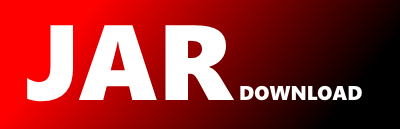
com.coherentlogic.fred.client.db.integration.services.SourcesService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fred-client-db-int Show documentation
Show all versions of fred-client-db-int Show documentation
Integration of the FRED Client with the h2 database for
testing purposes.
package com.coherentlogic.fred.client.db.integration.services;
import java.util.List;
import java.util.Optional;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Example;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.domain.Sort;
import org.springframework.stereotype.Repository;
import org.springframework.transaction.annotation.Transactional;
import com.coherentlogic.fred.client.core.domain.Sources;
import com.coherentlogic.fred.client.db.integration.dao.SourcesRepository;
@Repository(SourcesService.BEAN_NAME)
@Transactional
public class SourcesService {
public static final String BEAN_NAME = "sourcesService";
@PersistenceContext
private EntityManager entityManager;
@Autowired
private SourcesRepository sourcesRepository;
protected EntityManager getEntityManager() {
return entityManager;
}
protected void setEntityManager(EntityManager entityManager) {
this.entityManager = entityManager;
}
public SourcesRepository getSourcesRepository() {
return sourcesRepository;
}
public void setSourcesRepository(SourcesRepository sourcesRepository) {
this.sourcesRepository = sourcesRepository;
}
public long count() {
return sourcesRepository.count();
}
public long count(Example arg0) {
return sourcesRepository.count(arg0);
}
public void delete(Long arg0) {
sourcesRepository.deleteById(arg0);
}
public void delete(Sources arg0) {
sourcesRepository.delete(arg0);
}
public void delete(Iterable extends Sources> arg0) {
sourcesRepository.deleteAll(arg0);
}
public void deleteAll() {
sourcesRepository.deleteAll();
}
public boolean exists(Long arg0) {
return sourcesRepository.existsById(arg0);
}
public boolean exists(Example arg0) {
return sourcesRepository.exists(arg0);
}
public List findAll() {
return sourcesRepository.findAll();
}
public List findAll(Sort sort) {
return sourcesRepository.findAll(sort);
}
public List findAll(Iterable ids) {
return sourcesRepository.findAllById(ids);
}
public Page findAll(Pageable arg0) {
return sourcesRepository.findAll(arg0);
}
public Page findAll(Example arg0, Pageable arg1) {
return sourcesRepository.findAll(arg0, arg1);
}
public List save(Iterable entities) {
return sourcesRepository.saveAll(entities);
}
public void flush() {
sourcesRepository.flush();
}
public S saveAndFlush(S entity) {
return sourcesRepository.saveAndFlush(entity);
}
public void deleteInBatch(Iterable entities) {
sourcesRepository.deleteInBatch(entities);
}
public void deleteAllInBatch() {
sourcesRepository.deleteAllInBatch();
}
public Sources getOne(Long id) {
return sourcesRepository.getOne(id);
}
public List findAll(Example example) {
return sourcesRepository.findAll(example);
}
public List findAll(Example example, Sort sort) {
return sourcesRepository.findAll(example, sort);
}
public Optional findOne(Long arg0) {
return sourcesRepository.findById(arg0);
}
public Optional findOne(Example arg0) {
return sourcesRepository.findOne(arg0);
}
public S save(S arg0) {
return sourcesRepository.save(arg0);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy