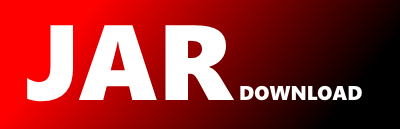
com.cohere.api.resources.finetuning.FinetuningClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cohere-java Show documentation
Show all versions of cohere-java Show documentation
The official Java library for Cohere's API.
/**
* This file was auto-generated by Fern from our API Definition.
*/
package com.cohere.api.resources.finetuning;
import com.cohere.api.core.ClientOptions;
import com.cohere.api.core.CohereApiApiError;
import com.cohere.api.core.CohereApiError;
import com.cohere.api.core.MediaTypes;
import com.cohere.api.core.ObjectMappers;
import com.cohere.api.core.RequestOptions;
import com.cohere.api.errors.CohereApiBadRequestError;
import com.cohere.api.errors.CohereApiForbiddenError;
import com.cohere.api.errors.CohereApiInternalServerError;
import com.cohere.api.errors.CohereApiNotFoundError;
import com.cohere.api.errors.CohereApiServiceUnavailableError;
import com.cohere.api.errors.CohereApiUnauthorizedError;
import com.cohere.api.resources.finetuning.finetuning.types.CreateFinetunedModelResponse;
import com.cohere.api.resources.finetuning.finetuning.types.FinetunedModel;
import com.cohere.api.resources.finetuning.finetuning.types.GetFinetunedModelResponse;
import com.cohere.api.resources.finetuning.finetuning.types.ListEventsResponse;
import com.cohere.api.resources.finetuning.finetuning.types.ListFinetunedModelsResponse;
import com.cohere.api.resources.finetuning.finetuning.types.ListTrainingStepMetricsResponse;
import com.cohere.api.resources.finetuning.finetuning.types.UpdateFinetunedModelResponse;
import com.cohere.api.resources.finetuning.requests.FinetuningListEventsRequest;
import com.cohere.api.resources.finetuning.requests.FinetuningListFinetunedModelsRequest;
import com.cohere.api.resources.finetuning.requests.FinetuningListTrainingStepMetricsRequest;
import com.cohere.api.resources.finetuning.requests.FinetuningUpdateFinetunedModelRequest;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.IOException;
import java.util.Map;
import okhttp3.Headers;
import okhttp3.HttpUrl;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
import okhttp3.ResponseBody;
public class FinetuningClient {
protected final ClientOptions clientOptions;
public FinetuningClient(ClientOptions clientOptions) {
this.clientOptions = clientOptions;
}
public ListFinetunedModelsResponse listFinetunedModels() {
return listFinetunedModels(
FinetuningListFinetunedModelsRequest.builder().build());
}
public ListFinetunedModelsResponse listFinetunedModels(FinetuningListFinetunedModelsRequest request) {
return listFinetunedModels(request, null);
}
public ListFinetunedModelsResponse listFinetunedModels(
FinetuningListFinetunedModelsRequest request, RequestOptions requestOptions) {
HttpUrl.Builder httpUrl = HttpUrl.parse(this.clientOptions.environment().getUrl())
.newBuilder()
.addPathSegments("v1/finetuning/finetuned-models");
if (request.getPageSize().isPresent()) {
httpUrl.addQueryParameter("page_size", request.getPageSize().get().toString());
}
if (request.getPageToken().isPresent()) {
httpUrl.addQueryParameter("page_token", request.getPageToken().get());
}
if (request.getOrderBy().isPresent()) {
httpUrl.addQueryParameter("order_by", request.getOrderBy().get());
}
Request.Builder _requestBuilder = new Request.Builder()
.url(httpUrl.build())
.method("GET", null)
.headers(Headers.of(clientOptions.headers(requestOptions)))
.addHeader("Content-Type", "application/json");
Request okhttpRequest = _requestBuilder.build();
OkHttpClient client = clientOptions.httpClient();
if (requestOptions != null && requestOptions.getTimeout().isPresent()) {
client = clientOptions.httpClientWithTimeout(requestOptions);
}
try (Response response = client.newCall(okhttpRequest).execute()) {
ResponseBody responseBody = response.body();
if (response.isSuccessful()) {
return ObjectMappers.JSON_MAPPER.readValue(responseBody.string(), ListFinetunedModelsResponse.class);
}
String responseBodyString = responseBody != null ? responseBody.string() : "{}";
try {
switch (response.code()) {
case 400:
throw new CohereApiBadRequestError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 401:
throw new CohereApiUnauthorizedError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 403:
throw new CohereApiForbiddenError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 404:
throw new CohereApiNotFoundError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 500:
throw new CohereApiInternalServerError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 503:
throw new CohereApiServiceUnavailableError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
}
} catch (JsonProcessingException ignored) {
// unable to map error response, throwing generic error
}
throw new CohereApiApiError(
"Error with status code " + response.code(),
response.code(),
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
} catch (IOException e) {
throw new CohereApiError("Network error executing HTTP request", e);
}
}
public CreateFinetunedModelResponse createFinetunedModel(FinetunedModel request) {
return createFinetunedModel(request, null);
}
public CreateFinetunedModelResponse createFinetunedModel(FinetunedModel request, RequestOptions requestOptions) {
HttpUrl httpUrl = HttpUrl.parse(this.clientOptions.environment().getUrl())
.newBuilder()
.addPathSegments("v1/finetuning/finetuned-models")
.build();
RequestBody body;
try {
body = RequestBody.create(
ObjectMappers.JSON_MAPPER.writeValueAsBytes(request), MediaTypes.APPLICATION_JSON);
} catch (JsonProcessingException e) {
throw new CohereApiError("Failed to serialize request", e);
}
Request okhttpRequest = new Request.Builder()
.url(httpUrl)
.method("POST", body)
.headers(Headers.of(clientOptions.headers(requestOptions)))
.addHeader("Content-Type", "application/json")
.build();
OkHttpClient client = clientOptions.httpClient();
if (requestOptions != null && requestOptions.getTimeout().isPresent()) {
client = clientOptions.httpClientWithTimeout(requestOptions);
}
try (Response response = client.newCall(okhttpRequest).execute()) {
ResponseBody responseBody = response.body();
if (response.isSuccessful()) {
return ObjectMappers.JSON_MAPPER.readValue(responseBody.string(), CreateFinetunedModelResponse.class);
}
String responseBodyString = responseBody != null ? responseBody.string() : "{}";
try {
switch (response.code()) {
case 400:
throw new CohereApiBadRequestError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 401:
throw new CohereApiUnauthorizedError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 403:
throw new CohereApiForbiddenError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 404:
throw new CohereApiNotFoundError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 500:
throw new CohereApiInternalServerError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 503:
throw new CohereApiServiceUnavailableError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
}
} catch (JsonProcessingException ignored) {
// unable to map error response, throwing generic error
}
throw new CohereApiApiError(
"Error with status code " + response.code(),
response.code(),
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
} catch (IOException e) {
throw new CohereApiError("Network error executing HTTP request", e);
}
}
public GetFinetunedModelResponse getFinetunedModel(String id) {
return getFinetunedModel(id, null);
}
public GetFinetunedModelResponse getFinetunedModel(String id, RequestOptions requestOptions) {
HttpUrl httpUrl = HttpUrl.parse(this.clientOptions.environment().getUrl())
.newBuilder()
.addPathSegments("v1/finetuning/finetuned-models")
.addPathSegment(id)
.build();
Request okhttpRequest = new Request.Builder()
.url(httpUrl)
.method("GET", null)
.headers(Headers.of(clientOptions.headers(requestOptions)))
.addHeader("Content-Type", "application/json")
.build();
OkHttpClient client = clientOptions.httpClient();
if (requestOptions != null && requestOptions.getTimeout().isPresent()) {
client = clientOptions.httpClientWithTimeout(requestOptions);
}
try (Response response = client.newCall(okhttpRequest).execute()) {
ResponseBody responseBody = response.body();
if (response.isSuccessful()) {
return ObjectMappers.JSON_MAPPER.readValue(responseBody.string(), GetFinetunedModelResponse.class);
}
String responseBodyString = responseBody != null ? responseBody.string() : "{}";
try {
switch (response.code()) {
case 400:
throw new CohereApiBadRequestError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 401:
throw new CohereApiUnauthorizedError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 403:
throw new CohereApiForbiddenError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 404:
throw new CohereApiNotFoundError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 500:
throw new CohereApiInternalServerError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
case 503:
throw new CohereApiServiceUnavailableError(
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
}
} catch (JsonProcessingException ignored) {
// unable to map error response, throwing generic error
}
throw new CohereApiApiError(
"Error with status code " + response.code(),
response.code(),
ObjectMappers.JSON_MAPPER.readValue(responseBodyString, Object.class));
} catch (IOException e) {
throw new CohereApiError("Network error executing HTTP request", e);
}
}
public Map deleteFinetunedModel(String id) {
return deleteFinetunedModel(id, null);
}
public Map deleteFinetunedModel(String id, RequestOptions requestOptions) {
HttpUrl httpUrl = HttpUrl.parse(this.clientOptions.environment().getUrl())
.newBuilder()
.addPathSegments("v1/finetuning/finetuned-models")
.addPathSegment(id)
.build();
Request okhttpRequest = new Request.Builder()
.url(httpUrl)
.method("DELETE", null)
.headers(Headers.of(clientOptions.headers(requestOptions)))
.addHeader("Content-Type", "application/json")
.build();
OkHttpClient client = clientOptions.httpClient();
if (requestOptions != null && requestOptions.getTimeout().isPresent()) {
client = clientOptions.httpClientWithTimeout(requestOptions);
}
try (Response response = client.newCall(okhttpRequest).execute()) {
ResponseBody responseBody = response.body();
if (response.isSuccessful()) {
return ObjectMappers.JSON_MAPPER.readValue(
responseBody.string(), new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy