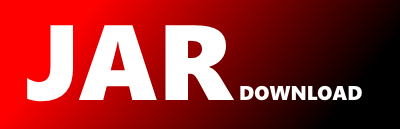
com.coherentlogic.coherent.data.model.demo.application.ClientGUI Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of demo-application Show documentation
Show all versions of demo-application Show documentation
This is the foundation for several data model demonstration
applications.
The newest version!
package com.coherentlogic.coherent.data.model.demo.application;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Frame;
import java.awt.GraphicsEnvironment;
import java.awt.Rectangle;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.TimeUnit;
import javax.swing.BoxLayout;
import javax.swing.ButtonGroup;
import javax.swing.ButtonModel;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JRadioButtonMenuItem;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.ScrollPaneConstants;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.coherentlogic.coherent.data.model.core.builders.HttpMethodsSpecification;
import com.coherentlogic.coherent.data.model.core.exceptions.InvalidURIException;
import com.coherentlogic.coherent.data.model.core.factories.AbstractQueryBuilderFactory;
import com.coherentlogic.coherent.data.model.demo.exceptions.IORuntimeException;
import com.jamonapi.MonKey;
import com.jamonapi.MonKeyImp;
import com.jamonapi.Monitor;
import com.jamonapi.MonitorFactory;
/**
* The front-end for the FRED Client that allows users to directly work with the
* {@link com.coherentlogic.fred.client.core.builders.QueryBuilder}.
*
* @author Support
*/
public abstract class ClientGUI
extends JFrame {
private static final long serialVersionUID = 1L;
private static final Logger log =
LoggerFactory.getLogger(ClientGUI.class);
private static final String QUERY_BUILDER = "queryBuilder";
private final URI uri;
private final JTextArea outputTextArea = new JTextArea();
private final JButton runScriptButton = new JButton("Run script");
private final ButtonGroup requestMenuItemsGroup = new ButtonGroup();
private final Map radioButtonMap =
new HashMap ();
private final GroovyEngine groovyEngine;
private final Map>
queryBuilderFactoryMap;
private final Map exampleMap;
/**
* FRED: http://bit.ly/15cPyLD api java docs
*/
private final String javaDocDestination;
private final AboutDialog aboutDialog;
private final ObjectStringifier objectStringifier =
new ObjectStringifier ();
private final MonKey monKey = new MonKeyImp(
"Time to call web method and return an instance of a domain class.",
TimeUnit.MILLISECONDS.toString());
/**
* @throws URISyntaxException
* @todo Remove the init method from the constructor.
*/
public ClientGUI(
GroovyEngine groovyEngine,
Map>
queryBuilderFactoryMap,
Map exampleMap,
String javaDocDestination,
AboutDialog aboutDialog
) throws URISyntaxException {
this.groovyEngine = groovyEngine;
this.queryBuilderFactoryMap = queryBuilderFactoryMap;
this.exampleMap = exampleMap;
this.javaDocDestination = javaDocDestination;
this.aboutDialog = aboutDialog;
uri = new URI("https://twitter.com/CoherentMktData");
setTitle(getApplicationTitle ());
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
protected abstract String getApplicationTitle ();
/**
* Method is used to add a menu bar and all necessary menu items.
*/
protected abstract void initializeMenu (JTextArea inputTextArea);
protected void addHelpAbout (JMenuBar menuBar) {
JMenu helpMenu = new JMenu("Help");
menuBar.add(helpMenu);
addAPIMenuItem (helpMenu);
JMenuItem mntmAbout = new JMenuItem("About");
helpMenu.add(mntmAbout);
mntmAbout.addActionListener(
new ActionListener () {
@Override
public void actionPerformed(ActionEvent actionEvent) {
aboutDialog.setVisible(true);
}
}
);
}
protected void addAPIMenuItem (JMenu helpMenu) {
JMenuItem apiJavadocs = new JMenuItem("API Javadocs");
apiJavadocs.setForeground(Color.blue);
helpMenu.add(apiJavadocs);
apiJavadocs.addActionListener(
new ActionListener () {
@Override
public void actionPerformed(ActionEvent actionEvent) {
// This is for tracking purposes and will direct the user
// to http://coherentlogic.com/fredJavaDoc/
try {
AboutDialog.open(javaDocDestination);
} catch (URISyntaxException uriSyntaxException) {
throw new InvalidURIException(
"Unable to open the destination: " +
javaDocDestination,
uriSyntaxException
);
}
}
}
);
}
/**
* Method configures the Swing components that are added to this object's
* JFrame.
*/
public void initialize () {
final JPanel parent = new JPanel();
parent.setLayout(new BorderLayout());
final JPanel panel = new JPanel();
panel.setLayout(new BoxLayout(panel, BoxLayout.PAGE_AXIS));
parent.add(panel);
getContentPane().add(parent, BorderLayout.CENTER);
setExtendedState(Frame.MAXIMIZED_BOTH);
JLabel enterYourQueryLabel = new JLabel(
"Enter your query here (context contains references to: " +
"requestBuilder):");
panel.add(enterYourQueryLabel);
final JTextArea inputTextArea = new JTextArea();
JScrollPane inputTextAreaScrollPane = new JScrollPane(inputTextArea);
inputTextAreaScrollPane.
setVerticalScrollBarPolicy(
ScrollPaneConstants.VERTICAL_SCROLLBAR_ALWAYS);
inputTextAreaScrollPane.
setHorizontalScrollBarPolicy(
ScrollPaneConstants.HORIZONTAL_SCROLLBAR_AS_NEEDED);
initializeMenu(inputTextArea);
String exampleText = getDefaultExampleApplicationText ();
inputTextArea.setText(exampleText);
inputTextArea.setColumns(80);
inputTextArea.setRows(40);
panel.add(inputTextAreaScrollPane);
panel.add(runScriptButton);
JLabel outputAppearsBelowLabel = new JLabel(
"The output appears below:");
panel.add(outputAppearsBelowLabel);
outputTextArea.setColumns(80);
outputTextArea.setRows(40);
JScrollPane outputTextAreaScrollPane = new JScrollPane(outputTextArea);
outputTextAreaScrollPane.
setVerticalScrollBarPolicy(
ScrollPaneConstants.VERTICAL_SCROLLBAR_ALWAYS);
panel.add(outputTextAreaScrollPane);
GraphicsEnvironment env =
GraphicsEnvironment.getLocalGraphicsEnvironment();
Rectangle bounds = env.getMaximumWindowBounds();
setBounds(bounds);
addActionListener (runScriptButton, inputTextArea, panel);
}
/**
* Method is used to add an action listener to the runScriptButton. The
* listener
*/
public void addActionListener (
final JButton runScriptButton,
final JTextArea inputTextArea,
final JPanel panel
) {
runScriptButton.addActionListener(
new ActionListener() {
@Override
public void actionPerformed(ActionEvent actionEvent) {
String scriptText = inputTextArea.getText();
log.info("scriptText:\n\n" + scriptText);
ButtonModel buttonModel =
requestMenuItemsGroup.getSelection();
log.info("buttonModel: " + buttonModel);
JRadioButtonMenuItem selectedMenuItem =
radioButtonMap.get(buttonModel);
String selectedText = selectedMenuItem.getText();
AbstractQueryBuilderFactory queryBuilderFactory =
(AbstractQueryBuilderFactory)
queryBuilderFactoryMap.get(selectedText);
T requestBuilder =
queryBuilderFactory.getInstance();
groovyEngine.setVariable(QUERY_BUILDER, requestBuilder);
Object result = null;
Monitor monitor = MonitorFactory.start(monKey);
try {
result = groovyEngine.evaluate(scriptText);
} catch (Throwable throwable) {
log.error("Evaluation failed for the script:\n\n" +
scriptText, throwable);
JOptionPane.showMessageDialog(
panel,
throwable.getMessage(),
"Evaluation failed!",
JOptionPane.ERROR_MESSAGE);
return;
} finally {
monitor.stop();
log.info ("JAMon report: " + monitor);
}
log.info("result: " + result);
if (result != null) {
String stringifiedResult =
objectStringifier.toString(result);
String fullResult =
"// Note that null values are not indicative of " +
"a problem, per se, for \n" +
"// example the PrimaryKey is only ever assigned " +
"when the object has been \n" +
"// saved to a database and since this does not " +
"happen in this example.\n\n\n" +
stringifiedResult;
outputTextArea.setText(fullResult);
}
}
}
);
}
public GroovyEngine getGroovyEngine() {
return groovyEngine;
}
public Map>
getQueryBuilderFactoryMap() {
return queryBuilderFactoryMap;
}
public Map getExampleMap() {
return exampleMap;
}
public Map getRadioButtonMap() {
return radioButtonMap;
}
public ButtonGroup getRequestMenuItemsGroup() {
return requestMenuItemsGroup;
}
/**
* @return The default application -- ie. the first application the user
* sees when the demo application runs for the first time.
*/
protected abstract String getDefaultExampleApplicationText ();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy