinalStage length(Optional length);
_FinalStage length(SummarizeRequestLength length);
_FinalStage format(Optional format);
_FinalStage format(SummarizeRequestFormat format);
_FinalStage model(Optional model);
_FinalStage model(String model);
_FinalStage extractiveness(Optional extractiveness);
_FinalStage extractiveness(SummarizeRequestExtractiveness extractiveness);
_FinalStage temperature(Optional temperature);
_FinalStage temperature(Double temperature);
_FinalStage additionalCommand(Optional additionalCommand);
_FinalStage additionalCommand(String additionalCommand);
}
@JsonIgnoreProperties(ignoreUnknown = true)
public static final class Builder implements TextStage, _FinalStage {
private String text;
private Optional additionalCommand = Optional.empty();
private Optional temperature = Optional.empty();
private Optional extractiveness = Optional.empty();
private Optional model = Optional.empty();
private Optional format = Optional.empty();
private Optional length = Optional.empty();
@JsonAnySetter
private Map additionalProperties = new HashMap<>();
private Builder() {}
@java.lang.Override
public Builder from(SummarizeRequest other) {
text(other.getText());
length(other.getLength());
format(other.getFormat());
model(other.getModel());
extractiveness(other.getExtractiveness());
temperature(other.getTemperature());
additionalCommand(other.getAdditionalCommand());
return this;
}
/**
* The text to generate a summary for. Can be up to 100,000 characters long. Currently the only supported language is English.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
@JsonSetter("text")
public _FinalStage text(String text) {
this.text = text;
return this;
}
/**
* A free-form instruction for modifying how the summaries get generated. Should complete the sentence "Generate a summary _". Eg. "focusing on the next steps" or "written by Yoda"
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage additionalCommand(String additionalCommand) {
this.additionalCommand = Optional.of(additionalCommand);
return this;
}
@java.lang.Override
@JsonSetter(value = "additional_command", nulls = Nulls.SKIP)
public _FinalStage additionalCommand(Optional additionalCommand) {
this.additionalCommand = additionalCommand;
return this;
}
/**
* Ranges from 0 to 5. Controls the randomness of the output. Lower values tend to generate more “predictable” output, while higher values tend to generate more “creative” output. The sweet spot is typically between 0 and 1.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage temperature(Double temperature) {
this.temperature = Optional.of(temperature);
return this;
}
@java.lang.Override
@JsonSetter(value = "temperature", nulls = Nulls.SKIP)
public _FinalStage temperature(Optional temperature) {
this.temperature = temperature;
return this;
}
/**
* One of low
, medium
, high
, or auto
, defaults to auto
. Controls how close to the original text the summary is. high
extractiveness summaries will lean towards reusing sentences verbatim, while low
extractiveness summaries will tend to paraphrase more. If auto
is selected, the best option will be picked based on the input text.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage extractiveness(SummarizeRequestExtractiveness extractiveness) {
this.extractiveness = Optional.of(extractiveness);
return this;
}
@java.lang.Override
@JsonSetter(value = "extractiveness", nulls = Nulls.SKIP)
public _FinalStage extractiveness(Optional extractiveness) {
this.extractiveness = extractiveness;
return this;
}
/**
* The identifier of the model to generate the summary with. Currently available models are command
(default), command-nightly
(experimental), command-light
, and command-light-nightly
(experimental). Smaller, "light" models are faster, while larger models will perform better.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage model(String model) {
this.model = Optional.of(model);
return this;
}
@java.lang.Override
@JsonSetter(value = "model", nulls = Nulls.SKIP)
public _FinalStage model(Optional model) {
this.model = model;
return this;
}
/**
* One of paragraph
, bullets
, or auto
, defaults to auto
. Indicates the style in which the summary will be delivered - in a free form paragraph or in bullet points. If auto
is selected, the best option will be picked based on the input text.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage format(SummarizeRequestFormat format) {
this.format = Optional.of(format);
return this;
}
@java.lang.Override
@JsonSetter(value = "format", nulls = Nulls.SKIP)
public _FinalStage format(Optional format) {
this.format = format;
return this;
}
/**
* One of short
, medium
, long
, or auto
defaults to auto
. Indicates the approximate length of the summary. If auto
is selected, the best option will be picked based on the input text.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage length(SummarizeRequestLength length) {
this.length = Optional.of(length);
return this;
}
@java.lang.Override
@JsonSetter(value = "length", nulls = Nulls.SKIP)
public _FinalStage length(Optional length) {
this.length = length;
return this;
}
@java.lang.Override
public SummarizeRequest build() {
return new SummarizeRequest(
text, length, format, model, extractiveness, temperature, additionalCommand, additionalProperties);
}
}
}