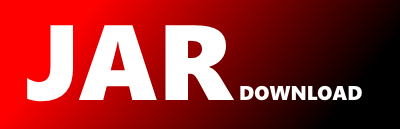
com.commercetools.payment.payone.methods.PayoneCreatePaymentMethodBase Maven / Gradle / Ivy
package com.commercetools.payment.payone.methods;
import com.commercetools.payment.payone.config.PayoneConfigurationNames;
import com.commercetools.payment.methods.CreatePaymentMethodBase;
import com.commercetools.payment.model.CreatePaymentData;
import io.sphere.sdk.carts.Cart;
import io.sphere.sdk.types.CustomFieldsDraftBuilder;
import java.util.Locale;
import java.util.Optional;
import static com.commercetools.payment.payone.config.PayoneConfigurationNames.LANGUAGE_CODE;
import static com.commercetools.payment.payone.config.PayoneConfigurationNames.REFERENCE;
/**
* Base class for Payone related payment methods.
*/
public abstract class PayoneCreatePaymentMethodBase extends CreatePaymentMethodBase {
/**
* @return String Payone payment method name.
* @see PayonePaymentMethodType
*/
abstract protected String getMethodType();
/**
* @return new instance of {@link CustomFieldsDraftBuilder} with implementation specific type key
* (see {@link #getMethodType()})
*/
protected CustomFieldsDraftBuilder createCustomFieldsBuilder() {
return CustomFieldsDraftBuilder.ofTypeKey(getMethodType());
}
/**
* By default all Payone custom fields have "languageCode" and "reference" values from payment data.
* @param cpd payment data from which fetch languageCode and reference
* @return CustomFieldsDraftBuilder of related type key (see {@link #getMethodType()})
* with pre-filled default values from {@code cpd}
* @see #createCustomFieldsBuilder()
*/
protected CustomFieldsDraftBuilder createCustomFieldsBuilder(CreatePaymentData cpd) {
return createCustomFieldsBuilder()
.addObject(REFERENCE, cpd.getReference())
.addObject(LANGUAGE_CODE, getLanguageFromPaymentDataOrFallback(cpd));
}
/**
* Try to define payment locale (two characters ISO 639) in the next order:
* - from custom payment data config, e.g. {@code cpd.getConfigByName(LANGUAGE_CODE)}
* (if not null)
* - else try {@link #getLanguageFromCart(Cart)} with {@code cpd.getCart()}
* - else fallback to "en" locale
*
* @param cpd payment-cart data from which define the locale
* @return non-null 2 characters string language name according to ISO 639 format
* @see #getLanguageFromCart(Cart)
*/
protected static String getLanguageFromPaymentDataOrFallback(CreatePaymentData cpd) {
Optional optionalCpd = Optional.ofNullable(cpd);
return optionalCpd
.map(paymentData -> paymentData.getConfigByName(LANGUAGE_CODE)) // if LANGUAGE_CODE is set in config
.orElseGet(() -> optionalCpd
.map(CreatePaymentData::getCart)
.map(CreatePaymentMethodBase::getLanguageFromCart)
.orElseGet(Locale.ENGLISH::getLanguage));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy