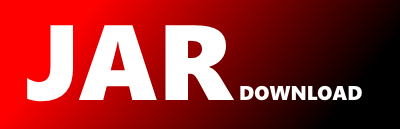
io.sphere.sdk.carts.CartDraftBuilder Maven / Gradle / Ivy
package io.sphere.sdk.carts;
import com.neovisionaries.i18n.CountryCode;
import io.sphere.sdk.models.*;
import io.sphere.sdk.shippingmethods.ShippingMethod;
import io.sphere.sdk.types.CustomFieldsDraft;
import javax.annotation.Nullable;
import javax.money.CurrencyUnit;
import java.util.List;
import java.util.Optional;
/**
* Builder to create {@link CartDraft}s.
*
* Example of creating a cart with custom fields:
* {@include.example io.sphere.sdk.carts.CartsCustomFieldsIntegrationTest#createCartWithCustomType()}
*/
public final class CartDraftBuilder extends Base implements Builder {
private final CurrencyUnit currency;
@Nullable
private String customerId;
@Nullable
private String customerEmail;
@Nullable
private CountryCode country;
@Nullable
private InventoryMode inventoryMode;
@Nullable
private CustomFieldsDraft custom;
@Nullable
private List lineItems;
@Nullable
private List customLineItems;
@Nullable
private Address shippingAddress;
@Nullable
private Address billingAddress;
@Nullable
private Reference shippingMethod;
CartDraftBuilder(final CurrencyUnit currency, @Nullable final String customerId,
@Nullable final CountryCode country, @Nullable final InventoryMode inventoryMode,
@Nullable final CustomFieldsDraft custom, @Nullable String customerEmail,
@Nullable final List lineItems,
@Nullable final List customLineItems,
@Nullable final Address shippingAddress,
@Nullable final Address billingAddress,
@Nullable final Reference shippingMethod) {
this.currency = currency;
this.customerId = customerId;
this.country = country;
this.inventoryMode = inventoryMode;
this.custom = custom;
this.customerEmail = customerEmail;
this.lineItems = lineItems;
this.customLineItems = customLineItems;
this.shippingAddress = shippingAddress;
this.billingAddress = billingAddress;
this.shippingMethod = shippingMethod;
}
CartDraftBuilder(final CartDraft template) {
this(template.getCurrency(), template.getCustomerId(), template.getCountry(), template.getInventoryMode(), template.getCustom(), template.getCustomerEmail(), template.getLineItems(), template.getCustomLineItems(), template.getShippingAddress(), template.getBillingAddress(), template.getShippingMethod());
}
public static CartDraftBuilder of(final CartDraft template) {
return new CartDraftBuilder(template);
}
public static CartDraftBuilder of(final CurrencyUnit currency) {
return new CartDraftBuilder(CartDraft.of(currency));
}
public CartDraftBuilder customerId(@Nullable final String customerId) {
this.customerId = customerId;
return this;
}
public CartDraftBuilder customerEmail(@Nullable final String customerEmail) {
this.customerEmail = customerEmail;
return this;
}
public CartDraftBuilder country(@Nullable final CountryCode country) {
this.country = country;
return this;
}
public CartDraftBuilder inventoryMode(@Nullable final InventoryMode inventoryMode) {
this.inventoryMode = inventoryMode;
return this;
}
@Override
public CartDraftDsl build() {
return new CartDraftDsl(currency, customerId, country, inventoryMode, custom, customerEmail, lineItems, customLineItems, shippingAddress, billingAddress, shippingMethod);
}
public CartDraftBuilder custom(@Nullable final CustomFieldsDraft custom) {
this.custom = custom;
return this;
}
public CartDraftBuilder lineItems(@Nullable final List lineItems) {
this.lineItems = lineItems;
return this;
}
public CartDraftBuilder customLineItems(@Nullable final List customLineItems) {
this.customLineItems = customLineItems;
return this;
}
public CartDraftBuilder shippingAddress(@Nullable final Address shippingAddress) {
this.shippingAddress = shippingAddress;
return this;
}
public CartDraftBuilder billingAddress(@Nullable final Address billingAddress) {
this.billingAddress = billingAddress;
return this;
}
public CartDraftBuilder shippingMethod(@Nullable final Referenceable shippingMethod) {
final Reference reference = Optional.ofNullable(shippingMethod)
.map(Referenceable::toReference)
.orElse(null);
this.shippingMethod = reference;
return this;
}
@Nullable
public Address getBillingAddress() {
return billingAddress;
}
@Nullable
public CountryCode getCountry() {
return country;
}
public CurrencyUnit getCurrency() {
return currency;
}
@Nullable
public CustomFieldsDraft getCustom() {
return custom;
}
@Nullable
public String getCustomerEmail() {
return customerEmail;
}
@Nullable
public String getCustomerId() {
return customerId;
}
@Nullable
public List getCustomLineItems() {
return customLineItems;
}
@Nullable
public InventoryMode getInventoryMode() {
return inventoryMode;
}
@Nullable
public List getLineItems() {
return lineItems;
}
@Nullable
public Address getShippingAddress() {
return shippingAddress;
}
@Nullable
public Reference getShippingMethod() {
return shippingMethod;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy