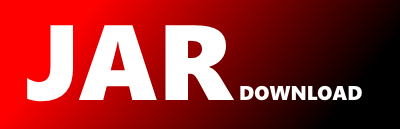
io.sphere.sdk.discountcodes.DiscountCodeImpl Maven / Gradle / Ivy
package io.sphere.sdk.discountcodes;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.databind.JsonNode;
import io.sphere.sdk.cartdiscounts.CartDiscount;
import io.sphere.sdk.models.LocalizedString;
import io.sphere.sdk.models.ResourceImpl;
import io.sphere.sdk.models.Reference;
import javax.annotation.Nullable;
import java.time.ZonedDateTime;
import java.util.List;
final class DiscountCodeImpl extends ResourceImpl implements DiscountCode {
private final String code;
@Nullable
private final LocalizedString name;
@Nullable
private final LocalizedString description;
private final List> cartDiscounts;
private final Boolean isActive;
@Nullable
private final Long maxApplications;
@Nullable
private final Long maxApplicationsPerCustomer;
@Nullable
private final String cartPredicate;
private final List> references;
@JsonCreator
public DiscountCodeImpl(final String id, final Long version, final ZonedDateTime createdAt, final ZonedDateTime lastModifiedAt, final List> cartDiscounts, final String code, @Nullable final LocalizedString name, @Nullable final LocalizedString description, final Boolean isActive, @Nullable final Long maxApplications, @Nullable final Long maxApplicationsPerCustomer, @Nullable final String cartPredicate, final List> references) {
super(id, version, createdAt, lastModifiedAt);
this.cartDiscounts = cartDiscounts;
this.code = code;
this.name = name;
this.description = description;
this.isActive = isActive;
this.maxApplications = maxApplications;
this.maxApplicationsPerCustomer = maxApplicationsPerCustomer;
this.cartPredicate = cartPredicate;
this.references = references;
}
@Override
public List> getCartDiscounts() {
return cartDiscounts;
}
@Override
@Nullable
public String getCartPredicate() {
return cartPredicate;
}
@Override
public String getCode() {
return code;
}
@Override
@Nullable
public LocalizedString getDescription() {
return description;
}
@Override
public Boolean isActive() {
return isActive;
}
@Nullable
@Override
public Long getMaxApplications() {
return maxApplications;
}
@Nullable
@Override
public Long getMaxApplicationsPerCustomer() {
return maxApplicationsPerCustomer;
}
@Override
@Nullable
public LocalizedString getName() {
return name;
}
@Override
public List> getReferences() {
return references;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy