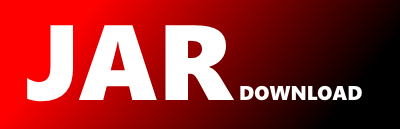
com.cohere.api.resources.embedjobs.requests.CreateEmbedJobRequest Maven / Gradle / Ivy
Show all versions of cohere-java Show documentation
/**
* This file was auto-generated by Fern from our API Definition.
*/
package com.cohere.api.resources.embedjobs.requests;
import com.cohere.api.core.ObjectMappers;
import com.cohere.api.resources.embedjobs.types.CreateEmbedJobRequestTruncate;
import com.cohere.api.types.EmbedInputType;
import com.cohere.api.types.EmbeddingType;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSetter;
import com.fasterxml.jackson.annotation.Nulls;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@JsonDeserialize(builder = CreateEmbedJobRequest.Builder.class)
public final class CreateEmbedJobRequest {
private final String model;
private final String datasetId;
private final EmbedInputType inputType;
private final Optional name;
private final Optional> embeddingTypes;
private final Optional truncate;
private final Map additionalProperties;
private CreateEmbedJobRequest(
String model,
String datasetId,
EmbedInputType inputType,
Optional name,
Optional> embeddingTypes,
Optional truncate,
Map additionalProperties) {
this.model = model;
this.datasetId = datasetId;
this.inputType = inputType;
this.name = name;
this.embeddingTypes = embeddingTypes;
this.truncate = truncate;
this.additionalProperties = additionalProperties;
}
/**
* @return ID of the embedding model.
* Available models and corresponding embedding dimensions:
*
* embed-english-v3.0
: 1024
* embed-multilingual-v3.0
: 1024
* embed-english-light-v3.0
: 384
* embed-multilingual-light-v3.0
: 384
*
*/
@JsonProperty("model")
public String getModel() {
return model;
}
/**
* @return ID of a Dataset. The Dataset must be of type embed-input
and must have a validation status Validated
*/
@JsonProperty("dataset_id")
public String getDatasetId() {
return datasetId;
}
@JsonProperty("input_type")
public EmbedInputType getInputType() {
return inputType;
}
/**
* @return The name of the embed job.
*/
@JsonProperty("name")
public Optional getName() {
return name;
}
/**
* @return Specifies the types of embeddings you want to get back. Not required and default is None, which returns the Embed Floats response type. Can be one or more of the following types.
*
* "float"
: Use this when you want to get back the default float embeddings. Valid for all models.
* "int8"
: Use this when you want to get back signed int8 embeddings. Valid for only v3 models.
* "uint8"
: Use this when you want to get back unsigned int8 embeddings. Valid for only v3 models.
* "binary"
: Use this when you want to get back signed binary embeddings. Valid for only v3 models.
* "ubinary"
: Use this when you want to get back unsigned binary embeddings. Valid for only v3 models.
*
*/
@JsonProperty("embedding_types")
public Optional> getEmbeddingTypes() {
return embeddingTypes;
}
/**
* @return One of START|END
to specify how the API will handle inputs longer than the maximum token length.
* Passing START
will discard the start of the input. END
will discard the end of the input. In both cases, input is discarded until the remaining input is exactly the maximum input token length for the model.
*/
@JsonProperty("truncate")
public Optional getTruncate() {
return truncate;
}
@java.lang.Override
public boolean equals(Object other) {
if (this == other) return true;
return other instanceof CreateEmbedJobRequest && equalTo((CreateEmbedJobRequest) other);
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
private boolean equalTo(CreateEmbedJobRequest other) {
return model.equals(other.model)
&& datasetId.equals(other.datasetId)
&& inputType.equals(other.inputType)
&& name.equals(other.name)
&& embeddingTypes.equals(other.embeddingTypes)
&& truncate.equals(other.truncate);
}
@java.lang.Override
public int hashCode() {
return Objects.hash(this.model, this.datasetId, this.inputType, this.name, this.embeddingTypes, this.truncate);
}
@java.lang.Override
public String toString() {
return ObjectMappers.stringify(this);
}
public static ModelStage builder() {
return new Builder();
}
public interface ModelStage {
DatasetIdStage model(String model);
Builder from(CreateEmbedJobRequest other);
}
public interface DatasetIdStage {
InputTypeStage datasetId(String datasetId);
}
public interface InputTypeStage {
_FinalStage inputType(EmbedInputType inputType);
}
public interface _FinalStage {
CreateEmbedJobRequest build();
_FinalStage name(Optional name);
_FinalStage name(String name);
_FinalStage embeddingTypes(Optional> embeddingTypes);
_FinalStage embeddingTypes(List embeddingTypes);
_FinalStage truncate(Optional truncate);
_FinalStage truncate(CreateEmbedJobRequestTruncate truncate);
}
@JsonIgnoreProperties(ignoreUnknown = true)
public static final class Builder implements ModelStage, DatasetIdStage, InputTypeStage, _FinalStage {
private String model;
private String datasetId;
private EmbedInputType inputType;
private Optional truncate = Optional.empty();
private Optional> embeddingTypes = Optional.empty();
private Optional name = Optional.empty();
@JsonAnySetter
private Map additionalProperties = new HashMap<>();
private Builder() {}
@java.lang.Override
public Builder from(CreateEmbedJobRequest other) {
model(other.getModel());
datasetId(other.getDatasetId());
inputType(other.getInputType());
name(other.getName());
embeddingTypes(other.getEmbeddingTypes());
truncate(other.getTruncate());
return this;
}
/**
* ID of the embedding model.
* Available models and corresponding embedding dimensions:
*
* embed-english-v3.0
: 1024
* embed-multilingual-v3.0
: 1024
* embed-english-light-v3.0
: 384
* embed-multilingual-light-v3.0
: 384
*
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
@JsonSetter("model")
public DatasetIdStage model(String model) {
this.model = model;
return this;
}
/**
* ID of a Dataset. The Dataset must be of type embed-input
and must have a validation status Validated
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
@JsonSetter("dataset_id")
public InputTypeStage datasetId(String datasetId) {
this.datasetId = datasetId;
return this;
}
@java.lang.Override
@JsonSetter("input_type")
public _FinalStage inputType(EmbedInputType inputType) {
this.inputType = inputType;
return this;
}
/**
* One of START|END
to specify how the API will handle inputs longer than the maximum token length.
* Passing START
will discard the start of the input. END
will discard the end of the input. In both cases, input is discarded until the remaining input is exactly the maximum input token length for the model.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage truncate(CreateEmbedJobRequestTruncate truncate) {
this.truncate = Optional.of(truncate);
return this;
}
@java.lang.Override
@JsonSetter(value = "truncate", nulls = Nulls.SKIP)
public _FinalStage truncate(Optional truncate) {
this.truncate = truncate;
return this;
}
/**
* Specifies the types of embeddings you want to get back. Not required and default is None, which returns the Embed Floats response type. Can be one or more of the following types.
*
* "float"
: Use this when you want to get back the default float embeddings. Valid for all models.
* "int8"
: Use this when you want to get back signed int8 embeddings. Valid for only v3 models.
* "uint8"
: Use this when you want to get back unsigned int8 embeddings. Valid for only v3 models.
* "binary"
: Use this when you want to get back signed binary embeddings. Valid for only v3 models.
* "ubinary"
: Use this when you want to get back unsigned binary embeddings. Valid for only v3 models.
*
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage embeddingTypes(List embeddingTypes) {
this.embeddingTypes = Optional.of(embeddingTypes);
return this;
}
@java.lang.Override
@JsonSetter(value = "embedding_types", nulls = Nulls.SKIP)
public _FinalStage embeddingTypes(Optional> embeddingTypes) {
this.embeddingTypes = embeddingTypes;
return this;
}
/**
* The name of the embed job.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage name(String name) {
this.name = Optional.of(name);
return this;
}
@java.lang.Override
@JsonSetter(value = "name", nulls = Nulls.SKIP)
public _FinalStage name(Optional name) {
this.name = name;
return this;
}
@java.lang.Override
public CreateEmbedJobRequest build() {
return new CreateEmbedJobRequest(
model, datasetId, inputType, name, embeddingTypes, truncate, additionalProperties);
}
}
}