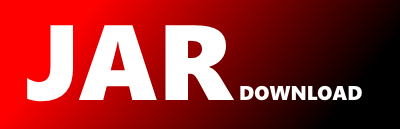
com.cohere.api.resources.v2.requests.V2ChatStreamRequest Maven / Gradle / Ivy
Show all versions of cohere-java Show documentation
/**
* This file was auto-generated by Fern from our API Definition.
*/
package com.cohere.api.resources.v2.requests;
import com.cohere.api.core.ObjectMappers;
import com.cohere.api.resources.v2.types.V2ChatStreamRequestDocumentsItem;
import com.cohere.api.resources.v2.types.V2ChatStreamRequestSafetyMode;
import com.cohere.api.types.ChatMessageV2;
import com.cohere.api.types.CitationOptions;
import com.cohere.api.types.ResponseFormatV2;
import com.cohere.api.types.ToolV2;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSetter;
import com.fasterxml.jackson.annotation.Nulls;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@JsonDeserialize(builder = V2ChatStreamRequest.Builder.class)
public final class V2ChatStreamRequest {
private final String model;
private final List messages;
private final Optional> tools;
private final Optional> documents;
private final Optional citationOptions;
private final Optional responseFormat;
private final Optional safetyMode;
private final Optional maxTokens;
private final Optional> stopSequences;
private final Optional temperature;
private final Optional seed;
private final Optional frequencyPenalty;
private final Optional presencePenalty;
private final Optional k;
private final Optional p;
private final Optional returnPrompt;
private final Map additionalProperties;
private V2ChatStreamRequest(
String model,
List messages,
Optional> tools,
Optional> documents,
Optional citationOptions,
Optional responseFormat,
Optional safetyMode,
Optional maxTokens,
Optional> stopSequences,
Optional temperature,
Optional seed,
Optional frequencyPenalty,
Optional presencePenalty,
Optional k,
Optional p,
Optional returnPrompt,
Map additionalProperties) {
this.model = model;
this.messages = messages;
this.tools = tools;
this.documents = documents;
this.citationOptions = citationOptions;
this.responseFormat = responseFormat;
this.safetyMode = safetyMode;
this.maxTokens = maxTokens;
this.stopSequences = stopSequences;
this.temperature = temperature;
this.seed = seed;
this.frequencyPenalty = frequencyPenalty;
this.presencePenalty = presencePenalty;
this.k = k;
this.p = p;
this.returnPrompt = returnPrompt;
this.additionalProperties = additionalProperties;
}
/**
* @return The name of a compatible Cohere model (such as command-r or command-r-plus) or the ID of a fine-tuned model.
*/
@JsonProperty("model")
public String getModel() {
return model;
}
@JsonProperty("messages")
public List getMessages() {
return messages;
}
/**
* @return A list of available tools (functions) that the model may suggest invoking before producing a text response.
* When tools
is passed (without tool_results
), the text
content in the response will be empty and the tool_calls
field in the response will be populated with a list of tool calls that need to be made. If no calls need to be made, the tool_calls
array will be empty.
*/
@JsonProperty("tools")
public Optional> getTools() {
return tools;
}
/**
* @return A list of relevant documents that the model can cite to generate a more accurate reply. Each document is either a string or document object with content and metadata.
*/
@JsonProperty("documents")
public Optional> getDocuments() {
return documents;
}
@JsonProperty("citation_options")
public Optional getCitationOptions() {
return citationOptions;
}
@JsonProperty("response_format")
public Optional getResponseFormat() {
return responseFormat;
}
/**
* @return Used to select the safety instruction inserted into the prompt. Defaults to CONTEXTUAL
.
* When NONE
is specified, the safety instruction will be omitted.
* Safety modes are not yet configurable in combination with tools
, tool_results
and documents
parameters.
* Note: This parameter is only compatible with models Command R 08-2024, Command R+ 08-2024 and newer.
* Compatible Deployments: Cohere Platform, Azure, AWS Sagemaker/Bedrock, Private Deployments
*/
@JsonProperty("safety_mode")
public Optional getSafetyMode() {
return safetyMode;
}
/**
* @return The maximum number of tokens the model will generate as part of the response. Note: Setting a low value may result in incomplete generations.
*/
@JsonProperty("max_tokens")
public Optional getMaxTokens() {
return maxTokens;
}
/**
* @return A list of up to 5 strings that the model will use to stop generation. If the model generates a string that matches any of the strings in the list, it will stop generating tokens and return the generated text up to that point not including the stop sequence.
*/
@JsonProperty("stop_sequences")
public Optional> getStopSequences() {
return stopSequences;
}
/**
* @return Defaults to 0.3
.
* A non-negative float that tunes the degree of randomness in generation. Lower temperatures mean less random generations, and higher temperatures mean more random generations.
* Randomness can be further maximized by increasing the value of the p
parameter.
*/
@JsonProperty("temperature")
public Optional getTemperature() {
return temperature;
}
/**
* @return If specified, the backend will make a best effort to sample tokens
* deterministically, such that repeated requests with the same
* seed and parameters should return the same result. However,
* determinism cannot be totally guaranteed.
*/
@JsonProperty("seed")
public Optional getSeed() {
return seed;
}
/**
* @return Defaults to 0.0
, min value of 0.0
, max value of 1.0
.
* Used to reduce repetitiveness of generated tokens. The higher the value, the stronger a penalty is applied to previously present tokens, proportional to how many times they have already appeared in the prompt or prior generation.
*/
@JsonProperty("frequency_penalty")
public Optional getFrequencyPenalty() {
return frequencyPenalty;
}
/**
* @return Defaults to 0.0
, min value of 0.0
, max value of 1.0
.
* Used to reduce repetitiveness of generated tokens. Similar to frequency_penalty
, except that this penalty is applied equally to all tokens that have already appeared, regardless of their exact frequencies.
*/
@JsonProperty("presence_penalty")
public Optional getPresencePenalty() {
return presencePenalty;
}
/**
* @return Ensures only the top k
most likely tokens are considered for generation at each step.
* Defaults to 0
, min value of 0
, max value of 500
.
*/
@JsonProperty("k")
public Optional getK() {
return k;
}
/**
* @return Ensures that only the most likely tokens, with total probability mass of p
, are considered for generation at each step. If both k
and p
are enabled, p
acts after k
.
* Defaults to 0.75
. min value of 0.01
, max value of 0.99
.
*/
@JsonProperty("p")
public Optional getP() {
return p;
}
/**
* @return Whether to return the prompt in the response.
*/
@JsonProperty("return_prompt")
public Optional getReturnPrompt() {
return returnPrompt;
}
@JsonProperty("stream")
public Boolean getStream() {
return true;
}
@java.lang.Override
public boolean equals(Object other) {
if (this == other) return true;
return other instanceof V2ChatStreamRequest && equalTo((V2ChatStreamRequest) other);
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
private boolean equalTo(V2ChatStreamRequest other) {
return model.equals(other.model)
&& messages.equals(other.messages)
&& tools.equals(other.tools)
&& documents.equals(other.documents)
&& citationOptions.equals(other.citationOptions)
&& responseFormat.equals(other.responseFormat)
&& safetyMode.equals(other.safetyMode)
&& maxTokens.equals(other.maxTokens)
&& stopSequences.equals(other.stopSequences)
&& temperature.equals(other.temperature)
&& seed.equals(other.seed)
&& frequencyPenalty.equals(other.frequencyPenalty)
&& presencePenalty.equals(other.presencePenalty)
&& k.equals(other.k)
&& p.equals(other.p)
&& returnPrompt.equals(other.returnPrompt);
}
@java.lang.Override
public int hashCode() {
return Objects.hash(
this.model,
this.messages,
this.tools,
this.documents,
this.citationOptions,
this.responseFormat,
this.safetyMode,
this.maxTokens,
this.stopSequences,
this.temperature,
this.seed,
this.frequencyPenalty,
this.presencePenalty,
this.k,
this.p,
this.returnPrompt);
}
@java.lang.Override
public String toString() {
return ObjectMappers.stringify(this);
}
public static ModelStage builder() {
return new Builder();
}
public interface ModelStage {
_FinalStage model(String model);
Builder from(V2ChatStreamRequest other);
}
public interface _FinalStage {
V2ChatStreamRequest build();
_FinalStage messages(List messages);
_FinalStage addMessages(ChatMessageV2 messages);
_FinalStage addAllMessages(List messages);
_FinalStage tools(Optional> tools);
_FinalStage tools(List tools);
_FinalStage documents(Optional> documents);
_FinalStage documents(List documents);
_FinalStage citationOptions(Optional citationOptions);
_FinalStage citationOptions(CitationOptions citationOptions);
_FinalStage responseFormat(Optional responseFormat);
_FinalStage responseFormat(ResponseFormatV2 responseFormat);
_FinalStage safetyMode(Optional safetyMode);
_FinalStage safetyMode(V2ChatStreamRequestSafetyMode safetyMode);
_FinalStage maxTokens(Optional maxTokens);
_FinalStage maxTokens(Integer maxTokens);
_FinalStage stopSequences(Optional> stopSequences);
_FinalStage stopSequences(List stopSequences);
_FinalStage temperature(Optional temperature);
_FinalStage temperature(Double temperature);
_FinalStage seed(Optional seed);
_FinalStage seed(Integer seed);
_FinalStage frequencyPenalty(Optional frequencyPenalty);
_FinalStage frequencyPenalty(Double frequencyPenalty);
_FinalStage presencePenalty(Optional presencePenalty);
_FinalStage presencePenalty(Double presencePenalty);
_FinalStage k(Optional k);
_FinalStage k(Double k);
_FinalStage p(Optional p);
_FinalStage p(Double p);
_FinalStage returnPrompt(Optional returnPrompt);
_FinalStage returnPrompt(Boolean returnPrompt);
}
@JsonIgnoreProperties(ignoreUnknown = true)
public static final class Builder implements ModelStage, _FinalStage {
private String model;
private Optional returnPrompt = Optional.empty();
private Optional p = Optional.empty();
private Optional k = Optional.empty();
private Optional presencePenalty = Optional.empty();
private Optional frequencyPenalty = Optional.empty();
private Optional seed = Optional.empty();
private Optional temperature = Optional.empty();
private Optional> stopSequences = Optional.empty();
private Optional maxTokens = Optional.empty();
private Optional safetyMode = Optional.empty();
private Optional responseFormat = Optional.empty();
private Optional citationOptions = Optional.empty();
private Optional> documents = Optional.empty();
private Optional> tools = Optional.empty();
private List messages = new ArrayList<>();
@JsonAnySetter
private Map additionalProperties = new HashMap<>();
private Builder() {}
@java.lang.Override
public Builder from(V2ChatStreamRequest other) {
model(other.getModel());
messages(other.getMessages());
tools(other.getTools());
documents(other.getDocuments());
citationOptions(other.getCitationOptions());
responseFormat(other.getResponseFormat());
safetyMode(other.getSafetyMode());
maxTokens(other.getMaxTokens());
stopSequences(other.getStopSequences());
temperature(other.getTemperature());
seed(other.getSeed());
frequencyPenalty(other.getFrequencyPenalty());
presencePenalty(other.getPresencePenalty());
k(other.getK());
p(other.getP());
returnPrompt(other.getReturnPrompt());
return this;
}
/**
* The name of a compatible Cohere model (such as command-r or command-r-plus) or the ID of a fine-tuned model.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
@JsonSetter("model")
public _FinalStage model(String model) {
this.model = model;
return this;
}
/**
* Whether to return the prompt in the response.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage returnPrompt(Boolean returnPrompt) {
this.returnPrompt = Optional.of(returnPrompt);
return this;
}
@java.lang.Override
@JsonSetter(value = "return_prompt", nulls = Nulls.SKIP)
public _FinalStage returnPrompt(Optional returnPrompt) {
this.returnPrompt = returnPrompt;
return this;
}
/**
* Ensures that only the most likely tokens, with total probability mass of p
, are considered for generation at each step. If both k
and p
are enabled, p
acts after k
.
* Defaults to 0.75
. min value of 0.01
, max value of 0.99
.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage p(Double p) {
this.p = Optional.of(p);
return this;
}
@java.lang.Override
@JsonSetter(value = "p", nulls = Nulls.SKIP)
public _FinalStage p(Optional p) {
this.p = p;
return this;
}
/**
* Ensures only the top k
most likely tokens are considered for generation at each step.
* Defaults to 0
, min value of 0
, max value of 500
.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage k(Double k) {
this.k = Optional.of(k);
return this;
}
@java.lang.Override
@JsonSetter(value = "k", nulls = Nulls.SKIP)
public _FinalStage k(Optional k) {
this.k = k;
return this;
}
/**
* Defaults to 0.0
, min value of 0.0
, max value of 1.0
.
* Used to reduce repetitiveness of generated tokens. Similar to frequency_penalty
, except that this penalty is applied equally to all tokens that have already appeared, regardless of their exact frequencies.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage presencePenalty(Double presencePenalty) {
this.presencePenalty = Optional.of(presencePenalty);
return this;
}
@java.lang.Override
@JsonSetter(value = "presence_penalty", nulls = Nulls.SKIP)
public _FinalStage presencePenalty(Optional presencePenalty) {
this.presencePenalty = presencePenalty;
return this;
}
/**
* Defaults to 0.0
, min value of 0.0
, max value of 1.0
.
* Used to reduce repetitiveness of generated tokens. The higher the value, the stronger a penalty is applied to previously present tokens, proportional to how many times they have already appeared in the prompt or prior generation.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage frequencyPenalty(Double frequencyPenalty) {
this.frequencyPenalty = Optional.of(frequencyPenalty);
return this;
}
@java.lang.Override
@JsonSetter(value = "frequency_penalty", nulls = Nulls.SKIP)
public _FinalStage frequencyPenalty(Optional frequencyPenalty) {
this.frequencyPenalty = frequencyPenalty;
return this;
}
/**
* If specified, the backend will make a best effort to sample tokens
* deterministically, such that repeated requests with the same
* seed and parameters should return the same result. However,
* determinism cannot be totally guaranteed.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage seed(Integer seed) {
this.seed = Optional.of(seed);
return this;
}
@java.lang.Override
@JsonSetter(value = "seed", nulls = Nulls.SKIP)
public _FinalStage seed(Optional seed) {
this.seed = seed;
return this;
}
/**
* Defaults to 0.3
.
* A non-negative float that tunes the degree of randomness in generation. Lower temperatures mean less random generations, and higher temperatures mean more random generations.
* Randomness can be further maximized by increasing the value of the p
parameter.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage temperature(Double temperature) {
this.temperature = Optional.of(temperature);
return this;
}
@java.lang.Override
@JsonSetter(value = "temperature", nulls = Nulls.SKIP)
public _FinalStage temperature(Optional temperature) {
this.temperature = temperature;
return this;
}
/**
* A list of up to 5 strings that the model will use to stop generation. If the model generates a string that matches any of the strings in the list, it will stop generating tokens and return the generated text up to that point not including the stop sequence.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage stopSequences(List stopSequences) {
this.stopSequences = Optional.of(stopSequences);
return this;
}
@java.lang.Override
@JsonSetter(value = "stop_sequences", nulls = Nulls.SKIP)
public _FinalStage stopSequences(Optional> stopSequences) {
this.stopSequences = stopSequences;
return this;
}
/**
* The maximum number of tokens the model will generate as part of the response. Note: Setting a low value may result in incomplete generations.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage maxTokens(Integer maxTokens) {
this.maxTokens = Optional.of(maxTokens);
return this;
}
@java.lang.Override
@JsonSetter(value = "max_tokens", nulls = Nulls.SKIP)
public _FinalStage maxTokens(Optional maxTokens) {
this.maxTokens = maxTokens;
return this;
}
/**
* Used to select the safety instruction inserted into the prompt. Defaults to CONTEXTUAL
.
* When NONE
is specified, the safety instruction will be omitted.
* Safety modes are not yet configurable in combination with tools
, tool_results
and documents
parameters.
* Note: This parameter is only compatible with models Command R 08-2024, Command R+ 08-2024 and newer.
* Compatible Deployments: Cohere Platform, Azure, AWS Sagemaker/Bedrock, Private Deployments
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage safetyMode(V2ChatStreamRequestSafetyMode safetyMode) {
this.safetyMode = Optional.of(safetyMode);
return this;
}
@java.lang.Override
@JsonSetter(value = "safety_mode", nulls = Nulls.SKIP)
public _FinalStage safetyMode(Optional safetyMode) {
this.safetyMode = safetyMode;
return this;
}
@java.lang.Override
public _FinalStage responseFormat(ResponseFormatV2 responseFormat) {
this.responseFormat = Optional.of(responseFormat);
return this;
}
@java.lang.Override
@JsonSetter(value = "response_format", nulls = Nulls.SKIP)
public _FinalStage responseFormat(Optional responseFormat) {
this.responseFormat = responseFormat;
return this;
}
@java.lang.Override
public _FinalStage citationOptions(CitationOptions citationOptions) {
this.citationOptions = Optional.of(citationOptions);
return this;
}
@java.lang.Override
@JsonSetter(value = "citation_options", nulls = Nulls.SKIP)
public _FinalStage citationOptions(Optional citationOptions) {
this.citationOptions = citationOptions;
return this;
}
/**
* A list of relevant documents that the model can cite to generate a more accurate reply. Each document is either a string or document object with content and metadata.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage documents(List documents) {
this.documents = Optional.of(documents);
return this;
}
@java.lang.Override
@JsonSetter(value = "documents", nulls = Nulls.SKIP)
public _FinalStage documents(Optional> documents) {
this.documents = documents;
return this;
}
/**
* A list of available tools (functions) that the model may suggest invoking before producing a text response.
* When tools
is passed (without tool_results
), the text
content in the response will be empty and the tool_calls
field in the response will be populated with a list of tool calls that need to be made. If no calls need to be made, the tool_calls
array will be empty.
* @return Reference to {@code this} so that method calls can be chained together.
*/
@java.lang.Override
public _FinalStage tools(List tools) {
this.tools = Optional.of(tools);
return this;
}
@java.lang.Override
@JsonSetter(value = "tools", nulls = Nulls.SKIP)
public _FinalStage tools(Optional> tools) {
this.tools = tools;
return this;
}
@java.lang.Override
public _FinalStage addAllMessages(List messages) {
this.messages.addAll(messages);
return this;
}
@java.lang.Override
public _FinalStage addMessages(ChatMessageV2 messages) {
this.messages.add(messages);
return this;
}
@java.lang.Override
@JsonSetter(value = "messages", nulls = Nulls.SKIP)
public _FinalStage messages(List messages) {
this.messages.clear();
this.messages.addAll(messages);
return this;
}
@java.lang.Override
public V2ChatStreamRequest build() {
return new V2ChatStreamRequest(
model,
messages,
tools,
documents,
citationOptions,
responseFormat,
safetyMode,
maxTokens,
stopSequences,
temperature,
seed,
frequencyPenalty,
presencePenalty,
k,
p,
returnPrompt,
additionalProperties);
}
}
}